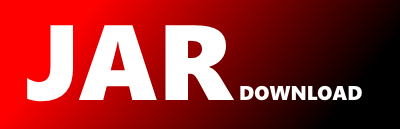
com.amazonaws.services.computeoptimizer.model.ECSServiceRecommendation Maven / Gradle / Ivy
Show all versions of aws-java-sdk-computeoptimizer Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.computeoptimizer.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes an Amazon ECS service recommendation.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ECSServiceRecommendation implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Resource Name (ARN) of the current Amazon ECS service.
*
*
* The following is the format of the ARN:
*
*
* arn:aws:ecs:region:aws_account_id:service/cluster-name/service-name
*
*/
private String serviceArn;
/**
*
* The Amazon Web Services account ID of the Amazon ECS service.
*
*/
private String accountId;
/**
*
* The configuration of the current Amazon ECS service.
*
*/
private ServiceConfiguration currentServiceConfiguration;
/**
*
* An array of objects that describe the utilization metrics of the Amazon ECS service.
*
*/
private java.util.List utilizationMetrics;
/**
*
* The number of days the Amazon ECS service utilization metrics were analyzed.
*
*/
private Double lookbackPeriodInDays;
/**
*
* The launch type the Amazon ECS service is using.
*
*
*
* Compute Optimizer only supports the Fargate launch type.
*
*
*/
private String launchType;
/**
*
* The timestamp of when the Amazon ECS service recommendation was last generated.
*
*/
private java.util.Date lastRefreshTimestamp;
/**
*
* The finding classification of an Amazon ECS service.
*
*
* Findings for Amazon ECS services include:
*
*
* -
*
* Underprovisioned
— When Compute Optimizer detects that there’s not enough memory or CPU, an
* Amazon ECS service is considered under-provisioned. An under-provisioned service might result in poor application
* performance.
*
*
* -
*
* Overprovisioned
— When Compute Optimizer detects that there’s excessive memory or CPU, an
* Amazon ECS service is considered over-provisioned. An over-provisioned service might result in additional
* infrastructure costs.
*
*
* -
*
* Optimized
— When both the CPU and memory of your Amazon ECS service meet the performance
* requirements of your workload, the service is considered optimized.
*
*
*
*/
private String finding;
/**
*
* The reason for the finding classification of an Amazon ECS service.
*
*
* Finding reason codes for Amazon ECS services include:
*
*
* -
*
* CPUUnderprovisioned
— The service CPU configuration can be sized up to enhance the
* performance of your workload. This is identified by analyzing the CPUUtilization
metric of the
* current service during the look-back period.
*
*
* -
*
* CPUOverprovisioned
— The service CPU configuration can be sized down while still meeting
* the performance requirements of your workload. This is identified by analyzing the CPUUtilization
* metric of the current service during the look-back period.
*
*
* -
*
* MemoryUnderprovisioned
— The service memory configuration can be sized up to enhance the
* performance of your workload. This is identified by analyzing the MemoryUtilization
metric of the
* current service during the look-back period.
*
*
* -
*
* MemoryOverprovisioned
— The service memory configuration can be sized down while still
* meeting the performance requirements of your workload. This is identified by analyzing the
* MemoryUtilization
metric of the current service during the look-back period.
*
*
*
*/
private java.util.List findingReasonCodes;
/**
*
* An array of objects that describe the recommendation options for the Amazon ECS service.
*
*/
private java.util.List serviceRecommendationOptions;
/**
*
* The risk of the current Amazon ECS service not meeting the performance needs of its workloads. The higher the
* risk, the more likely the current service can't meet the performance requirements of its workload.
*
*/
private String currentPerformanceRisk;
/**
*
* Describes the effective recommendation preferences for Amazon ECS services.
*
*/
private ECSEffectiveRecommendationPreferences effectiveRecommendationPreferences;
/**
*
* A list of tags assigned to your Amazon ECS service recommendations.
*
*/
private java.util.List tags;
/**
*
* The Amazon Resource Name (ARN) of the current Amazon ECS service.
*
*
* The following is the format of the ARN:
*
*
* arn:aws:ecs:region:aws_account_id:service/cluster-name/service-name
*
*
* @param serviceArn
* The Amazon Resource Name (ARN) of the current Amazon ECS service.
*
* The following is the format of the ARN:
*
*
* arn:aws:ecs:region:aws_account_id:service/cluster-name/service-name
*/
public void setServiceArn(String serviceArn) {
this.serviceArn = serviceArn;
}
/**
*
* The Amazon Resource Name (ARN) of the current Amazon ECS service.
*
*
* The following is the format of the ARN:
*
*
* arn:aws:ecs:region:aws_account_id:service/cluster-name/service-name
*
*
* @return The Amazon Resource Name (ARN) of the current Amazon ECS service.
*
* The following is the format of the ARN:
*
*
* arn:aws:ecs:region:aws_account_id:service/cluster-name/service-name
*/
public String getServiceArn() {
return this.serviceArn;
}
/**
*
* The Amazon Resource Name (ARN) of the current Amazon ECS service.
*
*
* The following is the format of the ARN:
*
*
* arn:aws:ecs:region:aws_account_id:service/cluster-name/service-name
*
*
* @param serviceArn
* The Amazon Resource Name (ARN) of the current Amazon ECS service.
*
* The following is the format of the ARN:
*
*
* arn:aws:ecs:region:aws_account_id:service/cluster-name/service-name
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ECSServiceRecommendation withServiceArn(String serviceArn) {
setServiceArn(serviceArn);
return this;
}
/**
*
* The Amazon Web Services account ID of the Amazon ECS service.
*
*
* @param accountId
* The Amazon Web Services account ID of the Amazon ECS service.
*/
public void setAccountId(String accountId) {
this.accountId = accountId;
}
/**
*
* The Amazon Web Services account ID of the Amazon ECS service.
*
*
* @return The Amazon Web Services account ID of the Amazon ECS service.
*/
public String getAccountId() {
return this.accountId;
}
/**
*
* The Amazon Web Services account ID of the Amazon ECS service.
*
*
* @param accountId
* The Amazon Web Services account ID of the Amazon ECS service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ECSServiceRecommendation withAccountId(String accountId) {
setAccountId(accountId);
return this;
}
/**
*
* The configuration of the current Amazon ECS service.
*
*
* @param currentServiceConfiguration
* The configuration of the current Amazon ECS service.
*/
public void setCurrentServiceConfiguration(ServiceConfiguration currentServiceConfiguration) {
this.currentServiceConfiguration = currentServiceConfiguration;
}
/**
*
* The configuration of the current Amazon ECS service.
*
*
* @return The configuration of the current Amazon ECS service.
*/
public ServiceConfiguration getCurrentServiceConfiguration() {
return this.currentServiceConfiguration;
}
/**
*
* The configuration of the current Amazon ECS service.
*
*
* @param currentServiceConfiguration
* The configuration of the current Amazon ECS service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ECSServiceRecommendation withCurrentServiceConfiguration(ServiceConfiguration currentServiceConfiguration) {
setCurrentServiceConfiguration(currentServiceConfiguration);
return this;
}
/**
*
* An array of objects that describe the utilization metrics of the Amazon ECS service.
*
*
* @return An array of objects that describe the utilization metrics of the Amazon ECS service.
*/
public java.util.List getUtilizationMetrics() {
return utilizationMetrics;
}
/**
*
* An array of objects that describe the utilization metrics of the Amazon ECS service.
*
*
* @param utilizationMetrics
* An array of objects that describe the utilization metrics of the Amazon ECS service.
*/
public void setUtilizationMetrics(java.util.Collection utilizationMetrics) {
if (utilizationMetrics == null) {
this.utilizationMetrics = null;
return;
}
this.utilizationMetrics = new java.util.ArrayList(utilizationMetrics);
}
/**
*
* An array of objects that describe the utilization metrics of the Amazon ECS service.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setUtilizationMetrics(java.util.Collection)} or {@link #withUtilizationMetrics(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param utilizationMetrics
* An array of objects that describe the utilization metrics of the Amazon ECS service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ECSServiceRecommendation withUtilizationMetrics(ECSServiceUtilizationMetric... utilizationMetrics) {
if (this.utilizationMetrics == null) {
setUtilizationMetrics(new java.util.ArrayList(utilizationMetrics.length));
}
for (ECSServiceUtilizationMetric ele : utilizationMetrics) {
this.utilizationMetrics.add(ele);
}
return this;
}
/**
*
* An array of objects that describe the utilization metrics of the Amazon ECS service.
*
*
* @param utilizationMetrics
* An array of objects that describe the utilization metrics of the Amazon ECS service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ECSServiceRecommendation withUtilizationMetrics(java.util.Collection utilizationMetrics) {
setUtilizationMetrics(utilizationMetrics);
return this;
}
/**
*
* The number of days the Amazon ECS service utilization metrics were analyzed.
*
*
* @param lookbackPeriodInDays
* The number of days the Amazon ECS service utilization metrics were analyzed.
*/
public void setLookbackPeriodInDays(Double lookbackPeriodInDays) {
this.lookbackPeriodInDays = lookbackPeriodInDays;
}
/**
*
* The number of days the Amazon ECS service utilization metrics were analyzed.
*
*
* @return The number of days the Amazon ECS service utilization metrics were analyzed.
*/
public Double getLookbackPeriodInDays() {
return this.lookbackPeriodInDays;
}
/**
*
* The number of days the Amazon ECS service utilization metrics were analyzed.
*
*
* @param lookbackPeriodInDays
* The number of days the Amazon ECS service utilization metrics were analyzed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ECSServiceRecommendation withLookbackPeriodInDays(Double lookbackPeriodInDays) {
setLookbackPeriodInDays(lookbackPeriodInDays);
return this;
}
/**
*
* The launch type the Amazon ECS service is using.
*
*
*
* Compute Optimizer only supports the Fargate launch type.
*
*
*
* @param launchType
* The launch type the Amazon ECS service is using.
*
* Compute Optimizer only supports the Fargate launch type.
*
* @see ECSServiceLaunchType
*/
public void setLaunchType(String launchType) {
this.launchType = launchType;
}
/**
*
* The launch type the Amazon ECS service is using.
*
*
*
* Compute Optimizer only supports the Fargate launch type.
*
*
*
* @return The launch type the Amazon ECS service is using.
*
* Compute Optimizer only supports the Fargate launch type.
*
* @see ECSServiceLaunchType
*/
public String getLaunchType() {
return this.launchType;
}
/**
*
* The launch type the Amazon ECS service is using.
*
*
*
* Compute Optimizer only supports the Fargate launch type.
*
*
*
* @param launchType
* The launch type the Amazon ECS service is using.
*
* Compute Optimizer only supports the Fargate launch type.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ECSServiceLaunchType
*/
public ECSServiceRecommendation withLaunchType(String launchType) {
setLaunchType(launchType);
return this;
}
/**
*
* The launch type the Amazon ECS service is using.
*
*
*
* Compute Optimizer only supports the Fargate launch type.
*
*
*
* @param launchType
* The launch type the Amazon ECS service is using.
*
* Compute Optimizer only supports the Fargate launch type.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ECSServiceLaunchType
*/
public ECSServiceRecommendation withLaunchType(ECSServiceLaunchType launchType) {
this.launchType = launchType.toString();
return this;
}
/**
*
* The timestamp of when the Amazon ECS service recommendation was last generated.
*
*
* @param lastRefreshTimestamp
* The timestamp of when the Amazon ECS service recommendation was last generated.
*/
public void setLastRefreshTimestamp(java.util.Date lastRefreshTimestamp) {
this.lastRefreshTimestamp = lastRefreshTimestamp;
}
/**
*
* The timestamp of when the Amazon ECS service recommendation was last generated.
*
*
* @return The timestamp of when the Amazon ECS service recommendation was last generated.
*/
public java.util.Date getLastRefreshTimestamp() {
return this.lastRefreshTimestamp;
}
/**
*
* The timestamp of when the Amazon ECS service recommendation was last generated.
*
*
* @param lastRefreshTimestamp
* The timestamp of when the Amazon ECS service recommendation was last generated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ECSServiceRecommendation withLastRefreshTimestamp(java.util.Date lastRefreshTimestamp) {
setLastRefreshTimestamp(lastRefreshTimestamp);
return this;
}
/**
*
* The finding classification of an Amazon ECS service.
*
*
* Findings for Amazon ECS services include:
*
*
* -
*
* Underprovisioned
— When Compute Optimizer detects that there’s not enough memory or CPU, an
* Amazon ECS service is considered under-provisioned. An under-provisioned service might result in poor application
* performance.
*
*
* -
*
* Overprovisioned
— When Compute Optimizer detects that there’s excessive memory or CPU, an
* Amazon ECS service is considered over-provisioned. An over-provisioned service might result in additional
* infrastructure costs.
*
*
* -
*
* Optimized
— When both the CPU and memory of your Amazon ECS service meet the performance
* requirements of your workload, the service is considered optimized.
*
*
*
*
* @param finding
* The finding classification of an Amazon ECS service.
*
* Findings for Amazon ECS services include:
*
*
* -
*
* Underprovisioned
— When Compute Optimizer detects that there’s not enough memory or
* CPU, an Amazon ECS service is considered under-provisioned. An under-provisioned service might result in
* poor application performance.
*
*
* -
*
* Overprovisioned
— When Compute Optimizer detects that there’s excessive memory or
* CPU, an Amazon ECS service is considered over-provisioned. An over-provisioned service might result in
* additional infrastructure costs.
*
*
* -
*
* Optimized
— When both the CPU and memory of your Amazon ECS service meet the
* performance requirements of your workload, the service is considered optimized.
*
*
* @see ECSServiceRecommendationFinding
*/
public void setFinding(String finding) {
this.finding = finding;
}
/**
*
* The finding classification of an Amazon ECS service.
*
*
* Findings for Amazon ECS services include:
*
*
* -
*
* Underprovisioned
— When Compute Optimizer detects that there’s not enough memory or CPU, an
* Amazon ECS service is considered under-provisioned. An under-provisioned service might result in poor application
* performance.
*
*
* -
*
* Overprovisioned
— When Compute Optimizer detects that there’s excessive memory or CPU, an
* Amazon ECS service is considered over-provisioned. An over-provisioned service might result in additional
* infrastructure costs.
*
*
* -
*
* Optimized
— When both the CPU and memory of your Amazon ECS service meet the performance
* requirements of your workload, the service is considered optimized.
*
*
*
*
* @return The finding classification of an Amazon ECS service.
*
* Findings for Amazon ECS services include:
*
*
* -
*
* Underprovisioned
— When Compute Optimizer detects that there’s not enough memory or
* CPU, an Amazon ECS service is considered under-provisioned. An under-provisioned service might result in
* poor application performance.
*
*
* -
*
* Overprovisioned
— When Compute Optimizer detects that there’s excessive memory or
* CPU, an Amazon ECS service is considered over-provisioned. An over-provisioned service might result in
* additional infrastructure costs.
*
*
* -
*
* Optimized
— When both the CPU and memory of your Amazon ECS service meet the
* performance requirements of your workload, the service is considered optimized.
*
*
* @see ECSServiceRecommendationFinding
*/
public String getFinding() {
return this.finding;
}
/**
*
* The finding classification of an Amazon ECS service.
*
*
* Findings for Amazon ECS services include:
*
*
* -
*
* Underprovisioned
— When Compute Optimizer detects that there’s not enough memory or CPU, an
* Amazon ECS service is considered under-provisioned. An under-provisioned service might result in poor application
* performance.
*
*
* -
*
* Overprovisioned
— When Compute Optimizer detects that there’s excessive memory or CPU, an
* Amazon ECS service is considered over-provisioned. An over-provisioned service might result in additional
* infrastructure costs.
*
*
* -
*
* Optimized
— When both the CPU and memory of your Amazon ECS service meet the performance
* requirements of your workload, the service is considered optimized.
*
*
*
*
* @param finding
* The finding classification of an Amazon ECS service.
*
* Findings for Amazon ECS services include:
*
*
* -
*
* Underprovisioned
— When Compute Optimizer detects that there’s not enough memory or
* CPU, an Amazon ECS service is considered under-provisioned. An under-provisioned service might result in
* poor application performance.
*
*
* -
*
* Overprovisioned
— When Compute Optimizer detects that there’s excessive memory or
* CPU, an Amazon ECS service is considered over-provisioned. An over-provisioned service might result in
* additional infrastructure costs.
*
*
* -
*
* Optimized
— When both the CPU and memory of your Amazon ECS service meet the
* performance requirements of your workload, the service is considered optimized.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ECSServiceRecommendationFinding
*/
public ECSServiceRecommendation withFinding(String finding) {
setFinding(finding);
return this;
}
/**
*
* The finding classification of an Amazon ECS service.
*
*
* Findings for Amazon ECS services include:
*
*
* -
*
* Underprovisioned
— When Compute Optimizer detects that there’s not enough memory or CPU, an
* Amazon ECS service is considered under-provisioned. An under-provisioned service might result in poor application
* performance.
*
*
* -
*
* Overprovisioned
— When Compute Optimizer detects that there’s excessive memory or CPU, an
* Amazon ECS service is considered over-provisioned. An over-provisioned service might result in additional
* infrastructure costs.
*
*
* -
*
* Optimized
— When both the CPU and memory of your Amazon ECS service meet the performance
* requirements of your workload, the service is considered optimized.
*
*
*
*
* @param finding
* The finding classification of an Amazon ECS service.
*
* Findings for Amazon ECS services include:
*
*
* -
*
* Underprovisioned
— When Compute Optimizer detects that there’s not enough memory or
* CPU, an Amazon ECS service is considered under-provisioned. An under-provisioned service might result in
* poor application performance.
*
*
* -
*
* Overprovisioned
— When Compute Optimizer detects that there’s excessive memory or
* CPU, an Amazon ECS service is considered over-provisioned. An over-provisioned service might result in
* additional infrastructure costs.
*
*
* -
*
* Optimized
— When both the CPU and memory of your Amazon ECS service meet the
* performance requirements of your workload, the service is considered optimized.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ECSServiceRecommendationFinding
*/
public ECSServiceRecommendation withFinding(ECSServiceRecommendationFinding finding) {
this.finding = finding.toString();
return this;
}
/**
*
* The reason for the finding classification of an Amazon ECS service.
*
*
* Finding reason codes for Amazon ECS services include:
*
*
* -
*
* CPUUnderprovisioned
— The service CPU configuration can be sized up to enhance the
* performance of your workload. This is identified by analyzing the CPUUtilization
metric of the
* current service during the look-back period.
*
*
* -
*
* CPUOverprovisioned
— The service CPU configuration can be sized down while still meeting
* the performance requirements of your workload. This is identified by analyzing the CPUUtilization
* metric of the current service during the look-back period.
*
*
* -
*
* MemoryUnderprovisioned
— The service memory configuration can be sized up to enhance the
* performance of your workload. This is identified by analyzing the MemoryUtilization
metric of the
* current service during the look-back period.
*
*
* -
*
* MemoryOverprovisioned
— The service memory configuration can be sized down while still
* meeting the performance requirements of your workload. This is identified by analyzing the
* MemoryUtilization
metric of the current service during the look-back period.
*
*
*
*
* @return The reason for the finding classification of an Amazon ECS service.
*
* Finding reason codes for Amazon ECS services include:
*
*
* -
*
* CPUUnderprovisioned
— The service CPU configuration can be sized up to enhance the
* performance of your workload. This is identified by analyzing the CPUUtilization
metric of
* the current service during the look-back period.
*
*
* -
*
* CPUOverprovisioned
— The service CPU configuration can be sized down while still
* meeting the performance requirements of your workload. This is identified by analyzing the
* CPUUtilization
metric of the current service during the look-back period.
*
*
* -
*
* MemoryUnderprovisioned
— The service memory configuration can be sized up to
* enhance the performance of your workload. This is identified by analyzing the
* MemoryUtilization
metric of the current service during the look-back period.
*
*
* -
*
* MemoryOverprovisioned
— The service memory configuration can be sized down while
* still meeting the performance requirements of your workload. This is identified by analyzing the
* MemoryUtilization
metric of the current service during the look-back period.
*
*
* @see ECSServiceRecommendationFindingReasonCode
*/
public java.util.List getFindingReasonCodes() {
return findingReasonCodes;
}
/**
*
* The reason for the finding classification of an Amazon ECS service.
*
*
* Finding reason codes for Amazon ECS services include:
*
*
* -
*
* CPUUnderprovisioned
— The service CPU configuration can be sized up to enhance the
* performance of your workload. This is identified by analyzing the CPUUtilization
metric of the
* current service during the look-back period.
*
*
* -
*
* CPUOverprovisioned
— The service CPU configuration can be sized down while still meeting
* the performance requirements of your workload. This is identified by analyzing the CPUUtilization
* metric of the current service during the look-back period.
*
*
* -
*
* MemoryUnderprovisioned
— The service memory configuration can be sized up to enhance the
* performance of your workload. This is identified by analyzing the MemoryUtilization
metric of the
* current service during the look-back period.
*
*
* -
*
* MemoryOverprovisioned
— The service memory configuration can be sized down while still
* meeting the performance requirements of your workload. This is identified by analyzing the
* MemoryUtilization
metric of the current service during the look-back period.
*
*
*
*
* @param findingReasonCodes
* The reason for the finding classification of an Amazon ECS service.
*
* Finding reason codes for Amazon ECS services include:
*
*
* -
*
* CPUUnderprovisioned
— The service CPU configuration can be sized up to enhance the
* performance of your workload. This is identified by analyzing the CPUUtilization
metric of
* the current service during the look-back period.
*
*
* -
*
* CPUOverprovisioned
— The service CPU configuration can be sized down while still
* meeting the performance requirements of your workload. This is identified by analyzing the
* CPUUtilization
metric of the current service during the look-back period.
*
*
* -
*
* MemoryUnderprovisioned
— The service memory configuration can be sized up to enhance
* the performance of your workload. This is identified by analyzing the MemoryUtilization
* metric of the current service during the look-back period.
*
*
* -
*
* MemoryOverprovisioned
— The service memory configuration can be sized down while
* still meeting the performance requirements of your workload. This is identified by analyzing the
* MemoryUtilization
metric of the current service during the look-back period.
*
*
* @see ECSServiceRecommendationFindingReasonCode
*/
public void setFindingReasonCodes(java.util.Collection findingReasonCodes) {
if (findingReasonCodes == null) {
this.findingReasonCodes = null;
return;
}
this.findingReasonCodes = new java.util.ArrayList(findingReasonCodes);
}
/**
*
* The reason for the finding classification of an Amazon ECS service.
*
*
* Finding reason codes for Amazon ECS services include:
*
*
* -
*
* CPUUnderprovisioned
— The service CPU configuration can be sized up to enhance the
* performance of your workload. This is identified by analyzing the CPUUtilization
metric of the
* current service during the look-back period.
*
*
* -
*
* CPUOverprovisioned
— The service CPU configuration can be sized down while still meeting
* the performance requirements of your workload. This is identified by analyzing the CPUUtilization
* metric of the current service during the look-back period.
*
*
* -
*
* MemoryUnderprovisioned
— The service memory configuration can be sized up to enhance the
* performance of your workload. This is identified by analyzing the MemoryUtilization
metric of the
* current service during the look-back period.
*
*
* -
*
* MemoryOverprovisioned
— The service memory configuration can be sized down while still
* meeting the performance requirements of your workload. This is identified by analyzing the
* MemoryUtilization
metric of the current service during the look-back period.
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFindingReasonCodes(java.util.Collection)} or {@link #withFindingReasonCodes(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param findingReasonCodes
* The reason for the finding classification of an Amazon ECS service.
*
* Finding reason codes for Amazon ECS services include:
*
*
* -
*
* CPUUnderprovisioned
— The service CPU configuration can be sized up to enhance the
* performance of your workload. This is identified by analyzing the CPUUtilization
metric of
* the current service during the look-back period.
*
*
* -
*
* CPUOverprovisioned
— The service CPU configuration can be sized down while still
* meeting the performance requirements of your workload. This is identified by analyzing the
* CPUUtilization
metric of the current service during the look-back period.
*
*
* -
*
* MemoryUnderprovisioned
— The service memory configuration can be sized up to enhance
* the performance of your workload. This is identified by analyzing the MemoryUtilization
* metric of the current service during the look-back period.
*
*
* -
*
* MemoryOverprovisioned
— The service memory configuration can be sized down while
* still meeting the performance requirements of your workload. This is identified by analyzing the
* MemoryUtilization
metric of the current service during the look-back period.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ECSServiceRecommendationFindingReasonCode
*/
public ECSServiceRecommendation withFindingReasonCodes(String... findingReasonCodes) {
if (this.findingReasonCodes == null) {
setFindingReasonCodes(new java.util.ArrayList(findingReasonCodes.length));
}
for (String ele : findingReasonCodes) {
this.findingReasonCodes.add(ele);
}
return this;
}
/**
*
* The reason for the finding classification of an Amazon ECS service.
*
*
* Finding reason codes for Amazon ECS services include:
*
*
* -
*
* CPUUnderprovisioned
— The service CPU configuration can be sized up to enhance the
* performance of your workload. This is identified by analyzing the CPUUtilization
metric of the
* current service during the look-back period.
*
*
* -
*
* CPUOverprovisioned
— The service CPU configuration can be sized down while still meeting
* the performance requirements of your workload. This is identified by analyzing the CPUUtilization
* metric of the current service during the look-back period.
*
*
* -
*
* MemoryUnderprovisioned
— The service memory configuration can be sized up to enhance the
* performance of your workload. This is identified by analyzing the MemoryUtilization
metric of the
* current service during the look-back period.
*
*
* -
*
* MemoryOverprovisioned
— The service memory configuration can be sized down while still
* meeting the performance requirements of your workload. This is identified by analyzing the
* MemoryUtilization
metric of the current service during the look-back period.
*
*
*
*
* @param findingReasonCodes
* The reason for the finding classification of an Amazon ECS service.
*
* Finding reason codes for Amazon ECS services include:
*
*
* -
*
* CPUUnderprovisioned
— The service CPU configuration can be sized up to enhance the
* performance of your workload. This is identified by analyzing the CPUUtilization
metric of
* the current service during the look-back period.
*
*
* -
*
* CPUOverprovisioned
— The service CPU configuration can be sized down while still
* meeting the performance requirements of your workload. This is identified by analyzing the
* CPUUtilization
metric of the current service during the look-back period.
*
*
* -
*
* MemoryUnderprovisioned
— The service memory configuration can be sized up to enhance
* the performance of your workload. This is identified by analyzing the MemoryUtilization
* metric of the current service during the look-back period.
*
*
* -
*
* MemoryOverprovisioned
— The service memory configuration can be sized down while
* still meeting the performance requirements of your workload. This is identified by analyzing the
* MemoryUtilization
metric of the current service during the look-back period.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ECSServiceRecommendationFindingReasonCode
*/
public ECSServiceRecommendation withFindingReasonCodes(java.util.Collection findingReasonCodes) {
setFindingReasonCodes(findingReasonCodes);
return this;
}
/**
*
* The reason for the finding classification of an Amazon ECS service.
*
*
* Finding reason codes for Amazon ECS services include:
*
*
* -
*
* CPUUnderprovisioned
— The service CPU configuration can be sized up to enhance the
* performance of your workload. This is identified by analyzing the CPUUtilization
metric of the
* current service during the look-back period.
*
*
* -
*
* CPUOverprovisioned
— The service CPU configuration can be sized down while still meeting
* the performance requirements of your workload. This is identified by analyzing the CPUUtilization
* metric of the current service during the look-back period.
*
*
* -
*
* MemoryUnderprovisioned
— The service memory configuration can be sized up to enhance the
* performance of your workload. This is identified by analyzing the MemoryUtilization
metric of the
* current service during the look-back period.
*
*
* -
*
* MemoryOverprovisioned
— The service memory configuration can be sized down while still
* meeting the performance requirements of your workload. This is identified by analyzing the
* MemoryUtilization
metric of the current service during the look-back period.
*
*
*
*
* @param findingReasonCodes
* The reason for the finding classification of an Amazon ECS service.
*
* Finding reason codes for Amazon ECS services include:
*
*
* -
*
* CPUUnderprovisioned
— The service CPU configuration can be sized up to enhance the
* performance of your workload. This is identified by analyzing the CPUUtilization
metric of
* the current service during the look-back period.
*
*
* -
*
* CPUOverprovisioned
— The service CPU configuration can be sized down while still
* meeting the performance requirements of your workload. This is identified by analyzing the
* CPUUtilization
metric of the current service during the look-back period.
*
*
* -
*
* MemoryUnderprovisioned
— The service memory configuration can be sized up to enhance
* the performance of your workload. This is identified by analyzing the MemoryUtilization
* metric of the current service during the look-back period.
*
*
* -
*
* MemoryOverprovisioned
— The service memory configuration can be sized down while
* still meeting the performance requirements of your workload. This is identified by analyzing the
* MemoryUtilization
metric of the current service during the look-back period.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ECSServiceRecommendationFindingReasonCode
*/
public ECSServiceRecommendation withFindingReasonCodes(ECSServiceRecommendationFindingReasonCode... findingReasonCodes) {
java.util.ArrayList findingReasonCodesCopy = new java.util.ArrayList(findingReasonCodes.length);
for (ECSServiceRecommendationFindingReasonCode value : findingReasonCodes) {
findingReasonCodesCopy.add(value.toString());
}
if (getFindingReasonCodes() == null) {
setFindingReasonCodes(findingReasonCodesCopy);
} else {
getFindingReasonCodes().addAll(findingReasonCodesCopy);
}
return this;
}
/**
*
* An array of objects that describe the recommendation options for the Amazon ECS service.
*
*
* @return An array of objects that describe the recommendation options for the Amazon ECS service.
*/
public java.util.List getServiceRecommendationOptions() {
return serviceRecommendationOptions;
}
/**
*
* An array of objects that describe the recommendation options for the Amazon ECS service.
*
*
* @param serviceRecommendationOptions
* An array of objects that describe the recommendation options for the Amazon ECS service.
*/
public void setServiceRecommendationOptions(java.util.Collection serviceRecommendationOptions) {
if (serviceRecommendationOptions == null) {
this.serviceRecommendationOptions = null;
return;
}
this.serviceRecommendationOptions = new java.util.ArrayList(serviceRecommendationOptions);
}
/**
*
* An array of objects that describe the recommendation options for the Amazon ECS service.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setServiceRecommendationOptions(java.util.Collection)} or
* {@link #withServiceRecommendationOptions(java.util.Collection)} if you want to override the existing values.
*
*
* @param serviceRecommendationOptions
* An array of objects that describe the recommendation options for the Amazon ECS service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ECSServiceRecommendation withServiceRecommendationOptions(ECSServiceRecommendationOption... serviceRecommendationOptions) {
if (this.serviceRecommendationOptions == null) {
setServiceRecommendationOptions(new java.util.ArrayList(serviceRecommendationOptions.length));
}
for (ECSServiceRecommendationOption ele : serviceRecommendationOptions) {
this.serviceRecommendationOptions.add(ele);
}
return this;
}
/**
*
* An array of objects that describe the recommendation options for the Amazon ECS service.
*
*
* @param serviceRecommendationOptions
* An array of objects that describe the recommendation options for the Amazon ECS service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ECSServiceRecommendation withServiceRecommendationOptions(java.util.Collection serviceRecommendationOptions) {
setServiceRecommendationOptions(serviceRecommendationOptions);
return this;
}
/**
*
* The risk of the current Amazon ECS service not meeting the performance needs of its workloads. The higher the
* risk, the more likely the current service can't meet the performance requirements of its workload.
*
*
* @param currentPerformanceRisk
* The risk of the current Amazon ECS service not meeting the performance needs of its workloads. The higher
* the risk, the more likely the current service can't meet the performance requirements of its workload.
* @see CurrentPerformanceRisk
*/
public void setCurrentPerformanceRisk(String currentPerformanceRisk) {
this.currentPerformanceRisk = currentPerformanceRisk;
}
/**
*
* The risk of the current Amazon ECS service not meeting the performance needs of its workloads. The higher the
* risk, the more likely the current service can't meet the performance requirements of its workload.
*
*
* @return The risk of the current Amazon ECS service not meeting the performance needs of its workloads. The higher
* the risk, the more likely the current service can't meet the performance requirements of its workload.
* @see CurrentPerformanceRisk
*/
public String getCurrentPerformanceRisk() {
return this.currentPerformanceRisk;
}
/**
*
* The risk of the current Amazon ECS service not meeting the performance needs of its workloads. The higher the
* risk, the more likely the current service can't meet the performance requirements of its workload.
*
*
* @param currentPerformanceRisk
* The risk of the current Amazon ECS service not meeting the performance needs of its workloads. The higher
* the risk, the more likely the current service can't meet the performance requirements of its workload.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CurrentPerformanceRisk
*/
public ECSServiceRecommendation withCurrentPerformanceRisk(String currentPerformanceRisk) {
setCurrentPerformanceRisk(currentPerformanceRisk);
return this;
}
/**
*
* The risk of the current Amazon ECS service not meeting the performance needs of its workloads. The higher the
* risk, the more likely the current service can't meet the performance requirements of its workload.
*
*
* @param currentPerformanceRisk
* The risk of the current Amazon ECS service not meeting the performance needs of its workloads. The higher
* the risk, the more likely the current service can't meet the performance requirements of its workload.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CurrentPerformanceRisk
*/
public ECSServiceRecommendation withCurrentPerformanceRisk(CurrentPerformanceRisk currentPerformanceRisk) {
this.currentPerformanceRisk = currentPerformanceRisk.toString();
return this;
}
/**
*
* Describes the effective recommendation preferences for Amazon ECS services.
*
*
* @param effectiveRecommendationPreferences
* Describes the effective recommendation preferences for Amazon ECS services.
*/
public void setEffectiveRecommendationPreferences(ECSEffectiveRecommendationPreferences effectiveRecommendationPreferences) {
this.effectiveRecommendationPreferences = effectiveRecommendationPreferences;
}
/**
*
* Describes the effective recommendation preferences for Amazon ECS services.
*
*
* @return Describes the effective recommendation preferences for Amazon ECS services.
*/
public ECSEffectiveRecommendationPreferences getEffectiveRecommendationPreferences() {
return this.effectiveRecommendationPreferences;
}
/**
*
* Describes the effective recommendation preferences for Amazon ECS services.
*
*
* @param effectiveRecommendationPreferences
* Describes the effective recommendation preferences for Amazon ECS services.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ECSServiceRecommendation withEffectiveRecommendationPreferences(ECSEffectiveRecommendationPreferences effectiveRecommendationPreferences) {
setEffectiveRecommendationPreferences(effectiveRecommendationPreferences);
return this;
}
/**
*
* A list of tags assigned to your Amazon ECS service recommendations.
*
*
* @return A list of tags assigned to your Amazon ECS service recommendations.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* A list of tags assigned to your Amazon ECS service recommendations.
*
*
* @param tags
* A list of tags assigned to your Amazon ECS service recommendations.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* A list of tags assigned to your Amazon ECS service recommendations.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* A list of tags assigned to your Amazon ECS service recommendations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ECSServiceRecommendation withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* A list of tags assigned to your Amazon ECS service recommendations.
*
*
* @param tags
* A list of tags assigned to your Amazon ECS service recommendations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ECSServiceRecommendation withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getServiceArn() != null)
sb.append("ServiceArn: ").append(getServiceArn()).append(",");
if (getAccountId() != null)
sb.append("AccountId: ").append(getAccountId()).append(",");
if (getCurrentServiceConfiguration() != null)
sb.append("CurrentServiceConfiguration: ").append(getCurrentServiceConfiguration()).append(",");
if (getUtilizationMetrics() != null)
sb.append("UtilizationMetrics: ").append(getUtilizationMetrics()).append(",");
if (getLookbackPeriodInDays() != null)
sb.append("LookbackPeriodInDays: ").append(getLookbackPeriodInDays()).append(",");
if (getLaunchType() != null)
sb.append("LaunchType: ").append(getLaunchType()).append(",");
if (getLastRefreshTimestamp() != null)
sb.append("LastRefreshTimestamp: ").append(getLastRefreshTimestamp()).append(",");
if (getFinding() != null)
sb.append("Finding: ").append(getFinding()).append(",");
if (getFindingReasonCodes() != null)
sb.append("FindingReasonCodes: ").append(getFindingReasonCodes()).append(",");
if (getServiceRecommendationOptions() != null)
sb.append("ServiceRecommendationOptions: ").append(getServiceRecommendationOptions()).append(",");
if (getCurrentPerformanceRisk() != null)
sb.append("CurrentPerformanceRisk: ").append(getCurrentPerformanceRisk()).append(",");
if (getEffectiveRecommendationPreferences() != null)
sb.append("EffectiveRecommendationPreferences: ").append(getEffectiveRecommendationPreferences()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ECSServiceRecommendation == false)
return false;
ECSServiceRecommendation other = (ECSServiceRecommendation) obj;
if (other.getServiceArn() == null ^ this.getServiceArn() == null)
return false;
if (other.getServiceArn() != null && other.getServiceArn().equals(this.getServiceArn()) == false)
return false;
if (other.getAccountId() == null ^ this.getAccountId() == null)
return false;
if (other.getAccountId() != null && other.getAccountId().equals(this.getAccountId()) == false)
return false;
if (other.getCurrentServiceConfiguration() == null ^ this.getCurrentServiceConfiguration() == null)
return false;
if (other.getCurrentServiceConfiguration() != null && other.getCurrentServiceConfiguration().equals(this.getCurrentServiceConfiguration()) == false)
return false;
if (other.getUtilizationMetrics() == null ^ this.getUtilizationMetrics() == null)
return false;
if (other.getUtilizationMetrics() != null && other.getUtilizationMetrics().equals(this.getUtilizationMetrics()) == false)
return false;
if (other.getLookbackPeriodInDays() == null ^ this.getLookbackPeriodInDays() == null)
return false;
if (other.getLookbackPeriodInDays() != null && other.getLookbackPeriodInDays().equals(this.getLookbackPeriodInDays()) == false)
return false;
if (other.getLaunchType() == null ^ this.getLaunchType() == null)
return false;
if (other.getLaunchType() != null && other.getLaunchType().equals(this.getLaunchType()) == false)
return false;
if (other.getLastRefreshTimestamp() == null ^ this.getLastRefreshTimestamp() == null)
return false;
if (other.getLastRefreshTimestamp() != null && other.getLastRefreshTimestamp().equals(this.getLastRefreshTimestamp()) == false)
return false;
if (other.getFinding() == null ^ this.getFinding() == null)
return false;
if (other.getFinding() != null && other.getFinding().equals(this.getFinding()) == false)
return false;
if (other.getFindingReasonCodes() == null ^ this.getFindingReasonCodes() == null)
return false;
if (other.getFindingReasonCodes() != null && other.getFindingReasonCodes().equals(this.getFindingReasonCodes()) == false)
return false;
if (other.getServiceRecommendationOptions() == null ^ this.getServiceRecommendationOptions() == null)
return false;
if (other.getServiceRecommendationOptions() != null && other.getServiceRecommendationOptions().equals(this.getServiceRecommendationOptions()) == false)
return false;
if (other.getCurrentPerformanceRisk() == null ^ this.getCurrentPerformanceRisk() == null)
return false;
if (other.getCurrentPerformanceRisk() != null && other.getCurrentPerformanceRisk().equals(this.getCurrentPerformanceRisk()) == false)
return false;
if (other.getEffectiveRecommendationPreferences() == null ^ this.getEffectiveRecommendationPreferences() == null)
return false;
if (other.getEffectiveRecommendationPreferences() != null
&& other.getEffectiveRecommendationPreferences().equals(this.getEffectiveRecommendationPreferences()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getServiceArn() == null) ? 0 : getServiceArn().hashCode());
hashCode = prime * hashCode + ((getAccountId() == null) ? 0 : getAccountId().hashCode());
hashCode = prime * hashCode + ((getCurrentServiceConfiguration() == null) ? 0 : getCurrentServiceConfiguration().hashCode());
hashCode = prime * hashCode + ((getUtilizationMetrics() == null) ? 0 : getUtilizationMetrics().hashCode());
hashCode = prime * hashCode + ((getLookbackPeriodInDays() == null) ? 0 : getLookbackPeriodInDays().hashCode());
hashCode = prime * hashCode + ((getLaunchType() == null) ? 0 : getLaunchType().hashCode());
hashCode = prime * hashCode + ((getLastRefreshTimestamp() == null) ? 0 : getLastRefreshTimestamp().hashCode());
hashCode = prime * hashCode + ((getFinding() == null) ? 0 : getFinding().hashCode());
hashCode = prime * hashCode + ((getFindingReasonCodes() == null) ? 0 : getFindingReasonCodes().hashCode());
hashCode = prime * hashCode + ((getServiceRecommendationOptions() == null) ? 0 : getServiceRecommendationOptions().hashCode());
hashCode = prime * hashCode + ((getCurrentPerformanceRisk() == null) ? 0 : getCurrentPerformanceRisk().hashCode());
hashCode = prime * hashCode + ((getEffectiveRecommendationPreferences() == null) ? 0 : getEffectiveRecommendationPreferences().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public ECSServiceRecommendation clone() {
try {
return (ECSServiceRecommendation) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.computeoptimizer.model.transform.ECSServiceRecommendationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}