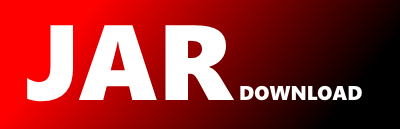
com.amazonaws.services.computeoptimizer.model.EffectiveRecommendationPreferences Maven / Gradle / Ivy
Show all versions of aws-java-sdk-computeoptimizer Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.computeoptimizer.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes the effective recommendation preferences for a resource.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class EffectiveRecommendationPreferences implements Serializable, Cloneable, StructuredPojo {
/**
*
* Describes the CPU vendor and architecture for an instance or Auto Scaling group recommendations.
*
*
* For example, when you specify AWS_ARM64
with:
*
*
* -
*
* A GetEC2InstanceRecommendations or GetAutoScalingGroupRecommendations request, Compute Optimizer
* returns recommendations that consist of Graviton instance types only.
*
*
* -
*
* A GetEC2RecommendationProjectedMetrics request, Compute Optimizer returns projected utilization metrics
* for Graviton instance type recommendations only.
*
*
* -
*
* A ExportEC2InstanceRecommendations or ExportAutoScalingGroupRecommendations request, Compute
* Optimizer exports recommendations that consist of Graviton instance types only.
*
*
*
*/
private java.util.List cpuVendorArchitectures;
/**
*
* Describes the activation status of the enhanced infrastructure metrics preference.
*
*
* A status of Active
confirms that the preference is applied in the latest recommendation refresh, and
* a status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* For more information, see Enhanced
* infrastructure metrics in the Compute Optimizer User Guide.
*
*/
private String enhancedInfrastructureMetrics;
/**
*
* Describes the activation status of the inferred workload types preference.
*
*
* A status of Active
confirms that the preference is applied in the latest recommendation refresh. A
* status of Inactive
confirms that it's not yet applied to recommendations.
*
*/
private String inferredWorkloadTypes;
/**
*
* An object that describes the external metrics recommendation preference.
*
*
* If the preference is applied in the latest recommendation refresh, an object with a valid source
* value appears in the response. If the preference isn't applied to the recommendations already, then this object
* doesn't appear in the response.
*
*/
private ExternalMetricsPreference externalMetricsPreference;
/**
*
* The number of days the utilization metrics of the Amazon Web Services resource are analyzed.
*
*/
private String lookBackPeriod;
/**
*
* The resource’s CPU and memory utilization preferences, such as threshold and headroom, that are used to generate
* rightsizing recommendations.
*
*
*
* This preference is only available for the Amazon EC2 instance resource type.
*
*
*/
private java.util.List utilizationPreferences;
/**
*
* The resource type values that are considered as candidates when generating rightsizing recommendations.
*
*/
private java.util.List preferredResources;
/**
*
* Describes the savings estimation mode applied for calculating savings opportunity for a resource.
*
*/
private InstanceSavingsEstimationMode savingsEstimationMode;
/**
*
* Describes the CPU vendor and architecture for an instance or Auto Scaling group recommendations.
*
*
* For example, when you specify AWS_ARM64
with:
*
*
* -
*
* A GetEC2InstanceRecommendations or GetAutoScalingGroupRecommendations request, Compute Optimizer
* returns recommendations that consist of Graviton instance types only.
*
*
* -
*
* A GetEC2RecommendationProjectedMetrics request, Compute Optimizer returns projected utilization metrics
* for Graviton instance type recommendations only.
*
*
* -
*
* A ExportEC2InstanceRecommendations or ExportAutoScalingGroupRecommendations request, Compute
* Optimizer exports recommendations that consist of Graviton instance types only.
*
*
*
*
* @return Describes the CPU vendor and architecture for an instance or Auto Scaling group recommendations.
*
* For example, when you specify AWS_ARM64
with:
*
*
* -
*
* A GetEC2InstanceRecommendations or GetAutoScalingGroupRecommendations request, Compute
* Optimizer returns recommendations that consist of Graviton instance types only.
*
*
* -
*
* A GetEC2RecommendationProjectedMetrics request, Compute Optimizer returns projected utilization
* metrics for Graviton instance type recommendations only.
*
*
* -
*
* A ExportEC2InstanceRecommendations or ExportAutoScalingGroupRecommendations request,
* Compute Optimizer exports recommendations that consist of Graviton instance types only.
*
*
* @see CpuVendorArchitecture
*/
public java.util.List getCpuVendorArchitectures() {
return cpuVendorArchitectures;
}
/**
*
* Describes the CPU vendor and architecture for an instance or Auto Scaling group recommendations.
*
*
* For example, when you specify AWS_ARM64
with:
*
*
* -
*
* A GetEC2InstanceRecommendations or GetAutoScalingGroupRecommendations request, Compute Optimizer
* returns recommendations that consist of Graviton instance types only.
*
*
* -
*
* A GetEC2RecommendationProjectedMetrics request, Compute Optimizer returns projected utilization metrics
* for Graviton instance type recommendations only.
*
*
* -
*
* A ExportEC2InstanceRecommendations or ExportAutoScalingGroupRecommendations request, Compute
* Optimizer exports recommendations that consist of Graviton instance types only.
*
*
*
*
* @param cpuVendorArchitectures
* Describes the CPU vendor and architecture for an instance or Auto Scaling group recommendations.
*
* For example, when you specify AWS_ARM64
with:
*
*
* -
*
* A GetEC2InstanceRecommendations or GetAutoScalingGroupRecommendations request, Compute
* Optimizer returns recommendations that consist of Graviton instance types only.
*
*
* -
*
* A GetEC2RecommendationProjectedMetrics request, Compute Optimizer returns projected utilization
* metrics for Graviton instance type recommendations only.
*
*
* -
*
* A ExportEC2InstanceRecommendations or ExportAutoScalingGroupRecommendations request, Compute
* Optimizer exports recommendations that consist of Graviton instance types only.
*
*
* @see CpuVendorArchitecture
*/
public void setCpuVendorArchitectures(java.util.Collection cpuVendorArchitectures) {
if (cpuVendorArchitectures == null) {
this.cpuVendorArchitectures = null;
return;
}
this.cpuVendorArchitectures = new java.util.ArrayList(cpuVendorArchitectures);
}
/**
*
* Describes the CPU vendor and architecture for an instance or Auto Scaling group recommendations.
*
*
* For example, when you specify AWS_ARM64
with:
*
*
* -
*
* A GetEC2InstanceRecommendations or GetAutoScalingGroupRecommendations request, Compute Optimizer
* returns recommendations that consist of Graviton instance types only.
*
*
* -
*
* A GetEC2RecommendationProjectedMetrics request, Compute Optimizer returns projected utilization metrics
* for Graviton instance type recommendations only.
*
*
* -
*
* A ExportEC2InstanceRecommendations or ExportAutoScalingGroupRecommendations request, Compute
* Optimizer exports recommendations that consist of Graviton instance types only.
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCpuVendorArchitectures(java.util.Collection)} or
* {@link #withCpuVendorArchitectures(java.util.Collection)} if you want to override the existing values.
*
*
* @param cpuVendorArchitectures
* Describes the CPU vendor and architecture for an instance or Auto Scaling group recommendations.
*
* For example, when you specify AWS_ARM64
with:
*
*
* -
*
* A GetEC2InstanceRecommendations or GetAutoScalingGroupRecommendations request, Compute
* Optimizer returns recommendations that consist of Graviton instance types only.
*
*
* -
*
* A GetEC2RecommendationProjectedMetrics request, Compute Optimizer returns projected utilization
* metrics for Graviton instance type recommendations only.
*
*
* -
*
* A ExportEC2InstanceRecommendations or ExportAutoScalingGroupRecommendations request, Compute
* Optimizer exports recommendations that consist of Graviton instance types only.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see CpuVendorArchitecture
*/
public EffectiveRecommendationPreferences withCpuVendorArchitectures(String... cpuVendorArchitectures) {
if (this.cpuVendorArchitectures == null) {
setCpuVendorArchitectures(new java.util.ArrayList(cpuVendorArchitectures.length));
}
for (String ele : cpuVendorArchitectures) {
this.cpuVendorArchitectures.add(ele);
}
return this;
}
/**
*
* Describes the CPU vendor and architecture for an instance or Auto Scaling group recommendations.
*
*
* For example, when you specify AWS_ARM64
with:
*
*
* -
*
* A GetEC2InstanceRecommendations or GetAutoScalingGroupRecommendations request, Compute Optimizer
* returns recommendations that consist of Graviton instance types only.
*
*
* -
*
* A GetEC2RecommendationProjectedMetrics request, Compute Optimizer returns projected utilization metrics
* for Graviton instance type recommendations only.
*
*
* -
*
* A ExportEC2InstanceRecommendations or ExportAutoScalingGroupRecommendations request, Compute
* Optimizer exports recommendations that consist of Graviton instance types only.
*
*
*
*
* @param cpuVendorArchitectures
* Describes the CPU vendor and architecture for an instance or Auto Scaling group recommendations.
*
* For example, when you specify AWS_ARM64
with:
*
*
* -
*
* A GetEC2InstanceRecommendations or GetAutoScalingGroupRecommendations request, Compute
* Optimizer returns recommendations that consist of Graviton instance types only.
*
*
* -
*
* A GetEC2RecommendationProjectedMetrics request, Compute Optimizer returns projected utilization
* metrics for Graviton instance type recommendations only.
*
*
* -
*
* A ExportEC2InstanceRecommendations or ExportAutoScalingGroupRecommendations request, Compute
* Optimizer exports recommendations that consist of Graviton instance types only.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see CpuVendorArchitecture
*/
public EffectiveRecommendationPreferences withCpuVendorArchitectures(java.util.Collection cpuVendorArchitectures) {
setCpuVendorArchitectures(cpuVendorArchitectures);
return this;
}
/**
*
* Describes the CPU vendor and architecture for an instance or Auto Scaling group recommendations.
*
*
* For example, when you specify AWS_ARM64
with:
*
*
* -
*
* A GetEC2InstanceRecommendations or GetAutoScalingGroupRecommendations request, Compute Optimizer
* returns recommendations that consist of Graviton instance types only.
*
*
* -
*
* A GetEC2RecommendationProjectedMetrics request, Compute Optimizer returns projected utilization metrics
* for Graviton instance type recommendations only.
*
*
* -
*
* A ExportEC2InstanceRecommendations or ExportAutoScalingGroupRecommendations request, Compute
* Optimizer exports recommendations that consist of Graviton instance types only.
*
*
*
*
* @param cpuVendorArchitectures
* Describes the CPU vendor and architecture for an instance or Auto Scaling group recommendations.
*
* For example, when you specify AWS_ARM64
with:
*
*
* -
*
* A GetEC2InstanceRecommendations or GetAutoScalingGroupRecommendations request, Compute
* Optimizer returns recommendations that consist of Graviton instance types only.
*
*
* -
*
* A GetEC2RecommendationProjectedMetrics request, Compute Optimizer returns projected utilization
* metrics for Graviton instance type recommendations only.
*
*
* -
*
* A ExportEC2InstanceRecommendations or ExportAutoScalingGroupRecommendations request, Compute
* Optimizer exports recommendations that consist of Graviton instance types only.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see CpuVendorArchitecture
*/
public EffectiveRecommendationPreferences withCpuVendorArchitectures(CpuVendorArchitecture... cpuVendorArchitectures) {
java.util.ArrayList cpuVendorArchitecturesCopy = new java.util.ArrayList(cpuVendorArchitectures.length);
for (CpuVendorArchitecture value : cpuVendorArchitectures) {
cpuVendorArchitecturesCopy.add(value.toString());
}
if (getCpuVendorArchitectures() == null) {
setCpuVendorArchitectures(cpuVendorArchitecturesCopy);
} else {
getCpuVendorArchitectures().addAll(cpuVendorArchitecturesCopy);
}
return this;
}
/**
*
* Describes the activation status of the enhanced infrastructure metrics preference.
*
*
* A status of Active
confirms that the preference is applied in the latest recommendation refresh, and
* a status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* For more information, see Enhanced
* infrastructure metrics in the Compute Optimizer User Guide.
*
*
* @param enhancedInfrastructureMetrics
* Describes the activation status of the enhanced infrastructure metrics preference.
*
* A status of Active
confirms that the preference is applied in the latest recommendation
* refresh, and a status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* For more information, see Enhanced infrastructure metrics in the Compute Optimizer User Guide.
* @see EnhancedInfrastructureMetrics
*/
public void setEnhancedInfrastructureMetrics(String enhancedInfrastructureMetrics) {
this.enhancedInfrastructureMetrics = enhancedInfrastructureMetrics;
}
/**
*
* Describes the activation status of the enhanced infrastructure metrics preference.
*
*
* A status of Active
confirms that the preference is applied in the latest recommendation refresh, and
* a status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* For more information, see Enhanced
* infrastructure metrics in the Compute Optimizer User Guide.
*
*
* @return Describes the activation status of the enhanced infrastructure metrics preference.
*
* A status of Active
confirms that the preference is applied in the latest recommendation
* refresh, and a status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* For more information, see Enhanced infrastructure metrics in the Compute Optimizer User Guide.
* @see EnhancedInfrastructureMetrics
*/
public String getEnhancedInfrastructureMetrics() {
return this.enhancedInfrastructureMetrics;
}
/**
*
* Describes the activation status of the enhanced infrastructure metrics preference.
*
*
* A status of Active
confirms that the preference is applied in the latest recommendation refresh, and
* a status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* For more information, see Enhanced
* infrastructure metrics in the Compute Optimizer User Guide.
*
*
* @param enhancedInfrastructureMetrics
* Describes the activation status of the enhanced infrastructure metrics preference.
*
* A status of Active
confirms that the preference is applied in the latest recommendation
* refresh, and a status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* For more information, see Enhanced infrastructure metrics in the Compute Optimizer User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnhancedInfrastructureMetrics
*/
public EffectiveRecommendationPreferences withEnhancedInfrastructureMetrics(String enhancedInfrastructureMetrics) {
setEnhancedInfrastructureMetrics(enhancedInfrastructureMetrics);
return this;
}
/**
*
* Describes the activation status of the enhanced infrastructure metrics preference.
*
*
* A status of Active
confirms that the preference is applied in the latest recommendation refresh, and
* a status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* For more information, see Enhanced
* infrastructure metrics in the Compute Optimizer User Guide.
*
*
* @param enhancedInfrastructureMetrics
* Describes the activation status of the enhanced infrastructure metrics preference.
*
* A status of Active
confirms that the preference is applied in the latest recommendation
* refresh, and a status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* For more information, see Enhanced infrastructure metrics in the Compute Optimizer User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnhancedInfrastructureMetrics
*/
public EffectiveRecommendationPreferences withEnhancedInfrastructureMetrics(EnhancedInfrastructureMetrics enhancedInfrastructureMetrics) {
this.enhancedInfrastructureMetrics = enhancedInfrastructureMetrics.toString();
return this;
}
/**
*
* Describes the activation status of the inferred workload types preference.
*
*
* A status of Active
confirms that the preference is applied in the latest recommendation refresh. A
* status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* @param inferredWorkloadTypes
* Describes the activation status of the inferred workload types preference.
*
* A status of Active
confirms that the preference is applied in the latest recommendation
* refresh. A status of Inactive
confirms that it's not yet applied to recommendations.
* @see InferredWorkloadTypesPreference
*/
public void setInferredWorkloadTypes(String inferredWorkloadTypes) {
this.inferredWorkloadTypes = inferredWorkloadTypes;
}
/**
*
* Describes the activation status of the inferred workload types preference.
*
*
* A status of Active
confirms that the preference is applied in the latest recommendation refresh. A
* status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* @return Describes the activation status of the inferred workload types preference.
*
* A status of Active
confirms that the preference is applied in the latest recommendation
* refresh. A status of Inactive
confirms that it's not yet applied to recommendations.
* @see InferredWorkloadTypesPreference
*/
public String getInferredWorkloadTypes() {
return this.inferredWorkloadTypes;
}
/**
*
* Describes the activation status of the inferred workload types preference.
*
*
* A status of Active
confirms that the preference is applied in the latest recommendation refresh. A
* status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* @param inferredWorkloadTypes
* Describes the activation status of the inferred workload types preference.
*
* A status of Active
confirms that the preference is applied in the latest recommendation
* refresh. A status of Inactive
confirms that it's not yet applied to recommendations.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InferredWorkloadTypesPreference
*/
public EffectiveRecommendationPreferences withInferredWorkloadTypes(String inferredWorkloadTypes) {
setInferredWorkloadTypes(inferredWorkloadTypes);
return this;
}
/**
*
* Describes the activation status of the inferred workload types preference.
*
*
* A status of Active
confirms that the preference is applied in the latest recommendation refresh. A
* status of Inactive
confirms that it's not yet applied to recommendations.
*
*
* @param inferredWorkloadTypes
* Describes the activation status of the inferred workload types preference.
*
* A status of Active
confirms that the preference is applied in the latest recommendation
* refresh. A status of Inactive
confirms that it's not yet applied to recommendations.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InferredWorkloadTypesPreference
*/
public EffectiveRecommendationPreferences withInferredWorkloadTypes(InferredWorkloadTypesPreference inferredWorkloadTypes) {
this.inferredWorkloadTypes = inferredWorkloadTypes.toString();
return this;
}
/**
*
* An object that describes the external metrics recommendation preference.
*
*
* If the preference is applied in the latest recommendation refresh, an object with a valid source
* value appears in the response. If the preference isn't applied to the recommendations already, then this object
* doesn't appear in the response.
*
*
* @param externalMetricsPreference
* An object that describes the external metrics recommendation preference.
*
* If the preference is applied in the latest recommendation refresh, an object with a valid
* source
value appears in the response. If the preference isn't applied to the recommendations
* already, then this object doesn't appear in the response.
*/
public void setExternalMetricsPreference(ExternalMetricsPreference externalMetricsPreference) {
this.externalMetricsPreference = externalMetricsPreference;
}
/**
*
* An object that describes the external metrics recommendation preference.
*
*
* If the preference is applied in the latest recommendation refresh, an object with a valid source
* value appears in the response. If the preference isn't applied to the recommendations already, then this object
* doesn't appear in the response.
*
*
* @return An object that describes the external metrics recommendation preference.
*
* If the preference is applied in the latest recommendation refresh, an object with a valid
* source
value appears in the response. If the preference isn't applied to the recommendations
* already, then this object doesn't appear in the response.
*/
public ExternalMetricsPreference getExternalMetricsPreference() {
return this.externalMetricsPreference;
}
/**
*
* An object that describes the external metrics recommendation preference.
*
*
* If the preference is applied in the latest recommendation refresh, an object with a valid source
* value appears in the response. If the preference isn't applied to the recommendations already, then this object
* doesn't appear in the response.
*
*
* @param externalMetricsPreference
* An object that describes the external metrics recommendation preference.
*
* If the preference is applied in the latest recommendation refresh, an object with a valid
* source
value appears in the response. If the preference isn't applied to the recommendations
* already, then this object doesn't appear in the response.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EffectiveRecommendationPreferences withExternalMetricsPreference(ExternalMetricsPreference externalMetricsPreference) {
setExternalMetricsPreference(externalMetricsPreference);
return this;
}
/**
*
* The number of days the utilization metrics of the Amazon Web Services resource are analyzed.
*
*
* @param lookBackPeriod
* The number of days the utilization metrics of the Amazon Web Services resource are analyzed.
* @see LookBackPeriodPreference
*/
public void setLookBackPeriod(String lookBackPeriod) {
this.lookBackPeriod = lookBackPeriod;
}
/**
*
* The number of days the utilization metrics of the Amazon Web Services resource are analyzed.
*
*
* @return The number of days the utilization metrics of the Amazon Web Services resource are analyzed.
* @see LookBackPeriodPreference
*/
public String getLookBackPeriod() {
return this.lookBackPeriod;
}
/**
*
* The number of days the utilization metrics of the Amazon Web Services resource are analyzed.
*
*
* @param lookBackPeriod
* The number of days the utilization metrics of the Amazon Web Services resource are analyzed.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LookBackPeriodPreference
*/
public EffectiveRecommendationPreferences withLookBackPeriod(String lookBackPeriod) {
setLookBackPeriod(lookBackPeriod);
return this;
}
/**
*
* The number of days the utilization metrics of the Amazon Web Services resource are analyzed.
*
*
* @param lookBackPeriod
* The number of days the utilization metrics of the Amazon Web Services resource are analyzed.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LookBackPeriodPreference
*/
public EffectiveRecommendationPreferences withLookBackPeriod(LookBackPeriodPreference lookBackPeriod) {
this.lookBackPeriod = lookBackPeriod.toString();
return this;
}
/**
*
* The resource’s CPU and memory utilization preferences, such as threshold and headroom, that are used to generate
* rightsizing recommendations.
*
*
*
* This preference is only available for the Amazon EC2 instance resource type.
*
*
*
* @return The resource’s CPU and memory utilization preferences, such as threshold and headroom, that are used to
* generate rightsizing recommendations.
*
* This preference is only available for the Amazon EC2 instance resource type.
*
*/
public java.util.List getUtilizationPreferences() {
return utilizationPreferences;
}
/**
*
* The resource’s CPU and memory utilization preferences, such as threshold and headroom, that are used to generate
* rightsizing recommendations.
*
*
*
* This preference is only available for the Amazon EC2 instance resource type.
*
*
*
* @param utilizationPreferences
* The resource’s CPU and memory utilization preferences, such as threshold and headroom, that are used to
* generate rightsizing recommendations.
*
* This preference is only available for the Amazon EC2 instance resource type.
*
*/
public void setUtilizationPreferences(java.util.Collection utilizationPreferences) {
if (utilizationPreferences == null) {
this.utilizationPreferences = null;
return;
}
this.utilizationPreferences = new java.util.ArrayList(utilizationPreferences);
}
/**
*
* The resource’s CPU and memory utilization preferences, such as threshold and headroom, that are used to generate
* rightsizing recommendations.
*
*
*
* This preference is only available for the Amazon EC2 instance resource type.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setUtilizationPreferences(java.util.Collection)} or
* {@link #withUtilizationPreferences(java.util.Collection)} if you want to override the existing values.
*
*
* @param utilizationPreferences
* The resource’s CPU and memory utilization preferences, such as threshold and headroom, that are used to
* generate rightsizing recommendations.
*
* This preference is only available for the Amazon EC2 instance resource type.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EffectiveRecommendationPreferences withUtilizationPreferences(UtilizationPreference... utilizationPreferences) {
if (this.utilizationPreferences == null) {
setUtilizationPreferences(new java.util.ArrayList(utilizationPreferences.length));
}
for (UtilizationPreference ele : utilizationPreferences) {
this.utilizationPreferences.add(ele);
}
return this;
}
/**
*
* The resource’s CPU and memory utilization preferences, such as threshold and headroom, that are used to generate
* rightsizing recommendations.
*
*
*
* This preference is only available for the Amazon EC2 instance resource type.
*
*
*
* @param utilizationPreferences
* The resource’s CPU and memory utilization preferences, such as threshold and headroom, that are used to
* generate rightsizing recommendations.
*
* This preference is only available for the Amazon EC2 instance resource type.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EffectiveRecommendationPreferences withUtilizationPreferences(java.util.Collection utilizationPreferences) {
setUtilizationPreferences(utilizationPreferences);
return this;
}
/**
*
* The resource type values that are considered as candidates when generating rightsizing recommendations.
*
*
* @return The resource type values that are considered as candidates when generating rightsizing recommendations.
*/
public java.util.List getPreferredResources() {
return preferredResources;
}
/**
*
* The resource type values that are considered as candidates when generating rightsizing recommendations.
*
*
* @param preferredResources
* The resource type values that are considered as candidates when generating rightsizing recommendations.
*/
public void setPreferredResources(java.util.Collection preferredResources) {
if (preferredResources == null) {
this.preferredResources = null;
return;
}
this.preferredResources = new java.util.ArrayList(preferredResources);
}
/**
*
* The resource type values that are considered as candidates when generating rightsizing recommendations.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPreferredResources(java.util.Collection)} or {@link #withPreferredResources(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param preferredResources
* The resource type values that are considered as candidates when generating rightsizing recommendations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EffectiveRecommendationPreferences withPreferredResources(EffectivePreferredResource... preferredResources) {
if (this.preferredResources == null) {
setPreferredResources(new java.util.ArrayList(preferredResources.length));
}
for (EffectivePreferredResource ele : preferredResources) {
this.preferredResources.add(ele);
}
return this;
}
/**
*
* The resource type values that are considered as candidates when generating rightsizing recommendations.
*
*
* @param preferredResources
* The resource type values that are considered as candidates when generating rightsizing recommendations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EffectiveRecommendationPreferences withPreferredResources(java.util.Collection preferredResources) {
setPreferredResources(preferredResources);
return this;
}
/**
*
* Describes the savings estimation mode applied for calculating savings opportunity for a resource.
*
*
* @param savingsEstimationMode
* Describes the savings estimation mode applied for calculating savings opportunity for a resource.
*/
public void setSavingsEstimationMode(InstanceSavingsEstimationMode savingsEstimationMode) {
this.savingsEstimationMode = savingsEstimationMode;
}
/**
*
* Describes the savings estimation mode applied for calculating savings opportunity for a resource.
*
*
* @return Describes the savings estimation mode applied for calculating savings opportunity for a resource.
*/
public InstanceSavingsEstimationMode getSavingsEstimationMode() {
return this.savingsEstimationMode;
}
/**
*
* Describes the savings estimation mode applied for calculating savings opportunity for a resource.
*
*
* @param savingsEstimationMode
* Describes the savings estimation mode applied for calculating savings opportunity for a resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EffectiveRecommendationPreferences withSavingsEstimationMode(InstanceSavingsEstimationMode savingsEstimationMode) {
setSavingsEstimationMode(savingsEstimationMode);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCpuVendorArchitectures() != null)
sb.append("CpuVendorArchitectures: ").append(getCpuVendorArchitectures()).append(",");
if (getEnhancedInfrastructureMetrics() != null)
sb.append("EnhancedInfrastructureMetrics: ").append(getEnhancedInfrastructureMetrics()).append(",");
if (getInferredWorkloadTypes() != null)
sb.append("InferredWorkloadTypes: ").append(getInferredWorkloadTypes()).append(",");
if (getExternalMetricsPreference() != null)
sb.append("ExternalMetricsPreference: ").append(getExternalMetricsPreference()).append(",");
if (getLookBackPeriod() != null)
sb.append("LookBackPeriod: ").append(getLookBackPeriod()).append(",");
if (getUtilizationPreferences() != null)
sb.append("UtilizationPreferences: ").append(getUtilizationPreferences()).append(",");
if (getPreferredResources() != null)
sb.append("PreferredResources: ").append(getPreferredResources()).append(",");
if (getSavingsEstimationMode() != null)
sb.append("SavingsEstimationMode: ").append(getSavingsEstimationMode());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof EffectiveRecommendationPreferences == false)
return false;
EffectiveRecommendationPreferences other = (EffectiveRecommendationPreferences) obj;
if (other.getCpuVendorArchitectures() == null ^ this.getCpuVendorArchitectures() == null)
return false;
if (other.getCpuVendorArchitectures() != null && other.getCpuVendorArchitectures().equals(this.getCpuVendorArchitectures()) == false)
return false;
if (other.getEnhancedInfrastructureMetrics() == null ^ this.getEnhancedInfrastructureMetrics() == null)
return false;
if (other.getEnhancedInfrastructureMetrics() != null
&& other.getEnhancedInfrastructureMetrics().equals(this.getEnhancedInfrastructureMetrics()) == false)
return false;
if (other.getInferredWorkloadTypes() == null ^ this.getInferredWorkloadTypes() == null)
return false;
if (other.getInferredWorkloadTypes() != null && other.getInferredWorkloadTypes().equals(this.getInferredWorkloadTypes()) == false)
return false;
if (other.getExternalMetricsPreference() == null ^ this.getExternalMetricsPreference() == null)
return false;
if (other.getExternalMetricsPreference() != null && other.getExternalMetricsPreference().equals(this.getExternalMetricsPreference()) == false)
return false;
if (other.getLookBackPeriod() == null ^ this.getLookBackPeriod() == null)
return false;
if (other.getLookBackPeriod() != null && other.getLookBackPeriod().equals(this.getLookBackPeriod()) == false)
return false;
if (other.getUtilizationPreferences() == null ^ this.getUtilizationPreferences() == null)
return false;
if (other.getUtilizationPreferences() != null && other.getUtilizationPreferences().equals(this.getUtilizationPreferences()) == false)
return false;
if (other.getPreferredResources() == null ^ this.getPreferredResources() == null)
return false;
if (other.getPreferredResources() != null && other.getPreferredResources().equals(this.getPreferredResources()) == false)
return false;
if (other.getSavingsEstimationMode() == null ^ this.getSavingsEstimationMode() == null)
return false;
if (other.getSavingsEstimationMode() != null && other.getSavingsEstimationMode().equals(this.getSavingsEstimationMode()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCpuVendorArchitectures() == null) ? 0 : getCpuVendorArchitectures().hashCode());
hashCode = prime * hashCode + ((getEnhancedInfrastructureMetrics() == null) ? 0 : getEnhancedInfrastructureMetrics().hashCode());
hashCode = prime * hashCode + ((getInferredWorkloadTypes() == null) ? 0 : getInferredWorkloadTypes().hashCode());
hashCode = prime * hashCode + ((getExternalMetricsPreference() == null) ? 0 : getExternalMetricsPreference().hashCode());
hashCode = prime * hashCode + ((getLookBackPeriod() == null) ? 0 : getLookBackPeriod().hashCode());
hashCode = prime * hashCode + ((getUtilizationPreferences() == null) ? 0 : getUtilizationPreferences().hashCode());
hashCode = prime * hashCode + ((getPreferredResources() == null) ? 0 : getPreferredResources().hashCode());
hashCode = prime * hashCode + ((getSavingsEstimationMode() == null) ? 0 : getSavingsEstimationMode().hashCode());
return hashCode;
}
@Override
public EffectiveRecommendationPreferences clone() {
try {
return (EffectiveRecommendationPreferences) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.computeoptimizer.model.transform.EffectiveRecommendationPreferencesMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}