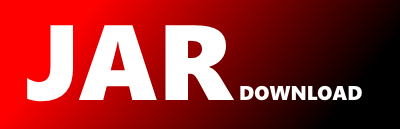
com.amazonaws.services.computeoptimizer.model.LicenseRecommendation Maven / Gradle / Ivy
Show all versions of aws-java-sdk-computeoptimizer Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.computeoptimizer.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes a license recommendation for an EC2 instance.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class LicenseRecommendation implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ARN that identifies the Amazon EC2 instance.
*
*/
private String resourceArn;
/**
*
* The Amazon Web Services account ID of the license.
*
*/
private String accountId;
/**
*
* An object that describes the current configuration of an instance that runs on a license.
*
*/
private LicenseConfiguration currentLicenseConfiguration;
/**
*
* The number of days for which utilization metrics were analyzed for an instance that runs on a license.
*
*/
private Double lookbackPeriodInDays;
/**
*
* The timestamp of when the license recommendation was last generated.
*
*/
private java.util.Date lastRefreshTimestamp;
/**
*
* The finding classification for an instance that runs on a license.
*
*
* Findings include:
*
*
* -
*
* InsufficentMetrics
— When Compute Optimizer detects that your CloudWatch Application Insights isn't
* enabled or is enabled with insufficient permissions.
*
*
* -
*
* NotOptimized
— When Compute Optimizer detects that your EC2 infrastructure isn't using any of the
* SQL server license features you're paying for, a license is considered not optimized.
*
*
* -
*
* Optimized
— When Compute Optimizer detects that all specifications of your license meet the
* performance requirements of your workload.
*
*
*
*/
private String finding;
/**
*
* The reason for the finding classification for an instance that runs on a license.
*
*
* Finding reason codes include:
*
*
* -
*
* Optimized
— All specifications of your license meet the performance requirements of your workload.
*
*
* -
*
* LicenseOverprovisioned
— A license is considered over-provisioned when your license can be
* downgraded while still meeting the performance requirements of your workload.
*
*
* -
*
* InvalidCloudwatchApplicationInsights
— CloudWatch Application Insights isn't configured properly.
*
*
* -
*
* CloudwatchApplicationInsightsError
— There is a CloudWatch Application Insights error.
*
*
*
*/
private java.util.List findingReasonCodes;
/**
*
* An array of objects that describe the license recommendation options.
*
*/
private java.util.List licenseRecommendationOptions;
/**
*
* A list of tags assigned to an EC2 instance.
*
*/
private java.util.List tags;
/**
*
* The ARN that identifies the Amazon EC2 instance.
*
*
* @param resourceArn
* The ARN that identifies the Amazon EC2 instance.
*/
public void setResourceArn(String resourceArn) {
this.resourceArn = resourceArn;
}
/**
*
* The ARN that identifies the Amazon EC2 instance.
*
*
* @return The ARN that identifies the Amazon EC2 instance.
*/
public String getResourceArn() {
return this.resourceArn;
}
/**
*
* The ARN that identifies the Amazon EC2 instance.
*
*
* @param resourceArn
* The ARN that identifies the Amazon EC2 instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LicenseRecommendation withResourceArn(String resourceArn) {
setResourceArn(resourceArn);
return this;
}
/**
*
* The Amazon Web Services account ID of the license.
*
*
* @param accountId
* The Amazon Web Services account ID of the license.
*/
public void setAccountId(String accountId) {
this.accountId = accountId;
}
/**
*
* The Amazon Web Services account ID of the license.
*
*
* @return The Amazon Web Services account ID of the license.
*/
public String getAccountId() {
return this.accountId;
}
/**
*
* The Amazon Web Services account ID of the license.
*
*
* @param accountId
* The Amazon Web Services account ID of the license.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LicenseRecommendation withAccountId(String accountId) {
setAccountId(accountId);
return this;
}
/**
*
* An object that describes the current configuration of an instance that runs on a license.
*
*
* @param currentLicenseConfiguration
* An object that describes the current configuration of an instance that runs on a license.
*/
public void setCurrentLicenseConfiguration(LicenseConfiguration currentLicenseConfiguration) {
this.currentLicenseConfiguration = currentLicenseConfiguration;
}
/**
*
* An object that describes the current configuration of an instance that runs on a license.
*
*
* @return An object that describes the current configuration of an instance that runs on a license.
*/
public LicenseConfiguration getCurrentLicenseConfiguration() {
return this.currentLicenseConfiguration;
}
/**
*
* An object that describes the current configuration of an instance that runs on a license.
*
*
* @param currentLicenseConfiguration
* An object that describes the current configuration of an instance that runs on a license.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LicenseRecommendation withCurrentLicenseConfiguration(LicenseConfiguration currentLicenseConfiguration) {
setCurrentLicenseConfiguration(currentLicenseConfiguration);
return this;
}
/**
*
* The number of days for which utilization metrics were analyzed for an instance that runs on a license.
*
*
* @param lookbackPeriodInDays
* The number of days for which utilization metrics were analyzed for an instance that runs on a license.
*/
public void setLookbackPeriodInDays(Double lookbackPeriodInDays) {
this.lookbackPeriodInDays = lookbackPeriodInDays;
}
/**
*
* The number of days for which utilization metrics were analyzed for an instance that runs on a license.
*
*
* @return The number of days for which utilization metrics were analyzed for an instance that runs on a license.
*/
public Double getLookbackPeriodInDays() {
return this.lookbackPeriodInDays;
}
/**
*
* The number of days for which utilization metrics were analyzed for an instance that runs on a license.
*
*
* @param lookbackPeriodInDays
* The number of days for which utilization metrics were analyzed for an instance that runs on a license.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LicenseRecommendation withLookbackPeriodInDays(Double lookbackPeriodInDays) {
setLookbackPeriodInDays(lookbackPeriodInDays);
return this;
}
/**
*
* The timestamp of when the license recommendation was last generated.
*
*
* @param lastRefreshTimestamp
* The timestamp of when the license recommendation was last generated.
*/
public void setLastRefreshTimestamp(java.util.Date lastRefreshTimestamp) {
this.lastRefreshTimestamp = lastRefreshTimestamp;
}
/**
*
* The timestamp of when the license recommendation was last generated.
*
*
* @return The timestamp of when the license recommendation was last generated.
*/
public java.util.Date getLastRefreshTimestamp() {
return this.lastRefreshTimestamp;
}
/**
*
* The timestamp of when the license recommendation was last generated.
*
*
* @param lastRefreshTimestamp
* The timestamp of when the license recommendation was last generated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LicenseRecommendation withLastRefreshTimestamp(java.util.Date lastRefreshTimestamp) {
setLastRefreshTimestamp(lastRefreshTimestamp);
return this;
}
/**
*
* The finding classification for an instance that runs on a license.
*
*
* Findings include:
*
*
* -
*
* InsufficentMetrics
— When Compute Optimizer detects that your CloudWatch Application Insights isn't
* enabled or is enabled with insufficient permissions.
*
*
* -
*
* NotOptimized
— When Compute Optimizer detects that your EC2 infrastructure isn't using any of the
* SQL server license features you're paying for, a license is considered not optimized.
*
*
* -
*
* Optimized
— When Compute Optimizer detects that all specifications of your license meet the
* performance requirements of your workload.
*
*
*
*
* @param finding
* The finding classification for an instance that runs on a license.
*
* Findings include:
*
*
* -
*
* InsufficentMetrics
— When Compute Optimizer detects that your CloudWatch Application Insights
* isn't enabled or is enabled with insufficient permissions.
*
*
* -
*
* NotOptimized
— When Compute Optimizer detects that your EC2 infrastructure isn't using any of
* the SQL server license features you're paying for, a license is considered not optimized.
*
*
* -
*
* Optimized
— When Compute Optimizer detects that all specifications of your license meet the
* performance requirements of your workload.
*
*
* @see LicenseFinding
*/
public void setFinding(String finding) {
this.finding = finding;
}
/**
*
* The finding classification for an instance that runs on a license.
*
*
* Findings include:
*
*
* -
*
* InsufficentMetrics
— When Compute Optimizer detects that your CloudWatch Application Insights isn't
* enabled or is enabled with insufficient permissions.
*
*
* -
*
* NotOptimized
— When Compute Optimizer detects that your EC2 infrastructure isn't using any of the
* SQL server license features you're paying for, a license is considered not optimized.
*
*
* -
*
* Optimized
— When Compute Optimizer detects that all specifications of your license meet the
* performance requirements of your workload.
*
*
*
*
* @return The finding classification for an instance that runs on a license.
*
* Findings include:
*
*
* -
*
* InsufficentMetrics
— When Compute Optimizer detects that your CloudWatch Application
* Insights isn't enabled or is enabled with insufficient permissions.
*
*
* -
*
* NotOptimized
— When Compute Optimizer detects that your EC2 infrastructure isn't using any
* of the SQL server license features you're paying for, a license is considered not optimized.
*
*
* -
*
* Optimized
— When Compute Optimizer detects that all specifications of your license meet the
* performance requirements of your workload.
*
*
* @see LicenseFinding
*/
public String getFinding() {
return this.finding;
}
/**
*
* The finding classification for an instance that runs on a license.
*
*
* Findings include:
*
*
* -
*
* InsufficentMetrics
— When Compute Optimizer detects that your CloudWatch Application Insights isn't
* enabled or is enabled with insufficient permissions.
*
*
* -
*
* NotOptimized
— When Compute Optimizer detects that your EC2 infrastructure isn't using any of the
* SQL server license features you're paying for, a license is considered not optimized.
*
*
* -
*
* Optimized
— When Compute Optimizer detects that all specifications of your license meet the
* performance requirements of your workload.
*
*
*
*
* @param finding
* The finding classification for an instance that runs on a license.
*
* Findings include:
*
*
* -
*
* InsufficentMetrics
— When Compute Optimizer detects that your CloudWatch Application Insights
* isn't enabled or is enabled with insufficient permissions.
*
*
* -
*
* NotOptimized
— When Compute Optimizer detects that your EC2 infrastructure isn't using any of
* the SQL server license features you're paying for, a license is considered not optimized.
*
*
* -
*
* Optimized
— When Compute Optimizer detects that all specifications of your license meet the
* performance requirements of your workload.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see LicenseFinding
*/
public LicenseRecommendation withFinding(String finding) {
setFinding(finding);
return this;
}
/**
*
* The finding classification for an instance that runs on a license.
*
*
* Findings include:
*
*
* -
*
* InsufficentMetrics
— When Compute Optimizer detects that your CloudWatch Application Insights isn't
* enabled or is enabled with insufficient permissions.
*
*
* -
*
* NotOptimized
— When Compute Optimizer detects that your EC2 infrastructure isn't using any of the
* SQL server license features you're paying for, a license is considered not optimized.
*
*
* -
*
* Optimized
— When Compute Optimizer detects that all specifications of your license meet the
* performance requirements of your workload.
*
*
*
*
* @param finding
* The finding classification for an instance that runs on a license.
*
* Findings include:
*
*
* -
*
* InsufficentMetrics
— When Compute Optimizer detects that your CloudWatch Application Insights
* isn't enabled or is enabled with insufficient permissions.
*
*
* -
*
* NotOptimized
— When Compute Optimizer detects that your EC2 infrastructure isn't using any of
* the SQL server license features you're paying for, a license is considered not optimized.
*
*
* -
*
* Optimized
— When Compute Optimizer detects that all specifications of your license meet the
* performance requirements of your workload.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see LicenseFinding
*/
public LicenseRecommendation withFinding(LicenseFinding finding) {
this.finding = finding.toString();
return this;
}
/**
*
* The reason for the finding classification for an instance that runs on a license.
*
*
* Finding reason codes include:
*
*
* -
*
* Optimized
— All specifications of your license meet the performance requirements of your workload.
*
*
* -
*
* LicenseOverprovisioned
— A license is considered over-provisioned when your license can be
* downgraded while still meeting the performance requirements of your workload.
*
*
* -
*
* InvalidCloudwatchApplicationInsights
— CloudWatch Application Insights isn't configured properly.
*
*
* -
*
* CloudwatchApplicationInsightsError
— There is a CloudWatch Application Insights error.
*
*
*
*
* @return The reason for the finding classification for an instance that runs on a license.
*
* Finding reason codes include:
*
*
* -
*
* Optimized
— All specifications of your license meet the performance requirements of your
* workload.
*
*
* -
*
* LicenseOverprovisioned
— A license is considered over-provisioned when your license can be
* downgraded while still meeting the performance requirements of your workload.
*
*
* -
*
* InvalidCloudwatchApplicationInsights
— CloudWatch Application Insights isn't configured
* properly.
*
*
* -
*
* CloudwatchApplicationInsightsError
— There is a CloudWatch Application Insights error.
*
*
* @see LicenseFindingReasonCode
*/
public java.util.List getFindingReasonCodes() {
return findingReasonCodes;
}
/**
*
* The reason for the finding classification for an instance that runs on a license.
*
*
* Finding reason codes include:
*
*
* -
*
* Optimized
— All specifications of your license meet the performance requirements of your workload.
*
*
* -
*
* LicenseOverprovisioned
— A license is considered over-provisioned when your license can be
* downgraded while still meeting the performance requirements of your workload.
*
*
* -
*
* InvalidCloudwatchApplicationInsights
— CloudWatch Application Insights isn't configured properly.
*
*
* -
*
* CloudwatchApplicationInsightsError
— There is a CloudWatch Application Insights error.
*
*
*
*
* @param findingReasonCodes
* The reason for the finding classification for an instance that runs on a license.
*
* Finding reason codes include:
*
*
* -
*
* Optimized
— All specifications of your license meet the performance requirements of your
* workload.
*
*
* -
*
* LicenseOverprovisioned
— A license is considered over-provisioned when your license can be
* downgraded while still meeting the performance requirements of your workload.
*
*
* -
*
* InvalidCloudwatchApplicationInsights
— CloudWatch Application Insights isn't configured
* properly.
*
*
* -
*
* CloudwatchApplicationInsightsError
— There is a CloudWatch Application Insights error.
*
*
* @see LicenseFindingReasonCode
*/
public void setFindingReasonCodes(java.util.Collection findingReasonCodes) {
if (findingReasonCodes == null) {
this.findingReasonCodes = null;
return;
}
this.findingReasonCodes = new java.util.ArrayList(findingReasonCodes);
}
/**
*
* The reason for the finding classification for an instance that runs on a license.
*
*
* Finding reason codes include:
*
*
* -
*
* Optimized
— All specifications of your license meet the performance requirements of your workload.
*
*
* -
*
* LicenseOverprovisioned
— A license is considered over-provisioned when your license can be
* downgraded while still meeting the performance requirements of your workload.
*
*
* -
*
* InvalidCloudwatchApplicationInsights
— CloudWatch Application Insights isn't configured properly.
*
*
* -
*
* CloudwatchApplicationInsightsError
— There is a CloudWatch Application Insights error.
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFindingReasonCodes(java.util.Collection)} or {@link #withFindingReasonCodes(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param findingReasonCodes
* The reason for the finding classification for an instance that runs on a license.
*
* Finding reason codes include:
*
*
* -
*
* Optimized
— All specifications of your license meet the performance requirements of your
* workload.
*
*
* -
*
* LicenseOverprovisioned
— A license is considered over-provisioned when your license can be
* downgraded while still meeting the performance requirements of your workload.
*
*
* -
*
* InvalidCloudwatchApplicationInsights
— CloudWatch Application Insights isn't configured
* properly.
*
*
* -
*
* CloudwatchApplicationInsightsError
— There is a CloudWatch Application Insights error.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see LicenseFindingReasonCode
*/
public LicenseRecommendation withFindingReasonCodes(String... findingReasonCodes) {
if (this.findingReasonCodes == null) {
setFindingReasonCodes(new java.util.ArrayList(findingReasonCodes.length));
}
for (String ele : findingReasonCodes) {
this.findingReasonCodes.add(ele);
}
return this;
}
/**
*
* The reason for the finding classification for an instance that runs on a license.
*
*
* Finding reason codes include:
*
*
* -
*
* Optimized
— All specifications of your license meet the performance requirements of your workload.
*
*
* -
*
* LicenseOverprovisioned
— A license is considered over-provisioned when your license can be
* downgraded while still meeting the performance requirements of your workload.
*
*
* -
*
* InvalidCloudwatchApplicationInsights
— CloudWatch Application Insights isn't configured properly.
*
*
* -
*
* CloudwatchApplicationInsightsError
— There is a CloudWatch Application Insights error.
*
*
*
*
* @param findingReasonCodes
* The reason for the finding classification for an instance that runs on a license.
*
* Finding reason codes include:
*
*
* -
*
* Optimized
— All specifications of your license meet the performance requirements of your
* workload.
*
*
* -
*
* LicenseOverprovisioned
— A license is considered over-provisioned when your license can be
* downgraded while still meeting the performance requirements of your workload.
*
*
* -
*
* InvalidCloudwatchApplicationInsights
— CloudWatch Application Insights isn't configured
* properly.
*
*
* -
*
* CloudwatchApplicationInsightsError
— There is a CloudWatch Application Insights error.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see LicenseFindingReasonCode
*/
public LicenseRecommendation withFindingReasonCodes(java.util.Collection findingReasonCodes) {
setFindingReasonCodes(findingReasonCodes);
return this;
}
/**
*
* The reason for the finding classification for an instance that runs on a license.
*
*
* Finding reason codes include:
*
*
* -
*
* Optimized
— All specifications of your license meet the performance requirements of your workload.
*
*
* -
*
* LicenseOverprovisioned
— A license is considered over-provisioned when your license can be
* downgraded while still meeting the performance requirements of your workload.
*
*
* -
*
* InvalidCloudwatchApplicationInsights
— CloudWatch Application Insights isn't configured properly.
*
*
* -
*
* CloudwatchApplicationInsightsError
— There is a CloudWatch Application Insights error.
*
*
*
*
* @param findingReasonCodes
* The reason for the finding classification for an instance that runs on a license.
*
* Finding reason codes include:
*
*
* -
*
* Optimized
— All specifications of your license meet the performance requirements of your
* workload.
*
*
* -
*
* LicenseOverprovisioned
— A license is considered over-provisioned when your license can be
* downgraded while still meeting the performance requirements of your workload.
*
*
* -
*
* InvalidCloudwatchApplicationInsights
— CloudWatch Application Insights isn't configured
* properly.
*
*
* -
*
* CloudwatchApplicationInsightsError
— There is a CloudWatch Application Insights error.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see LicenseFindingReasonCode
*/
public LicenseRecommendation withFindingReasonCodes(LicenseFindingReasonCode... findingReasonCodes) {
java.util.ArrayList findingReasonCodesCopy = new java.util.ArrayList(findingReasonCodes.length);
for (LicenseFindingReasonCode value : findingReasonCodes) {
findingReasonCodesCopy.add(value.toString());
}
if (getFindingReasonCodes() == null) {
setFindingReasonCodes(findingReasonCodesCopy);
} else {
getFindingReasonCodes().addAll(findingReasonCodesCopy);
}
return this;
}
/**
*
* An array of objects that describe the license recommendation options.
*
*
* @return An array of objects that describe the license recommendation options.
*/
public java.util.List getLicenseRecommendationOptions() {
return licenseRecommendationOptions;
}
/**
*
* An array of objects that describe the license recommendation options.
*
*
* @param licenseRecommendationOptions
* An array of objects that describe the license recommendation options.
*/
public void setLicenseRecommendationOptions(java.util.Collection licenseRecommendationOptions) {
if (licenseRecommendationOptions == null) {
this.licenseRecommendationOptions = null;
return;
}
this.licenseRecommendationOptions = new java.util.ArrayList(licenseRecommendationOptions);
}
/**
*
* An array of objects that describe the license recommendation options.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setLicenseRecommendationOptions(java.util.Collection)} or
* {@link #withLicenseRecommendationOptions(java.util.Collection)} if you want to override the existing values.
*
*
* @param licenseRecommendationOptions
* An array of objects that describe the license recommendation options.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LicenseRecommendation withLicenseRecommendationOptions(LicenseRecommendationOption... licenseRecommendationOptions) {
if (this.licenseRecommendationOptions == null) {
setLicenseRecommendationOptions(new java.util.ArrayList(licenseRecommendationOptions.length));
}
for (LicenseRecommendationOption ele : licenseRecommendationOptions) {
this.licenseRecommendationOptions.add(ele);
}
return this;
}
/**
*
* An array of objects that describe the license recommendation options.
*
*
* @param licenseRecommendationOptions
* An array of objects that describe the license recommendation options.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LicenseRecommendation withLicenseRecommendationOptions(java.util.Collection licenseRecommendationOptions) {
setLicenseRecommendationOptions(licenseRecommendationOptions);
return this;
}
/**
*
* A list of tags assigned to an EC2 instance.
*
*
* @return A list of tags assigned to an EC2 instance.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* A list of tags assigned to an EC2 instance.
*
*
* @param tags
* A list of tags assigned to an EC2 instance.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* A list of tags assigned to an EC2 instance.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* A list of tags assigned to an EC2 instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LicenseRecommendation withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* A list of tags assigned to an EC2 instance.
*
*
* @param tags
* A list of tags assigned to an EC2 instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LicenseRecommendation withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getResourceArn() != null)
sb.append("ResourceArn: ").append(getResourceArn()).append(",");
if (getAccountId() != null)
sb.append("AccountId: ").append(getAccountId()).append(",");
if (getCurrentLicenseConfiguration() != null)
sb.append("CurrentLicenseConfiguration: ").append(getCurrentLicenseConfiguration()).append(",");
if (getLookbackPeriodInDays() != null)
sb.append("LookbackPeriodInDays: ").append(getLookbackPeriodInDays()).append(",");
if (getLastRefreshTimestamp() != null)
sb.append("LastRefreshTimestamp: ").append(getLastRefreshTimestamp()).append(",");
if (getFinding() != null)
sb.append("Finding: ").append(getFinding()).append(",");
if (getFindingReasonCodes() != null)
sb.append("FindingReasonCodes: ").append(getFindingReasonCodes()).append(",");
if (getLicenseRecommendationOptions() != null)
sb.append("LicenseRecommendationOptions: ").append(getLicenseRecommendationOptions()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof LicenseRecommendation == false)
return false;
LicenseRecommendation other = (LicenseRecommendation) obj;
if (other.getResourceArn() == null ^ this.getResourceArn() == null)
return false;
if (other.getResourceArn() != null && other.getResourceArn().equals(this.getResourceArn()) == false)
return false;
if (other.getAccountId() == null ^ this.getAccountId() == null)
return false;
if (other.getAccountId() != null && other.getAccountId().equals(this.getAccountId()) == false)
return false;
if (other.getCurrentLicenseConfiguration() == null ^ this.getCurrentLicenseConfiguration() == null)
return false;
if (other.getCurrentLicenseConfiguration() != null && other.getCurrentLicenseConfiguration().equals(this.getCurrentLicenseConfiguration()) == false)
return false;
if (other.getLookbackPeriodInDays() == null ^ this.getLookbackPeriodInDays() == null)
return false;
if (other.getLookbackPeriodInDays() != null && other.getLookbackPeriodInDays().equals(this.getLookbackPeriodInDays()) == false)
return false;
if (other.getLastRefreshTimestamp() == null ^ this.getLastRefreshTimestamp() == null)
return false;
if (other.getLastRefreshTimestamp() != null && other.getLastRefreshTimestamp().equals(this.getLastRefreshTimestamp()) == false)
return false;
if (other.getFinding() == null ^ this.getFinding() == null)
return false;
if (other.getFinding() != null && other.getFinding().equals(this.getFinding()) == false)
return false;
if (other.getFindingReasonCodes() == null ^ this.getFindingReasonCodes() == null)
return false;
if (other.getFindingReasonCodes() != null && other.getFindingReasonCodes().equals(this.getFindingReasonCodes()) == false)
return false;
if (other.getLicenseRecommendationOptions() == null ^ this.getLicenseRecommendationOptions() == null)
return false;
if (other.getLicenseRecommendationOptions() != null && other.getLicenseRecommendationOptions().equals(this.getLicenseRecommendationOptions()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getResourceArn() == null) ? 0 : getResourceArn().hashCode());
hashCode = prime * hashCode + ((getAccountId() == null) ? 0 : getAccountId().hashCode());
hashCode = prime * hashCode + ((getCurrentLicenseConfiguration() == null) ? 0 : getCurrentLicenseConfiguration().hashCode());
hashCode = prime * hashCode + ((getLookbackPeriodInDays() == null) ? 0 : getLookbackPeriodInDays().hashCode());
hashCode = prime * hashCode + ((getLastRefreshTimestamp() == null) ? 0 : getLastRefreshTimestamp().hashCode());
hashCode = prime * hashCode + ((getFinding() == null) ? 0 : getFinding().hashCode());
hashCode = prime * hashCode + ((getFindingReasonCodes() == null) ? 0 : getFindingReasonCodes().hashCode());
hashCode = prime * hashCode + ((getLicenseRecommendationOptions() == null) ? 0 : getLicenseRecommendationOptions().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public LicenseRecommendation clone() {
try {
return (LicenseRecommendation) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.computeoptimizer.model.transform.LicenseRecommendationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}