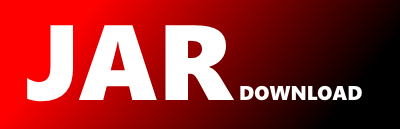
com.amazonaws.services.connectcases.AmazonConnectCases Maven / Gradle / Ivy
Show all versions of aws-java-sdk-connectcases Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.connectcases;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.connectcases.model.*;
/**
* Interface for accessing ConnectCases.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.connectcases.AbstractAmazonConnectCases} instead.
*
*
*
* With Amazon Connect Cases, your agents can track and manage customer issues that require multiple interactions,
* follow-up tasks, and teams in your contact center. A case represents a customer issue. It records the issue, the
* steps and interactions taken to resolve the issue, and the outcome. For more information, see Amazon Connect Cases in the Amazon
* Connect Administrator Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonConnectCases {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "cases";
/**
*
* Returns the description for the list of fields in the request parameters.
*
*
* @param batchGetFieldRequest
* @return Result of the BatchGetField operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonConnectCases.BatchGetField
* @see AWS API
* Documentation
*/
BatchGetFieldResult batchGetField(BatchGetFieldRequest batchGetFieldRequest);
/**
*
* Creates and updates a set of field options for a single select field in a Cases domain.
*
*
* @param batchPutFieldOptionsRequest
* @return Result of the BatchPutFieldOptions operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded. For a list of service quotas, see Amazon
* Connect Service Quotas in the Amazon Connect Administrator Guide.
* @sample AmazonConnectCases.BatchPutFieldOptions
* @see AWS API Documentation
*/
BatchPutFieldOptionsResult batchPutFieldOptions(BatchPutFieldOptionsRequest batchPutFieldOptionsRequest);
/**
*
*
* If you provide a value for PerformedBy.UserArn
you must also have connect:DescribeUser
* permission on the User ARN resource that you provide
*
*
*
*
* <p>Creates a case in the specified Cases domain. Case system and custom fields are taken as an array id/value pairs with a declared data types.</p> <p>The following fields are required when creating a case:</p> <ul> <li> <p> <code>customer_id</code> - You must provide the full customer profile ARN in this format: <code>arn:aws:profile:your_AWS_Region:your_AWS_account ID:domains/your_profiles_domain_name/profiles/profile_ID</code> </p> </li> <li> <p> <code>title</code> </p> </li> </ul>
*
*
* @param createCaseRequest
* @return Result of the CreateCase operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The requested operation would cause a conflict with the current state of a service resource associated
* with the request. Resolve the conflict before retrying this request. See the accompanying error message
* for details.
* @sample AmazonConnectCases.CreateCase
* @see AWS API
* Documentation
*/
CreateCaseResult createCase(CreateCaseRequest createCaseRequest);
/**
*
* Creates a domain, which is a container for all case data, such as cases, fields, templates and layouts. Each
* Amazon Connect instance can be associated with only one Cases domain.
*
*
*
* This will not associate your connect instance to Cases domain. Instead, use the Amazon Connect CreateIntegrationAssociation API. You need specific IAM permissions to successfully associate the Cases
* domain. For more information, see Onboard to Cases.
*
*
*
* </important>
*
*
* @param createDomainRequest
* @return Result of the CreateDomain operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The requested operation would cause a conflict with the current state of a service resource associated
* with the request. Resolve the conflict before retrying this request. See the accompanying error message
* for details.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded. For a list of service quotas, see Amazon
* Connect Service Quotas in the Amazon Connect Administrator Guide.
* @sample AmazonConnectCases.CreateDomain
* @see AWS API
* Documentation
*/
CreateDomainResult createDomain(CreateDomainRequest createDomainRequest);
/**
*
* Creates a field in the Cases domain. This field is used to define the case object model (that is, defines what
* data can be captured on cases) in a Cases domain.
*
*
* @param createFieldRequest
* @return Result of the CreateField operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The requested operation would cause a conflict with the current state of a service resource associated
* with the request. Resolve the conflict before retrying this request. See the accompanying error message
* for details.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded. For a list of service quotas, see Amazon
* Connect Service Quotas in the Amazon Connect Administrator Guide.
* @sample AmazonConnectCases.CreateField
* @see AWS API
* Documentation
*/
CreateFieldResult createField(CreateFieldRequest createFieldRequest);
/**
*
* Creates a layout in the Cases domain. Layouts define the following configuration in the top section and More Info
* tab of the Cases user interface:
*
*
* -
*
* Fields to display to the users
*
*
* -
*
* Field ordering
*
*
*
*
*
* Title and Status fields cannot be part of layouts since they are not configurable.
*
*
*
* @param createLayoutRequest
* @return Result of the CreateLayout operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The requested operation would cause a conflict with the current state of a service resource associated
* with the request. Resolve the conflict before retrying this request. See the accompanying error message
* for details.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded. For a list of service quotas, see Amazon
* Connect Service Quotas in the Amazon Connect Administrator Guide.
* @sample AmazonConnectCases.CreateLayout
* @see AWS API
* Documentation
*/
CreateLayoutResult createLayout(CreateLayoutRequest createLayoutRequest);
/**
*
* Creates a related item (comments, tasks, and contacts) and associates it with a case.
*
*
*
* -
*
* A Related Item is a resource that is associated with a case. It may or may not have an external identifier
* linking it to an external resource (for example, a contactArn
). All Related Items have their own
* internal identifier, the relatedItemArn
. Examples of related items include comments
and
* contacts
.
*
*
* -
*
* If you provide a value for performedBy.userArn
you must also have DescribeUser permission
* on the ARN of the user that you provide.
*
*
*
*
*
* </note>
*
*
* @param createRelatedItemRequest
* @return Result of the CreateRelatedItem operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded. For a list of service quotas, see Amazon
* Connect Service Quotas in the Amazon Connect Administrator Guide.
* @sample AmazonConnectCases.CreateRelatedItem
* @see AWS
* API Documentation
*/
CreateRelatedItemResult createRelatedItem(CreateRelatedItemRequest createRelatedItemRequest);
/**
*
* Creates a template in the Cases domain. This template is used to define the case object model (that is, to define
* what data can be captured on cases) in a Cases domain. A template must have a unique name within a domain, and it
* must reference existing field IDs and layout IDs. Additionally, multiple fields with same IDs are not allowed
* within the same Template. A template can be either Active or Inactive, as indicated by its status. Inactive
* templates cannot be used to create cases.
*
*
* @param createTemplateRequest
* @return Result of the CreateTemplate operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The requested operation would cause a conflict with the current state of a service resource associated
* with the request. Resolve the conflict before retrying this request. See the accompanying error message
* for details.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded. For a list of service quotas, see Amazon
* Connect Service Quotas in the Amazon Connect Administrator Guide.
* @sample AmazonConnectCases.CreateTemplate
* @see AWS
* API Documentation
*/
CreateTemplateResult createTemplate(CreateTemplateRequest createTemplateRequest);
/**
*
* Deletes a Cases domain.
*
*
*
* <note> <p>After deleting your domain you must disassociate the deleted domain from your Amazon Connect instance with another API call before being able to use Cases again with this Amazon Connect instance. See <a href="https://docs.aws.amazon.com/connect/latest/APIReference/API_DeleteIntegrationAssociation.html">DeleteIntegrationAssociation</a>.</p> </note>
*
*
* @param deleteDomainRequest
* @return Result of the DeleteDomain operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The requested operation would cause a conflict with the current state of a service resource associated
* with the request. Resolve the conflict before retrying this request. See the accompanying error message
* for details.
* @sample AmazonConnectCases.DeleteDomain
* @see AWS API
* Documentation
*/
DeleteDomainResult deleteDomain(DeleteDomainRequest deleteDomainRequest);
/**
*
* Deletes a field from a cases template. You can delete up to 100 fields per domain.
*
*
* After a field is deleted:
*
*
* -
*
* You can still retrieve the field by calling BatchGetField
.
*
*
* -
*
* You cannot update a deleted field by calling UpdateField
; it throws a
* ValidationException
.
*
*
* -
*
* Deleted fields are not included in the ListFields
response.
*
*
* -
*
* Calling CreateCase
with a deleted field throws a ValidationException
denoting which
* field IDs in the request have been deleted.
*
*
* -
*
* Calling GetCase
with a deleted field ID returns the deleted field's value if one exists.
*
*
* -
*
* Calling UpdateCase
with a deleted field ID throws a ValidationException
if the case
* does not already contain a value for the deleted field. Otherwise it succeeds, allowing you to update or remove
* (using emptyValue: {}
) the field's value from the case.
*
*
* -
*
* GetTemplate
does not return field IDs for deleted fields.
*
*
* -
*
* GetLayout
does not return field IDs for deleted fields.
*
*
* -
*
* Calling SearchCases
with the deleted field ID as a filter returns any cases that have a value for
* the deleted field that matches the filter criteria.
*
*
* -
*
* Calling SearchCases
with a searchTerm
value that matches a deleted field's value on a
* case returns the case in the response.
*
*
* -
*
* Calling BatchPutFieldOptions
with a deleted field ID throw a ValidationException
.
*
*
* -
*
* Calling GetCaseEventConfiguration
does not return field IDs for deleted fields.
*
*
*
*
* @param deleteFieldRequest
* @return Result of the DeleteField operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The requested operation would cause a conflict with the current state of a service resource associated
* with the request. Resolve the conflict before retrying this request. See the accompanying error message
* for details.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded. For a list of service quotas, see Amazon
* Connect Service Quotas in the Amazon Connect Administrator Guide.
* @sample AmazonConnectCases.DeleteField
* @see AWS API
* Documentation
*/
DeleteFieldResult deleteField(DeleteFieldRequest deleteFieldRequest);
/**
*
* Deletes a layout from a cases template. You can delete up to 100 layouts per domain.
*
*
*
* <p>After a layout is deleted:</p> <ul> <li> <p>You can still retrieve the layout by calling <code>GetLayout</code>.</p> </li> <li> <p>You cannot update a deleted layout by calling <code>UpdateLayout</code>; it throws a <code>ValidationException</code>.</p> </li> <li> <p>Deleted layouts are not included in the <code>ListLayouts</code> response.</p> </li> </ul>
*
*
* @param deleteLayoutRequest
* @return Result of the DeleteLayout operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The requested operation would cause a conflict with the current state of a service resource associated
* with the request. Resolve the conflict before retrying this request. See the accompanying error message
* for details.
* @sample AmazonConnectCases.DeleteLayout
* @see AWS API
* Documentation
*/
DeleteLayoutResult deleteLayout(DeleteLayoutRequest deleteLayoutRequest);
/**
*
* Deletes a cases template. You can delete up to 100 templates per domain.
*
*
*
* <p>After a cases template is deleted:</p> <ul> <li> <p>You can still retrieve the template by calling <code>GetTemplate</code>.</p> </li> <li> <p>You cannot update the template. </p> </li> <li> <p>You cannot create a case by using the deleted template.</p> </li> <li> <p>Deleted templates are not included in the <code>ListTemplates</code> response.</p> </li> </ul>
*
*
* @param deleteTemplateRequest
* @return Result of the DeleteTemplate operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The requested operation would cause a conflict with the current state of a service resource associated
* with the request. Resolve the conflict before retrying this request. See the accompanying error message
* for details.
* @sample AmazonConnectCases.DeleteTemplate
* @see AWS
* API Documentation
*/
DeleteTemplateResult deleteTemplate(DeleteTemplateRequest deleteTemplateRequest);
/**
*
* Returns information about a specific case if it exists.
*
*
* @param getCaseRequest
* @return Result of the GetCase operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonConnectCases.GetCase
* @see AWS API
* Documentation
*/
GetCaseResult getCase(GetCaseRequest getCaseRequest);
/**
*
* Returns the audit history about a specific case if it exists.
*
*
* @param getCaseAuditEventsRequest
* @return Result of the GetCaseAuditEvents operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonConnectCases.GetCaseAuditEvents
* @see AWS API Documentation
*/
GetCaseAuditEventsResult getCaseAuditEvents(GetCaseAuditEventsRequest getCaseAuditEventsRequest);
/**
*
* Returns the case event publishing configuration.
*
*
* @param getCaseEventConfigurationRequest
* @return Result of the GetCaseEventConfiguration operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonConnectCases.GetCaseEventConfiguration
* @see AWS API Documentation
*/
GetCaseEventConfigurationResult getCaseEventConfiguration(GetCaseEventConfigurationRequest getCaseEventConfigurationRequest);
/**
*
* Returns information about a specific domain if it exists.
*
*
* @param getDomainRequest
* @return Result of the GetDomain operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonConnectCases.GetDomain
* @see AWS API
* Documentation
*/
GetDomainResult getDomain(GetDomainRequest getDomainRequest);
/**
*
* Returns the details for the requested layout.
*
*
* @param getLayoutRequest
* @return Result of the GetLayout operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonConnectCases.GetLayout
* @see AWS API
* Documentation
*/
GetLayoutResult getLayout(GetLayoutRequest getLayoutRequest);
/**
*
* Returns the details for the requested template.
*
*
* @param getTemplateRequest
* @return Result of the GetTemplate operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonConnectCases.GetTemplate
* @see AWS API
* Documentation
*/
GetTemplateResult getTemplate(GetTemplateRequest getTemplateRequest);
/**
*
* Lists cases for a given contact.
*
*
* @param listCasesForContactRequest
* @return Result of the ListCasesForContact operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonConnectCases.ListCasesForContact
* @see AWS API Documentation
*/
ListCasesForContactResult listCasesForContact(ListCasesForContactRequest listCasesForContactRequest);
/**
*
* Lists all cases domains in the Amazon Web Services account. Each list item is a condensed summary object of the
* domain.
*
*
* @param listDomainsRequest
* @return Result of the ListDomains operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonConnectCases.ListDomains
* @see AWS API
* Documentation
*/
ListDomainsResult listDomains(ListDomainsRequest listDomainsRequest);
/**
*
* Lists all of the field options for a field identifier in the domain.
*
*
* @param listFieldOptionsRequest
* @return Result of the ListFieldOptions operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonConnectCases.ListFieldOptions
* @see AWS
* API Documentation
*/
ListFieldOptionsResult listFieldOptions(ListFieldOptionsRequest listFieldOptionsRequest);
/**
*
* Lists all fields in a Cases domain.
*
*
* @param listFieldsRequest
* @return Result of the ListFields operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonConnectCases.ListFields
* @see AWS API
* Documentation
*/
ListFieldsResult listFields(ListFieldsRequest listFieldsRequest);
/**
*
* Lists all layouts in the given cases domain. Each list item is a condensed summary object of the layout.
*
*
* @param listLayoutsRequest
* @return Result of the ListLayouts operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonConnectCases.ListLayouts
* @see AWS API
* Documentation
*/
ListLayoutsResult listLayouts(ListLayoutsRequest listLayoutsRequest);
/**
*
* Lists tags for a resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonConnectCases.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists all of the templates in a Cases domain. Each list item is a condensed summary object of the template.
*
*
* @param listTemplatesRequest
* @return Result of the ListTemplates operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonConnectCases.ListTemplates
* @see AWS API
* Documentation
*/
ListTemplatesResult listTemplates(ListTemplatesRequest listTemplatesRequest);
/**
*
* Adds case event publishing configuration. For a complete list of fields you can add to the event message, see Create case fields in the
* Amazon Connect Administrator Guide
*
*
* @param putCaseEventConfigurationRequest
* @return Result of the PutCaseEventConfiguration operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonConnectCases.PutCaseEventConfiguration
* @see AWS API Documentation
*/
PutCaseEventConfigurationResult putCaseEventConfiguration(PutCaseEventConfigurationRequest putCaseEventConfigurationRequest);
/**
*
* Searches for cases within their associated Cases domain. Search results are returned as a paginated list of
* abridged case documents.
*
*
*
* For customer_id
you must provide the full customer profile ARN in this format:
* arn:aws:profile:your AWS Region:your AWS account ID:domains/profiles domain name/profiles/profile ID
* .
*
*
*
* @param searchCasesRequest
* @return Result of the SearchCases operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonConnectCases.SearchCases
* @see AWS API
* Documentation
*/
SearchCasesResult searchCases(SearchCasesRequest searchCasesRequest);
/**
*
* Searches for related items that are associated with a case.
*
*
*
* If no filters are provided, this returns all related items associated with a case.
*
*
*
* @param searchRelatedItemsRequest
* @return Result of the SearchRelatedItems operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonConnectCases.SearchRelatedItems
* @see AWS API Documentation
*/
SearchRelatedItemsResult searchRelatedItems(SearchRelatedItemsRequest searchRelatedItemsRequest);
/**
*
* Adds tags to a resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonConnectCases.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Untags a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonConnectCases.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
*
* If you provide a value for PerformedBy.UserArn
you must also have connect:DescribeUser
* permission on the User ARN resource that you provide
*
*
*
*
* <p>Updates the values of fields on a case. Fields to be updated are received as an array of id/value pairs identical to the <code>CreateCase</code> input .</p> <p>If the action is successful, the service sends back an HTTP 200 response with an empty HTTP body.</p>
*
*
* @param updateCaseRequest
* @return Result of the UpdateCase operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonConnectCases.UpdateCase
* @see AWS API
* Documentation
*/
UpdateCaseResult updateCase(UpdateCaseRequest updateCaseRequest);
/**
*
* Updates the properties of an existing field.
*
*
* @param updateFieldRequest
* @return Result of the UpdateField operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The requested operation would cause a conflict with the current state of a service resource associated
* with the request. Resolve the conflict before retrying this request. See the accompanying error message
* for details.
* @sample AmazonConnectCases.UpdateField
* @see AWS API
* Documentation
*/
UpdateFieldResult updateField(UpdateFieldRequest updateFieldRequest);
/**
*
* Updates the attributes of an existing layout.
*
*
* If the action is successful, the service sends back an HTTP 200 response with an empty HTTP body.
*
*
* A ValidationException
is returned when you add non-existent fieldIds
to a layout.
*
*
*
* Title and Status fields cannot be part of layouts because they are not configurable.
*
*
*
* @param updateLayoutRequest
* @return Result of the UpdateLayout operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The requested operation would cause a conflict with the current state of a service resource associated
* with the request. Resolve the conflict before retrying this request. See the accompanying error message
* for details.
* @throws ServiceQuotaExceededException
* The service quota has been exceeded. For a list of service quotas, see Amazon
* Connect Service Quotas in the Amazon Connect Administrator Guide.
* @sample AmazonConnectCases.UpdateLayout
* @see AWS API
* Documentation
*/
UpdateLayoutResult updateLayout(UpdateLayoutRequest updateLayoutRequest);
/**
*
* Updates the attributes of an existing template. The template attributes that can be modified include
* name
, description
, layoutConfiguration
, requiredFields
, and
* status
. At least one of these attributes must not be null. If a null value is provided for a given
* attribute, that attribute is ignored and its current value is preserved.
*
*
* @param updateTemplateRequest
* @return Result of the UpdateTemplate operation returned by the service.
* @throws InternalServerException
* We couldn't process your request because of an issue with the server. Try again later.
* @throws ResourceNotFoundException
* We couldn't find the requested resource. Check that your resources exists and were created in the same
* Amazon Web Services Region as your request, and try your request again.
* @throws ValidationException
* The request isn't valid. Check the syntax and try again.
* @throws ThrottlingException
* The rate has been exceeded for this API. Please try again after a few minutes.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The requested operation would cause a conflict with the current state of a service resource associated
* with the request. Resolve the conflict before retrying this request. See the accompanying error message
* for details.
* @sample AmazonConnectCases.UpdateTemplate
* @see AWS
* API Documentation
*/
UpdateTemplateResult updateTemplate(UpdateTemplateRequest updateTemplateRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}