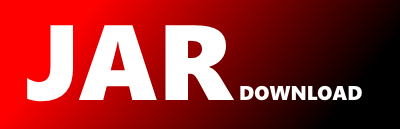
com.amazonaws.services.connectcontactlens.model.RealtimeContactAnalysisSegment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-java-sdk-connectcontactlens Show documentation
Show all versions of aws-java-sdk-connectcontactlens Show documentation
The AWS Java SDK for Amazon Connect Contact Lens module holds the client classes that are used for communicating with Amazon Connect Contact Lens Service
The newest version!
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.connectcontactlens.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* An analyzed segment for a real-time analysis session.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class RealtimeContactAnalysisSegment implements Serializable, Cloneable, StructuredPojo {
/**
*
* The analyzed transcript.
*
*/
private Transcript transcript;
/**
*
* The matched category rules.
*
*/
private Categories categories;
/**
*
* Information about the post-contact summary.
*
*/
private PostContactSummary postContactSummary;
/**
*
* The analyzed transcript.
*
*
* @param transcript
* The analyzed transcript.
*/
public void setTranscript(Transcript transcript) {
this.transcript = transcript;
}
/**
*
* The analyzed transcript.
*
*
* @return The analyzed transcript.
*/
public Transcript getTranscript() {
return this.transcript;
}
/**
*
* The analyzed transcript.
*
*
* @param transcript
* The analyzed transcript.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RealtimeContactAnalysisSegment withTranscript(Transcript transcript) {
setTranscript(transcript);
return this;
}
/**
*
* The matched category rules.
*
*
* @param categories
* The matched category rules.
*/
public void setCategories(Categories categories) {
this.categories = categories;
}
/**
*
* The matched category rules.
*
*
* @return The matched category rules.
*/
public Categories getCategories() {
return this.categories;
}
/**
*
* The matched category rules.
*
*
* @param categories
* The matched category rules.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RealtimeContactAnalysisSegment withCategories(Categories categories) {
setCategories(categories);
return this;
}
/**
*
* Information about the post-contact summary.
*
*
* @param postContactSummary
* Information about the post-contact summary.
*/
public void setPostContactSummary(PostContactSummary postContactSummary) {
this.postContactSummary = postContactSummary;
}
/**
*
* Information about the post-contact summary.
*
*
* @return Information about the post-contact summary.
*/
public PostContactSummary getPostContactSummary() {
return this.postContactSummary;
}
/**
*
* Information about the post-contact summary.
*
*
* @param postContactSummary
* Information about the post-contact summary.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RealtimeContactAnalysisSegment withPostContactSummary(PostContactSummary postContactSummary) {
setPostContactSummary(postContactSummary);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTranscript() != null)
sb.append("Transcript: ").append(getTranscript()).append(",");
if (getCategories() != null)
sb.append("Categories: ").append(getCategories()).append(",");
if (getPostContactSummary() != null)
sb.append("PostContactSummary: ").append(getPostContactSummary());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof RealtimeContactAnalysisSegment == false)
return false;
RealtimeContactAnalysisSegment other = (RealtimeContactAnalysisSegment) obj;
if (other.getTranscript() == null ^ this.getTranscript() == null)
return false;
if (other.getTranscript() != null && other.getTranscript().equals(this.getTranscript()) == false)
return false;
if (other.getCategories() == null ^ this.getCategories() == null)
return false;
if (other.getCategories() != null && other.getCategories().equals(this.getCategories()) == false)
return false;
if (other.getPostContactSummary() == null ^ this.getPostContactSummary() == null)
return false;
if (other.getPostContactSummary() != null && other.getPostContactSummary().equals(this.getPostContactSummary()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTranscript() == null) ? 0 : getTranscript().hashCode());
hashCode = prime * hashCode + ((getCategories() == null) ? 0 : getCategories().hashCode());
hashCode = prime * hashCode + ((getPostContactSummary() == null) ? 0 : getPostContactSummary().hashCode());
return hashCode;
}
@Override
public RealtimeContactAnalysisSegment clone() {
try {
return (RealtimeContactAnalysisSegment) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.connectcontactlens.model.transform.RealtimeContactAnalysisSegmentMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy