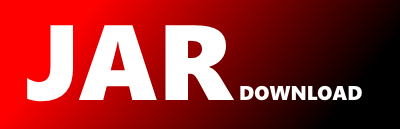
com.amazonaws.services.connectcontactlens.model.Transcript Maven / Gradle / Ivy
Show all versions of aws-java-sdk-connectcontactlens Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.connectcontactlens.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* A list of messages in the session.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Transcript implements Serializable, Cloneable, StructuredPojo {
/**
*
* The identifier of the transcript.
*
*/
private String id;
/**
*
* The identifier of the participant. Valid values are CUSTOMER or AGENT.
*
*/
private String participantId;
/**
*
* The role of participant. For example, is it a customer, agent, or system.
*
*/
private String participantRole;
/**
*
* The content of the transcript.
*
*/
private String content;
/**
*
* The beginning offset in the contact for this transcript.
*
*/
private Integer beginOffsetMillis;
/**
*
* The end offset in the contact for this transcript.
*
*/
private Integer endOffsetMillis;
/**
*
* The sentiment detected for this piece of transcript.
*
*/
private String sentiment;
/**
*
* List of positions where issues were detected on the transcript.
*
*/
private java.util.List issuesDetected;
/**
*
* The identifier of the transcript.
*
*
* @param id
* The identifier of the transcript.
*/
public void setId(String id) {
this.id = id;
}
/**
*
* The identifier of the transcript.
*
*
* @return The identifier of the transcript.
*/
public String getId() {
return this.id;
}
/**
*
* The identifier of the transcript.
*
*
* @param id
* The identifier of the transcript.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Transcript withId(String id) {
setId(id);
return this;
}
/**
*
* The identifier of the participant. Valid values are CUSTOMER or AGENT.
*
*
* @param participantId
* The identifier of the participant. Valid values are CUSTOMER or AGENT.
*/
public void setParticipantId(String participantId) {
this.participantId = participantId;
}
/**
*
* The identifier of the participant. Valid values are CUSTOMER or AGENT.
*
*
* @return The identifier of the participant. Valid values are CUSTOMER or AGENT.
*/
public String getParticipantId() {
return this.participantId;
}
/**
*
* The identifier of the participant. Valid values are CUSTOMER or AGENT.
*
*
* @param participantId
* The identifier of the participant. Valid values are CUSTOMER or AGENT.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Transcript withParticipantId(String participantId) {
setParticipantId(participantId);
return this;
}
/**
*
* The role of participant. For example, is it a customer, agent, or system.
*
*
* @param participantRole
* The role of participant. For example, is it a customer, agent, or system.
*/
public void setParticipantRole(String participantRole) {
this.participantRole = participantRole;
}
/**
*
* The role of participant. For example, is it a customer, agent, or system.
*
*
* @return The role of participant. For example, is it a customer, agent, or system.
*/
public String getParticipantRole() {
return this.participantRole;
}
/**
*
* The role of participant. For example, is it a customer, agent, or system.
*
*
* @param participantRole
* The role of participant. For example, is it a customer, agent, or system.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Transcript withParticipantRole(String participantRole) {
setParticipantRole(participantRole);
return this;
}
/**
*
* The content of the transcript.
*
*
* @param content
* The content of the transcript.
*/
public void setContent(String content) {
this.content = content;
}
/**
*
* The content of the transcript.
*
*
* @return The content of the transcript.
*/
public String getContent() {
return this.content;
}
/**
*
* The content of the transcript.
*
*
* @param content
* The content of the transcript.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Transcript withContent(String content) {
setContent(content);
return this;
}
/**
*
* The beginning offset in the contact for this transcript.
*
*
* @param beginOffsetMillis
* The beginning offset in the contact for this transcript.
*/
public void setBeginOffsetMillis(Integer beginOffsetMillis) {
this.beginOffsetMillis = beginOffsetMillis;
}
/**
*
* The beginning offset in the contact for this transcript.
*
*
* @return The beginning offset in the contact for this transcript.
*/
public Integer getBeginOffsetMillis() {
return this.beginOffsetMillis;
}
/**
*
* The beginning offset in the contact for this transcript.
*
*
* @param beginOffsetMillis
* The beginning offset in the contact for this transcript.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Transcript withBeginOffsetMillis(Integer beginOffsetMillis) {
setBeginOffsetMillis(beginOffsetMillis);
return this;
}
/**
*
* The end offset in the contact for this transcript.
*
*
* @param endOffsetMillis
* The end offset in the contact for this transcript.
*/
public void setEndOffsetMillis(Integer endOffsetMillis) {
this.endOffsetMillis = endOffsetMillis;
}
/**
*
* The end offset in the contact for this transcript.
*
*
* @return The end offset in the contact for this transcript.
*/
public Integer getEndOffsetMillis() {
return this.endOffsetMillis;
}
/**
*
* The end offset in the contact for this transcript.
*
*
* @param endOffsetMillis
* The end offset in the contact for this transcript.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Transcript withEndOffsetMillis(Integer endOffsetMillis) {
setEndOffsetMillis(endOffsetMillis);
return this;
}
/**
*
* The sentiment detected for this piece of transcript.
*
*
* @param sentiment
* The sentiment detected for this piece of transcript.
* @see SentimentValue
*/
public void setSentiment(String sentiment) {
this.sentiment = sentiment;
}
/**
*
* The sentiment detected for this piece of transcript.
*
*
* @return The sentiment detected for this piece of transcript.
* @see SentimentValue
*/
public String getSentiment() {
return this.sentiment;
}
/**
*
* The sentiment detected for this piece of transcript.
*
*
* @param sentiment
* The sentiment detected for this piece of transcript.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SentimentValue
*/
public Transcript withSentiment(String sentiment) {
setSentiment(sentiment);
return this;
}
/**
*
* The sentiment detected for this piece of transcript.
*
*
* @param sentiment
* The sentiment detected for this piece of transcript.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SentimentValue
*/
public Transcript withSentiment(SentimentValue sentiment) {
this.sentiment = sentiment.toString();
return this;
}
/**
*
* List of positions where issues were detected on the transcript.
*
*
* @return List of positions where issues were detected on the transcript.
*/
public java.util.List getIssuesDetected() {
return issuesDetected;
}
/**
*
* List of positions where issues were detected on the transcript.
*
*
* @param issuesDetected
* List of positions where issues were detected on the transcript.
*/
public void setIssuesDetected(java.util.Collection issuesDetected) {
if (issuesDetected == null) {
this.issuesDetected = null;
return;
}
this.issuesDetected = new java.util.ArrayList(issuesDetected);
}
/**
*
* List of positions where issues were detected on the transcript.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setIssuesDetected(java.util.Collection)} or {@link #withIssuesDetected(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param issuesDetected
* List of positions where issues were detected on the transcript.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Transcript withIssuesDetected(IssueDetected... issuesDetected) {
if (this.issuesDetected == null) {
setIssuesDetected(new java.util.ArrayList(issuesDetected.length));
}
for (IssueDetected ele : issuesDetected) {
this.issuesDetected.add(ele);
}
return this;
}
/**
*
* List of positions where issues were detected on the transcript.
*
*
* @param issuesDetected
* List of positions where issues were detected on the transcript.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Transcript withIssuesDetected(java.util.Collection issuesDetected) {
setIssuesDetected(issuesDetected);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getId() != null)
sb.append("Id: ").append(getId()).append(",");
if (getParticipantId() != null)
sb.append("ParticipantId: ").append(getParticipantId()).append(",");
if (getParticipantRole() != null)
sb.append("ParticipantRole: ").append(getParticipantRole()).append(",");
if (getContent() != null)
sb.append("Content: ").append(getContent()).append(",");
if (getBeginOffsetMillis() != null)
sb.append("BeginOffsetMillis: ").append(getBeginOffsetMillis()).append(",");
if (getEndOffsetMillis() != null)
sb.append("EndOffsetMillis: ").append(getEndOffsetMillis()).append(",");
if (getSentiment() != null)
sb.append("Sentiment: ").append(getSentiment()).append(",");
if (getIssuesDetected() != null)
sb.append("IssuesDetected: ").append(getIssuesDetected());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Transcript == false)
return false;
Transcript other = (Transcript) obj;
if (other.getId() == null ^ this.getId() == null)
return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false)
return false;
if (other.getParticipantId() == null ^ this.getParticipantId() == null)
return false;
if (other.getParticipantId() != null && other.getParticipantId().equals(this.getParticipantId()) == false)
return false;
if (other.getParticipantRole() == null ^ this.getParticipantRole() == null)
return false;
if (other.getParticipantRole() != null && other.getParticipantRole().equals(this.getParticipantRole()) == false)
return false;
if (other.getContent() == null ^ this.getContent() == null)
return false;
if (other.getContent() != null && other.getContent().equals(this.getContent()) == false)
return false;
if (other.getBeginOffsetMillis() == null ^ this.getBeginOffsetMillis() == null)
return false;
if (other.getBeginOffsetMillis() != null && other.getBeginOffsetMillis().equals(this.getBeginOffsetMillis()) == false)
return false;
if (other.getEndOffsetMillis() == null ^ this.getEndOffsetMillis() == null)
return false;
if (other.getEndOffsetMillis() != null && other.getEndOffsetMillis().equals(this.getEndOffsetMillis()) == false)
return false;
if (other.getSentiment() == null ^ this.getSentiment() == null)
return false;
if (other.getSentiment() != null && other.getSentiment().equals(this.getSentiment()) == false)
return false;
if (other.getIssuesDetected() == null ^ this.getIssuesDetected() == null)
return false;
if (other.getIssuesDetected() != null && other.getIssuesDetected().equals(this.getIssuesDetected()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getParticipantId() == null) ? 0 : getParticipantId().hashCode());
hashCode = prime * hashCode + ((getParticipantRole() == null) ? 0 : getParticipantRole().hashCode());
hashCode = prime * hashCode + ((getContent() == null) ? 0 : getContent().hashCode());
hashCode = prime * hashCode + ((getBeginOffsetMillis() == null) ? 0 : getBeginOffsetMillis().hashCode());
hashCode = prime * hashCode + ((getEndOffsetMillis() == null) ? 0 : getEndOffsetMillis().hashCode());
hashCode = prime * hashCode + ((getSentiment() == null) ? 0 : getSentiment().hashCode());
hashCode = prime * hashCode + ((getIssuesDetected() == null) ? 0 : getIssuesDetected().hashCode());
return hashCode;
}
@Override
public Transcript clone() {
try {
return (Transcript) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.connectcontactlens.model.transform.TranscriptMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}