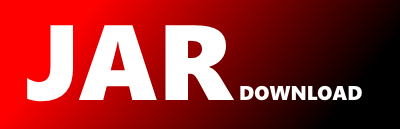
com.amazonaws.services.customerprofiles.model.UpdateAddress Maven / Gradle / Ivy
Show all versions of aws-java-sdk-customerprofiles Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.customerprofiles.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Updates associated with the address properties of a customer profile.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateAddress implements Serializable, Cloneable, StructuredPojo {
/**
*
* The first line of a customer address.
*
*/
private String address1;
/**
*
* The second line of a customer address.
*
*/
private String address2;
/**
*
* The third line of a customer address.
*
*/
private String address3;
/**
*
* The fourth line of a customer address.
*
*/
private String address4;
/**
*
* The city in which a customer lives.
*
*/
private String city;
/**
*
* The county in which a customer lives.
*
*/
private String county;
/**
*
* The state in which a customer lives.
*
*/
private String state;
/**
*
* The province in which a customer lives.
*
*/
private String province;
/**
*
* The country in which a customer lives.
*
*/
private String country;
/**
*
* The postal code of a customer address.
*
*/
private String postalCode;
/**
*
* The first line of a customer address.
*
*
* @param address1
* The first line of a customer address.
*/
public void setAddress1(String address1) {
this.address1 = address1;
}
/**
*
* The first line of a customer address.
*
*
* @return The first line of a customer address.
*/
public String getAddress1() {
return this.address1;
}
/**
*
* The first line of a customer address.
*
*
* @param address1
* The first line of a customer address.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateAddress withAddress1(String address1) {
setAddress1(address1);
return this;
}
/**
*
* The second line of a customer address.
*
*
* @param address2
* The second line of a customer address.
*/
public void setAddress2(String address2) {
this.address2 = address2;
}
/**
*
* The second line of a customer address.
*
*
* @return The second line of a customer address.
*/
public String getAddress2() {
return this.address2;
}
/**
*
* The second line of a customer address.
*
*
* @param address2
* The second line of a customer address.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateAddress withAddress2(String address2) {
setAddress2(address2);
return this;
}
/**
*
* The third line of a customer address.
*
*
* @param address3
* The third line of a customer address.
*/
public void setAddress3(String address3) {
this.address3 = address3;
}
/**
*
* The third line of a customer address.
*
*
* @return The third line of a customer address.
*/
public String getAddress3() {
return this.address3;
}
/**
*
* The third line of a customer address.
*
*
* @param address3
* The third line of a customer address.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateAddress withAddress3(String address3) {
setAddress3(address3);
return this;
}
/**
*
* The fourth line of a customer address.
*
*
* @param address4
* The fourth line of a customer address.
*/
public void setAddress4(String address4) {
this.address4 = address4;
}
/**
*
* The fourth line of a customer address.
*
*
* @return The fourth line of a customer address.
*/
public String getAddress4() {
return this.address4;
}
/**
*
* The fourth line of a customer address.
*
*
* @param address4
* The fourth line of a customer address.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateAddress withAddress4(String address4) {
setAddress4(address4);
return this;
}
/**
*
* The city in which a customer lives.
*
*
* @param city
* The city in which a customer lives.
*/
public void setCity(String city) {
this.city = city;
}
/**
*
* The city in which a customer lives.
*
*
* @return The city in which a customer lives.
*/
public String getCity() {
return this.city;
}
/**
*
* The city in which a customer lives.
*
*
* @param city
* The city in which a customer lives.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateAddress withCity(String city) {
setCity(city);
return this;
}
/**
*
* The county in which a customer lives.
*
*
* @param county
* The county in which a customer lives.
*/
public void setCounty(String county) {
this.county = county;
}
/**
*
* The county in which a customer lives.
*
*
* @return The county in which a customer lives.
*/
public String getCounty() {
return this.county;
}
/**
*
* The county in which a customer lives.
*
*
* @param county
* The county in which a customer lives.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateAddress withCounty(String county) {
setCounty(county);
return this;
}
/**
*
* The state in which a customer lives.
*
*
* @param state
* The state in which a customer lives.
*/
public void setState(String state) {
this.state = state;
}
/**
*
* The state in which a customer lives.
*
*
* @return The state in which a customer lives.
*/
public String getState() {
return this.state;
}
/**
*
* The state in which a customer lives.
*
*
* @param state
* The state in which a customer lives.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateAddress withState(String state) {
setState(state);
return this;
}
/**
*
* The province in which a customer lives.
*
*
* @param province
* The province in which a customer lives.
*/
public void setProvince(String province) {
this.province = province;
}
/**
*
* The province in which a customer lives.
*
*
* @return The province in which a customer lives.
*/
public String getProvince() {
return this.province;
}
/**
*
* The province in which a customer lives.
*
*
* @param province
* The province in which a customer lives.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateAddress withProvince(String province) {
setProvince(province);
return this;
}
/**
*
* The country in which a customer lives.
*
*
* @param country
* The country in which a customer lives.
*/
public void setCountry(String country) {
this.country = country;
}
/**
*
* The country in which a customer lives.
*
*
* @return The country in which a customer lives.
*/
public String getCountry() {
return this.country;
}
/**
*
* The country in which a customer lives.
*
*
* @param country
* The country in which a customer lives.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateAddress withCountry(String country) {
setCountry(country);
return this;
}
/**
*
* The postal code of a customer address.
*
*
* @param postalCode
* The postal code of a customer address.
*/
public void setPostalCode(String postalCode) {
this.postalCode = postalCode;
}
/**
*
* The postal code of a customer address.
*
*
* @return The postal code of a customer address.
*/
public String getPostalCode() {
return this.postalCode;
}
/**
*
* The postal code of a customer address.
*
*
* @param postalCode
* The postal code of a customer address.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateAddress withPostalCode(String postalCode) {
setPostalCode(postalCode);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAddress1() != null)
sb.append("Address1: ").append(getAddress1()).append(",");
if (getAddress2() != null)
sb.append("Address2: ").append(getAddress2()).append(",");
if (getAddress3() != null)
sb.append("Address3: ").append(getAddress3()).append(",");
if (getAddress4() != null)
sb.append("Address4: ").append(getAddress4()).append(",");
if (getCity() != null)
sb.append("City: ").append(getCity()).append(",");
if (getCounty() != null)
sb.append("County: ").append(getCounty()).append(",");
if (getState() != null)
sb.append("State: ").append(getState()).append(",");
if (getProvince() != null)
sb.append("Province: ").append(getProvince()).append(",");
if (getCountry() != null)
sb.append("Country: ").append(getCountry()).append(",");
if (getPostalCode() != null)
sb.append("PostalCode: ").append(getPostalCode());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateAddress == false)
return false;
UpdateAddress other = (UpdateAddress) obj;
if (other.getAddress1() == null ^ this.getAddress1() == null)
return false;
if (other.getAddress1() != null && other.getAddress1().equals(this.getAddress1()) == false)
return false;
if (other.getAddress2() == null ^ this.getAddress2() == null)
return false;
if (other.getAddress2() != null && other.getAddress2().equals(this.getAddress2()) == false)
return false;
if (other.getAddress3() == null ^ this.getAddress3() == null)
return false;
if (other.getAddress3() != null && other.getAddress3().equals(this.getAddress3()) == false)
return false;
if (other.getAddress4() == null ^ this.getAddress4() == null)
return false;
if (other.getAddress4() != null && other.getAddress4().equals(this.getAddress4()) == false)
return false;
if (other.getCity() == null ^ this.getCity() == null)
return false;
if (other.getCity() != null && other.getCity().equals(this.getCity()) == false)
return false;
if (other.getCounty() == null ^ this.getCounty() == null)
return false;
if (other.getCounty() != null && other.getCounty().equals(this.getCounty()) == false)
return false;
if (other.getState() == null ^ this.getState() == null)
return false;
if (other.getState() != null && other.getState().equals(this.getState()) == false)
return false;
if (other.getProvince() == null ^ this.getProvince() == null)
return false;
if (other.getProvince() != null && other.getProvince().equals(this.getProvince()) == false)
return false;
if (other.getCountry() == null ^ this.getCountry() == null)
return false;
if (other.getCountry() != null && other.getCountry().equals(this.getCountry()) == false)
return false;
if (other.getPostalCode() == null ^ this.getPostalCode() == null)
return false;
if (other.getPostalCode() != null && other.getPostalCode().equals(this.getPostalCode()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAddress1() == null) ? 0 : getAddress1().hashCode());
hashCode = prime * hashCode + ((getAddress2() == null) ? 0 : getAddress2().hashCode());
hashCode = prime * hashCode + ((getAddress3() == null) ? 0 : getAddress3().hashCode());
hashCode = prime * hashCode + ((getAddress4() == null) ? 0 : getAddress4().hashCode());
hashCode = prime * hashCode + ((getCity() == null) ? 0 : getCity().hashCode());
hashCode = prime * hashCode + ((getCounty() == null) ? 0 : getCounty().hashCode());
hashCode = prime * hashCode + ((getState() == null) ? 0 : getState().hashCode());
hashCode = prime * hashCode + ((getProvince() == null) ? 0 : getProvince().hashCode());
hashCode = prime * hashCode + ((getCountry() == null) ? 0 : getCountry().hashCode());
hashCode = prime * hashCode + ((getPostalCode() == null) ? 0 : getPostalCode().hashCode());
return hashCode;
}
@Override
public UpdateAddress clone() {
try {
return (UpdateAddress) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.customerprofiles.model.transform.UpdateAddressMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}