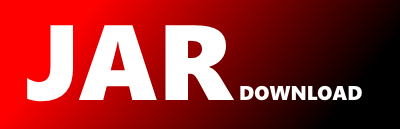
com.amazonaws.services.customerprofiles.model.FlowDefinition Maven / Gradle / Ivy
Show all versions of aws-java-sdk-customerprofiles Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.customerprofiles.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The configurations that control how Customer Profiles retrieves data from the source, Amazon AppFlow. Customer
* Profiles uses this information to create an AppFlow flow on behalf of customers.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class FlowDefinition implements Serializable, Cloneable, StructuredPojo {
/**
*
* A description of the flow you want to create.
*
*/
private String description;
/**
*
* The specified name of the flow. Use underscores (_) or hyphens (-) only. Spaces are not allowed.
*
*/
private String flowName;
/**
*
* The Amazon Resource Name of the AWS Key Management Service (KMS) key you provide for encryption.
*
*/
private String kmsArn;
/**
*
* The configuration that controls how Customer Profiles retrieves data from the source.
*
*/
private SourceFlowConfig sourceFlowConfig;
/**
*
* A list of tasks that Customer Profiles performs while transferring the data in the flow run.
*
*/
private java.util.List tasks;
/**
*
* The trigger settings that determine how and when the flow runs.
*
*/
private TriggerConfig triggerConfig;
/**
*
* A description of the flow you want to create.
*
*
* @param description
* A description of the flow you want to create.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the flow you want to create.
*
*
* @return A description of the flow you want to create.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the flow you want to create.
*
*
* @param description
* A description of the flow you want to create.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FlowDefinition withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The specified name of the flow. Use underscores (_) or hyphens (-) only. Spaces are not allowed.
*
*
* @param flowName
* The specified name of the flow. Use underscores (_) or hyphens (-) only. Spaces are not allowed.
*/
public void setFlowName(String flowName) {
this.flowName = flowName;
}
/**
*
* The specified name of the flow. Use underscores (_) or hyphens (-) only. Spaces are not allowed.
*
*
* @return The specified name of the flow. Use underscores (_) or hyphens (-) only. Spaces are not allowed.
*/
public String getFlowName() {
return this.flowName;
}
/**
*
* The specified name of the flow. Use underscores (_) or hyphens (-) only. Spaces are not allowed.
*
*
* @param flowName
* The specified name of the flow. Use underscores (_) or hyphens (-) only. Spaces are not allowed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FlowDefinition withFlowName(String flowName) {
setFlowName(flowName);
return this;
}
/**
*
* The Amazon Resource Name of the AWS Key Management Service (KMS) key you provide for encryption.
*
*
* @param kmsArn
* The Amazon Resource Name of the AWS Key Management Service (KMS) key you provide for encryption.
*/
public void setKmsArn(String kmsArn) {
this.kmsArn = kmsArn;
}
/**
*
* The Amazon Resource Name of the AWS Key Management Service (KMS) key you provide for encryption.
*
*
* @return The Amazon Resource Name of the AWS Key Management Service (KMS) key you provide for encryption.
*/
public String getKmsArn() {
return this.kmsArn;
}
/**
*
* The Amazon Resource Name of the AWS Key Management Service (KMS) key you provide for encryption.
*
*
* @param kmsArn
* The Amazon Resource Name of the AWS Key Management Service (KMS) key you provide for encryption.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FlowDefinition withKmsArn(String kmsArn) {
setKmsArn(kmsArn);
return this;
}
/**
*
* The configuration that controls how Customer Profiles retrieves data from the source.
*
*
* @param sourceFlowConfig
* The configuration that controls how Customer Profiles retrieves data from the source.
*/
public void setSourceFlowConfig(SourceFlowConfig sourceFlowConfig) {
this.sourceFlowConfig = sourceFlowConfig;
}
/**
*
* The configuration that controls how Customer Profiles retrieves data from the source.
*
*
* @return The configuration that controls how Customer Profiles retrieves data from the source.
*/
public SourceFlowConfig getSourceFlowConfig() {
return this.sourceFlowConfig;
}
/**
*
* The configuration that controls how Customer Profiles retrieves data from the source.
*
*
* @param sourceFlowConfig
* The configuration that controls how Customer Profiles retrieves data from the source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FlowDefinition withSourceFlowConfig(SourceFlowConfig sourceFlowConfig) {
setSourceFlowConfig(sourceFlowConfig);
return this;
}
/**
*
* A list of tasks that Customer Profiles performs while transferring the data in the flow run.
*
*
* @return A list of tasks that Customer Profiles performs while transferring the data in the flow run.
*/
public java.util.List getTasks() {
return tasks;
}
/**
*
* A list of tasks that Customer Profiles performs while transferring the data in the flow run.
*
*
* @param tasks
* A list of tasks that Customer Profiles performs while transferring the data in the flow run.
*/
public void setTasks(java.util.Collection tasks) {
if (tasks == null) {
this.tasks = null;
return;
}
this.tasks = new java.util.ArrayList(tasks);
}
/**
*
* A list of tasks that Customer Profiles performs while transferring the data in the flow run.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTasks(java.util.Collection)} or {@link #withTasks(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tasks
* A list of tasks that Customer Profiles performs while transferring the data in the flow run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FlowDefinition withTasks(Task... tasks) {
if (this.tasks == null) {
setTasks(new java.util.ArrayList(tasks.length));
}
for (Task ele : tasks) {
this.tasks.add(ele);
}
return this;
}
/**
*
* A list of tasks that Customer Profiles performs while transferring the data in the flow run.
*
*
* @param tasks
* A list of tasks that Customer Profiles performs while transferring the data in the flow run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FlowDefinition withTasks(java.util.Collection tasks) {
setTasks(tasks);
return this;
}
/**
*
* The trigger settings that determine how and when the flow runs.
*
*
* @param triggerConfig
* The trigger settings that determine how and when the flow runs.
*/
public void setTriggerConfig(TriggerConfig triggerConfig) {
this.triggerConfig = triggerConfig;
}
/**
*
* The trigger settings that determine how and when the flow runs.
*
*
* @return The trigger settings that determine how and when the flow runs.
*/
public TriggerConfig getTriggerConfig() {
return this.triggerConfig;
}
/**
*
* The trigger settings that determine how and when the flow runs.
*
*
* @param triggerConfig
* The trigger settings that determine how and when the flow runs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FlowDefinition withTriggerConfig(TriggerConfig triggerConfig) {
setTriggerConfig(triggerConfig);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getFlowName() != null)
sb.append("FlowName: ").append(getFlowName()).append(",");
if (getKmsArn() != null)
sb.append("KmsArn: ").append(getKmsArn()).append(",");
if (getSourceFlowConfig() != null)
sb.append("SourceFlowConfig: ").append(getSourceFlowConfig()).append(",");
if (getTasks() != null)
sb.append("Tasks: ").append(getTasks()).append(",");
if (getTriggerConfig() != null)
sb.append("TriggerConfig: ").append(getTriggerConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof FlowDefinition == false)
return false;
FlowDefinition other = (FlowDefinition) obj;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getFlowName() == null ^ this.getFlowName() == null)
return false;
if (other.getFlowName() != null && other.getFlowName().equals(this.getFlowName()) == false)
return false;
if (other.getKmsArn() == null ^ this.getKmsArn() == null)
return false;
if (other.getKmsArn() != null && other.getKmsArn().equals(this.getKmsArn()) == false)
return false;
if (other.getSourceFlowConfig() == null ^ this.getSourceFlowConfig() == null)
return false;
if (other.getSourceFlowConfig() != null && other.getSourceFlowConfig().equals(this.getSourceFlowConfig()) == false)
return false;
if (other.getTasks() == null ^ this.getTasks() == null)
return false;
if (other.getTasks() != null && other.getTasks().equals(this.getTasks()) == false)
return false;
if (other.getTriggerConfig() == null ^ this.getTriggerConfig() == null)
return false;
if (other.getTriggerConfig() != null && other.getTriggerConfig().equals(this.getTriggerConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getFlowName() == null) ? 0 : getFlowName().hashCode());
hashCode = prime * hashCode + ((getKmsArn() == null) ? 0 : getKmsArn().hashCode());
hashCode = prime * hashCode + ((getSourceFlowConfig() == null) ? 0 : getSourceFlowConfig().hashCode());
hashCode = prime * hashCode + ((getTasks() == null) ? 0 : getTasks().hashCode());
hashCode = prime * hashCode + ((getTriggerConfig() == null) ? 0 : getTriggerConfig().hashCode());
return hashCode;
}
@Override
public FlowDefinition clone() {
try {
return (FlowDefinition) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.customerprofiles.model.transform.FlowDefinitionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}