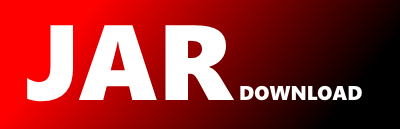
com.amazonaws.services.customerprofiles.model.GetAutoMergingPreviewResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-customerprofiles Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.customerprofiles.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetAutoMergingPreviewResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The unique name of the domain.
*
*/
private String domainName;
/**
*
* The number of match groups in the domain that have been reviewed in this preview dry run.
*
*/
private Long numberOfMatchesInSample;
/**
*
* The number of profiles found in this preview dry run.
*
*/
private Long numberOfProfilesInSample;
/**
*
* The number of profiles that would be merged if this wasn't a preview dry run.
*
*/
private Long numberOfProfilesWillBeMerged;
/**
*
* The unique name of the domain.
*
*
* @param domainName
* The unique name of the domain.
*/
public void setDomainName(String domainName) {
this.domainName = domainName;
}
/**
*
* The unique name of the domain.
*
*
* @return The unique name of the domain.
*/
public String getDomainName() {
return this.domainName;
}
/**
*
* The unique name of the domain.
*
*
* @param domainName
* The unique name of the domain.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetAutoMergingPreviewResult withDomainName(String domainName) {
setDomainName(domainName);
return this;
}
/**
*
* The number of match groups in the domain that have been reviewed in this preview dry run.
*
*
* @param numberOfMatchesInSample
* The number of match groups in the domain that have been reviewed in this preview dry run.
*/
public void setNumberOfMatchesInSample(Long numberOfMatchesInSample) {
this.numberOfMatchesInSample = numberOfMatchesInSample;
}
/**
*
* The number of match groups in the domain that have been reviewed in this preview dry run.
*
*
* @return The number of match groups in the domain that have been reviewed in this preview dry run.
*/
public Long getNumberOfMatchesInSample() {
return this.numberOfMatchesInSample;
}
/**
*
* The number of match groups in the domain that have been reviewed in this preview dry run.
*
*
* @param numberOfMatchesInSample
* The number of match groups in the domain that have been reviewed in this preview dry run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetAutoMergingPreviewResult withNumberOfMatchesInSample(Long numberOfMatchesInSample) {
setNumberOfMatchesInSample(numberOfMatchesInSample);
return this;
}
/**
*
* The number of profiles found in this preview dry run.
*
*
* @param numberOfProfilesInSample
* The number of profiles found in this preview dry run.
*/
public void setNumberOfProfilesInSample(Long numberOfProfilesInSample) {
this.numberOfProfilesInSample = numberOfProfilesInSample;
}
/**
*
* The number of profiles found in this preview dry run.
*
*
* @return The number of profiles found in this preview dry run.
*/
public Long getNumberOfProfilesInSample() {
return this.numberOfProfilesInSample;
}
/**
*
* The number of profiles found in this preview dry run.
*
*
* @param numberOfProfilesInSample
* The number of profiles found in this preview dry run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetAutoMergingPreviewResult withNumberOfProfilesInSample(Long numberOfProfilesInSample) {
setNumberOfProfilesInSample(numberOfProfilesInSample);
return this;
}
/**
*
* The number of profiles that would be merged if this wasn't a preview dry run.
*
*
* @param numberOfProfilesWillBeMerged
* The number of profiles that would be merged if this wasn't a preview dry run.
*/
public void setNumberOfProfilesWillBeMerged(Long numberOfProfilesWillBeMerged) {
this.numberOfProfilesWillBeMerged = numberOfProfilesWillBeMerged;
}
/**
*
* The number of profiles that would be merged if this wasn't a preview dry run.
*
*
* @return The number of profiles that would be merged if this wasn't a preview dry run.
*/
public Long getNumberOfProfilesWillBeMerged() {
return this.numberOfProfilesWillBeMerged;
}
/**
*
* The number of profiles that would be merged if this wasn't a preview dry run.
*
*
* @param numberOfProfilesWillBeMerged
* The number of profiles that would be merged if this wasn't a preview dry run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetAutoMergingPreviewResult withNumberOfProfilesWillBeMerged(Long numberOfProfilesWillBeMerged) {
setNumberOfProfilesWillBeMerged(numberOfProfilesWillBeMerged);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDomainName() != null)
sb.append("DomainName: ").append(getDomainName()).append(",");
if (getNumberOfMatchesInSample() != null)
sb.append("NumberOfMatchesInSample: ").append(getNumberOfMatchesInSample()).append(",");
if (getNumberOfProfilesInSample() != null)
sb.append("NumberOfProfilesInSample: ").append(getNumberOfProfilesInSample()).append(",");
if (getNumberOfProfilesWillBeMerged() != null)
sb.append("NumberOfProfilesWillBeMerged: ").append(getNumberOfProfilesWillBeMerged());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetAutoMergingPreviewResult == false)
return false;
GetAutoMergingPreviewResult other = (GetAutoMergingPreviewResult) obj;
if (other.getDomainName() == null ^ this.getDomainName() == null)
return false;
if (other.getDomainName() != null && other.getDomainName().equals(this.getDomainName()) == false)
return false;
if (other.getNumberOfMatchesInSample() == null ^ this.getNumberOfMatchesInSample() == null)
return false;
if (other.getNumberOfMatchesInSample() != null && other.getNumberOfMatchesInSample().equals(this.getNumberOfMatchesInSample()) == false)
return false;
if (other.getNumberOfProfilesInSample() == null ^ this.getNumberOfProfilesInSample() == null)
return false;
if (other.getNumberOfProfilesInSample() != null && other.getNumberOfProfilesInSample().equals(this.getNumberOfProfilesInSample()) == false)
return false;
if (other.getNumberOfProfilesWillBeMerged() == null ^ this.getNumberOfProfilesWillBeMerged() == null)
return false;
if (other.getNumberOfProfilesWillBeMerged() != null && other.getNumberOfProfilesWillBeMerged().equals(this.getNumberOfProfilesWillBeMerged()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDomainName() == null) ? 0 : getDomainName().hashCode());
hashCode = prime * hashCode + ((getNumberOfMatchesInSample() == null) ? 0 : getNumberOfMatchesInSample().hashCode());
hashCode = prime * hashCode + ((getNumberOfProfilesInSample() == null) ? 0 : getNumberOfProfilesInSample().hashCode());
hashCode = prime * hashCode + ((getNumberOfProfilesWillBeMerged() == null) ? 0 : getNumberOfProfilesWillBeMerged().hashCode());
return hashCode;
}
@Override
public GetAutoMergingPreviewResult clone() {
try {
return (GetAutoMergingPreviewResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}