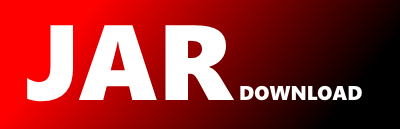
com.amazonaws.services.customerprofiles.model.GetWorkflowResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-customerprofiles Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.customerprofiles.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetWorkflowResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* Unique identifier for the workflow.
*
*/
private String workflowId;
/**
*
* The type of workflow. The only supported value is APPFLOW_INTEGRATION.
*
*/
private String workflowType;
/**
*
* Status of workflow execution.
*
*/
private String status;
/**
*
* Workflow error messages during execution (if any).
*
*/
private String errorDescription;
/**
*
* The timestamp that represents when workflow execution started.
*
*/
private java.util.Date startDate;
/**
*
* The timestamp that represents when workflow execution last updated.
*
*/
private java.util.Date lastUpdatedAt;
/**
*
* Attributes provided for workflow execution.
*
*/
private WorkflowAttributes attributes;
/**
*
* Workflow specific execution metrics.
*
*/
private WorkflowMetrics metrics;
/**
*
* Unique identifier for the workflow.
*
*
* @param workflowId
* Unique identifier for the workflow.
*/
public void setWorkflowId(String workflowId) {
this.workflowId = workflowId;
}
/**
*
* Unique identifier for the workflow.
*
*
* @return Unique identifier for the workflow.
*/
public String getWorkflowId() {
return this.workflowId;
}
/**
*
* Unique identifier for the workflow.
*
*
* @param workflowId
* Unique identifier for the workflow.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetWorkflowResult withWorkflowId(String workflowId) {
setWorkflowId(workflowId);
return this;
}
/**
*
* The type of workflow. The only supported value is APPFLOW_INTEGRATION.
*
*
* @param workflowType
* The type of workflow. The only supported value is APPFLOW_INTEGRATION.
* @see WorkflowType
*/
public void setWorkflowType(String workflowType) {
this.workflowType = workflowType;
}
/**
*
* The type of workflow. The only supported value is APPFLOW_INTEGRATION.
*
*
* @return The type of workflow. The only supported value is APPFLOW_INTEGRATION.
* @see WorkflowType
*/
public String getWorkflowType() {
return this.workflowType;
}
/**
*
* The type of workflow. The only supported value is APPFLOW_INTEGRATION.
*
*
* @param workflowType
* The type of workflow. The only supported value is APPFLOW_INTEGRATION.
* @return Returns a reference to this object so that method calls can be chained together.
* @see WorkflowType
*/
public GetWorkflowResult withWorkflowType(String workflowType) {
setWorkflowType(workflowType);
return this;
}
/**
*
* The type of workflow. The only supported value is APPFLOW_INTEGRATION.
*
*
* @param workflowType
* The type of workflow. The only supported value is APPFLOW_INTEGRATION.
* @return Returns a reference to this object so that method calls can be chained together.
* @see WorkflowType
*/
public GetWorkflowResult withWorkflowType(WorkflowType workflowType) {
this.workflowType = workflowType.toString();
return this;
}
/**
*
* Status of workflow execution.
*
*
* @param status
* Status of workflow execution.
* @see Status
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* Status of workflow execution.
*
*
* @return Status of workflow execution.
* @see Status
*/
public String getStatus() {
return this.status;
}
/**
*
* Status of workflow execution.
*
*
* @param status
* Status of workflow execution.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Status
*/
public GetWorkflowResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* Status of workflow execution.
*
*
* @param status
* Status of workflow execution.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Status
*/
public GetWorkflowResult withStatus(Status status) {
this.status = status.toString();
return this;
}
/**
*
* Workflow error messages during execution (if any).
*
*
* @param errorDescription
* Workflow error messages during execution (if any).
*/
public void setErrorDescription(String errorDescription) {
this.errorDescription = errorDescription;
}
/**
*
* Workflow error messages during execution (if any).
*
*
* @return Workflow error messages during execution (if any).
*/
public String getErrorDescription() {
return this.errorDescription;
}
/**
*
* Workflow error messages during execution (if any).
*
*
* @param errorDescription
* Workflow error messages during execution (if any).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetWorkflowResult withErrorDescription(String errorDescription) {
setErrorDescription(errorDescription);
return this;
}
/**
*
* The timestamp that represents when workflow execution started.
*
*
* @param startDate
* The timestamp that represents when workflow execution started.
*/
public void setStartDate(java.util.Date startDate) {
this.startDate = startDate;
}
/**
*
* The timestamp that represents when workflow execution started.
*
*
* @return The timestamp that represents when workflow execution started.
*/
public java.util.Date getStartDate() {
return this.startDate;
}
/**
*
* The timestamp that represents when workflow execution started.
*
*
* @param startDate
* The timestamp that represents when workflow execution started.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetWorkflowResult withStartDate(java.util.Date startDate) {
setStartDate(startDate);
return this;
}
/**
*
* The timestamp that represents when workflow execution last updated.
*
*
* @param lastUpdatedAt
* The timestamp that represents when workflow execution last updated.
*/
public void setLastUpdatedAt(java.util.Date lastUpdatedAt) {
this.lastUpdatedAt = lastUpdatedAt;
}
/**
*
* The timestamp that represents when workflow execution last updated.
*
*
* @return The timestamp that represents when workflow execution last updated.
*/
public java.util.Date getLastUpdatedAt() {
return this.lastUpdatedAt;
}
/**
*
* The timestamp that represents when workflow execution last updated.
*
*
* @param lastUpdatedAt
* The timestamp that represents when workflow execution last updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetWorkflowResult withLastUpdatedAt(java.util.Date lastUpdatedAt) {
setLastUpdatedAt(lastUpdatedAt);
return this;
}
/**
*
* Attributes provided for workflow execution.
*
*
* @param attributes
* Attributes provided for workflow execution.
*/
public void setAttributes(WorkflowAttributes attributes) {
this.attributes = attributes;
}
/**
*
* Attributes provided for workflow execution.
*
*
* @return Attributes provided for workflow execution.
*/
public WorkflowAttributes getAttributes() {
return this.attributes;
}
/**
*
* Attributes provided for workflow execution.
*
*
* @param attributes
* Attributes provided for workflow execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetWorkflowResult withAttributes(WorkflowAttributes attributes) {
setAttributes(attributes);
return this;
}
/**
*
* Workflow specific execution metrics.
*
*
* @param metrics
* Workflow specific execution metrics.
*/
public void setMetrics(WorkflowMetrics metrics) {
this.metrics = metrics;
}
/**
*
* Workflow specific execution metrics.
*
*
* @return Workflow specific execution metrics.
*/
public WorkflowMetrics getMetrics() {
return this.metrics;
}
/**
*
* Workflow specific execution metrics.
*
*
* @param metrics
* Workflow specific execution metrics.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetWorkflowResult withMetrics(WorkflowMetrics metrics) {
setMetrics(metrics);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getWorkflowId() != null)
sb.append("WorkflowId: ").append(getWorkflowId()).append(",");
if (getWorkflowType() != null)
sb.append("WorkflowType: ").append(getWorkflowType()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getErrorDescription() != null)
sb.append("ErrorDescription: ").append(getErrorDescription()).append(",");
if (getStartDate() != null)
sb.append("StartDate: ").append(getStartDate()).append(",");
if (getLastUpdatedAt() != null)
sb.append("LastUpdatedAt: ").append(getLastUpdatedAt()).append(",");
if (getAttributes() != null)
sb.append("Attributes: ").append(getAttributes()).append(",");
if (getMetrics() != null)
sb.append("Metrics: ").append(getMetrics());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetWorkflowResult == false)
return false;
GetWorkflowResult other = (GetWorkflowResult) obj;
if (other.getWorkflowId() == null ^ this.getWorkflowId() == null)
return false;
if (other.getWorkflowId() != null && other.getWorkflowId().equals(this.getWorkflowId()) == false)
return false;
if (other.getWorkflowType() == null ^ this.getWorkflowType() == null)
return false;
if (other.getWorkflowType() != null && other.getWorkflowType().equals(this.getWorkflowType()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getErrorDescription() == null ^ this.getErrorDescription() == null)
return false;
if (other.getErrorDescription() != null && other.getErrorDescription().equals(this.getErrorDescription()) == false)
return false;
if (other.getStartDate() == null ^ this.getStartDate() == null)
return false;
if (other.getStartDate() != null && other.getStartDate().equals(this.getStartDate()) == false)
return false;
if (other.getLastUpdatedAt() == null ^ this.getLastUpdatedAt() == null)
return false;
if (other.getLastUpdatedAt() != null && other.getLastUpdatedAt().equals(this.getLastUpdatedAt()) == false)
return false;
if (other.getAttributes() == null ^ this.getAttributes() == null)
return false;
if (other.getAttributes() != null && other.getAttributes().equals(this.getAttributes()) == false)
return false;
if (other.getMetrics() == null ^ this.getMetrics() == null)
return false;
if (other.getMetrics() != null && other.getMetrics().equals(this.getMetrics()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getWorkflowId() == null) ? 0 : getWorkflowId().hashCode());
hashCode = prime * hashCode + ((getWorkflowType() == null) ? 0 : getWorkflowType().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getErrorDescription() == null) ? 0 : getErrorDescription().hashCode());
hashCode = prime * hashCode + ((getStartDate() == null) ? 0 : getStartDate().hashCode());
hashCode = prime * hashCode + ((getLastUpdatedAt() == null) ? 0 : getLastUpdatedAt().hashCode());
hashCode = prime * hashCode + ((getAttributes() == null) ? 0 : getAttributes().hashCode());
hashCode = prime * hashCode + ((getMetrics() == null) ? 0 : getMetrics().hashCode());
return hashCode;
}
@Override
public GetWorkflowResult clone() {
try {
return (GetWorkflowResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}