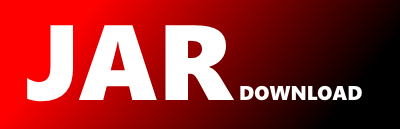
com.amazonaws.services.customerprofiles.AmazonCustomerProfiles Maven / Gradle / Ivy
Show all versions of aws-java-sdk-customerprofiles Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.customerprofiles;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.customerprofiles.model.*;
/**
* Interface for accessing Customer Profiles.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.customerprofiles.AbstractAmazonCustomerProfiles} instead.
*
*
* Amazon Connect Customer Profiles
*
* Amazon Connect Customer Profiles is a unified customer profile for your contact center that has pre-built connectors
* powered by AppFlow that make it easy to combine customer information from third party applications, such as
* Salesforce (CRM), ServiceNow (ITSM), and your enterprise resource planning (ERP), with contact history from your
* Amazon Connect contact center.
*
*
* For more information about the Amazon Connect Customer Profiles feature, see Use Customer Profiles in the
* Amazon Connect Administrator's Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonCustomerProfiles {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "profile";
/**
*
* Associates a new key value with a specific profile, such as a Contact Record ContactId.
*
*
* A profile object can have a single unique key and any number of additional keys that can be used to identify the
* profile that it belongs to.
*
*
* @param addProfileKeyRequest
* @return Result of the AddProfileKey operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.AddProfileKey
* @see AWS API Documentation
*/
AddProfileKeyResult addProfileKey(AddProfileKeyRequest addProfileKeyRequest);
/**
*
* Creates a new calculated attribute definition. After creation, new object data ingested into Customer Profiles
* will be included in the calculated attribute, which can be retrieved for a profile using the GetCalculatedAttributeForProfile API. Defining a calculated attribute makes it available for all profiles
* within a domain. Each calculated attribute can only reference one ObjectType
and at most, two fields
* from that ObjectType
.
*
*
* @param createCalculatedAttributeDefinitionRequest
* @return Result of the CreateCalculatedAttributeDefinition operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.CreateCalculatedAttributeDefinition
* @see AWS API Documentation
*/
CreateCalculatedAttributeDefinitionResult createCalculatedAttributeDefinition(
CreateCalculatedAttributeDefinitionRequest createCalculatedAttributeDefinitionRequest);
/**
*
* Creates a domain, which is a container for all customer data, such as customer profile attributes, object types,
* profile keys, and encryption keys. You can create multiple domains, and each domain can have multiple third-party
* integrations.
*
*
* Each Amazon Connect instance can be associated with only one domain. Multiple Amazon Connect instances can be
* associated with one domain.
*
*
* Use this API or UpdateDomain to
* enable identity
* resolution: set Matching
to true.
*
*
* To prevent cross-service impersonation when you call this API, see Cross-service confused deputy prevention for sample policies that you should apply.
*
*
*
* It is not possible to associate a Customer Profiles domain with an Amazon Connect Instance directly from the API.
* If you would like to create a domain and associate a Customer Profiles domain, use the Amazon Connect admin
* website. For more information, see Enable Customer Profiles.
*
*
* Each Amazon Connect instance can be associated with only one domain. Multiple Amazon Connect instances can be
* associated with one domain.
*
*
*
* @param createDomainRequest
* @return Result of the CreateDomain operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.CreateDomain
* @see AWS
* API Documentation
*/
CreateDomainResult createDomain(CreateDomainRequest createDomainRequest);
/**
*
* Creates an event stream, which is a subscription to real-time events, such as when profiles are created and
* updated through Amazon Connect Customer Profiles.
*
*
* Each event stream can be associated with only one Kinesis Data Stream destination in the same region and Amazon
* Web Services account as the customer profiles domain
*
*
* @param createEventStreamRequest
* @return Result of the CreateEventStream operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.CreateEventStream
* @see AWS API Documentation
*/
CreateEventStreamResult createEventStream(CreateEventStreamRequest createEventStreamRequest);
/**
*
* Creates an integration workflow. An integration workflow is an async process which ingests historic data and sets
* up an integration for ongoing updates. The supported Amazon AppFlow sources are Salesforce, ServiceNow, and
* Marketo.
*
*
* @param createIntegrationWorkflowRequest
* @return Result of the CreateIntegrationWorkflow operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.CreateIntegrationWorkflow
* @see AWS API Documentation
*/
CreateIntegrationWorkflowResult createIntegrationWorkflow(CreateIntegrationWorkflowRequest createIntegrationWorkflowRequest);
/**
*
* Creates a standard profile.
*
*
* A standard profile represents the following attributes for a customer profile in a domain.
*
*
* @param createProfileRequest
* @return Result of the CreateProfile operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.CreateProfile
* @see AWS API Documentation
*/
CreateProfileResult createProfile(CreateProfileRequest createProfileRequest);
/**
*
* Deletes an existing calculated attribute definition. Note that deleting a default calculated attribute is
* possible, however once deleted, you will be unable to undo that action and will need to recreate it on your own
* using the CreateCalculatedAttributeDefinition API if you want it back.
*
*
* @param deleteCalculatedAttributeDefinitionRequest
* @return Result of the DeleteCalculatedAttributeDefinition operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.DeleteCalculatedAttributeDefinition
* @see AWS API Documentation
*/
DeleteCalculatedAttributeDefinitionResult deleteCalculatedAttributeDefinition(
DeleteCalculatedAttributeDefinitionRequest deleteCalculatedAttributeDefinitionRequest);
/**
*
* Deletes a specific domain and all of its customer data, such as customer profile attributes and their related
* objects.
*
*
* @param deleteDomainRequest
* @return Result of the DeleteDomain operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.DeleteDomain
* @see AWS
* API Documentation
*/
DeleteDomainResult deleteDomain(DeleteDomainRequest deleteDomainRequest);
/**
*
* Disables and deletes the specified event stream.
*
*
* @param deleteEventStreamRequest
* @return Result of the DeleteEventStream operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.DeleteEventStream
* @see AWS API Documentation
*/
DeleteEventStreamResult deleteEventStream(DeleteEventStreamRequest deleteEventStreamRequest);
/**
*
* Removes an integration from a specific domain.
*
*
* @param deleteIntegrationRequest
* @return Result of the DeleteIntegration operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.DeleteIntegration
* @see AWS API Documentation
*/
DeleteIntegrationResult deleteIntegration(DeleteIntegrationRequest deleteIntegrationRequest);
/**
*
* Deletes the standard customer profile and all data pertaining to the profile.
*
*
* @param deleteProfileRequest
* @return Result of the DeleteProfile operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.DeleteProfile
* @see AWS API Documentation
*/
DeleteProfileResult deleteProfile(DeleteProfileRequest deleteProfileRequest);
/**
*
* Removes a searchable key from a customer profile.
*
*
* @param deleteProfileKeyRequest
* @return Result of the DeleteProfileKey operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.DeleteProfileKey
* @see AWS API Documentation
*/
DeleteProfileKeyResult deleteProfileKey(DeleteProfileKeyRequest deleteProfileKeyRequest);
/**
*
* Removes an object associated with a profile of a given ProfileObjectType.
*
*
* @param deleteProfileObjectRequest
* @return Result of the DeleteProfileObject operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.DeleteProfileObject
* @see AWS API Documentation
*/
DeleteProfileObjectResult deleteProfileObject(DeleteProfileObjectRequest deleteProfileObjectRequest);
/**
*
* Removes a ProfileObjectType from a specific domain as well as removes all the ProfileObjects of that type. It
* also disables integrations from this specific ProfileObjectType. In addition, it scrubs all of the fields of the
* standard profile that were populated from this ProfileObjectType.
*
*
* @param deleteProfileObjectTypeRequest
* @return Result of the DeleteProfileObjectType operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.DeleteProfileObjectType
* @see AWS API Documentation
*/
DeleteProfileObjectTypeResult deleteProfileObjectType(DeleteProfileObjectTypeRequest deleteProfileObjectTypeRequest);
/**
*
* Deletes the specified workflow and all its corresponding resources. This is an async process.
*
*
* @param deleteWorkflowRequest
* @return Result of the DeleteWorkflow operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.DeleteWorkflow
* @see AWS API Documentation
*/
DeleteWorkflowResult deleteWorkflow(DeleteWorkflowRequest deleteWorkflowRequest);
/**
*
* The process of detecting profile object type mapping by using given objects.
*
*
* @param detectProfileObjectTypeRequest
* @return Result of the DetectProfileObjectType operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.DetectProfileObjectType
* @see AWS API Documentation
*/
DetectProfileObjectTypeResult detectProfileObjectType(DetectProfileObjectTypeRequest detectProfileObjectTypeRequest);
/**
*
* Tests the auto-merging settings of your Identity Resolution Job without merging your data. It randomly selects a
* sample of matching groups from the existing matching results, and applies the automerging settings that you
* provided. You can then view the number of profiles in the sample, the number of matches, and the number of
* profiles identified to be merged. This enables you to evaluate the accuracy of the attributes in your matching
* list.
*
*
* You can't view which profiles are matched and would be merged.
*
*
*
* We strongly recommend you use this API to do a dry run of the automerging process before running the Identity
* Resolution Job. Include at least two matching attributes. If your matching list includes too few
* attributes (such as only FirstName
or only LastName
), there may be a large number of
* matches. This increases the chances of erroneous merges.
*
*
*
* @param getAutoMergingPreviewRequest
* @return Result of the GetAutoMergingPreview operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.GetAutoMergingPreview
* @see AWS API Documentation
*/
GetAutoMergingPreviewResult getAutoMergingPreview(GetAutoMergingPreviewRequest getAutoMergingPreviewRequest);
/**
*
* Provides more information on a calculated attribute definition for Customer Profiles.
*
*
* @param getCalculatedAttributeDefinitionRequest
* @return Result of the GetCalculatedAttributeDefinition operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.GetCalculatedAttributeDefinition
* @see AWS API Documentation
*/
GetCalculatedAttributeDefinitionResult getCalculatedAttributeDefinition(GetCalculatedAttributeDefinitionRequest getCalculatedAttributeDefinitionRequest);
/**
*
* Retrieve a calculated attribute for a customer profile.
*
*
* @param getCalculatedAttributeForProfileRequest
* @return Result of the GetCalculatedAttributeForProfile operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.GetCalculatedAttributeForProfile
* @see AWS API Documentation
*/
GetCalculatedAttributeForProfileResult getCalculatedAttributeForProfile(GetCalculatedAttributeForProfileRequest getCalculatedAttributeForProfileRequest);
/**
*
* Returns information about a specific domain.
*
*
* @param getDomainRequest
* @return Result of the GetDomain operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.GetDomain
* @see AWS
* API Documentation
*/
GetDomainResult getDomain(GetDomainRequest getDomainRequest);
/**
*
* Returns information about the specified event stream in a specific domain.
*
*
* @param getEventStreamRequest
* @return Result of the GetEventStream operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.GetEventStream
* @see AWS API Documentation
*/
GetEventStreamResult getEventStream(GetEventStreamRequest getEventStreamRequest);
/**
*
* Returns information about an Identity Resolution Job in a specific domain.
*
*
* Identity Resolution Jobs are set up using the Amazon Connect admin console. For more information, see Use Identity Resolution
* to consolidate similar profiles.
*
*
* @param getIdentityResolutionJobRequest
* @return Result of the GetIdentityResolutionJob operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.GetIdentityResolutionJob
* @see AWS API Documentation
*/
GetIdentityResolutionJobResult getIdentityResolutionJob(GetIdentityResolutionJobRequest getIdentityResolutionJobRequest);
/**
*
* Returns an integration for a domain.
*
*
* @param getIntegrationRequest
* @return Result of the GetIntegration operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.GetIntegration
* @see AWS API Documentation
*/
GetIntegrationResult getIntegration(GetIntegrationRequest getIntegrationRequest);
/**
*
* Before calling this API, use CreateDomain or
* UpdateDomain
* to enable identity resolution: set Matching
to true.
*
*
* GetMatches returns potentially matching profiles, based on the results of the latest run of a machine learning
* process.
*
*
*
* The process of matching duplicate profiles. If Matching
= true
, Amazon Connect Customer
* Profiles starts a weekly batch process called Identity Resolution Job. If you do not specify a date and time for
* Identity Resolution Job to run, by default it runs every Saturday at 12AM UTC to detect duplicate profiles in
* your domains.
*
*
* After the Identity Resolution Job completes, use the GetMatches API to
* return and review the results. Or, if you have configured ExportingConfig
in the
* MatchingRequest
, you can download the results from S3.
*
*
*
* Amazon Connect uses the following profile attributes to identify matches:
*
*
* -
*
* PhoneNumber
*
*
* -
*
* HomePhoneNumber
*
*
* -
*
* BusinessPhoneNumber
*
*
* -
*
* MobilePhoneNumber
*
*
* -
*
* EmailAddress
*
*
* -
*
* PersonalEmailAddress
*
*
* -
*
* BusinessEmailAddress
*
*
* -
*
* FullName
*
*
*
*
* For example, two or more profiles—with spelling mistakes such as John Doe and Jhn Doe, or different
* casing email addresses such as [email protected] and [email protected], or different
* phone number formats such as 555-010-0000 and +1-555-010-0000—can be detected as belonging to the
* same customer John Doe and merged into a unified profile.
*
*
* @param getMatchesRequest
* @return Result of the GetMatches operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.GetMatches
* @see AWS
* API Documentation
*/
GetMatchesResult getMatches(GetMatchesRequest getMatchesRequest);
/**
*
* Returns the object types for a specific domain.
*
*
* @param getProfileObjectTypeRequest
* @return Result of the GetProfileObjectType operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.GetProfileObjectType
* @see AWS API Documentation
*/
GetProfileObjectTypeResult getProfileObjectType(GetProfileObjectTypeRequest getProfileObjectTypeRequest);
/**
*
* Returns the template information for a specific object type.
*
*
* A template is a predefined ProfileObjectType, such as “Salesforce-Account” or “Salesforce-Contact.” When a user
* sends a ProfileObject, using the PutProfileObject API, with an ObjectTypeName that matches one of the
* TemplateIds, it uses the mappings from the template.
*
*
* @param getProfileObjectTypeTemplateRequest
* @return Result of the GetProfileObjectTypeTemplate operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.GetProfileObjectTypeTemplate
* @see AWS API Documentation
*/
GetProfileObjectTypeTemplateResult getProfileObjectTypeTemplate(GetProfileObjectTypeTemplateRequest getProfileObjectTypeTemplateRequest);
/**
*
* Returns a set of profiles that belong to the same matching group using the matchId
or
* profileId
. You can also specify the type of matching that you want for finding similar profiles
* using either RULE_BASED_MATCHING
or ML_BASED_MATCHING
.
*
*
* @param getSimilarProfilesRequest
* @return Result of the GetSimilarProfiles operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.GetSimilarProfiles
* @see AWS API Documentation
*/
GetSimilarProfilesResult getSimilarProfiles(GetSimilarProfilesRequest getSimilarProfilesRequest);
/**
*
* Get details of specified workflow.
*
*
* @param getWorkflowRequest
* @return Result of the GetWorkflow operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.GetWorkflow
* @see AWS
* API Documentation
*/
GetWorkflowResult getWorkflow(GetWorkflowRequest getWorkflowRequest);
/**
*
* Get granular list of steps in workflow.
*
*
* @param getWorkflowStepsRequest
* @return Result of the GetWorkflowSteps operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.GetWorkflowSteps
* @see AWS API Documentation
*/
GetWorkflowStepsResult getWorkflowSteps(GetWorkflowStepsRequest getWorkflowStepsRequest);
/**
*
* Lists all of the integrations associated to a specific URI in the AWS account.
*
*
* @param listAccountIntegrationsRequest
* @return Result of the ListAccountIntegrations operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.ListAccountIntegrations
* @see AWS API Documentation
*/
ListAccountIntegrationsResult listAccountIntegrations(ListAccountIntegrationsRequest listAccountIntegrationsRequest);
/**
*
* Lists calculated attribute definitions for Customer Profiles
*
*
* @param listCalculatedAttributeDefinitionsRequest
* @return Result of the ListCalculatedAttributeDefinitions operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.ListCalculatedAttributeDefinitions
* @see AWS API Documentation
*/
ListCalculatedAttributeDefinitionsResult listCalculatedAttributeDefinitions(
ListCalculatedAttributeDefinitionsRequest listCalculatedAttributeDefinitionsRequest);
/**
*
* Retrieve a list of calculated attributes for a customer profile.
*
*
* @param listCalculatedAttributesForProfileRequest
* @return Result of the ListCalculatedAttributesForProfile operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.ListCalculatedAttributesForProfile
* @see AWS API Documentation
*/
ListCalculatedAttributesForProfileResult listCalculatedAttributesForProfile(
ListCalculatedAttributesForProfileRequest listCalculatedAttributesForProfileRequest);
/**
*
* Returns a list of all the domains for an AWS account that have been created.
*
*
* @param listDomainsRequest
* @return Result of the ListDomains operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.ListDomains
* @see AWS
* API Documentation
*/
ListDomainsResult listDomains(ListDomainsRequest listDomainsRequest);
/**
*
* Returns a list of all the event streams in a specific domain.
*
*
* @param listEventStreamsRequest
* @return Result of the ListEventStreams operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.ListEventStreams
* @see AWS API Documentation
*/
ListEventStreamsResult listEventStreams(ListEventStreamsRequest listEventStreamsRequest);
/**
*
* Lists all of the Identity Resolution Jobs in your domain. The response sorts the list by
* JobStartTime
.
*
*
* @param listIdentityResolutionJobsRequest
* @return Result of the ListIdentityResolutionJobs operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.ListIdentityResolutionJobs
* @see AWS API Documentation
*/
ListIdentityResolutionJobsResult listIdentityResolutionJobs(ListIdentityResolutionJobsRequest listIdentityResolutionJobsRequest);
/**
*
* Lists all of the integrations in your domain.
*
*
* @param listIntegrationsRequest
* @return Result of the ListIntegrations operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.ListIntegrations
* @see AWS API Documentation
*/
ListIntegrationsResult listIntegrations(ListIntegrationsRequest listIntegrationsRequest);
/**
*
* Lists all of the template information for object types.
*
*
* @param listProfileObjectTypeTemplatesRequest
* @return Result of the ListProfileObjectTypeTemplates operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.ListProfileObjectTypeTemplates
* @see AWS API Documentation
*/
ListProfileObjectTypeTemplatesResult listProfileObjectTypeTemplates(ListProfileObjectTypeTemplatesRequest listProfileObjectTypeTemplatesRequest);
/**
*
* Lists all of the templates available within the service.
*
*
* @param listProfileObjectTypesRequest
* @return Result of the ListProfileObjectTypes operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.ListProfileObjectTypes
* @see AWS API Documentation
*/
ListProfileObjectTypesResult listProfileObjectTypes(ListProfileObjectTypesRequest listProfileObjectTypesRequest);
/**
*
* Returns a list of objects associated with a profile of a given ProfileObjectType.
*
*
* @param listProfileObjectsRequest
* @return Result of the ListProfileObjects operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.ListProfileObjects
* @see AWS API Documentation
*/
ListProfileObjectsResult listProfileObjects(ListProfileObjectsRequest listProfileObjectsRequest);
/**
*
* Returns a set of MatchIds
that belong to the given domain.
*
*
* @param listRuleBasedMatchesRequest
* @return Result of the ListRuleBasedMatches operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.ListRuleBasedMatches
* @see AWS API Documentation
*/
ListRuleBasedMatchesResult listRuleBasedMatches(ListRuleBasedMatchesRequest listRuleBasedMatchesRequest);
/**
*
* Displays the tags associated with an Amazon Connect Customer Profiles resource. In Connect Customer Profiles,
* domains, profile object types, and integrations can be tagged.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServerException
* An internal service error occurred.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @sample AmazonCustomerProfiles.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Query to list all workflows.
*
*
* @param listWorkflowsRequest
* @return Result of the ListWorkflows operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.ListWorkflows
* @see AWS API Documentation
*/
ListWorkflowsResult listWorkflows(ListWorkflowsRequest listWorkflowsRequest);
/**
*
* Runs an AWS Lambda job that does the following:
*
*
* -
*
* All the profileKeys in the ProfileToBeMerged
will be moved to the main profile.
*
*
* -
*
* All the objects in the ProfileToBeMerged
will be moved to the main profile.
*
*
* -
*
* All the ProfileToBeMerged
will be deleted at the end.
*
*
* -
*
* All the profileKeys in the ProfileIdsToBeMerged
will be moved to the main profile.
*
*
* -
*
* Standard fields are merged as follows:
*
*
* -
*
* Fields are always "union"-ed if there are no conflicts in standard fields or attributeKeys.
*
*
* -
*
* When there are conflicting fields:
*
*
* -
*
* If no SourceProfileIds
entry is specified, the main Profile value is always taken.
*
*
* -
*
* If a SourceProfileIds
entry is specified, the specified profileId is always taken, even if it is a
* NULL value.
*
*
*
*
*
*
*
*
* You can use MergeProfiles together with GetMatches, which
* returns potentially matching profiles, or use it with the results of another matching system. After profiles have
* been merged, they cannot be separated (unmerged).
*
*
* @param mergeProfilesRequest
* @return Result of the MergeProfiles operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.MergeProfiles
* @see AWS API Documentation
*/
MergeProfilesResult mergeProfiles(MergeProfilesRequest mergeProfilesRequest);
/**
*
* Adds an integration between the service and a third-party service, which includes Amazon AppFlow and Amazon
* Connect.
*
*
* An integration can belong to only one domain.
*
*
* To add or remove tags on an existing Integration, see TagResource /
* UntagResource.
*
*
* @param putIntegrationRequest
* @return Result of the PutIntegration operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.PutIntegration
* @see AWS API Documentation
*/
PutIntegrationResult putIntegration(PutIntegrationRequest putIntegrationRequest);
/**
*
* Adds additional objects to customer profiles of a given ObjectType.
*
*
* When adding a specific profile object, like a Contact Record, an inferred profile can get created if it is not
* mapped to an existing profile. The resulting profile will only have a phone number populated in the standard
* ProfileObject. Any additional Contact Records with the same phone number will be mapped to the same inferred
* profile.
*
*
* When a ProfileObject is created and if a ProfileObjectType already exists for the ProfileObject, it will provide
* data to a standard profile depending on the ProfileObjectType definition.
*
*
* PutProfileObject needs an ObjectType, which can be created using PutProfileObjectType.
*
*
* @param putProfileObjectRequest
* @return Result of the PutProfileObject operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.PutProfileObject
* @see AWS API Documentation
*/
PutProfileObjectResult putProfileObject(PutProfileObjectRequest putProfileObjectRequest);
/**
*
* Defines a ProfileObjectType.
*
*
* To add or remove tags on an existing ObjectType, see TagResource/UntagResource.
*
*
* @param putProfileObjectTypeRequest
* @return Result of the PutProfileObjectType operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.PutProfileObjectType
* @see AWS API Documentation
*/
PutProfileObjectTypeResult putProfileObjectType(PutProfileObjectTypeRequest putProfileObjectTypeRequest);
/**
*
* Searches for profiles within a specific domain using one or more predefined search keys (e.g., _fullName, _phone,
* _email, _account, etc.) and/or custom-defined search keys. A search key is a data type pair that consists of a
* KeyName
and Values
list.
*
*
* This operation supports searching for profiles with a minimum of 1 key-value(s) pair and up to 5 key-value(s)
* pairs using either AND
or OR
logic.
*
*
* @param searchProfilesRequest
* @return Result of the SearchProfiles operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.SearchProfiles
* @see AWS API Documentation
*/
SearchProfilesResult searchProfiles(SearchProfilesRequest searchProfilesRequest);
/**
*
* Assigns one or more tags (key-value pairs) to the specified Amazon Connect Customer Profiles resource. Tags can
* help you organize and categorize your resources. You can also use them to scope user permissions by granting a
* user permission to access or change only resources with certain tag values. In Connect Customer Profiles,
* domains, profile object types, and integrations can be tagged.
*
*
* Tags don't have any semantic meaning to AWS and are interpreted strictly as strings of characters.
*
*
* You can use the TagResource action with a resource that already has tags. If you specify a new tag key, this tag
* is appended to the list of tags associated with the resource. If you specify a tag key that is already associated
* with the resource, the new tag value that you specify replaces the previous value for that tag.
*
*
* You can associate as many as 50 tags with a resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalServerException
* An internal service error occurred.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @sample AmazonCustomerProfiles.TagResource
* @see AWS
* API Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes one or more tags from the specified Amazon Connect Customer Profiles resource. In Connect Customer
* Profiles, domains, profile object types, and integrations can be tagged.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServerException
* An internal service error occurred.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @sample AmazonCustomerProfiles.UntagResource
* @see AWS API Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates an existing calculated attribute definition. When updating the Conditions, note that increasing the date
* range of a calculated attribute will not trigger inclusion of historical data greater than the current date
* range.
*
*
* @param updateCalculatedAttributeDefinitionRequest
* @return Result of the UpdateCalculatedAttributeDefinition operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.UpdateCalculatedAttributeDefinition
* @see AWS API Documentation
*/
UpdateCalculatedAttributeDefinitionResult updateCalculatedAttributeDefinition(
UpdateCalculatedAttributeDefinitionRequest updateCalculatedAttributeDefinitionRequest);
/**
*
* Updates the properties of a domain, including creating or selecting a dead letter queue or an encryption key.
*
*
* After a domain is created, the name can’t be changed.
*
*
* Use this API or CreateDomain to
* enable identity
* resolution: set Matching
to true.
*
*
* To prevent cross-service impersonation when you call this API, see Cross-service confused deputy prevention for sample policies that you should apply.
*
*
* To add or remove tags on an existing Domain, see TagResource/UntagResource.
*
*
* @param updateDomainRequest
* @return Result of the UpdateDomain operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.UpdateDomain
* @see AWS
* API Documentation
*/
UpdateDomainResult updateDomain(UpdateDomainRequest updateDomainRequest);
/**
*
* Updates the properties of a profile. The ProfileId is required for updating a customer profile.
*
*
* When calling the UpdateProfile API, specifying an empty string value means that any existing value will be
* removed. Not specifying a string value means that any value already there will be kept.
*
*
* @param updateProfileRequest
* @return Result of the UpdateProfile operation returned by the service.
* @throws BadRequestException
* The input you provided is invalid.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The requested resource does not exist, or access was denied.
* @throws ThrottlingException
* You exceeded the maximum number of requests.
* @throws InternalServerException
* An internal service error occurred.
* @sample AmazonCustomerProfiles.UpdateProfile
* @see AWS API Documentation
*/
UpdateProfileResult updateProfile(UpdateProfileRequest updateProfileRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}