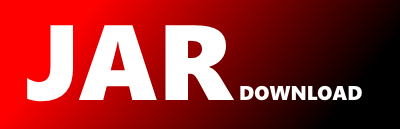
com.amazonaws.services.customerprofiles.AmazonCustomerProfilesAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-customerprofiles Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.customerprofiles;
import javax.annotation.Generated;
import com.amazonaws.services.customerprofiles.model.*;
/**
* Interface for accessing Customer Profiles asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.customerprofiles.AbstractAmazonCustomerProfilesAsync} instead.
*
*
* Amazon Connect Customer Profiles
*
* Amazon Connect Customer Profiles is a unified customer profile for your contact center that has pre-built connectors
* powered by AppFlow that make it easy to combine customer information from third party applications, such as
* Salesforce (CRM), ServiceNow (ITSM), and your enterprise resource planning (ERP), with contact history from your
* Amazon Connect contact center.
*
*
* For more information about the Amazon Connect Customer Profiles feature, see Use Customer Profiles in the
* Amazon Connect Administrator's Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonCustomerProfilesAsync extends AmazonCustomerProfiles {
/**
*
* Associates a new key value with a specific profile, such as a Contact Record ContactId.
*
*
* A profile object can have a single unique key and any number of additional keys that can be used to identify the
* profile that it belongs to.
*
*
* @param addProfileKeyRequest
* @return A Java Future containing the result of the AddProfileKey operation returned by the service.
* @sample AmazonCustomerProfilesAsync.AddProfileKey
* @see AWS API Documentation
*/
java.util.concurrent.Future addProfileKeyAsync(AddProfileKeyRequest addProfileKeyRequest);
/**
*
* Associates a new key value with a specific profile, such as a Contact Record ContactId.
*
*
* A profile object can have a single unique key and any number of additional keys that can be used to identify the
* profile that it belongs to.
*
*
* @param addProfileKeyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddProfileKey operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.AddProfileKey
* @see AWS API Documentation
*/
java.util.concurrent.Future addProfileKeyAsync(AddProfileKeyRequest addProfileKeyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new calculated attribute definition. After creation, new object data ingested into Customer Profiles
* will be included in the calculated attribute, which can be retrieved for a profile using the GetCalculatedAttributeForProfile API. Defining a calculated attribute makes it available for all profiles
* within a domain. Each calculated attribute can only reference one ObjectType
and at most, two fields
* from that ObjectType
.
*
*
* @param createCalculatedAttributeDefinitionRequest
* @return A Java Future containing the result of the CreateCalculatedAttributeDefinition operation returned by the
* service.
* @sample AmazonCustomerProfilesAsync.CreateCalculatedAttributeDefinition
* @see AWS API Documentation
*/
java.util.concurrent.Future createCalculatedAttributeDefinitionAsync(
CreateCalculatedAttributeDefinitionRequest createCalculatedAttributeDefinitionRequest);
/**
*
* Creates a new calculated attribute definition. After creation, new object data ingested into Customer Profiles
* will be included in the calculated attribute, which can be retrieved for a profile using the GetCalculatedAttributeForProfile API. Defining a calculated attribute makes it available for all profiles
* within a domain. Each calculated attribute can only reference one ObjectType
and at most, two fields
* from that ObjectType
.
*
*
* @param createCalculatedAttributeDefinitionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCalculatedAttributeDefinition operation returned by the
* service.
* @sample AmazonCustomerProfilesAsyncHandler.CreateCalculatedAttributeDefinition
* @see AWS API Documentation
*/
java.util.concurrent.Future createCalculatedAttributeDefinitionAsync(
CreateCalculatedAttributeDefinitionRequest createCalculatedAttributeDefinitionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a domain, which is a container for all customer data, such as customer profile attributes, object types,
* profile keys, and encryption keys. You can create multiple domains, and each domain can have multiple third-party
* integrations.
*
*
* Each Amazon Connect instance can be associated with only one domain. Multiple Amazon Connect instances can be
* associated with one domain.
*
*
* Use this API or UpdateDomain to
* enable identity
* resolution: set Matching
to true.
*
*
* To prevent cross-service impersonation when you call this API, see Cross-service confused deputy prevention for sample policies that you should apply.
*
*
*
* It is not possible to associate a Customer Profiles domain with an Amazon Connect Instance directly from the API.
* If you would like to create a domain and associate a Customer Profiles domain, use the Amazon Connect admin
* website. For more information, see Enable Customer Profiles.
*
*
* Each Amazon Connect instance can be associated with only one domain. Multiple Amazon Connect instances can be
* associated with one domain.
*
*
*
* @param createDomainRequest
* @return A Java Future containing the result of the CreateDomain operation returned by the service.
* @sample AmazonCustomerProfilesAsync.CreateDomain
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDomainAsync(CreateDomainRequest createDomainRequest);
/**
*
* Creates a domain, which is a container for all customer data, such as customer profile attributes, object types,
* profile keys, and encryption keys. You can create multiple domains, and each domain can have multiple third-party
* integrations.
*
*
* Each Amazon Connect instance can be associated with only one domain. Multiple Amazon Connect instances can be
* associated with one domain.
*
*
* Use this API or UpdateDomain to
* enable identity
* resolution: set Matching
to true.
*
*
* To prevent cross-service impersonation when you call this API, see Cross-service confused deputy prevention for sample policies that you should apply.
*
*
*
* It is not possible to associate a Customer Profiles domain with an Amazon Connect Instance directly from the API.
* If you would like to create a domain and associate a Customer Profiles domain, use the Amazon Connect admin
* website. For more information, see Enable Customer Profiles.
*
*
* Each Amazon Connect instance can be associated with only one domain. Multiple Amazon Connect instances can be
* associated with one domain.
*
*
*
* @param createDomainRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDomain operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.CreateDomain
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDomainAsync(CreateDomainRequest createDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an event stream, which is a subscription to real-time events, such as when profiles are created and
* updated through Amazon Connect Customer Profiles.
*
*
* Each event stream can be associated with only one Kinesis Data Stream destination in the same region and Amazon
* Web Services account as the customer profiles domain
*
*
* @param createEventStreamRequest
* @return A Java Future containing the result of the CreateEventStream operation returned by the service.
* @sample AmazonCustomerProfilesAsync.CreateEventStream
* @see AWS API Documentation
*/
java.util.concurrent.Future createEventStreamAsync(CreateEventStreamRequest createEventStreamRequest);
/**
*
* Creates an event stream, which is a subscription to real-time events, such as when profiles are created and
* updated through Amazon Connect Customer Profiles.
*
*
* Each event stream can be associated with only one Kinesis Data Stream destination in the same region and Amazon
* Web Services account as the customer profiles domain
*
*
* @param createEventStreamRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEventStream operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.CreateEventStream
* @see AWS API Documentation
*/
java.util.concurrent.Future createEventStreamAsync(CreateEventStreamRequest createEventStreamRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an integration workflow. An integration workflow is an async process which ingests historic data and sets
* up an integration for ongoing updates. The supported Amazon AppFlow sources are Salesforce, ServiceNow, and
* Marketo.
*
*
* @param createIntegrationWorkflowRequest
* @return A Java Future containing the result of the CreateIntegrationWorkflow operation returned by the service.
* @sample AmazonCustomerProfilesAsync.CreateIntegrationWorkflow
* @see AWS API Documentation
*/
java.util.concurrent.Future createIntegrationWorkflowAsync(
CreateIntegrationWorkflowRequest createIntegrationWorkflowRequest);
/**
*
* Creates an integration workflow. An integration workflow is an async process which ingests historic data and sets
* up an integration for ongoing updates. The supported Amazon AppFlow sources are Salesforce, ServiceNow, and
* Marketo.
*
*
* @param createIntegrationWorkflowRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateIntegrationWorkflow operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.CreateIntegrationWorkflow
* @see AWS API Documentation
*/
java.util.concurrent.Future createIntegrationWorkflowAsync(
CreateIntegrationWorkflowRequest createIntegrationWorkflowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a standard profile.
*
*
* A standard profile represents the following attributes for a customer profile in a domain.
*
*
* @param createProfileRequest
* @return A Java Future containing the result of the CreateProfile operation returned by the service.
* @sample AmazonCustomerProfilesAsync.CreateProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future createProfileAsync(CreateProfileRequest createProfileRequest);
/**
*
* Creates a standard profile.
*
*
* A standard profile represents the following attributes for a customer profile in a domain.
*
*
* @param createProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateProfile operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.CreateProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future createProfileAsync(CreateProfileRequest createProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing calculated attribute definition. Note that deleting a default calculated attribute is
* possible, however once deleted, you will be unable to undo that action and will need to recreate it on your own
* using the CreateCalculatedAttributeDefinition API if you want it back.
*
*
* @param deleteCalculatedAttributeDefinitionRequest
* @return A Java Future containing the result of the DeleteCalculatedAttributeDefinition operation returned by the
* service.
* @sample AmazonCustomerProfilesAsync.DeleteCalculatedAttributeDefinition
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCalculatedAttributeDefinitionAsync(
DeleteCalculatedAttributeDefinitionRequest deleteCalculatedAttributeDefinitionRequest);
/**
*
* Deletes an existing calculated attribute definition. Note that deleting a default calculated attribute is
* possible, however once deleted, you will be unable to undo that action and will need to recreate it on your own
* using the CreateCalculatedAttributeDefinition API if you want it back.
*
*
* @param deleteCalculatedAttributeDefinitionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCalculatedAttributeDefinition operation returned by the
* service.
* @sample AmazonCustomerProfilesAsyncHandler.DeleteCalculatedAttributeDefinition
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCalculatedAttributeDefinitionAsync(
DeleteCalculatedAttributeDefinitionRequest deleteCalculatedAttributeDefinitionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a specific domain and all of its customer data, such as customer profile attributes and their related
* objects.
*
*
* @param deleteDomainRequest
* @return A Java Future containing the result of the DeleteDomain operation returned by the service.
* @sample AmazonCustomerProfilesAsync.DeleteDomain
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDomainAsync(DeleteDomainRequest deleteDomainRequest);
/**
*
* Deletes a specific domain and all of its customer data, such as customer profile attributes and their related
* objects.
*
*
* @param deleteDomainRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDomain operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.DeleteDomain
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDomainAsync(DeleteDomainRequest deleteDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disables and deletes the specified event stream.
*
*
* @param deleteEventStreamRequest
* @return A Java Future containing the result of the DeleteEventStream operation returned by the service.
* @sample AmazonCustomerProfilesAsync.DeleteEventStream
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEventStreamAsync(DeleteEventStreamRequest deleteEventStreamRequest);
/**
*
* Disables and deletes the specified event stream.
*
*
* @param deleteEventStreamRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEventStream operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.DeleteEventStream
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEventStreamAsync(DeleteEventStreamRequest deleteEventStreamRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes an integration from a specific domain.
*
*
* @param deleteIntegrationRequest
* @return A Java Future containing the result of the DeleteIntegration operation returned by the service.
* @sample AmazonCustomerProfilesAsync.DeleteIntegration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteIntegrationAsync(DeleteIntegrationRequest deleteIntegrationRequest);
/**
*
* Removes an integration from a specific domain.
*
*
* @param deleteIntegrationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteIntegration operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.DeleteIntegration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteIntegrationAsync(DeleteIntegrationRequest deleteIntegrationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the standard customer profile and all data pertaining to the profile.
*
*
* @param deleteProfileRequest
* @return A Java Future containing the result of the DeleteProfile operation returned by the service.
* @sample AmazonCustomerProfilesAsync.DeleteProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteProfileAsync(DeleteProfileRequest deleteProfileRequest);
/**
*
* Deletes the standard customer profile and all data pertaining to the profile.
*
*
* @param deleteProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteProfile operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.DeleteProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteProfileAsync(DeleteProfileRequest deleteProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a searchable key from a customer profile.
*
*
* @param deleteProfileKeyRequest
* @return A Java Future containing the result of the DeleteProfileKey operation returned by the service.
* @sample AmazonCustomerProfilesAsync.DeleteProfileKey
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteProfileKeyAsync(DeleteProfileKeyRequest deleteProfileKeyRequest);
/**
*
* Removes a searchable key from a customer profile.
*
*
* @param deleteProfileKeyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteProfileKey operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.DeleteProfileKey
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteProfileKeyAsync(DeleteProfileKeyRequest deleteProfileKeyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes an object associated with a profile of a given ProfileObjectType.
*
*
* @param deleteProfileObjectRequest
* @return A Java Future containing the result of the DeleteProfileObject operation returned by the service.
* @sample AmazonCustomerProfilesAsync.DeleteProfileObject
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteProfileObjectAsync(DeleteProfileObjectRequest deleteProfileObjectRequest);
/**
*
* Removes an object associated with a profile of a given ProfileObjectType.
*
*
* @param deleteProfileObjectRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteProfileObject operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.DeleteProfileObject
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteProfileObjectAsync(DeleteProfileObjectRequest deleteProfileObjectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a ProfileObjectType from a specific domain as well as removes all the ProfileObjects of that type. It
* also disables integrations from this specific ProfileObjectType. In addition, it scrubs all of the fields of the
* standard profile that were populated from this ProfileObjectType.
*
*
* @param deleteProfileObjectTypeRequest
* @return A Java Future containing the result of the DeleteProfileObjectType operation returned by the service.
* @sample AmazonCustomerProfilesAsync.DeleteProfileObjectType
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteProfileObjectTypeAsync(DeleteProfileObjectTypeRequest deleteProfileObjectTypeRequest);
/**
*
* Removes a ProfileObjectType from a specific domain as well as removes all the ProfileObjects of that type. It
* also disables integrations from this specific ProfileObjectType. In addition, it scrubs all of the fields of the
* standard profile that were populated from this ProfileObjectType.
*
*
* @param deleteProfileObjectTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteProfileObjectType operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.DeleteProfileObjectType
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteProfileObjectTypeAsync(DeleteProfileObjectTypeRequest deleteProfileObjectTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified workflow and all its corresponding resources. This is an async process.
*
*
* @param deleteWorkflowRequest
* @return A Java Future containing the result of the DeleteWorkflow operation returned by the service.
* @sample AmazonCustomerProfilesAsync.DeleteWorkflow
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteWorkflowAsync(DeleteWorkflowRequest deleteWorkflowRequest);
/**
*
* Deletes the specified workflow and all its corresponding resources. This is an async process.
*
*
* @param deleteWorkflowRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteWorkflow operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.DeleteWorkflow
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteWorkflowAsync(DeleteWorkflowRequest deleteWorkflowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The process of detecting profile object type mapping by using given objects.
*
*
* @param detectProfileObjectTypeRequest
* @return A Java Future containing the result of the DetectProfileObjectType operation returned by the service.
* @sample AmazonCustomerProfilesAsync.DetectProfileObjectType
* @see AWS API Documentation
*/
java.util.concurrent.Future detectProfileObjectTypeAsync(DetectProfileObjectTypeRequest detectProfileObjectTypeRequest);
/**
*
* The process of detecting profile object type mapping by using given objects.
*
*
* @param detectProfileObjectTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DetectProfileObjectType operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.DetectProfileObjectType
* @see AWS API Documentation
*/
java.util.concurrent.Future detectProfileObjectTypeAsync(DetectProfileObjectTypeRequest detectProfileObjectTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Tests the auto-merging settings of your Identity Resolution Job without merging your data. It randomly selects a
* sample of matching groups from the existing matching results, and applies the automerging settings that you
* provided. You can then view the number of profiles in the sample, the number of matches, and the number of
* profiles identified to be merged. This enables you to evaluate the accuracy of the attributes in your matching
* list.
*
*
* You can't view which profiles are matched and would be merged.
*
*
*
* We strongly recommend you use this API to do a dry run of the automerging process before running the Identity
* Resolution Job. Include at least two matching attributes. If your matching list includes too few
* attributes (such as only FirstName
or only LastName
), there may be a large number of
* matches. This increases the chances of erroneous merges.
*
*
*
* @param getAutoMergingPreviewRequest
* @return A Java Future containing the result of the GetAutoMergingPreview operation returned by the service.
* @sample AmazonCustomerProfilesAsync.GetAutoMergingPreview
* @see AWS API Documentation
*/
java.util.concurrent.Future getAutoMergingPreviewAsync(GetAutoMergingPreviewRequest getAutoMergingPreviewRequest);
/**
*
* Tests the auto-merging settings of your Identity Resolution Job without merging your data. It randomly selects a
* sample of matching groups from the existing matching results, and applies the automerging settings that you
* provided. You can then view the number of profiles in the sample, the number of matches, and the number of
* profiles identified to be merged. This enables you to evaluate the accuracy of the attributes in your matching
* list.
*
*
* You can't view which profiles are matched and would be merged.
*
*
*
* We strongly recommend you use this API to do a dry run of the automerging process before running the Identity
* Resolution Job. Include at least two matching attributes. If your matching list includes too few
* attributes (such as only FirstName
or only LastName
), there may be a large number of
* matches. This increases the chances of erroneous merges.
*
*
*
* @param getAutoMergingPreviewRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAutoMergingPreview operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.GetAutoMergingPreview
* @see AWS API Documentation
*/
java.util.concurrent.Future getAutoMergingPreviewAsync(GetAutoMergingPreviewRequest getAutoMergingPreviewRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides more information on a calculated attribute definition for Customer Profiles.
*
*
* @param getCalculatedAttributeDefinitionRequest
* @return A Java Future containing the result of the GetCalculatedAttributeDefinition operation returned by the
* service.
* @sample AmazonCustomerProfilesAsync.GetCalculatedAttributeDefinition
* @see AWS API Documentation
*/
java.util.concurrent.Future getCalculatedAttributeDefinitionAsync(
GetCalculatedAttributeDefinitionRequest getCalculatedAttributeDefinitionRequest);
/**
*
* Provides more information on a calculated attribute definition for Customer Profiles.
*
*
* @param getCalculatedAttributeDefinitionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetCalculatedAttributeDefinition operation returned by the
* service.
* @sample AmazonCustomerProfilesAsyncHandler.GetCalculatedAttributeDefinition
* @see AWS API Documentation
*/
java.util.concurrent.Future getCalculatedAttributeDefinitionAsync(
GetCalculatedAttributeDefinitionRequest getCalculatedAttributeDefinitionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve a calculated attribute for a customer profile.
*
*
* @param getCalculatedAttributeForProfileRequest
* @return A Java Future containing the result of the GetCalculatedAttributeForProfile operation returned by the
* service.
* @sample AmazonCustomerProfilesAsync.GetCalculatedAttributeForProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future getCalculatedAttributeForProfileAsync(
GetCalculatedAttributeForProfileRequest getCalculatedAttributeForProfileRequest);
/**
*
* Retrieve a calculated attribute for a customer profile.
*
*
* @param getCalculatedAttributeForProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetCalculatedAttributeForProfile operation returned by the
* service.
* @sample AmazonCustomerProfilesAsyncHandler.GetCalculatedAttributeForProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future getCalculatedAttributeForProfileAsync(
GetCalculatedAttributeForProfileRequest getCalculatedAttributeForProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a specific domain.
*
*
* @param getDomainRequest
* @return A Java Future containing the result of the GetDomain operation returned by the service.
* @sample AmazonCustomerProfilesAsync.GetDomain
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getDomainAsync(GetDomainRequest getDomainRequest);
/**
*
* Returns information about a specific domain.
*
*
* @param getDomainRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDomain operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.GetDomain
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getDomainAsync(GetDomainRequest getDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the specified event stream in a specific domain.
*
*
* @param getEventStreamRequest
* @return A Java Future containing the result of the GetEventStream operation returned by the service.
* @sample AmazonCustomerProfilesAsync.GetEventStream
* @see AWS API Documentation
*/
java.util.concurrent.Future getEventStreamAsync(GetEventStreamRequest getEventStreamRequest);
/**
*
* Returns information about the specified event stream in a specific domain.
*
*
* @param getEventStreamRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEventStream operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.GetEventStream
* @see AWS API Documentation
*/
java.util.concurrent.Future getEventStreamAsync(GetEventStreamRequest getEventStreamRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about an Identity Resolution Job in a specific domain.
*
*
* Identity Resolution Jobs are set up using the Amazon Connect admin console. For more information, see Use Identity Resolution
* to consolidate similar profiles.
*
*
* @param getIdentityResolutionJobRequest
* @return A Java Future containing the result of the GetIdentityResolutionJob operation returned by the service.
* @sample AmazonCustomerProfilesAsync.GetIdentityResolutionJob
* @see AWS API Documentation
*/
java.util.concurrent.Future getIdentityResolutionJobAsync(GetIdentityResolutionJobRequest getIdentityResolutionJobRequest);
/**
*
* Returns information about an Identity Resolution Job in a specific domain.
*
*
* Identity Resolution Jobs are set up using the Amazon Connect admin console. For more information, see Use Identity Resolution
* to consolidate similar profiles.
*
*
* @param getIdentityResolutionJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetIdentityResolutionJob operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.GetIdentityResolutionJob
* @see AWS API Documentation
*/
java.util.concurrent.Future getIdentityResolutionJobAsync(GetIdentityResolutionJobRequest getIdentityResolutionJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns an integration for a domain.
*
*
* @param getIntegrationRequest
* @return A Java Future containing the result of the GetIntegration operation returned by the service.
* @sample AmazonCustomerProfilesAsync.GetIntegration
* @see AWS API Documentation
*/
java.util.concurrent.Future getIntegrationAsync(GetIntegrationRequest getIntegrationRequest);
/**
*
* Returns an integration for a domain.
*
*
* @param getIntegrationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetIntegration operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.GetIntegration
* @see AWS API Documentation
*/
java.util.concurrent.Future getIntegrationAsync(GetIntegrationRequest getIntegrationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Before calling this API, use CreateDomain or
* UpdateDomain
* to enable identity resolution: set Matching
to true.
*
*
* GetMatches returns potentially matching profiles, based on the results of the latest run of a machine learning
* process.
*
*
*
* The process of matching duplicate profiles. If Matching
= true
, Amazon Connect Customer
* Profiles starts a weekly batch process called Identity Resolution Job. If you do not specify a date and time for
* Identity Resolution Job to run, by default it runs every Saturday at 12AM UTC to detect duplicate profiles in
* your domains.
*
*
* After the Identity Resolution Job completes, use the GetMatches API to
* return and review the results. Or, if you have configured ExportingConfig
in the
* MatchingRequest
, you can download the results from S3.
*
*
*
* Amazon Connect uses the following profile attributes to identify matches:
*
*
* -
*
* PhoneNumber
*
*
* -
*
* HomePhoneNumber
*
*
* -
*
* BusinessPhoneNumber
*
*
* -
*
* MobilePhoneNumber
*
*
* -
*
* EmailAddress
*
*
* -
*
* PersonalEmailAddress
*
*
* -
*
* BusinessEmailAddress
*
*
* -
*
* FullName
*
*
*
*
* For example, two or more profiles—with spelling mistakes such as John Doe and Jhn Doe, or different
* casing email addresses such as [email protected] and [email protected], or different
* phone number formats such as 555-010-0000 and +1-555-010-0000—can be detected as belonging to the
* same customer John Doe and merged into a unified profile.
*
*
* @param getMatchesRequest
* @return A Java Future containing the result of the GetMatches operation returned by the service.
* @sample AmazonCustomerProfilesAsync.GetMatches
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getMatchesAsync(GetMatchesRequest getMatchesRequest);
/**
*
* Before calling this API, use CreateDomain or
* UpdateDomain
* to enable identity resolution: set Matching
to true.
*
*
* GetMatches returns potentially matching profiles, based on the results of the latest run of a machine learning
* process.
*
*
*
* The process of matching duplicate profiles. If Matching
= true
, Amazon Connect Customer
* Profiles starts a weekly batch process called Identity Resolution Job. If you do not specify a date and time for
* Identity Resolution Job to run, by default it runs every Saturday at 12AM UTC to detect duplicate profiles in
* your domains.
*
*
* After the Identity Resolution Job completes, use the GetMatches API to
* return and review the results. Or, if you have configured ExportingConfig
in the
* MatchingRequest
, you can download the results from S3.
*
*
*
* Amazon Connect uses the following profile attributes to identify matches:
*
*
* -
*
* PhoneNumber
*
*
* -
*
* HomePhoneNumber
*
*
* -
*
* BusinessPhoneNumber
*
*
* -
*
* MobilePhoneNumber
*
*
* -
*
* EmailAddress
*
*
* -
*
* PersonalEmailAddress
*
*
* -
*
* BusinessEmailAddress
*
*
* -
*
* FullName
*
*
*
*
* For example, two or more profiles—with spelling mistakes such as John Doe and Jhn Doe, or different
* casing email addresses such as [email protected] and [email protected], or different
* phone number formats such as 555-010-0000 and +1-555-010-0000—can be detected as belonging to the
* same customer John Doe and merged into a unified profile.
*
*
* @param getMatchesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMatches operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.GetMatches
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getMatchesAsync(GetMatchesRequest getMatchesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the object types for a specific domain.
*
*
* @param getProfileObjectTypeRequest
* @return A Java Future containing the result of the GetProfileObjectType operation returned by the service.
* @sample AmazonCustomerProfilesAsync.GetProfileObjectType
* @see AWS API Documentation
*/
java.util.concurrent.Future getProfileObjectTypeAsync(GetProfileObjectTypeRequest getProfileObjectTypeRequest);
/**
*
* Returns the object types for a specific domain.
*
*
* @param getProfileObjectTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetProfileObjectType operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.GetProfileObjectType
* @see AWS API Documentation
*/
java.util.concurrent.Future getProfileObjectTypeAsync(GetProfileObjectTypeRequest getProfileObjectTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the template information for a specific object type.
*
*
* A template is a predefined ProfileObjectType, such as “Salesforce-Account” or “Salesforce-Contact.” When a user
* sends a ProfileObject, using the PutProfileObject API, with an ObjectTypeName that matches one of the
* TemplateIds, it uses the mappings from the template.
*
*
* @param getProfileObjectTypeTemplateRequest
* @return A Java Future containing the result of the GetProfileObjectTypeTemplate operation returned by the
* service.
* @sample AmazonCustomerProfilesAsync.GetProfileObjectTypeTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future getProfileObjectTypeTemplateAsync(
GetProfileObjectTypeTemplateRequest getProfileObjectTypeTemplateRequest);
/**
*
* Returns the template information for a specific object type.
*
*
* A template is a predefined ProfileObjectType, such as “Salesforce-Account” or “Salesforce-Contact.” When a user
* sends a ProfileObject, using the PutProfileObject API, with an ObjectTypeName that matches one of the
* TemplateIds, it uses the mappings from the template.
*
*
* @param getProfileObjectTypeTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetProfileObjectTypeTemplate operation returned by the
* service.
* @sample AmazonCustomerProfilesAsyncHandler.GetProfileObjectTypeTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future getProfileObjectTypeTemplateAsync(
GetProfileObjectTypeTemplateRequest getProfileObjectTypeTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a set of profiles that belong to the same matching group using the matchId
or
* profileId
. You can also specify the type of matching that you want for finding similar profiles
* using either RULE_BASED_MATCHING
or ML_BASED_MATCHING
.
*
*
* @param getSimilarProfilesRequest
* @return A Java Future containing the result of the GetSimilarProfiles operation returned by the service.
* @sample AmazonCustomerProfilesAsync.GetSimilarProfiles
* @see AWS API Documentation
*/
java.util.concurrent.Future getSimilarProfilesAsync(GetSimilarProfilesRequest getSimilarProfilesRequest);
/**
*
* Returns a set of profiles that belong to the same matching group using the matchId
or
* profileId
. You can also specify the type of matching that you want for finding similar profiles
* using either RULE_BASED_MATCHING
or ML_BASED_MATCHING
.
*
*
* @param getSimilarProfilesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSimilarProfiles operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.GetSimilarProfiles
* @see AWS API Documentation
*/
java.util.concurrent.Future getSimilarProfilesAsync(GetSimilarProfilesRequest getSimilarProfilesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get details of specified workflow.
*
*
* @param getWorkflowRequest
* @return A Java Future containing the result of the GetWorkflow operation returned by the service.
* @sample AmazonCustomerProfilesAsync.GetWorkflow
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getWorkflowAsync(GetWorkflowRequest getWorkflowRequest);
/**
*
* Get details of specified workflow.
*
*
* @param getWorkflowRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetWorkflow operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.GetWorkflow
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getWorkflowAsync(GetWorkflowRequest getWorkflowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get granular list of steps in workflow.
*
*
* @param getWorkflowStepsRequest
* @return A Java Future containing the result of the GetWorkflowSteps operation returned by the service.
* @sample AmazonCustomerProfilesAsync.GetWorkflowSteps
* @see AWS API Documentation
*/
java.util.concurrent.Future getWorkflowStepsAsync(GetWorkflowStepsRequest getWorkflowStepsRequest);
/**
*
* Get granular list of steps in workflow.
*
*
* @param getWorkflowStepsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetWorkflowSteps operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.GetWorkflowSteps
* @see AWS API Documentation
*/
java.util.concurrent.Future getWorkflowStepsAsync(GetWorkflowStepsRequest getWorkflowStepsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all of the integrations associated to a specific URI in the AWS account.
*
*
* @param listAccountIntegrationsRequest
* @return A Java Future containing the result of the ListAccountIntegrations operation returned by the service.
* @sample AmazonCustomerProfilesAsync.ListAccountIntegrations
* @see AWS API Documentation
*/
java.util.concurrent.Future listAccountIntegrationsAsync(ListAccountIntegrationsRequest listAccountIntegrationsRequest);
/**
*
* Lists all of the integrations associated to a specific URI in the AWS account.
*
*
* @param listAccountIntegrationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAccountIntegrations operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.ListAccountIntegrations
* @see AWS API Documentation
*/
java.util.concurrent.Future listAccountIntegrationsAsync(ListAccountIntegrationsRequest listAccountIntegrationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists calculated attribute definitions for Customer Profiles
*
*
* @param listCalculatedAttributeDefinitionsRequest
* @return A Java Future containing the result of the ListCalculatedAttributeDefinitions operation returned by the
* service.
* @sample AmazonCustomerProfilesAsync.ListCalculatedAttributeDefinitions
* @see AWS API Documentation
*/
java.util.concurrent.Future listCalculatedAttributeDefinitionsAsync(
ListCalculatedAttributeDefinitionsRequest listCalculatedAttributeDefinitionsRequest);
/**
*
* Lists calculated attribute definitions for Customer Profiles
*
*
* @param listCalculatedAttributeDefinitionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListCalculatedAttributeDefinitions operation returned by the
* service.
* @sample AmazonCustomerProfilesAsyncHandler.ListCalculatedAttributeDefinitions
* @see AWS API Documentation
*/
java.util.concurrent.Future listCalculatedAttributeDefinitionsAsync(
ListCalculatedAttributeDefinitionsRequest listCalculatedAttributeDefinitionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve a list of calculated attributes for a customer profile.
*
*
* @param listCalculatedAttributesForProfileRequest
* @return A Java Future containing the result of the ListCalculatedAttributesForProfile operation returned by the
* service.
* @sample AmazonCustomerProfilesAsync.ListCalculatedAttributesForProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future listCalculatedAttributesForProfileAsync(
ListCalculatedAttributesForProfileRequest listCalculatedAttributesForProfileRequest);
/**
*
* Retrieve a list of calculated attributes for a customer profile.
*
*
* @param listCalculatedAttributesForProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListCalculatedAttributesForProfile operation returned by the
* service.
* @sample AmazonCustomerProfilesAsyncHandler.ListCalculatedAttributesForProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future listCalculatedAttributesForProfileAsync(
ListCalculatedAttributesForProfileRequest listCalculatedAttributesForProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all the domains for an AWS account that have been created.
*
*
* @param listDomainsRequest
* @return A Java Future containing the result of the ListDomains operation returned by the service.
* @sample AmazonCustomerProfilesAsync.ListDomains
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDomainsAsync(ListDomainsRequest listDomainsRequest);
/**
*
* Returns a list of all the domains for an AWS account that have been created.
*
*
* @param listDomainsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDomains operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.ListDomains
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDomainsAsync(ListDomainsRequest listDomainsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all the event streams in a specific domain.
*
*
* @param listEventStreamsRequest
* @return A Java Future containing the result of the ListEventStreams operation returned by the service.
* @sample AmazonCustomerProfilesAsync.ListEventStreams
* @see AWS API Documentation
*/
java.util.concurrent.Future listEventStreamsAsync(ListEventStreamsRequest listEventStreamsRequest);
/**
*
* Returns a list of all the event streams in a specific domain.
*
*
* @param listEventStreamsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListEventStreams operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.ListEventStreams
* @see AWS API Documentation
*/
java.util.concurrent.Future listEventStreamsAsync(ListEventStreamsRequest listEventStreamsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all of the Identity Resolution Jobs in your domain. The response sorts the list by
* JobStartTime
.
*
*
* @param listIdentityResolutionJobsRequest
* @return A Java Future containing the result of the ListIdentityResolutionJobs operation returned by the service.
* @sample AmazonCustomerProfilesAsync.ListIdentityResolutionJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listIdentityResolutionJobsAsync(
ListIdentityResolutionJobsRequest listIdentityResolutionJobsRequest);
/**
*
* Lists all of the Identity Resolution Jobs in your domain. The response sorts the list by
* JobStartTime
.
*
*
* @param listIdentityResolutionJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListIdentityResolutionJobs operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.ListIdentityResolutionJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listIdentityResolutionJobsAsync(
ListIdentityResolutionJobsRequest listIdentityResolutionJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all of the integrations in your domain.
*
*
* @param listIntegrationsRequest
* @return A Java Future containing the result of the ListIntegrations operation returned by the service.
* @sample AmazonCustomerProfilesAsync.ListIntegrations
* @see AWS API Documentation
*/
java.util.concurrent.Future listIntegrationsAsync(ListIntegrationsRequest listIntegrationsRequest);
/**
*
* Lists all of the integrations in your domain.
*
*
* @param listIntegrationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListIntegrations operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.ListIntegrations
* @see AWS API Documentation
*/
java.util.concurrent.Future listIntegrationsAsync(ListIntegrationsRequest listIntegrationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all of the template information for object types.
*
*
* @param listProfileObjectTypeTemplatesRequest
* @return A Java Future containing the result of the ListProfileObjectTypeTemplates operation returned by the
* service.
* @sample AmazonCustomerProfilesAsync.ListProfileObjectTypeTemplates
* @see AWS API Documentation
*/
java.util.concurrent.Future listProfileObjectTypeTemplatesAsync(
ListProfileObjectTypeTemplatesRequest listProfileObjectTypeTemplatesRequest);
/**
*
* Lists all of the template information for object types.
*
*
* @param listProfileObjectTypeTemplatesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListProfileObjectTypeTemplates operation returned by the
* service.
* @sample AmazonCustomerProfilesAsyncHandler.ListProfileObjectTypeTemplates
* @see AWS API Documentation
*/
java.util.concurrent.Future listProfileObjectTypeTemplatesAsync(
ListProfileObjectTypeTemplatesRequest listProfileObjectTypeTemplatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all of the templates available within the service.
*
*
* @param listProfileObjectTypesRequest
* @return A Java Future containing the result of the ListProfileObjectTypes operation returned by the service.
* @sample AmazonCustomerProfilesAsync.ListProfileObjectTypes
* @see AWS API Documentation
*/
java.util.concurrent.Future listProfileObjectTypesAsync(ListProfileObjectTypesRequest listProfileObjectTypesRequest);
/**
*
* Lists all of the templates available within the service.
*
*
* @param listProfileObjectTypesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListProfileObjectTypes operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.ListProfileObjectTypes
* @see AWS API Documentation
*/
java.util.concurrent.Future listProfileObjectTypesAsync(ListProfileObjectTypesRequest listProfileObjectTypesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of objects associated with a profile of a given ProfileObjectType.
*
*
* @param listProfileObjectsRequest
* @return A Java Future containing the result of the ListProfileObjects operation returned by the service.
* @sample AmazonCustomerProfilesAsync.ListProfileObjects
* @see AWS API Documentation
*/
java.util.concurrent.Future listProfileObjectsAsync(ListProfileObjectsRequest listProfileObjectsRequest);
/**
*
* Returns a list of objects associated with a profile of a given ProfileObjectType.
*
*
* @param listProfileObjectsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListProfileObjects operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.ListProfileObjects
* @see AWS API Documentation
*/
java.util.concurrent.Future listProfileObjectsAsync(ListProfileObjectsRequest listProfileObjectsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a set of MatchIds
that belong to the given domain.
*
*
* @param listRuleBasedMatchesRequest
* @return A Java Future containing the result of the ListRuleBasedMatches operation returned by the service.
* @sample AmazonCustomerProfilesAsync.ListRuleBasedMatches
* @see AWS API Documentation
*/
java.util.concurrent.Future listRuleBasedMatchesAsync(ListRuleBasedMatchesRequest listRuleBasedMatchesRequest);
/**
*
* Returns a set of MatchIds
that belong to the given domain.
*
*
* @param listRuleBasedMatchesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRuleBasedMatches operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.ListRuleBasedMatches
* @see AWS API Documentation
*/
java.util.concurrent.Future listRuleBasedMatchesAsync(ListRuleBasedMatchesRequest listRuleBasedMatchesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Displays the tags associated with an Amazon Connect Customer Profiles resource. In Connect Customer Profiles,
* domains, profile object types, and integrations can be tagged.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonCustomerProfilesAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Displays the tags associated with an Amazon Connect Customer Profiles resource. In Connect Customer Profiles,
* domains, profile object types, and integrations can be tagged.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Query to list all workflows.
*
*
* @param listWorkflowsRequest
* @return A Java Future containing the result of the ListWorkflows operation returned by the service.
* @sample AmazonCustomerProfilesAsync.ListWorkflows
* @see AWS API Documentation
*/
java.util.concurrent.Future listWorkflowsAsync(ListWorkflowsRequest listWorkflowsRequest);
/**
*
* Query to list all workflows.
*
*
* @param listWorkflowsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListWorkflows operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.ListWorkflows
* @see AWS API Documentation
*/
java.util.concurrent.Future listWorkflowsAsync(ListWorkflowsRequest listWorkflowsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Runs an AWS Lambda job that does the following:
*
*
* -
*
* All the profileKeys in the ProfileToBeMerged
will be moved to the main profile.
*
*
* -
*
* All the objects in the ProfileToBeMerged
will be moved to the main profile.
*
*
* -
*
* All the ProfileToBeMerged
will be deleted at the end.
*
*
* -
*
* All the profileKeys in the ProfileIdsToBeMerged
will be moved to the main profile.
*
*
* -
*
* Standard fields are merged as follows:
*
*
* -
*
* Fields are always "union"-ed if there are no conflicts in standard fields or attributeKeys.
*
*
* -
*
* When there are conflicting fields:
*
*
* -
*
* If no SourceProfileIds
entry is specified, the main Profile value is always taken.
*
*
* -
*
* If a SourceProfileIds
entry is specified, the specified profileId is always taken, even if it is a
* NULL value.
*
*
*
*
*
*
*
*
* You can use MergeProfiles together with GetMatches, which
* returns potentially matching profiles, or use it with the results of another matching system. After profiles have
* been merged, they cannot be separated (unmerged).
*
*
* @param mergeProfilesRequest
* @return A Java Future containing the result of the MergeProfiles operation returned by the service.
* @sample AmazonCustomerProfilesAsync.MergeProfiles
* @see AWS API Documentation
*/
java.util.concurrent.Future mergeProfilesAsync(MergeProfilesRequest mergeProfilesRequest);
/**
*
* Runs an AWS Lambda job that does the following:
*
*
* -
*
* All the profileKeys in the ProfileToBeMerged
will be moved to the main profile.
*
*
* -
*
* All the objects in the ProfileToBeMerged
will be moved to the main profile.
*
*
* -
*
* All the ProfileToBeMerged
will be deleted at the end.
*
*
* -
*
* All the profileKeys in the ProfileIdsToBeMerged
will be moved to the main profile.
*
*
* -
*
* Standard fields are merged as follows:
*
*
* -
*
* Fields are always "union"-ed if there are no conflicts in standard fields or attributeKeys.
*
*
* -
*
* When there are conflicting fields:
*
*
* -
*
* If no SourceProfileIds
entry is specified, the main Profile value is always taken.
*
*
* -
*
* If a SourceProfileIds
entry is specified, the specified profileId is always taken, even if it is a
* NULL value.
*
*
*
*
*
*
*
*
* You can use MergeProfiles together with GetMatches, which
* returns potentially matching profiles, or use it with the results of another matching system. After profiles have
* been merged, they cannot be separated (unmerged).
*
*
* @param mergeProfilesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the MergeProfiles operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.MergeProfiles
* @see AWS API Documentation
*/
java.util.concurrent.Future mergeProfilesAsync(MergeProfilesRequest mergeProfilesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds an integration between the service and a third-party service, which includes Amazon AppFlow and Amazon
* Connect.
*
*
* An integration can belong to only one domain.
*
*
* To add or remove tags on an existing Integration, see TagResource /
* UntagResource.
*
*
* @param putIntegrationRequest
* @return A Java Future containing the result of the PutIntegration operation returned by the service.
* @sample AmazonCustomerProfilesAsync.PutIntegration
* @see AWS API Documentation
*/
java.util.concurrent.Future putIntegrationAsync(PutIntegrationRequest putIntegrationRequest);
/**
*
* Adds an integration between the service and a third-party service, which includes Amazon AppFlow and Amazon
* Connect.
*
*
* An integration can belong to only one domain.
*
*
* To add or remove tags on an existing Integration, see TagResource /
* UntagResource.
*
*
* @param putIntegrationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutIntegration operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.PutIntegration
* @see AWS API Documentation
*/
java.util.concurrent.Future putIntegrationAsync(PutIntegrationRequest putIntegrationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds additional objects to customer profiles of a given ObjectType.
*
*
* When adding a specific profile object, like a Contact Record, an inferred profile can get created if it is not
* mapped to an existing profile. The resulting profile will only have a phone number populated in the standard
* ProfileObject. Any additional Contact Records with the same phone number will be mapped to the same inferred
* profile.
*
*
* When a ProfileObject is created and if a ProfileObjectType already exists for the ProfileObject, it will provide
* data to a standard profile depending on the ProfileObjectType definition.
*
*
* PutProfileObject needs an ObjectType, which can be created using PutProfileObjectType.
*
*
* @param putProfileObjectRequest
* @return A Java Future containing the result of the PutProfileObject operation returned by the service.
* @sample AmazonCustomerProfilesAsync.PutProfileObject
* @see AWS API Documentation
*/
java.util.concurrent.Future putProfileObjectAsync(PutProfileObjectRequest putProfileObjectRequest);
/**
*
* Adds additional objects to customer profiles of a given ObjectType.
*
*
* When adding a specific profile object, like a Contact Record, an inferred profile can get created if it is not
* mapped to an existing profile. The resulting profile will only have a phone number populated in the standard
* ProfileObject. Any additional Contact Records with the same phone number will be mapped to the same inferred
* profile.
*
*
* When a ProfileObject is created and if a ProfileObjectType already exists for the ProfileObject, it will provide
* data to a standard profile depending on the ProfileObjectType definition.
*
*
* PutProfileObject needs an ObjectType, which can be created using PutProfileObjectType.
*
*
* @param putProfileObjectRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutProfileObject operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.PutProfileObject
* @see AWS API Documentation
*/
java.util.concurrent.Future putProfileObjectAsync(PutProfileObjectRequest putProfileObjectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Defines a ProfileObjectType.
*
*
* To add or remove tags on an existing ObjectType, see TagResource/UntagResource.
*
*
* @param putProfileObjectTypeRequest
* @return A Java Future containing the result of the PutProfileObjectType operation returned by the service.
* @sample AmazonCustomerProfilesAsync.PutProfileObjectType
* @see AWS API Documentation
*/
java.util.concurrent.Future putProfileObjectTypeAsync(PutProfileObjectTypeRequest putProfileObjectTypeRequest);
/**
*
* Defines a ProfileObjectType.
*
*
* To add or remove tags on an existing ObjectType, see TagResource/UntagResource.
*
*
* @param putProfileObjectTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutProfileObjectType operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.PutProfileObjectType
* @see AWS API Documentation
*/
java.util.concurrent.Future putProfileObjectTypeAsync(PutProfileObjectTypeRequest putProfileObjectTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Searches for profiles within a specific domain using one or more predefined search keys (e.g., _fullName, _phone,
* _email, _account, etc.) and/or custom-defined search keys. A search key is a data type pair that consists of a
* KeyName
and Values
list.
*
*
* This operation supports searching for profiles with a minimum of 1 key-value(s) pair and up to 5 key-value(s)
* pairs using either AND
or OR
logic.
*
*
* @param searchProfilesRequest
* @return A Java Future containing the result of the SearchProfiles operation returned by the service.
* @sample AmazonCustomerProfilesAsync.SearchProfiles
* @see AWS API Documentation
*/
java.util.concurrent.Future searchProfilesAsync(SearchProfilesRequest searchProfilesRequest);
/**
*
* Searches for profiles within a specific domain using one or more predefined search keys (e.g., _fullName, _phone,
* _email, _account, etc.) and/or custom-defined search keys. A search key is a data type pair that consists of a
* KeyName
and Values
list.
*
*
* This operation supports searching for profiles with a minimum of 1 key-value(s) pair and up to 5 key-value(s)
* pairs using either AND
or OR
logic.
*
*
* @param searchProfilesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchProfiles operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.SearchProfiles
* @see AWS API Documentation
*/
java.util.concurrent.Future searchProfilesAsync(SearchProfilesRequest searchProfilesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns one or more tags (key-value pairs) to the specified Amazon Connect Customer Profiles resource. Tags can
* help you organize and categorize your resources. You can also use them to scope user permissions by granting a
* user permission to access or change only resources with certain tag values. In Connect Customer Profiles,
* domains, profile object types, and integrations can be tagged.
*
*
* Tags don't have any semantic meaning to AWS and are interpreted strictly as strings of characters.
*
*
* You can use the TagResource action with a resource that already has tags. If you specify a new tag key, this tag
* is appended to the list of tags associated with the resource. If you specify a tag key that is already associated
* with the resource, the new tag value that you specify replaces the previous value for that tag.
*
*
* You can associate as many as 50 tags with a resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonCustomerProfilesAsync.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Assigns one or more tags (key-value pairs) to the specified Amazon Connect Customer Profiles resource. Tags can
* help you organize and categorize your resources. You can also use them to scope user permissions by granting a
* user permission to access or change only resources with certain tag values. In Connect Customer Profiles,
* domains, profile object types, and integrations can be tagged.
*
*
* Tags don't have any semantic meaning to AWS and are interpreted strictly as strings of characters.
*
*
* You can use the TagResource action with a resource that already has tags. If you specify a new tag key, this tag
* is appended to the list of tags associated with the resource. If you specify a tag key that is already associated
* with the resource, the new tag value that you specify replaces the previous value for that tag.
*
*
* You can associate as many as 50 tags with a resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes one or more tags from the specified Amazon Connect Customer Profiles resource. In Connect Customer
* Profiles, domains, profile object types, and integrations can be tagged.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonCustomerProfilesAsync.UntagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes one or more tags from the specified Amazon Connect Customer Profiles resource. In Connect Customer
* Profiles, domains, profile object types, and integrations can be tagged.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.UntagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing calculated attribute definition. When updating the Conditions, note that increasing the date
* range of a calculated attribute will not trigger inclusion of historical data greater than the current date
* range.
*
*
* @param updateCalculatedAttributeDefinitionRequest
* @return A Java Future containing the result of the UpdateCalculatedAttributeDefinition operation returned by the
* service.
* @sample AmazonCustomerProfilesAsync.UpdateCalculatedAttributeDefinition
* @see AWS API Documentation
*/
java.util.concurrent.Future updateCalculatedAttributeDefinitionAsync(
UpdateCalculatedAttributeDefinitionRequest updateCalculatedAttributeDefinitionRequest);
/**
*
* Updates an existing calculated attribute definition. When updating the Conditions, note that increasing the date
* range of a calculated attribute will not trigger inclusion of historical data greater than the current date
* range.
*
*
* @param updateCalculatedAttributeDefinitionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateCalculatedAttributeDefinition operation returned by the
* service.
* @sample AmazonCustomerProfilesAsyncHandler.UpdateCalculatedAttributeDefinition
* @see AWS API Documentation
*/
java.util.concurrent.Future updateCalculatedAttributeDefinitionAsync(
UpdateCalculatedAttributeDefinitionRequest updateCalculatedAttributeDefinitionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the properties of a domain, including creating or selecting a dead letter queue or an encryption key.
*
*
* After a domain is created, the name can’t be changed.
*
*
* Use this API or CreateDomain to
* enable identity
* resolution: set Matching
to true.
*
*
* To prevent cross-service impersonation when you call this API, see Cross-service confused deputy prevention for sample policies that you should apply.
*
*
* To add or remove tags on an existing Domain, see TagResource/UntagResource.
*
*
* @param updateDomainRequest
* @return A Java Future containing the result of the UpdateDomain operation returned by the service.
* @sample AmazonCustomerProfilesAsync.UpdateDomain
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateDomainAsync(UpdateDomainRequest updateDomainRequest);
/**
*
* Updates the properties of a domain, including creating or selecting a dead letter queue or an encryption key.
*
*
* After a domain is created, the name can’t be changed.
*
*
* Use this API or CreateDomain to
* enable identity
* resolution: set Matching
to true.
*
*
* To prevent cross-service impersonation when you call this API, see Cross-service confused deputy prevention for sample policies that you should apply.
*
*
* To add or remove tags on an existing Domain, see TagResource/UntagResource.
*
*
* @param updateDomainRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDomain operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.UpdateDomain
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateDomainAsync(UpdateDomainRequest updateDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the properties of a profile. The ProfileId is required for updating a customer profile.
*
*
* When calling the UpdateProfile API, specifying an empty string value means that any existing value will be
* removed. Not specifying a string value means that any value already there will be kept.
*
*
* @param updateProfileRequest
* @return A Java Future containing the result of the UpdateProfile operation returned by the service.
* @sample AmazonCustomerProfilesAsync.UpdateProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future updateProfileAsync(UpdateProfileRequest updateProfileRequest);
/**
*
* Updates the properties of a profile. The ProfileId is required for updating a customer profile.
*
*
* When calling the UpdateProfile API, specifying an empty string value means that any existing value will be
* removed. Not specifying a string value means that any value already there will be kept.
*
*
* @param updateProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateProfile operation returned by the service.
* @sample AmazonCustomerProfilesAsyncHandler.UpdateProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future updateProfileAsync(UpdateProfileRequest updateProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}