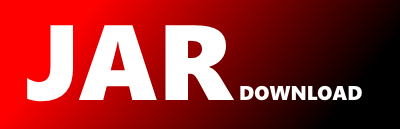
com.amazonaws.services.customerprofiles.model.CreateProfileRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-customerprofiles Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.customerprofiles.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateProfileRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The unique name of the domain.
*
*/
private String domainName;
/**
*
* An account number that you have given to the customer.
*
*/
private String accountNumber;
/**
*
* Any additional information relevant to the customer’s profile.
*
*/
private String additionalInformation;
/**
*
* The type of profile used to describe the customer.
*
*/
private String partyType;
/**
*
* The name of the customer’s business.
*
*/
private String businessName;
/**
*
* The customer’s first name.
*
*/
private String firstName;
/**
*
* The customer’s middle name.
*
*/
private String middleName;
/**
*
* The customer’s last name.
*
*/
private String lastName;
/**
*
* The customer’s birth date.
*
*/
private String birthDate;
/**
*
* The gender with which the customer identifies.
*
*/
private String gender;
/**
*
* The customer’s phone number, which has not been specified as a mobile, home, or business number.
*
*/
private String phoneNumber;
/**
*
* The customer’s mobile phone number.
*
*/
private String mobilePhoneNumber;
/**
*
* The customer’s home phone number.
*
*/
private String homePhoneNumber;
/**
*
* The customer’s business phone number.
*
*/
private String businessPhoneNumber;
/**
*
* The customer’s email address, which has not been specified as a personal or business address.
*
*/
private String emailAddress;
/**
*
* The customer’s personal email address.
*
*/
private String personalEmailAddress;
/**
*
* The customer’s business email address.
*
*/
private String businessEmailAddress;
/**
*
* A generic address associated with the customer that is not mailing, shipping, or billing.
*
*/
private Address address;
/**
*
* The customer’s shipping address.
*
*/
private Address shippingAddress;
/**
*
* The customer’s mailing address.
*
*/
private Address mailingAddress;
/**
*
* The customer’s billing address.
*
*/
private Address billingAddress;
/**
*
* A key value pair of attributes of a customer profile.
*
*/
private java.util.Map attributes;
/**
*
* An alternative to PartyType
which accepts any string as input.
*
*/
private String partyTypeString;
/**
*
* An alternative to Gender
which accepts any string as input.
*
*/
private String genderString;
/**
*
* The unique name of the domain.
*
*
* @param domainName
* The unique name of the domain.
*/
public void setDomainName(String domainName) {
this.domainName = domainName;
}
/**
*
* The unique name of the domain.
*
*
* @return The unique name of the domain.
*/
public String getDomainName() {
return this.domainName;
}
/**
*
* The unique name of the domain.
*
*
* @param domainName
* The unique name of the domain.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest withDomainName(String domainName) {
setDomainName(domainName);
return this;
}
/**
*
* An account number that you have given to the customer.
*
*
* @param accountNumber
* An account number that you have given to the customer.
*/
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
/**
*
* An account number that you have given to the customer.
*
*
* @return An account number that you have given to the customer.
*/
public String getAccountNumber() {
return this.accountNumber;
}
/**
*
* An account number that you have given to the customer.
*
*
* @param accountNumber
* An account number that you have given to the customer.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest withAccountNumber(String accountNumber) {
setAccountNumber(accountNumber);
return this;
}
/**
*
* Any additional information relevant to the customer’s profile.
*
*
* @param additionalInformation
* Any additional information relevant to the customer’s profile.
*/
public void setAdditionalInformation(String additionalInformation) {
this.additionalInformation = additionalInformation;
}
/**
*
* Any additional information relevant to the customer’s profile.
*
*
* @return Any additional information relevant to the customer’s profile.
*/
public String getAdditionalInformation() {
return this.additionalInformation;
}
/**
*
* Any additional information relevant to the customer’s profile.
*
*
* @param additionalInformation
* Any additional information relevant to the customer’s profile.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest withAdditionalInformation(String additionalInformation) {
setAdditionalInformation(additionalInformation);
return this;
}
/**
*
* The type of profile used to describe the customer.
*
*
* @param partyType
* The type of profile used to describe the customer.
* @see PartyType
*/
@Deprecated
public void setPartyType(String partyType) {
this.partyType = partyType;
}
/**
*
* The type of profile used to describe the customer.
*
*
* @return The type of profile used to describe the customer.
* @see PartyType
*/
@Deprecated
public String getPartyType() {
return this.partyType;
}
/**
*
* The type of profile used to describe the customer.
*
*
* @param partyType
* The type of profile used to describe the customer.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PartyType
*/
@Deprecated
public CreateProfileRequest withPartyType(String partyType) {
setPartyType(partyType);
return this;
}
/**
*
* The type of profile used to describe the customer.
*
*
* @param partyType
* The type of profile used to describe the customer.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PartyType
*/
@Deprecated
public CreateProfileRequest withPartyType(PartyType partyType) {
this.partyType = partyType.toString();
return this;
}
/**
*
* The name of the customer’s business.
*
*
* @param businessName
* The name of the customer’s business.
*/
public void setBusinessName(String businessName) {
this.businessName = businessName;
}
/**
*
* The name of the customer’s business.
*
*
* @return The name of the customer’s business.
*/
public String getBusinessName() {
return this.businessName;
}
/**
*
* The name of the customer’s business.
*
*
* @param businessName
* The name of the customer’s business.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest withBusinessName(String businessName) {
setBusinessName(businessName);
return this;
}
/**
*
* The customer’s first name.
*
*
* @param firstName
* The customer’s first name.
*/
public void setFirstName(String firstName) {
this.firstName = firstName;
}
/**
*
* The customer’s first name.
*
*
* @return The customer’s first name.
*/
public String getFirstName() {
return this.firstName;
}
/**
*
* The customer’s first name.
*
*
* @param firstName
* The customer’s first name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest withFirstName(String firstName) {
setFirstName(firstName);
return this;
}
/**
*
* The customer’s middle name.
*
*
* @param middleName
* The customer’s middle name.
*/
public void setMiddleName(String middleName) {
this.middleName = middleName;
}
/**
*
* The customer’s middle name.
*
*
* @return The customer’s middle name.
*/
public String getMiddleName() {
return this.middleName;
}
/**
*
* The customer’s middle name.
*
*
* @param middleName
* The customer’s middle name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest withMiddleName(String middleName) {
setMiddleName(middleName);
return this;
}
/**
*
* The customer’s last name.
*
*
* @param lastName
* The customer’s last name.
*/
public void setLastName(String lastName) {
this.lastName = lastName;
}
/**
*
* The customer’s last name.
*
*
* @return The customer’s last name.
*/
public String getLastName() {
return this.lastName;
}
/**
*
* The customer’s last name.
*
*
* @param lastName
* The customer’s last name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest withLastName(String lastName) {
setLastName(lastName);
return this;
}
/**
*
* The customer’s birth date.
*
*
* @param birthDate
* The customer’s birth date.
*/
public void setBirthDate(String birthDate) {
this.birthDate = birthDate;
}
/**
*
* The customer’s birth date.
*
*
* @return The customer’s birth date.
*/
public String getBirthDate() {
return this.birthDate;
}
/**
*
* The customer’s birth date.
*
*
* @param birthDate
* The customer’s birth date.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest withBirthDate(String birthDate) {
setBirthDate(birthDate);
return this;
}
/**
*
* The gender with which the customer identifies.
*
*
* @param gender
* The gender with which the customer identifies.
* @see Gender
*/
@Deprecated
public void setGender(String gender) {
this.gender = gender;
}
/**
*
* The gender with which the customer identifies.
*
*
* @return The gender with which the customer identifies.
* @see Gender
*/
@Deprecated
public String getGender() {
return this.gender;
}
/**
*
* The gender with which the customer identifies.
*
*
* @param gender
* The gender with which the customer identifies.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Gender
*/
@Deprecated
public CreateProfileRequest withGender(String gender) {
setGender(gender);
return this;
}
/**
*
* The gender with which the customer identifies.
*
*
* @param gender
* The gender with which the customer identifies.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Gender
*/
@Deprecated
public CreateProfileRequest withGender(Gender gender) {
this.gender = gender.toString();
return this;
}
/**
*
* The customer’s phone number, which has not been specified as a mobile, home, or business number.
*
*
* @param phoneNumber
* The customer’s phone number, which has not been specified as a mobile, home, or business number.
*/
public void setPhoneNumber(String phoneNumber) {
this.phoneNumber = phoneNumber;
}
/**
*
* The customer’s phone number, which has not been specified as a mobile, home, or business number.
*
*
* @return The customer’s phone number, which has not been specified as a mobile, home, or business number.
*/
public String getPhoneNumber() {
return this.phoneNumber;
}
/**
*
* The customer’s phone number, which has not been specified as a mobile, home, or business number.
*
*
* @param phoneNumber
* The customer’s phone number, which has not been specified as a mobile, home, or business number.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest withPhoneNumber(String phoneNumber) {
setPhoneNumber(phoneNumber);
return this;
}
/**
*
* The customer’s mobile phone number.
*
*
* @param mobilePhoneNumber
* The customer’s mobile phone number.
*/
public void setMobilePhoneNumber(String mobilePhoneNumber) {
this.mobilePhoneNumber = mobilePhoneNumber;
}
/**
*
* The customer’s mobile phone number.
*
*
* @return The customer’s mobile phone number.
*/
public String getMobilePhoneNumber() {
return this.mobilePhoneNumber;
}
/**
*
* The customer’s mobile phone number.
*
*
* @param mobilePhoneNumber
* The customer’s mobile phone number.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest withMobilePhoneNumber(String mobilePhoneNumber) {
setMobilePhoneNumber(mobilePhoneNumber);
return this;
}
/**
*
* The customer’s home phone number.
*
*
* @param homePhoneNumber
* The customer’s home phone number.
*/
public void setHomePhoneNumber(String homePhoneNumber) {
this.homePhoneNumber = homePhoneNumber;
}
/**
*
* The customer’s home phone number.
*
*
* @return The customer’s home phone number.
*/
public String getHomePhoneNumber() {
return this.homePhoneNumber;
}
/**
*
* The customer’s home phone number.
*
*
* @param homePhoneNumber
* The customer’s home phone number.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest withHomePhoneNumber(String homePhoneNumber) {
setHomePhoneNumber(homePhoneNumber);
return this;
}
/**
*
* The customer’s business phone number.
*
*
* @param businessPhoneNumber
* The customer’s business phone number.
*/
public void setBusinessPhoneNumber(String businessPhoneNumber) {
this.businessPhoneNumber = businessPhoneNumber;
}
/**
*
* The customer’s business phone number.
*
*
* @return The customer’s business phone number.
*/
public String getBusinessPhoneNumber() {
return this.businessPhoneNumber;
}
/**
*
* The customer’s business phone number.
*
*
* @param businessPhoneNumber
* The customer’s business phone number.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest withBusinessPhoneNumber(String businessPhoneNumber) {
setBusinessPhoneNumber(businessPhoneNumber);
return this;
}
/**
*
* The customer’s email address, which has not been specified as a personal or business address.
*
*
* @param emailAddress
* The customer’s email address, which has not been specified as a personal or business address.
*/
public void setEmailAddress(String emailAddress) {
this.emailAddress = emailAddress;
}
/**
*
* The customer’s email address, which has not been specified as a personal or business address.
*
*
* @return The customer’s email address, which has not been specified as a personal or business address.
*/
public String getEmailAddress() {
return this.emailAddress;
}
/**
*
* The customer’s email address, which has not been specified as a personal or business address.
*
*
* @param emailAddress
* The customer’s email address, which has not been specified as a personal or business address.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest withEmailAddress(String emailAddress) {
setEmailAddress(emailAddress);
return this;
}
/**
*
* The customer’s personal email address.
*
*
* @param personalEmailAddress
* The customer’s personal email address.
*/
public void setPersonalEmailAddress(String personalEmailAddress) {
this.personalEmailAddress = personalEmailAddress;
}
/**
*
* The customer’s personal email address.
*
*
* @return The customer’s personal email address.
*/
public String getPersonalEmailAddress() {
return this.personalEmailAddress;
}
/**
*
* The customer’s personal email address.
*
*
* @param personalEmailAddress
* The customer’s personal email address.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest withPersonalEmailAddress(String personalEmailAddress) {
setPersonalEmailAddress(personalEmailAddress);
return this;
}
/**
*
* The customer’s business email address.
*
*
* @param businessEmailAddress
* The customer’s business email address.
*/
public void setBusinessEmailAddress(String businessEmailAddress) {
this.businessEmailAddress = businessEmailAddress;
}
/**
*
* The customer’s business email address.
*
*
* @return The customer’s business email address.
*/
public String getBusinessEmailAddress() {
return this.businessEmailAddress;
}
/**
*
* The customer’s business email address.
*
*
* @param businessEmailAddress
* The customer’s business email address.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest withBusinessEmailAddress(String businessEmailAddress) {
setBusinessEmailAddress(businessEmailAddress);
return this;
}
/**
*
* A generic address associated with the customer that is not mailing, shipping, or billing.
*
*
* @param address
* A generic address associated with the customer that is not mailing, shipping, or billing.
*/
public void setAddress(Address address) {
this.address = address;
}
/**
*
* A generic address associated with the customer that is not mailing, shipping, or billing.
*
*
* @return A generic address associated with the customer that is not mailing, shipping, or billing.
*/
public Address getAddress() {
return this.address;
}
/**
*
* A generic address associated with the customer that is not mailing, shipping, or billing.
*
*
* @param address
* A generic address associated with the customer that is not mailing, shipping, or billing.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest withAddress(Address address) {
setAddress(address);
return this;
}
/**
*
* The customer’s shipping address.
*
*
* @param shippingAddress
* The customer’s shipping address.
*/
public void setShippingAddress(Address shippingAddress) {
this.shippingAddress = shippingAddress;
}
/**
*
* The customer’s shipping address.
*
*
* @return The customer’s shipping address.
*/
public Address getShippingAddress() {
return this.shippingAddress;
}
/**
*
* The customer’s shipping address.
*
*
* @param shippingAddress
* The customer’s shipping address.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest withShippingAddress(Address shippingAddress) {
setShippingAddress(shippingAddress);
return this;
}
/**
*
* The customer’s mailing address.
*
*
* @param mailingAddress
* The customer’s mailing address.
*/
public void setMailingAddress(Address mailingAddress) {
this.mailingAddress = mailingAddress;
}
/**
*
* The customer’s mailing address.
*
*
* @return The customer’s mailing address.
*/
public Address getMailingAddress() {
return this.mailingAddress;
}
/**
*
* The customer’s mailing address.
*
*
* @param mailingAddress
* The customer’s mailing address.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest withMailingAddress(Address mailingAddress) {
setMailingAddress(mailingAddress);
return this;
}
/**
*
* The customer’s billing address.
*
*
* @param billingAddress
* The customer’s billing address.
*/
public void setBillingAddress(Address billingAddress) {
this.billingAddress = billingAddress;
}
/**
*
* The customer’s billing address.
*
*
* @return The customer’s billing address.
*/
public Address getBillingAddress() {
return this.billingAddress;
}
/**
*
* The customer’s billing address.
*
*
* @param billingAddress
* The customer’s billing address.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest withBillingAddress(Address billingAddress) {
setBillingAddress(billingAddress);
return this;
}
/**
*
* A key value pair of attributes of a customer profile.
*
*
* @return A key value pair of attributes of a customer profile.
*/
public java.util.Map getAttributes() {
return attributes;
}
/**
*
* A key value pair of attributes of a customer profile.
*
*
* @param attributes
* A key value pair of attributes of a customer profile.
*/
public void setAttributes(java.util.Map attributes) {
this.attributes = attributes;
}
/**
*
* A key value pair of attributes of a customer profile.
*
*
* @param attributes
* A key value pair of attributes of a customer profile.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest withAttributes(java.util.Map attributes) {
setAttributes(attributes);
return this;
}
/**
* Add a single Attributes entry
*
* @see CreateProfileRequest#withAttributes
* @returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest addAttributesEntry(String key, String value) {
if (null == this.attributes) {
this.attributes = new java.util.HashMap();
}
if (this.attributes.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.attributes.put(key, value);
return this;
}
/**
* Removes all the entries added into Attributes.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest clearAttributesEntries() {
this.attributes = null;
return this;
}
/**
*
* An alternative to PartyType
which accepts any string as input.
*
*
* @param partyTypeString
* An alternative to PartyType
which accepts any string as input.
*/
public void setPartyTypeString(String partyTypeString) {
this.partyTypeString = partyTypeString;
}
/**
*
* An alternative to PartyType
which accepts any string as input.
*
*
* @return An alternative to PartyType
which accepts any string as input.
*/
public String getPartyTypeString() {
return this.partyTypeString;
}
/**
*
* An alternative to PartyType
which accepts any string as input.
*
*
* @param partyTypeString
* An alternative to PartyType
which accepts any string as input.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest withPartyTypeString(String partyTypeString) {
setPartyTypeString(partyTypeString);
return this;
}
/**
*
* An alternative to Gender
which accepts any string as input.
*
*
* @param genderString
* An alternative to Gender
which accepts any string as input.
*/
public void setGenderString(String genderString) {
this.genderString = genderString;
}
/**
*
* An alternative to Gender
which accepts any string as input.
*
*
* @return An alternative to Gender
which accepts any string as input.
*/
public String getGenderString() {
return this.genderString;
}
/**
*
* An alternative to Gender
which accepts any string as input.
*
*
* @param genderString
* An alternative to Gender
which accepts any string as input.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateProfileRequest withGenderString(String genderString) {
setGenderString(genderString);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDomainName() != null)
sb.append("DomainName: ").append(getDomainName()).append(",");
if (getAccountNumber() != null)
sb.append("AccountNumber: ").append("***Sensitive Data Redacted***").append(",");
if (getAdditionalInformation() != null)
sb.append("AdditionalInformation: ").append("***Sensitive Data Redacted***").append(",");
if (getPartyType() != null)
sb.append("PartyType: ").append("***Sensitive Data Redacted***").append(",");
if (getBusinessName() != null)
sb.append("BusinessName: ").append("***Sensitive Data Redacted***").append(",");
if (getFirstName() != null)
sb.append("FirstName: ").append("***Sensitive Data Redacted***").append(",");
if (getMiddleName() != null)
sb.append("MiddleName: ").append("***Sensitive Data Redacted***").append(",");
if (getLastName() != null)
sb.append("LastName: ").append("***Sensitive Data Redacted***").append(",");
if (getBirthDate() != null)
sb.append("BirthDate: ").append("***Sensitive Data Redacted***").append(",");
if (getGender() != null)
sb.append("Gender: ").append("***Sensitive Data Redacted***").append(",");
if (getPhoneNumber() != null)
sb.append("PhoneNumber: ").append("***Sensitive Data Redacted***").append(",");
if (getMobilePhoneNumber() != null)
sb.append("MobilePhoneNumber: ").append("***Sensitive Data Redacted***").append(",");
if (getHomePhoneNumber() != null)
sb.append("HomePhoneNumber: ").append("***Sensitive Data Redacted***").append(",");
if (getBusinessPhoneNumber() != null)
sb.append("BusinessPhoneNumber: ").append("***Sensitive Data Redacted***").append(",");
if (getEmailAddress() != null)
sb.append("EmailAddress: ").append("***Sensitive Data Redacted***").append(",");
if (getPersonalEmailAddress() != null)
sb.append("PersonalEmailAddress: ").append("***Sensitive Data Redacted***").append(",");
if (getBusinessEmailAddress() != null)
sb.append("BusinessEmailAddress: ").append("***Sensitive Data Redacted***").append(",");
if (getAddress() != null)
sb.append("Address: ").append("***Sensitive Data Redacted***").append(",");
if (getShippingAddress() != null)
sb.append("ShippingAddress: ").append("***Sensitive Data Redacted***").append(",");
if (getMailingAddress() != null)
sb.append("MailingAddress: ").append("***Sensitive Data Redacted***").append(",");
if (getBillingAddress() != null)
sb.append("BillingAddress: ").append("***Sensitive Data Redacted***").append(",");
if (getAttributes() != null)
sb.append("Attributes: ").append("***Sensitive Data Redacted***").append(",");
if (getPartyTypeString() != null)
sb.append("PartyTypeString: ").append("***Sensitive Data Redacted***").append(",");
if (getGenderString() != null)
sb.append("GenderString: ").append("***Sensitive Data Redacted***");
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateProfileRequest == false)
return false;
CreateProfileRequest other = (CreateProfileRequest) obj;
if (other.getDomainName() == null ^ this.getDomainName() == null)
return false;
if (other.getDomainName() != null && other.getDomainName().equals(this.getDomainName()) == false)
return false;
if (other.getAccountNumber() == null ^ this.getAccountNumber() == null)
return false;
if (other.getAccountNumber() != null && other.getAccountNumber().equals(this.getAccountNumber()) == false)
return false;
if (other.getAdditionalInformation() == null ^ this.getAdditionalInformation() == null)
return false;
if (other.getAdditionalInformation() != null && other.getAdditionalInformation().equals(this.getAdditionalInformation()) == false)
return false;
if (other.getPartyType() == null ^ this.getPartyType() == null)
return false;
if (other.getPartyType() != null && other.getPartyType().equals(this.getPartyType()) == false)
return false;
if (other.getBusinessName() == null ^ this.getBusinessName() == null)
return false;
if (other.getBusinessName() != null && other.getBusinessName().equals(this.getBusinessName()) == false)
return false;
if (other.getFirstName() == null ^ this.getFirstName() == null)
return false;
if (other.getFirstName() != null && other.getFirstName().equals(this.getFirstName()) == false)
return false;
if (other.getMiddleName() == null ^ this.getMiddleName() == null)
return false;
if (other.getMiddleName() != null && other.getMiddleName().equals(this.getMiddleName()) == false)
return false;
if (other.getLastName() == null ^ this.getLastName() == null)
return false;
if (other.getLastName() != null && other.getLastName().equals(this.getLastName()) == false)
return false;
if (other.getBirthDate() == null ^ this.getBirthDate() == null)
return false;
if (other.getBirthDate() != null && other.getBirthDate().equals(this.getBirthDate()) == false)
return false;
if (other.getGender() == null ^ this.getGender() == null)
return false;
if (other.getGender() != null && other.getGender().equals(this.getGender()) == false)
return false;
if (other.getPhoneNumber() == null ^ this.getPhoneNumber() == null)
return false;
if (other.getPhoneNumber() != null && other.getPhoneNumber().equals(this.getPhoneNumber()) == false)
return false;
if (other.getMobilePhoneNumber() == null ^ this.getMobilePhoneNumber() == null)
return false;
if (other.getMobilePhoneNumber() != null && other.getMobilePhoneNumber().equals(this.getMobilePhoneNumber()) == false)
return false;
if (other.getHomePhoneNumber() == null ^ this.getHomePhoneNumber() == null)
return false;
if (other.getHomePhoneNumber() != null && other.getHomePhoneNumber().equals(this.getHomePhoneNumber()) == false)
return false;
if (other.getBusinessPhoneNumber() == null ^ this.getBusinessPhoneNumber() == null)
return false;
if (other.getBusinessPhoneNumber() != null && other.getBusinessPhoneNumber().equals(this.getBusinessPhoneNumber()) == false)
return false;
if (other.getEmailAddress() == null ^ this.getEmailAddress() == null)
return false;
if (other.getEmailAddress() != null && other.getEmailAddress().equals(this.getEmailAddress()) == false)
return false;
if (other.getPersonalEmailAddress() == null ^ this.getPersonalEmailAddress() == null)
return false;
if (other.getPersonalEmailAddress() != null && other.getPersonalEmailAddress().equals(this.getPersonalEmailAddress()) == false)
return false;
if (other.getBusinessEmailAddress() == null ^ this.getBusinessEmailAddress() == null)
return false;
if (other.getBusinessEmailAddress() != null && other.getBusinessEmailAddress().equals(this.getBusinessEmailAddress()) == false)
return false;
if (other.getAddress() == null ^ this.getAddress() == null)
return false;
if (other.getAddress() != null && other.getAddress().equals(this.getAddress()) == false)
return false;
if (other.getShippingAddress() == null ^ this.getShippingAddress() == null)
return false;
if (other.getShippingAddress() != null && other.getShippingAddress().equals(this.getShippingAddress()) == false)
return false;
if (other.getMailingAddress() == null ^ this.getMailingAddress() == null)
return false;
if (other.getMailingAddress() != null && other.getMailingAddress().equals(this.getMailingAddress()) == false)
return false;
if (other.getBillingAddress() == null ^ this.getBillingAddress() == null)
return false;
if (other.getBillingAddress() != null && other.getBillingAddress().equals(this.getBillingAddress()) == false)
return false;
if (other.getAttributes() == null ^ this.getAttributes() == null)
return false;
if (other.getAttributes() != null && other.getAttributes().equals(this.getAttributes()) == false)
return false;
if (other.getPartyTypeString() == null ^ this.getPartyTypeString() == null)
return false;
if (other.getPartyTypeString() != null && other.getPartyTypeString().equals(this.getPartyTypeString()) == false)
return false;
if (other.getGenderString() == null ^ this.getGenderString() == null)
return false;
if (other.getGenderString() != null && other.getGenderString().equals(this.getGenderString()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDomainName() == null) ? 0 : getDomainName().hashCode());
hashCode = prime * hashCode + ((getAccountNumber() == null) ? 0 : getAccountNumber().hashCode());
hashCode = prime * hashCode + ((getAdditionalInformation() == null) ? 0 : getAdditionalInformation().hashCode());
hashCode = prime * hashCode + ((getPartyType() == null) ? 0 : getPartyType().hashCode());
hashCode = prime * hashCode + ((getBusinessName() == null) ? 0 : getBusinessName().hashCode());
hashCode = prime * hashCode + ((getFirstName() == null) ? 0 : getFirstName().hashCode());
hashCode = prime * hashCode + ((getMiddleName() == null) ? 0 : getMiddleName().hashCode());
hashCode = prime * hashCode + ((getLastName() == null) ? 0 : getLastName().hashCode());
hashCode = prime * hashCode + ((getBirthDate() == null) ? 0 : getBirthDate().hashCode());
hashCode = prime * hashCode + ((getGender() == null) ? 0 : getGender().hashCode());
hashCode = prime * hashCode + ((getPhoneNumber() == null) ? 0 : getPhoneNumber().hashCode());
hashCode = prime * hashCode + ((getMobilePhoneNumber() == null) ? 0 : getMobilePhoneNumber().hashCode());
hashCode = prime * hashCode + ((getHomePhoneNumber() == null) ? 0 : getHomePhoneNumber().hashCode());
hashCode = prime * hashCode + ((getBusinessPhoneNumber() == null) ? 0 : getBusinessPhoneNumber().hashCode());
hashCode = prime * hashCode + ((getEmailAddress() == null) ? 0 : getEmailAddress().hashCode());
hashCode = prime * hashCode + ((getPersonalEmailAddress() == null) ? 0 : getPersonalEmailAddress().hashCode());
hashCode = prime * hashCode + ((getBusinessEmailAddress() == null) ? 0 : getBusinessEmailAddress().hashCode());
hashCode = prime * hashCode + ((getAddress() == null) ? 0 : getAddress().hashCode());
hashCode = prime * hashCode + ((getShippingAddress() == null) ? 0 : getShippingAddress().hashCode());
hashCode = prime * hashCode + ((getMailingAddress() == null) ? 0 : getMailingAddress().hashCode());
hashCode = prime * hashCode + ((getBillingAddress() == null) ? 0 : getBillingAddress().hashCode());
hashCode = prime * hashCode + ((getAttributes() == null) ? 0 : getAttributes().hashCode());
hashCode = prime * hashCode + ((getPartyTypeString() == null) ? 0 : getPartyTypeString().hashCode());
hashCode = prime * hashCode + ((getGenderString() == null) ? 0 : getGenderString().hashCode());
return hashCode;
}
@Override
public CreateProfileRequest clone() {
return (CreateProfileRequest) super.clone();
}
}