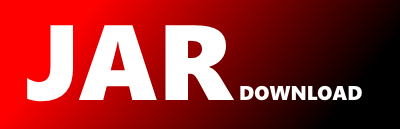
com.amazonaws.services.customerprofiles.model.ListIntegrationItem Maven / Gradle / Ivy
Show all versions of aws-java-sdk-customerprofiles Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.customerprofiles.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* An integration in list of integrations.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ListIntegrationItem implements Serializable, Cloneable, StructuredPojo {
/**
*
* The unique name of the domain.
*
*/
private String domainName;
/**
*
* The URI of the S3 bucket or any other type of data source.
*
*/
private String uri;
/**
*
* The name of the profile object type.
*
*/
private String objectTypeName;
/**
*
* The timestamp of when the domain was created.
*
*/
private java.util.Date createdAt;
/**
*
* The timestamp of when the domain was most recently edited.
*
*/
private java.util.Date lastUpdatedAt;
/**
*
* The tags used to organize, track, or control access for this resource.
*
*/
private java.util.Map tags;
/**
*
* A map in which each key is an event type from an external application such as Segment or Shopify, and each value
* is an ObjectTypeName
(template) used to ingest the event. It supports the following event types:
* SegmentIdentify
, ShopifyCreateCustomers
, ShopifyUpdateCustomers
,
* ShopifyCreateDraftOrders
, ShopifyUpdateDraftOrders
, ShopifyCreateOrders
,
* and ShopifyUpdatedOrders
.
*
*/
private java.util.Map objectTypeNames;
/**
*
* Unique identifier for the workflow.
*
*/
private String workflowId;
/**
*
* Boolean that shows if the Flow that's associated with the Integration is created in Amazon Appflow, or with
* ObjectTypeName equals _unstructured via API/CLI in flowDefinition.
*
*/
private Boolean isUnstructured;
/**
*
* The unique name of the domain.
*
*
* @param domainName
* The unique name of the domain.
*/
public void setDomainName(String domainName) {
this.domainName = domainName;
}
/**
*
* The unique name of the domain.
*
*
* @return The unique name of the domain.
*/
public String getDomainName() {
return this.domainName;
}
/**
*
* The unique name of the domain.
*
*
* @param domainName
* The unique name of the domain.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListIntegrationItem withDomainName(String domainName) {
setDomainName(domainName);
return this;
}
/**
*
* The URI of the S3 bucket or any other type of data source.
*
*
* @param uri
* The URI of the S3 bucket or any other type of data source.
*/
public void setUri(String uri) {
this.uri = uri;
}
/**
*
* The URI of the S3 bucket or any other type of data source.
*
*
* @return The URI of the S3 bucket or any other type of data source.
*/
public String getUri() {
return this.uri;
}
/**
*
* The URI of the S3 bucket or any other type of data source.
*
*
* @param uri
* The URI of the S3 bucket or any other type of data source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListIntegrationItem withUri(String uri) {
setUri(uri);
return this;
}
/**
*
* The name of the profile object type.
*
*
* @param objectTypeName
* The name of the profile object type.
*/
public void setObjectTypeName(String objectTypeName) {
this.objectTypeName = objectTypeName;
}
/**
*
* The name of the profile object type.
*
*
* @return The name of the profile object type.
*/
public String getObjectTypeName() {
return this.objectTypeName;
}
/**
*
* The name of the profile object type.
*
*
* @param objectTypeName
* The name of the profile object type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListIntegrationItem withObjectTypeName(String objectTypeName) {
setObjectTypeName(objectTypeName);
return this;
}
/**
*
* The timestamp of when the domain was created.
*
*
* @param createdAt
* The timestamp of when the domain was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The timestamp of when the domain was created.
*
*
* @return The timestamp of when the domain was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The timestamp of when the domain was created.
*
*
* @param createdAt
* The timestamp of when the domain was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListIntegrationItem withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The timestamp of when the domain was most recently edited.
*
*
* @param lastUpdatedAt
* The timestamp of when the domain was most recently edited.
*/
public void setLastUpdatedAt(java.util.Date lastUpdatedAt) {
this.lastUpdatedAt = lastUpdatedAt;
}
/**
*
* The timestamp of when the domain was most recently edited.
*
*
* @return The timestamp of when the domain was most recently edited.
*/
public java.util.Date getLastUpdatedAt() {
return this.lastUpdatedAt;
}
/**
*
* The timestamp of when the domain was most recently edited.
*
*
* @param lastUpdatedAt
* The timestamp of when the domain was most recently edited.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListIntegrationItem withLastUpdatedAt(java.util.Date lastUpdatedAt) {
setLastUpdatedAt(lastUpdatedAt);
return this;
}
/**
*
* The tags used to organize, track, or control access for this resource.
*
*
* @return The tags used to organize, track, or control access for this resource.
*/
public java.util.Map getTags() {
return tags;
}
/**
*
* The tags used to organize, track, or control access for this resource.
*
*
* @param tags
* The tags used to organize, track, or control access for this resource.
*/
public void setTags(java.util.Map tags) {
this.tags = tags;
}
/**
*
* The tags used to organize, track, or control access for this resource.
*
*
* @param tags
* The tags used to organize, track, or control access for this resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListIntegrationItem withTags(java.util.Map tags) {
setTags(tags);
return this;
}
/**
* Add a single Tags entry
*
* @see ListIntegrationItem#withTags
* @returns a reference to this object so that method calls can be chained together.
*/
public ListIntegrationItem addTagsEntry(String key, String value) {
if (null == this.tags) {
this.tags = new java.util.HashMap();
}
if (this.tags.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.tags.put(key, value);
return this;
}
/**
* Removes all the entries added into Tags.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListIntegrationItem clearTagsEntries() {
this.tags = null;
return this;
}
/**
*
* A map in which each key is an event type from an external application such as Segment or Shopify, and each value
* is an ObjectTypeName
(template) used to ingest the event. It supports the following event types:
* SegmentIdentify
, ShopifyCreateCustomers
, ShopifyUpdateCustomers
,
* ShopifyCreateDraftOrders
, ShopifyUpdateDraftOrders
, ShopifyCreateOrders
,
* and ShopifyUpdatedOrders
.
*
*
* @return A map in which each key is an event type from an external application such as Segment or Shopify, and
* each value is an ObjectTypeName
(template) used to ingest the event. It supports the
* following event types: SegmentIdentify
, ShopifyCreateCustomers
,
* ShopifyUpdateCustomers
, ShopifyCreateDraftOrders
,
* ShopifyUpdateDraftOrders
, ShopifyCreateOrders
, and
* ShopifyUpdatedOrders
.
*/
public java.util.Map getObjectTypeNames() {
return objectTypeNames;
}
/**
*
* A map in which each key is an event type from an external application such as Segment or Shopify, and each value
* is an ObjectTypeName
(template) used to ingest the event. It supports the following event types:
* SegmentIdentify
, ShopifyCreateCustomers
, ShopifyUpdateCustomers
,
* ShopifyCreateDraftOrders
, ShopifyUpdateDraftOrders
, ShopifyCreateOrders
,
* and ShopifyUpdatedOrders
.
*
*
* @param objectTypeNames
* A map in which each key is an event type from an external application such as Segment or Shopify, and each
* value is an ObjectTypeName
(template) used to ingest the event. It supports the following
* event types: SegmentIdentify
, ShopifyCreateCustomers
,
* ShopifyUpdateCustomers
, ShopifyCreateDraftOrders
,
* ShopifyUpdateDraftOrders
, ShopifyCreateOrders
, and
* ShopifyUpdatedOrders
.
*/
public void setObjectTypeNames(java.util.Map objectTypeNames) {
this.objectTypeNames = objectTypeNames;
}
/**
*
* A map in which each key is an event type from an external application such as Segment or Shopify, and each value
* is an ObjectTypeName
(template) used to ingest the event. It supports the following event types:
* SegmentIdentify
, ShopifyCreateCustomers
, ShopifyUpdateCustomers
,
* ShopifyCreateDraftOrders
, ShopifyUpdateDraftOrders
, ShopifyCreateOrders
,
* and ShopifyUpdatedOrders
.
*
*
* @param objectTypeNames
* A map in which each key is an event type from an external application such as Segment or Shopify, and each
* value is an ObjectTypeName
(template) used to ingest the event. It supports the following
* event types: SegmentIdentify
, ShopifyCreateCustomers
,
* ShopifyUpdateCustomers
, ShopifyCreateDraftOrders
,
* ShopifyUpdateDraftOrders
, ShopifyCreateOrders
, and
* ShopifyUpdatedOrders
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListIntegrationItem withObjectTypeNames(java.util.Map objectTypeNames) {
setObjectTypeNames(objectTypeNames);
return this;
}
/**
* Add a single ObjectTypeNames entry
*
* @see ListIntegrationItem#withObjectTypeNames
* @returns a reference to this object so that method calls can be chained together.
*/
public ListIntegrationItem addObjectTypeNamesEntry(String key, String value) {
if (null == this.objectTypeNames) {
this.objectTypeNames = new java.util.HashMap();
}
if (this.objectTypeNames.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.objectTypeNames.put(key, value);
return this;
}
/**
* Removes all the entries added into ObjectTypeNames.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListIntegrationItem clearObjectTypeNamesEntries() {
this.objectTypeNames = null;
return this;
}
/**
*
* Unique identifier for the workflow.
*
*
* @param workflowId
* Unique identifier for the workflow.
*/
public void setWorkflowId(String workflowId) {
this.workflowId = workflowId;
}
/**
*
* Unique identifier for the workflow.
*
*
* @return Unique identifier for the workflow.
*/
public String getWorkflowId() {
return this.workflowId;
}
/**
*
* Unique identifier for the workflow.
*
*
* @param workflowId
* Unique identifier for the workflow.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListIntegrationItem withWorkflowId(String workflowId) {
setWorkflowId(workflowId);
return this;
}
/**
*
* Boolean that shows if the Flow that's associated with the Integration is created in Amazon Appflow, or with
* ObjectTypeName equals _unstructured via API/CLI in flowDefinition.
*
*
* @param isUnstructured
* Boolean that shows if the Flow that's associated with the Integration is created in Amazon Appflow, or
* with ObjectTypeName equals _unstructured via API/CLI in flowDefinition.
*/
public void setIsUnstructured(Boolean isUnstructured) {
this.isUnstructured = isUnstructured;
}
/**
*
* Boolean that shows if the Flow that's associated with the Integration is created in Amazon Appflow, or with
* ObjectTypeName equals _unstructured via API/CLI in flowDefinition.
*
*
* @return Boolean that shows if the Flow that's associated with the Integration is created in Amazon Appflow, or
* with ObjectTypeName equals _unstructured via API/CLI in flowDefinition.
*/
public Boolean getIsUnstructured() {
return this.isUnstructured;
}
/**
*
* Boolean that shows if the Flow that's associated with the Integration is created in Amazon Appflow, or with
* ObjectTypeName equals _unstructured via API/CLI in flowDefinition.
*
*
* @param isUnstructured
* Boolean that shows if the Flow that's associated with the Integration is created in Amazon Appflow, or
* with ObjectTypeName equals _unstructured via API/CLI in flowDefinition.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListIntegrationItem withIsUnstructured(Boolean isUnstructured) {
setIsUnstructured(isUnstructured);
return this;
}
/**
*
* Boolean that shows if the Flow that's associated with the Integration is created in Amazon Appflow, or with
* ObjectTypeName equals _unstructured via API/CLI in flowDefinition.
*
*
* @return Boolean that shows if the Flow that's associated with the Integration is created in Amazon Appflow, or
* with ObjectTypeName equals _unstructured via API/CLI in flowDefinition.
*/
public Boolean isUnstructured() {
return this.isUnstructured;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDomainName() != null)
sb.append("DomainName: ").append(getDomainName()).append(",");
if (getUri() != null)
sb.append("Uri: ").append(getUri()).append(",");
if (getObjectTypeName() != null)
sb.append("ObjectTypeName: ").append(getObjectTypeName()).append(",");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getLastUpdatedAt() != null)
sb.append("LastUpdatedAt: ").append(getLastUpdatedAt()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getObjectTypeNames() != null)
sb.append("ObjectTypeNames: ").append(getObjectTypeNames()).append(",");
if (getWorkflowId() != null)
sb.append("WorkflowId: ").append(getWorkflowId()).append(",");
if (getIsUnstructured() != null)
sb.append("IsUnstructured: ").append(getIsUnstructured());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ListIntegrationItem == false)
return false;
ListIntegrationItem other = (ListIntegrationItem) obj;
if (other.getDomainName() == null ^ this.getDomainName() == null)
return false;
if (other.getDomainName() != null && other.getDomainName().equals(this.getDomainName()) == false)
return false;
if (other.getUri() == null ^ this.getUri() == null)
return false;
if (other.getUri() != null && other.getUri().equals(this.getUri()) == false)
return false;
if (other.getObjectTypeName() == null ^ this.getObjectTypeName() == null)
return false;
if (other.getObjectTypeName() != null && other.getObjectTypeName().equals(this.getObjectTypeName()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getLastUpdatedAt() == null ^ this.getLastUpdatedAt() == null)
return false;
if (other.getLastUpdatedAt() != null && other.getLastUpdatedAt().equals(this.getLastUpdatedAt()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getObjectTypeNames() == null ^ this.getObjectTypeNames() == null)
return false;
if (other.getObjectTypeNames() != null && other.getObjectTypeNames().equals(this.getObjectTypeNames()) == false)
return false;
if (other.getWorkflowId() == null ^ this.getWorkflowId() == null)
return false;
if (other.getWorkflowId() != null && other.getWorkflowId().equals(this.getWorkflowId()) == false)
return false;
if (other.getIsUnstructured() == null ^ this.getIsUnstructured() == null)
return false;
if (other.getIsUnstructured() != null && other.getIsUnstructured().equals(this.getIsUnstructured()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDomainName() == null) ? 0 : getDomainName().hashCode());
hashCode = prime * hashCode + ((getUri() == null) ? 0 : getUri().hashCode());
hashCode = prime * hashCode + ((getObjectTypeName() == null) ? 0 : getObjectTypeName().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getLastUpdatedAt() == null) ? 0 : getLastUpdatedAt().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getObjectTypeNames() == null) ? 0 : getObjectTypeNames().hashCode());
hashCode = prime * hashCode + ((getWorkflowId() == null) ? 0 : getWorkflowId().hashCode());
hashCode = prime * hashCode + ((getIsUnstructured() == null) ? 0 : getIsUnstructured().hashCode());
return hashCode;
}
@Override
public ListIntegrationItem clone() {
try {
return (ListIntegrationItem) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.customerprofiles.model.transform.ListIntegrationItemMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}