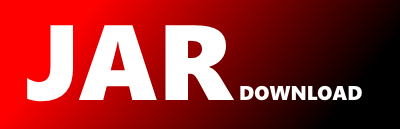
com.amazonaws.services.dataexchange.AWSDataExchange Maven / Gradle / Ivy
Show all versions of aws-java-sdk-dataexchange Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.dataexchange;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.dataexchange.model.*;
/**
* Interface for accessing AWS Data Exchange.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.dataexchange.AbstractAWSDataExchange} instead.
*
*
*
* AWS Data Exchange is a service that makes it easy for AWS customers to exchange data in the cloud. You can use the
* AWS Data Exchange APIs to create, update, manage, and access file-based data set in the AWS Cloud.
*
*
* As a subscriber, you can view and access the data sets that you have an entitlement to through a subscription. You
* can use the APIS to download or copy your entitled data sets to Amazon S3 for use across a variety of AWS analytics
* and machine learning services.
*
*
* As a provider, you can create and manage your data sets that you would like to publish to a product. Being able to
* package and provide your data sets into products requires a few steps to determine eligibility. For more information,
* visit the AWS Data Exchange User Guide.
*
*
* A data set is a collection of data that can be changed or updated over time. Data sets can be updated using
* revisions, which represent a new version or incremental change to a data set. A revision contains one or more assets.
* An asset in AWS Data Exchange is a piece of data that can be stored as an Amazon S3 object. The asset can be a
* structured data file, an image file, or some other data file. Jobs are asynchronous import or export operations used
* to create or copy assets.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSDataExchange {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "dataexchange";
/**
*
* This operation cancels a job. Jobs can be cancelled only when they are in the WAITING state.
*
*
* @param cancelJobRequest
* @return Result of the CancelJob operation returned by the service.
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @throws ConflictException
* 409 response
* @sample AWSDataExchange.CancelJob
* @see AWS API
* Documentation
*/
CancelJobResult cancelJob(CancelJobRequest cancelJobRequest);
/**
*
* This operation creates a data set.
*
*
* @param createDataSetRequest
* The request body for CreateDataSet.
* @return Result of the CreateDataSet operation returned by the service.
* @throws ThrottlingException
* 429 response
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @throws ServiceLimitExceededException
* 402 response
* @throws AccessDeniedException
* 403 response
* @sample AWSDataExchange.CreateDataSet
* @see AWS API
* Documentation
*/
CreateDataSetResult createDataSet(CreateDataSetRequest createDataSetRequest);
/**
*
* This operation creates an event action.
*
*
* @param createEventActionRequest
* The request body for CreateEventAction.
* @return Result of the CreateEventAction operation returned by the service.
* @throws ThrottlingException
* 429 response
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @throws ServiceLimitExceededException
* 402 response
* @throws AccessDeniedException
* 403 response
* @sample AWSDataExchange.CreateEventAction
* @see AWS
* API Documentation
*/
CreateEventActionResult createEventAction(CreateEventActionRequest createEventActionRequest);
/**
*
* This operation creates a job.
*
*
* @param createJobRequest
* The request body for CreateJob.
* @return Result of the CreateJob operation returned by the service.
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @throws AccessDeniedException
* 403 response
* @throws ConflictException
* 409 response
* @sample AWSDataExchange.CreateJob
* @see AWS API
* Documentation
*/
CreateJobResult createJob(CreateJobRequest createJobRequest);
/**
*
* This operation creates a revision for a data set.
*
*
* @param createRevisionRequest
* The request body for CreateRevision.
* @return Result of the CreateRevision operation returned by the service.
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @throws AccessDeniedException
* 403 response
* @sample AWSDataExchange.CreateRevision
* @see AWS
* API Documentation
*/
CreateRevisionResult createRevision(CreateRevisionRequest createRevisionRequest);
/**
*
* This operation deletes an asset.
*
*
* @param deleteAssetRequest
* @return Result of the DeleteAsset operation returned by the service.
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @throws AccessDeniedException
* 403 response
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ConflictException
* 409 response
* @sample AWSDataExchange.DeleteAsset
* @see AWS API
* Documentation
*/
DeleteAssetResult deleteAsset(DeleteAssetRequest deleteAssetRequest);
/**
*
* This operation deletes a data set.
*
*
* @param deleteDataSetRequest
* @return Result of the DeleteDataSet operation returned by the service.
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @throws AccessDeniedException
* 403 response
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ConflictException
* 409 response
* @sample AWSDataExchange.DeleteDataSet
* @see AWS API
* Documentation
*/
DeleteDataSetResult deleteDataSet(DeleteDataSetRequest deleteDataSetRequest);
/**
*
* This operation deletes the event action.
*
*
* @param deleteEventActionRequest
* @return Result of the DeleteEventAction operation returned by the service.
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @sample AWSDataExchange.DeleteEventAction
* @see AWS
* API Documentation
*/
DeleteEventActionResult deleteEventAction(DeleteEventActionRequest deleteEventActionRequest);
/**
*
* This operation deletes a revision.
*
*
* @param deleteRevisionRequest
* @return Result of the DeleteRevision operation returned by the service.
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @throws AccessDeniedException
* 403 response
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ConflictException
* 409 response
* @sample AWSDataExchange.DeleteRevision
* @see AWS
* API Documentation
*/
DeleteRevisionResult deleteRevision(DeleteRevisionRequest deleteRevisionRequest);
/**
*
* This operation returns information about an asset.
*
*
* @param getAssetRequest
* @return Result of the GetAsset operation returned by the service.
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @sample AWSDataExchange.GetAsset
* @see AWS API
* Documentation
*/
GetAssetResult getAsset(GetAssetRequest getAssetRequest);
/**
*
* This operation returns information about a data set.
*
*
* @param getDataSetRequest
* @return Result of the GetDataSet operation returned by the service.
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @sample AWSDataExchange.GetDataSet
* @see AWS API
* Documentation
*/
GetDataSetResult getDataSet(GetDataSetRequest getDataSetRequest);
/**
*
* This operation retrieves information about an event action.
*
*
* @param getEventActionRequest
* @return Result of the GetEventAction operation returned by the service.
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @sample AWSDataExchange.GetEventAction
* @see AWS
* API Documentation
*/
GetEventActionResult getEventAction(GetEventActionRequest getEventActionRequest);
/**
*
* This operation returns information about a job.
*
*
* @param getJobRequest
* @return Result of the GetJob operation returned by the service.
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @sample AWSDataExchange.GetJob
* @see AWS API
* Documentation
*/
GetJobResult getJob(GetJobRequest getJobRequest);
/**
*
* This operation returns information about a revision.
*
*
* @param getRevisionRequest
* @return Result of the GetRevision operation returned by the service.
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @sample AWSDataExchange.GetRevision
* @see AWS API
* Documentation
*/
GetRevisionResult getRevision(GetRevisionRequest getRevisionRequest);
/**
*
* This operation lists a data set's revisions sorted by CreatedAt in descending order.
*
*
* @param listDataSetRevisionsRequest
* @return Result of the ListDataSetRevisions operation returned by the service.
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @sample AWSDataExchange.ListDataSetRevisions
* @see AWS API Documentation
*/
ListDataSetRevisionsResult listDataSetRevisions(ListDataSetRevisionsRequest listDataSetRevisionsRequest);
/**
*
* This operation lists your data sets. When listing by origin OWNED, results are sorted by CreatedAt in descending
* order. When listing by origin ENTITLED, there is no order and the maxResults parameter is ignored.
*
*
* @param listDataSetsRequest
* @return Result of the ListDataSets operation returned by the service.
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @sample AWSDataExchange.ListDataSets
* @see AWS API
* Documentation
*/
ListDataSetsResult listDataSets(ListDataSetsRequest listDataSetsRequest);
/**
*
* This operation lists your event actions.
*
*
* @param listEventActionsRequest
* @return Result of the ListEventActions operation returned by the service.
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @sample AWSDataExchange.ListEventActions
* @see AWS
* API Documentation
*/
ListEventActionsResult listEventActions(ListEventActionsRequest listEventActionsRequest);
/**
*
* This operation lists your jobs sorted by CreatedAt in descending order.
*
*
* @param listJobsRequest
* @return Result of the ListJobs operation returned by the service.
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @sample AWSDataExchange.ListJobs
* @see AWS API
* Documentation
*/
ListJobsResult listJobs(ListJobsRequest listJobsRequest);
/**
*
* This operation lists a revision's assets sorted alphabetically in descending order.
*
*
* @param listRevisionAssetsRequest
* @return Result of the ListRevisionAssets operation returned by the service.
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @sample AWSDataExchange.ListRevisionAssets
* @see AWS API Documentation
*/
ListRevisionAssetsResult listRevisionAssets(ListRevisionAssetsRequest listRevisionAssetsRequest);
/**
*
* This operation lists the tags on the resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @sample AWSDataExchange.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* This operation revokes subscribers' access to a revision.
*
*
* @param revokeRevisionRequest
* The request body for RevokeRevision.
* @return Result of the RevokeRevision operation returned by the service.
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @throws AccessDeniedException
* 403 response
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ConflictException
* 409 response
* @sample AWSDataExchange.RevokeRevision
* @see AWS
* API Documentation
*/
RevokeRevisionResult revokeRevision(RevokeRevisionRequest revokeRevisionRequest);
/**
*
* This operation starts a job.
*
*
* @param startJobRequest
* @return Result of the StartJob operation returned by the service.
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @throws AccessDeniedException
* 403 response
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ConflictException
* 409 response
* @sample AWSDataExchange.StartJob
* @see AWS API
* Documentation
*/
StartJobResult startJob(StartJobRequest startJobRequest);
/**
*
* This operation tags a resource.
*
*
* @param tagResourceRequest
* The request body for TagResource.
* @return Result of the TagResource operation returned by the service.
* @sample AWSDataExchange.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* This operation removes one or more tags from a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @sample AWSDataExchange.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* This operation updates an asset.
*
*
* @param updateAssetRequest
* The request body for UpdateAsset.
* @return Result of the UpdateAsset operation returned by the service.
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @throws AccessDeniedException
* 403 response
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ConflictException
* 409 response
* @sample AWSDataExchange.UpdateAsset
* @see AWS API
* Documentation
*/
UpdateAssetResult updateAsset(UpdateAssetRequest updateAssetRequest);
/**
*
* This operation updates a data set.
*
*
* @param updateDataSetRequest
* The request body for UpdateDataSet.
* @return Result of the UpdateDataSet operation returned by the service.
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @throws AccessDeniedException
* 403 response
* @sample AWSDataExchange.UpdateDataSet
* @see AWS API
* Documentation
*/
UpdateDataSetResult updateDataSet(UpdateDataSetRequest updateDataSetRequest);
/**
*
* This operation updates the event action.
*
*
* @param updateEventActionRequest
* The request body for UpdateEventAction.
* @return Result of the UpdateEventAction operation returned by the service.
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @throws AccessDeniedException
* 403 response
* @sample AWSDataExchange.UpdateEventAction
* @see AWS
* API Documentation
*/
UpdateEventActionResult updateEventAction(UpdateEventActionRequest updateEventActionRequest);
/**
*
* This operation updates a revision.
*
*
* @param updateRevisionRequest
* The request body for UpdateRevision.
* @return Result of the UpdateRevision operation returned by the service.
* @throws ValidationException
* 400 response
* @throws InternalServerException
* 500 response
* @throws AccessDeniedException
* 403 response
* @throws ResourceNotFoundException
* 404 response
* @throws ThrottlingException
* 429 response
* @throws ConflictException
* 409 response
* @sample AWSDataExchange.UpdateRevision
* @see AWS
* API Documentation
*/
UpdateRevisionResult updateRevision(UpdateRevisionRequest updateRevisionRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}