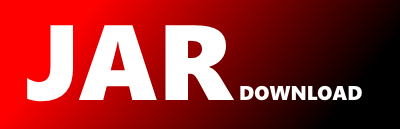
com.amazonaws.services.dataexchange.AWSDataExchangeAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-dataexchange Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.dataexchange;
import javax.annotation.Generated;
import com.amazonaws.services.dataexchange.model.*;
/**
* Interface for accessing AWS Data Exchange asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.dataexchange.AbstractAWSDataExchangeAsync} instead.
*
*
*
* AWS Data Exchange is a service that makes it easy for AWS customers to exchange data in the cloud. You can use the
* AWS Data Exchange APIs to create, update, manage, and access file-based data set in the AWS Cloud.
*
*
* As a subscriber, you can view and access the data sets that you have an entitlement to through a subscription. You
* can use the APIS to download or copy your entitled data sets to Amazon S3 for use across a variety of AWS analytics
* and machine learning services.
*
*
* As a provider, you can create and manage your data sets that you would like to publish to a product. Being able to
* package and provide your data sets into products requires a few steps to determine eligibility. For more information,
* visit the AWS Data Exchange User Guide.
*
*
* A data set is a collection of data that can be changed or updated over time. Data sets can be updated using
* revisions, which represent a new version or incremental change to a data set. A revision contains one or more assets.
* An asset in AWS Data Exchange is a piece of data that can be stored as an Amazon S3 object. The asset can be a
* structured data file, an image file, or some other data file. Jobs are asynchronous import or export operations used
* to create or copy assets.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSDataExchangeAsync extends AWSDataExchange {
/**
*
* This operation cancels a job. Jobs can be cancelled only when they are in the WAITING state.
*
*
* @param cancelJobRequest
* @return A Java Future containing the result of the CancelJob operation returned by the service.
* @sample AWSDataExchangeAsync.CancelJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelJobAsync(CancelJobRequest cancelJobRequest);
/**
*
* This operation cancels a job. Jobs can be cancelled only when they are in the WAITING state.
*
*
* @param cancelJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelJob operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.CancelJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelJobAsync(CancelJobRequest cancelJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation creates a data set.
*
*
* @param createDataSetRequest
* @return A Java Future containing the result of the CreateDataSet operation returned by the service.
* @sample AWSDataExchangeAsync.CreateDataSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDataSetAsync(CreateDataSetRequest createDataSetRequest);
/**
*
* This operation creates a data set.
*
*
* @param createDataSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDataSet operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.CreateDataSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDataSetAsync(CreateDataSetRequest createDataSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation creates an event action.
*
*
* @param createEventActionRequest
* @return A Java Future containing the result of the CreateEventAction operation returned by the service.
* @sample AWSDataExchangeAsync.CreateEventAction
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createEventActionAsync(CreateEventActionRequest createEventActionRequest);
/**
*
* This operation creates an event action.
*
*
* @param createEventActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEventAction operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.CreateEventAction
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createEventActionAsync(CreateEventActionRequest createEventActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation creates a job.
*
*
* @param createJobRequest
* @return A Java Future containing the result of the CreateJob operation returned by the service.
* @sample AWSDataExchangeAsync.CreateJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createJobAsync(CreateJobRequest createJobRequest);
/**
*
* This operation creates a job.
*
*
* @param createJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateJob operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.CreateJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createJobAsync(CreateJobRequest createJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation creates a revision for a data set.
*
*
* @param createRevisionRequest
* @return A Java Future containing the result of the CreateRevision operation returned by the service.
* @sample AWSDataExchangeAsync.CreateRevision
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createRevisionAsync(CreateRevisionRequest createRevisionRequest);
/**
*
* This operation creates a revision for a data set.
*
*
* @param createRevisionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRevision operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.CreateRevision
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createRevisionAsync(CreateRevisionRequest createRevisionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation deletes an asset.
*
*
* @param deleteAssetRequest
* @return A Java Future containing the result of the DeleteAsset operation returned by the service.
* @sample AWSDataExchangeAsync.DeleteAsset
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAssetAsync(DeleteAssetRequest deleteAssetRequest);
/**
*
* This operation deletes an asset.
*
*
* @param deleteAssetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAsset operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.DeleteAsset
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAssetAsync(DeleteAssetRequest deleteAssetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation deletes a data set.
*
*
* @param deleteDataSetRequest
* @return A Java Future containing the result of the DeleteDataSet operation returned by the service.
* @sample AWSDataExchangeAsync.DeleteDataSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDataSetAsync(DeleteDataSetRequest deleteDataSetRequest);
/**
*
* This operation deletes a data set.
*
*
* @param deleteDataSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDataSet operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.DeleteDataSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDataSetAsync(DeleteDataSetRequest deleteDataSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation deletes the event action.
*
*
* @param deleteEventActionRequest
* @return A Java Future containing the result of the DeleteEventAction operation returned by the service.
* @sample AWSDataExchangeAsync.DeleteEventAction
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteEventActionAsync(DeleteEventActionRequest deleteEventActionRequest);
/**
*
* This operation deletes the event action.
*
*
* @param deleteEventActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEventAction operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.DeleteEventAction
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteEventActionAsync(DeleteEventActionRequest deleteEventActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation deletes a revision.
*
*
* @param deleteRevisionRequest
* @return A Java Future containing the result of the DeleteRevision operation returned by the service.
* @sample AWSDataExchangeAsync.DeleteRevision
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteRevisionAsync(DeleteRevisionRequest deleteRevisionRequest);
/**
*
* This operation deletes a revision.
*
*
* @param deleteRevisionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRevision operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.DeleteRevision
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteRevisionAsync(DeleteRevisionRequest deleteRevisionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation returns information about an asset.
*
*
* @param getAssetRequest
* @return A Java Future containing the result of the GetAsset operation returned by the service.
* @sample AWSDataExchangeAsync.GetAsset
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAssetAsync(GetAssetRequest getAssetRequest);
/**
*
* This operation returns information about an asset.
*
*
* @param getAssetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAsset operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.GetAsset
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAssetAsync(GetAssetRequest getAssetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation returns information about a data set.
*
*
* @param getDataSetRequest
* @return A Java Future containing the result of the GetDataSet operation returned by the service.
* @sample AWSDataExchangeAsync.GetDataSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDataSetAsync(GetDataSetRequest getDataSetRequest);
/**
*
* This operation returns information about a data set.
*
*
* @param getDataSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDataSet operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.GetDataSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDataSetAsync(GetDataSetRequest getDataSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation retrieves information about an event action.
*
*
* @param getEventActionRequest
* @return A Java Future containing the result of the GetEventAction operation returned by the service.
* @sample AWSDataExchangeAsync.GetEventAction
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getEventActionAsync(GetEventActionRequest getEventActionRequest);
/**
*
* This operation retrieves information about an event action.
*
*
* @param getEventActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEventAction operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.GetEventAction
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getEventActionAsync(GetEventActionRequest getEventActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation returns information about a job.
*
*
* @param getJobRequest
* @return A Java Future containing the result of the GetJob operation returned by the service.
* @sample AWSDataExchangeAsync.GetJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getJobAsync(GetJobRequest getJobRequest);
/**
*
* This operation returns information about a job.
*
*
* @param getJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetJob operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.GetJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getJobAsync(GetJobRequest getJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation returns information about a revision.
*
*
* @param getRevisionRequest
* @return A Java Future containing the result of the GetRevision operation returned by the service.
* @sample AWSDataExchangeAsync.GetRevision
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getRevisionAsync(GetRevisionRequest getRevisionRequest);
/**
*
* This operation returns information about a revision.
*
*
* @param getRevisionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetRevision operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.GetRevision
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getRevisionAsync(GetRevisionRequest getRevisionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation lists a data set's revisions sorted by CreatedAt in descending order.
*
*
* @param listDataSetRevisionsRequest
* @return A Java Future containing the result of the ListDataSetRevisions operation returned by the service.
* @sample AWSDataExchangeAsync.ListDataSetRevisions
* @see AWS API Documentation
*/
java.util.concurrent.Future listDataSetRevisionsAsync(ListDataSetRevisionsRequest listDataSetRevisionsRequest);
/**
*
* This operation lists a data set's revisions sorted by CreatedAt in descending order.
*
*
* @param listDataSetRevisionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDataSetRevisions operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.ListDataSetRevisions
* @see AWS API Documentation
*/
java.util.concurrent.Future listDataSetRevisionsAsync(ListDataSetRevisionsRequest listDataSetRevisionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation lists your data sets. When listing by origin OWNED, results are sorted by CreatedAt in descending
* order. When listing by origin ENTITLED, there is no order and the maxResults parameter is ignored.
*
*
* @param listDataSetsRequest
* @return A Java Future containing the result of the ListDataSets operation returned by the service.
* @sample AWSDataExchangeAsync.ListDataSets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDataSetsAsync(ListDataSetsRequest listDataSetsRequest);
/**
*
* This operation lists your data sets. When listing by origin OWNED, results are sorted by CreatedAt in descending
* order. When listing by origin ENTITLED, there is no order and the maxResults parameter is ignored.
*
*
* @param listDataSetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDataSets operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.ListDataSets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDataSetsAsync(ListDataSetsRequest listDataSetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation lists your event actions.
*
*
* @param listEventActionsRequest
* @return A Java Future containing the result of the ListEventActions operation returned by the service.
* @sample AWSDataExchangeAsync.ListEventActions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listEventActionsAsync(ListEventActionsRequest listEventActionsRequest);
/**
*
* This operation lists your event actions.
*
*
* @param listEventActionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListEventActions operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.ListEventActions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listEventActionsAsync(ListEventActionsRequest listEventActionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation lists your jobs sorted by CreatedAt in descending order.
*
*
* @param listJobsRequest
* @return A Java Future containing the result of the ListJobs operation returned by the service.
* @sample AWSDataExchangeAsync.ListJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listJobsAsync(ListJobsRequest listJobsRequest);
/**
*
* This operation lists your jobs sorted by CreatedAt in descending order.
*
*
* @param listJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListJobs operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.ListJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listJobsAsync(ListJobsRequest listJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation lists a revision's assets sorted alphabetically in descending order.
*
*
* @param listRevisionAssetsRequest
* @return A Java Future containing the result of the ListRevisionAssets operation returned by the service.
* @sample AWSDataExchangeAsync.ListRevisionAssets
* @see AWS API Documentation
*/
java.util.concurrent.Future listRevisionAssetsAsync(ListRevisionAssetsRequest listRevisionAssetsRequest);
/**
*
* This operation lists a revision's assets sorted alphabetically in descending order.
*
*
* @param listRevisionAssetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRevisionAssets operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.ListRevisionAssets
* @see AWS API Documentation
*/
java.util.concurrent.Future listRevisionAssetsAsync(ListRevisionAssetsRequest listRevisionAssetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation lists the tags on the resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSDataExchangeAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* This operation lists the tags on the resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation revokes subscribers' access to a revision.
*
*
* @param revokeRevisionRequest
* @return A Java Future containing the result of the RevokeRevision operation returned by the service.
* @sample AWSDataExchangeAsync.RevokeRevision
* @see AWS
* API Documentation
*/
java.util.concurrent.Future revokeRevisionAsync(RevokeRevisionRequest revokeRevisionRequest);
/**
*
* This operation revokes subscribers' access to a revision.
*
*
* @param revokeRevisionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RevokeRevision operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.RevokeRevision
* @see AWS
* API Documentation
*/
java.util.concurrent.Future revokeRevisionAsync(RevokeRevisionRequest revokeRevisionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation starts a job.
*
*
* @param startJobRequest
* @return A Java Future containing the result of the StartJob operation returned by the service.
* @sample AWSDataExchangeAsync.StartJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startJobAsync(StartJobRequest startJobRequest);
/**
*
* This operation starts a job.
*
*
* @param startJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartJob operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.StartJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startJobAsync(StartJobRequest startJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation tags a resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSDataExchangeAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* This operation tags a resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation removes one or more tags from a resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSDataExchangeAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* This operation removes one or more tags from a resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation updates an asset.
*
*
* @param updateAssetRequest
* @return A Java Future containing the result of the UpdateAsset operation returned by the service.
* @sample AWSDataExchangeAsync.UpdateAsset
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateAssetAsync(UpdateAssetRequest updateAssetRequest);
/**
*
* This operation updates an asset.
*
*
* @param updateAssetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAsset operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.UpdateAsset
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateAssetAsync(UpdateAssetRequest updateAssetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation updates a data set.
*
*
* @param updateDataSetRequest
* @return A Java Future containing the result of the UpdateDataSet operation returned by the service.
* @sample AWSDataExchangeAsync.UpdateDataSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateDataSetAsync(UpdateDataSetRequest updateDataSetRequest);
/**
*
* This operation updates a data set.
*
*
* @param updateDataSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDataSet operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.UpdateDataSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateDataSetAsync(UpdateDataSetRequest updateDataSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation updates the event action.
*
*
* @param updateEventActionRequest
* @return A Java Future containing the result of the UpdateEventAction operation returned by the service.
* @sample AWSDataExchangeAsync.UpdateEventAction
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateEventActionAsync(UpdateEventActionRequest updateEventActionRequest);
/**
*
* This operation updates the event action.
*
*
* @param updateEventActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateEventAction operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.UpdateEventAction
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateEventActionAsync(UpdateEventActionRequest updateEventActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation updates a revision.
*
*
* @param updateRevisionRequest
* @return A Java Future containing the result of the UpdateRevision operation returned by the service.
* @sample AWSDataExchangeAsync.UpdateRevision
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateRevisionAsync(UpdateRevisionRequest updateRevisionRequest);
/**
*
* This operation updates a revision.
*
*
* @param updateRevisionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateRevision operation returned by the service.
* @sample AWSDataExchangeAsyncHandler.UpdateRevision
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateRevisionAsync(UpdateRevisionRequest updateRevisionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}