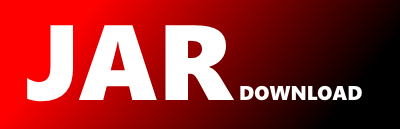
com.amazonaws.services.dataexchange.model.RevokeRevisionResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-dataexchange Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.dataexchange.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class RevokeRevisionResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The ARN for the revision.
*
*/
private String arn;
/**
*
* An optional comment about the revision.
*
*/
private String comment;
/**
*
* The date and time that the revision was created, in ISO 8601 format.
*
*/
private java.util.Date createdAt;
/**
*
* The unique identifier for the data set associated with this revision.
*
*/
private String dataSetId;
/**
*
* To publish a revision to a data set in a product, the revision must first be finalized. Finalizing a revision
* tells AWS Data Exchange that changes to the assets in the revision are complete. After it's in this read-only
* state, you can publish the revision to your products. Finalized revisions can be published through the AWS Data
* Exchange console or the AWS Marketplace Catalog API, using the StartChangeSet AWS Marketplace Catalog API action.
* When using the API, revisions are uniquely identified by their ARN.
*
*/
private Boolean finalized;
/**
*
* The unique identifier for the revision.
*
*/
private String id;
/**
*
* The revision ID of the owned revision corresponding to the entitled revision being viewed. This parameter is
* returned when a revision owner is viewing the entitled copy of its owned revision.
*
*/
private String sourceId;
/**
*
* The date and time that the revision was last updated, in ISO 8601 format.
*
*/
private java.util.Date updatedAt;
/**
*
* A required comment to inform subscribers of the reason their access to the revision was revoked.
*
*/
private String revocationComment;
/**
*
* A status indicating that subscribers' access to the revision was revoked.
*
*/
private Boolean revoked;
/**
*
* The date and time that the revision was revoked, in ISO 8601 format.
*
*/
private java.util.Date revokedAt;
/**
*
* The ARN for the revision.
*
*
* @param arn
* The ARN for the revision.
*/
public void setArn(String arn) {
this.arn = arn;
}
/**
*
* The ARN for the revision.
*
*
* @return The ARN for the revision.
*/
public String getArn() {
return this.arn;
}
/**
*
* The ARN for the revision.
*
*
* @param arn
* The ARN for the revision.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RevokeRevisionResult withArn(String arn) {
setArn(arn);
return this;
}
/**
*
* An optional comment about the revision.
*
*
* @param comment
* An optional comment about the revision.
*/
public void setComment(String comment) {
this.comment = comment;
}
/**
*
* An optional comment about the revision.
*
*
* @return An optional comment about the revision.
*/
public String getComment() {
return this.comment;
}
/**
*
* An optional comment about the revision.
*
*
* @param comment
* An optional comment about the revision.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RevokeRevisionResult withComment(String comment) {
setComment(comment);
return this;
}
/**
*
* The date and time that the revision was created, in ISO 8601 format.
*
*
* @param createdAt
* The date and time that the revision was created, in ISO 8601 format.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The date and time that the revision was created, in ISO 8601 format.
*
*
* @return The date and time that the revision was created, in ISO 8601 format.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The date and time that the revision was created, in ISO 8601 format.
*
*
* @param createdAt
* The date and time that the revision was created, in ISO 8601 format.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RevokeRevisionResult withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The unique identifier for the data set associated with this revision.
*
*
* @param dataSetId
* The unique identifier for the data set associated with this revision.
*/
public void setDataSetId(String dataSetId) {
this.dataSetId = dataSetId;
}
/**
*
* The unique identifier for the data set associated with this revision.
*
*
* @return The unique identifier for the data set associated with this revision.
*/
public String getDataSetId() {
return this.dataSetId;
}
/**
*
* The unique identifier for the data set associated with this revision.
*
*
* @param dataSetId
* The unique identifier for the data set associated with this revision.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RevokeRevisionResult withDataSetId(String dataSetId) {
setDataSetId(dataSetId);
return this;
}
/**
*
* To publish a revision to a data set in a product, the revision must first be finalized. Finalizing a revision
* tells AWS Data Exchange that changes to the assets in the revision are complete. After it's in this read-only
* state, you can publish the revision to your products. Finalized revisions can be published through the AWS Data
* Exchange console or the AWS Marketplace Catalog API, using the StartChangeSet AWS Marketplace Catalog API action.
* When using the API, revisions are uniquely identified by their ARN.
*
*
* @param finalized
* To publish a revision to a data set in a product, the revision must first be finalized. Finalizing a
* revision tells AWS Data Exchange that changes to the assets in the revision are complete. After it's in
* this read-only state, you can publish the revision to your products. Finalized revisions can be published
* through the AWS Data Exchange console or the AWS Marketplace Catalog API, using the StartChangeSet AWS
* Marketplace Catalog API action. When using the API, revisions are uniquely identified by their ARN.
*/
public void setFinalized(Boolean finalized) {
this.finalized = finalized;
}
/**
*
* To publish a revision to a data set in a product, the revision must first be finalized. Finalizing a revision
* tells AWS Data Exchange that changes to the assets in the revision are complete. After it's in this read-only
* state, you can publish the revision to your products. Finalized revisions can be published through the AWS Data
* Exchange console or the AWS Marketplace Catalog API, using the StartChangeSet AWS Marketplace Catalog API action.
* When using the API, revisions are uniquely identified by their ARN.
*
*
* @return To publish a revision to a data set in a product, the revision must first be finalized. Finalizing a
* revision tells AWS Data Exchange that changes to the assets in the revision are complete. After it's in
* this read-only state, you can publish the revision to your products. Finalized revisions can be published
* through the AWS Data Exchange console or the AWS Marketplace Catalog API, using the StartChangeSet AWS
* Marketplace Catalog API action. When using the API, revisions are uniquely identified by their ARN.
*/
public Boolean getFinalized() {
return this.finalized;
}
/**
*
* To publish a revision to a data set in a product, the revision must first be finalized. Finalizing a revision
* tells AWS Data Exchange that changes to the assets in the revision are complete. After it's in this read-only
* state, you can publish the revision to your products. Finalized revisions can be published through the AWS Data
* Exchange console or the AWS Marketplace Catalog API, using the StartChangeSet AWS Marketplace Catalog API action.
* When using the API, revisions are uniquely identified by their ARN.
*
*
* @param finalized
* To publish a revision to a data set in a product, the revision must first be finalized. Finalizing a
* revision tells AWS Data Exchange that changes to the assets in the revision are complete. After it's in
* this read-only state, you can publish the revision to your products. Finalized revisions can be published
* through the AWS Data Exchange console or the AWS Marketplace Catalog API, using the StartChangeSet AWS
* Marketplace Catalog API action. When using the API, revisions are uniquely identified by their ARN.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RevokeRevisionResult withFinalized(Boolean finalized) {
setFinalized(finalized);
return this;
}
/**
*
* To publish a revision to a data set in a product, the revision must first be finalized. Finalizing a revision
* tells AWS Data Exchange that changes to the assets in the revision are complete. After it's in this read-only
* state, you can publish the revision to your products. Finalized revisions can be published through the AWS Data
* Exchange console or the AWS Marketplace Catalog API, using the StartChangeSet AWS Marketplace Catalog API action.
* When using the API, revisions are uniquely identified by their ARN.
*
*
* @return To publish a revision to a data set in a product, the revision must first be finalized. Finalizing a
* revision tells AWS Data Exchange that changes to the assets in the revision are complete. After it's in
* this read-only state, you can publish the revision to your products. Finalized revisions can be published
* through the AWS Data Exchange console or the AWS Marketplace Catalog API, using the StartChangeSet AWS
* Marketplace Catalog API action. When using the API, revisions are uniquely identified by their ARN.
*/
public Boolean isFinalized() {
return this.finalized;
}
/**
*
* The unique identifier for the revision.
*
*
* @param id
* The unique identifier for the revision.
*/
public void setId(String id) {
this.id = id;
}
/**
*
* The unique identifier for the revision.
*
*
* @return The unique identifier for the revision.
*/
public String getId() {
return this.id;
}
/**
*
* The unique identifier for the revision.
*
*
* @param id
* The unique identifier for the revision.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RevokeRevisionResult withId(String id) {
setId(id);
return this;
}
/**
*
* The revision ID of the owned revision corresponding to the entitled revision being viewed. This parameter is
* returned when a revision owner is viewing the entitled copy of its owned revision.
*
*
* @param sourceId
* The revision ID of the owned revision corresponding to the entitled revision being viewed. This parameter
* is returned when a revision owner is viewing the entitled copy of its owned revision.
*/
public void setSourceId(String sourceId) {
this.sourceId = sourceId;
}
/**
*
* The revision ID of the owned revision corresponding to the entitled revision being viewed. This parameter is
* returned when a revision owner is viewing the entitled copy of its owned revision.
*
*
* @return The revision ID of the owned revision corresponding to the entitled revision being viewed. This parameter
* is returned when a revision owner is viewing the entitled copy of its owned revision.
*/
public String getSourceId() {
return this.sourceId;
}
/**
*
* The revision ID of the owned revision corresponding to the entitled revision being viewed. This parameter is
* returned when a revision owner is viewing the entitled copy of its owned revision.
*
*
* @param sourceId
* The revision ID of the owned revision corresponding to the entitled revision being viewed. This parameter
* is returned when a revision owner is viewing the entitled copy of its owned revision.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RevokeRevisionResult withSourceId(String sourceId) {
setSourceId(sourceId);
return this;
}
/**
*
* The date and time that the revision was last updated, in ISO 8601 format.
*
*
* @param updatedAt
* The date and time that the revision was last updated, in ISO 8601 format.
*/
public void setUpdatedAt(java.util.Date updatedAt) {
this.updatedAt = updatedAt;
}
/**
*
* The date and time that the revision was last updated, in ISO 8601 format.
*
*
* @return The date and time that the revision was last updated, in ISO 8601 format.
*/
public java.util.Date getUpdatedAt() {
return this.updatedAt;
}
/**
*
* The date and time that the revision was last updated, in ISO 8601 format.
*
*
* @param updatedAt
* The date and time that the revision was last updated, in ISO 8601 format.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RevokeRevisionResult withUpdatedAt(java.util.Date updatedAt) {
setUpdatedAt(updatedAt);
return this;
}
/**
*
* A required comment to inform subscribers of the reason their access to the revision was revoked.
*
*
* @param revocationComment
* A required comment to inform subscribers of the reason their access to the revision was revoked.
*/
public void setRevocationComment(String revocationComment) {
this.revocationComment = revocationComment;
}
/**
*
* A required comment to inform subscribers of the reason their access to the revision was revoked.
*
*
* @return A required comment to inform subscribers of the reason their access to the revision was revoked.
*/
public String getRevocationComment() {
return this.revocationComment;
}
/**
*
* A required comment to inform subscribers of the reason their access to the revision was revoked.
*
*
* @param revocationComment
* A required comment to inform subscribers of the reason their access to the revision was revoked.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RevokeRevisionResult withRevocationComment(String revocationComment) {
setRevocationComment(revocationComment);
return this;
}
/**
*
* A status indicating that subscribers' access to the revision was revoked.
*
*
* @param revoked
* A status indicating that subscribers' access to the revision was revoked.
*/
public void setRevoked(Boolean revoked) {
this.revoked = revoked;
}
/**
*
* A status indicating that subscribers' access to the revision was revoked.
*
*
* @return A status indicating that subscribers' access to the revision was revoked.
*/
public Boolean getRevoked() {
return this.revoked;
}
/**
*
* A status indicating that subscribers' access to the revision was revoked.
*
*
* @param revoked
* A status indicating that subscribers' access to the revision was revoked.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RevokeRevisionResult withRevoked(Boolean revoked) {
setRevoked(revoked);
return this;
}
/**
*
* A status indicating that subscribers' access to the revision was revoked.
*
*
* @return A status indicating that subscribers' access to the revision was revoked.
*/
public Boolean isRevoked() {
return this.revoked;
}
/**
*
* The date and time that the revision was revoked, in ISO 8601 format.
*
*
* @param revokedAt
* The date and time that the revision was revoked, in ISO 8601 format.
*/
public void setRevokedAt(java.util.Date revokedAt) {
this.revokedAt = revokedAt;
}
/**
*
* The date and time that the revision was revoked, in ISO 8601 format.
*
*
* @return The date and time that the revision was revoked, in ISO 8601 format.
*/
public java.util.Date getRevokedAt() {
return this.revokedAt;
}
/**
*
* The date and time that the revision was revoked, in ISO 8601 format.
*
*
* @param revokedAt
* The date and time that the revision was revoked, in ISO 8601 format.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RevokeRevisionResult withRevokedAt(java.util.Date revokedAt) {
setRevokedAt(revokedAt);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getArn() != null)
sb.append("Arn: ").append(getArn()).append(",");
if (getComment() != null)
sb.append("Comment: ").append(getComment()).append(",");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getDataSetId() != null)
sb.append("DataSetId: ").append(getDataSetId()).append(",");
if (getFinalized() != null)
sb.append("Finalized: ").append(getFinalized()).append(",");
if (getId() != null)
sb.append("Id: ").append(getId()).append(",");
if (getSourceId() != null)
sb.append("SourceId: ").append(getSourceId()).append(",");
if (getUpdatedAt() != null)
sb.append("UpdatedAt: ").append(getUpdatedAt()).append(",");
if (getRevocationComment() != null)
sb.append("RevocationComment: ").append(getRevocationComment()).append(",");
if (getRevoked() != null)
sb.append("Revoked: ").append(getRevoked()).append(",");
if (getRevokedAt() != null)
sb.append("RevokedAt: ").append(getRevokedAt());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof RevokeRevisionResult == false)
return false;
RevokeRevisionResult other = (RevokeRevisionResult) obj;
if (other.getArn() == null ^ this.getArn() == null)
return false;
if (other.getArn() != null && other.getArn().equals(this.getArn()) == false)
return false;
if (other.getComment() == null ^ this.getComment() == null)
return false;
if (other.getComment() != null && other.getComment().equals(this.getComment()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getDataSetId() == null ^ this.getDataSetId() == null)
return false;
if (other.getDataSetId() != null && other.getDataSetId().equals(this.getDataSetId()) == false)
return false;
if (other.getFinalized() == null ^ this.getFinalized() == null)
return false;
if (other.getFinalized() != null && other.getFinalized().equals(this.getFinalized()) == false)
return false;
if (other.getId() == null ^ this.getId() == null)
return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false)
return false;
if (other.getSourceId() == null ^ this.getSourceId() == null)
return false;
if (other.getSourceId() != null && other.getSourceId().equals(this.getSourceId()) == false)
return false;
if (other.getUpdatedAt() == null ^ this.getUpdatedAt() == null)
return false;
if (other.getUpdatedAt() != null && other.getUpdatedAt().equals(this.getUpdatedAt()) == false)
return false;
if (other.getRevocationComment() == null ^ this.getRevocationComment() == null)
return false;
if (other.getRevocationComment() != null && other.getRevocationComment().equals(this.getRevocationComment()) == false)
return false;
if (other.getRevoked() == null ^ this.getRevoked() == null)
return false;
if (other.getRevoked() != null && other.getRevoked().equals(this.getRevoked()) == false)
return false;
if (other.getRevokedAt() == null ^ this.getRevokedAt() == null)
return false;
if (other.getRevokedAt() != null && other.getRevokedAt().equals(this.getRevokedAt()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getArn() == null) ? 0 : getArn().hashCode());
hashCode = prime * hashCode + ((getComment() == null) ? 0 : getComment().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getDataSetId() == null) ? 0 : getDataSetId().hashCode());
hashCode = prime * hashCode + ((getFinalized() == null) ? 0 : getFinalized().hashCode());
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getSourceId() == null) ? 0 : getSourceId().hashCode());
hashCode = prime * hashCode + ((getUpdatedAt() == null) ? 0 : getUpdatedAt().hashCode());
hashCode = prime * hashCode + ((getRevocationComment() == null) ? 0 : getRevocationComment().hashCode());
hashCode = prime * hashCode + ((getRevoked() == null) ? 0 : getRevoked().hashCode());
hashCode = prime * hashCode + ((getRevokedAt() == null) ? 0 : getRevokedAt().hashCode());
return hashCode;
}
@Override
public RevokeRevisionResult clone() {
try {
return (RevokeRevisionResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}