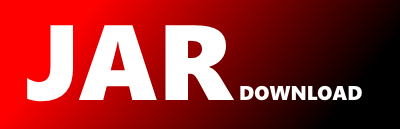
com.amazonaws.services.dataexchange.model.S3DataAccessAssetSourceEntry Maven / Gradle / Ivy
Show all versions of aws-java-sdk-dataexchange Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.dataexchange.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Source details for an Amazon S3 data access asset.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class S3DataAccessAssetSourceEntry implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon S3 bucket used for hosting shared data in the Amazon S3 data access.
*
*/
private String bucket;
/**
*
* Organizes Amazon S3 asset key prefixes stored in an Amazon S3 bucket.
*
*/
private java.util.List keyPrefixes;
/**
*
* The keys used to create the Amazon S3 data access.
*
*/
private java.util.List keys;
/**
*
* List of AWS KMS CMKs (Key Management System Customer Managed Keys) and ARNs used to encrypt S3 objects being
* shared in this S3 Data Access asset.
*
*/
private java.util.List kmsKeysToGrant;
/**
*
* The Amazon S3 bucket used for hosting shared data in the Amazon S3 data access.
*
*
* @param bucket
* The Amazon S3 bucket used for hosting shared data in the Amazon S3 data access.
*/
public void setBucket(String bucket) {
this.bucket = bucket;
}
/**
*
* The Amazon S3 bucket used for hosting shared data in the Amazon S3 data access.
*
*
* @return The Amazon S3 bucket used for hosting shared data in the Amazon S3 data access.
*/
public String getBucket() {
return this.bucket;
}
/**
*
* The Amazon S3 bucket used for hosting shared data in the Amazon S3 data access.
*
*
* @param bucket
* The Amazon S3 bucket used for hosting shared data in the Amazon S3 data access.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3DataAccessAssetSourceEntry withBucket(String bucket) {
setBucket(bucket);
return this;
}
/**
*
* Organizes Amazon S3 asset key prefixes stored in an Amazon S3 bucket.
*
*
* @return Organizes Amazon S3 asset key prefixes stored in an Amazon S3 bucket.
*/
public java.util.List getKeyPrefixes() {
return keyPrefixes;
}
/**
*
* Organizes Amazon S3 asset key prefixes stored in an Amazon S3 bucket.
*
*
* @param keyPrefixes
* Organizes Amazon S3 asset key prefixes stored in an Amazon S3 bucket.
*/
public void setKeyPrefixes(java.util.Collection keyPrefixes) {
if (keyPrefixes == null) {
this.keyPrefixes = null;
return;
}
this.keyPrefixes = new java.util.ArrayList(keyPrefixes);
}
/**
*
* Organizes Amazon S3 asset key prefixes stored in an Amazon S3 bucket.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setKeyPrefixes(java.util.Collection)} or {@link #withKeyPrefixes(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param keyPrefixes
* Organizes Amazon S3 asset key prefixes stored in an Amazon S3 bucket.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3DataAccessAssetSourceEntry withKeyPrefixes(String... keyPrefixes) {
if (this.keyPrefixes == null) {
setKeyPrefixes(new java.util.ArrayList(keyPrefixes.length));
}
for (String ele : keyPrefixes) {
this.keyPrefixes.add(ele);
}
return this;
}
/**
*
* Organizes Amazon S3 asset key prefixes stored in an Amazon S3 bucket.
*
*
* @param keyPrefixes
* Organizes Amazon S3 asset key prefixes stored in an Amazon S3 bucket.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3DataAccessAssetSourceEntry withKeyPrefixes(java.util.Collection keyPrefixes) {
setKeyPrefixes(keyPrefixes);
return this;
}
/**
*
* The keys used to create the Amazon S3 data access.
*
*
* @return The keys used to create the Amazon S3 data access.
*/
public java.util.List getKeys() {
return keys;
}
/**
*
* The keys used to create the Amazon S3 data access.
*
*
* @param keys
* The keys used to create the Amazon S3 data access.
*/
public void setKeys(java.util.Collection keys) {
if (keys == null) {
this.keys = null;
return;
}
this.keys = new java.util.ArrayList(keys);
}
/**
*
* The keys used to create the Amazon S3 data access.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setKeys(java.util.Collection)} or {@link #withKeys(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param keys
* The keys used to create the Amazon S3 data access.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3DataAccessAssetSourceEntry withKeys(String... keys) {
if (this.keys == null) {
setKeys(new java.util.ArrayList(keys.length));
}
for (String ele : keys) {
this.keys.add(ele);
}
return this;
}
/**
*
* The keys used to create the Amazon S3 data access.
*
*
* @param keys
* The keys used to create the Amazon S3 data access.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3DataAccessAssetSourceEntry withKeys(java.util.Collection keys) {
setKeys(keys);
return this;
}
/**
*
* List of AWS KMS CMKs (Key Management System Customer Managed Keys) and ARNs used to encrypt S3 objects being
* shared in this S3 Data Access asset.
*
*
* @return List of AWS KMS CMKs (Key Management System Customer Managed Keys) and ARNs used to encrypt S3 objects
* being shared in this S3 Data Access asset.
*/
public java.util.List getKmsKeysToGrant() {
return kmsKeysToGrant;
}
/**
*
* List of AWS KMS CMKs (Key Management System Customer Managed Keys) and ARNs used to encrypt S3 objects being
* shared in this S3 Data Access asset.
*
*
* @param kmsKeysToGrant
* List of AWS KMS CMKs (Key Management System Customer Managed Keys) and ARNs used to encrypt S3 objects
* being shared in this S3 Data Access asset.
*/
public void setKmsKeysToGrant(java.util.Collection kmsKeysToGrant) {
if (kmsKeysToGrant == null) {
this.kmsKeysToGrant = null;
return;
}
this.kmsKeysToGrant = new java.util.ArrayList(kmsKeysToGrant);
}
/**
*
* List of AWS KMS CMKs (Key Management System Customer Managed Keys) and ARNs used to encrypt S3 objects being
* shared in this S3 Data Access asset.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setKmsKeysToGrant(java.util.Collection)} or {@link #withKmsKeysToGrant(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param kmsKeysToGrant
* List of AWS KMS CMKs (Key Management System Customer Managed Keys) and ARNs used to encrypt S3 objects
* being shared in this S3 Data Access asset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3DataAccessAssetSourceEntry withKmsKeysToGrant(KmsKeyToGrant... kmsKeysToGrant) {
if (this.kmsKeysToGrant == null) {
setKmsKeysToGrant(new java.util.ArrayList(kmsKeysToGrant.length));
}
for (KmsKeyToGrant ele : kmsKeysToGrant) {
this.kmsKeysToGrant.add(ele);
}
return this;
}
/**
*
* List of AWS KMS CMKs (Key Management System Customer Managed Keys) and ARNs used to encrypt S3 objects being
* shared in this S3 Data Access asset.
*
*
* @param kmsKeysToGrant
* List of AWS KMS CMKs (Key Management System Customer Managed Keys) and ARNs used to encrypt S3 objects
* being shared in this S3 Data Access asset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3DataAccessAssetSourceEntry withKmsKeysToGrant(java.util.Collection kmsKeysToGrant) {
setKmsKeysToGrant(kmsKeysToGrant);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getBucket() != null)
sb.append("Bucket: ").append(getBucket()).append(",");
if (getKeyPrefixes() != null)
sb.append("KeyPrefixes: ").append(getKeyPrefixes()).append(",");
if (getKeys() != null)
sb.append("Keys: ").append(getKeys()).append(",");
if (getKmsKeysToGrant() != null)
sb.append("KmsKeysToGrant: ").append(getKmsKeysToGrant());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof S3DataAccessAssetSourceEntry == false)
return false;
S3DataAccessAssetSourceEntry other = (S3DataAccessAssetSourceEntry) obj;
if (other.getBucket() == null ^ this.getBucket() == null)
return false;
if (other.getBucket() != null && other.getBucket().equals(this.getBucket()) == false)
return false;
if (other.getKeyPrefixes() == null ^ this.getKeyPrefixes() == null)
return false;
if (other.getKeyPrefixes() != null && other.getKeyPrefixes().equals(this.getKeyPrefixes()) == false)
return false;
if (other.getKeys() == null ^ this.getKeys() == null)
return false;
if (other.getKeys() != null && other.getKeys().equals(this.getKeys()) == false)
return false;
if (other.getKmsKeysToGrant() == null ^ this.getKmsKeysToGrant() == null)
return false;
if (other.getKmsKeysToGrant() != null && other.getKmsKeysToGrant().equals(this.getKmsKeysToGrant()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getBucket() == null) ? 0 : getBucket().hashCode());
hashCode = prime * hashCode + ((getKeyPrefixes() == null) ? 0 : getKeyPrefixes().hashCode());
hashCode = prime * hashCode + ((getKeys() == null) ? 0 : getKeys().hashCode());
hashCode = prime * hashCode + ((getKmsKeysToGrant() == null) ? 0 : getKmsKeysToGrant().hashCode());
return hashCode;
}
@Override
public S3DataAccessAssetSourceEntry clone() {
try {
return (S3DataAccessAssetSourceEntry) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.dataexchange.model.transform.S3DataAccessAssetSourceEntryMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}