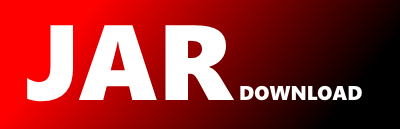
com.amazonaws.services.datapipeline.model.PollForTaskRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-datapipeline Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.datapipeline.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* Contains the parameters for PollForTask.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PollForTaskRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The type of task the task runner is configured to accept and process. The worker group is set as a field on
* objects in the pipeline when they are created. You can only specify a single value for workerGroup
* in the call to PollForTask
. There are no wildcard values permitted in workerGroup
; the
* string must be an exact, case-sensitive, match.
*
*/
private String workerGroup;
/**
*
* The public DNS name of the calling task runner.
*
*/
private String hostname;
/**
*
* Identity information for the EC2 instance that is hosting the task runner. You can get this value from the
* instance using http://169.254.169.254/latest/meta-data/instance-id
. For more information, see Instance Metadata
* in the Amazon Elastic Compute Cloud User Guide. Passing in this value proves that your task runner is
* running on an EC2 instance, and ensures the proper AWS Data Pipeline service charges are applied to your
* pipeline.
*
*/
private InstanceIdentity instanceIdentity;
/**
*
* The type of task the task runner is configured to accept and process. The worker group is set as a field on
* objects in the pipeline when they are created. You can only specify a single value for workerGroup
* in the call to PollForTask
. There are no wildcard values permitted in workerGroup
; the
* string must be an exact, case-sensitive, match.
*
*
* @param workerGroup
* The type of task the task runner is configured to accept and process. The worker group is set as a field
* on objects in the pipeline when they are created. You can only specify a single value for
* workerGroup
in the call to PollForTask
. There are no wildcard values permitted
* in workerGroup
; the string must be an exact, case-sensitive, match.
*/
public void setWorkerGroup(String workerGroup) {
this.workerGroup = workerGroup;
}
/**
*
* The type of task the task runner is configured to accept and process. The worker group is set as a field on
* objects in the pipeline when they are created. You can only specify a single value for workerGroup
* in the call to PollForTask
. There are no wildcard values permitted in workerGroup
; the
* string must be an exact, case-sensitive, match.
*
*
* @return The type of task the task runner is configured to accept and process. The worker group is set as a field
* on objects in the pipeline when they are created. You can only specify a single value for
* workerGroup
in the call to PollForTask
. There are no wildcard values permitted
* in workerGroup
; the string must be an exact, case-sensitive, match.
*/
public String getWorkerGroup() {
return this.workerGroup;
}
/**
*
* The type of task the task runner is configured to accept and process. The worker group is set as a field on
* objects in the pipeline when they are created. You can only specify a single value for workerGroup
* in the call to PollForTask
. There are no wildcard values permitted in workerGroup
; the
* string must be an exact, case-sensitive, match.
*
*
* @param workerGroup
* The type of task the task runner is configured to accept and process. The worker group is set as a field
* on objects in the pipeline when they are created. You can only specify a single value for
* workerGroup
in the call to PollForTask
. There are no wildcard values permitted
* in workerGroup
; the string must be an exact, case-sensitive, match.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PollForTaskRequest withWorkerGroup(String workerGroup) {
setWorkerGroup(workerGroup);
return this;
}
/**
*
* The public DNS name of the calling task runner.
*
*
* @param hostname
* The public DNS name of the calling task runner.
*/
public void setHostname(String hostname) {
this.hostname = hostname;
}
/**
*
* The public DNS name of the calling task runner.
*
*
* @return The public DNS name of the calling task runner.
*/
public String getHostname() {
return this.hostname;
}
/**
*
* The public DNS name of the calling task runner.
*
*
* @param hostname
* The public DNS name of the calling task runner.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PollForTaskRequest withHostname(String hostname) {
setHostname(hostname);
return this;
}
/**
*
* Identity information for the EC2 instance that is hosting the task runner. You can get this value from the
* instance using http://169.254.169.254/latest/meta-data/instance-id
. For more information, see Instance Metadata
* in the Amazon Elastic Compute Cloud User Guide. Passing in this value proves that your task runner is
* running on an EC2 instance, and ensures the proper AWS Data Pipeline service charges are applied to your
* pipeline.
*
*
* @param instanceIdentity
* Identity information for the EC2 instance that is hosting the task runner. You can get this value from the
* instance using http://169.254.169.254/latest/meta-data/instance-id
. For more information, see
* Instance
* Metadata in the Amazon Elastic Compute Cloud User Guide. Passing in this value proves that your
* task runner is running on an EC2 instance, and ensures the proper AWS Data Pipeline service charges are
* applied to your pipeline.
*/
public void setInstanceIdentity(InstanceIdentity instanceIdentity) {
this.instanceIdentity = instanceIdentity;
}
/**
*
* Identity information for the EC2 instance that is hosting the task runner. You can get this value from the
* instance using http://169.254.169.254/latest/meta-data/instance-id
. For more information, see Instance Metadata
* in the Amazon Elastic Compute Cloud User Guide. Passing in this value proves that your task runner is
* running on an EC2 instance, and ensures the proper AWS Data Pipeline service charges are applied to your
* pipeline.
*
*
* @return Identity information for the EC2 instance that is hosting the task runner. You can get this value from
* the instance using http://169.254.169.254/latest/meta-data/instance-id
. For more
* information, see Instance
* Metadata in the Amazon Elastic Compute Cloud User Guide. Passing in this value proves that
* your task runner is running on an EC2 instance, and ensures the proper AWS Data Pipeline service charges
* are applied to your pipeline.
*/
public InstanceIdentity getInstanceIdentity() {
return this.instanceIdentity;
}
/**
*
* Identity information for the EC2 instance that is hosting the task runner. You can get this value from the
* instance using http://169.254.169.254/latest/meta-data/instance-id
. For more information, see Instance Metadata
* in the Amazon Elastic Compute Cloud User Guide. Passing in this value proves that your task runner is
* running on an EC2 instance, and ensures the proper AWS Data Pipeline service charges are applied to your
* pipeline.
*
*
* @param instanceIdentity
* Identity information for the EC2 instance that is hosting the task runner. You can get this value from the
* instance using http://169.254.169.254/latest/meta-data/instance-id
. For more information, see
* Instance
* Metadata in the Amazon Elastic Compute Cloud User Guide. Passing in this value proves that your
* task runner is running on an EC2 instance, and ensures the proper AWS Data Pipeline service charges are
* applied to your pipeline.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PollForTaskRequest withInstanceIdentity(InstanceIdentity instanceIdentity) {
setInstanceIdentity(instanceIdentity);
return this;
}
/**
* Returns a string representation of this object; useful for testing and debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getWorkerGroup() != null)
sb.append("WorkerGroup: ").append(getWorkerGroup()).append(",");
if (getHostname() != null)
sb.append("Hostname: ").append(getHostname()).append(",");
if (getInstanceIdentity() != null)
sb.append("InstanceIdentity: ").append(getInstanceIdentity());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PollForTaskRequest == false)
return false;
PollForTaskRequest other = (PollForTaskRequest) obj;
if (other.getWorkerGroup() == null ^ this.getWorkerGroup() == null)
return false;
if (other.getWorkerGroup() != null && other.getWorkerGroup().equals(this.getWorkerGroup()) == false)
return false;
if (other.getHostname() == null ^ this.getHostname() == null)
return false;
if (other.getHostname() != null && other.getHostname().equals(this.getHostname()) == false)
return false;
if (other.getInstanceIdentity() == null ^ this.getInstanceIdentity() == null)
return false;
if (other.getInstanceIdentity() != null && other.getInstanceIdentity().equals(this.getInstanceIdentity()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getWorkerGroup() == null) ? 0 : getWorkerGroup().hashCode());
hashCode = prime * hashCode + ((getHostname() == null) ? 0 : getHostname().hashCode());
hashCode = prime * hashCode + ((getInstanceIdentity() == null) ? 0 : getInstanceIdentity().hashCode());
return hashCode;
}
@Override
public PollForTaskRequest clone() {
return (PollForTaskRequest) super.clone();
}
}