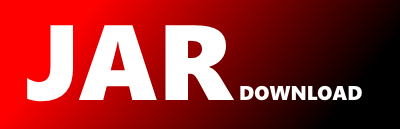
com.amazonaws.services.datasync.AWSDataSyncAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-datasync Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.datasync;
import javax.annotation.Generated;
import com.amazonaws.services.datasync.model.*;
/**
* Interface for accessing DataSync asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.datasync.AbstractAWSDataSyncAsync} instead.
*
*
* DataSync
*
* DataSync is a managed data transfer service that makes it simpler for you to automate moving data between on-premises
* storage and Amazon Simple Storage Service (Amazon S3) or Amazon Elastic File System (Amazon EFS).
*
*
* This API interface reference for DataSync contains documentation for a programming interface that you can use to
* manage DataSync.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSDataSyncAsync extends AWSDataSync {
/**
*
* Cancels execution of a task.
*
*
* When you cancel a task execution, the transfer of some files is abruptly interrupted. The contents of files that
* are transferred to the destination might be incomplete or inconsistent with the source files. However, if you
* start a new task execution on the same task and you allow the task execution to complete, file content on the
* destination is complete and consistent. This applies to other unexpected failures that interrupt a task
* execution. In all of these cases, DataSync successfully complete the transfer when you start the next task
* execution.
*
*
* @param cancelTaskExecutionRequest
* CancelTaskExecutionRequest
* @return A Java Future containing the result of the CancelTaskExecution operation returned by the service.
* @sample AWSDataSyncAsync.CancelTaskExecution
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelTaskExecutionAsync(CancelTaskExecutionRequest cancelTaskExecutionRequest);
/**
*
* Cancels execution of a task.
*
*
* When you cancel a task execution, the transfer of some files is abruptly interrupted. The contents of files that
* are transferred to the destination might be incomplete or inconsistent with the source files. However, if you
* start a new task execution on the same task and you allow the task execution to complete, file content on the
* destination is complete and consistent. This applies to other unexpected failures that interrupt a task
* execution. In all of these cases, DataSync successfully complete the transfer when you start the next task
* execution.
*
*
* @param cancelTaskExecutionRequest
* CancelTaskExecutionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelTaskExecution operation returned by the service.
* @sample AWSDataSyncAsyncHandler.CancelTaskExecution
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelTaskExecutionAsync(CancelTaskExecutionRequest cancelTaskExecutionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Activates an DataSync agent that you have deployed on your host. The activation process associates your agent
* with your account. In the activation process, you specify information such as the Amazon Web Services Region that
* you want to activate the agent in. You activate the agent in the Amazon Web Services Region where your target
* locations (in Amazon S3 or Amazon EFS) reside. Your tasks are created in this Amazon Web Services Region.
*
*
* You can activate the agent in a VPC (virtual private cloud) or provide the agent access to a VPC endpoint so you
* can run tasks without going over the public internet.
*
*
* You can use an agent for more than one location. If a task uses multiple agents, all of them need to have status
* AVAILABLE for the task to run. If you use multiple agents for a source location, the status of all the agents
* must be AVAILABLE for the task to run.
*
*
* Agents are automatically updated by Amazon Web Services on a regular basis, using a mechanism that ensures
* minimal interruption to your tasks.
*
*
*
* @param createAgentRequest
* CreateAgentRequest
* @return A Java Future containing the result of the CreateAgent operation returned by the service.
* @sample AWSDataSyncAsync.CreateAgent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAgentAsync(CreateAgentRequest createAgentRequest);
/**
*
* Activates an DataSync agent that you have deployed on your host. The activation process associates your agent
* with your account. In the activation process, you specify information such as the Amazon Web Services Region that
* you want to activate the agent in. You activate the agent in the Amazon Web Services Region where your target
* locations (in Amazon S3 or Amazon EFS) reside. Your tasks are created in this Amazon Web Services Region.
*
*
* You can activate the agent in a VPC (virtual private cloud) or provide the agent access to a VPC endpoint so you
* can run tasks without going over the public internet.
*
*
* You can use an agent for more than one location. If a task uses multiple agents, all of them need to have status
* AVAILABLE for the task to run. If you use multiple agents for a source location, the status of all the agents
* must be AVAILABLE for the task to run.
*
*
* Agents are automatically updated by Amazon Web Services on a regular basis, using a mechanism that ensures
* minimal interruption to your tasks.
*
*
*
* @param createAgentRequest
* CreateAgentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAgent operation returned by the service.
* @sample AWSDataSyncAsyncHandler.CreateAgent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAgentAsync(CreateAgentRequest createAgentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an endpoint for an Amazon EFS file system.
*
*
* @param createLocationEfsRequest
* CreateLocationEfsRequest
* @return A Java Future containing the result of the CreateLocationEfs operation returned by the service.
* @sample AWSDataSyncAsync.CreateLocationEfs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createLocationEfsAsync(CreateLocationEfsRequest createLocationEfsRequest);
/**
*
* Creates an endpoint for an Amazon EFS file system.
*
*
* @param createLocationEfsRequest
* CreateLocationEfsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLocationEfs operation returned by the service.
* @sample AWSDataSyncAsyncHandler.CreateLocationEfs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createLocationEfsAsync(CreateLocationEfsRequest createLocationEfsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an endpoint for an Amazon FSx for Lustre file system.
*
*
* @param createLocationFsxLustreRequest
* @return A Java Future containing the result of the CreateLocationFsxLustre operation returned by the service.
* @sample AWSDataSyncAsync.CreateLocationFsxLustre
* @see AWS API Documentation
*/
java.util.concurrent.Future createLocationFsxLustreAsync(CreateLocationFsxLustreRequest createLocationFsxLustreRequest);
/**
*
* Creates an endpoint for an Amazon FSx for Lustre file system.
*
*
* @param createLocationFsxLustreRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLocationFsxLustre operation returned by the service.
* @sample AWSDataSyncAsyncHandler.CreateLocationFsxLustre
* @see AWS API Documentation
*/
java.util.concurrent.Future createLocationFsxLustreAsync(CreateLocationFsxLustreRequest createLocationFsxLustreRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an endpoint for an Amazon FSx for Windows File Server file system.
*
*
* @param createLocationFsxWindowsRequest
* @return A Java Future containing the result of the CreateLocationFsxWindows operation returned by the service.
* @sample AWSDataSyncAsync.CreateLocationFsxWindows
* @see AWS API Documentation
*/
java.util.concurrent.Future createLocationFsxWindowsAsync(CreateLocationFsxWindowsRequest createLocationFsxWindowsRequest);
/**
*
* Creates an endpoint for an Amazon FSx for Windows File Server file system.
*
*
* @param createLocationFsxWindowsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLocationFsxWindows operation returned by the service.
* @sample AWSDataSyncAsyncHandler.CreateLocationFsxWindows
* @see AWS API Documentation
*/
java.util.concurrent.Future createLocationFsxWindowsAsync(CreateLocationFsxWindowsRequest createLocationFsxWindowsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an endpoint for a Hadoop Distributed File System (HDFS).
*
*
* @param createLocationHdfsRequest
* @return A Java Future containing the result of the CreateLocationHdfs operation returned by the service.
* @sample AWSDataSyncAsync.CreateLocationHdfs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createLocationHdfsAsync(CreateLocationHdfsRequest createLocationHdfsRequest);
/**
*
* Creates an endpoint for a Hadoop Distributed File System (HDFS).
*
*
* @param createLocationHdfsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLocationHdfs operation returned by the service.
* @sample AWSDataSyncAsyncHandler.CreateLocationHdfs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createLocationHdfsAsync(CreateLocationHdfsRequest createLocationHdfsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Defines a file system on a Network File System (NFS) server that can be read from or written to.
*
*
* @param createLocationNfsRequest
* CreateLocationNfsRequest
* @return A Java Future containing the result of the CreateLocationNfs operation returned by the service.
* @sample AWSDataSyncAsync.CreateLocationNfs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createLocationNfsAsync(CreateLocationNfsRequest createLocationNfsRequest);
/**
*
* Defines a file system on a Network File System (NFS) server that can be read from or written to.
*
*
* @param createLocationNfsRequest
* CreateLocationNfsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLocationNfs operation returned by the service.
* @sample AWSDataSyncAsyncHandler.CreateLocationNfs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createLocationNfsAsync(CreateLocationNfsRequest createLocationNfsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an endpoint for a self-managed object storage bucket. For more information about self-managed object
* storage locations, see Creating a location for
* object storage.
*
*
* @param createLocationObjectStorageRequest
* CreateLocationObjectStorageRequest
* @return A Java Future containing the result of the CreateLocationObjectStorage operation returned by the service.
* @sample AWSDataSyncAsync.CreateLocationObjectStorage
* @see AWS API Documentation
*/
java.util.concurrent.Future createLocationObjectStorageAsync(
CreateLocationObjectStorageRequest createLocationObjectStorageRequest);
/**
*
* Creates an endpoint for a self-managed object storage bucket. For more information about self-managed object
* storage locations, see Creating a location for
* object storage.
*
*
* @param createLocationObjectStorageRequest
* CreateLocationObjectStorageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLocationObjectStorage operation returned by the service.
* @sample AWSDataSyncAsyncHandler.CreateLocationObjectStorage
* @see AWS API Documentation
*/
java.util.concurrent.Future createLocationObjectStorageAsync(
CreateLocationObjectStorageRequest createLocationObjectStorageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an endpoint for an Amazon S3 bucket.
*
*
* For more information, see Create an Amazon S3 location in the DataSync User Guide.
*
*
* @param createLocationS3Request
* CreateLocationS3Request
* @return A Java Future containing the result of the CreateLocationS3 operation returned by the service.
* @sample AWSDataSyncAsync.CreateLocationS3
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createLocationS3Async(CreateLocationS3Request createLocationS3Request);
/**
*
* Creates an endpoint for an Amazon S3 bucket.
*
*
* For more information, see Create an Amazon S3 location in the DataSync User Guide.
*
*
* @param createLocationS3Request
* CreateLocationS3Request
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLocationS3 operation returned by the service.
* @sample AWSDataSyncAsyncHandler.CreateLocationS3
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createLocationS3Async(CreateLocationS3Request createLocationS3Request,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Defines a file system on a Server Message Block (SMB) server that can be read from or written to.
*
*
* @param createLocationSmbRequest
* CreateLocationSmbRequest
* @return A Java Future containing the result of the CreateLocationSmb operation returned by the service.
* @sample AWSDataSyncAsync.CreateLocationSmb
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createLocationSmbAsync(CreateLocationSmbRequest createLocationSmbRequest);
/**
*
* Defines a file system on a Server Message Block (SMB) server that can be read from or written to.
*
*
* @param createLocationSmbRequest
* CreateLocationSmbRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLocationSmb operation returned by the service.
* @sample AWSDataSyncAsyncHandler.CreateLocationSmb
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createLocationSmbAsync(CreateLocationSmbRequest createLocationSmbRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a task.
*
*
* A task includes a source location and a destination location, and a configuration that specifies how data is
* transferred. A task always transfers data from the source location to the destination location. The configuration
* specifies options such as task scheduling, bandwidth limits, etc. A task is the complete definition of a data
* transfer.
*
*
* When you create a task that transfers data between Amazon Web Services services in different Amazon Web Services
* Regions, one of the two locations that you specify must reside in the Region where DataSync is being used. The
* other location must be specified in a different Region.
*
*
* You can transfer data between commercial Amazon Web Services Regions except for China, or between Amazon Web
* Services GovCloud (US) Regions.
*
*
*
* When you use DataSync to copy files or objects between Amazon Web Services Regions, you pay for data transfer
* between Regions. This is billed as data transfer OUT from your source Region to your destination Region. For more
* information, see Data Transfer pricing.
*
*
*
* @param createTaskRequest
* CreateTaskRequest
* @return A Java Future containing the result of the CreateTask operation returned by the service.
* @sample AWSDataSyncAsync.CreateTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTaskAsync(CreateTaskRequest createTaskRequest);
/**
*
* Creates a task.
*
*
* A task includes a source location and a destination location, and a configuration that specifies how data is
* transferred. A task always transfers data from the source location to the destination location. The configuration
* specifies options such as task scheduling, bandwidth limits, etc. A task is the complete definition of a data
* transfer.
*
*
* When you create a task that transfers data between Amazon Web Services services in different Amazon Web Services
* Regions, one of the two locations that you specify must reside in the Region where DataSync is being used. The
* other location must be specified in a different Region.
*
*
* You can transfer data between commercial Amazon Web Services Regions except for China, or between Amazon Web
* Services GovCloud (US) Regions.
*
*
*
* When you use DataSync to copy files or objects between Amazon Web Services Regions, you pay for data transfer
* between Regions. This is billed as data transfer OUT from your source Region to your destination Region. For more
* information, see Data Transfer pricing.
*
*
*
* @param createTaskRequest
* CreateTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTask operation returned by the service.
* @sample AWSDataSyncAsyncHandler.CreateTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTaskAsync(CreateTaskRequest createTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an agent. To specify which agent to delete, use the Amazon Resource Name (ARN) of the agent in your
* request. The operation disassociates the agent from your Amazon Web Services account. However, it doesn't delete
* the agent virtual machine (VM) from your on-premises environment.
*
*
* @param deleteAgentRequest
* DeleteAgentRequest
* @return A Java Future containing the result of the DeleteAgent operation returned by the service.
* @sample AWSDataSyncAsync.DeleteAgent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAgentAsync(DeleteAgentRequest deleteAgentRequest);
/**
*
* Deletes an agent. To specify which agent to delete, use the Amazon Resource Name (ARN) of the agent in your
* request. The operation disassociates the agent from your Amazon Web Services account. However, it doesn't delete
* the agent virtual machine (VM) from your on-premises environment.
*
*
* @param deleteAgentRequest
* DeleteAgentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAgent operation returned by the service.
* @sample AWSDataSyncAsyncHandler.DeleteAgent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAgentAsync(DeleteAgentRequest deleteAgentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the configuration of a location used by DataSync.
*
*
* @param deleteLocationRequest
* DeleteLocation
* @return A Java Future containing the result of the DeleteLocation operation returned by the service.
* @sample AWSDataSyncAsync.DeleteLocation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteLocationAsync(DeleteLocationRequest deleteLocationRequest);
/**
*
* Deletes the configuration of a location used by DataSync.
*
*
* @param deleteLocationRequest
* DeleteLocation
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteLocation operation returned by the service.
* @sample AWSDataSyncAsyncHandler.DeleteLocation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteLocationAsync(DeleteLocationRequest deleteLocationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a task.
*
*
* @param deleteTaskRequest
* DeleteTask
* @return A Java Future containing the result of the DeleteTask operation returned by the service.
* @sample AWSDataSyncAsync.DeleteTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteTaskAsync(DeleteTaskRequest deleteTaskRequest);
/**
*
* Deletes a task.
*
*
* @param deleteTaskRequest
* DeleteTask
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTask operation returned by the service.
* @sample AWSDataSyncAsyncHandler.DeleteTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteTaskAsync(DeleteTaskRequest deleteTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns metadata such as the name, the network interfaces, and the status (that is, whether the agent is running
* or not) for an agent. To specify which agent to describe, use the Amazon Resource Name (ARN) of the agent in your
* request.
*
*
* @param describeAgentRequest
* DescribeAgent
* @return A Java Future containing the result of the DescribeAgent operation returned by the service.
* @sample AWSDataSyncAsync.DescribeAgent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeAgentAsync(DescribeAgentRequest describeAgentRequest);
/**
*
* Returns metadata such as the name, the network interfaces, and the status (that is, whether the agent is running
* or not) for an agent. To specify which agent to describe, use the Amazon Resource Name (ARN) of the agent in your
* request.
*
*
* @param describeAgentRequest
* DescribeAgent
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAgent operation returned by the service.
* @sample AWSDataSyncAsyncHandler.DescribeAgent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeAgentAsync(DescribeAgentRequest describeAgentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns metadata, such as the path information about an Amazon EFS location.
*
*
* @param describeLocationEfsRequest
* DescribeLocationEfsRequest
* @return A Java Future containing the result of the DescribeLocationEfs operation returned by the service.
* @sample AWSDataSyncAsync.DescribeLocationEfs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeLocationEfsAsync(DescribeLocationEfsRequest describeLocationEfsRequest);
/**
*
* Returns metadata, such as the path information about an Amazon EFS location.
*
*
* @param describeLocationEfsRequest
* DescribeLocationEfsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeLocationEfs operation returned by the service.
* @sample AWSDataSyncAsyncHandler.DescribeLocationEfs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeLocationEfsAsync(DescribeLocationEfsRequest describeLocationEfsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns metadata, such as the path information about an Amazon FSx for Lustre location.
*
*
* @param describeLocationFsxLustreRequest
* @return A Java Future containing the result of the DescribeLocationFsxLustre operation returned by the service.
* @sample AWSDataSyncAsync.DescribeLocationFsxLustre
* @see AWS API Documentation
*/
java.util.concurrent.Future describeLocationFsxLustreAsync(
DescribeLocationFsxLustreRequest describeLocationFsxLustreRequest);
/**
*
* Returns metadata, such as the path information about an Amazon FSx for Lustre location.
*
*
* @param describeLocationFsxLustreRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeLocationFsxLustre operation returned by the service.
* @sample AWSDataSyncAsyncHandler.DescribeLocationFsxLustre
* @see AWS API Documentation
*/
java.util.concurrent.Future describeLocationFsxLustreAsync(
DescribeLocationFsxLustreRequest describeLocationFsxLustreRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns metadata, such as the path information about an Amazon FSx for Windows File Server location.
*
*
* @param describeLocationFsxWindowsRequest
* @return A Java Future containing the result of the DescribeLocationFsxWindows operation returned by the service.
* @sample AWSDataSyncAsync.DescribeLocationFsxWindows
* @see AWS API Documentation
*/
java.util.concurrent.Future describeLocationFsxWindowsAsync(
DescribeLocationFsxWindowsRequest describeLocationFsxWindowsRequest);
/**
*
* Returns metadata, such as the path information about an Amazon FSx for Windows File Server location.
*
*
* @param describeLocationFsxWindowsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeLocationFsxWindows operation returned by the service.
* @sample AWSDataSyncAsyncHandler.DescribeLocationFsxWindows
* @see AWS API Documentation
*/
java.util.concurrent.Future describeLocationFsxWindowsAsync(
DescribeLocationFsxWindowsRequest describeLocationFsxWindowsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns metadata, such as the authentication information about the Hadoop Distributed File System (HDFS)
* location.
*
*
* @param describeLocationHdfsRequest
* @return A Java Future containing the result of the DescribeLocationHdfs operation returned by the service.
* @sample AWSDataSyncAsync.DescribeLocationHdfs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeLocationHdfsAsync(DescribeLocationHdfsRequest describeLocationHdfsRequest);
/**
*
* Returns metadata, such as the authentication information about the Hadoop Distributed File System (HDFS)
* location.
*
*
* @param describeLocationHdfsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeLocationHdfs operation returned by the service.
* @sample AWSDataSyncAsyncHandler.DescribeLocationHdfs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeLocationHdfsAsync(DescribeLocationHdfsRequest describeLocationHdfsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns metadata, such as the path information, about an NFS location.
*
*
* @param describeLocationNfsRequest
* DescribeLocationNfsRequest
* @return A Java Future containing the result of the DescribeLocationNfs operation returned by the service.
* @sample AWSDataSyncAsync.DescribeLocationNfs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeLocationNfsAsync(DescribeLocationNfsRequest describeLocationNfsRequest);
/**
*
* Returns metadata, such as the path information, about an NFS location.
*
*
* @param describeLocationNfsRequest
* DescribeLocationNfsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeLocationNfs operation returned by the service.
* @sample AWSDataSyncAsyncHandler.DescribeLocationNfs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeLocationNfsAsync(DescribeLocationNfsRequest describeLocationNfsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns metadata about a self-managed object storage server location. For more information about self-managed
* object storage locations, see Creating a location for
* object storage.
*
*
* @param describeLocationObjectStorageRequest
* DescribeLocationObjectStorageRequest
* @return A Java Future containing the result of the DescribeLocationObjectStorage operation returned by the
* service.
* @sample AWSDataSyncAsync.DescribeLocationObjectStorage
* @see AWS API Documentation
*/
java.util.concurrent.Future describeLocationObjectStorageAsync(
DescribeLocationObjectStorageRequest describeLocationObjectStorageRequest);
/**
*
* Returns metadata about a self-managed object storage server location. For more information about self-managed
* object storage locations, see Creating a location for
* object storage.
*
*
* @param describeLocationObjectStorageRequest
* DescribeLocationObjectStorageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeLocationObjectStorage operation returned by the
* service.
* @sample AWSDataSyncAsyncHandler.DescribeLocationObjectStorage
* @see AWS API Documentation
*/
java.util.concurrent.Future describeLocationObjectStorageAsync(
DescribeLocationObjectStorageRequest describeLocationObjectStorageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns metadata, such as bucket name, about an Amazon S3 bucket location.
*
*
* @param describeLocationS3Request
* DescribeLocationS3Request
* @return A Java Future containing the result of the DescribeLocationS3 operation returned by the service.
* @sample AWSDataSyncAsync.DescribeLocationS3
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeLocationS3Async(DescribeLocationS3Request describeLocationS3Request);
/**
*
* Returns metadata, such as bucket name, about an Amazon S3 bucket location.
*
*
* @param describeLocationS3Request
* DescribeLocationS3Request
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeLocationS3 operation returned by the service.
* @sample AWSDataSyncAsyncHandler.DescribeLocationS3
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeLocationS3Async(DescribeLocationS3Request describeLocationS3Request,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns metadata, such as the path and user information about an SMB location.
*
*
* @param describeLocationSmbRequest
* DescribeLocationSmbRequest
* @return A Java Future containing the result of the DescribeLocationSmb operation returned by the service.
* @sample AWSDataSyncAsync.DescribeLocationSmb
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeLocationSmbAsync(DescribeLocationSmbRequest describeLocationSmbRequest);
/**
*
* Returns metadata, such as the path and user information about an SMB location.
*
*
* @param describeLocationSmbRequest
* DescribeLocationSmbRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeLocationSmb operation returned by the service.
* @sample AWSDataSyncAsyncHandler.DescribeLocationSmb
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeLocationSmbAsync(DescribeLocationSmbRequest describeLocationSmbRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns metadata about a task.
*
*
* @param describeTaskRequest
* DescribeTaskRequest
* @return A Java Future containing the result of the DescribeTask operation returned by the service.
* @sample AWSDataSyncAsync.DescribeTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeTaskAsync(DescribeTaskRequest describeTaskRequest);
/**
*
* Returns metadata about a task.
*
*
* @param describeTaskRequest
* DescribeTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTask operation returned by the service.
* @sample AWSDataSyncAsyncHandler.DescribeTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeTaskAsync(DescribeTaskRequest describeTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns detailed metadata about a task that is being executed.
*
*
* @param describeTaskExecutionRequest
* DescribeTaskExecutionRequest
* @return A Java Future containing the result of the DescribeTaskExecution operation returned by the service.
* @sample AWSDataSyncAsync.DescribeTaskExecution
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeTaskExecutionAsync(DescribeTaskExecutionRequest describeTaskExecutionRequest);
/**
*
* Returns detailed metadata about a task that is being executed.
*
*
* @param describeTaskExecutionRequest
* DescribeTaskExecutionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTaskExecution operation returned by the service.
* @sample AWSDataSyncAsyncHandler.DescribeTaskExecution
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeTaskExecutionAsync(DescribeTaskExecutionRequest describeTaskExecutionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of agents owned by an Amazon Web Services account in the Amazon Web Services Region specified in
* the request. The returned list is ordered by agent Amazon Resource Name (ARN).
*
*
* By default, this operation returns a maximum of 100 agents. This operation supports pagination that enables you
* to optionally reduce the number of agents returned in a response.
*
*
* If you have more agents than are returned in a response (that is, the response returns only a truncated list of
* your agents), the response contains a marker that you can specify in your next request to fetch the next page of
* agents.
*
*
* @param listAgentsRequest
* ListAgentsRequest
* @return A Java Future containing the result of the ListAgents operation returned by the service.
* @sample AWSDataSyncAsync.ListAgents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAgentsAsync(ListAgentsRequest listAgentsRequest);
/**
*
* Returns a list of agents owned by an Amazon Web Services account in the Amazon Web Services Region specified in
* the request. The returned list is ordered by agent Amazon Resource Name (ARN).
*
*
* By default, this operation returns a maximum of 100 agents. This operation supports pagination that enables you
* to optionally reduce the number of agents returned in a response.
*
*
* If you have more agents than are returned in a response (that is, the response returns only a truncated list of
* your agents), the response contains a marker that you can specify in your next request to fetch the next page of
* agents.
*
*
* @param listAgentsRequest
* ListAgentsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAgents operation returned by the service.
* @sample AWSDataSyncAsyncHandler.ListAgents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAgentsAsync(ListAgentsRequest listAgentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of source and destination locations.
*
*
* If you have more locations than are returned in a response (that is, the response returns only a truncated list
* of your agents), the response contains a token that you can specify in your next request to fetch the next page
* of locations.
*
*
* @param listLocationsRequest
* ListLocationsRequest
* @return A Java Future containing the result of the ListLocations operation returned by the service.
* @sample AWSDataSyncAsync.ListLocations
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listLocationsAsync(ListLocationsRequest listLocationsRequest);
/**
*
* Returns a list of source and destination locations.
*
*
* If you have more locations than are returned in a response (that is, the response returns only a truncated list
* of your agents), the response contains a token that you can specify in your next request to fetch the next page
* of locations.
*
*
* @param listLocationsRequest
* ListLocationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListLocations operation returned by the service.
* @sample AWSDataSyncAsyncHandler.ListLocations
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listLocationsAsync(ListLocationsRequest listLocationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns all the tags associated with a specified resource.
*
*
* @param listTagsForResourceRequest
* ListTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSDataSyncAsync.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Returns all the tags associated with a specified resource.
*
*
* @param listTagsForResourceRequest
* ListTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSDataSyncAsyncHandler.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of executed tasks.
*
*
* @param listTaskExecutionsRequest
* ListTaskExecutions
* @return A Java Future containing the result of the ListTaskExecutions operation returned by the service.
* @sample AWSDataSyncAsync.ListTaskExecutions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTaskExecutionsAsync(ListTaskExecutionsRequest listTaskExecutionsRequest);
/**
*
* Returns a list of executed tasks.
*
*
* @param listTaskExecutionsRequest
* ListTaskExecutions
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTaskExecutions operation returned by the service.
* @sample AWSDataSyncAsyncHandler.ListTaskExecutions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTaskExecutionsAsync(ListTaskExecutionsRequest listTaskExecutionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all the tasks.
*
*
* @param listTasksRequest
* ListTasksRequest
* @return A Java Future containing the result of the ListTasks operation returned by the service.
* @sample AWSDataSyncAsync.ListTasks
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTasksAsync(ListTasksRequest listTasksRequest);
/**
*
* Returns a list of all the tasks.
*
*
* @param listTasksRequest
* ListTasksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTasks operation returned by the service.
* @sample AWSDataSyncAsyncHandler.ListTasks
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTasksAsync(ListTasksRequest listTasksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts a specific invocation of a task. A TaskExecution
value represents an individual run of a
* task. Each task can have at most one TaskExecution
at a time.
*
*
* TaskExecution
has the following transition phases: INITIALIZING | PREPARING | TRANSFERRING |
* VERIFYING | SUCCESS/FAILURE.
*
*
* For detailed information, see the Task Execution section in the Components and Terminology topic in the
* DataSync User Guide.
*
*
* @param startTaskExecutionRequest
* StartTaskExecutionRequest
* @return A Java Future containing the result of the StartTaskExecution operation returned by the service.
* @sample AWSDataSyncAsync.StartTaskExecution
* @see AWS
* API Documentation
*/
java.util.concurrent.Future startTaskExecutionAsync(StartTaskExecutionRequest startTaskExecutionRequest);
/**
*
* Starts a specific invocation of a task. A TaskExecution
value represents an individual run of a
* task. Each task can have at most one TaskExecution
at a time.
*
*
* TaskExecution
has the following transition phases: INITIALIZING | PREPARING | TRANSFERRING |
* VERIFYING | SUCCESS/FAILURE.
*
*
* For detailed information, see the Task Execution section in the Components and Terminology topic in the
* DataSync User Guide.
*
*
* @param startTaskExecutionRequest
* StartTaskExecutionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartTaskExecution operation returned by the service.
* @sample AWSDataSyncAsyncHandler.StartTaskExecution
* @see AWS
* API Documentation
*/
java.util.concurrent.Future startTaskExecutionAsync(StartTaskExecutionRequest startTaskExecutionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Applies a key-value pair to an Amazon Web Services resource.
*
*
* @param tagResourceRequest
* TagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSDataSyncAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Applies a key-value pair to an Amazon Web Services resource.
*
*
* @param tagResourceRequest
* TagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSDataSyncAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a tag from an Amazon Web Services resource.
*
*
* @param untagResourceRequest
* UntagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSDataSyncAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes a tag from an Amazon Web Services resource.
*
*
* @param untagResourceRequest
* UntagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSDataSyncAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the name of an agent.
*
*
* @param updateAgentRequest
* UpdateAgentRequest
* @return A Java Future containing the result of the UpdateAgent operation returned by the service.
* @sample AWSDataSyncAsync.UpdateAgent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateAgentAsync(UpdateAgentRequest updateAgentRequest);
/**
*
* Updates the name of an agent.
*
*
* @param updateAgentRequest
* UpdateAgentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAgent operation returned by the service.
* @sample AWSDataSyncAsyncHandler.UpdateAgent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateAgentAsync(UpdateAgentRequest updateAgentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates some parameters of a previously created location for a Hadoop Distributed File System cluster.
*
*
* @param updateLocationHdfsRequest
* @return A Java Future containing the result of the UpdateLocationHdfs operation returned by the service.
* @sample AWSDataSyncAsync.UpdateLocationHdfs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateLocationHdfsAsync(UpdateLocationHdfsRequest updateLocationHdfsRequest);
/**
*
* Updates some parameters of a previously created location for a Hadoop Distributed File System cluster.
*
*
* @param updateLocationHdfsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateLocationHdfs operation returned by the service.
* @sample AWSDataSyncAsyncHandler.UpdateLocationHdfs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateLocationHdfsAsync(UpdateLocationHdfsRequest updateLocationHdfsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates some of the parameters of a previously created location for Network File System (NFS) access. For
* information about creating an NFS location, see Creating a location for
* NFS.
*
*
* @param updateLocationNfsRequest
* @return A Java Future containing the result of the UpdateLocationNfs operation returned by the service.
* @sample AWSDataSyncAsync.UpdateLocationNfs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateLocationNfsAsync(UpdateLocationNfsRequest updateLocationNfsRequest);
/**
*
* Updates some of the parameters of a previously created location for Network File System (NFS) access. For
* information about creating an NFS location, see Creating a location for
* NFS.
*
*
* @param updateLocationNfsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateLocationNfs operation returned by the service.
* @sample AWSDataSyncAsyncHandler.UpdateLocationNfs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateLocationNfsAsync(UpdateLocationNfsRequest updateLocationNfsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates some of the parameters of a previously created location for self-managed object storage server access.
* For information about creating a self-managed object storage location, see Creating a location for
* object storage.
*
*
* @param updateLocationObjectStorageRequest
* @return A Java Future containing the result of the UpdateLocationObjectStorage operation returned by the service.
* @sample AWSDataSyncAsync.UpdateLocationObjectStorage
* @see AWS API Documentation
*/
java.util.concurrent.Future updateLocationObjectStorageAsync(
UpdateLocationObjectStorageRequest updateLocationObjectStorageRequest);
/**
*
* Updates some of the parameters of a previously created location for self-managed object storage server access.
* For information about creating a self-managed object storage location, see Creating a location for
* object storage.
*
*
* @param updateLocationObjectStorageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateLocationObjectStorage operation returned by the service.
* @sample AWSDataSyncAsyncHandler.UpdateLocationObjectStorage
* @see AWS API Documentation
*/
java.util.concurrent.Future updateLocationObjectStorageAsync(
UpdateLocationObjectStorageRequest updateLocationObjectStorageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates some of the parameters of a previously created location for Server Message Block (SMB) file system
* access. For information about creating an SMB location, see Creating a location for
* SMB.
*
*
* @param updateLocationSmbRequest
* @return A Java Future containing the result of the UpdateLocationSmb operation returned by the service.
* @sample AWSDataSyncAsync.UpdateLocationSmb
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateLocationSmbAsync(UpdateLocationSmbRequest updateLocationSmbRequest);
/**
*
* Updates some of the parameters of a previously created location for Server Message Block (SMB) file system
* access. For information about creating an SMB location, see Creating a location for
* SMB.
*
*
* @param updateLocationSmbRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateLocationSmb operation returned by the service.
* @sample AWSDataSyncAsyncHandler.UpdateLocationSmb
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateLocationSmbAsync(UpdateLocationSmbRequest updateLocationSmbRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the metadata associated with a task.
*
*
* @param updateTaskRequest
* UpdateTaskResponse
* @return A Java Future containing the result of the UpdateTask operation returned by the service.
* @sample AWSDataSyncAsync.UpdateTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateTaskAsync(UpdateTaskRequest updateTaskRequest);
/**
*
* Updates the metadata associated with a task.
*
*
* @param updateTaskRequest
* UpdateTaskResponse
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateTask operation returned by the service.
* @sample AWSDataSyncAsyncHandler.UpdateTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateTaskAsync(UpdateTaskRequest updateTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates execution of a task.
*
*
* You can modify bandwidth throttling for a task execution that is running or queued. For more information, see Adjusting Bandwidth Throttling for a Task Execution.
*
*
*
* The only Option
that can be modified by UpdateTaskExecution
is
* BytesPerSecond
* .
*
*
*
* @param updateTaskExecutionRequest
* @return A Java Future containing the result of the UpdateTaskExecution operation returned by the service.
* @sample AWSDataSyncAsync.UpdateTaskExecution
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateTaskExecutionAsync(UpdateTaskExecutionRequest updateTaskExecutionRequest);
/**
*
* Updates execution of a task.
*
*
* You can modify bandwidth throttling for a task execution that is running or queued. For more information, see Adjusting Bandwidth Throttling for a Task Execution.
*
*
*
* The only Option
that can be modified by UpdateTaskExecution
is
* BytesPerSecond
* .
*
*
*
* @param updateTaskExecutionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateTaskExecution operation returned by the service.
* @sample AWSDataSyncAsyncHandler.UpdateTaskExecution
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateTaskExecutionAsync(UpdateTaskExecutionRequest updateTaskExecutionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}