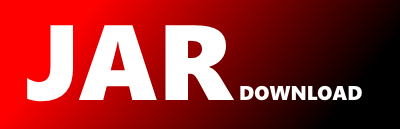
com.amazonaws.services.datasync.model.DescribeTaskExecutionResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-datasync Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.datasync.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* DescribeTaskExecutionResponse
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DescribeTaskExecutionResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The Amazon Resource Name (ARN) of the task execution that was described. TaskExecutionArn
is
* hierarchical and includes TaskArn
for the task that was executed.
*
*
* For example, a TaskExecution
value with the ARN
* arn:aws:datasync:us-east-1:111222333444:task/task-0208075f79cedf4a2/execution/exec-08ef1e88ec491019b
* executed the task with the ARN arn:aws:datasync:us-east-1:111222333444:task/task-0208075f79cedf4a2
.
*
*/
private String taskExecutionArn;
/**
*
* The status of the task execution.
*
*
* For detailed information about task execution statuses, see Understanding Task Statuses in the DataSync User
* Guide.
*
*/
private String status;
private Options options;
/**
*
* A list of filter rules that determines which files to exclude from a task. The list should contain a single
* filter string that consists of the patterns to exclude. The patterns are delimited by "|" (that is, a pipe), for
* example: "/folder1|/folder2"
*
*
*
*/
private java.util.List excludes;
/**
*
* A list of filter rules that determines which files to include when running a task. The list should contain a
* single filter string that consists of the patterns to include. The patterns are delimited by "|" (that is, a
* pipe), for example: "/folder1|/folder2"
*
*
*
*/
private java.util.List includes;
/**
*
* The time that the task execution was started.
*
*/
private java.util.Date startTime;
/**
*
* The expected number of files that is to be transferred over the network. This value is calculated during the
* PREPARING phase, before the TRANSFERRING phase. This value is the expected number of files to be transferred.
* It's calculated based on comparing the content of the source and destination locations and finding the delta that
* needs to be transferred.
*
*/
private Long estimatedFilesToTransfer;
/**
*
* The estimated physical number of bytes that is to be transferred over the network.
*
*/
private Long estimatedBytesToTransfer;
/**
*
* The actual number of files that was transferred over the network. This value is calculated and updated on an
* ongoing basis during the TRANSFERRING phase. It's updated periodically when each file is read from the source and
* sent over the network.
*
*
* If failures occur during a transfer, this value can be less than EstimatedFilesToTransfer
. This
* value can also be greater than EstimatedFilesTransferred
in some cases. This element is
* implementation-specific for some location types, so don't use it as an indicator for a correct file number or to
* monitor your task execution.
*
*/
private Long filesTransferred;
/**
*
* The number of logical bytes written to the destination Amazon Web Services storage resource.
*
*/
private Long bytesWritten;
/**
*
* The physical number of bytes transferred over the network.
*
*/
private Long bytesTransferred;
/**
*
* The result of the task execution.
*
*/
private TaskExecutionResultDetail result;
/**
*
* The Amazon Resource Name (ARN) of the task execution that was described. TaskExecutionArn
is
* hierarchical and includes TaskArn
for the task that was executed.
*
*
* For example, a TaskExecution
value with the ARN
* arn:aws:datasync:us-east-1:111222333444:task/task-0208075f79cedf4a2/execution/exec-08ef1e88ec491019b
* executed the task with the ARN arn:aws:datasync:us-east-1:111222333444:task/task-0208075f79cedf4a2
.
*
*
* @param taskExecutionArn
* The Amazon Resource Name (ARN) of the task execution that was described. TaskExecutionArn
is
* hierarchical and includes TaskArn
for the task that was executed.
*
* For example, a TaskExecution
value with the ARN
* arn:aws:datasync:us-east-1:111222333444:task/task-0208075f79cedf4a2/execution/exec-08ef1e88ec491019b
* executed the task with the ARN
* arn:aws:datasync:us-east-1:111222333444:task/task-0208075f79cedf4a2
.
*/
public void setTaskExecutionArn(String taskExecutionArn) {
this.taskExecutionArn = taskExecutionArn;
}
/**
*
* The Amazon Resource Name (ARN) of the task execution that was described. TaskExecutionArn
is
* hierarchical and includes TaskArn
for the task that was executed.
*
*
* For example, a TaskExecution
value with the ARN
* arn:aws:datasync:us-east-1:111222333444:task/task-0208075f79cedf4a2/execution/exec-08ef1e88ec491019b
* executed the task with the ARN arn:aws:datasync:us-east-1:111222333444:task/task-0208075f79cedf4a2
.
*
*
* @return The Amazon Resource Name (ARN) of the task execution that was described. TaskExecutionArn
is
* hierarchical and includes TaskArn
for the task that was executed.
*
* For example, a TaskExecution
value with the ARN
* arn:aws:datasync:us-east-1:111222333444:task/task-0208075f79cedf4a2/execution/exec-08ef1e88ec491019b
* executed the task with the ARN
* arn:aws:datasync:us-east-1:111222333444:task/task-0208075f79cedf4a2
.
*/
public String getTaskExecutionArn() {
return this.taskExecutionArn;
}
/**
*
* The Amazon Resource Name (ARN) of the task execution that was described. TaskExecutionArn
is
* hierarchical and includes TaskArn
for the task that was executed.
*
*
* For example, a TaskExecution
value with the ARN
* arn:aws:datasync:us-east-1:111222333444:task/task-0208075f79cedf4a2/execution/exec-08ef1e88ec491019b
* executed the task with the ARN arn:aws:datasync:us-east-1:111222333444:task/task-0208075f79cedf4a2
.
*
*
* @param taskExecutionArn
* The Amazon Resource Name (ARN) of the task execution that was described. TaskExecutionArn
is
* hierarchical and includes TaskArn
for the task that was executed.
*
* For example, a TaskExecution
value with the ARN
* arn:aws:datasync:us-east-1:111222333444:task/task-0208075f79cedf4a2/execution/exec-08ef1e88ec491019b
* executed the task with the ARN
* arn:aws:datasync:us-east-1:111222333444:task/task-0208075f79cedf4a2
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskExecutionResult withTaskExecutionArn(String taskExecutionArn) {
setTaskExecutionArn(taskExecutionArn);
return this;
}
/**
*
* The status of the task execution.
*
*
* For detailed information about task execution statuses, see Understanding Task Statuses in the DataSync User
* Guide.
*
*
* @param status
* The status of the task execution.
*
* For detailed information about task execution statuses, see Understanding Task Statuses in the DataSync
* User Guide.
* @see TaskExecutionStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the task execution.
*
*
* For detailed information about task execution statuses, see Understanding Task Statuses in the DataSync User
* Guide.
*
*
* @return The status of the task execution.
*
* For detailed information about task execution statuses, see Understanding Task Statuses in the
* DataSync User Guide.
* @see TaskExecutionStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the task execution.
*
*
* For detailed information about task execution statuses, see Understanding Task Statuses in the DataSync User
* Guide.
*
*
* @param status
* The status of the task execution.
*
* For detailed information about task execution statuses, see Understanding Task Statuses in the DataSync
* User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TaskExecutionStatus
*/
public DescribeTaskExecutionResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of the task execution.
*
*
* For detailed information about task execution statuses, see Understanding Task Statuses in the DataSync User
* Guide.
*
*
* @param status
* The status of the task execution.
*
* For detailed information about task execution statuses, see Understanding Task Statuses in the DataSync
* User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TaskExecutionStatus
*/
public DescribeTaskExecutionResult withStatus(TaskExecutionStatus status) {
this.status = status.toString();
return this;
}
/**
* @param options
*/
public void setOptions(Options options) {
this.options = options;
}
/**
* @return
*/
public Options getOptions() {
return this.options;
}
/**
* @param options
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskExecutionResult withOptions(Options options) {
setOptions(options);
return this;
}
/**
*
* A list of filter rules that determines which files to exclude from a task. The list should contain a single
* filter string that consists of the patterns to exclude. The patterns are delimited by "|" (that is, a pipe), for
* example: "/folder1|/folder2"
*
*
*
*
* @return A list of filter rules that determines which files to exclude from a task. The list should contain a
* single filter string that consists of the patterns to exclude. The patterns are delimited by "|" (that
* is, a pipe), for example: "/folder1|/folder2"
*
*/
public java.util.List getExcludes() {
return excludes;
}
/**
*
* A list of filter rules that determines which files to exclude from a task. The list should contain a single
* filter string that consists of the patterns to exclude. The patterns are delimited by "|" (that is, a pipe), for
* example: "/folder1|/folder2"
*
*
*
*
* @param excludes
* A list of filter rules that determines which files to exclude from a task. The list should contain a
* single filter string that consists of the patterns to exclude. The patterns are delimited by "|" (that is,
* a pipe), for example: "/folder1|/folder2"
*
*/
public void setExcludes(java.util.Collection excludes) {
if (excludes == null) {
this.excludes = null;
return;
}
this.excludes = new java.util.ArrayList(excludes);
}
/**
*
* A list of filter rules that determines which files to exclude from a task. The list should contain a single
* filter string that consists of the patterns to exclude. The patterns are delimited by "|" (that is, a pipe), for
* example: "/folder1|/folder2"
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setExcludes(java.util.Collection)} or {@link #withExcludes(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param excludes
* A list of filter rules that determines which files to exclude from a task. The list should contain a
* single filter string that consists of the patterns to exclude. The patterns are delimited by "|" (that is,
* a pipe), for example: "/folder1|/folder2"
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskExecutionResult withExcludes(FilterRule... excludes) {
if (this.excludes == null) {
setExcludes(new java.util.ArrayList(excludes.length));
}
for (FilterRule ele : excludes) {
this.excludes.add(ele);
}
return this;
}
/**
*
* A list of filter rules that determines which files to exclude from a task. The list should contain a single
* filter string that consists of the patterns to exclude. The patterns are delimited by "|" (that is, a pipe), for
* example: "/folder1|/folder2"
*
*
*
*
* @param excludes
* A list of filter rules that determines which files to exclude from a task. The list should contain a
* single filter string that consists of the patterns to exclude. The patterns are delimited by "|" (that is,
* a pipe), for example: "/folder1|/folder2"
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskExecutionResult withExcludes(java.util.Collection excludes) {
setExcludes(excludes);
return this;
}
/**
*
* A list of filter rules that determines which files to include when running a task. The list should contain a
* single filter string that consists of the patterns to include. The patterns are delimited by "|" (that is, a
* pipe), for example: "/folder1|/folder2"
*
*
*
*
* @return A list of filter rules that determines which files to include when running a task. The list should
* contain a single filter string that consists of the patterns to include. The patterns are delimited by
* "|" (that is, a pipe), for example: "/folder1|/folder2"
*
*/
public java.util.List getIncludes() {
return includes;
}
/**
*
* A list of filter rules that determines which files to include when running a task. The list should contain a
* single filter string that consists of the patterns to include. The patterns are delimited by "|" (that is, a
* pipe), for example: "/folder1|/folder2"
*
*
*
*
* @param includes
* A list of filter rules that determines which files to include when running a task. The list should contain
* a single filter string that consists of the patterns to include. The patterns are delimited by "|" (that
* is, a pipe), for example: "/folder1|/folder2"
*
*/
public void setIncludes(java.util.Collection includes) {
if (includes == null) {
this.includes = null;
return;
}
this.includes = new java.util.ArrayList(includes);
}
/**
*
* A list of filter rules that determines which files to include when running a task. The list should contain a
* single filter string that consists of the patterns to include. The patterns are delimited by "|" (that is, a
* pipe), for example: "/folder1|/folder2"
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setIncludes(java.util.Collection)} or {@link #withIncludes(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param includes
* A list of filter rules that determines which files to include when running a task. The list should contain
* a single filter string that consists of the patterns to include. The patterns are delimited by "|" (that
* is, a pipe), for example: "/folder1|/folder2"
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskExecutionResult withIncludes(FilterRule... includes) {
if (this.includes == null) {
setIncludes(new java.util.ArrayList(includes.length));
}
for (FilterRule ele : includes) {
this.includes.add(ele);
}
return this;
}
/**
*
* A list of filter rules that determines which files to include when running a task. The list should contain a
* single filter string that consists of the patterns to include. The patterns are delimited by "|" (that is, a
* pipe), for example: "/folder1|/folder2"
*
*
*
*
* @param includes
* A list of filter rules that determines which files to include when running a task. The list should contain
* a single filter string that consists of the patterns to include. The patterns are delimited by "|" (that
* is, a pipe), for example: "/folder1|/folder2"
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskExecutionResult withIncludes(java.util.Collection includes) {
setIncludes(includes);
return this;
}
/**
*
* The time that the task execution was started.
*
*
* @param startTime
* The time that the task execution was started.
*/
public void setStartTime(java.util.Date startTime) {
this.startTime = startTime;
}
/**
*
* The time that the task execution was started.
*
*
* @return The time that the task execution was started.
*/
public java.util.Date getStartTime() {
return this.startTime;
}
/**
*
* The time that the task execution was started.
*
*
* @param startTime
* The time that the task execution was started.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskExecutionResult withStartTime(java.util.Date startTime) {
setStartTime(startTime);
return this;
}
/**
*
* The expected number of files that is to be transferred over the network. This value is calculated during the
* PREPARING phase, before the TRANSFERRING phase. This value is the expected number of files to be transferred.
* It's calculated based on comparing the content of the source and destination locations and finding the delta that
* needs to be transferred.
*
*
* @param estimatedFilesToTransfer
* The expected number of files that is to be transferred over the network. This value is calculated during
* the PREPARING phase, before the TRANSFERRING phase. This value is the expected number of files to be
* transferred. It's calculated based on comparing the content of the source and destination locations and
* finding the delta that needs to be transferred.
*/
public void setEstimatedFilesToTransfer(Long estimatedFilesToTransfer) {
this.estimatedFilesToTransfer = estimatedFilesToTransfer;
}
/**
*
* The expected number of files that is to be transferred over the network. This value is calculated during the
* PREPARING phase, before the TRANSFERRING phase. This value is the expected number of files to be transferred.
* It's calculated based on comparing the content of the source and destination locations and finding the delta that
* needs to be transferred.
*
*
* @return The expected number of files that is to be transferred over the network. This value is calculated during
* the PREPARING phase, before the TRANSFERRING phase. This value is the expected number of files to be
* transferred. It's calculated based on comparing the content of the source and destination locations and
* finding the delta that needs to be transferred.
*/
public Long getEstimatedFilesToTransfer() {
return this.estimatedFilesToTransfer;
}
/**
*
* The expected number of files that is to be transferred over the network. This value is calculated during the
* PREPARING phase, before the TRANSFERRING phase. This value is the expected number of files to be transferred.
* It's calculated based on comparing the content of the source and destination locations and finding the delta that
* needs to be transferred.
*
*
* @param estimatedFilesToTransfer
* The expected number of files that is to be transferred over the network. This value is calculated during
* the PREPARING phase, before the TRANSFERRING phase. This value is the expected number of files to be
* transferred. It's calculated based on comparing the content of the source and destination locations and
* finding the delta that needs to be transferred.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskExecutionResult withEstimatedFilesToTransfer(Long estimatedFilesToTransfer) {
setEstimatedFilesToTransfer(estimatedFilesToTransfer);
return this;
}
/**
*
* The estimated physical number of bytes that is to be transferred over the network.
*
*
* @param estimatedBytesToTransfer
* The estimated physical number of bytes that is to be transferred over the network.
*/
public void setEstimatedBytesToTransfer(Long estimatedBytesToTransfer) {
this.estimatedBytesToTransfer = estimatedBytesToTransfer;
}
/**
*
* The estimated physical number of bytes that is to be transferred over the network.
*
*
* @return The estimated physical number of bytes that is to be transferred over the network.
*/
public Long getEstimatedBytesToTransfer() {
return this.estimatedBytesToTransfer;
}
/**
*
* The estimated physical number of bytes that is to be transferred over the network.
*
*
* @param estimatedBytesToTransfer
* The estimated physical number of bytes that is to be transferred over the network.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskExecutionResult withEstimatedBytesToTransfer(Long estimatedBytesToTransfer) {
setEstimatedBytesToTransfer(estimatedBytesToTransfer);
return this;
}
/**
*
* The actual number of files that was transferred over the network. This value is calculated and updated on an
* ongoing basis during the TRANSFERRING phase. It's updated periodically when each file is read from the source and
* sent over the network.
*
*
* If failures occur during a transfer, this value can be less than EstimatedFilesToTransfer
. This
* value can also be greater than EstimatedFilesTransferred
in some cases. This element is
* implementation-specific for some location types, so don't use it as an indicator for a correct file number or to
* monitor your task execution.
*
*
* @param filesTransferred
* The actual number of files that was transferred over the network. This value is calculated and updated on
* an ongoing basis during the TRANSFERRING phase. It's updated periodically when each file is read from the
* source and sent over the network.
*
* If failures occur during a transfer, this value can be less than EstimatedFilesToTransfer
.
* This value can also be greater than EstimatedFilesTransferred
in some cases. This element is
* implementation-specific for some location types, so don't use it as an indicator for a correct file number
* or to monitor your task execution.
*/
public void setFilesTransferred(Long filesTransferred) {
this.filesTransferred = filesTransferred;
}
/**
*
* The actual number of files that was transferred over the network. This value is calculated and updated on an
* ongoing basis during the TRANSFERRING phase. It's updated periodically when each file is read from the source and
* sent over the network.
*
*
* If failures occur during a transfer, this value can be less than EstimatedFilesToTransfer
. This
* value can also be greater than EstimatedFilesTransferred
in some cases. This element is
* implementation-specific for some location types, so don't use it as an indicator for a correct file number or to
* monitor your task execution.
*
*
* @return The actual number of files that was transferred over the network. This value is calculated and updated on
* an ongoing basis during the TRANSFERRING phase. It's updated periodically when each file is read from the
* source and sent over the network.
*
* If failures occur during a transfer, this value can be less than EstimatedFilesToTransfer
.
* This value can also be greater than EstimatedFilesTransferred
in some cases. This element is
* implementation-specific for some location types, so don't use it as an indicator for a correct file
* number or to monitor your task execution.
*/
public Long getFilesTransferred() {
return this.filesTransferred;
}
/**
*
* The actual number of files that was transferred over the network. This value is calculated and updated on an
* ongoing basis during the TRANSFERRING phase. It's updated periodically when each file is read from the source and
* sent over the network.
*
*
* If failures occur during a transfer, this value can be less than EstimatedFilesToTransfer
. This
* value can also be greater than EstimatedFilesTransferred
in some cases. This element is
* implementation-specific for some location types, so don't use it as an indicator for a correct file number or to
* monitor your task execution.
*
*
* @param filesTransferred
* The actual number of files that was transferred over the network. This value is calculated and updated on
* an ongoing basis during the TRANSFERRING phase. It's updated periodically when each file is read from the
* source and sent over the network.
*
* If failures occur during a transfer, this value can be less than EstimatedFilesToTransfer
.
* This value can also be greater than EstimatedFilesTransferred
in some cases. This element is
* implementation-specific for some location types, so don't use it as an indicator for a correct file number
* or to monitor your task execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskExecutionResult withFilesTransferred(Long filesTransferred) {
setFilesTransferred(filesTransferred);
return this;
}
/**
*
* The number of logical bytes written to the destination Amazon Web Services storage resource.
*
*
* @param bytesWritten
* The number of logical bytes written to the destination Amazon Web Services storage resource.
*/
public void setBytesWritten(Long bytesWritten) {
this.bytesWritten = bytesWritten;
}
/**
*
* The number of logical bytes written to the destination Amazon Web Services storage resource.
*
*
* @return The number of logical bytes written to the destination Amazon Web Services storage resource.
*/
public Long getBytesWritten() {
return this.bytesWritten;
}
/**
*
* The number of logical bytes written to the destination Amazon Web Services storage resource.
*
*
* @param bytesWritten
* The number of logical bytes written to the destination Amazon Web Services storage resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskExecutionResult withBytesWritten(Long bytesWritten) {
setBytesWritten(bytesWritten);
return this;
}
/**
*
* The physical number of bytes transferred over the network.
*
*
* @param bytesTransferred
* The physical number of bytes transferred over the network.
*/
public void setBytesTransferred(Long bytesTransferred) {
this.bytesTransferred = bytesTransferred;
}
/**
*
* The physical number of bytes transferred over the network.
*
*
* @return The physical number of bytes transferred over the network.
*/
public Long getBytesTransferred() {
return this.bytesTransferred;
}
/**
*
* The physical number of bytes transferred over the network.
*
*
* @param bytesTransferred
* The physical number of bytes transferred over the network.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskExecutionResult withBytesTransferred(Long bytesTransferred) {
setBytesTransferred(bytesTransferred);
return this;
}
/**
*
* The result of the task execution.
*
*
* @param result
* The result of the task execution.
*/
public void setResult(TaskExecutionResultDetail result) {
this.result = result;
}
/**
*
* The result of the task execution.
*
*
* @return The result of the task execution.
*/
public TaskExecutionResultDetail getResult() {
return this.result;
}
/**
*
* The result of the task execution.
*
*
* @param result
* The result of the task execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskExecutionResult withResult(TaskExecutionResultDetail result) {
setResult(result);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTaskExecutionArn() != null)
sb.append("TaskExecutionArn: ").append(getTaskExecutionArn()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getOptions() != null)
sb.append("Options: ").append(getOptions()).append(",");
if (getExcludes() != null)
sb.append("Excludes: ").append(getExcludes()).append(",");
if (getIncludes() != null)
sb.append("Includes: ").append(getIncludes()).append(",");
if (getStartTime() != null)
sb.append("StartTime: ").append(getStartTime()).append(",");
if (getEstimatedFilesToTransfer() != null)
sb.append("EstimatedFilesToTransfer: ").append(getEstimatedFilesToTransfer()).append(",");
if (getEstimatedBytesToTransfer() != null)
sb.append("EstimatedBytesToTransfer: ").append(getEstimatedBytesToTransfer()).append(",");
if (getFilesTransferred() != null)
sb.append("FilesTransferred: ").append(getFilesTransferred()).append(",");
if (getBytesWritten() != null)
sb.append("BytesWritten: ").append(getBytesWritten()).append(",");
if (getBytesTransferred() != null)
sb.append("BytesTransferred: ").append(getBytesTransferred()).append(",");
if (getResult() != null)
sb.append("Result: ").append(getResult());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeTaskExecutionResult == false)
return false;
DescribeTaskExecutionResult other = (DescribeTaskExecutionResult) obj;
if (other.getTaskExecutionArn() == null ^ this.getTaskExecutionArn() == null)
return false;
if (other.getTaskExecutionArn() != null && other.getTaskExecutionArn().equals(this.getTaskExecutionArn()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getOptions() == null ^ this.getOptions() == null)
return false;
if (other.getOptions() != null && other.getOptions().equals(this.getOptions()) == false)
return false;
if (other.getExcludes() == null ^ this.getExcludes() == null)
return false;
if (other.getExcludes() != null && other.getExcludes().equals(this.getExcludes()) == false)
return false;
if (other.getIncludes() == null ^ this.getIncludes() == null)
return false;
if (other.getIncludes() != null && other.getIncludes().equals(this.getIncludes()) == false)
return false;
if (other.getStartTime() == null ^ this.getStartTime() == null)
return false;
if (other.getStartTime() != null && other.getStartTime().equals(this.getStartTime()) == false)
return false;
if (other.getEstimatedFilesToTransfer() == null ^ this.getEstimatedFilesToTransfer() == null)
return false;
if (other.getEstimatedFilesToTransfer() != null && other.getEstimatedFilesToTransfer().equals(this.getEstimatedFilesToTransfer()) == false)
return false;
if (other.getEstimatedBytesToTransfer() == null ^ this.getEstimatedBytesToTransfer() == null)
return false;
if (other.getEstimatedBytesToTransfer() != null && other.getEstimatedBytesToTransfer().equals(this.getEstimatedBytesToTransfer()) == false)
return false;
if (other.getFilesTransferred() == null ^ this.getFilesTransferred() == null)
return false;
if (other.getFilesTransferred() != null && other.getFilesTransferred().equals(this.getFilesTransferred()) == false)
return false;
if (other.getBytesWritten() == null ^ this.getBytesWritten() == null)
return false;
if (other.getBytesWritten() != null && other.getBytesWritten().equals(this.getBytesWritten()) == false)
return false;
if (other.getBytesTransferred() == null ^ this.getBytesTransferred() == null)
return false;
if (other.getBytesTransferred() != null && other.getBytesTransferred().equals(this.getBytesTransferred()) == false)
return false;
if (other.getResult() == null ^ this.getResult() == null)
return false;
if (other.getResult() != null && other.getResult().equals(this.getResult()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTaskExecutionArn() == null) ? 0 : getTaskExecutionArn().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getOptions() == null) ? 0 : getOptions().hashCode());
hashCode = prime * hashCode + ((getExcludes() == null) ? 0 : getExcludes().hashCode());
hashCode = prime * hashCode + ((getIncludes() == null) ? 0 : getIncludes().hashCode());
hashCode = prime * hashCode + ((getStartTime() == null) ? 0 : getStartTime().hashCode());
hashCode = prime * hashCode + ((getEstimatedFilesToTransfer() == null) ? 0 : getEstimatedFilesToTransfer().hashCode());
hashCode = prime * hashCode + ((getEstimatedBytesToTransfer() == null) ? 0 : getEstimatedBytesToTransfer().hashCode());
hashCode = prime * hashCode + ((getFilesTransferred() == null) ? 0 : getFilesTransferred().hashCode());
hashCode = prime * hashCode + ((getBytesWritten() == null) ? 0 : getBytesWritten().hashCode());
hashCode = prime * hashCode + ((getBytesTransferred() == null) ? 0 : getBytesTransferred().hashCode());
hashCode = prime * hashCode + ((getResult() == null) ? 0 : getResult().hashCode());
return hashCode;
}
@Override
public DescribeTaskExecutionResult clone() {
try {
return (DescribeTaskExecutionResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}