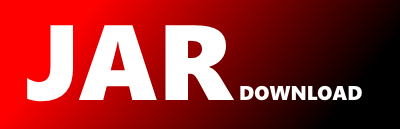
com.amazonaws.services.datasync.model.DescribeTaskResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-datasync Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.datasync.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* DescribeTaskResponse
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DescribeTaskResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The Amazon Resource Name (ARN) of the task that was described.
*
*/
private String taskArn;
/**
*
* The status of the task that was described.
*
*
* For detailed information about task execution statuses, see Understanding Task Statuses in the DataSync User
* Guide.
*
*/
private String status;
/**
*
* The name of the task that was described.
*
*/
private String name;
/**
*
* The Amazon Resource Name (ARN) of the task execution that is transferring files.
*
*/
private String currentTaskExecutionArn;
/**
*
* The Amazon Resource Name (ARN) of the source file system's location.
*
*/
private String sourceLocationArn;
/**
*
* The Amazon Resource Name (ARN) of the Amazon Web Services storage resource's location.
*
*/
private String destinationLocationArn;
/**
*
* The Amazon Resource Name (ARN) of the Amazon CloudWatch log group that was used to monitor and log events in the
* task.
*
*
* For more information on these groups, see Working with Log Groups and Log Streams in the Amazon CloudWatch
* User Guide.
*
*/
private String cloudWatchLogGroupArn;
/**
*
* The Amazon Resource Names (ARNs) of the network interfaces created for your source location. For more
* information, see Network interface requirements.
*
*/
private java.util.List sourceNetworkInterfaceArns;
/**
*
* The Amazon Resource Names (ARNs) of the network interfaces created for your destination location. For more
* information, see Network interface requirements.
*
*/
private java.util.List destinationNetworkInterfaceArns;
/**
*
* The configuration options that control the behavior of the StartTaskExecution
operation. Some
* options include preserving file or object metadata and verifying data integrity.
*
*
* You can override these options for each task execution. For more information, see StartTaskExecution.
*
*/
private Options options;
/**
*
* A list of filter rules that exclude specific data during your transfer. For more information and examples, see Filtering data transferred by
* DataSync.
*
*/
private java.util.List excludes;
/**
*
* The schedule used to periodically transfer files from a source to a destination location.
*
*/
private TaskSchedule schedule;
/**
*
* Errors that DataSync encountered during execution of the task. You can use this error code to help troubleshoot
* issues.
*
*/
private String errorCode;
/**
*
* Detailed description of an error that was encountered during the task execution. You can use this information to
* help troubleshoot issues.
*
*/
private String errorDetail;
/**
*
* The time that the task was created.
*
*/
private java.util.Date creationTime;
/**
*
* A list of filter rules that include specific data during your transfer. For more information and examples, see Filtering data transferred by
* DataSync.
*
*/
private java.util.List includes;
/**
*
* The configuration of your task report. For more information, see Creating a task report.
*
*/
private TaskReportConfig taskReportConfig;
/**
*
* The Amazon Resource Name (ARN) of the task that was described.
*
*
* @param taskArn
* The Amazon Resource Name (ARN) of the task that was described.
*/
public void setTaskArn(String taskArn) {
this.taskArn = taskArn;
}
/**
*
* The Amazon Resource Name (ARN) of the task that was described.
*
*
* @return The Amazon Resource Name (ARN) of the task that was described.
*/
public String getTaskArn() {
return this.taskArn;
}
/**
*
* The Amazon Resource Name (ARN) of the task that was described.
*
*
* @param taskArn
* The Amazon Resource Name (ARN) of the task that was described.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskResult withTaskArn(String taskArn) {
setTaskArn(taskArn);
return this;
}
/**
*
* The status of the task that was described.
*
*
* For detailed information about task execution statuses, see Understanding Task Statuses in the DataSync User
* Guide.
*
*
* @param status
* The status of the task that was described.
*
* For detailed information about task execution statuses, see Understanding Task Statuses in the DataSync
* User Guide.
* @see TaskStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the task that was described.
*
*
* For detailed information about task execution statuses, see Understanding Task Statuses in the DataSync User
* Guide.
*
*
* @return The status of the task that was described.
*
* For detailed information about task execution statuses, see Understanding Task Statuses in the
* DataSync User Guide.
* @see TaskStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the task that was described.
*
*
* For detailed information about task execution statuses, see Understanding Task Statuses in the DataSync User
* Guide.
*
*
* @param status
* The status of the task that was described.
*
* For detailed information about task execution statuses, see Understanding Task Statuses in the DataSync
* User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TaskStatus
*/
public DescribeTaskResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of the task that was described.
*
*
* For detailed information about task execution statuses, see Understanding Task Statuses in the DataSync User
* Guide.
*
*
* @param status
* The status of the task that was described.
*
* For detailed information about task execution statuses, see Understanding Task Statuses in the DataSync
* User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TaskStatus
*/
public DescribeTaskResult withStatus(TaskStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The name of the task that was described.
*
*
* @param name
* The name of the task that was described.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the task that was described.
*
*
* @return The name of the task that was described.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the task that was described.
*
*
* @param name
* The name of the task that was described.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskResult withName(String name) {
setName(name);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the task execution that is transferring files.
*
*
* @param currentTaskExecutionArn
* The Amazon Resource Name (ARN) of the task execution that is transferring files.
*/
public void setCurrentTaskExecutionArn(String currentTaskExecutionArn) {
this.currentTaskExecutionArn = currentTaskExecutionArn;
}
/**
*
* The Amazon Resource Name (ARN) of the task execution that is transferring files.
*
*
* @return The Amazon Resource Name (ARN) of the task execution that is transferring files.
*/
public String getCurrentTaskExecutionArn() {
return this.currentTaskExecutionArn;
}
/**
*
* The Amazon Resource Name (ARN) of the task execution that is transferring files.
*
*
* @param currentTaskExecutionArn
* The Amazon Resource Name (ARN) of the task execution that is transferring files.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskResult withCurrentTaskExecutionArn(String currentTaskExecutionArn) {
setCurrentTaskExecutionArn(currentTaskExecutionArn);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the source file system's location.
*
*
* @param sourceLocationArn
* The Amazon Resource Name (ARN) of the source file system's location.
*/
public void setSourceLocationArn(String sourceLocationArn) {
this.sourceLocationArn = sourceLocationArn;
}
/**
*
* The Amazon Resource Name (ARN) of the source file system's location.
*
*
* @return The Amazon Resource Name (ARN) of the source file system's location.
*/
public String getSourceLocationArn() {
return this.sourceLocationArn;
}
/**
*
* The Amazon Resource Name (ARN) of the source file system's location.
*
*
* @param sourceLocationArn
* The Amazon Resource Name (ARN) of the source file system's location.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskResult withSourceLocationArn(String sourceLocationArn) {
setSourceLocationArn(sourceLocationArn);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon Web Services storage resource's location.
*
*
* @param destinationLocationArn
* The Amazon Resource Name (ARN) of the Amazon Web Services storage resource's location.
*/
public void setDestinationLocationArn(String destinationLocationArn) {
this.destinationLocationArn = destinationLocationArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon Web Services storage resource's location.
*
*
* @return The Amazon Resource Name (ARN) of the Amazon Web Services storage resource's location.
*/
public String getDestinationLocationArn() {
return this.destinationLocationArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon Web Services storage resource's location.
*
*
* @param destinationLocationArn
* The Amazon Resource Name (ARN) of the Amazon Web Services storage resource's location.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskResult withDestinationLocationArn(String destinationLocationArn) {
setDestinationLocationArn(destinationLocationArn);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon CloudWatch log group that was used to monitor and log events in the
* task.
*
*
* For more information on these groups, see Working with Log Groups and Log Streams in the Amazon CloudWatch
* User Guide.
*
*
* @param cloudWatchLogGroupArn
* The Amazon Resource Name (ARN) of the Amazon CloudWatch log group that was used to monitor and log events
* in the task.
*
* For more information on these groups, see Working with Log Groups and Log Streams in the Amazon
* CloudWatch User Guide.
*/
public void setCloudWatchLogGroupArn(String cloudWatchLogGroupArn) {
this.cloudWatchLogGroupArn = cloudWatchLogGroupArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon CloudWatch log group that was used to monitor and log events in the
* task.
*
*
* For more information on these groups, see Working with Log Groups and Log Streams in the Amazon CloudWatch
* User Guide.
*
*
* @return The Amazon Resource Name (ARN) of the Amazon CloudWatch log group that was used to monitor and log events
* in the task.
*
* For more information on these groups, see Working with Log Groups and Log Streams in the Amazon
* CloudWatch User Guide.
*/
public String getCloudWatchLogGroupArn() {
return this.cloudWatchLogGroupArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon CloudWatch log group that was used to monitor and log events in the
* task.
*
*
* For more information on these groups, see Working with Log Groups and Log Streams in the Amazon CloudWatch
* User Guide.
*
*
* @param cloudWatchLogGroupArn
* The Amazon Resource Name (ARN) of the Amazon CloudWatch log group that was used to monitor and log events
* in the task.
*
* For more information on these groups, see Working with Log Groups and Log Streams in the Amazon
* CloudWatch User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskResult withCloudWatchLogGroupArn(String cloudWatchLogGroupArn) {
setCloudWatchLogGroupArn(cloudWatchLogGroupArn);
return this;
}
/**
*
* The Amazon Resource Names (ARNs) of the network interfaces created for your source location. For more
* information, see Network interface requirements.
*
*
* @return The Amazon Resource Names (ARNs) of the network interfaces created for your source location. For more
* information, see Network interface requirements.
*/
public java.util.List getSourceNetworkInterfaceArns() {
return sourceNetworkInterfaceArns;
}
/**
*
* The Amazon Resource Names (ARNs) of the network interfaces created for your source location. For more
* information, see Network interface requirements.
*
*
* @param sourceNetworkInterfaceArns
* The Amazon Resource Names (ARNs) of the network interfaces created for your source location. For more
* information, see Network interface requirements.
*/
public void setSourceNetworkInterfaceArns(java.util.Collection sourceNetworkInterfaceArns) {
if (sourceNetworkInterfaceArns == null) {
this.sourceNetworkInterfaceArns = null;
return;
}
this.sourceNetworkInterfaceArns = new java.util.ArrayList(sourceNetworkInterfaceArns);
}
/**
*
* The Amazon Resource Names (ARNs) of the network interfaces created for your source location. For more
* information, see Network interface requirements.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSourceNetworkInterfaceArns(java.util.Collection)} or
* {@link #withSourceNetworkInterfaceArns(java.util.Collection)} if you want to override the existing values.
*
*
* @param sourceNetworkInterfaceArns
* The Amazon Resource Names (ARNs) of the network interfaces created for your source location. For more
* information, see Network interface requirements.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskResult withSourceNetworkInterfaceArns(String... sourceNetworkInterfaceArns) {
if (this.sourceNetworkInterfaceArns == null) {
setSourceNetworkInterfaceArns(new java.util.ArrayList(sourceNetworkInterfaceArns.length));
}
for (String ele : sourceNetworkInterfaceArns) {
this.sourceNetworkInterfaceArns.add(ele);
}
return this;
}
/**
*
* The Amazon Resource Names (ARNs) of the network interfaces created for your source location. For more
* information, see Network interface requirements.
*
*
* @param sourceNetworkInterfaceArns
* The Amazon Resource Names (ARNs) of the network interfaces created for your source location. For more
* information, see Network interface requirements.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskResult withSourceNetworkInterfaceArns(java.util.Collection sourceNetworkInterfaceArns) {
setSourceNetworkInterfaceArns(sourceNetworkInterfaceArns);
return this;
}
/**
*
* The Amazon Resource Names (ARNs) of the network interfaces created for your destination location. For more
* information, see Network interface requirements.
*
*
* @return The Amazon Resource Names (ARNs) of the network interfaces created for your destination location. For
* more information, see Network interface requirements.
*/
public java.util.List getDestinationNetworkInterfaceArns() {
return destinationNetworkInterfaceArns;
}
/**
*
* The Amazon Resource Names (ARNs) of the network interfaces created for your destination location. For more
* information, see Network interface requirements.
*
*
* @param destinationNetworkInterfaceArns
* The Amazon Resource Names (ARNs) of the network interfaces created for your destination location. For more
* information, see Network interface requirements.
*/
public void setDestinationNetworkInterfaceArns(java.util.Collection destinationNetworkInterfaceArns) {
if (destinationNetworkInterfaceArns == null) {
this.destinationNetworkInterfaceArns = null;
return;
}
this.destinationNetworkInterfaceArns = new java.util.ArrayList(destinationNetworkInterfaceArns);
}
/**
*
* The Amazon Resource Names (ARNs) of the network interfaces created for your destination location. For more
* information, see Network interface requirements.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDestinationNetworkInterfaceArns(java.util.Collection)} or
* {@link #withDestinationNetworkInterfaceArns(java.util.Collection)} if you want to override the existing values.
*
*
* @param destinationNetworkInterfaceArns
* The Amazon Resource Names (ARNs) of the network interfaces created for your destination location. For more
* information, see Network interface requirements.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskResult withDestinationNetworkInterfaceArns(String... destinationNetworkInterfaceArns) {
if (this.destinationNetworkInterfaceArns == null) {
setDestinationNetworkInterfaceArns(new java.util.ArrayList(destinationNetworkInterfaceArns.length));
}
for (String ele : destinationNetworkInterfaceArns) {
this.destinationNetworkInterfaceArns.add(ele);
}
return this;
}
/**
*
* The Amazon Resource Names (ARNs) of the network interfaces created for your destination location. For more
* information, see Network interface requirements.
*
*
* @param destinationNetworkInterfaceArns
* The Amazon Resource Names (ARNs) of the network interfaces created for your destination location. For more
* information, see Network interface requirements.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskResult withDestinationNetworkInterfaceArns(java.util.Collection destinationNetworkInterfaceArns) {
setDestinationNetworkInterfaceArns(destinationNetworkInterfaceArns);
return this;
}
/**
*
* The configuration options that control the behavior of the StartTaskExecution
operation. Some
* options include preserving file or object metadata and verifying data integrity.
*
*
* You can override these options for each task execution. For more information, see StartTaskExecution.
*
*
* @param options
* The configuration options that control the behavior of the StartTaskExecution
operation. Some
* options include preserving file or object metadata and verifying data integrity.
*
* You can override these options for each task execution. For more information, see StartTaskExecution.
*/
public void setOptions(Options options) {
this.options = options;
}
/**
*
* The configuration options that control the behavior of the StartTaskExecution
operation. Some
* options include preserving file or object metadata and verifying data integrity.
*
*
* You can override these options for each task execution. For more information, see StartTaskExecution.
*
*
* @return The configuration options that control the behavior of the StartTaskExecution
operation.
* Some options include preserving file or object metadata and verifying data integrity.
*
* You can override these options for each task execution. For more information, see StartTaskExecution.
*/
public Options getOptions() {
return this.options;
}
/**
*
* The configuration options that control the behavior of the StartTaskExecution
operation. Some
* options include preserving file or object metadata and verifying data integrity.
*
*
* You can override these options for each task execution. For more information, see StartTaskExecution.
*
*
* @param options
* The configuration options that control the behavior of the StartTaskExecution
operation. Some
* options include preserving file or object metadata and verifying data integrity.
*
* You can override these options for each task execution. For more information, see StartTaskExecution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskResult withOptions(Options options) {
setOptions(options);
return this;
}
/**
*
* A list of filter rules that exclude specific data during your transfer. For more information and examples, see Filtering data transferred by
* DataSync.
*
*
* @return A list of filter rules that exclude specific data during your transfer. For more information and
* examples, see Filtering
* data transferred by DataSync.
*/
public java.util.List getExcludes() {
return excludes;
}
/**
*
* A list of filter rules that exclude specific data during your transfer. For more information and examples, see Filtering data transferred by
* DataSync.
*
*
* @param excludes
* A list of filter rules that exclude specific data during your transfer. For more information and examples,
* see Filtering data
* transferred by DataSync.
*/
public void setExcludes(java.util.Collection excludes) {
if (excludes == null) {
this.excludes = null;
return;
}
this.excludes = new java.util.ArrayList(excludes);
}
/**
*
* A list of filter rules that exclude specific data during your transfer. For more information and examples, see Filtering data transferred by
* DataSync.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setExcludes(java.util.Collection)} or {@link #withExcludes(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param excludes
* A list of filter rules that exclude specific data during your transfer. For more information and examples,
* see Filtering data
* transferred by DataSync.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskResult withExcludes(FilterRule... excludes) {
if (this.excludes == null) {
setExcludes(new java.util.ArrayList(excludes.length));
}
for (FilterRule ele : excludes) {
this.excludes.add(ele);
}
return this;
}
/**
*
* A list of filter rules that exclude specific data during your transfer. For more information and examples, see Filtering data transferred by
* DataSync.
*
*
* @param excludes
* A list of filter rules that exclude specific data during your transfer. For more information and examples,
* see Filtering data
* transferred by DataSync.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskResult withExcludes(java.util.Collection excludes) {
setExcludes(excludes);
return this;
}
/**
*
* The schedule used to periodically transfer files from a source to a destination location.
*
*
* @param schedule
* The schedule used to periodically transfer files from a source to a destination location.
*/
public void setSchedule(TaskSchedule schedule) {
this.schedule = schedule;
}
/**
*
* The schedule used to periodically transfer files from a source to a destination location.
*
*
* @return The schedule used to periodically transfer files from a source to a destination location.
*/
public TaskSchedule getSchedule() {
return this.schedule;
}
/**
*
* The schedule used to periodically transfer files from a source to a destination location.
*
*
* @param schedule
* The schedule used to periodically transfer files from a source to a destination location.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskResult withSchedule(TaskSchedule schedule) {
setSchedule(schedule);
return this;
}
/**
*
* Errors that DataSync encountered during execution of the task. You can use this error code to help troubleshoot
* issues.
*
*
* @param errorCode
* Errors that DataSync encountered during execution of the task. You can use this error code to help
* troubleshoot issues.
*/
public void setErrorCode(String errorCode) {
this.errorCode = errorCode;
}
/**
*
* Errors that DataSync encountered during execution of the task. You can use this error code to help troubleshoot
* issues.
*
*
* @return Errors that DataSync encountered during execution of the task. You can use this error code to help
* troubleshoot issues.
*/
public String getErrorCode() {
return this.errorCode;
}
/**
*
* Errors that DataSync encountered during execution of the task. You can use this error code to help troubleshoot
* issues.
*
*
* @param errorCode
* Errors that DataSync encountered during execution of the task. You can use this error code to help
* troubleshoot issues.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskResult withErrorCode(String errorCode) {
setErrorCode(errorCode);
return this;
}
/**
*
* Detailed description of an error that was encountered during the task execution. You can use this information to
* help troubleshoot issues.
*
*
* @param errorDetail
* Detailed description of an error that was encountered during the task execution. You can use this
* information to help troubleshoot issues.
*/
public void setErrorDetail(String errorDetail) {
this.errorDetail = errorDetail;
}
/**
*
* Detailed description of an error that was encountered during the task execution. You can use this information to
* help troubleshoot issues.
*
*
* @return Detailed description of an error that was encountered during the task execution. You can use this
* information to help troubleshoot issues.
*/
public String getErrorDetail() {
return this.errorDetail;
}
/**
*
* Detailed description of an error that was encountered during the task execution. You can use this information to
* help troubleshoot issues.
*
*
* @param errorDetail
* Detailed description of an error that was encountered during the task execution. You can use this
* information to help troubleshoot issues.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskResult withErrorDetail(String errorDetail) {
setErrorDetail(errorDetail);
return this;
}
/**
*
* The time that the task was created.
*
*
* @param creationTime
* The time that the task was created.
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
*
* The time that the task was created.
*
*
* @return The time that the task was created.
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
*
* The time that the task was created.
*
*
* @param creationTime
* The time that the task was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskResult withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
*
* A list of filter rules that include specific data during your transfer. For more information and examples, see Filtering data transferred by
* DataSync.
*
*
* @return A list of filter rules that include specific data during your transfer. For more information and
* examples, see Filtering
* data transferred by DataSync.
*/
public java.util.List getIncludes() {
return includes;
}
/**
*
* A list of filter rules that include specific data during your transfer. For more information and examples, see Filtering data transferred by
* DataSync.
*
*
* @param includes
* A list of filter rules that include specific data during your transfer. For more information and examples,
* see Filtering data
* transferred by DataSync.
*/
public void setIncludes(java.util.Collection includes) {
if (includes == null) {
this.includes = null;
return;
}
this.includes = new java.util.ArrayList(includes);
}
/**
*
* A list of filter rules that include specific data during your transfer. For more information and examples, see Filtering data transferred by
* DataSync.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setIncludes(java.util.Collection)} or {@link #withIncludes(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param includes
* A list of filter rules that include specific data during your transfer. For more information and examples,
* see Filtering data
* transferred by DataSync.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskResult withIncludes(FilterRule... includes) {
if (this.includes == null) {
setIncludes(new java.util.ArrayList(includes.length));
}
for (FilterRule ele : includes) {
this.includes.add(ele);
}
return this;
}
/**
*
* A list of filter rules that include specific data during your transfer. For more information and examples, see Filtering data transferred by
* DataSync.
*
*
* @param includes
* A list of filter rules that include specific data during your transfer. For more information and examples,
* see Filtering data
* transferred by DataSync.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskResult withIncludes(java.util.Collection includes) {
setIncludes(includes);
return this;
}
/**
*
* The configuration of your task report. For more information, see Creating a task report.
*
*
* @param taskReportConfig
* The configuration of your task report. For more information, see Creating a task report.
*/
public void setTaskReportConfig(TaskReportConfig taskReportConfig) {
this.taskReportConfig = taskReportConfig;
}
/**
*
* The configuration of your task report. For more information, see Creating a task report.
*
*
* @return The configuration of your task report. For more information, see Creating a task
* report.
*/
public TaskReportConfig getTaskReportConfig() {
return this.taskReportConfig;
}
/**
*
* The configuration of your task report. For more information, see Creating a task report.
*
*
* @param taskReportConfig
* The configuration of your task report. For more information, see Creating a task report.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTaskResult withTaskReportConfig(TaskReportConfig taskReportConfig) {
setTaskReportConfig(taskReportConfig);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTaskArn() != null)
sb.append("TaskArn: ").append(getTaskArn()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getCurrentTaskExecutionArn() != null)
sb.append("CurrentTaskExecutionArn: ").append(getCurrentTaskExecutionArn()).append(",");
if (getSourceLocationArn() != null)
sb.append("SourceLocationArn: ").append(getSourceLocationArn()).append(",");
if (getDestinationLocationArn() != null)
sb.append("DestinationLocationArn: ").append(getDestinationLocationArn()).append(",");
if (getCloudWatchLogGroupArn() != null)
sb.append("CloudWatchLogGroupArn: ").append(getCloudWatchLogGroupArn()).append(",");
if (getSourceNetworkInterfaceArns() != null)
sb.append("SourceNetworkInterfaceArns: ").append(getSourceNetworkInterfaceArns()).append(",");
if (getDestinationNetworkInterfaceArns() != null)
sb.append("DestinationNetworkInterfaceArns: ").append(getDestinationNetworkInterfaceArns()).append(",");
if (getOptions() != null)
sb.append("Options: ").append(getOptions()).append(",");
if (getExcludes() != null)
sb.append("Excludes: ").append(getExcludes()).append(",");
if (getSchedule() != null)
sb.append("Schedule: ").append(getSchedule()).append(",");
if (getErrorCode() != null)
sb.append("ErrorCode: ").append(getErrorCode()).append(",");
if (getErrorDetail() != null)
sb.append("ErrorDetail: ").append(getErrorDetail()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime()).append(",");
if (getIncludes() != null)
sb.append("Includes: ").append(getIncludes()).append(",");
if (getTaskReportConfig() != null)
sb.append("TaskReportConfig: ").append(getTaskReportConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeTaskResult == false)
return false;
DescribeTaskResult other = (DescribeTaskResult) obj;
if (other.getTaskArn() == null ^ this.getTaskArn() == null)
return false;
if (other.getTaskArn() != null && other.getTaskArn().equals(this.getTaskArn()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getCurrentTaskExecutionArn() == null ^ this.getCurrentTaskExecutionArn() == null)
return false;
if (other.getCurrentTaskExecutionArn() != null && other.getCurrentTaskExecutionArn().equals(this.getCurrentTaskExecutionArn()) == false)
return false;
if (other.getSourceLocationArn() == null ^ this.getSourceLocationArn() == null)
return false;
if (other.getSourceLocationArn() != null && other.getSourceLocationArn().equals(this.getSourceLocationArn()) == false)
return false;
if (other.getDestinationLocationArn() == null ^ this.getDestinationLocationArn() == null)
return false;
if (other.getDestinationLocationArn() != null && other.getDestinationLocationArn().equals(this.getDestinationLocationArn()) == false)
return false;
if (other.getCloudWatchLogGroupArn() == null ^ this.getCloudWatchLogGroupArn() == null)
return false;
if (other.getCloudWatchLogGroupArn() != null && other.getCloudWatchLogGroupArn().equals(this.getCloudWatchLogGroupArn()) == false)
return false;
if (other.getSourceNetworkInterfaceArns() == null ^ this.getSourceNetworkInterfaceArns() == null)
return false;
if (other.getSourceNetworkInterfaceArns() != null && other.getSourceNetworkInterfaceArns().equals(this.getSourceNetworkInterfaceArns()) == false)
return false;
if (other.getDestinationNetworkInterfaceArns() == null ^ this.getDestinationNetworkInterfaceArns() == null)
return false;
if (other.getDestinationNetworkInterfaceArns() != null
&& other.getDestinationNetworkInterfaceArns().equals(this.getDestinationNetworkInterfaceArns()) == false)
return false;
if (other.getOptions() == null ^ this.getOptions() == null)
return false;
if (other.getOptions() != null && other.getOptions().equals(this.getOptions()) == false)
return false;
if (other.getExcludes() == null ^ this.getExcludes() == null)
return false;
if (other.getExcludes() != null && other.getExcludes().equals(this.getExcludes()) == false)
return false;
if (other.getSchedule() == null ^ this.getSchedule() == null)
return false;
if (other.getSchedule() != null && other.getSchedule().equals(this.getSchedule()) == false)
return false;
if (other.getErrorCode() == null ^ this.getErrorCode() == null)
return false;
if (other.getErrorCode() != null && other.getErrorCode().equals(this.getErrorCode()) == false)
return false;
if (other.getErrorDetail() == null ^ this.getErrorDetail() == null)
return false;
if (other.getErrorDetail() != null && other.getErrorDetail().equals(this.getErrorDetail()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
if (other.getIncludes() == null ^ this.getIncludes() == null)
return false;
if (other.getIncludes() != null && other.getIncludes().equals(this.getIncludes()) == false)
return false;
if (other.getTaskReportConfig() == null ^ this.getTaskReportConfig() == null)
return false;
if (other.getTaskReportConfig() != null && other.getTaskReportConfig().equals(this.getTaskReportConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTaskArn() == null) ? 0 : getTaskArn().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getCurrentTaskExecutionArn() == null) ? 0 : getCurrentTaskExecutionArn().hashCode());
hashCode = prime * hashCode + ((getSourceLocationArn() == null) ? 0 : getSourceLocationArn().hashCode());
hashCode = prime * hashCode + ((getDestinationLocationArn() == null) ? 0 : getDestinationLocationArn().hashCode());
hashCode = prime * hashCode + ((getCloudWatchLogGroupArn() == null) ? 0 : getCloudWatchLogGroupArn().hashCode());
hashCode = prime * hashCode + ((getSourceNetworkInterfaceArns() == null) ? 0 : getSourceNetworkInterfaceArns().hashCode());
hashCode = prime * hashCode + ((getDestinationNetworkInterfaceArns() == null) ? 0 : getDestinationNetworkInterfaceArns().hashCode());
hashCode = prime * hashCode + ((getOptions() == null) ? 0 : getOptions().hashCode());
hashCode = prime * hashCode + ((getExcludes() == null) ? 0 : getExcludes().hashCode());
hashCode = prime * hashCode + ((getSchedule() == null) ? 0 : getSchedule().hashCode());
hashCode = prime * hashCode + ((getErrorCode() == null) ? 0 : getErrorCode().hashCode());
hashCode = prime * hashCode + ((getErrorDetail() == null) ? 0 : getErrorDetail().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
hashCode = prime * hashCode + ((getIncludes() == null) ? 0 : getIncludes().hashCode());
hashCode = prime * hashCode + ((getTaskReportConfig() == null) ? 0 : getTaskReportConfig().hashCode());
return hashCode;
}
@Override
public DescribeTaskResult clone() {
try {
return (DescribeTaskResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}