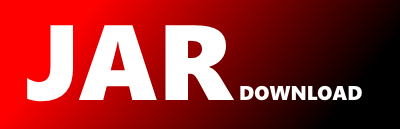
com.amazonaws.services.datasync.model.UpdateLocationAzureBlobRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-datasync Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.datasync.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateLocationAzureBlobRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* Specifies the ARN of the Azure Blob Storage transfer location that you're updating.
*
*/
private String locationArn;
/**
*
* Specifies path segments if you want to limit your transfer to a virtual directory in your container (for example,
* /my/images
).
*
*/
private String subdirectory;
/**
*
* Specifies the authentication method DataSync uses to access your Azure Blob Storage. DataSync can access blob
* storage using a shared access signature (SAS).
*
*/
private String authenticationType;
/**
*
* Specifies the SAS configuration that allows DataSync to access your Azure Blob Storage.
*
*/
private AzureBlobSasConfiguration sasConfiguration;
/**
*
* Specifies the type of blob that you want your objects or files to be when transferring them into Azure Blob
* Storage. Currently, DataSync only supports moving data into Azure Blob Storage as block blobs. For more
* information on blob types, see the Azure Blob Storage documentation.
*
*/
private String blobType;
/**
*
* Specifies the access tier that you want your objects or files transferred into. This only applies when using the
* location as a transfer destination. For more information, see Access tiers.
*
*/
private String accessTier;
/**
*
* Specifies the Amazon Resource Name (ARN) of the DataSync agent that can connect with your Azure Blob Storage
* container.
*
*
* You can specify more than one agent. For more information, see Using multiple agents for your
* transfer.
*
*/
private java.util.List agentArns;
/**
*
* Specifies the ARN of the Azure Blob Storage transfer location that you're updating.
*
*
* @param locationArn
* Specifies the ARN of the Azure Blob Storage transfer location that you're updating.
*/
public void setLocationArn(String locationArn) {
this.locationArn = locationArn;
}
/**
*
* Specifies the ARN of the Azure Blob Storage transfer location that you're updating.
*
*
* @return Specifies the ARN of the Azure Blob Storage transfer location that you're updating.
*/
public String getLocationArn() {
return this.locationArn;
}
/**
*
* Specifies the ARN of the Azure Blob Storage transfer location that you're updating.
*
*
* @param locationArn
* Specifies the ARN of the Azure Blob Storage transfer location that you're updating.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateLocationAzureBlobRequest withLocationArn(String locationArn) {
setLocationArn(locationArn);
return this;
}
/**
*
* Specifies path segments if you want to limit your transfer to a virtual directory in your container (for example,
* /my/images
).
*
*
* @param subdirectory
* Specifies path segments if you want to limit your transfer to a virtual directory in your container (for
* example, /my/images
).
*/
public void setSubdirectory(String subdirectory) {
this.subdirectory = subdirectory;
}
/**
*
* Specifies path segments if you want to limit your transfer to a virtual directory in your container (for example,
* /my/images
).
*
*
* @return Specifies path segments if you want to limit your transfer to a virtual directory in your container (for
* example, /my/images
).
*/
public String getSubdirectory() {
return this.subdirectory;
}
/**
*
* Specifies path segments if you want to limit your transfer to a virtual directory in your container (for example,
* /my/images
).
*
*
* @param subdirectory
* Specifies path segments if you want to limit your transfer to a virtual directory in your container (for
* example, /my/images
).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateLocationAzureBlobRequest withSubdirectory(String subdirectory) {
setSubdirectory(subdirectory);
return this;
}
/**
*
* Specifies the authentication method DataSync uses to access your Azure Blob Storage. DataSync can access blob
* storage using a shared access signature (SAS).
*
*
* @param authenticationType
* Specifies the authentication method DataSync uses to access your Azure Blob Storage. DataSync can access
* blob storage using a shared access signature (SAS).
* @see AzureBlobAuthenticationType
*/
public void setAuthenticationType(String authenticationType) {
this.authenticationType = authenticationType;
}
/**
*
* Specifies the authentication method DataSync uses to access your Azure Blob Storage. DataSync can access blob
* storage using a shared access signature (SAS).
*
*
* @return Specifies the authentication method DataSync uses to access your Azure Blob Storage. DataSync can access
* blob storage using a shared access signature (SAS).
* @see AzureBlobAuthenticationType
*/
public String getAuthenticationType() {
return this.authenticationType;
}
/**
*
* Specifies the authentication method DataSync uses to access your Azure Blob Storage. DataSync can access blob
* storage using a shared access signature (SAS).
*
*
* @param authenticationType
* Specifies the authentication method DataSync uses to access your Azure Blob Storage. DataSync can access
* blob storage using a shared access signature (SAS).
* @return Returns a reference to this object so that method calls can be chained together.
* @see AzureBlobAuthenticationType
*/
public UpdateLocationAzureBlobRequest withAuthenticationType(String authenticationType) {
setAuthenticationType(authenticationType);
return this;
}
/**
*
* Specifies the authentication method DataSync uses to access your Azure Blob Storage. DataSync can access blob
* storage using a shared access signature (SAS).
*
*
* @param authenticationType
* Specifies the authentication method DataSync uses to access your Azure Blob Storage. DataSync can access
* blob storage using a shared access signature (SAS).
* @return Returns a reference to this object so that method calls can be chained together.
* @see AzureBlobAuthenticationType
*/
public UpdateLocationAzureBlobRequest withAuthenticationType(AzureBlobAuthenticationType authenticationType) {
this.authenticationType = authenticationType.toString();
return this;
}
/**
*
* Specifies the SAS configuration that allows DataSync to access your Azure Blob Storage.
*
*
* @param sasConfiguration
* Specifies the SAS configuration that allows DataSync to access your Azure Blob Storage.
*/
public void setSasConfiguration(AzureBlobSasConfiguration sasConfiguration) {
this.sasConfiguration = sasConfiguration;
}
/**
*
* Specifies the SAS configuration that allows DataSync to access your Azure Blob Storage.
*
*
* @return Specifies the SAS configuration that allows DataSync to access your Azure Blob Storage.
*/
public AzureBlobSasConfiguration getSasConfiguration() {
return this.sasConfiguration;
}
/**
*
* Specifies the SAS configuration that allows DataSync to access your Azure Blob Storage.
*
*
* @param sasConfiguration
* Specifies the SAS configuration that allows DataSync to access your Azure Blob Storage.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateLocationAzureBlobRequest withSasConfiguration(AzureBlobSasConfiguration sasConfiguration) {
setSasConfiguration(sasConfiguration);
return this;
}
/**
*
* Specifies the type of blob that you want your objects or files to be when transferring them into Azure Blob
* Storage. Currently, DataSync only supports moving data into Azure Blob Storage as block blobs. For more
* information on blob types, see the Azure Blob Storage documentation.
*
*
* @param blobType
* Specifies the type of blob that you want your objects or files to be when transferring them into Azure
* Blob Storage. Currently, DataSync only supports moving data into Azure Blob Storage as block blobs. For
* more information on blob types, see the Azure Blob Storage documentation.
* @see AzureBlobType
*/
public void setBlobType(String blobType) {
this.blobType = blobType;
}
/**
*
* Specifies the type of blob that you want your objects or files to be when transferring them into Azure Blob
* Storage. Currently, DataSync only supports moving data into Azure Blob Storage as block blobs. For more
* information on blob types, see the Azure Blob Storage documentation.
*
*
* @return Specifies the type of blob that you want your objects or files to be when transferring them into Azure
* Blob Storage. Currently, DataSync only supports moving data into Azure Blob Storage as block blobs. For
* more information on blob types, see the Azure Blob Storage documentation.
* @see AzureBlobType
*/
public String getBlobType() {
return this.blobType;
}
/**
*
* Specifies the type of blob that you want your objects or files to be when transferring them into Azure Blob
* Storage. Currently, DataSync only supports moving data into Azure Blob Storage as block blobs. For more
* information on blob types, see the Azure Blob Storage documentation.
*
*
* @param blobType
* Specifies the type of blob that you want your objects or files to be when transferring them into Azure
* Blob Storage. Currently, DataSync only supports moving data into Azure Blob Storage as block blobs. For
* more information on blob types, see the Azure Blob Storage documentation.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AzureBlobType
*/
public UpdateLocationAzureBlobRequest withBlobType(String blobType) {
setBlobType(blobType);
return this;
}
/**
*
* Specifies the type of blob that you want your objects or files to be when transferring them into Azure Blob
* Storage. Currently, DataSync only supports moving data into Azure Blob Storage as block blobs. For more
* information on blob types, see the Azure Blob Storage documentation.
*
*
* @param blobType
* Specifies the type of blob that you want your objects or files to be when transferring them into Azure
* Blob Storage. Currently, DataSync only supports moving data into Azure Blob Storage as block blobs. For
* more information on blob types, see the Azure Blob Storage documentation.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AzureBlobType
*/
public UpdateLocationAzureBlobRequest withBlobType(AzureBlobType blobType) {
this.blobType = blobType.toString();
return this;
}
/**
*
* Specifies the access tier that you want your objects or files transferred into. This only applies when using the
* location as a transfer destination. For more information, see Access tiers.
*
*
* @param accessTier
* Specifies the access tier that you want your objects or files transferred into. This only applies when
* using the location as a transfer destination. For more information, see Access tiers.
* @see AzureAccessTier
*/
public void setAccessTier(String accessTier) {
this.accessTier = accessTier;
}
/**
*
* Specifies the access tier that you want your objects or files transferred into. This only applies when using the
* location as a transfer destination. For more information, see Access tiers.
*
*
* @return Specifies the access tier that you want your objects or files transferred into. This only applies when
* using the location as a transfer destination. For more information, see Access tiers.
* @see AzureAccessTier
*/
public String getAccessTier() {
return this.accessTier;
}
/**
*
* Specifies the access tier that you want your objects or files transferred into. This only applies when using the
* location as a transfer destination. For more information, see Access tiers.
*
*
* @param accessTier
* Specifies the access tier that you want your objects or files transferred into. This only applies when
* using the location as a transfer destination. For more information, see Access tiers.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AzureAccessTier
*/
public UpdateLocationAzureBlobRequest withAccessTier(String accessTier) {
setAccessTier(accessTier);
return this;
}
/**
*
* Specifies the access tier that you want your objects or files transferred into. This only applies when using the
* location as a transfer destination. For more information, see Access tiers.
*
*
* @param accessTier
* Specifies the access tier that you want your objects or files transferred into. This only applies when
* using the location as a transfer destination. For more information, see Access tiers.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AzureAccessTier
*/
public UpdateLocationAzureBlobRequest withAccessTier(AzureAccessTier accessTier) {
this.accessTier = accessTier.toString();
return this;
}
/**
*
* Specifies the Amazon Resource Name (ARN) of the DataSync agent that can connect with your Azure Blob Storage
* container.
*
*
* You can specify more than one agent. For more information, see Using multiple agents for your
* transfer.
*
*
* @return Specifies the Amazon Resource Name (ARN) of the DataSync agent that can connect with your Azure Blob
* Storage container.
*
* You can specify more than one agent. For more information, see Using multiple agents
* for your transfer.
*/
public java.util.List getAgentArns() {
return agentArns;
}
/**
*
* Specifies the Amazon Resource Name (ARN) of the DataSync agent that can connect with your Azure Blob Storage
* container.
*
*
* You can specify more than one agent. For more information, see Using multiple agents for your
* transfer.
*
*
* @param agentArns
* Specifies the Amazon Resource Name (ARN) of the DataSync agent that can connect with your Azure Blob
* Storage container.
*
* You can specify more than one agent. For more information, see Using multiple agents
* for your transfer.
*/
public void setAgentArns(java.util.Collection agentArns) {
if (agentArns == null) {
this.agentArns = null;
return;
}
this.agentArns = new java.util.ArrayList(agentArns);
}
/**
*
* Specifies the Amazon Resource Name (ARN) of the DataSync agent that can connect with your Azure Blob Storage
* container.
*
*
* You can specify more than one agent. For more information, see Using multiple agents for your
* transfer.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAgentArns(java.util.Collection)} or {@link #withAgentArns(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param agentArns
* Specifies the Amazon Resource Name (ARN) of the DataSync agent that can connect with your Azure Blob
* Storage container.
*
* You can specify more than one agent. For more information, see Using multiple agents
* for your transfer.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateLocationAzureBlobRequest withAgentArns(String... agentArns) {
if (this.agentArns == null) {
setAgentArns(new java.util.ArrayList(agentArns.length));
}
for (String ele : agentArns) {
this.agentArns.add(ele);
}
return this;
}
/**
*
* Specifies the Amazon Resource Name (ARN) of the DataSync agent that can connect with your Azure Blob Storage
* container.
*
*
* You can specify more than one agent. For more information, see Using multiple agents for your
* transfer.
*
*
* @param agentArns
* Specifies the Amazon Resource Name (ARN) of the DataSync agent that can connect with your Azure Blob
* Storage container.
*
* You can specify more than one agent. For more information, see Using multiple agents
* for your transfer.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateLocationAzureBlobRequest withAgentArns(java.util.Collection agentArns) {
setAgentArns(agentArns);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getLocationArn() != null)
sb.append("LocationArn: ").append(getLocationArn()).append(",");
if (getSubdirectory() != null)
sb.append("Subdirectory: ").append(getSubdirectory()).append(",");
if (getAuthenticationType() != null)
sb.append("AuthenticationType: ").append(getAuthenticationType()).append(",");
if (getSasConfiguration() != null)
sb.append("SasConfiguration: ").append(getSasConfiguration()).append(",");
if (getBlobType() != null)
sb.append("BlobType: ").append(getBlobType()).append(",");
if (getAccessTier() != null)
sb.append("AccessTier: ").append(getAccessTier()).append(",");
if (getAgentArns() != null)
sb.append("AgentArns: ").append(getAgentArns());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateLocationAzureBlobRequest == false)
return false;
UpdateLocationAzureBlobRequest other = (UpdateLocationAzureBlobRequest) obj;
if (other.getLocationArn() == null ^ this.getLocationArn() == null)
return false;
if (other.getLocationArn() != null && other.getLocationArn().equals(this.getLocationArn()) == false)
return false;
if (other.getSubdirectory() == null ^ this.getSubdirectory() == null)
return false;
if (other.getSubdirectory() != null && other.getSubdirectory().equals(this.getSubdirectory()) == false)
return false;
if (other.getAuthenticationType() == null ^ this.getAuthenticationType() == null)
return false;
if (other.getAuthenticationType() != null && other.getAuthenticationType().equals(this.getAuthenticationType()) == false)
return false;
if (other.getSasConfiguration() == null ^ this.getSasConfiguration() == null)
return false;
if (other.getSasConfiguration() != null && other.getSasConfiguration().equals(this.getSasConfiguration()) == false)
return false;
if (other.getBlobType() == null ^ this.getBlobType() == null)
return false;
if (other.getBlobType() != null && other.getBlobType().equals(this.getBlobType()) == false)
return false;
if (other.getAccessTier() == null ^ this.getAccessTier() == null)
return false;
if (other.getAccessTier() != null && other.getAccessTier().equals(this.getAccessTier()) == false)
return false;
if (other.getAgentArns() == null ^ this.getAgentArns() == null)
return false;
if (other.getAgentArns() != null && other.getAgentArns().equals(this.getAgentArns()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getLocationArn() == null) ? 0 : getLocationArn().hashCode());
hashCode = prime * hashCode + ((getSubdirectory() == null) ? 0 : getSubdirectory().hashCode());
hashCode = prime * hashCode + ((getAuthenticationType() == null) ? 0 : getAuthenticationType().hashCode());
hashCode = prime * hashCode + ((getSasConfiguration() == null) ? 0 : getSasConfiguration().hashCode());
hashCode = prime * hashCode + ((getBlobType() == null) ? 0 : getBlobType().hashCode());
hashCode = prime * hashCode + ((getAccessTier() == null) ? 0 : getAccessTier().hashCode());
hashCode = prime * hashCode + ((getAgentArns() == null) ? 0 : getAgentArns().hashCode());
return hashCode;
}
@Override
public UpdateLocationAzureBlobRequest clone() {
return (UpdateLocationAzureBlobRequest) super.clone();
}
}