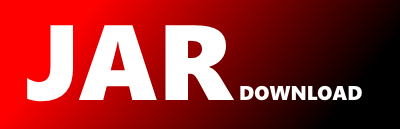
com.amazonaws.services.datazone.AmazonDataZone Maven / Gradle / Ivy
Show all versions of aws-java-sdk-datazoneexternal Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.datazone;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.datazone.model.*;
/**
* Interface for accessing Amazon DataZone.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.datazone.AbstractAmazonDataZone} instead.
*
*
*
* Amazon DataZone is a data management service that enables you to catalog, discover, govern, share, and analyze your
* data. With Amazon DataZone, you can share and access your data across accounts and supported regions. Amazon DataZone
* simplifies your experience across Amazon Web Services services, including, but not limited to, Amazon Redshift,
* Amazon Athena, Amazon Web Services Glue, and Amazon Web Services Lake Formation.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonDataZone {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "datazone";
/**
*
* Accepts automatically generated business-friendly metadata for your Amazon DataZone assets.
*
*
* @param acceptPredictionsRequest
* @return Result of the AcceptPredictions operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.AcceptPredictions
* @see AWS API
* Documentation
*/
AcceptPredictionsResult acceptPredictions(AcceptPredictionsRequest acceptPredictionsRequest);
/**
*
* Accepts a subscription request to a specific asset.
*
*
* @param acceptSubscriptionRequestRequest
* @return Result of the AcceptSubscriptionRequest operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.AcceptSubscriptionRequest
* @see AWS API Documentation
*/
AcceptSubscriptionRequestResult acceptSubscriptionRequest(AcceptSubscriptionRequestRequest acceptSubscriptionRequestRequest);
/**
*
* Associates the environment role in Amazon DataZone.
*
*
* @param associateEnvironmentRoleRequest
* @return Result of the AssociateEnvironmentRole operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.AssociateEnvironmentRole
* @see AWS API Documentation
*/
AssociateEnvironmentRoleResult associateEnvironmentRole(AssociateEnvironmentRoleRequest associateEnvironmentRoleRequest);
/**
*
* Cancels the metadata generation run.
*
*
* @param cancelMetadataGenerationRunRequest
* @return Result of the CancelMetadataGenerationRun operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CancelMetadataGenerationRun
* @see AWS API Documentation
*/
CancelMetadataGenerationRunResult cancelMetadataGenerationRun(CancelMetadataGenerationRunRequest cancelMetadataGenerationRunRequest);
/**
*
* Cancels the subscription to the specified asset.
*
*
* @param cancelSubscriptionRequest
* @return Result of the CancelSubscription operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CancelSubscription
* @see AWS
* API Documentation
*/
CancelSubscriptionResult cancelSubscription(CancelSubscriptionRequest cancelSubscriptionRequest);
/**
*
* Creates an asset in Amazon DataZone catalog.
*
*
* @param createAssetRequest
* @return Result of the CreateAsset operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateAsset
* @see AWS API
* Documentation
*/
CreateAssetResult createAsset(CreateAssetRequest createAssetRequest);
/**
*
* Creates a data asset filter.
*
*
* @param createAssetFilterRequest
* @return Result of the CreateAssetFilter operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateAssetFilter
* @see AWS API
* Documentation
*/
CreateAssetFilterResult createAssetFilter(CreateAssetFilterRequest createAssetFilterRequest);
/**
*
* Creates a revision of the asset.
*
*
* @param createAssetRevisionRequest
* @return Result of the CreateAssetRevision operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateAssetRevision
* @see AWS
* API Documentation
*/
CreateAssetRevisionResult createAssetRevision(CreateAssetRevisionRequest createAssetRevisionRequest);
/**
*
* Creates a custom asset type.
*
*
* @param createAssetTypeRequest
* @return Result of the CreateAssetType operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateAssetType
* @see AWS API
* Documentation
*/
CreateAssetTypeResult createAssetType(CreateAssetTypeRequest createAssetTypeRequest);
/**
*
* Creates an Amazon DataZone data source.
*
*
* @param createDataSourceRequest
* @return Result of the CreateDataSource operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateDataSource
* @see AWS API
* Documentation
*/
CreateDataSourceResult createDataSource(CreateDataSourceRequest createDataSourceRequest);
/**
*
* Creates an Amazon DataZone domain.
*
*
* @param createDomainRequest
* @return Result of the CreateDomain operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateDomain
* @see AWS API
* Documentation
*/
CreateDomainResult createDomain(CreateDomainRequest createDomainRequest);
/**
*
* Create an Amazon DataZone environment.
*
*
* @param createEnvironmentRequest
* @return Result of the CreateEnvironment operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateEnvironment
* @see AWS API
* Documentation
*/
CreateEnvironmentResult createEnvironment(CreateEnvironmentRequest createEnvironmentRequest);
/**
*
* Creates an action for the environment, for example, creates a console link for an analytics tool that is
* available in this environment.
*
*
* @param createEnvironmentActionRequest
* @return Result of the CreateEnvironmentAction operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateEnvironmentAction
* @see AWS API Documentation
*/
CreateEnvironmentActionResult createEnvironmentAction(CreateEnvironmentActionRequest createEnvironmentActionRequest);
/**
*
* Creates an Amazon DataZone environment profile.
*
*
* @param createEnvironmentProfileRequest
* @return Result of the CreateEnvironmentProfile operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateEnvironmentProfile
* @see AWS API Documentation
*/
CreateEnvironmentProfileResult createEnvironmentProfile(CreateEnvironmentProfileRequest createEnvironmentProfileRequest);
/**
*
* Creates a metadata form type.
*
*
* @param createFormTypeRequest
* @return Result of the CreateFormType operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateFormType
* @see AWS API
* Documentation
*/
CreateFormTypeResult createFormType(CreateFormTypeRequest createFormTypeRequest);
/**
*
* Creates an Amazon DataZone business glossary.
*
*
* @param createGlossaryRequest
* @return Result of the CreateGlossary operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateGlossary
* @see AWS API
* Documentation
*/
CreateGlossaryResult createGlossary(CreateGlossaryRequest createGlossaryRequest);
/**
*
* Creates a business glossary term.
*
*
* @param createGlossaryTermRequest
* @return Result of the CreateGlossaryTerm operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateGlossaryTerm
* @see AWS
* API Documentation
*/
CreateGlossaryTermResult createGlossaryTerm(CreateGlossaryTermRequest createGlossaryTermRequest);
/**
*
* Creates a group profile in Amazon DataZone.
*
*
* @param createGroupProfileRequest
* @return Result of the CreateGroupProfile operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateGroupProfile
* @see AWS
* API Documentation
*/
CreateGroupProfileResult createGroupProfile(CreateGroupProfileRequest createGroupProfileRequest);
/**
*
* Publishes a listing (a record of an asset at a given time) or removes a listing from the catalog.
*
*
* @param createListingChangeSetRequest
* @return Result of the CreateListingChangeSet operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateListingChangeSet
* @see AWS API Documentation
*/
CreateListingChangeSetResult createListingChangeSet(CreateListingChangeSetRequest createListingChangeSetRequest);
/**
*
* Creates an Amazon DataZone project.
*
*
* @param createProjectRequest
* @return Result of the CreateProject operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateProject
* @see AWS API
* Documentation
*/
CreateProjectResult createProject(CreateProjectRequest createProjectRequest);
/**
*
* Creates a project membership in Amazon DataZone.
*
*
* @param createProjectMembershipRequest
* @return Result of the CreateProjectMembership operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateProjectMembership
* @see AWS API Documentation
*/
CreateProjectMembershipResult createProjectMembership(CreateProjectMembershipRequest createProjectMembershipRequest);
/**
*
* Creates a subsscription grant in Amazon DataZone.
*
*
* @param createSubscriptionGrantRequest
* @return Result of the CreateSubscriptionGrant operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateSubscriptionGrant
* @see AWS API Documentation
*/
CreateSubscriptionGrantResult createSubscriptionGrant(CreateSubscriptionGrantRequest createSubscriptionGrantRequest);
/**
*
* Creates a subscription request in Amazon DataZone.
*
*
* @param createSubscriptionRequestRequest
* @return Result of the CreateSubscriptionRequest operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateSubscriptionRequest
* @see AWS API Documentation
*/
CreateSubscriptionRequestResult createSubscriptionRequest(CreateSubscriptionRequestRequest createSubscriptionRequestRequest);
/**
*
* Creates a subscription target in Amazon DataZone.
*
*
* @param createSubscriptionTargetRequest
* @return Result of the CreateSubscriptionTarget operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateSubscriptionTarget
* @see AWS API Documentation
*/
CreateSubscriptionTargetResult createSubscriptionTarget(CreateSubscriptionTargetRequest createSubscriptionTargetRequest);
/**
*
* Creates a user profile in Amazon DataZone.
*
*
* @param createUserProfileRequest
* @return Result of the CreateUserProfile operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateUserProfile
* @see AWS API
* Documentation
*/
CreateUserProfileResult createUserProfile(CreateUserProfileRequest createUserProfileRequest);
/**
*
* Delets an asset in Amazon DataZone.
*
*
* @param deleteAssetRequest
* @return Result of the DeleteAsset operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteAsset
* @see AWS API
* Documentation
*/
DeleteAssetResult deleteAsset(DeleteAssetRequest deleteAssetRequest);
/**
*
* Deletes an asset filter.
*
*
* @param deleteAssetFilterRequest
* @return Result of the DeleteAssetFilter operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteAssetFilter
* @see AWS API
* Documentation
*/
DeleteAssetFilterResult deleteAssetFilter(DeleteAssetFilterRequest deleteAssetFilterRequest);
/**
*
* Deletes an asset type in Amazon DataZone.
*
*
* @param deleteAssetTypeRequest
* @return Result of the DeleteAssetType operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteAssetType
* @see AWS API
* Documentation
*/
DeleteAssetTypeResult deleteAssetType(DeleteAssetTypeRequest deleteAssetTypeRequest);
/**
*
* Deletes a data source in Amazon DataZone.
*
*
* @param deleteDataSourceRequest
* @return Result of the DeleteDataSource operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteDataSource
* @see AWS API
* Documentation
*/
DeleteDataSourceResult deleteDataSource(DeleteDataSourceRequest deleteDataSourceRequest);
/**
*
* Deletes a Amazon DataZone domain.
*
*
* @param deleteDomainRequest
* @return Result of the DeleteDomain operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteDomain
* @see AWS API
* Documentation
*/
DeleteDomainResult deleteDomain(DeleteDomainRequest deleteDomainRequest);
/**
*
* Deletes an environment in Amazon DataZone.
*
*
* @param deleteEnvironmentRequest
* @return Result of the DeleteEnvironment operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteEnvironment
* @see AWS API
* Documentation
*/
DeleteEnvironmentResult deleteEnvironment(DeleteEnvironmentRequest deleteEnvironmentRequest);
/**
*
* Deletes an action for the environment, for example, deletes a console link for an analytics tool that is
* available in this environment.
*
*
* @param deleteEnvironmentActionRequest
* @return Result of the DeleteEnvironmentAction operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteEnvironmentAction
* @see AWS API Documentation
*/
DeleteEnvironmentActionResult deleteEnvironmentAction(DeleteEnvironmentActionRequest deleteEnvironmentActionRequest);
/**
*
* Deletes the blueprint configuration in Amazon DataZone.
*
*
* @param deleteEnvironmentBlueprintConfigurationRequest
* @return Result of the DeleteEnvironmentBlueprintConfiguration operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteEnvironmentBlueprintConfiguration
* @see AWS API Documentation
*/
DeleteEnvironmentBlueprintConfigurationResult deleteEnvironmentBlueprintConfiguration(
DeleteEnvironmentBlueprintConfigurationRequest deleteEnvironmentBlueprintConfigurationRequest);
/**
*
* Deletes an environment profile in Amazon DataZone.
*
*
* @param deleteEnvironmentProfileRequest
* @return Result of the DeleteEnvironmentProfile operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteEnvironmentProfile
* @see AWS API Documentation
*/
DeleteEnvironmentProfileResult deleteEnvironmentProfile(DeleteEnvironmentProfileRequest deleteEnvironmentProfileRequest);
/**
*
* Delets and metadata form type in Amazon DataZone.
*
*
* @param deleteFormTypeRequest
* @return Result of the DeleteFormType operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteFormType
* @see AWS API
* Documentation
*/
DeleteFormTypeResult deleteFormType(DeleteFormTypeRequest deleteFormTypeRequest);
/**
*
* Deletes a business glossary in Amazon DataZone.
*
*
* @param deleteGlossaryRequest
* @return Result of the DeleteGlossary operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteGlossary
* @see AWS API
* Documentation
*/
DeleteGlossaryResult deleteGlossary(DeleteGlossaryRequest deleteGlossaryRequest);
/**
*
* Deletes a business glossary term in Amazon DataZone.
*
*
* @param deleteGlossaryTermRequest
* @return Result of the DeleteGlossaryTerm operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteGlossaryTerm
* @see AWS
* API Documentation
*/
DeleteGlossaryTermResult deleteGlossaryTerm(DeleteGlossaryTermRequest deleteGlossaryTermRequest);
/**
*
* Deletes a listing (a record of an asset at a given time).
*
*
* @param deleteListingRequest
* @return Result of the DeleteListing operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteListing
* @see AWS API
* Documentation
*/
DeleteListingResult deleteListing(DeleteListingRequest deleteListingRequest);
/**
*
* Deletes a project in Amazon DataZone.
*
*
* @param deleteProjectRequest
* @return Result of the DeleteProject operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteProject
* @see AWS API
* Documentation
*/
DeleteProjectResult deleteProject(DeleteProjectRequest deleteProjectRequest);
/**
*
* Deletes project membership in Amazon DataZone.
*
*
* @param deleteProjectMembershipRequest
* @return Result of the DeleteProjectMembership operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteProjectMembership
* @see AWS API Documentation
*/
DeleteProjectMembershipResult deleteProjectMembership(DeleteProjectMembershipRequest deleteProjectMembershipRequest);
/**
*
* Deletes and subscription grant in Amazon DataZone.
*
*
* @param deleteSubscriptionGrantRequest
* @return Result of the DeleteSubscriptionGrant operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteSubscriptionGrant
* @see AWS API Documentation
*/
DeleteSubscriptionGrantResult deleteSubscriptionGrant(DeleteSubscriptionGrantRequest deleteSubscriptionGrantRequest);
/**
*
* Deletes a subscription request in Amazon DataZone.
*
*
* @param deleteSubscriptionRequestRequest
* @return Result of the DeleteSubscriptionRequest operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteSubscriptionRequest
* @see AWS API Documentation
*/
DeleteSubscriptionRequestResult deleteSubscriptionRequest(DeleteSubscriptionRequestRequest deleteSubscriptionRequestRequest);
/**
*
* Deletes a subscription target in Amazon DataZone.
*
*
* @param deleteSubscriptionTargetRequest
* @return Result of the DeleteSubscriptionTarget operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteSubscriptionTarget
* @see AWS API Documentation
*/
DeleteSubscriptionTargetResult deleteSubscriptionTarget(DeleteSubscriptionTargetRequest deleteSubscriptionTargetRequest);
/**
*
* Deletes the specified time series form for the specified asset.
*
*
* @param deleteTimeSeriesDataPointsRequest
* @return Result of the DeleteTimeSeriesDataPoints operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteTimeSeriesDataPoints
* @see AWS API Documentation
*/
DeleteTimeSeriesDataPointsResult deleteTimeSeriesDataPoints(DeleteTimeSeriesDataPointsRequest deleteTimeSeriesDataPointsRequest);
/**
*
* Disassociates the environment role in Amazon DataZone.
*
*
* @param disassociateEnvironmentRoleRequest
* @return Result of the DisassociateEnvironmentRole operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DisassociateEnvironmentRole
* @see AWS API Documentation
*/
DisassociateEnvironmentRoleResult disassociateEnvironmentRole(DisassociateEnvironmentRoleRequest disassociateEnvironmentRoleRequest);
/**
*
* Gets an Amazon DataZone asset.
*
*
* @param getAssetRequest
* @return Result of the GetAsset operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetAsset
* @see AWS API
* Documentation
*/
GetAssetResult getAsset(GetAssetRequest getAssetRequest);
/**
*
* Gets an asset filter.
*
*
* @param getAssetFilterRequest
* @return Result of the GetAssetFilter operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetAssetFilter
* @see AWS API
* Documentation
*/
GetAssetFilterResult getAssetFilter(GetAssetFilterRequest getAssetFilterRequest);
/**
*
* Gets an Amazon DataZone asset type.
*
*
* @param getAssetTypeRequest
* @return Result of the GetAssetType operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetAssetType
* @see AWS API
* Documentation
*/
GetAssetTypeResult getAssetType(GetAssetTypeRequest getAssetTypeRequest);
/**
*
* Gets an Amazon DataZone data source.
*
*
* @param getDataSourceRequest
* @return Result of the GetDataSource operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetDataSource
* @see AWS API
* Documentation
*/
GetDataSourceResult getDataSource(GetDataSourceRequest getDataSourceRequest);
/**
*
* Gets an Amazon DataZone data source run.
*
*
* @param getDataSourceRunRequest
* @return Result of the GetDataSourceRun operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetDataSourceRun
* @see AWS API
* Documentation
*/
GetDataSourceRunResult getDataSourceRun(GetDataSourceRunRequest getDataSourceRunRequest);
/**
*
* Gets an Amazon DataZone domain.
*
*
* @param getDomainRequest
* @return Result of the GetDomain operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetDomain
* @see AWS API
* Documentation
*/
GetDomainResult getDomain(GetDomainRequest getDomainRequest);
/**
*
* Gets an Amazon DataZone environment.
*
*
* @param getEnvironmentRequest
* @return Result of the GetEnvironment operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetEnvironment
* @see AWS API
* Documentation
*/
GetEnvironmentResult getEnvironment(GetEnvironmentRequest getEnvironmentRequest);
/**
*
* Gets the specified environment action.
*
*
* @param getEnvironmentActionRequest
* @return Result of the GetEnvironmentAction operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetEnvironmentAction
* @see AWS
* API Documentation
*/
GetEnvironmentActionResult getEnvironmentAction(GetEnvironmentActionRequest getEnvironmentActionRequest);
/**
*
* Gets an Amazon DataZone blueprint.
*
*
* @param getEnvironmentBlueprintRequest
* @return Result of the GetEnvironmentBlueprint operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetEnvironmentBlueprint
* @see AWS API Documentation
*/
GetEnvironmentBlueprintResult getEnvironmentBlueprint(GetEnvironmentBlueprintRequest getEnvironmentBlueprintRequest);
/**
*
* Gets the blueprint configuration in Amazon DataZone.
*
*
* @param getEnvironmentBlueprintConfigurationRequest
* @return Result of the GetEnvironmentBlueprintConfiguration operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetEnvironmentBlueprintConfiguration
* @see AWS API Documentation
*/
GetEnvironmentBlueprintConfigurationResult getEnvironmentBlueprintConfiguration(
GetEnvironmentBlueprintConfigurationRequest getEnvironmentBlueprintConfigurationRequest);
/**
*
* Gets the credentials of an environment in Amazon DataZone.
*
*
* @param getEnvironmentCredentialsRequest
* @return Result of the GetEnvironmentCredentials operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetEnvironmentCredentials
* @see AWS API Documentation
*/
GetEnvironmentCredentialsResult getEnvironmentCredentials(GetEnvironmentCredentialsRequest getEnvironmentCredentialsRequest);
/**
*
* Gets an evinronment profile in Amazon DataZone.
*
*
* @param getEnvironmentProfileRequest
* @return Result of the GetEnvironmentProfile operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetEnvironmentProfile
* @see AWS
* API Documentation
*/
GetEnvironmentProfileResult getEnvironmentProfile(GetEnvironmentProfileRequest getEnvironmentProfileRequest);
/**
*
* Gets a metadata form type in Amazon DataZone.
*
*
* @param getFormTypeRequest
* @return Result of the GetFormType operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetFormType
* @see AWS API
* Documentation
*/
GetFormTypeResult getFormType(GetFormTypeRequest getFormTypeRequest);
/**
*
* Gets a business glossary in Amazon DataZone.
*
*
* @param getGlossaryRequest
* @return Result of the GetGlossary operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetGlossary
* @see AWS API
* Documentation
*/
GetGlossaryResult getGlossary(GetGlossaryRequest getGlossaryRequest);
/**
*
* Gets a business glossary term in Amazon DataZone.
*
*
* @param getGlossaryTermRequest
* @return Result of the GetGlossaryTerm operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetGlossaryTerm
* @see AWS API
* Documentation
*/
GetGlossaryTermResult getGlossaryTerm(GetGlossaryTermRequest getGlossaryTermRequest);
/**
*
* Gets a group profile in Amazon DataZone.
*
*
* @param getGroupProfileRequest
* @return Result of the GetGroupProfile operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetGroupProfile
* @see AWS API
* Documentation
*/
GetGroupProfileResult getGroupProfile(GetGroupProfileRequest getGroupProfileRequest);
/**
*
* Gets the data portal URL for the specified Amazon DataZone domain.
*
*
* @param getIamPortalLoginUrlRequest
* @return Result of the GetIamPortalLoginUrl operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetIamPortalLoginUrl
* @see AWS
* API Documentation
*/
GetIamPortalLoginUrlResult getIamPortalLoginUrl(GetIamPortalLoginUrlRequest getIamPortalLoginUrlRequest);
/**
*
* Gets the data lineage node.
*
*
* @param getLineageNodeRequest
* @return Result of the GetLineageNode operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetLineageNode
* @see AWS API
* Documentation
*/
GetLineageNodeResult getLineageNode(GetLineageNodeRequest getLineageNodeRequest);
/**
*
* Gets a listing (a record of an asset at a given time).
*
*
* @param getListingRequest
* @return Result of the GetListing operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetListing
* @see AWS API
* Documentation
*/
GetListingResult getListing(GetListingRequest getListingRequest);
/**
*
* Gets a metadata generation run in Amazon DataZone.
*
*
* @param getMetadataGenerationRunRequest
* @return Result of the GetMetadataGenerationRun operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetMetadataGenerationRun
* @see AWS API Documentation
*/
GetMetadataGenerationRunResult getMetadataGenerationRun(GetMetadataGenerationRunRequest getMetadataGenerationRunRequest);
/**
*
* Gets a project in Amazon DataZone.
*
*
* @param getProjectRequest
* @return Result of the GetProject operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetProject
* @see AWS API
* Documentation
*/
GetProjectResult getProject(GetProjectRequest getProjectRequest);
/**
*
* Gets a subscription in Amazon DataZone.
*
*
* @param getSubscriptionRequest
* @return Result of the GetSubscription operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetSubscription
* @see AWS API
* Documentation
*/
GetSubscriptionResult getSubscription(GetSubscriptionRequest getSubscriptionRequest);
/**
*
* Gets the subscription grant in Amazon DataZone.
*
*
* @param getSubscriptionGrantRequest
* @return Result of the GetSubscriptionGrant operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetSubscriptionGrant
* @see AWS
* API Documentation
*/
GetSubscriptionGrantResult getSubscriptionGrant(GetSubscriptionGrantRequest getSubscriptionGrantRequest);
/**
*
* Gets the details of the specified subscription request.
*
*
* @param getSubscriptionRequestDetailsRequest
* @return Result of the GetSubscriptionRequestDetails operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetSubscriptionRequestDetails
* @see AWS API Documentation
*/
GetSubscriptionRequestDetailsResult getSubscriptionRequestDetails(GetSubscriptionRequestDetailsRequest getSubscriptionRequestDetailsRequest);
/**
*
* Gets the subscription target in Amazon DataZone.
*
*
* @param getSubscriptionTargetRequest
* @return Result of the GetSubscriptionTarget operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetSubscriptionTarget
* @see AWS
* API Documentation
*/
GetSubscriptionTargetResult getSubscriptionTarget(GetSubscriptionTargetRequest getSubscriptionTargetRequest);
/**
*
* Gets the existing data point for the asset.
*
*
* @param getTimeSeriesDataPointRequest
* @return Result of the GetTimeSeriesDataPoint operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetTimeSeriesDataPoint
* @see AWS API Documentation
*/
GetTimeSeriesDataPointResult getTimeSeriesDataPoint(GetTimeSeriesDataPointRequest getTimeSeriesDataPointRequest);
/**
*
* Gets a user profile in Amazon DataZone.
*
*
* @param getUserProfileRequest
* @return Result of the GetUserProfile operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetUserProfile
* @see AWS API
* Documentation
*/
GetUserProfileResult getUserProfile(GetUserProfileRequest getUserProfileRequest);
/**
*
* Lists asset filters.
*
*
* @param listAssetFiltersRequest
* @return Result of the ListAssetFilters operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListAssetFilters
* @see AWS API
* Documentation
*/
ListAssetFiltersResult listAssetFilters(ListAssetFiltersRequest listAssetFiltersRequest);
/**
*
* Lists the revisions for the asset.
*
*
* @param listAssetRevisionsRequest
* @return Result of the ListAssetRevisions operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListAssetRevisions
* @see AWS
* API Documentation
*/
ListAssetRevisionsResult listAssetRevisions(ListAssetRevisionsRequest listAssetRevisionsRequest);
/**
*
* Lists data source run activities.
*
*
* @param listDataSourceRunActivitiesRequest
* @return Result of the ListDataSourceRunActivities operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListDataSourceRunActivities
* @see AWS API Documentation
*/
ListDataSourceRunActivitiesResult listDataSourceRunActivities(ListDataSourceRunActivitiesRequest listDataSourceRunActivitiesRequest);
/**
*
* Lists data source runs in Amazon DataZone.
*
*
* @param listDataSourceRunsRequest
* @return Result of the ListDataSourceRuns operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListDataSourceRuns
* @see AWS
* API Documentation
*/
ListDataSourceRunsResult listDataSourceRuns(ListDataSourceRunsRequest listDataSourceRunsRequest);
/**
*
* Lists data sources in Amazon DataZone.
*
*
* @param listDataSourcesRequest
* @return Result of the ListDataSources operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListDataSources
* @see AWS API
* Documentation
*/
ListDataSourcesResult listDataSources(ListDataSourcesRequest listDataSourcesRequest);
/**
*
* Lists Amazon DataZone domains.
*
*
* @param listDomainsRequest
* @return Result of the ListDomains operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListDomains
* @see AWS API
* Documentation
*/
ListDomainsResult listDomains(ListDomainsRequest listDomainsRequest);
/**
*
* Lists existing environment actions.
*
*
* @param listEnvironmentActionsRequest
* @return Result of the ListEnvironmentActions operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListEnvironmentActions
* @see AWS API Documentation
*/
ListEnvironmentActionsResult listEnvironmentActions(ListEnvironmentActionsRequest listEnvironmentActionsRequest);
/**
*
* Lists blueprint configurations for a Amazon DataZone environment.
*
*
* @param listEnvironmentBlueprintConfigurationsRequest
* @return Result of the ListEnvironmentBlueprintConfigurations operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListEnvironmentBlueprintConfigurations
* @see AWS API Documentation
*/
ListEnvironmentBlueprintConfigurationsResult listEnvironmentBlueprintConfigurations(
ListEnvironmentBlueprintConfigurationsRequest listEnvironmentBlueprintConfigurationsRequest);
/**
*
* Lists blueprints in an Amazon DataZone environment.
*
*
* @param listEnvironmentBlueprintsRequest
* @return Result of the ListEnvironmentBlueprints operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListEnvironmentBlueprints
* @see AWS API Documentation
*/
ListEnvironmentBlueprintsResult listEnvironmentBlueprints(ListEnvironmentBlueprintsRequest listEnvironmentBlueprintsRequest);
/**
*
* Lists Amazon DataZone environment profiles.
*
*
* @param listEnvironmentProfilesRequest
* @return Result of the ListEnvironmentProfiles operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListEnvironmentProfiles
* @see AWS API Documentation
*/
ListEnvironmentProfilesResult listEnvironmentProfiles(ListEnvironmentProfilesRequest listEnvironmentProfilesRequest);
/**
*
* Lists Amazon DataZone environments.
*
*
* @param listEnvironmentsRequest
* @return Result of the ListEnvironments operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListEnvironments
* @see AWS API
* Documentation
*/
ListEnvironmentsResult listEnvironments(ListEnvironmentsRequest listEnvironmentsRequest);
/**
*
* Lists the history of the specified data lineage node.
*
*
* @param listLineageNodeHistoryRequest
* @return Result of the ListLineageNodeHistory operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListLineageNodeHistory
* @see AWS API Documentation
*/
ListLineageNodeHistoryResult listLineageNodeHistory(ListLineageNodeHistoryRequest listLineageNodeHistoryRequest);
/**
*
* Lists all metadata generation runs.
*
*
* @param listMetadataGenerationRunsRequest
* @return Result of the ListMetadataGenerationRuns operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListMetadataGenerationRuns
* @see AWS API Documentation
*/
ListMetadataGenerationRunsResult listMetadataGenerationRuns(ListMetadataGenerationRunsRequest listMetadataGenerationRunsRequest);
/**
*
* Lists all Amazon DataZone notifications.
*
*
* @param listNotificationsRequest
* @return Result of the ListNotifications operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListNotifications
* @see AWS API
* Documentation
*/
ListNotificationsResult listNotifications(ListNotificationsRequest listNotificationsRequest);
/**
*
* Lists all members of the specified project.
*
*
* @param listProjectMembershipsRequest
* @return Result of the ListProjectMemberships operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListProjectMemberships
* @see AWS API Documentation
*/
ListProjectMembershipsResult listProjectMemberships(ListProjectMembershipsRequest listProjectMembershipsRequest);
/**
*
* Lists Amazon DataZone projects.
*
*
* @param listProjectsRequest
* @return Result of the ListProjects operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListProjects
* @see AWS API
* Documentation
*/
ListProjectsResult listProjects(ListProjectsRequest listProjectsRequest);
/**
*
* Lists subscription grants.
*
*
* @param listSubscriptionGrantsRequest
* @return Result of the ListSubscriptionGrants operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListSubscriptionGrants
* @see AWS API Documentation
*/
ListSubscriptionGrantsResult listSubscriptionGrants(ListSubscriptionGrantsRequest listSubscriptionGrantsRequest);
/**
*
* Lists Amazon DataZone subscription requests.
*
*
* @param listSubscriptionRequestsRequest
* @return Result of the ListSubscriptionRequests operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListSubscriptionRequests
* @see AWS API Documentation
*/
ListSubscriptionRequestsResult listSubscriptionRequests(ListSubscriptionRequestsRequest listSubscriptionRequestsRequest);
/**
*
* Lists subscription targets in Amazon DataZone.
*
*
* @param listSubscriptionTargetsRequest
* @return Result of the ListSubscriptionTargets operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListSubscriptionTargets
* @see AWS API Documentation
*/
ListSubscriptionTargetsResult listSubscriptionTargets(ListSubscriptionTargetsRequest listSubscriptionTargetsRequest);
/**
*
* Lists subscriptions in Amazon DataZone.
*
*
* @param listSubscriptionsRequest
* @return Result of the ListSubscriptions operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListSubscriptions
* @see AWS API
* Documentation
*/
ListSubscriptionsResult listSubscriptions(ListSubscriptionsRequest listSubscriptionsRequest);
/**
*
* Lists tags for the specified resource in Amazon DataZone.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListTagsForResource
* @see AWS
* API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists time series data points.
*
*
* @param listTimeSeriesDataPointsRequest
* @return Result of the ListTimeSeriesDataPoints operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListTimeSeriesDataPoints
* @see AWS API Documentation
*/
ListTimeSeriesDataPointsResult listTimeSeriesDataPoints(ListTimeSeriesDataPointsRequest listTimeSeriesDataPointsRequest);
/**
*
* Posts a data lineage event.
*
*
* @param postLineageEventRequest
* @return Result of the PostLineageEvent operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.PostLineageEvent
* @see AWS API
* Documentation
*/
PostLineageEventResult postLineageEvent(PostLineageEventRequest postLineageEventRequest);
/**
*
* Posts time series data points to Amazon DataZone for the specified asset.
*
*
* @param postTimeSeriesDataPointsRequest
* @return Result of the PostTimeSeriesDataPoints operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.PostTimeSeriesDataPoints
* @see AWS API Documentation
*/
PostTimeSeriesDataPointsResult postTimeSeriesDataPoints(PostTimeSeriesDataPointsRequest postTimeSeriesDataPointsRequest);
/**
*
* Writes the configuration for the specified environment blueprint in Amazon DataZone.
*
*
* @param putEnvironmentBlueprintConfigurationRequest
* @return Result of the PutEnvironmentBlueprintConfiguration operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.PutEnvironmentBlueprintConfiguration
* @see AWS API Documentation
*/
PutEnvironmentBlueprintConfigurationResult putEnvironmentBlueprintConfiguration(
PutEnvironmentBlueprintConfigurationRequest putEnvironmentBlueprintConfigurationRequest);
/**
*
* Rejects automatically generated business-friendly metadata for your Amazon DataZone assets.
*
*
* @param rejectPredictionsRequest
* @return Result of the RejectPredictions operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.RejectPredictions
* @see AWS API
* Documentation
*/
RejectPredictionsResult rejectPredictions(RejectPredictionsRequest rejectPredictionsRequest);
/**
*
* Rejects the specified subscription request.
*
*
* @param rejectSubscriptionRequestRequest
* @return Result of the RejectSubscriptionRequest operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.RejectSubscriptionRequest
* @see AWS API Documentation
*/
RejectSubscriptionRequestResult rejectSubscriptionRequest(RejectSubscriptionRequestRequest rejectSubscriptionRequestRequest);
/**
*
* Revokes a specified subscription in Amazon DataZone.
*
*
* @param revokeSubscriptionRequest
* @return Result of the RevokeSubscription operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.RevokeSubscription
* @see AWS
* API Documentation
*/
RevokeSubscriptionResult revokeSubscription(RevokeSubscriptionRequest revokeSubscriptionRequest);
/**
*
* Searches for assets in Amazon DataZone.
*
*
* @param searchRequest
* @return Result of the Search operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.Search
* @see AWS API
* Documentation
*/
SearchResult search(SearchRequest searchRequest);
/**
*
* Searches group profiles in Amazon DataZone.
*
*
* @param searchGroupProfilesRequest
* @return Result of the SearchGroupProfiles operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.SearchGroupProfiles
* @see AWS
* API Documentation
*/
SearchGroupProfilesResult searchGroupProfiles(SearchGroupProfilesRequest searchGroupProfilesRequest);
/**
*
* Searches listings (records of an asset at a given time) in Amazon DataZone.
*
*
* @param searchListingsRequest
* @return Result of the SearchListings operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.SearchListings
* @see AWS API
* Documentation
*/
SearchListingsResult searchListings(SearchListingsRequest searchListingsRequest);
/**
*
* Searches for types in Amazon DataZone.
*
*
* @param searchTypesRequest
* @return Result of the SearchTypes operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.SearchTypes
* @see AWS API
* Documentation
*/
SearchTypesResult searchTypes(SearchTypesRequest searchTypesRequest);
/**
*
* Searches user profiles in Amazon DataZone.
*
*
* @param searchUserProfilesRequest
* @return Result of the SearchUserProfiles operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.SearchUserProfiles
* @see AWS
* API Documentation
*/
SearchUserProfilesResult searchUserProfiles(SearchUserProfilesRequest searchUserProfilesRequest);
/**
*
* Start the run of the specified data source in Amazon DataZone.
*
*
* @param startDataSourceRunRequest
* @return Result of the StartDataSourceRun operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.StartDataSourceRun
* @see AWS
* API Documentation
*/
StartDataSourceRunResult startDataSourceRun(StartDataSourceRunRequest startDataSourceRunRequest);
/**
*
* Starts the metadata generation run.
*
*
* @param startMetadataGenerationRunRequest
* @return Result of the StartMetadataGenerationRun operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.StartMetadataGenerationRun
* @see AWS API Documentation
*/
StartMetadataGenerationRunResult startMetadataGenerationRun(StartMetadataGenerationRunRequest startMetadataGenerationRunRequest);
/**
*
* Tags a resource in Amazon DataZone.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Untags a resource in Amazon DataZone.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates an asset filter.
*
*
* @param updateAssetFilterRequest
* @return Result of the UpdateAssetFilter operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.UpdateAssetFilter
* @see AWS API
* Documentation
*/
UpdateAssetFilterResult updateAssetFilter(UpdateAssetFilterRequest updateAssetFilterRequest);
/**
*
* Updates the specified data source in Amazon DataZone.
*
*
* @param updateDataSourceRequest
* @return Result of the UpdateDataSource operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.UpdateDataSource
* @see AWS API
* Documentation
*/
UpdateDataSourceResult updateDataSource(UpdateDataSourceRequest updateDataSourceRequest);
/**
*
* Updates a Amazon DataZone domain.
*
*
* @param updateDomainRequest
* @return Result of the UpdateDomain operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.UpdateDomain
* @see AWS API
* Documentation
*/
UpdateDomainResult updateDomain(UpdateDomainRequest updateDomainRequest);
/**
*
* Updates the specified environment in Amazon DataZone.
*
*
* @param updateEnvironmentRequest
* @return Result of the UpdateEnvironment operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.UpdateEnvironment
* @see AWS API
* Documentation
*/
UpdateEnvironmentResult updateEnvironment(UpdateEnvironmentRequest updateEnvironmentRequest);
/**
*
* Updates an environment action.
*
*
* @param updateEnvironmentActionRequest
* @return Result of the UpdateEnvironmentAction operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.UpdateEnvironmentAction
* @see AWS API Documentation
*/
UpdateEnvironmentActionResult updateEnvironmentAction(UpdateEnvironmentActionRequest updateEnvironmentActionRequest);
/**
*
* Updates the specified environment profile in Amazon DataZone.
*
*
* @param updateEnvironmentProfileRequest
* @return Result of the UpdateEnvironmentProfile operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.UpdateEnvironmentProfile
* @see AWS API Documentation
*/
UpdateEnvironmentProfileResult updateEnvironmentProfile(UpdateEnvironmentProfileRequest updateEnvironmentProfileRequest);
/**
*
* Updates the business glossary in Amazon DataZone.
*
*
* @param updateGlossaryRequest
* @return Result of the UpdateGlossary operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.UpdateGlossary
* @see AWS API
* Documentation
*/
UpdateGlossaryResult updateGlossary(UpdateGlossaryRequest updateGlossaryRequest);
/**
*
* Updates a business glossary term in Amazon DataZone.
*
*
* @param updateGlossaryTermRequest
* @return Result of the UpdateGlossaryTerm operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.UpdateGlossaryTerm
* @see AWS
* API Documentation
*/
UpdateGlossaryTermResult updateGlossaryTerm(UpdateGlossaryTermRequest updateGlossaryTermRequest);
/**
*
* Updates the specified group profile in Amazon DataZone.
*
*
* @param updateGroupProfileRequest
* @return Result of the UpdateGroupProfile operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.UpdateGroupProfile
* @see AWS
* API Documentation
*/
UpdateGroupProfileResult updateGroupProfile(UpdateGroupProfileRequest updateGroupProfileRequest);
/**
*
* Updates the specified project in Amazon DataZone.
*
*
* @param updateProjectRequest
* @return Result of the UpdateProject operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.UpdateProject
* @see AWS API
* Documentation
*/
UpdateProjectResult updateProject(UpdateProjectRequest updateProjectRequest);
/**
*
* Updates the status of the specified subscription grant status in Amazon DataZone.
*
*
* @param updateSubscriptionGrantStatusRequest
* @return Result of the UpdateSubscriptionGrantStatus operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.UpdateSubscriptionGrantStatus
* @see AWS API Documentation
*/
UpdateSubscriptionGrantStatusResult updateSubscriptionGrantStatus(UpdateSubscriptionGrantStatusRequest updateSubscriptionGrantStatusRequest);
/**
*
* Updates a specified subscription request in Amazon DataZone.
*
*
* @param updateSubscriptionRequestRequest
* @return Result of the UpdateSubscriptionRequest operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.UpdateSubscriptionRequest
* @see AWS API Documentation
*/
UpdateSubscriptionRequestResult updateSubscriptionRequest(UpdateSubscriptionRequestRequest updateSubscriptionRequestRequest);
/**
*
* Updates the specified subscription target in Amazon DataZone.
*
*
* @param updateSubscriptionTargetRequest
* @return Result of the UpdateSubscriptionTarget operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.UpdateSubscriptionTarget
* @see AWS API Documentation
*/
UpdateSubscriptionTargetResult updateSubscriptionTarget(UpdateSubscriptionTargetRequest updateSubscriptionTargetRequest);
/**
*
* Updates the specified user profile in Amazon DataZone.
*
*
* @param updateUserProfileRequest
* @return Result of the UpdateUserProfile operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.UpdateUserProfile
* @see AWS API
* Documentation
*/
UpdateUserProfileResult updateUserProfile(UpdateUserProfileRequest updateUserProfileRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}