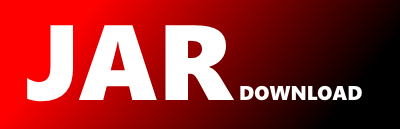
com.amazonaws.services.datazone.AmazonDataZoneAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-datazoneexternal Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.datazone;
import javax.annotation.Generated;
import com.amazonaws.services.datazone.model.*;
/**
* Interface for accessing Amazon DataZone asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.datazone.AbstractAmazonDataZoneAsync} instead.
*
*
*
* Amazon DataZone is a data management service that enables you to catalog, discover, govern, share, and analyze your
* data. With Amazon DataZone, you can share and access your data across accounts and supported regions. Amazon DataZone
* simplifies your experience across Amazon Web Services services, including, but not limited to, Amazon Redshift,
* Amazon Athena, Amazon Web Services Glue, and Amazon Web Services Lake Formation.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonDataZoneAsync extends AmazonDataZone {
/**
*
* Accepts automatically generated business-friendly metadata for your Amazon DataZone assets.
*
*
* @param acceptPredictionsRequest
* @return A Java Future containing the result of the AcceptPredictions operation returned by the service.
* @sample AmazonDataZoneAsync.AcceptPredictions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future acceptPredictionsAsync(AcceptPredictionsRequest acceptPredictionsRequest);
/**
*
* Accepts automatically generated business-friendly metadata for your Amazon DataZone assets.
*
*
* @param acceptPredictionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AcceptPredictions operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.AcceptPredictions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future acceptPredictionsAsync(AcceptPredictionsRequest acceptPredictionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Accepts a subscription request to a specific asset.
*
*
* @param acceptSubscriptionRequestRequest
* @return A Java Future containing the result of the AcceptSubscriptionRequest operation returned by the service.
* @sample AmazonDataZoneAsync.AcceptSubscriptionRequest
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptSubscriptionRequestAsync(
AcceptSubscriptionRequestRequest acceptSubscriptionRequestRequest);
/**
*
* Accepts a subscription request to a specific asset.
*
*
* @param acceptSubscriptionRequestRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AcceptSubscriptionRequest operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.AcceptSubscriptionRequest
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptSubscriptionRequestAsync(
AcceptSubscriptionRequestRequest acceptSubscriptionRequestRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates the environment role in Amazon DataZone.
*
*
* @param associateEnvironmentRoleRequest
* @return A Java Future containing the result of the AssociateEnvironmentRole operation returned by the service.
* @sample AmazonDataZoneAsync.AssociateEnvironmentRole
* @see AWS API Documentation
*/
java.util.concurrent.Future associateEnvironmentRoleAsync(AssociateEnvironmentRoleRequest associateEnvironmentRoleRequest);
/**
*
* Associates the environment role in Amazon DataZone.
*
*
* @param associateEnvironmentRoleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateEnvironmentRole operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.AssociateEnvironmentRole
* @see AWS API Documentation
*/
java.util.concurrent.Future associateEnvironmentRoleAsync(AssociateEnvironmentRoleRequest associateEnvironmentRoleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels the metadata generation run.
*
*
* @param cancelMetadataGenerationRunRequest
* @return A Java Future containing the result of the CancelMetadataGenerationRun operation returned by the service.
* @sample AmazonDataZoneAsync.CancelMetadataGenerationRun
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelMetadataGenerationRunAsync(
CancelMetadataGenerationRunRequest cancelMetadataGenerationRunRequest);
/**
*
* Cancels the metadata generation run.
*
*
* @param cancelMetadataGenerationRunRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelMetadataGenerationRun operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.CancelMetadataGenerationRun
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelMetadataGenerationRunAsync(
CancelMetadataGenerationRunRequest cancelMetadataGenerationRunRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels the subscription to the specified asset.
*
*
* @param cancelSubscriptionRequest
* @return A Java Future containing the result of the CancelSubscription operation returned by the service.
* @sample AmazonDataZoneAsync.CancelSubscription
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelSubscriptionAsync(CancelSubscriptionRequest cancelSubscriptionRequest);
/**
*
* Cancels the subscription to the specified asset.
*
*
* @param cancelSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelSubscription operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.CancelSubscription
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelSubscriptionAsync(CancelSubscriptionRequest cancelSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an asset in Amazon DataZone catalog.
*
*
* @param createAssetRequest
* @return A Java Future containing the result of the CreateAsset operation returned by the service.
* @sample AmazonDataZoneAsync.CreateAsset
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAssetAsync(CreateAssetRequest createAssetRequest);
/**
*
* Creates an asset in Amazon DataZone catalog.
*
*
* @param createAssetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAsset operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.CreateAsset
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAssetAsync(CreateAssetRequest createAssetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a data asset filter.
*
*
* @param createAssetFilterRequest
* @return A Java Future containing the result of the CreateAssetFilter operation returned by the service.
* @sample AmazonDataZoneAsync.CreateAssetFilter
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAssetFilterAsync(CreateAssetFilterRequest createAssetFilterRequest);
/**
*
* Creates a data asset filter.
*
*
* @param createAssetFilterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAssetFilter operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.CreateAssetFilter
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAssetFilterAsync(CreateAssetFilterRequest createAssetFilterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a revision of the asset.
*
*
* @param createAssetRevisionRequest
* @return A Java Future containing the result of the CreateAssetRevision operation returned by the service.
* @sample AmazonDataZoneAsync.CreateAssetRevision
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createAssetRevisionAsync(CreateAssetRevisionRequest createAssetRevisionRequest);
/**
*
* Creates a revision of the asset.
*
*
* @param createAssetRevisionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAssetRevision operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.CreateAssetRevision
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createAssetRevisionAsync(CreateAssetRevisionRequest createAssetRevisionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a custom asset type.
*
*
* @param createAssetTypeRequest
* @return A Java Future containing the result of the CreateAssetType operation returned by the service.
* @sample AmazonDataZoneAsync.CreateAssetType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAssetTypeAsync(CreateAssetTypeRequest createAssetTypeRequest);
/**
*
* Creates a custom asset type.
*
*
* @param createAssetTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAssetType operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.CreateAssetType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAssetTypeAsync(CreateAssetTypeRequest createAssetTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon DataZone data source.
*
*
* @param createDataSourceRequest
* @return A Java Future containing the result of the CreateDataSource operation returned by the service.
* @sample AmazonDataZoneAsync.CreateDataSource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDataSourceAsync(CreateDataSourceRequest createDataSourceRequest);
/**
*
* Creates an Amazon DataZone data source.
*
*
* @param createDataSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDataSource operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.CreateDataSource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDataSourceAsync(CreateDataSourceRequest createDataSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon DataZone domain.
*
*
* @param createDomainRequest
* @return A Java Future containing the result of the CreateDomain operation returned by the service.
* @sample AmazonDataZoneAsync.CreateDomain
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDomainAsync(CreateDomainRequest createDomainRequest);
/**
*
* Creates an Amazon DataZone domain.
*
*
* @param createDomainRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDomain operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.CreateDomain
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDomainAsync(CreateDomainRequest createDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create an Amazon DataZone environment.
*
*
* @param createEnvironmentRequest
* @return A Java Future containing the result of the CreateEnvironment operation returned by the service.
* @sample AmazonDataZoneAsync.CreateEnvironment
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createEnvironmentAsync(CreateEnvironmentRequest createEnvironmentRequest);
/**
*
* Create an Amazon DataZone environment.
*
*
* @param createEnvironmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEnvironment operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.CreateEnvironment
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createEnvironmentAsync(CreateEnvironmentRequest createEnvironmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an action for the environment, for example, creates a console link for an analytics tool that is
* available in this environment.
*
*
* @param createEnvironmentActionRequest
* @return A Java Future containing the result of the CreateEnvironmentAction operation returned by the service.
* @sample AmazonDataZoneAsync.CreateEnvironmentAction
* @see AWS API Documentation
*/
java.util.concurrent.Future createEnvironmentActionAsync(CreateEnvironmentActionRequest createEnvironmentActionRequest);
/**
*
* Creates an action for the environment, for example, creates a console link for an analytics tool that is
* available in this environment.
*
*
* @param createEnvironmentActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEnvironmentAction operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.CreateEnvironmentAction
* @see AWS API Documentation
*/
java.util.concurrent.Future createEnvironmentActionAsync(CreateEnvironmentActionRequest createEnvironmentActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon DataZone environment profile.
*
*
* @param createEnvironmentProfileRequest
* @return A Java Future containing the result of the CreateEnvironmentProfile operation returned by the service.
* @sample AmazonDataZoneAsync.CreateEnvironmentProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future createEnvironmentProfileAsync(CreateEnvironmentProfileRequest createEnvironmentProfileRequest);
/**
*
* Creates an Amazon DataZone environment profile.
*
*
* @param createEnvironmentProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEnvironmentProfile operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.CreateEnvironmentProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future createEnvironmentProfileAsync(CreateEnvironmentProfileRequest createEnvironmentProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a metadata form type.
*
*
* @param createFormTypeRequest
* @return A Java Future containing the result of the CreateFormType operation returned by the service.
* @sample AmazonDataZoneAsync.CreateFormType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFormTypeAsync(CreateFormTypeRequest createFormTypeRequest);
/**
*
* Creates a metadata form type.
*
*
* @param createFormTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFormType operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.CreateFormType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFormTypeAsync(CreateFormTypeRequest createFormTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon DataZone business glossary.
*
*
* @param createGlossaryRequest
* @return A Java Future containing the result of the CreateGlossary operation returned by the service.
* @sample AmazonDataZoneAsync.CreateGlossary
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createGlossaryAsync(CreateGlossaryRequest createGlossaryRequest);
/**
*
* Creates an Amazon DataZone business glossary.
*
*
* @param createGlossaryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateGlossary operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.CreateGlossary
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createGlossaryAsync(CreateGlossaryRequest createGlossaryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a business glossary term.
*
*
* @param createGlossaryTermRequest
* @return A Java Future containing the result of the CreateGlossaryTerm operation returned by the service.
* @sample AmazonDataZoneAsync.CreateGlossaryTerm
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createGlossaryTermAsync(CreateGlossaryTermRequest createGlossaryTermRequest);
/**
*
* Creates a business glossary term.
*
*
* @param createGlossaryTermRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateGlossaryTerm operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.CreateGlossaryTerm
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createGlossaryTermAsync(CreateGlossaryTermRequest createGlossaryTermRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a group profile in Amazon DataZone.
*
*
* @param createGroupProfileRequest
* @return A Java Future containing the result of the CreateGroupProfile operation returned by the service.
* @sample AmazonDataZoneAsync.CreateGroupProfile
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createGroupProfileAsync(CreateGroupProfileRequest createGroupProfileRequest);
/**
*
* Creates a group profile in Amazon DataZone.
*
*
* @param createGroupProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateGroupProfile operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.CreateGroupProfile
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createGroupProfileAsync(CreateGroupProfileRequest createGroupProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Publishes a listing (a record of an asset at a given time) or removes a listing from the catalog.
*
*
* @param createListingChangeSetRequest
* @return A Java Future containing the result of the CreateListingChangeSet operation returned by the service.
* @sample AmazonDataZoneAsync.CreateListingChangeSet
* @see AWS API Documentation
*/
java.util.concurrent.Future createListingChangeSetAsync(CreateListingChangeSetRequest createListingChangeSetRequest);
/**
*
* Publishes a listing (a record of an asset at a given time) or removes a listing from the catalog.
*
*
* @param createListingChangeSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateListingChangeSet operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.CreateListingChangeSet
* @see AWS API Documentation
*/
java.util.concurrent.Future createListingChangeSetAsync(CreateListingChangeSetRequest createListingChangeSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon DataZone project.
*
*
* @param createProjectRequest
* @return A Java Future containing the result of the CreateProject operation returned by the service.
* @sample AmazonDataZoneAsync.CreateProject
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createProjectAsync(CreateProjectRequest createProjectRequest);
/**
*
* Creates an Amazon DataZone project.
*
*
* @param createProjectRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateProject operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.CreateProject
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createProjectAsync(CreateProjectRequest createProjectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a project membership in Amazon DataZone.
*
*
* @param createProjectMembershipRequest
* @return A Java Future containing the result of the CreateProjectMembership operation returned by the service.
* @sample AmazonDataZoneAsync.CreateProjectMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future createProjectMembershipAsync(CreateProjectMembershipRequest createProjectMembershipRequest);
/**
*
* Creates a project membership in Amazon DataZone.
*
*
* @param createProjectMembershipRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateProjectMembership operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.CreateProjectMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future createProjectMembershipAsync(CreateProjectMembershipRequest createProjectMembershipRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a subsscription grant in Amazon DataZone.
*
*
* @param createSubscriptionGrantRequest
* @return A Java Future containing the result of the CreateSubscriptionGrant operation returned by the service.
* @sample AmazonDataZoneAsync.CreateSubscriptionGrant
* @see AWS API Documentation
*/
java.util.concurrent.Future createSubscriptionGrantAsync(CreateSubscriptionGrantRequest createSubscriptionGrantRequest);
/**
*
* Creates a subsscription grant in Amazon DataZone.
*
*
* @param createSubscriptionGrantRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSubscriptionGrant operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.CreateSubscriptionGrant
* @see AWS API Documentation
*/
java.util.concurrent.Future createSubscriptionGrantAsync(CreateSubscriptionGrantRequest createSubscriptionGrantRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a subscription request in Amazon DataZone.
*
*
* @param createSubscriptionRequestRequest
* @return A Java Future containing the result of the CreateSubscriptionRequest operation returned by the service.
* @sample AmazonDataZoneAsync.CreateSubscriptionRequest
* @see AWS API Documentation
*/
java.util.concurrent.Future createSubscriptionRequestAsync(
CreateSubscriptionRequestRequest createSubscriptionRequestRequest);
/**
*
* Creates a subscription request in Amazon DataZone.
*
*
* @param createSubscriptionRequestRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSubscriptionRequest operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.CreateSubscriptionRequest
* @see AWS API Documentation
*/
java.util.concurrent.Future createSubscriptionRequestAsync(
CreateSubscriptionRequestRequest createSubscriptionRequestRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a subscription target in Amazon DataZone.
*
*
* @param createSubscriptionTargetRequest
* @return A Java Future containing the result of the CreateSubscriptionTarget operation returned by the service.
* @sample AmazonDataZoneAsync.CreateSubscriptionTarget
* @see AWS API Documentation
*/
java.util.concurrent.Future createSubscriptionTargetAsync(CreateSubscriptionTargetRequest createSubscriptionTargetRequest);
/**
*
* Creates a subscription target in Amazon DataZone.
*
*
* @param createSubscriptionTargetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSubscriptionTarget operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.CreateSubscriptionTarget
* @see AWS API Documentation
*/
java.util.concurrent.Future createSubscriptionTargetAsync(CreateSubscriptionTargetRequest createSubscriptionTargetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a user profile in Amazon DataZone.
*
*
* @param createUserProfileRequest
* @return A Java Future containing the result of the CreateUserProfile operation returned by the service.
* @sample AmazonDataZoneAsync.CreateUserProfile
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createUserProfileAsync(CreateUserProfileRequest createUserProfileRequest);
/**
*
* Creates a user profile in Amazon DataZone.
*
*
* @param createUserProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateUserProfile operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.CreateUserProfile
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createUserProfileAsync(CreateUserProfileRequest createUserProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delets an asset in Amazon DataZone.
*
*
* @param deleteAssetRequest
* @return A Java Future containing the result of the DeleteAsset operation returned by the service.
* @sample AmazonDataZoneAsync.DeleteAsset
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAssetAsync(DeleteAssetRequest deleteAssetRequest);
/**
*
* Delets an asset in Amazon DataZone.
*
*
* @param deleteAssetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAsset operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.DeleteAsset
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAssetAsync(DeleteAssetRequest deleteAssetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an asset filter.
*
*
* @param deleteAssetFilterRequest
* @return A Java Future containing the result of the DeleteAssetFilter operation returned by the service.
* @sample AmazonDataZoneAsync.DeleteAssetFilter
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAssetFilterAsync(DeleteAssetFilterRequest deleteAssetFilterRequest);
/**
*
* Deletes an asset filter.
*
*
* @param deleteAssetFilterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAssetFilter operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.DeleteAssetFilter
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAssetFilterAsync(DeleteAssetFilterRequest deleteAssetFilterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an asset type in Amazon DataZone.
*
*
* @param deleteAssetTypeRequest
* @return A Java Future containing the result of the DeleteAssetType operation returned by the service.
* @sample AmazonDataZoneAsync.DeleteAssetType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAssetTypeAsync(DeleteAssetTypeRequest deleteAssetTypeRequest);
/**
*
* Deletes an asset type in Amazon DataZone.
*
*
* @param deleteAssetTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAssetType operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.DeleteAssetType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAssetTypeAsync(DeleteAssetTypeRequest deleteAssetTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a data source in Amazon DataZone.
*
*
* @param deleteDataSourceRequest
* @return A Java Future containing the result of the DeleteDataSource operation returned by the service.
* @sample AmazonDataZoneAsync.DeleteDataSource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDataSourceAsync(DeleteDataSourceRequest deleteDataSourceRequest);
/**
*
* Deletes a data source in Amazon DataZone.
*
*
* @param deleteDataSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDataSource operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.DeleteDataSource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDataSourceAsync(DeleteDataSourceRequest deleteDataSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a Amazon DataZone domain.
*
*
* @param deleteDomainRequest
* @return A Java Future containing the result of the DeleteDomain operation returned by the service.
* @sample AmazonDataZoneAsync.DeleteDomain
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDomainAsync(DeleteDomainRequest deleteDomainRequest);
/**
*
* Deletes a Amazon DataZone domain.
*
*
* @param deleteDomainRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDomain operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.DeleteDomain
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDomainAsync(DeleteDomainRequest deleteDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an environment in Amazon DataZone.
*
*
* @param deleteEnvironmentRequest
* @return A Java Future containing the result of the DeleteEnvironment operation returned by the service.
* @sample AmazonDataZoneAsync.DeleteEnvironment
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteEnvironmentAsync(DeleteEnvironmentRequest deleteEnvironmentRequest);
/**
*
* Deletes an environment in Amazon DataZone.
*
*
* @param deleteEnvironmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEnvironment operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.DeleteEnvironment
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteEnvironmentAsync(DeleteEnvironmentRequest deleteEnvironmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an action for the environment, for example, deletes a console link for an analytics tool that is
* available in this environment.
*
*
* @param deleteEnvironmentActionRequest
* @return A Java Future containing the result of the DeleteEnvironmentAction operation returned by the service.
* @sample AmazonDataZoneAsync.DeleteEnvironmentAction
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEnvironmentActionAsync(DeleteEnvironmentActionRequest deleteEnvironmentActionRequest);
/**
*
* Deletes an action for the environment, for example, deletes a console link for an analytics tool that is
* available in this environment.
*
*
* @param deleteEnvironmentActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEnvironmentAction operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.DeleteEnvironmentAction
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEnvironmentActionAsync(DeleteEnvironmentActionRequest deleteEnvironmentActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the blueprint configuration in Amazon DataZone.
*
*
* @param deleteEnvironmentBlueprintConfigurationRequest
* @return A Java Future containing the result of the DeleteEnvironmentBlueprintConfiguration operation returned by
* the service.
* @sample AmazonDataZoneAsync.DeleteEnvironmentBlueprintConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEnvironmentBlueprintConfigurationAsync(
DeleteEnvironmentBlueprintConfigurationRequest deleteEnvironmentBlueprintConfigurationRequest);
/**
*
* Deletes the blueprint configuration in Amazon DataZone.
*
*
* @param deleteEnvironmentBlueprintConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEnvironmentBlueprintConfiguration operation returned by
* the service.
* @sample AmazonDataZoneAsyncHandler.DeleteEnvironmentBlueprintConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEnvironmentBlueprintConfigurationAsync(
DeleteEnvironmentBlueprintConfigurationRequest deleteEnvironmentBlueprintConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an environment profile in Amazon DataZone.
*
*
* @param deleteEnvironmentProfileRequest
* @return A Java Future containing the result of the DeleteEnvironmentProfile operation returned by the service.
* @sample AmazonDataZoneAsync.DeleteEnvironmentProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEnvironmentProfileAsync(DeleteEnvironmentProfileRequest deleteEnvironmentProfileRequest);
/**
*
* Deletes an environment profile in Amazon DataZone.
*
*
* @param deleteEnvironmentProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEnvironmentProfile operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.DeleteEnvironmentProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEnvironmentProfileAsync(DeleteEnvironmentProfileRequest deleteEnvironmentProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delets and metadata form type in Amazon DataZone.
*
*
* @param deleteFormTypeRequest
* @return A Java Future containing the result of the DeleteFormType operation returned by the service.
* @sample AmazonDataZoneAsync.DeleteFormType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFormTypeAsync(DeleteFormTypeRequest deleteFormTypeRequest);
/**
*
* Delets and metadata form type in Amazon DataZone.
*
*
* @param deleteFormTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFormType operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.DeleteFormType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFormTypeAsync(DeleteFormTypeRequest deleteFormTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a business glossary in Amazon DataZone.
*
*
* @param deleteGlossaryRequest
* @return A Java Future containing the result of the DeleteGlossary operation returned by the service.
* @sample AmazonDataZoneAsync.DeleteGlossary
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteGlossaryAsync(DeleteGlossaryRequest deleteGlossaryRequest);
/**
*
* Deletes a business glossary in Amazon DataZone.
*
*
* @param deleteGlossaryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteGlossary operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.DeleteGlossary
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteGlossaryAsync(DeleteGlossaryRequest deleteGlossaryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a business glossary term in Amazon DataZone.
*
*
* @param deleteGlossaryTermRequest
* @return A Java Future containing the result of the DeleteGlossaryTerm operation returned by the service.
* @sample AmazonDataZoneAsync.DeleteGlossaryTerm
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteGlossaryTermAsync(DeleteGlossaryTermRequest deleteGlossaryTermRequest);
/**
*
* Deletes a business glossary term in Amazon DataZone.
*
*
* @param deleteGlossaryTermRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteGlossaryTerm operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.DeleteGlossaryTerm
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteGlossaryTermAsync(DeleteGlossaryTermRequest deleteGlossaryTermRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a listing (a record of an asset at a given time).
*
*
* @param deleteListingRequest
* @return A Java Future containing the result of the DeleteListing operation returned by the service.
* @sample AmazonDataZoneAsync.DeleteListing
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteListingAsync(DeleteListingRequest deleteListingRequest);
/**
*
* Deletes a listing (a record of an asset at a given time).
*
*
* @param deleteListingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteListing operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.DeleteListing
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteListingAsync(DeleteListingRequest deleteListingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a project in Amazon DataZone.
*
*
* @param deleteProjectRequest
* @return A Java Future containing the result of the DeleteProject operation returned by the service.
* @sample AmazonDataZoneAsync.DeleteProject
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteProjectAsync(DeleteProjectRequest deleteProjectRequest);
/**
*
* Deletes a project in Amazon DataZone.
*
*
* @param deleteProjectRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteProject operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.DeleteProject
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteProjectAsync(DeleteProjectRequest deleteProjectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes project membership in Amazon DataZone.
*
*
* @param deleteProjectMembershipRequest
* @return A Java Future containing the result of the DeleteProjectMembership operation returned by the service.
* @sample AmazonDataZoneAsync.DeleteProjectMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteProjectMembershipAsync(DeleteProjectMembershipRequest deleteProjectMembershipRequest);
/**
*
* Deletes project membership in Amazon DataZone.
*
*
* @param deleteProjectMembershipRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteProjectMembership operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.DeleteProjectMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteProjectMembershipAsync(DeleteProjectMembershipRequest deleteProjectMembershipRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes and subscription grant in Amazon DataZone.
*
*
* @param deleteSubscriptionGrantRequest
* @return A Java Future containing the result of the DeleteSubscriptionGrant operation returned by the service.
* @sample AmazonDataZoneAsync.DeleteSubscriptionGrant
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSubscriptionGrantAsync(DeleteSubscriptionGrantRequest deleteSubscriptionGrantRequest);
/**
*
* Deletes and subscription grant in Amazon DataZone.
*
*
* @param deleteSubscriptionGrantRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSubscriptionGrant operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.DeleteSubscriptionGrant
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSubscriptionGrantAsync(DeleteSubscriptionGrantRequest deleteSubscriptionGrantRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a subscription request in Amazon DataZone.
*
*
* @param deleteSubscriptionRequestRequest
* @return A Java Future containing the result of the DeleteSubscriptionRequest operation returned by the service.
* @sample AmazonDataZoneAsync.DeleteSubscriptionRequest
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSubscriptionRequestAsync(
DeleteSubscriptionRequestRequest deleteSubscriptionRequestRequest);
/**
*
* Deletes a subscription request in Amazon DataZone.
*
*
* @param deleteSubscriptionRequestRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSubscriptionRequest operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.DeleteSubscriptionRequest
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSubscriptionRequestAsync(
DeleteSubscriptionRequestRequest deleteSubscriptionRequestRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a subscription target in Amazon DataZone.
*
*
* @param deleteSubscriptionTargetRequest
* @return A Java Future containing the result of the DeleteSubscriptionTarget operation returned by the service.
* @sample AmazonDataZoneAsync.DeleteSubscriptionTarget
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSubscriptionTargetAsync(DeleteSubscriptionTargetRequest deleteSubscriptionTargetRequest);
/**
*
* Deletes a subscription target in Amazon DataZone.
*
*
* @param deleteSubscriptionTargetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSubscriptionTarget operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.DeleteSubscriptionTarget
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSubscriptionTargetAsync(DeleteSubscriptionTargetRequest deleteSubscriptionTargetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified time series form for the specified asset.
*
*
* @param deleteTimeSeriesDataPointsRequest
* @return A Java Future containing the result of the DeleteTimeSeriesDataPoints operation returned by the service.
* @sample AmazonDataZoneAsync.DeleteTimeSeriesDataPoints
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteTimeSeriesDataPointsAsync(
DeleteTimeSeriesDataPointsRequest deleteTimeSeriesDataPointsRequest);
/**
*
* Deletes the specified time series form for the specified asset.
*
*
* @param deleteTimeSeriesDataPointsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTimeSeriesDataPoints operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.DeleteTimeSeriesDataPoints
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteTimeSeriesDataPointsAsync(
DeleteTimeSeriesDataPointsRequest deleteTimeSeriesDataPointsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates the environment role in Amazon DataZone.
*
*
* @param disassociateEnvironmentRoleRequest
* @return A Java Future containing the result of the DisassociateEnvironmentRole operation returned by the service.
* @sample AmazonDataZoneAsync.DisassociateEnvironmentRole
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateEnvironmentRoleAsync(
DisassociateEnvironmentRoleRequest disassociateEnvironmentRoleRequest);
/**
*
* Disassociates the environment role in Amazon DataZone.
*
*
* @param disassociateEnvironmentRoleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateEnvironmentRole operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.DisassociateEnvironmentRole
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateEnvironmentRoleAsync(
DisassociateEnvironmentRoleRequest disassociateEnvironmentRoleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets an Amazon DataZone asset.
*
*
* @param getAssetRequest
* @return A Java Future containing the result of the GetAsset operation returned by the service.
* @sample AmazonDataZoneAsync.GetAsset
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAssetAsync(GetAssetRequest getAssetRequest);
/**
*
* Gets an Amazon DataZone asset.
*
*
* @param getAssetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAsset operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetAsset
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAssetAsync(GetAssetRequest getAssetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets an asset filter.
*
*
* @param getAssetFilterRequest
* @return A Java Future containing the result of the GetAssetFilter operation returned by the service.
* @sample AmazonDataZoneAsync.GetAssetFilter
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAssetFilterAsync(GetAssetFilterRequest getAssetFilterRequest);
/**
*
* Gets an asset filter.
*
*
* @param getAssetFilterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAssetFilter operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetAssetFilter
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAssetFilterAsync(GetAssetFilterRequest getAssetFilterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets an Amazon DataZone asset type.
*
*
* @param getAssetTypeRequest
* @return A Java Future containing the result of the GetAssetType operation returned by the service.
* @sample AmazonDataZoneAsync.GetAssetType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAssetTypeAsync(GetAssetTypeRequest getAssetTypeRequest);
/**
*
* Gets an Amazon DataZone asset type.
*
*
* @param getAssetTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAssetType operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetAssetType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAssetTypeAsync(GetAssetTypeRequest getAssetTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets an Amazon DataZone data source.
*
*
* @param getDataSourceRequest
* @return A Java Future containing the result of the GetDataSource operation returned by the service.
* @sample AmazonDataZoneAsync.GetDataSource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDataSourceAsync(GetDataSourceRequest getDataSourceRequest);
/**
*
* Gets an Amazon DataZone data source.
*
*
* @param getDataSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDataSource operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetDataSource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDataSourceAsync(GetDataSourceRequest getDataSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets an Amazon DataZone data source run.
*
*
* @param getDataSourceRunRequest
* @return A Java Future containing the result of the GetDataSourceRun operation returned by the service.
* @sample AmazonDataZoneAsync.GetDataSourceRun
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDataSourceRunAsync(GetDataSourceRunRequest getDataSourceRunRequest);
/**
*
* Gets an Amazon DataZone data source run.
*
*
* @param getDataSourceRunRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDataSourceRun operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetDataSourceRun
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDataSourceRunAsync(GetDataSourceRunRequest getDataSourceRunRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets an Amazon DataZone domain.
*
*
* @param getDomainRequest
* @return A Java Future containing the result of the GetDomain operation returned by the service.
* @sample AmazonDataZoneAsync.GetDomain
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDomainAsync(GetDomainRequest getDomainRequest);
/**
*
* Gets an Amazon DataZone domain.
*
*
* @param getDomainRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDomain operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetDomain
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDomainAsync(GetDomainRequest getDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets an Amazon DataZone environment.
*
*
* @param getEnvironmentRequest
* @return A Java Future containing the result of the GetEnvironment operation returned by the service.
* @sample AmazonDataZoneAsync.GetEnvironment
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getEnvironmentAsync(GetEnvironmentRequest getEnvironmentRequest);
/**
*
* Gets an Amazon DataZone environment.
*
*
* @param getEnvironmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEnvironment operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetEnvironment
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getEnvironmentAsync(GetEnvironmentRequest getEnvironmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the specified environment action.
*
*
* @param getEnvironmentActionRequest
* @return A Java Future containing the result of the GetEnvironmentAction operation returned by the service.
* @sample AmazonDataZoneAsync.GetEnvironmentAction
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getEnvironmentActionAsync(GetEnvironmentActionRequest getEnvironmentActionRequest);
/**
*
* Gets the specified environment action.
*
*
* @param getEnvironmentActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEnvironmentAction operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetEnvironmentAction
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getEnvironmentActionAsync(GetEnvironmentActionRequest getEnvironmentActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets an Amazon DataZone blueprint.
*
*
* @param getEnvironmentBlueprintRequest
* @return A Java Future containing the result of the GetEnvironmentBlueprint operation returned by the service.
* @sample AmazonDataZoneAsync.GetEnvironmentBlueprint
* @see AWS API Documentation
*/
java.util.concurrent.Future getEnvironmentBlueprintAsync(GetEnvironmentBlueprintRequest getEnvironmentBlueprintRequest);
/**
*
* Gets an Amazon DataZone blueprint.
*
*
* @param getEnvironmentBlueprintRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEnvironmentBlueprint operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetEnvironmentBlueprint
* @see AWS API Documentation
*/
java.util.concurrent.Future getEnvironmentBlueprintAsync(GetEnvironmentBlueprintRequest getEnvironmentBlueprintRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the blueprint configuration in Amazon DataZone.
*
*
* @param getEnvironmentBlueprintConfigurationRequest
* @return A Java Future containing the result of the GetEnvironmentBlueprintConfiguration operation returned by the
* service.
* @sample AmazonDataZoneAsync.GetEnvironmentBlueprintConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getEnvironmentBlueprintConfigurationAsync(
GetEnvironmentBlueprintConfigurationRequest getEnvironmentBlueprintConfigurationRequest);
/**
*
* Gets the blueprint configuration in Amazon DataZone.
*
*
* @param getEnvironmentBlueprintConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEnvironmentBlueprintConfiguration operation returned by the
* service.
* @sample AmazonDataZoneAsyncHandler.GetEnvironmentBlueprintConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getEnvironmentBlueprintConfigurationAsync(
GetEnvironmentBlueprintConfigurationRequest getEnvironmentBlueprintConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the credentials of an environment in Amazon DataZone.
*
*
* @param getEnvironmentCredentialsRequest
* @return A Java Future containing the result of the GetEnvironmentCredentials operation returned by the service.
* @sample AmazonDataZoneAsync.GetEnvironmentCredentials
* @see AWS API Documentation
*/
java.util.concurrent.Future getEnvironmentCredentialsAsync(
GetEnvironmentCredentialsRequest getEnvironmentCredentialsRequest);
/**
*
* Gets the credentials of an environment in Amazon DataZone.
*
*
* @param getEnvironmentCredentialsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEnvironmentCredentials operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetEnvironmentCredentials
* @see AWS API Documentation
*/
java.util.concurrent.Future getEnvironmentCredentialsAsync(
GetEnvironmentCredentialsRequest getEnvironmentCredentialsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets an evinronment profile in Amazon DataZone.
*
*
* @param getEnvironmentProfileRequest
* @return A Java Future containing the result of the GetEnvironmentProfile operation returned by the service.
* @sample AmazonDataZoneAsync.GetEnvironmentProfile
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getEnvironmentProfileAsync(GetEnvironmentProfileRequest getEnvironmentProfileRequest);
/**
*
* Gets an evinronment profile in Amazon DataZone.
*
*
* @param getEnvironmentProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEnvironmentProfile operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetEnvironmentProfile
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getEnvironmentProfileAsync(GetEnvironmentProfileRequest getEnvironmentProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a metadata form type in Amazon DataZone.
*
*
* @param getFormTypeRequest
* @return A Java Future containing the result of the GetFormType operation returned by the service.
* @sample AmazonDataZoneAsync.GetFormType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getFormTypeAsync(GetFormTypeRequest getFormTypeRequest);
/**
*
* Gets a metadata form type in Amazon DataZone.
*
*
* @param getFormTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetFormType operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetFormType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getFormTypeAsync(GetFormTypeRequest getFormTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a business glossary in Amazon DataZone.
*
*
* @param getGlossaryRequest
* @return A Java Future containing the result of the GetGlossary operation returned by the service.
* @sample AmazonDataZoneAsync.GetGlossary
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getGlossaryAsync(GetGlossaryRequest getGlossaryRequest);
/**
*
* Gets a business glossary in Amazon DataZone.
*
*
* @param getGlossaryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetGlossary operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetGlossary
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getGlossaryAsync(GetGlossaryRequest getGlossaryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a business glossary term in Amazon DataZone.
*
*
* @param getGlossaryTermRequest
* @return A Java Future containing the result of the GetGlossaryTerm operation returned by the service.
* @sample AmazonDataZoneAsync.GetGlossaryTerm
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getGlossaryTermAsync(GetGlossaryTermRequest getGlossaryTermRequest);
/**
*
* Gets a business glossary term in Amazon DataZone.
*
*
* @param getGlossaryTermRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetGlossaryTerm operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetGlossaryTerm
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getGlossaryTermAsync(GetGlossaryTermRequest getGlossaryTermRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a group profile in Amazon DataZone.
*
*
* @param getGroupProfileRequest
* @return A Java Future containing the result of the GetGroupProfile operation returned by the service.
* @sample AmazonDataZoneAsync.GetGroupProfile
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getGroupProfileAsync(GetGroupProfileRequest getGroupProfileRequest);
/**
*
* Gets a group profile in Amazon DataZone.
*
*
* @param getGroupProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetGroupProfile operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetGroupProfile
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getGroupProfileAsync(GetGroupProfileRequest getGroupProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the data portal URL for the specified Amazon DataZone domain.
*
*
* @param getIamPortalLoginUrlRequest
* @return A Java Future containing the result of the GetIamPortalLoginUrl operation returned by the service.
* @sample AmazonDataZoneAsync.GetIamPortalLoginUrl
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getIamPortalLoginUrlAsync(GetIamPortalLoginUrlRequest getIamPortalLoginUrlRequest);
/**
*
* Gets the data portal URL for the specified Amazon DataZone domain.
*
*
* @param getIamPortalLoginUrlRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetIamPortalLoginUrl operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetIamPortalLoginUrl
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getIamPortalLoginUrlAsync(GetIamPortalLoginUrlRequest getIamPortalLoginUrlRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the data lineage node.
*
*
* @param getLineageNodeRequest
* @return A Java Future containing the result of the GetLineageNode operation returned by the service.
* @sample AmazonDataZoneAsync.GetLineageNode
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getLineageNodeAsync(GetLineageNodeRequest getLineageNodeRequest);
/**
*
* Gets the data lineage node.
*
*
* @param getLineageNodeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetLineageNode operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetLineageNode
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getLineageNodeAsync(GetLineageNodeRequest getLineageNodeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a listing (a record of an asset at a given time).
*
*
* @param getListingRequest
* @return A Java Future containing the result of the GetListing operation returned by the service.
* @sample AmazonDataZoneAsync.GetListing
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getListingAsync(GetListingRequest getListingRequest);
/**
*
* Gets a listing (a record of an asset at a given time).
*
*
* @param getListingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetListing operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetListing
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getListingAsync(GetListingRequest getListingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a metadata generation run in Amazon DataZone.
*
*
* @param getMetadataGenerationRunRequest
* @return A Java Future containing the result of the GetMetadataGenerationRun operation returned by the service.
* @sample AmazonDataZoneAsync.GetMetadataGenerationRun
* @see AWS API Documentation
*/
java.util.concurrent.Future getMetadataGenerationRunAsync(GetMetadataGenerationRunRequest getMetadataGenerationRunRequest);
/**
*
* Gets a metadata generation run in Amazon DataZone.
*
*
* @param getMetadataGenerationRunRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMetadataGenerationRun operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetMetadataGenerationRun
* @see AWS API Documentation
*/
java.util.concurrent.Future getMetadataGenerationRunAsync(GetMetadataGenerationRunRequest getMetadataGenerationRunRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a project in Amazon DataZone.
*
*
* @param getProjectRequest
* @return A Java Future containing the result of the GetProject operation returned by the service.
* @sample AmazonDataZoneAsync.GetProject
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getProjectAsync(GetProjectRequest getProjectRequest);
/**
*
* Gets a project in Amazon DataZone.
*
*
* @param getProjectRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetProject operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetProject
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getProjectAsync(GetProjectRequest getProjectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a subscription in Amazon DataZone.
*
*
* @param getSubscriptionRequest
* @return A Java Future containing the result of the GetSubscription operation returned by the service.
* @sample AmazonDataZoneAsync.GetSubscription
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSubscriptionAsync(GetSubscriptionRequest getSubscriptionRequest);
/**
*
* Gets a subscription in Amazon DataZone.
*
*
* @param getSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSubscription operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetSubscription
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSubscriptionAsync(GetSubscriptionRequest getSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the subscription grant in Amazon DataZone.
*
*
* @param getSubscriptionGrantRequest
* @return A Java Future containing the result of the GetSubscriptionGrant operation returned by the service.
* @sample AmazonDataZoneAsync.GetSubscriptionGrant
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getSubscriptionGrantAsync(GetSubscriptionGrantRequest getSubscriptionGrantRequest);
/**
*
* Gets the subscription grant in Amazon DataZone.
*
*
* @param getSubscriptionGrantRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSubscriptionGrant operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetSubscriptionGrant
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getSubscriptionGrantAsync(GetSubscriptionGrantRequest getSubscriptionGrantRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the details of the specified subscription request.
*
*
* @param getSubscriptionRequestDetailsRequest
* @return A Java Future containing the result of the GetSubscriptionRequestDetails operation returned by the
* service.
* @sample AmazonDataZoneAsync.GetSubscriptionRequestDetails
* @see AWS API Documentation
*/
java.util.concurrent.Future getSubscriptionRequestDetailsAsync(
GetSubscriptionRequestDetailsRequest getSubscriptionRequestDetailsRequest);
/**
*
* Gets the details of the specified subscription request.
*
*
* @param getSubscriptionRequestDetailsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSubscriptionRequestDetails operation returned by the
* service.
* @sample AmazonDataZoneAsyncHandler.GetSubscriptionRequestDetails
* @see AWS API Documentation
*/
java.util.concurrent.Future getSubscriptionRequestDetailsAsync(
GetSubscriptionRequestDetailsRequest getSubscriptionRequestDetailsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the subscription target in Amazon DataZone.
*
*
* @param getSubscriptionTargetRequest
* @return A Java Future containing the result of the GetSubscriptionTarget operation returned by the service.
* @sample AmazonDataZoneAsync.GetSubscriptionTarget
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getSubscriptionTargetAsync(GetSubscriptionTargetRequest getSubscriptionTargetRequest);
/**
*
* Gets the subscription target in Amazon DataZone.
*
*
* @param getSubscriptionTargetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSubscriptionTarget operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetSubscriptionTarget
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getSubscriptionTargetAsync(GetSubscriptionTargetRequest getSubscriptionTargetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the existing data point for the asset.
*
*
* @param getTimeSeriesDataPointRequest
* @return A Java Future containing the result of the GetTimeSeriesDataPoint operation returned by the service.
* @sample AmazonDataZoneAsync.GetTimeSeriesDataPoint
* @see AWS API Documentation
*/
java.util.concurrent.Future getTimeSeriesDataPointAsync(GetTimeSeriesDataPointRequest getTimeSeriesDataPointRequest);
/**
*
* Gets the existing data point for the asset.
*
*
* @param getTimeSeriesDataPointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTimeSeriesDataPoint operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetTimeSeriesDataPoint
* @see AWS API Documentation
*/
java.util.concurrent.Future getTimeSeriesDataPointAsync(GetTimeSeriesDataPointRequest getTimeSeriesDataPointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a user profile in Amazon DataZone.
*
*
* @param getUserProfileRequest
* @return A Java Future containing the result of the GetUserProfile operation returned by the service.
* @sample AmazonDataZoneAsync.GetUserProfile
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getUserProfileAsync(GetUserProfileRequest getUserProfileRequest);
/**
*
* Gets a user profile in Amazon DataZone.
*
*
* @param getUserProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetUserProfile operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.GetUserProfile
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getUserProfileAsync(GetUserProfileRequest getUserProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists asset filters.
*
*
* @param listAssetFiltersRequest
* @return A Java Future containing the result of the ListAssetFilters operation returned by the service.
* @sample AmazonDataZoneAsync.ListAssetFilters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAssetFiltersAsync(ListAssetFiltersRequest listAssetFiltersRequest);
/**
*
* Lists asset filters.
*
*
* @param listAssetFiltersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAssetFilters operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.ListAssetFilters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAssetFiltersAsync(ListAssetFiltersRequest listAssetFiltersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the revisions for the asset.
*
*
* @param listAssetRevisionsRequest
* @return A Java Future containing the result of the ListAssetRevisions operation returned by the service.
* @sample AmazonDataZoneAsync.ListAssetRevisions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listAssetRevisionsAsync(ListAssetRevisionsRequest listAssetRevisionsRequest);
/**
*
* Lists the revisions for the asset.
*
*
* @param listAssetRevisionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAssetRevisions operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.ListAssetRevisions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listAssetRevisionsAsync(ListAssetRevisionsRequest listAssetRevisionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists data source run activities.
*
*
* @param listDataSourceRunActivitiesRequest
* @return A Java Future containing the result of the ListDataSourceRunActivities operation returned by the service.
* @sample AmazonDataZoneAsync.ListDataSourceRunActivities
* @see AWS API Documentation
*/
java.util.concurrent.Future listDataSourceRunActivitiesAsync(
ListDataSourceRunActivitiesRequest listDataSourceRunActivitiesRequest);
/**
*
* Lists data source run activities.
*
*
* @param listDataSourceRunActivitiesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDataSourceRunActivities operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.ListDataSourceRunActivities
* @see AWS API Documentation
*/
java.util.concurrent.Future listDataSourceRunActivitiesAsync(
ListDataSourceRunActivitiesRequest listDataSourceRunActivitiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists data source runs in Amazon DataZone.
*
*
* @param listDataSourceRunsRequest
* @return A Java Future containing the result of the ListDataSourceRuns operation returned by the service.
* @sample AmazonDataZoneAsync.ListDataSourceRuns
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDataSourceRunsAsync(ListDataSourceRunsRequest listDataSourceRunsRequest);
/**
*
* Lists data source runs in Amazon DataZone.
*
*
* @param listDataSourceRunsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDataSourceRuns operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.ListDataSourceRuns
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDataSourceRunsAsync(ListDataSourceRunsRequest listDataSourceRunsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists data sources in Amazon DataZone.
*
*
* @param listDataSourcesRequest
* @return A Java Future containing the result of the ListDataSources operation returned by the service.
* @sample AmazonDataZoneAsync.ListDataSources
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDataSourcesAsync(ListDataSourcesRequest listDataSourcesRequest);
/**
*
* Lists data sources in Amazon DataZone.
*
*
* @param listDataSourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDataSources operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.ListDataSources
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDataSourcesAsync(ListDataSourcesRequest listDataSourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists Amazon DataZone domains.
*
*
* @param listDomainsRequest
* @return A Java Future containing the result of the ListDomains operation returned by the service.
* @sample AmazonDataZoneAsync.ListDomains
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDomainsAsync(ListDomainsRequest listDomainsRequest);
/**
*
* Lists Amazon DataZone domains.
*
*
* @param listDomainsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDomains operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.ListDomains
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDomainsAsync(ListDomainsRequest listDomainsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists existing environment actions.
*
*
* @param listEnvironmentActionsRequest
* @return A Java Future containing the result of the ListEnvironmentActions operation returned by the service.
* @sample AmazonDataZoneAsync.ListEnvironmentActions
* @see AWS API Documentation
*/
java.util.concurrent.Future listEnvironmentActionsAsync(ListEnvironmentActionsRequest listEnvironmentActionsRequest);
/**
*
* Lists existing environment actions.
*
*
* @param listEnvironmentActionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListEnvironmentActions operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.ListEnvironmentActions
* @see AWS API Documentation
*/
java.util.concurrent.Future listEnvironmentActionsAsync(ListEnvironmentActionsRequest listEnvironmentActionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists blueprint configurations for a Amazon DataZone environment.
*
*
* @param listEnvironmentBlueprintConfigurationsRequest
* @return A Java Future containing the result of the ListEnvironmentBlueprintConfigurations operation returned by
* the service.
* @sample AmazonDataZoneAsync.ListEnvironmentBlueprintConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listEnvironmentBlueprintConfigurationsAsync(
ListEnvironmentBlueprintConfigurationsRequest listEnvironmentBlueprintConfigurationsRequest);
/**
*
* Lists blueprint configurations for a Amazon DataZone environment.
*
*
* @param listEnvironmentBlueprintConfigurationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListEnvironmentBlueprintConfigurations operation returned by
* the service.
* @sample AmazonDataZoneAsyncHandler.ListEnvironmentBlueprintConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listEnvironmentBlueprintConfigurationsAsync(
ListEnvironmentBlueprintConfigurationsRequest listEnvironmentBlueprintConfigurationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists blueprints in an Amazon DataZone environment.
*
*
* @param listEnvironmentBlueprintsRequest
* @return A Java Future containing the result of the ListEnvironmentBlueprints operation returned by the service.
* @sample AmazonDataZoneAsync.ListEnvironmentBlueprints
* @see AWS API Documentation
*/
java.util.concurrent.Future listEnvironmentBlueprintsAsync(
ListEnvironmentBlueprintsRequest listEnvironmentBlueprintsRequest);
/**
*
* Lists blueprints in an Amazon DataZone environment.
*
*
* @param listEnvironmentBlueprintsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListEnvironmentBlueprints operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.ListEnvironmentBlueprints
* @see AWS API Documentation
*/
java.util.concurrent.Future listEnvironmentBlueprintsAsync(
ListEnvironmentBlueprintsRequest listEnvironmentBlueprintsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists Amazon DataZone environment profiles.
*
*
* @param listEnvironmentProfilesRequest
* @return A Java Future containing the result of the ListEnvironmentProfiles operation returned by the service.
* @sample AmazonDataZoneAsync.ListEnvironmentProfiles
* @see AWS API Documentation
*/
java.util.concurrent.Future listEnvironmentProfilesAsync(ListEnvironmentProfilesRequest listEnvironmentProfilesRequest);
/**
*
* Lists Amazon DataZone environment profiles.
*
*
* @param listEnvironmentProfilesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListEnvironmentProfiles operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.ListEnvironmentProfiles
* @see AWS API Documentation
*/
java.util.concurrent.Future listEnvironmentProfilesAsync(ListEnvironmentProfilesRequest listEnvironmentProfilesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists Amazon DataZone environments.
*
*
* @param listEnvironmentsRequest
* @return A Java Future containing the result of the ListEnvironments operation returned by the service.
* @sample AmazonDataZoneAsync.ListEnvironments
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listEnvironmentsAsync(ListEnvironmentsRequest listEnvironmentsRequest);
/**
*
* Lists Amazon DataZone environments.
*
*
* @param listEnvironmentsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListEnvironments operation returned by the service.
* @sample AmazonDataZoneAsyncHandler.ListEnvironments
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listEnvironmentsAsync(ListEnvironmentsRequest listEnvironmentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the history of the specified data lineage node.
*
*
* @param listLineageNodeHistoryRequest
* @return A Java Future containing the result of the ListLineageNodeHistory operation returned by the service.
* @sample AmazonDataZoneAsync.ListLineageNodeHistory
* @see AWS API Documentation
*/
java.util.concurrent.Future listLineageNodeHistoryAsync(ListLineageNodeHistoryRequest listLineageNodeHistoryRequest);
/**
*