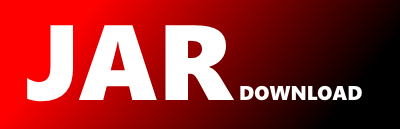
com.amazonaws.services.datazone.AmazonDataZoneClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-datazoneexternal Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.datazone;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.datazone.AmazonDataZoneClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.datazone.model.*;
import com.amazonaws.services.datazone.model.transform.*;
/**
* Client for accessing Amazon DataZone. All service calls made using this client are blocking, and will not return
* until the service call completes.
*
*
* Amazon DataZone is a data management service that enables you to catalog, discover, govern, share, and analyze your
* data. With Amazon DataZone, you can share and access your data across accounts and supported regions. Amazon DataZone
* simplifies your experience across Amazon Web Services services, including, but not limited to, Amazon Redshift,
* Amazon Athena, Amazon Web Services Glue, and Amazon Web Services Lake Formation.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonDataZoneClient extends AmazonWebServiceClient implements AmazonDataZone {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonDataZone.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "datazone";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceQuotaExceededException").withExceptionUnmarshaller(
com.amazonaws.services.datazone.model.transform.ServiceQuotaExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerException").withExceptionUnmarshaller(
com.amazonaws.services.datazone.model.transform.InternalServerExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ValidationException").withExceptionUnmarshaller(
com.amazonaws.services.datazone.model.transform.ValidationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottlingException").withExceptionUnmarshaller(
com.amazonaws.services.datazone.model.transform.ThrottlingExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.datazone.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.datazone.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnauthorizedException").withExceptionUnmarshaller(
com.amazonaws.services.datazone.model.transform.UnauthorizedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.datazone.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.datazone.model.AmazonDataZoneException.class));
public static AmazonDataZoneClientBuilder builder() {
return AmazonDataZoneClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on Amazon DataZone using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonDataZoneClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on Amazon DataZone using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonDataZoneClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("datazone.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/datazone/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/datazone/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Accepts automatically generated business-friendly metadata for your Amazon DataZone assets.
*
*
* @param acceptPredictionsRequest
* @return Result of the AcceptPredictions operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.AcceptPredictions
* @see AWS API
* Documentation
*/
@Override
public AcceptPredictionsResult acceptPredictions(AcceptPredictionsRequest request) {
request = beforeClientExecution(request);
return executeAcceptPredictions(request);
}
@SdkInternalApi
final AcceptPredictionsResult executeAcceptPredictions(AcceptPredictionsRequest acceptPredictionsRequest) {
ExecutionContext executionContext = createExecutionContext(acceptPredictionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AcceptPredictionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(acceptPredictionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AcceptPredictions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new AcceptPredictionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Accepts a subscription request to a specific asset.
*
*
* @param acceptSubscriptionRequestRequest
* @return Result of the AcceptSubscriptionRequest operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.AcceptSubscriptionRequest
* @see AWS API Documentation
*/
@Override
public AcceptSubscriptionRequestResult acceptSubscriptionRequest(AcceptSubscriptionRequestRequest request) {
request = beforeClientExecution(request);
return executeAcceptSubscriptionRequest(request);
}
@SdkInternalApi
final AcceptSubscriptionRequestResult executeAcceptSubscriptionRequest(AcceptSubscriptionRequestRequest acceptSubscriptionRequestRequest) {
ExecutionContext executionContext = createExecutionContext(acceptSubscriptionRequestRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AcceptSubscriptionRequestRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(acceptSubscriptionRequestRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AcceptSubscriptionRequest");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AcceptSubscriptionRequestResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates the environment role in Amazon DataZone.
*
*
* @param associateEnvironmentRoleRequest
* @return Result of the AssociateEnvironmentRole operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.AssociateEnvironmentRole
* @see AWS API Documentation
*/
@Override
public AssociateEnvironmentRoleResult associateEnvironmentRole(AssociateEnvironmentRoleRequest request) {
request = beforeClientExecution(request);
return executeAssociateEnvironmentRole(request);
}
@SdkInternalApi
final AssociateEnvironmentRoleResult executeAssociateEnvironmentRole(AssociateEnvironmentRoleRequest associateEnvironmentRoleRequest) {
ExecutionContext executionContext = createExecutionContext(associateEnvironmentRoleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateEnvironmentRoleRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associateEnvironmentRoleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateEnvironmentRole");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociateEnvironmentRoleResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Cancels the metadata generation run.
*
*
* @param cancelMetadataGenerationRunRequest
* @return Result of the CancelMetadataGenerationRun operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CancelMetadataGenerationRun
* @see AWS API Documentation
*/
@Override
public CancelMetadataGenerationRunResult cancelMetadataGenerationRun(CancelMetadataGenerationRunRequest request) {
request = beforeClientExecution(request);
return executeCancelMetadataGenerationRun(request);
}
@SdkInternalApi
final CancelMetadataGenerationRunResult executeCancelMetadataGenerationRun(CancelMetadataGenerationRunRequest cancelMetadataGenerationRunRequest) {
ExecutionContext executionContext = createExecutionContext(cancelMetadataGenerationRunRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CancelMetadataGenerationRunRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(cancelMetadataGenerationRunRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CancelMetadataGenerationRun");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CancelMetadataGenerationRunResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Cancels the subscription to the specified asset.
*
*
* @param cancelSubscriptionRequest
* @return Result of the CancelSubscription operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CancelSubscription
* @see AWS
* API Documentation
*/
@Override
public CancelSubscriptionResult cancelSubscription(CancelSubscriptionRequest request) {
request = beforeClientExecution(request);
return executeCancelSubscription(request);
}
@SdkInternalApi
final CancelSubscriptionResult executeCancelSubscription(CancelSubscriptionRequest cancelSubscriptionRequest) {
ExecutionContext executionContext = createExecutionContext(cancelSubscriptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CancelSubscriptionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(cancelSubscriptionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CancelSubscription");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CancelSubscriptionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an asset in Amazon DataZone catalog.
*
*
* @param createAssetRequest
* @return Result of the CreateAsset operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateAsset
* @see AWS API
* Documentation
*/
@Override
public CreateAssetResult createAsset(CreateAssetRequest request) {
request = beforeClientExecution(request);
return executeCreateAsset(request);
}
@SdkInternalApi
final CreateAssetResult executeCreateAsset(CreateAssetRequest createAssetRequest) {
ExecutionContext executionContext = createExecutionContext(createAssetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAssetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createAssetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAsset");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateAssetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a data asset filter.
*
*
* @param createAssetFilterRequest
* @return Result of the CreateAssetFilter operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateAssetFilter
* @see AWS API
* Documentation
*/
@Override
public CreateAssetFilterResult createAssetFilter(CreateAssetFilterRequest request) {
request = beforeClientExecution(request);
return executeCreateAssetFilter(request);
}
@SdkInternalApi
final CreateAssetFilterResult executeCreateAssetFilter(CreateAssetFilterRequest createAssetFilterRequest) {
ExecutionContext executionContext = createExecutionContext(createAssetFilterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAssetFilterRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createAssetFilterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAssetFilter");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateAssetFilterResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a revision of the asset.
*
*
* @param createAssetRevisionRequest
* @return Result of the CreateAssetRevision operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateAssetRevision
* @see AWS
* API Documentation
*/
@Override
public CreateAssetRevisionResult createAssetRevision(CreateAssetRevisionRequest request) {
request = beforeClientExecution(request);
return executeCreateAssetRevision(request);
}
@SdkInternalApi
final CreateAssetRevisionResult executeCreateAssetRevision(CreateAssetRevisionRequest createAssetRevisionRequest) {
ExecutionContext executionContext = createExecutionContext(createAssetRevisionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAssetRevisionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createAssetRevisionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAssetRevision");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateAssetRevisionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a custom asset type.
*
*
* @param createAssetTypeRequest
* @return Result of the CreateAssetType operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateAssetType
* @see AWS API
* Documentation
*/
@Override
public CreateAssetTypeResult createAssetType(CreateAssetTypeRequest request) {
request = beforeClientExecution(request);
return executeCreateAssetType(request);
}
@SdkInternalApi
final CreateAssetTypeResult executeCreateAssetType(CreateAssetTypeRequest createAssetTypeRequest) {
ExecutionContext executionContext = createExecutionContext(createAssetTypeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAssetTypeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createAssetTypeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAssetType");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateAssetTypeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon DataZone data source.
*
*
* @param createDataSourceRequest
* @return Result of the CreateDataSource operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateDataSource
* @see AWS API
* Documentation
*/
@Override
public CreateDataSourceResult createDataSource(CreateDataSourceRequest request) {
request = beforeClientExecution(request);
return executeCreateDataSource(request);
}
@SdkInternalApi
final CreateDataSourceResult executeCreateDataSource(CreateDataSourceRequest createDataSourceRequest) {
ExecutionContext executionContext = createExecutionContext(createDataSourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDataSourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createDataSourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDataSource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateDataSourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon DataZone domain.
*
*
* @param createDomainRequest
* @return Result of the CreateDomain operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateDomain
* @see AWS API
* Documentation
*/
@Override
public CreateDomainResult createDomain(CreateDomainRequest request) {
request = beforeClientExecution(request);
return executeCreateDomain(request);
}
@SdkInternalApi
final CreateDomainResult executeCreateDomain(CreateDomainRequest createDomainRequest) {
ExecutionContext executionContext = createExecutionContext(createDomainRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDomainRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createDomainRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDomain");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateDomainResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Create an Amazon DataZone environment.
*
*
* @param createEnvironmentRequest
* @return Result of the CreateEnvironment operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateEnvironment
* @see AWS API
* Documentation
*/
@Override
public CreateEnvironmentResult createEnvironment(CreateEnvironmentRequest request) {
request = beforeClientExecution(request);
return executeCreateEnvironment(request);
}
@SdkInternalApi
final CreateEnvironmentResult executeCreateEnvironment(CreateEnvironmentRequest createEnvironmentRequest) {
ExecutionContext executionContext = createExecutionContext(createEnvironmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateEnvironmentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createEnvironmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateEnvironment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateEnvironmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an action for the environment, for example, creates a console link for an analytics tool that is
* available in this environment.
*
*
* @param createEnvironmentActionRequest
* @return Result of the CreateEnvironmentAction operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateEnvironmentAction
* @see AWS API Documentation
*/
@Override
public CreateEnvironmentActionResult createEnvironmentAction(CreateEnvironmentActionRequest request) {
request = beforeClientExecution(request);
return executeCreateEnvironmentAction(request);
}
@SdkInternalApi
final CreateEnvironmentActionResult executeCreateEnvironmentAction(CreateEnvironmentActionRequest createEnvironmentActionRequest) {
ExecutionContext executionContext = createExecutionContext(createEnvironmentActionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateEnvironmentActionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createEnvironmentActionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateEnvironmentAction");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateEnvironmentActionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon DataZone environment profile.
*
*
* @param createEnvironmentProfileRequest
* @return Result of the CreateEnvironmentProfile operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateEnvironmentProfile
* @see AWS API Documentation
*/
@Override
public CreateEnvironmentProfileResult createEnvironmentProfile(CreateEnvironmentProfileRequest request) {
request = beforeClientExecution(request);
return executeCreateEnvironmentProfile(request);
}
@SdkInternalApi
final CreateEnvironmentProfileResult executeCreateEnvironmentProfile(CreateEnvironmentProfileRequest createEnvironmentProfileRequest) {
ExecutionContext executionContext = createExecutionContext(createEnvironmentProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateEnvironmentProfileRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createEnvironmentProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateEnvironmentProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateEnvironmentProfileResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a metadata form type.
*
*
* @param createFormTypeRequest
* @return Result of the CreateFormType operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateFormType
* @see AWS API
* Documentation
*/
@Override
public CreateFormTypeResult createFormType(CreateFormTypeRequest request) {
request = beforeClientExecution(request);
return executeCreateFormType(request);
}
@SdkInternalApi
final CreateFormTypeResult executeCreateFormType(CreateFormTypeRequest createFormTypeRequest) {
ExecutionContext executionContext = createExecutionContext(createFormTypeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateFormTypeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createFormTypeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateFormType");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateFormTypeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon DataZone business glossary.
*
*
* @param createGlossaryRequest
* @return Result of the CreateGlossary operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateGlossary
* @see AWS API
* Documentation
*/
@Override
public CreateGlossaryResult createGlossary(CreateGlossaryRequest request) {
request = beforeClientExecution(request);
return executeCreateGlossary(request);
}
@SdkInternalApi
final CreateGlossaryResult executeCreateGlossary(CreateGlossaryRequest createGlossaryRequest) {
ExecutionContext executionContext = createExecutionContext(createGlossaryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateGlossaryRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createGlossaryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateGlossary");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateGlossaryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a business glossary term.
*
*
* @param createGlossaryTermRequest
* @return Result of the CreateGlossaryTerm operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateGlossaryTerm
* @see AWS
* API Documentation
*/
@Override
public CreateGlossaryTermResult createGlossaryTerm(CreateGlossaryTermRequest request) {
request = beforeClientExecution(request);
return executeCreateGlossaryTerm(request);
}
@SdkInternalApi
final CreateGlossaryTermResult executeCreateGlossaryTerm(CreateGlossaryTermRequest createGlossaryTermRequest) {
ExecutionContext executionContext = createExecutionContext(createGlossaryTermRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateGlossaryTermRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createGlossaryTermRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateGlossaryTerm");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateGlossaryTermResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a group profile in Amazon DataZone.
*
*
* @param createGroupProfileRequest
* @return Result of the CreateGroupProfile operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateGroupProfile
* @see AWS
* API Documentation
*/
@Override
public CreateGroupProfileResult createGroupProfile(CreateGroupProfileRequest request) {
request = beforeClientExecution(request);
return executeCreateGroupProfile(request);
}
@SdkInternalApi
final CreateGroupProfileResult executeCreateGroupProfile(CreateGroupProfileRequest createGroupProfileRequest) {
ExecutionContext executionContext = createExecutionContext(createGroupProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateGroupProfileRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createGroupProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateGroupProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateGroupProfileResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Publishes a listing (a record of an asset at a given time) or removes a listing from the catalog.
*
*
* @param createListingChangeSetRequest
* @return Result of the CreateListingChangeSet operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateListingChangeSet
* @see AWS API Documentation
*/
@Override
public CreateListingChangeSetResult createListingChangeSet(CreateListingChangeSetRequest request) {
request = beforeClientExecution(request);
return executeCreateListingChangeSet(request);
}
@SdkInternalApi
final CreateListingChangeSetResult executeCreateListingChangeSet(CreateListingChangeSetRequest createListingChangeSetRequest) {
ExecutionContext executionContext = createExecutionContext(createListingChangeSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateListingChangeSetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createListingChangeSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateListingChangeSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateListingChangeSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon DataZone project.
*
*
* @param createProjectRequest
* @return Result of the CreateProject operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateProject
* @see AWS API
* Documentation
*/
@Override
public CreateProjectResult createProject(CreateProjectRequest request) {
request = beforeClientExecution(request);
return executeCreateProject(request);
}
@SdkInternalApi
final CreateProjectResult executeCreateProject(CreateProjectRequest createProjectRequest) {
ExecutionContext executionContext = createExecutionContext(createProjectRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateProjectRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createProjectRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateProject");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateProjectResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a project membership in Amazon DataZone.
*
*
* @param createProjectMembershipRequest
* @return Result of the CreateProjectMembership operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateProjectMembership
* @see AWS API Documentation
*/
@Override
public CreateProjectMembershipResult createProjectMembership(CreateProjectMembershipRequest request) {
request = beforeClientExecution(request);
return executeCreateProjectMembership(request);
}
@SdkInternalApi
final CreateProjectMembershipResult executeCreateProjectMembership(CreateProjectMembershipRequest createProjectMembershipRequest) {
ExecutionContext executionContext = createExecutionContext(createProjectMembershipRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateProjectMembershipRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createProjectMembershipRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateProjectMembership");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateProjectMembershipResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a subsscription grant in Amazon DataZone.
*
*
* @param createSubscriptionGrantRequest
* @return Result of the CreateSubscriptionGrant operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateSubscriptionGrant
* @see AWS API Documentation
*/
@Override
public CreateSubscriptionGrantResult createSubscriptionGrant(CreateSubscriptionGrantRequest request) {
request = beforeClientExecution(request);
return executeCreateSubscriptionGrant(request);
}
@SdkInternalApi
final CreateSubscriptionGrantResult executeCreateSubscriptionGrant(CreateSubscriptionGrantRequest createSubscriptionGrantRequest) {
ExecutionContext executionContext = createExecutionContext(createSubscriptionGrantRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSubscriptionGrantRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createSubscriptionGrantRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateSubscriptionGrant");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateSubscriptionGrantResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a subscription request in Amazon DataZone.
*
*
* @param createSubscriptionRequestRequest
* @return Result of the CreateSubscriptionRequest operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateSubscriptionRequest
* @see AWS API Documentation
*/
@Override
public CreateSubscriptionRequestResult createSubscriptionRequest(CreateSubscriptionRequestRequest request) {
request = beforeClientExecution(request);
return executeCreateSubscriptionRequest(request);
}
@SdkInternalApi
final CreateSubscriptionRequestResult executeCreateSubscriptionRequest(CreateSubscriptionRequestRequest createSubscriptionRequestRequest) {
ExecutionContext executionContext = createExecutionContext(createSubscriptionRequestRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSubscriptionRequestRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createSubscriptionRequestRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateSubscriptionRequest");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateSubscriptionRequestResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a subscription target in Amazon DataZone.
*
*
* @param createSubscriptionTargetRequest
* @return Result of the CreateSubscriptionTarget operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateSubscriptionTarget
* @see AWS API Documentation
*/
@Override
public CreateSubscriptionTargetResult createSubscriptionTarget(CreateSubscriptionTargetRequest request) {
request = beforeClientExecution(request);
return executeCreateSubscriptionTarget(request);
}
@SdkInternalApi
final CreateSubscriptionTargetResult executeCreateSubscriptionTarget(CreateSubscriptionTargetRequest createSubscriptionTargetRequest) {
ExecutionContext executionContext = createExecutionContext(createSubscriptionTargetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSubscriptionTargetRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createSubscriptionTargetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateSubscriptionTarget");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateSubscriptionTargetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a user profile in Amazon DataZone.
*
*
* @param createUserProfileRequest
* @return Result of the CreateUserProfile operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.CreateUserProfile
* @see AWS API
* Documentation
*/
@Override
public CreateUserProfileResult createUserProfile(CreateUserProfileRequest request) {
request = beforeClientExecution(request);
return executeCreateUserProfile(request);
}
@SdkInternalApi
final CreateUserProfileResult executeCreateUserProfile(CreateUserProfileRequest createUserProfileRequest) {
ExecutionContext executionContext = createExecutionContext(createUserProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateUserProfileRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createUserProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateUserProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateUserProfileResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Delets an asset in Amazon DataZone.
*
*
* @param deleteAssetRequest
* @return Result of the DeleteAsset operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteAsset
* @see AWS API
* Documentation
*/
@Override
public DeleteAssetResult deleteAsset(DeleteAssetRequest request) {
request = beforeClientExecution(request);
return executeDeleteAsset(request);
}
@SdkInternalApi
final DeleteAssetResult executeDeleteAsset(DeleteAssetRequest deleteAssetRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAssetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAssetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteAssetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAsset");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteAssetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an asset filter.
*
*
* @param deleteAssetFilterRequest
* @return Result of the DeleteAssetFilter operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteAssetFilter
* @see AWS API
* Documentation
*/
@Override
public DeleteAssetFilterResult deleteAssetFilter(DeleteAssetFilterRequest request) {
request = beforeClientExecution(request);
return executeDeleteAssetFilter(request);
}
@SdkInternalApi
final DeleteAssetFilterResult executeDeleteAssetFilter(DeleteAssetFilterRequest deleteAssetFilterRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAssetFilterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAssetFilterRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteAssetFilterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAssetFilter");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteAssetFilterResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an asset type in Amazon DataZone.
*
*
* @param deleteAssetTypeRequest
* @return Result of the DeleteAssetType operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteAssetType
* @see AWS API
* Documentation
*/
@Override
public DeleteAssetTypeResult deleteAssetType(DeleteAssetTypeRequest request) {
request = beforeClientExecution(request);
return executeDeleteAssetType(request);
}
@SdkInternalApi
final DeleteAssetTypeResult executeDeleteAssetType(DeleteAssetTypeRequest deleteAssetTypeRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAssetTypeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAssetTypeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteAssetTypeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAssetType");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteAssetTypeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a data source in Amazon DataZone.
*
*
* @param deleteDataSourceRequest
* @return Result of the DeleteDataSource operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteDataSource
* @see AWS API
* Documentation
*/
@Override
public DeleteDataSourceResult deleteDataSource(DeleteDataSourceRequest request) {
request = beforeClientExecution(request);
return executeDeleteDataSource(request);
}
@SdkInternalApi
final DeleteDataSourceResult executeDeleteDataSource(DeleteDataSourceRequest deleteDataSourceRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDataSourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDataSourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteDataSourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDataSource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteDataSourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a Amazon DataZone domain.
*
*
* @param deleteDomainRequest
* @return Result of the DeleteDomain operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteDomain
* @see AWS API
* Documentation
*/
@Override
public DeleteDomainResult deleteDomain(DeleteDomainRequest request) {
request = beforeClientExecution(request);
return executeDeleteDomain(request);
}
@SdkInternalApi
final DeleteDomainResult executeDeleteDomain(DeleteDomainRequest deleteDomainRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDomainRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDomainRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteDomainRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDomain");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteDomainResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an environment in Amazon DataZone.
*
*
* @param deleteEnvironmentRequest
* @return Result of the DeleteEnvironment operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteEnvironment
* @see AWS API
* Documentation
*/
@Override
public DeleteEnvironmentResult deleteEnvironment(DeleteEnvironmentRequest request) {
request = beforeClientExecution(request);
return executeDeleteEnvironment(request);
}
@SdkInternalApi
final DeleteEnvironmentResult executeDeleteEnvironment(DeleteEnvironmentRequest deleteEnvironmentRequest) {
ExecutionContext executionContext = createExecutionContext(deleteEnvironmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteEnvironmentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteEnvironmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteEnvironment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteEnvironmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an action for the environment, for example, deletes a console link for an analytics tool that is
* available in this environment.
*
*
* @param deleteEnvironmentActionRequest
* @return Result of the DeleteEnvironmentAction operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteEnvironmentAction
* @see AWS API Documentation
*/
@Override
public DeleteEnvironmentActionResult deleteEnvironmentAction(DeleteEnvironmentActionRequest request) {
request = beforeClientExecution(request);
return executeDeleteEnvironmentAction(request);
}
@SdkInternalApi
final DeleteEnvironmentActionResult executeDeleteEnvironmentAction(DeleteEnvironmentActionRequest deleteEnvironmentActionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteEnvironmentActionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteEnvironmentActionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteEnvironmentActionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteEnvironmentAction");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteEnvironmentActionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the blueprint configuration in Amazon DataZone.
*
*
* @param deleteEnvironmentBlueprintConfigurationRequest
* @return Result of the DeleteEnvironmentBlueprintConfiguration operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteEnvironmentBlueprintConfiguration
* @see AWS API Documentation
*/
@Override
public DeleteEnvironmentBlueprintConfigurationResult deleteEnvironmentBlueprintConfiguration(DeleteEnvironmentBlueprintConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDeleteEnvironmentBlueprintConfiguration(request);
}
@SdkInternalApi
final DeleteEnvironmentBlueprintConfigurationResult executeDeleteEnvironmentBlueprintConfiguration(
DeleteEnvironmentBlueprintConfigurationRequest deleteEnvironmentBlueprintConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteEnvironmentBlueprintConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteEnvironmentBlueprintConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteEnvironmentBlueprintConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteEnvironmentBlueprintConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteEnvironmentBlueprintConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an environment profile in Amazon DataZone.
*
*
* @param deleteEnvironmentProfileRequest
* @return Result of the DeleteEnvironmentProfile operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteEnvironmentProfile
* @see AWS API Documentation
*/
@Override
public DeleteEnvironmentProfileResult deleteEnvironmentProfile(DeleteEnvironmentProfileRequest request) {
request = beforeClientExecution(request);
return executeDeleteEnvironmentProfile(request);
}
@SdkInternalApi
final DeleteEnvironmentProfileResult executeDeleteEnvironmentProfile(DeleteEnvironmentProfileRequest deleteEnvironmentProfileRequest) {
ExecutionContext executionContext = createExecutionContext(deleteEnvironmentProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteEnvironmentProfileRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteEnvironmentProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteEnvironmentProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteEnvironmentProfileResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Delets and metadata form type in Amazon DataZone.
*
*
* @param deleteFormTypeRequest
* @return Result of the DeleteFormType operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteFormType
* @see AWS API
* Documentation
*/
@Override
public DeleteFormTypeResult deleteFormType(DeleteFormTypeRequest request) {
request = beforeClientExecution(request);
return executeDeleteFormType(request);
}
@SdkInternalApi
final DeleteFormTypeResult executeDeleteFormType(DeleteFormTypeRequest deleteFormTypeRequest) {
ExecutionContext executionContext = createExecutionContext(deleteFormTypeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteFormTypeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteFormTypeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteFormType");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteFormTypeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a business glossary in Amazon DataZone.
*
*
* @param deleteGlossaryRequest
* @return Result of the DeleteGlossary operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteGlossary
* @see AWS API
* Documentation
*/
@Override
public DeleteGlossaryResult deleteGlossary(DeleteGlossaryRequest request) {
request = beforeClientExecution(request);
return executeDeleteGlossary(request);
}
@SdkInternalApi
final DeleteGlossaryResult executeDeleteGlossary(DeleteGlossaryRequest deleteGlossaryRequest) {
ExecutionContext executionContext = createExecutionContext(deleteGlossaryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteGlossaryRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteGlossaryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteGlossary");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteGlossaryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a business glossary term in Amazon DataZone.
*
*
* @param deleteGlossaryTermRequest
* @return Result of the DeleteGlossaryTerm operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteGlossaryTerm
* @see AWS
* API Documentation
*/
@Override
public DeleteGlossaryTermResult deleteGlossaryTerm(DeleteGlossaryTermRequest request) {
request = beforeClientExecution(request);
return executeDeleteGlossaryTerm(request);
}
@SdkInternalApi
final DeleteGlossaryTermResult executeDeleteGlossaryTerm(DeleteGlossaryTermRequest deleteGlossaryTermRequest) {
ExecutionContext executionContext = createExecutionContext(deleteGlossaryTermRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteGlossaryTermRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteGlossaryTermRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteGlossaryTerm");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteGlossaryTermResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a listing (a record of an asset at a given time).
*
*
* @param deleteListingRequest
* @return Result of the DeleteListing operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteListing
* @see AWS API
* Documentation
*/
@Override
public DeleteListingResult deleteListing(DeleteListingRequest request) {
request = beforeClientExecution(request);
return executeDeleteListing(request);
}
@SdkInternalApi
final DeleteListingResult executeDeleteListing(DeleteListingRequest deleteListingRequest) {
ExecutionContext executionContext = createExecutionContext(deleteListingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteListingRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteListingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteListing");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteListingResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a project in Amazon DataZone.
*
*
* @param deleteProjectRequest
* @return Result of the DeleteProject operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteProject
* @see AWS API
* Documentation
*/
@Override
public DeleteProjectResult deleteProject(DeleteProjectRequest request) {
request = beforeClientExecution(request);
return executeDeleteProject(request);
}
@SdkInternalApi
final DeleteProjectResult executeDeleteProject(DeleteProjectRequest deleteProjectRequest) {
ExecutionContext executionContext = createExecutionContext(deleteProjectRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteProjectRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteProjectRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteProject");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteProjectResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes project membership in Amazon DataZone.
*
*
* @param deleteProjectMembershipRequest
* @return Result of the DeleteProjectMembership operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteProjectMembership
* @see AWS API Documentation
*/
@Override
public DeleteProjectMembershipResult deleteProjectMembership(DeleteProjectMembershipRequest request) {
request = beforeClientExecution(request);
return executeDeleteProjectMembership(request);
}
@SdkInternalApi
final DeleteProjectMembershipResult executeDeleteProjectMembership(DeleteProjectMembershipRequest deleteProjectMembershipRequest) {
ExecutionContext executionContext = createExecutionContext(deleteProjectMembershipRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteProjectMembershipRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteProjectMembershipRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteProjectMembership");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteProjectMembershipResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes and subscription grant in Amazon DataZone.
*
*
* @param deleteSubscriptionGrantRequest
* @return Result of the DeleteSubscriptionGrant operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteSubscriptionGrant
* @see AWS API Documentation
*/
@Override
public DeleteSubscriptionGrantResult deleteSubscriptionGrant(DeleteSubscriptionGrantRequest request) {
request = beforeClientExecution(request);
return executeDeleteSubscriptionGrant(request);
}
@SdkInternalApi
final DeleteSubscriptionGrantResult executeDeleteSubscriptionGrant(DeleteSubscriptionGrantRequest deleteSubscriptionGrantRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSubscriptionGrantRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteSubscriptionGrantRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteSubscriptionGrantRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteSubscriptionGrant");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteSubscriptionGrantResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a subscription request in Amazon DataZone.
*
*
* @param deleteSubscriptionRequestRequest
* @return Result of the DeleteSubscriptionRequest operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteSubscriptionRequest
* @see AWS API Documentation
*/
@Override
public DeleteSubscriptionRequestResult deleteSubscriptionRequest(DeleteSubscriptionRequestRequest request) {
request = beforeClientExecution(request);
return executeDeleteSubscriptionRequest(request);
}
@SdkInternalApi
final DeleteSubscriptionRequestResult executeDeleteSubscriptionRequest(DeleteSubscriptionRequestRequest deleteSubscriptionRequestRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSubscriptionRequestRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteSubscriptionRequestRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteSubscriptionRequestRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteSubscriptionRequest");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteSubscriptionRequestResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a subscription target in Amazon DataZone.
*
*
* @param deleteSubscriptionTargetRequest
* @return Result of the DeleteSubscriptionTarget operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteSubscriptionTarget
* @see AWS API Documentation
*/
@Override
public DeleteSubscriptionTargetResult deleteSubscriptionTarget(DeleteSubscriptionTargetRequest request) {
request = beforeClientExecution(request);
return executeDeleteSubscriptionTarget(request);
}
@SdkInternalApi
final DeleteSubscriptionTargetResult executeDeleteSubscriptionTarget(DeleteSubscriptionTargetRequest deleteSubscriptionTargetRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSubscriptionTargetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteSubscriptionTargetRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteSubscriptionTargetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteSubscriptionTarget");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteSubscriptionTargetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified time series form for the specified asset.
*
*
* @param deleteTimeSeriesDataPointsRequest
* @return Result of the DeleteTimeSeriesDataPoints operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DeleteTimeSeriesDataPoints
* @see AWS API Documentation
*/
@Override
public DeleteTimeSeriesDataPointsResult deleteTimeSeriesDataPoints(DeleteTimeSeriesDataPointsRequest request) {
request = beforeClientExecution(request);
return executeDeleteTimeSeriesDataPoints(request);
}
@SdkInternalApi
final DeleteTimeSeriesDataPointsResult executeDeleteTimeSeriesDataPoints(DeleteTimeSeriesDataPointsRequest deleteTimeSeriesDataPointsRequest) {
ExecutionContext executionContext = createExecutionContext(deleteTimeSeriesDataPointsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteTimeSeriesDataPointsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteTimeSeriesDataPointsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteTimeSeriesDataPoints");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteTimeSeriesDataPointsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates the environment role in Amazon DataZone.
*
*
* @param disassociateEnvironmentRoleRequest
* @return Result of the DisassociateEnvironmentRole operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.DisassociateEnvironmentRole
* @see AWS API Documentation
*/
@Override
public DisassociateEnvironmentRoleResult disassociateEnvironmentRole(DisassociateEnvironmentRoleRequest request) {
request = beforeClientExecution(request);
return executeDisassociateEnvironmentRole(request);
}
@SdkInternalApi
final DisassociateEnvironmentRoleResult executeDisassociateEnvironmentRole(DisassociateEnvironmentRoleRequest disassociateEnvironmentRoleRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateEnvironmentRoleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateEnvironmentRoleRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateEnvironmentRoleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateEnvironmentRole");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateEnvironmentRoleResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets an Amazon DataZone asset.
*
*
* @param getAssetRequest
* @return Result of the GetAsset operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetAsset
* @see AWS API
* Documentation
*/
@Override
public GetAssetResult getAsset(GetAssetRequest request) {
request = beforeClientExecution(request);
return executeGetAsset(request);
}
@SdkInternalApi
final GetAssetResult executeGetAsset(GetAssetRequest getAssetRequest) {
ExecutionContext executionContext = createExecutionContext(getAssetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAssetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getAssetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAsset");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetAssetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets an asset filter.
*
*
* @param getAssetFilterRequest
* @return Result of the GetAssetFilter operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetAssetFilter
* @see AWS API
* Documentation
*/
@Override
public GetAssetFilterResult getAssetFilter(GetAssetFilterRequest request) {
request = beforeClientExecution(request);
return executeGetAssetFilter(request);
}
@SdkInternalApi
final GetAssetFilterResult executeGetAssetFilter(GetAssetFilterRequest getAssetFilterRequest) {
ExecutionContext executionContext = createExecutionContext(getAssetFilterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAssetFilterRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getAssetFilterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAssetFilter");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetAssetFilterResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets an Amazon DataZone asset type.
*
*
* @param getAssetTypeRequest
* @return Result of the GetAssetType operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetAssetType
* @see AWS API
* Documentation
*/
@Override
public GetAssetTypeResult getAssetType(GetAssetTypeRequest request) {
request = beforeClientExecution(request);
return executeGetAssetType(request);
}
@SdkInternalApi
final GetAssetTypeResult executeGetAssetType(GetAssetTypeRequest getAssetTypeRequest) {
ExecutionContext executionContext = createExecutionContext(getAssetTypeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAssetTypeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getAssetTypeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAssetType");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetAssetTypeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets an Amazon DataZone data source.
*
*
* @param getDataSourceRequest
* @return Result of the GetDataSource operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetDataSource
* @see AWS API
* Documentation
*/
@Override
public GetDataSourceResult getDataSource(GetDataSourceRequest request) {
request = beforeClientExecution(request);
return executeGetDataSource(request);
}
@SdkInternalApi
final GetDataSourceResult executeGetDataSource(GetDataSourceRequest getDataSourceRequest) {
ExecutionContext executionContext = createExecutionContext(getDataSourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDataSourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getDataSourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDataSource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetDataSourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets an Amazon DataZone data source run.
*
*
* @param getDataSourceRunRequest
* @return Result of the GetDataSourceRun operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetDataSourceRun
* @see AWS API
* Documentation
*/
@Override
public GetDataSourceRunResult getDataSourceRun(GetDataSourceRunRequest request) {
request = beforeClientExecution(request);
return executeGetDataSourceRun(request);
}
@SdkInternalApi
final GetDataSourceRunResult executeGetDataSourceRun(GetDataSourceRunRequest getDataSourceRunRequest) {
ExecutionContext executionContext = createExecutionContext(getDataSourceRunRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDataSourceRunRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getDataSourceRunRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDataSourceRun");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetDataSourceRunResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets an Amazon DataZone domain.
*
*
* @param getDomainRequest
* @return Result of the GetDomain operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetDomain
* @see AWS API
* Documentation
*/
@Override
public GetDomainResult getDomain(GetDomainRequest request) {
request = beforeClientExecution(request);
return executeGetDomain(request);
}
@SdkInternalApi
final GetDomainResult executeGetDomain(GetDomainRequest getDomainRequest) {
ExecutionContext executionContext = createExecutionContext(getDomainRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDomainRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getDomainRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDomain");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetDomainResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets an Amazon DataZone environment.
*
*
* @param getEnvironmentRequest
* @return Result of the GetEnvironment operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetEnvironment
* @see AWS API
* Documentation
*/
@Override
public GetEnvironmentResult getEnvironment(GetEnvironmentRequest request) {
request = beforeClientExecution(request);
return executeGetEnvironment(request);
}
@SdkInternalApi
final GetEnvironmentResult executeGetEnvironment(GetEnvironmentRequest getEnvironmentRequest) {
ExecutionContext executionContext = createExecutionContext(getEnvironmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetEnvironmentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getEnvironmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetEnvironment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetEnvironmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the specified environment action.
*
*
* @param getEnvironmentActionRequest
* @return Result of the GetEnvironmentAction operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetEnvironmentAction
* @see AWS
* API Documentation
*/
@Override
public GetEnvironmentActionResult getEnvironmentAction(GetEnvironmentActionRequest request) {
request = beforeClientExecution(request);
return executeGetEnvironmentAction(request);
}
@SdkInternalApi
final GetEnvironmentActionResult executeGetEnvironmentAction(GetEnvironmentActionRequest getEnvironmentActionRequest) {
ExecutionContext executionContext = createExecutionContext(getEnvironmentActionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetEnvironmentActionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getEnvironmentActionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetEnvironmentAction");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetEnvironmentActionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets an Amazon DataZone blueprint.
*
*
* @param getEnvironmentBlueprintRequest
* @return Result of the GetEnvironmentBlueprint operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetEnvironmentBlueprint
* @see AWS API Documentation
*/
@Override
public GetEnvironmentBlueprintResult getEnvironmentBlueprint(GetEnvironmentBlueprintRequest request) {
request = beforeClientExecution(request);
return executeGetEnvironmentBlueprint(request);
}
@SdkInternalApi
final GetEnvironmentBlueprintResult executeGetEnvironmentBlueprint(GetEnvironmentBlueprintRequest getEnvironmentBlueprintRequest) {
ExecutionContext executionContext = createExecutionContext(getEnvironmentBlueprintRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetEnvironmentBlueprintRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getEnvironmentBlueprintRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetEnvironmentBlueprint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetEnvironmentBlueprintResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the blueprint configuration in Amazon DataZone.
*
*
* @param getEnvironmentBlueprintConfigurationRequest
* @return Result of the GetEnvironmentBlueprintConfiguration operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetEnvironmentBlueprintConfiguration
* @see AWS API Documentation
*/
@Override
public GetEnvironmentBlueprintConfigurationResult getEnvironmentBlueprintConfiguration(GetEnvironmentBlueprintConfigurationRequest request) {
request = beforeClientExecution(request);
return executeGetEnvironmentBlueprintConfiguration(request);
}
@SdkInternalApi
final GetEnvironmentBlueprintConfigurationResult executeGetEnvironmentBlueprintConfiguration(
GetEnvironmentBlueprintConfigurationRequest getEnvironmentBlueprintConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(getEnvironmentBlueprintConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetEnvironmentBlueprintConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getEnvironmentBlueprintConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetEnvironmentBlueprintConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetEnvironmentBlueprintConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the credentials of an environment in Amazon DataZone.
*
*
* @param getEnvironmentCredentialsRequest
* @return Result of the GetEnvironmentCredentials operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetEnvironmentCredentials
* @see AWS API Documentation
*/
@Override
public GetEnvironmentCredentialsResult getEnvironmentCredentials(GetEnvironmentCredentialsRequest request) {
request = beforeClientExecution(request);
return executeGetEnvironmentCredentials(request);
}
@SdkInternalApi
final GetEnvironmentCredentialsResult executeGetEnvironmentCredentials(GetEnvironmentCredentialsRequest getEnvironmentCredentialsRequest) {
ExecutionContext executionContext = createExecutionContext(getEnvironmentCredentialsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetEnvironmentCredentialsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getEnvironmentCredentialsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetEnvironmentCredentials");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetEnvironmentCredentialsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets an evinronment profile in Amazon DataZone.
*
*
* @param getEnvironmentProfileRequest
* @return Result of the GetEnvironmentProfile operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetEnvironmentProfile
* @see AWS
* API Documentation
*/
@Override
public GetEnvironmentProfileResult getEnvironmentProfile(GetEnvironmentProfileRequest request) {
request = beforeClientExecution(request);
return executeGetEnvironmentProfile(request);
}
@SdkInternalApi
final GetEnvironmentProfileResult executeGetEnvironmentProfile(GetEnvironmentProfileRequest getEnvironmentProfileRequest) {
ExecutionContext executionContext = createExecutionContext(getEnvironmentProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetEnvironmentProfileRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getEnvironmentProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetEnvironmentProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetEnvironmentProfileResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a metadata form type in Amazon DataZone.
*
*
* @param getFormTypeRequest
* @return Result of the GetFormType operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetFormType
* @see AWS API
* Documentation
*/
@Override
public GetFormTypeResult getFormType(GetFormTypeRequest request) {
request = beforeClientExecution(request);
return executeGetFormType(request);
}
@SdkInternalApi
final GetFormTypeResult executeGetFormType(GetFormTypeRequest getFormTypeRequest) {
ExecutionContext executionContext = createExecutionContext(getFormTypeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetFormTypeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getFormTypeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetFormType");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetFormTypeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a business glossary in Amazon DataZone.
*
*
* @param getGlossaryRequest
* @return Result of the GetGlossary operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetGlossary
* @see AWS API
* Documentation
*/
@Override
public GetGlossaryResult getGlossary(GetGlossaryRequest request) {
request = beforeClientExecution(request);
return executeGetGlossary(request);
}
@SdkInternalApi
final GetGlossaryResult executeGetGlossary(GetGlossaryRequest getGlossaryRequest) {
ExecutionContext executionContext = createExecutionContext(getGlossaryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetGlossaryRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getGlossaryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetGlossary");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetGlossaryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a business glossary term in Amazon DataZone.
*
*
* @param getGlossaryTermRequest
* @return Result of the GetGlossaryTerm operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetGlossaryTerm
* @see AWS API
* Documentation
*/
@Override
public GetGlossaryTermResult getGlossaryTerm(GetGlossaryTermRequest request) {
request = beforeClientExecution(request);
return executeGetGlossaryTerm(request);
}
@SdkInternalApi
final GetGlossaryTermResult executeGetGlossaryTerm(GetGlossaryTermRequest getGlossaryTermRequest) {
ExecutionContext executionContext = createExecutionContext(getGlossaryTermRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetGlossaryTermRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getGlossaryTermRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetGlossaryTerm");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetGlossaryTermResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a group profile in Amazon DataZone.
*
*
* @param getGroupProfileRequest
* @return Result of the GetGroupProfile operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetGroupProfile
* @see AWS API
* Documentation
*/
@Override
public GetGroupProfileResult getGroupProfile(GetGroupProfileRequest request) {
request = beforeClientExecution(request);
return executeGetGroupProfile(request);
}
@SdkInternalApi
final GetGroupProfileResult executeGetGroupProfile(GetGroupProfileRequest getGroupProfileRequest) {
ExecutionContext executionContext = createExecutionContext(getGroupProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetGroupProfileRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getGroupProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetGroupProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetGroupProfileResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the data portal URL for the specified Amazon DataZone domain.
*
*
* @param getIamPortalLoginUrlRequest
* @return Result of the GetIamPortalLoginUrl operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetIamPortalLoginUrl
* @see AWS
* API Documentation
*/
@Override
public GetIamPortalLoginUrlResult getIamPortalLoginUrl(GetIamPortalLoginUrlRequest request) {
request = beforeClientExecution(request);
return executeGetIamPortalLoginUrl(request);
}
@SdkInternalApi
final GetIamPortalLoginUrlResult executeGetIamPortalLoginUrl(GetIamPortalLoginUrlRequest getIamPortalLoginUrlRequest) {
ExecutionContext executionContext = createExecutionContext(getIamPortalLoginUrlRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetIamPortalLoginUrlRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getIamPortalLoginUrlRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetIamPortalLoginUrl");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetIamPortalLoginUrlResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the data lineage node.
*
*
* @param getLineageNodeRequest
* @return Result of the GetLineageNode operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetLineageNode
* @see AWS API
* Documentation
*/
@Override
public GetLineageNodeResult getLineageNode(GetLineageNodeRequest request) {
request = beforeClientExecution(request);
return executeGetLineageNode(request);
}
@SdkInternalApi
final GetLineageNodeResult executeGetLineageNode(GetLineageNodeRequest getLineageNodeRequest) {
ExecutionContext executionContext = createExecutionContext(getLineageNodeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetLineageNodeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getLineageNodeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetLineageNode");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetLineageNodeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a listing (a record of an asset at a given time).
*
*
* @param getListingRequest
* @return Result of the GetListing operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetListing
* @see AWS API
* Documentation
*/
@Override
public GetListingResult getListing(GetListingRequest request) {
request = beforeClientExecution(request);
return executeGetListing(request);
}
@SdkInternalApi
final GetListingResult executeGetListing(GetListingRequest getListingRequest) {
ExecutionContext executionContext = createExecutionContext(getListingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetListingRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getListingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetListing");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetListingResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a metadata generation run in Amazon DataZone.
*
*
* @param getMetadataGenerationRunRequest
* @return Result of the GetMetadataGenerationRun operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetMetadataGenerationRun
* @see AWS API Documentation
*/
@Override
public GetMetadataGenerationRunResult getMetadataGenerationRun(GetMetadataGenerationRunRequest request) {
request = beforeClientExecution(request);
return executeGetMetadataGenerationRun(request);
}
@SdkInternalApi
final GetMetadataGenerationRunResult executeGetMetadataGenerationRun(GetMetadataGenerationRunRequest getMetadataGenerationRunRequest) {
ExecutionContext executionContext = createExecutionContext(getMetadataGenerationRunRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetMetadataGenerationRunRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getMetadataGenerationRunRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetMetadataGenerationRun");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetMetadataGenerationRunResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a project in Amazon DataZone.
*
*
* @param getProjectRequest
* @return Result of the GetProject operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetProject
* @see AWS API
* Documentation
*/
@Override
public GetProjectResult getProject(GetProjectRequest request) {
request = beforeClientExecution(request);
return executeGetProject(request);
}
@SdkInternalApi
final GetProjectResult executeGetProject(GetProjectRequest getProjectRequest) {
ExecutionContext executionContext = createExecutionContext(getProjectRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetProjectRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getProjectRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetProject");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetProjectResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a subscription in Amazon DataZone.
*
*
* @param getSubscriptionRequest
* @return Result of the GetSubscription operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetSubscription
* @see AWS API
* Documentation
*/
@Override
public GetSubscriptionResult getSubscription(GetSubscriptionRequest request) {
request = beforeClientExecution(request);
return executeGetSubscription(request);
}
@SdkInternalApi
final GetSubscriptionResult executeGetSubscription(GetSubscriptionRequest getSubscriptionRequest) {
ExecutionContext executionContext = createExecutionContext(getSubscriptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSubscriptionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getSubscriptionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSubscription");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetSubscriptionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the subscription grant in Amazon DataZone.
*
*
* @param getSubscriptionGrantRequest
* @return Result of the GetSubscriptionGrant operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetSubscriptionGrant
* @see AWS
* API Documentation
*/
@Override
public GetSubscriptionGrantResult getSubscriptionGrant(GetSubscriptionGrantRequest request) {
request = beforeClientExecution(request);
return executeGetSubscriptionGrant(request);
}
@SdkInternalApi
final GetSubscriptionGrantResult executeGetSubscriptionGrant(GetSubscriptionGrantRequest getSubscriptionGrantRequest) {
ExecutionContext executionContext = createExecutionContext(getSubscriptionGrantRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSubscriptionGrantRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getSubscriptionGrantRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSubscriptionGrant");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetSubscriptionGrantResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the details of the specified subscription request.
*
*
* @param getSubscriptionRequestDetailsRequest
* @return Result of the GetSubscriptionRequestDetails operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetSubscriptionRequestDetails
* @see AWS API Documentation
*/
@Override
public GetSubscriptionRequestDetailsResult getSubscriptionRequestDetails(GetSubscriptionRequestDetailsRequest request) {
request = beforeClientExecution(request);
return executeGetSubscriptionRequestDetails(request);
}
@SdkInternalApi
final GetSubscriptionRequestDetailsResult executeGetSubscriptionRequestDetails(GetSubscriptionRequestDetailsRequest getSubscriptionRequestDetailsRequest) {
ExecutionContext executionContext = createExecutionContext(getSubscriptionRequestDetailsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSubscriptionRequestDetailsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getSubscriptionRequestDetailsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSubscriptionRequestDetails");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetSubscriptionRequestDetailsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the subscription target in Amazon DataZone.
*
*
* @param getSubscriptionTargetRequest
* @return Result of the GetSubscriptionTarget operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetSubscriptionTarget
* @see AWS
* API Documentation
*/
@Override
public GetSubscriptionTargetResult getSubscriptionTarget(GetSubscriptionTargetRequest request) {
request = beforeClientExecution(request);
return executeGetSubscriptionTarget(request);
}
@SdkInternalApi
final GetSubscriptionTargetResult executeGetSubscriptionTarget(GetSubscriptionTargetRequest getSubscriptionTargetRequest) {
ExecutionContext executionContext = createExecutionContext(getSubscriptionTargetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSubscriptionTargetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getSubscriptionTargetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSubscriptionTarget");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetSubscriptionTargetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the existing data point for the asset.
*
*
* @param getTimeSeriesDataPointRequest
* @return Result of the GetTimeSeriesDataPoint operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetTimeSeriesDataPoint
* @see AWS API Documentation
*/
@Override
public GetTimeSeriesDataPointResult getTimeSeriesDataPoint(GetTimeSeriesDataPointRequest request) {
request = beforeClientExecution(request);
return executeGetTimeSeriesDataPoint(request);
}
@SdkInternalApi
final GetTimeSeriesDataPointResult executeGetTimeSeriesDataPoint(GetTimeSeriesDataPointRequest getTimeSeriesDataPointRequest) {
ExecutionContext executionContext = createExecutionContext(getTimeSeriesDataPointRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetTimeSeriesDataPointRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getTimeSeriesDataPointRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetTimeSeriesDataPoint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetTimeSeriesDataPointResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a user profile in Amazon DataZone.
*
*
* @param getUserProfileRequest
* @return Result of the GetUserProfile operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.GetUserProfile
* @see AWS API
* Documentation
*/
@Override
public GetUserProfileResult getUserProfile(GetUserProfileRequest request) {
request = beforeClientExecution(request);
return executeGetUserProfile(request);
}
@SdkInternalApi
final GetUserProfileResult executeGetUserProfile(GetUserProfileRequest getUserProfileRequest) {
ExecutionContext executionContext = createExecutionContext(getUserProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetUserProfileRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getUserProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetUserProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetUserProfileResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists asset filters.
*
*
* @param listAssetFiltersRequest
* @return Result of the ListAssetFilters operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListAssetFilters
* @see AWS API
* Documentation
*/
@Override
public ListAssetFiltersResult listAssetFilters(ListAssetFiltersRequest request) {
request = beforeClientExecution(request);
return executeListAssetFilters(request);
}
@SdkInternalApi
final ListAssetFiltersResult executeListAssetFilters(ListAssetFiltersRequest listAssetFiltersRequest) {
ExecutionContext executionContext = createExecutionContext(listAssetFiltersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAssetFiltersRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listAssetFiltersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAssetFilters");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListAssetFiltersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the revisions for the asset.
*
*
* @param listAssetRevisionsRequest
* @return Result of the ListAssetRevisions operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListAssetRevisions
* @see AWS
* API Documentation
*/
@Override
public ListAssetRevisionsResult listAssetRevisions(ListAssetRevisionsRequest request) {
request = beforeClientExecution(request);
return executeListAssetRevisions(request);
}
@SdkInternalApi
final ListAssetRevisionsResult executeListAssetRevisions(ListAssetRevisionsRequest listAssetRevisionsRequest) {
ExecutionContext executionContext = createExecutionContext(listAssetRevisionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAssetRevisionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listAssetRevisionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAssetRevisions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListAssetRevisionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists data source run activities.
*
*
* @param listDataSourceRunActivitiesRequest
* @return Result of the ListDataSourceRunActivities operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListDataSourceRunActivities
* @see AWS API Documentation
*/
@Override
public ListDataSourceRunActivitiesResult listDataSourceRunActivities(ListDataSourceRunActivitiesRequest request) {
request = beforeClientExecution(request);
return executeListDataSourceRunActivities(request);
}
@SdkInternalApi
final ListDataSourceRunActivitiesResult executeListDataSourceRunActivities(ListDataSourceRunActivitiesRequest listDataSourceRunActivitiesRequest) {
ExecutionContext executionContext = createExecutionContext(listDataSourceRunActivitiesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDataSourceRunActivitiesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listDataSourceRunActivitiesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDataSourceRunActivities");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListDataSourceRunActivitiesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists data source runs in Amazon DataZone.
*
*
* @param listDataSourceRunsRequest
* @return Result of the ListDataSourceRuns operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListDataSourceRuns
* @see AWS
* API Documentation
*/
@Override
public ListDataSourceRunsResult listDataSourceRuns(ListDataSourceRunsRequest request) {
request = beforeClientExecution(request);
return executeListDataSourceRuns(request);
}
@SdkInternalApi
final ListDataSourceRunsResult executeListDataSourceRuns(ListDataSourceRunsRequest listDataSourceRunsRequest) {
ExecutionContext executionContext = createExecutionContext(listDataSourceRunsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDataSourceRunsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listDataSourceRunsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDataSourceRuns");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListDataSourceRunsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists data sources in Amazon DataZone.
*
*
* @param listDataSourcesRequest
* @return Result of the ListDataSources operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListDataSources
* @see AWS API
* Documentation
*/
@Override
public ListDataSourcesResult listDataSources(ListDataSourcesRequest request) {
request = beforeClientExecution(request);
return executeListDataSources(request);
}
@SdkInternalApi
final ListDataSourcesResult executeListDataSources(ListDataSourcesRequest listDataSourcesRequest) {
ExecutionContext executionContext = createExecutionContext(listDataSourcesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDataSourcesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listDataSourcesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDataSources");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListDataSourcesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists Amazon DataZone domains.
*
*
* @param listDomainsRequest
* @return Result of the ListDomains operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* The request has exceeded the specified service quota.
* @throws ConflictException
* There is a conflict while performing this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListDomains
* @see AWS API
* Documentation
*/
@Override
public ListDomainsResult listDomains(ListDomainsRequest request) {
request = beforeClientExecution(request);
return executeListDomains(request);
}
@SdkInternalApi
final ListDomainsResult executeListDomains(ListDomainsRequest listDomainsRequest) {
ExecutionContext executionContext = createExecutionContext(listDomainsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDomainsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listDomainsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDomains");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListDomainsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists existing environment actions.
*
*
* @param listEnvironmentActionsRequest
* @return Result of the ListEnvironmentActions operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListEnvironmentActions
* @see AWS API Documentation
*/
@Override
public ListEnvironmentActionsResult listEnvironmentActions(ListEnvironmentActionsRequest request) {
request = beforeClientExecution(request);
return executeListEnvironmentActions(request);
}
@SdkInternalApi
final ListEnvironmentActionsResult executeListEnvironmentActions(ListEnvironmentActionsRequest listEnvironmentActionsRequest) {
ExecutionContext executionContext = createExecutionContext(listEnvironmentActionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListEnvironmentActionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listEnvironmentActionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListEnvironmentActions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListEnvironmentActionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists blueprint configurations for a Amazon DataZone environment.
*
*
* @param listEnvironmentBlueprintConfigurationsRequest
* @return Result of the ListEnvironmentBlueprintConfigurations operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListEnvironmentBlueprintConfigurations
* @see AWS API Documentation
*/
@Override
public ListEnvironmentBlueprintConfigurationsResult listEnvironmentBlueprintConfigurations(ListEnvironmentBlueprintConfigurationsRequest request) {
request = beforeClientExecution(request);
return executeListEnvironmentBlueprintConfigurations(request);
}
@SdkInternalApi
final ListEnvironmentBlueprintConfigurationsResult executeListEnvironmentBlueprintConfigurations(
ListEnvironmentBlueprintConfigurationsRequest listEnvironmentBlueprintConfigurationsRequest) {
ExecutionContext executionContext = createExecutionContext(listEnvironmentBlueprintConfigurationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListEnvironmentBlueprintConfigurationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listEnvironmentBlueprintConfigurationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListEnvironmentBlueprintConfigurations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListEnvironmentBlueprintConfigurationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists blueprints in an Amazon DataZone environment.
*
*
* @param listEnvironmentBlueprintsRequest
* @return Result of the ListEnvironmentBlueprints operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListEnvironmentBlueprints
* @see AWS API Documentation
*/
@Override
public ListEnvironmentBlueprintsResult listEnvironmentBlueprints(ListEnvironmentBlueprintsRequest request) {
request = beforeClientExecution(request);
return executeListEnvironmentBlueprints(request);
}
@SdkInternalApi
final ListEnvironmentBlueprintsResult executeListEnvironmentBlueprints(ListEnvironmentBlueprintsRequest listEnvironmentBlueprintsRequest) {
ExecutionContext executionContext = createExecutionContext(listEnvironmentBlueprintsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListEnvironmentBlueprintsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listEnvironmentBlueprintsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListEnvironmentBlueprints");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListEnvironmentBlueprintsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists Amazon DataZone environment profiles.
*
*
* @param listEnvironmentProfilesRequest
* @return Result of the ListEnvironmentProfiles operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListEnvironmentProfiles
* @see AWS API Documentation
*/
@Override
public ListEnvironmentProfilesResult listEnvironmentProfiles(ListEnvironmentProfilesRequest request) {
request = beforeClientExecution(request);
return executeListEnvironmentProfiles(request);
}
@SdkInternalApi
final ListEnvironmentProfilesResult executeListEnvironmentProfiles(ListEnvironmentProfilesRequest listEnvironmentProfilesRequest) {
ExecutionContext executionContext = createExecutionContext(listEnvironmentProfilesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListEnvironmentProfilesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listEnvironmentProfilesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListEnvironmentProfiles");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListEnvironmentProfilesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists Amazon DataZone environments.
*
*
* @param listEnvironmentsRequest
* @return Result of the ListEnvironments operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListEnvironments
* @see AWS API
* Documentation
*/
@Override
public ListEnvironmentsResult listEnvironments(ListEnvironmentsRequest request) {
request = beforeClientExecution(request);
return executeListEnvironments(request);
}
@SdkInternalApi
final ListEnvironmentsResult executeListEnvironments(ListEnvironmentsRequest listEnvironmentsRequest) {
ExecutionContext executionContext = createExecutionContext(listEnvironmentsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListEnvironmentsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listEnvironmentsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "DataZone");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListEnvironments");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListEnvironmentsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the history of the specified data lineage node.
*
*
* @param listLineageNodeHistoryRequest
* @return Result of the ListLineageNodeHistory operation returned by the service.
* @throws InternalServerException
* The request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The specified resource cannot be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the Amazon Web Services service.
* @throws UnauthorizedException
* You do not have permission to perform this action.
* @sample AmazonDataZone.ListLineageNodeHistory
* @see AWS API Documentation
*/
@Override
public ListLineageNodeHistoryResult listLineageNodeHistory(ListLineageNodeHistoryRequest request) {
request = beforeClientExecution(request);
return executeListLineageNodeHistory(request);
}
@SdkInternalApi
final ListLineageNodeHistoryResult executeListLineageNodeHistory(ListLineageNodeHistoryRequest listLineageNodeHistoryRequest) {
ExecutionContext executionContext = createExecutionContext(listLineageNodeHistoryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response