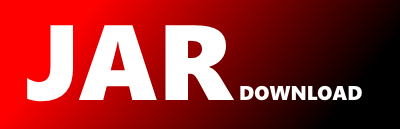
com.amazonaws.services.datazone.model.GetDataSourceResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-datazoneexternal Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.datazone.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetDataSourceResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The metadata forms attached to the assets created by this data source.
*
*/
private java.util.List assetFormsOutput;
/**
*
* The configuration of the data source.
*
*/
private DataSourceConfigurationOutput configuration;
/**
*
* The timestamp of when the data source was created.
*
*/
private java.util.Date createdAt;
/**
*
* The description of the data source.
*
*/
private String description;
/**
*
* The ID of the Amazon DataZone domain in which the data source exists.
*
*/
private String domainId;
/**
*
* Specifies whether this data source is enabled or not.
*
*/
private String enableSetting;
/**
*
* The ID of the environment where this data source creates and publishes assets,
*
*/
private String environmentId;
/**
*
* Specifies the error message that is returned if the operation cannot be successfully completed.
*
*/
private DataSourceErrorMessage errorMessage;
/**
*
* The ID of the data source.
*
*/
private String id;
/**
*
* The number of assets created by the data source during its last run.
*
*/
private Integer lastRunAssetCount;
/**
*
* The timestamp of the last run of the data source.
*
*/
private java.util.Date lastRunAt;
/**
*
* Specifies the error message that is returned if the operation cannot be successfully completed.
*
*/
private DataSourceErrorMessage lastRunErrorMessage;
/**
*
* The status of the last run of the data source.
*
*/
private String lastRunStatus;
/**
*
* The name of the data source.
*
*/
private String name;
/**
*
* The ID of the project where the data source creates and publishes assets.
*
*/
private String projectId;
/**
*
* Specifies whether the assets that this data source creates in the inventory are to be also automatically
* published to the catalog.
*
*/
private Boolean publishOnImport;
/**
*
* The recommendation configuration of the data source.
*
*/
private RecommendationConfiguration recommendation;
/**
*
* The schedule of the data source runs.
*
*/
private ScheduleConfiguration schedule;
/**
*
* Specifies the status of the self-granting functionality.
*
*/
private SelfGrantStatusOutput selfGrantStatus;
/**
*
* The status of the data source.
*
*/
private String status;
/**
*
* The type of the data source.
*
*/
private String type;
/**
*
* The timestamp of when the data source was updated.
*
*/
private java.util.Date updatedAt;
/**
*
* The metadata forms attached to the assets created by this data source.
*
*
* @return The metadata forms attached to the assets created by this data source.
*/
public java.util.List getAssetFormsOutput() {
return assetFormsOutput;
}
/**
*
* The metadata forms attached to the assets created by this data source.
*
*
* @param assetFormsOutput
* The metadata forms attached to the assets created by this data source.
*/
public void setAssetFormsOutput(java.util.Collection assetFormsOutput) {
if (assetFormsOutput == null) {
this.assetFormsOutput = null;
return;
}
this.assetFormsOutput = new java.util.ArrayList(assetFormsOutput);
}
/**
*
* The metadata forms attached to the assets created by this data source.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAssetFormsOutput(java.util.Collection)} or {@link #withAssetFormsOutput(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param assetFormsOutput
* The metadata forms attached to the assets created by this data source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDataSourceResult withAssetFormsOutput(FormOutput... assetFormsOutput) {
if (this.assetFormsOutput == null) {
setAssetFormsOutput(new java.util.ArrayList(assetFormsOutput.length));
}
for (FormOutput ele : assetFormsOutput) {
this.assetFormsOutput.add(ele);
}
return this;
}
/**
*
* The metadata forms attached to the assets created by this data source.
*
*
* @param assetFormsOutput
* The metadata forms attached to the assets created by this data source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDataSourceResult withAssetFormsOutput(java.util.Collection assetFormsOutput) {
setAssetFormsOutput(assetFormsOutput);
return this;
}
/**
*
* The configuration of the data source.
*
*
* @param configuration
* The configuration of the data source.
*/
public void setConfiguration(DataSourceConfigurationOutput configuration) {
this.configuration = configuration;
}
/**
*
* The configuration of the data source.
*
*
* @return The configuration of the data source.
*/
public DataSourceConfigurationOutput getConfiguration() {
return this.configuration;
}
/**
*
* The configuration of the data source.
*
*
* @param configuration
* The configuration of the data source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDataSourceResult withConfiguration(DataSourceConfigurationOutput configuration) {
setConfiguration(configuration);
return this;
}
/**
*
* The timestamp of when the data source was created.
*
*
* @param createdAt
* The timestamp of when the data source was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The timestamp of when the data source was created.
*
*
* @return The timestamp of when the data source was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The timestamp of when the data source was created.
*
*
* @param createdAt
* The timestamp of when the data source was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDataSourceResult withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The description of the data source.
*
*
* @param description
* The description of the data source.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The description of the data source.
*
*
* @return The description of the data source.
*/
public String getDescription() {
return this.description;
}
/**
*
* The description of the data source.
*
*
* @param description
* The description of the data source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDataSourceResult withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The ID of the Amazon DataZone domain in which the data source exists.
*
*
* @param domainId
* The ID of the Amazon DataZone domain in which the data source exists.
*/
public void setDomainId(String domainId) {
this.domainId = domainId;
}
/**
*
* The ID of the Amazon DataZone domain in which the data source exists.
*
*
* @return The ID of the Amazon DataZone domain in which the data source exists.
*/
public String getDomainId() {
return this.domainId;
}
/**
*
* The ID of the Amazon DataZone domain in which the data source exists.
*
*
* @param domainId
* The ID of the Amazon DataZone domain in which the data source exists.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDataSourceResult withDomainId(String domainId) {
setDomainId(domainId);
return this;
}
/**
*
* Specifies whether this data source is enabled or not.
*
*
* @param enableSetting
* Specifies whether this data source is enabled or not.
* @see EnableSetting
*/
public void setEnableSetting(String enableSetting) {
this.enableSetting = enableSetting;
}
/**
*
* Specifies whether this data source is enabled or not.
*
*
* @return Specifies whether this data source is enabled or not.
* @see EnableSetting
*/
public String getEnableSetting() {
return this.enableSetting;
}
/**
*
* Specifies whether this data source is enabled or not.
*
*
* @param enableSetting
* Specifies whether this data source is enabled or not.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnableSetting
*/
public GetDataSourceResult withEnableSetting(String enableSetting) {
setEnableSetting(enableSetting);
return this;
}
/**
*
* Specifies whether this data source is enabled or not.
*
*
* @param enableSetting
* Specifies whether this data source is enabled or not.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnableSetting
*/
public GetDataSourceResult withEnableSetting(EnableSetting enableSetting) {
this.enableSetting = enableSetting.toString();
return this;
}
/**
*
* The ID of the environment where this data source creates and publishes assets,
*
*
* @param environmentId
* The ID of the environment where this data source creates and publishes assets,
*/
public void setEnvironmentId(String environmentId) {
this.environmentId = environmentId;
}
/**
*
* The ID of the environment where this data source creates and publishes assets,
*
*
* @return The ID of the environment where this data source creates and publishes assets,
*/
public String getEnvironmentId() {
return this.environmentId;
}
/**
*
* The ID of the environment where this data source creates and publishes assets,
*
*
* @param environmentId
* The ID of the environment where this data source creates and publishes assets,
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDataSourceResult withEnvironmentId(String environmentId) {
setEnvironmentId(environmentId);
return this;
}
/**
*
* Specifies the error message that is returned if the operation cannot be successfully completed.
*
*
* @param errorMessage
* Specifies the error message that is returned if the operation cannot be successfully completed.
*/
public void setErrorMessage(DataSourceErrorMessage errorMessage) {
this.errorMessage = errorMessage;
}
/**
*
* Specifies the error message that is returned if the operation cannot be successfully completed.
*
*
* @return Specifies the error message that is returned if the operation cannot be successfully completed.
*/
public DataSourceErrorMessage getErrorMessage() {
return this.errorMessage;
}
/**
*
* Specifies the error message that is returned if the operation cannot be successfully completed.
*
*
* @param errorMessage
* Specifies the error message that is returned if the operation cannot be successfully completed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDataSourceResult withErrorMessage(DataSourceErrorMessage errorMessage) {
setErrorMessage(errorMessage);
return this;
}
/**
*
* The ID of the data source.
*
*
* @param id
* The ID of the data source.
*/
public void setId(String id) {
this.id = id;
}
/**
*
* The ID of the data source.
*
*
* @return The ID of the data source.
*/
public String getId() {
return this.id;
}
/**
*
* The ID of the data source.
*
*
* @param id
* The ID of the data source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDataSourceResult withId(String id) {
setId(id);
return this;
}
/**
*
* The number of assets created by the data source during its last run.
*
*
* @param lastRunAssetCount
* The number of assets created by the data source during its last run.
*/
public void setLastRunAssetCount(Integer lastRunAssetCount) {
this.lastRunAssetCount = lastRunAssetCount;
}
/**
*
* The number of assets created by the data source during its last run.
*
*
* @return The number of assets created by the data source during its last run.
*/
public Integer getLastRunAssetCount() {
return this.lastRunAssetCount;
}
/**
*
* The number of assets created by the data source during its last run.
*
*
* @param lastRunAssetCount
* The number of assets created by the data source during its last run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDataSourceResult withLastRunAssetCount(Integer lastRunAssetCount) {
setLastRunAssetCount(lastRunAssetCount);
return this;
}
/**
*
* The timestamp of the last run of the data source.
*
*
* @param lastRunAt
* The timestamp of the last run of the data source.
*/
public void setLastRunAt(java.util.Date lastRunAt) {
this.lastRunAt = lastRunAt;
}
/**
*
* The timestamp of the last run of the data source.
*
*
* @return The timestamp of the last run of the data source.
*/
public java.util.Date getLastRunAt() {
return this.lastRunAt;
}
/**
*
* The timestamp of the last run of the data source.
*
*
* @param lastRunAt
* The timestamp of the last run of the data source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDataSourceResult withLastRunAt(java.util.Date lastRunAt) {
setLastRunAt(lastRunAt);
return this;
}
/**
*
* Specifies the error message that is returned if the operation cannot be successfully completed.
*
*
* @param lastRunErrorMessage
* Specifies the error message that is returned if the operation cannot be successfully completed.
*/
public void setLastRunErrorMessage(DataSourceErrorMessage lastRunErrorMessage) {
this.lastRunErrorMessage = lastRunErrorMessage;
}
/**
*
* Specifies the error message that is returned if the operation cannot be successfully completed.
*
*
* @return Specifies the error message that is returned if the operation cannot be successfully completed.
*/
public DataSourceErrorMessage getLastRunErrorMessage() {
return this.lastRunErrorMessage;
}
/**
*
* Specifies the error message that is returned if the operation cannot be successfully completed.
*
*
* @param lastRunErrorMessage
* Specifies the error message that is returned if the operation cannot be successfully completed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDataSourceResult withLastRunErrorMessage(DataSourceErrorMessage lastRunErrorMessage) {
setLastRunErrorMessage(lastRunErrorMessage);
return this;
}
/**
*
* The status of the last run of the data source.
*
*
* @param lastRunStatus
* The status of the last run of the data source.
* @see DataSourceRunStatus
*/
public void setLastRunStatus(String lastRunStatus) {
this.lastRunStatus = lastRunStatus;
}
/**
*
* The status of the last run of the data source.
*
*
* @return The status of the last run of the data source.
* @see DataSourceRunStatus
*/
public String getLastRunStatus() {
return this.lastRunStatus;
}
/**
*
* The status of the last run of the data source.
*
*
* @param lastRunStatus
* The status of the last run of the data source.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataSourceRunStatus
*/
public GetDataSourceResult withLastRunStatus(String lastRunStatus) {
setLastRunStatus(lastRunStatus);
return this;
}
/**
*
* The status of the last run of the data source.
*
*
* @param lastRunStatus
* The status of the last run of the data source.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataSourceRunStatus
*/
public GetDataSourceResult withLastRunStatus(DataSourceRunStatus lastRunStatus) {
this.lastRunStatus = lastRunStatus.toString();
return this;
}
/**
*
* The name of the data source.
*
*
* @param name
* The name of the data source.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the data source.
*
*
* @return The name of the data source.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the data source.
*
*
* @param name
* The name of the data source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDataSourceResult withName(String name) {
setName(name);
return this;
}
/**
*
* The ID of the project where the data source creates and publishes assets.
*
*
* @param projectId
* The ID of the project where the data source creates and publishes assets.
*/
public void setProjectId(String projectId) {
this.projectId = projectId;
}
/**
*
* The ID of the project where the data source creates and publishes assets.
*
*
* @return The ID of the project where the data source creates and publishes assets.
*/
public String getProjectId() {
return this.projectId;
}
/**
*
* The ID of the project where the data source creates and publishes assets.
*
*
* @param projectId
* The ID of the project where the data source creates and publishes assets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDataSourceResult withProjectId(String projectId) {
setProjectId(projectId);
return this;
}
/**
*
* Specifies whether the assets that this data source creates in the inventory are to be also automatically
* published to the catalog.
*
*
* @param publishOnImport
* Specifies whether the assets that this data source creates in the inventory are to be also automatically
* published to the catalog.
*/
public void setPublishOnImport(Boolean publishOnImport) {
this.publishOnImport = publishOnImport;
}
/**
*
* Specifies whether the assets that this data source creates in the inventory are to be also automatically
* published to the catalog.
*
*
* @return Specifies whether the assets that this data source creates in the inventory are to be also automatically
* published to the catalog.
*/
public Boolean getPublishOnImport() {
return this.publishOnImport;
}
/**
*
* Specifies whether the assets that this data source creates in the inventory are to be also automatically
* published to the catalog.
*
*
* @param publishOnImport
* Specifies whether the assets that this data source creates in the inventory are to be also automatically
* published to the catalog.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDataSourceResult withPublishOnImport(Boolean publishOnImport) {
setPublishOnImport(publishOnImport);
return this;
}
/**
*
* Specifies whether the assets that this data source creates in the inventory are to be also automatically
* published to the catalog.
*
*
* @return Specifies whether the assets that this data source creates in the inventory are to be also automatically
* published to the catalog.
*/
public Boolean isPublishOnImport() {
return this.publishOnImport;
}
/**
*
* The recommendation configuration of the data source.
*
*
* @param recommendation
* The recommendation configuration of the data source.
*/
public void setRecommendation(RecommendationConfiguration recommendation) {
this.recommendation = recommendation;
}
/**
*
* The recommendation configuration of the data source.
*
*
* @return The recommendation configuration of the data source.
*/
public RecommendationConfiguration getRecommendation() {
return this.recommendation;
}
/**
*
* The recommendation configuration of the data source.
*
*
* @param recommendation
* The recommendation configuration of the data source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDataSourceResult withRecommendation(RecommendationConfiguration recommendation) {
setRecommendation(recommendation);
return this;
}
/**
*
* The schedule of the data source runs.
*
*
* @param schedule
* The schedule of the data source runs.
*/
public void setSchedule(ScheduleConfiguration schedule) {
this.schedule = schedule;
}
/**
*
* The schedule of the data source runs.
*
*
* @return The schedule of the data source runs.
*/
public ScheduleConfiguration getSchedule() {
return this.schedule;
}
/**
*
* The schedule of the data source runs.
*
*
* @param schedule
* The schedule of the data source runs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDataSourceResult withSchedule(ScheduleConfiguration schedule) {
setSchedule(schedule);
return this;
}
/**
*
* Specifies the status of the self-granting functionality.
*
*
* @param selfGrantStatus
* Specifies the status of the self-granting functionality.
*/
public void setSelfGrantStatus(SelfGrantStatusOutput selfGrantStatus) {
this.selfGrantStatus = selfGrantStatus;
}
/**
*
* Specifies the status of the self-granting functionality.
*
*
* @return Specifies the status of the self-granting functionality.
*/
public SelfGrantStatusOutput getSelfGrantStatus() {
return this.selfGrantStatus;
}
/**
*
* Specifies the status of the self-granting functionality.
*
*
* @param selfGrantStatus
* Specifies the status of the self-granting functionality.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDataSourceResult withSelfGrantStatus(SelfGrantStatusOutput selfGrantStatus) {
setSelfGrantStatus(selfGrantStatus);
return this;
}
/**
*
* The status of the data source.
*
*
* @param status
* The status of the data source.
* @see DataSourceStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the data source.
*
*
* @return The status of the data source.
* @see DataSourceStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the data source.
*
*
* @param status
* The status of the data source.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataSourceStatus
*/
public GetDataSourceResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of the data source.
*
*
* @param status
* The status of the data source.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataSourceStatus
*/
public GetDataSourceResult withStatus(DataSourceStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The type of the data source.
*
*
* @param type
* The type of the data source.
*/
public void setType(String type) {
this.type = type;
}
/**
*
* The type of the data source.
*
*
* @return The type of the data source.
*/
public String getType() {
return this.type;
}
/**
*
* The type of the data source.
*
*
* @param type
* The type of the data source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDataSourceResult withType(String type) {
setType(type);
return this;
}
/**
*
* The timestamp of when the data source was updated.
*
*
* @param updatedAt
* The timestamp of when the data source was updated.
*/
public void setUpdatedAt(java.util.Date updatedAt) {
this.updatedAt = updatedAt;
}
/**
*
* The timestamp of when the data source was updated.
*
*
* @return The timestamp of when the data source was updated.
*/
public java.util.Date getUpdatedAt() {
return this.updatedAt;
}
/**
*
* The timestamp of when the data source was updated.
*
*
* @param updatedAt
* The timestamp of when the data source was updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDataSourceResult withUpdatedAt(java.util.Date updatedAt) {
setUpdatedAt(updatedAt);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAssetFormsOutput() != null)
sb.append("AssetFormsOutput: ").append(getAssetFormsOutput()).append(",");
if (getConfiguration() != null)
sb.append("Configuration: ").append(getConfiguration()).append(",");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getDescription() != null)
sb.append("Description: ").append("***Sensitive Data Redacted***").append(",");
if (getDomainId() != null)
sb.append("DomainId: ").append(getDomainId()).append(",");
if (getEnableSetting() != null)
sb.append("EnableSetting: ").append(getEnableSetting()).append(",");
if (getEnvironmentId() != null)
sb.append("EnvironmentId: ").append(getEnvironmentId()).append(",");
if (getErrorMessage() != null)
sb.append("ErrorMessage: ").append(getErrorMessage()).append(",");
if (getId() != null)
sb.append("Id: ").append(getId()).append(",");
if (getLastRunAssetCount() != null)
sb.append("LastRunAssetCount: ").append(getLastRunAssetCount()).append(",");
if (getLastRunAt() != null)
sb.append("LastRunAt: ").append(getLastRunAt()).append(",");
if (getLastRunErrorMessage() != null)
sb.append("LastRunErrorMessage: ").append(getLastRunErrorMessage()).append(",");
if (getLastRunStatus() != null)
sb.append("LastRunStatus: ").append(getLastRunStatus()).append(",");
if (getName() != null)
sb.append("Name: ").append("***Sensitive Data Redacted***").append(",");
if (getProjectId() != null)
sb.append("ProjectId: ").append(getProjectId()).append(",");
if (getPublishOnImport() != null)
sb.append("PublishOnImport: ").append(getPublishOnImport()).append(",");
if (getRecommendation() != null)
sb.append("Recommendation: ").append(getRecommendation()).append(",");
if (getSchedule() != null)
sb.append("Schedule: ").append("***Sensitive Data Redacted***").append(",");
if (getSelfGrantStatus() != null)
sb.append("SelfGrantStatus: ").append(getSelfGrantStatus()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getType() != null)
sb.append("Type: ").append(getType()).append(",");
if (getUpdatedAt() != null)
sb.append("UpdatedAt: ").append(getUpdatedAt());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetDataSourceResult == false)
return false;
GetDataSourceResult other = (GetDataSourceResult) obj;
if (other.getAssetFormsOutput() == null ^ this.getAssetFormsOutput() == null)
return false;
if (other.getAssetFormsOutput() != null && other.getAssetFormsOutput().equals(this.getAssetFormsOutput()) == false)
return false;
if (other.getConfiguration() == null ^ this.getConfiguration() == null)
return false;
if (other.getConfiguration() != null && other.getConfiguration().equals(this.getConfiguration()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getDomainId() == null ^ this.getDomainId() == null)
return false;
if (other.getDomainId() != null && other.getDomainId().equals(this.getDomainId()) == false)
return false;
if (other.getEnableSetting() == null ^ this.getEnableSetting() == null)
return false;
if (other.getEnableSetting() != null && other.getEnableSetting().equals(this.getEnableSetting()) == false)
return false;
if (other.getEnvironmentId() == null ^ this.getEnvironmentId() == null)
return false;
if (other.getEnvironmentId() != null && other.getEnvironmentId().equals(this.getEnvironmentId()) == false)
return false;
if (other.getErrorMessage() == null ^ this.getErrorMessage() == null)
return false;
if (other.getErrorMessage() != null && other.getErrorMessage().equals(this.getErrorMessage()) == false)
return false;
if (other.getId() == null ^ this.getId() == null)
return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false)
return false;
if (other.getLastRunAssetCount() == null ^ this.getLastRunAssetCount() == null)
return false;
if (other.getLastRunAssetCount() != null && other.getLastRunAssetCount().equals(this.getLastRunAssetCount()) == false)
return false;
if (other.getLastRunAt() == null ^ this.getLastRunAt() == null)
return false;
if (other.getLastRunAt() != null && other.getLastRunAt().equals(this.getLastRunAt()) == false)
return false;
if (other.getLastRunErrorMessage() == null ^ this.getLastRunErrorMessage() == null)
return false;
if (other.getLastRunErrorMessage() != null && other.getLastRunErrorMessage().equals(this.getLastRunErrorMessage()) == false)
return false;
if (other.getLastRunStatus() == null ^ this.getLastRunStatus() == null)
return false;
if (other.getLastRunStatus() != null && other.getLastRunStatus().equals(this.getLastRunStatus()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getProjectId() == null ^ this.getProjectId() == null)
return false;
if (other.getProjectId() != null && other.getProjectId().equals(this.getProjectId()) == false)
return false;
if (other.getPublishOnImport() == null ^ this.getPublishOnImport() == null)
return false;
if (other.getPublishOnImport() != null && other.getPublishOnImport().equals(this.getPublishOnImport()) == false)
return false;
if (other.getRecommendation() == null ^ this.getRecommendation() == null)
return false;
if (other.getRecommendation() != null && other.getRecommendation().equals(this.getRecommendation()) == false)
return false;
if (other.getSchedule() == null ^ this.getSchedule() == null)
return false;
if (other.getSchedule() != null && other.getSchedule().equals(this.getSchedule()) == false)
return false;
if (other.getSelfGrantStatus() == null ^ this.getSelfGrantStatus() == null)
return false;
if (other.getSelfGrantStatus() != null && other.getSelfGrantStatus().equals(this.getSelfGrantStatus()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null && other.getType().equals(this.getType()) == false)
return false;
if (other.getUpdatedAt() == null ^ this.getUpdatedAt() == null)
return false;
if (other.getUpdatedAt() != null && other.getUpdatedAt().equals(this.getUpdatedAt()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAssetFormsOutput() == null) ? 0 : getAssetFormsOutput().hashCode());
hashCode = prime * hashCode + ((getConfiguration() == null) ? 0 : getConfiguration().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getDomainId() == null) ? 0 : getDomainId().hashCode());
hashCode = prime * hashCode + ((getEnableSetting() == null) ? 0 : getEnableSetting().hashCode());
hashCode = prime * hashCode + ((getEnvironmentId() == null) ? 0 : getEnvironmentId().hashCode());
hashCode = prime * hashCode + ((getErrorMessage() == null) ? 0 : getErrorMessage().hashCode());
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getLastRunAssetCount() == null) ? 0 : getLastRunAssetCount().hashCode());
hashCode = prime * hashCode + ((getLastRunAt() == null) ? 0 : getLastRunAt().hashCode());
hashCode = prime * hashCode + ((getLastRunErrorMessage() == null) ? 0 : getLastRunErrorMessage().hashCode());
hashCode = prime * hashCode + ((getLastRunStatus() == null) ? 0 : getLastRunStatus().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getProjectId() == null) ? 0 : getProjectId().hashCode());
hashCode = prime * hashCode + ((getPublishOnImport() == null) ? 0 : getPublishOnImport().hashCode());
hashCode = prime * hashCode + ((getRecommendation() == null) ? 0 : getRecommendation().hashCode());
hashCode = prime * hashCode + ((getSchedule() == null) ? 0 : getSchedule().hashCode());
hashCode = prime * hashCode + ((getSelfGrantStatus() == null) ? 0 : getSelfGrantStatus().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getType() == null) ? 0 : getType().hashCode());
hashCode = prime * hashCode + ((getUpdatedAt() == null) ? 0 : getUpdatedAt().hashCode());
return hashCode;
}
@Override
public GetDataSourceResult clone() {
try {
return (GetDataSourceResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}