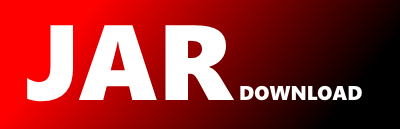
com.amazonaws.services.datazone.model.CreateAssetRevisionResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-datazoneexternal Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.datazone.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateAssetRevisionResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The timestamp of when the asset revision occured.
*
*/
private java.util.Date createdAt;
/**
*
* The Amazon DataZone user who performed the asset revision.
*
*/
private String createdBy;
/**
*
* The revised asset description.
*
*/
private String description;
/**
*
* The unique identifier of the Amazon DataZone domain where the asset was revised.
*
*/
private String domainId;
/**
*
* The external identifier of the asset.
*
*/
private String externalIdentifier;
/**
*
* The timestamp of when the first asset revision occured.
*
*/
private java.util.Date firstRevisionCreatedAt;
/**
*
* The Amazon DataZone user who performed the first asset revision.
*
*/
private String firstRevisionCreatedBy;
/**
*
* The metadata forms that were attached to the asset as part of the asset revision.
*
*/
private java.util.List formsOutput;
/**
*
* The glossary terms that were attached to the asset as part of asset revision.
*
*/
private java.util.List glossaryTerms;
/**
*
* The unique identifier of the asset revision.
*
*/
private String id;
/**
*
* The latest data point that was imported into the time series form for the asset.
*
*/
private java.util.List latestTimeSeriesDataPointFormsOutput;
/**
*
* The details of an asset published in an Amazon DataZone catalog.
*
*/
private AssetListingDetails listing;
/**
*
* The revised name of the asset.
*
*/
private String name;
/**
*
* The unique identifier of the revised project that owns the asset.
*
*/
private String owningProjectId;
/**
*
* The configuration of the automatically generated business-friendly metadata for the asset.
*
*/
private PredictionConfiguration predictionConfiguration;
/**
*
* The read-only metadata forms that were attached to the asset as part of the asset revision.
*
*/
private java.util.List readOnlyFormsOutput;
/**
*
* The revision of the asset.
*
*/
private String revision;
/**
*
* The identifier of the revision type.
*
*/
private String typeIdentifier;
/**
*
* The revision type of the asset.
*
*/
private String typeRevision;
/**
*
* The timestamp of when the asset revision occured.
*
*
* @param createdAt
* The timestamp of when the asset revision occured.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The timestamp of when the asset revision occured.
*
*
* @return The timestamp of when the asset revision occured.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The timestamp of when the asset revision occured.
*
*
* @param createdAt
* The timestamp of when the asset revision occured.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The Amazon DataZone user who performed the asset revision.
*
*
* @param createdBy
* The Amazon DataZone user who performed the asset revision.
*/
public void setCreatedBy(String createdBy) {
this.createdBy = createdBy;
}
/**
*
* The Amazon DataZone user who performed the asset revision.
*
*
* @return The Amazon DataZone user who performed the asset revision.
*/
public String getCreatedBy() {
return this.createdBy;
}
/**
*
* The Amazon DataZone user who performed the asset revision.
*
*
* @param createdBy
* The Amazon DataZone user who performed the asset revision.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withCreatedBy(String createdBy) {
setCreatedBy(createdBy);
return this;
}
/**
*
* The revised asset description.
*
*
* @param description
* The revised asset description.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The revised asset description.
*
*
* @return The revised asset description.
*/
public String getDescription() {
return this.description;
}
/**
*
* The revised asset description.
*
*
* @param description
* The revised asset description.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The unique identifier of the Amazon DataZone domain where the asset was revised.
*
*
* @param domainId
* The unique identifier of the Amazon DataZone domain where the asset was revised.
*/
public void setDomainId(String domainId) {
this.domainId = domainId;
}
/**
*
* The unique identifier of the Amazon DataZone domain where the asset was revised.
*
*
* @return The unique identifier of the Amazon DataZone domain where the asset was revised.
*/
public String getDomainId() {
return this.domainId;
}
/**
*
* The unique identifier of the Amazon DataZone domain where the asset was revised.
*
*
* @param domainId
* The unique identifier of the Amazon DataZone domain where the asset was revised.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withDomainId(String domainId) {
setDomainId(domainId);
return this;
}
/**
*
* The external identifier of the asset.
*
*
* @param externalIdentifier
* The external identifier of the asset.
*/
public void setExternalIdentifier(String externalIdentifier) {
this.externalIdentifier = externalIdentifier;
}
/**
*
* The external identifier of the asset.
*
*
* @return The external identifier of the asset.
*/
public String getExternalIdentifier() {
return this.externalIdentifier;
}
/**
*
* The external identifier of the asset.
*
*
* @param externalIdentifier
* The external identifier of the asset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withExternalIdentifier(String externalIdentifier) {
setExternalIdentifier(externalIdentifier);
return this;
}
/**
*
* The timestamp of when the first asset revision occured.
*
*
* @param firstRevisionCreatedAt
* The timestamp of when the first asset revision occured.
*/
public void setFirstRevisionCreatedAt(java.util.Date firstRevisionCreatedAt) {
this.firstRevisionCreatedAt = firstRevisionCreatedAt;
}
/**
*
* The timestamp of when the first asset revision occured.
*
*
* @return The timestamp of when the first asset revision occured.
*/
public java.util.Date getFirstRevisionCreatedAt() {
return this.firstRevisionCreatedAt;
}
/**
*
* The timestamp of when the first asset revision occured.
*
*
* @param firstRevisionCreatedAt
* The timestamp of when the first asset revision occured.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withFirstRevisionCreatedAt(java.util.Date firstRevisionCreatedAt) {
setFirstRevisionCreatedAt(firstRevisionCreatedAt);
return this;
}
/**
*
* The Amazon DataZone user who performed the first asset revision.
*
*
* @param firstRevisionCreatedBy
* The Amazon DataZone user who performed the first asset revision.
*/
public void setFirstRevisionCreatedBy(String firstRevisionCreatedBy) {
this.firstRevisionCreatedBy = firstRevisionCreatedBy;
}
/**
*
* The Amazon DataZone user who performed the first asset revision.
*
*
* @return The Amazon DataZone user who performed the first asset revision.
*/
public String getFirstRevisionCreatedBy() {
return this.firstRevisionCreatedBy;
}
/**
*
* The Amazon DataZone user who performed the first asset revision.
*
*
* @param firstRevisionCreatedBy
* The Amazon DataZone user who performed the first asset revision.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withFirstRevisionCreatedBy(String firstRevisionCreatedBy) {
setFirstRevisionCreatedBy(firstRevisionCreatedBy);
return this;
}
/**
*
* The metadata forms that were attached to the asset as part of the asset revision.
*
*
* @return The metadata forms that were attached to the asset as part of the asset revision.
*/
public java.util.List getFormsOutput() {
return formsOutput;
}
/**
*
* The metadata forms that were attached to the asset as part of the asset revision.
*
*
* @param formsOutput
* The metadata forms that were attached to the asset as part of the asset revision.
*/
public void setFormsOutput(java.util.Collection formsOutput) {
if (formsOutput == null) {
this.formsOutput = null;
return;
}
this.formsOutput = new java.util.ArrayList(formsOutput);
}
/**
*
* The metadata forms that were attached to the asset as part of the asset revision.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFormsOutput(java.util.Collection)} or {@link #withFormsOutput(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param formsOutput
* The metadata forms that were attached to the asset as part of the asset revision.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withFormsOutput(FormOutput... formsOutput) {
if (this.formsOutput == null) {
setFormsOutput(new java.util.ArrayList(formsOutput.length));
}
for (FormOutput ele : formsOutput) {
this.formsOutput.add(ele);
}
return this;
}
/**
*
* The metadata forms that were attached to the asset as part of the asset revision.
*
*
* @param formsOutput
* The metadata forms that were attached to the asset as part of the asset revision.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withFormsOutput(java.util.Collection formsOutput) {
setFormsOutput(formsOutput);
return this;
}
/**
*
* The glossary terms that were attached to the asset as part of asset revision.
*
*
* @return The glossary terms that were attached to the asset as part of asset revision.
*/
public java.util.List getGlossaryTerms() {
return glossaryTerms;
}
/**
*
* The glossary terms that were attached to the asset as part of asset revision.
*
*
* @param glossaryTerms
* The glossary terms that were attached to the asset as part of asset revision.
*/
public void setGlossaryTerms(java.util.Collection glossaryTerms) {
if (glossaryTerms == null) {
this.glossaryTerms = null;
return;
}
this.glossaryTerms = new java.util.ArrayList(glossaryTerms);
}
/**
*
* The glossary terms that were attached to the asset as part of asset revision.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setGlossaryTerms(java.util.Collection)} or {@link #withGlossaryTerms(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param glossaryTerms
* The glossary terms that were attached to the asset as part of asset revision.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withGlossaryTerms(String... glossaryTerms) {
if (this.glossaryTerms == null) {
setGlossaryTerms(new java.util.ArrayList(glossaryTerms.length));
}
for (String ele : glossaryTerms) {
this.glossaryTerms.add(ele);
}
return this;
}
/**
*
* The glossary terms that were attached to the asset as part of asset revision.
*
*
* @param glossaryTerms
* The glossary terms that were attached to the asset as part of asset revision.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withGlossaryTerms(java.util.Collection glossaryTerms) {
setGlossaryTerms(glossaryTerms);
return this;
}
/**
*
* The unique identifier of the asset revision.
*
*
* @param id
* The unique identifier of the asset revision.
*/
public void setId(String id) {
this.id = id;
}
/**
*
* The unique identifier of the asset revision.
*
*
* @return The unique identifier of the asset revision.
*/
public String getId() {
return this.id;
}
/**
*
* The unique identifier of the asset revision.
*
*
* @param id
* The unique identifier of the asset revision.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withId(String id) {
setId(id);
return this;
}
/**
*
* The latest data point that was imported into the time series form for the asset.
*
*
* @return The latest data point that was imported into the time series form for the asset.
*/
public java.util.List getLatestTimeSeriesDataPointFormsOutput() {
return latestTimeSeriesDataPointFormsOutput;
}
/**
*
* The latest data point that was imported into the time series form for the asset.
*
*
* @param latestTimeSeriesDataPointFormsOutput
* The latest data point that was imported into the time series form for the asset.
*/
public void setLatestTimeSeriesDataPointFormsOutput(java.util.Collection latestTimeSeriesDataPointFormsOutput) {
if (latestTimeSeriesDataPointFormsOutput == null) {
this.latestTimeSeriesDataPointFormsOutput = null;
return;
}
this.latestTimeSeriesDataPointFormsOutput = new java.util.ArrayList(latestTimeSeriesDataPointFormsOutput);
}
/**
*
* The latest data point that was imported into the time series form for the asset.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setLatestTimeSeriesDataPointFormsOutput(java.util.Collection)} or
* {@link #withLatestTimeSeriesDataPointFormsOutput(java.util.Collection)} if you want to override the existing
* values.
*
*
* @param latestTimeSeriesDataPointFormsOutput
* The latest data point that was imported into the time series form for the asset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withLatestTimeSeriesDataPointFormsOutput(TimeSeriesDataPointSummaryFormOutput... latestTimeSeriesDataPointFormsOutput) {
if (this.latestTimeSeriesDataPointFormsOutput == null) {
setLatestTimeSeriesDataPointFormsOutput(new java.util.ArrayList(latestTimeSeriesDataPointFormsOutput.length));
}
for (TimeSeriesDataPointSummaryFormOutput ele : latestTimeSeriesDataPointFormsOutput) {
this.latestTimeSeriesDataPointFormsOutput.add(ele);
}
return this;
}
/**
*
* The latest data point that was imported into the time series form for the asset.
*
*
* @param latestTimeSeriesDataPointFormsOutput
* The latest data point that was imported into the time series form for the asset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withLatestTimeSeriesDataPointFormsOutput(
java.util.Collection latestTimeSeriesDataPointFormsOutput) {
setLatestTimeSeriesDataPointFormsOutput(latestTimeSeriesDataPointFormsOutput);
return this;
}
/**
*
* The details of an asset published in an Amazon DataZone catalog.
*
*
* @param listing
* The details of an asset published in an Amazon DataZone catalog.
*/
public void setListing(AssetListingDetails listing) {
this.listing = listing;
}
/**
*
* The details of an asset published in an Amazon DataZone catalog.
*
*
* @return The details of an asset published in an Amazon DataZone catalog.
*/
public AssetListingDetails getListing() {
return this.listing;
}
/**
*
* The details of an asset published in an Amazon DataZone catalog.
*
*
* @param listing
* The details of an asset published in an Amazon DataZone catalog.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withListing(AssetListingDetails listing) {
setListing(listing);
return this;
}
/**
*
* The revised name of the asset.
*
*
* @param name
* The revised name of the asset.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The revised name of the asset.
*
*
* @return The revised name of the asset.
*/
public String getName() {
return this.name;
}
/**
*
* The revised name of the asset.
*
*
* @param name
* The revised name of the asset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withName(String name) {
setName(name);
return this;
}
/**
*
* The unique identifier of the revised project that owns the asset.
*
*
* @param owningProjectId
* The unique identifier of the revised project that owns the asset.
*/
public void setOwningProjectId(String owningProjectId) {
this.owningProjectId = owningProjectId;
}
/**
*
* The unique identifier of the revised project that owns the asset.
*
*
* @return The unique identifier of the revised project that owns the asset.
*/
public String getOwningProjectId() {
return this.owningProjectId;
}
/**
*
* The unique identifier of the revised project that owns the asset.
*
*
* @param owningProjectId
* The unique identifier of the revised project that owns the asset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withOwningProjectId(String owningProjectId) {
setOwningProjectId(owningProjectId);
return this;
}
/**
*
* The configuration of the automatically generated business-friendly metadata for the asset.
*
*
* @param predictionConfiguration
* The configuration of the automatically generated business-friendly metadata for the asset.
*/
public void setPredictionConfiguration(PredictionConfiguration predictionConfiguration) {
this.predictionConfiguration = predictionConfiguration;
}
/**
*
* The configuration of the automatically generated business-friendly metadata for the asset.
*
*
* @return The configuration of the automatically generated business-friendly metadata for the asset.
*/
public PredictionConfiguration getPredictionConfiguration() {
return this.predictionConfiguration;
}
/**
*
* The configuration of the automatically generated business-friendly metadata for the asset.
*
*
* @param predictionConfiguration
* The configuration of the automatically generated business-friendly metadata for the asset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withPredictionConfiguration(PredictionConfiguration predictionConfiguration) {
setPredictionConfiguration(predictionConfiguration);
return this;
}
/**
*
* The read-only metadata forms that were attached to the asset as part of the asset revision.
*
*
* @return The read-only metadata forms that were attached to the asset as part of the asset revision.
*/
public java.util.List getReadOnlyFormsOutput() {
return readOnlyFormsOutput;
}
/**
*
* The read-only metadata forms that were attached to the asset as part of the asset revision.
*
*
* @param readOnlyFormsOutput
* The read-only metadata forms that were attached to the asset as part of the asset revision.
*/
public void setReadOnlyFormsOutput(java.util.Collection readOnlyFormsOutput) {
if (readOnlyFormsOutput == null) {
this.readOnlyFormsOutput = null;
return;
}
this.readOnlyFormsOutput = new java.util.ArrayList(readOnlyFormsOutput);
}
/**
*
* The read-only metadata forms that were attached to the asset as part of the asset revision.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setReadOnlyFormsOutput(java.util.Collection)} or {@link #withReadOnlyFormsOutput(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param readOnlyFormsOutput
* The read-only metadata forms that were attached to the asset as part of the asset revision.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withReadOnlyFormsOutput(FormOutput... readOnlyFormsOutput) {
if (this.readOnlyFormsOutput == null) {
setReadOnlyFormsOutput(new java.util.ArrayList(readOnlyFormsOutput.length));
}
for (FormOutput ele : readOnlyFormsOutput) {
this.readOnlyFormsOutput.add(ele);
}
return this;
}
/**
*
* The read-only metadata forms that were attached to the asset as part of the asset revision.
*
*
* @param readOnlyFormsOutput
* The read-only metadata forms that were attached to the asset as part of the asset revision.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withReadOnlyFormsOutput(java.util.Collection readOnlyFormsOutput) {
setReadOnlyFormsOutput(readOnlyFormsOutput);
return this;
}
/**
*
* The revision of the asset.
*
*
* @param revision
* The revision of the asset.
*/
public void setRevision(String revision) {
this.revision = revision;
}
/**
*
* The revision of the asset.
*
*
* @return The revision of the asset.
*/
public String getRevision() {
return this.revision;
}
/**
*
* The revision of the asset.
*
*
* @param revision
* The revision of the asset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withRevision(String revision) {
setRevision(revision);
return this;
}
/**
*
* The identifier of the revision type.
*
*
* @param typeIdentifier
* The identifier of the revision type.
*/
public void setTypeIdentifier(String typeIdentifier) {
this.typeIdentifier = typeIdentifier;
}
/**
*
* The identifier of the revision type.
*
*
* @return The identifier of the revision type.
*/
public String getTypeIdentifier() {
return this.typeIdentifier;
}
/**
*
* The identifier of the revision type.
*
*
* @param typeIdentifier
* The identifier of the revision type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withTypeIdentifier(String typeIdentifier) {
setTypeIdentifier(typeIdentifier);
return this;
}
/**
*
* The revision type of the asset.
*
*
* @param typeRevision
* The revision type of the asset.
*/
public void setTypeRevision(String typeRevision) {
this.typeRevision = typeRevision;
}
/**
*
* The revision type of the asset.
*
*
* @return The revision type of the asset.
*/
public String getTypeRevision() {
return this.typeRevision;
}
/**
*
* The revision type of the asset.
*
*
* @param typeRevision
* The revision type of the asset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAssetRevisionResult withTypeRevision(String typeRevision) {
setTypeRevision(typeRevision);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getCreatedBy() != null)
sb.append("CreatedBy: ").append(getCreatedBy()).append(",");
if (getDescription() != null)
sb.append("Description: ").append("***Sensitive Data Redacted***").append(",");
if (getDomainId() != null)
sb.append("DomainId: ").append(getDomainId()).append(",");
if (getExternalIdentifier() != null)
sb.append("ExternalIdentifier: ").append("***Sensitive Data Redacted***").append(",");
if (getFirstRevisionCreatedAt() != null)
sb.append("FirstRevisionCreatedAt: ").append(getFirstRevisionCreatedAt()).append(",");
if (getFirstRevisionCreatedBy() != null)
sb.append("FirstRevisionCreatedBy: ").append(getFirstRevisionCreatedBy()).append(",");
if (getFormsOutput() != null)
sb.append("FormsOutput: ").append(getFormsOutput()).append(",");
if (getGlossaryTerms() != null)
sb.append("GlossaryTerms: ").append(getGlossaryTerms()).append(",");
if (getId() != null)
sb.append("Id: ").append(getId()).append(",");
if (getLatestTimeSeriesDataPointFormsOutput() != null)
sb.append("LatestTimeSeriesDataPointFormsOutput: ").append(getLatestTimeSeriesDataPointFormsOutput()).append(",");
if (getListing() != null)
sb.append("Listing: ").append(getListing()).append(",");
if (getName() != null)
sb.append("Name: ").append("***Sensitive Data Redacted***").append(",");
if (getOwningProjectId() != null)
sb.append("OwningProjectId: ").append(getOwningProjectId()).append(",");
if (getPredictionConfiguration() != null)
sb.append("PredictionConfiguration: ").append(getPredictionConfiguration()).append(",");
if (getReadOnlyFormsOutput() != null)
sb.append("ReadOnlyFormsOutput: ").append(getReadOnlyFormsOutput()).append(",");
if (getRevision() != null)
sb.append("Revision: ").append(getRevision()).append(",");
if (getTypeIdentifier() != null)
sb.append("TypeIdentifier: ").append(getTypeIdentifier()).append(",");
if (getTypeRevision() != null)
sb.append("TypeRevision: ").append(getTypeRevision());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateAssetRevisionResult == false)
return false;
CreateAssetRevisionResult other = (CreateAssetRevisionResult) obj;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getCreatedBy() == null ^ this.getCreatedBy() == null)
return false;
if (other.getCreatedBy() != null && other.getCreatedBy().equals(this.getCreatedBy()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getDomainId() == null ^ this.getDomainId() == null)
return false;
if (other.getDomainId() != null && other.getDomainId().equals(this.getDomainId()) == false)
return false;
if (other.getExternalIdentifier() == null ^ this.getExternalIdentifier() == null)
return false;
if (other.getExternalIdentifier() != null && other.getExternalIdentifier().equals(this.getExternalIdentifier()) == false)
return false;
if (other.getFirstRevisionCreatedAt() == null ^ this.getFirstRevisionCreatedAt() == null)
return false;
if (other.getFirstRevisionCreatedAt() != null && other.getFirstRevisionCreatedAt().equals(this.getFirstRevisionCreatedAt()) == false)
return false;
if (other.getFirstRevisionCreatedBy() == null ^ this.getFirstRevisionCreatedBy() == null)
return false;
if (other.getFirstRevisionCreatedBy() != null && other.getFirstRevisionCreatedBy().equals(this.getFirstRevisionCreatedBy()) == false)
return false;
if (other.getFormsOutput() == null ^ this.getFormsOutput() == null)
return false;
if (other.getFormsOutput() != null && other.getFormsOutput().equals(this.getFormsOutput()) == false)
return false;
if (other.getGlossaryTerms() == null ^ this.getGlossaryTerms() == null)
return false;
if (other.getGlossaryTerms() != null && other.getGlossaryTerms().equals(this.getGlossaryTerms()) == false)
return false;
if (other.getId() == null ^ this.getId() == null)
return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false)
return false;
if (other.getLatestTimeSeriesDataPointFormsOutput() == null ^ this.getLatestTimeSeriesDataPointFormsOutput() == null)
return false;
if (other.getLatestTimeSeriesDataPointFormsOutput() != null
&& other.getLatestTimeSeriesDataPointFormsOutput().equals(this.getLatestTimeSeriesDataPointFormsOutput()) == false)
return false;
if (other.getListing() == null ^ this.getListing() == null)
return false;
if (other.getListing() != null && other.getListing().equals(this.getListing()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getOwningProjectId() == null ^ this.getOwningProjectId() == null)
return false;
if (other.getOwningProjectId() != null && other.getOwningProjectId().equals(this.getOwningProjectId()) == false)
return false;
if (other.getPredictionConfiguration() == null ^ this.getPredictionConfiguration() == null)
return false;
if (other.getPredictionConfiguration() != null && other.getPredictionConfiguration().equals(this.getPredictionConfiguration()) == false)
return false;
if (other.getReadOnlyFormsOutput() == null ^ this.getReadOnlyFormsOutput() == null)
return false;
if (other.getReadOnlyFormsOutput() != null && other.getReadOnlyFormsOutput().equals(this.getReadOnlyFormsOutput()) == false)
return false;
if (other.getRevision() == null ^ this.getRevision() == null)
return false;
if (other.getRevision() != null && other.getRevision().equals(this.getRevision()) == false)
return false;
if (other.getTypeIdentifier() == null ^ this.getTypeIdentifier() == null)
return false;
if (other.getTypeIdentifier() != null && other.getTypeIdentifier().equals(this.getTypeIdentifier()) == false)
return false;
if (other.getTypeRevision() == null ^ this.getTypeRevision() == null)
return false;
if (other.getTypeRevision() != null && other.getTypeRevision().equals(this.getTypeRevision()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getCreatedBy() == null) ? 0 : getCreatedBy().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getDomainId() == null) ? 0 : getDomainId().hashCode());
hashCode = prime * hashCode + ((getExternalIdentifier() == null) ? 0 : getExternalIdentifier().hashCode());
hashCode = prime * hashCode + ((getFirstRevisionCreatedAt() == null) ? 0 : getFirstRevisionCreatedAt().hashCode());
hashCode = prime * hashCode + ((getFirstRevisionCreatedBy() == null) ? 0 : getFirstRevisionCreatedBy().hashCode());
hashCode = prime * hashCode + ((getFormsOutput() == null) ? 0 : getFormsOutput().hashCode());
hashCode = prime * hashCode + ((getGlossaryTerms() == null) ? 0 : getGlossaryTerms().hashCode());
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getLatestTimeSeriesDataPointFormsOutput() == null) ? 0 : getLatestTimeSeriesDataPointFormsOutput().hashCode());
hashCode = prime * hashCode + ((getListing() == null) ? 0 : getListing().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getOwningProjectId() == null) ? 0 : getOwningProjectId().hashCode());
hashCode = prime * hashCode + ((getPredictionConfiguration() == null) ? 0 : getPredictionConfiguration().hashCode());
hashCode = prime * hashCode + ((getReadOnlyFormsOutput() == null) ? 0 : getReadOnlyFormsOutput().hashCode());
hashCode = prime * hashCode + ((getRevision() == null) ? 0 : getRevision().hashCode());
hashCode = prime * hashCode + ((getTypeIdentifier() == null) ? 0 : getTypeIdentifier().hashCode());
hashCode = prime * hashCode + ((getTypeRevision() == null) ? 0 : getTypeRevision().hashCode());
return hashCode;
}
@Override
public CreateAssetRevisionResult clone() {
try {
return (CreateAssetRevisionResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}