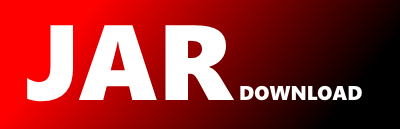
com.amazonaws.services.datazone.model.GetEnvironmentBlueprintConfigurationResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-datazoneexternal Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.datazone.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetEnvironmentBlueprintConfigurationResult extends com.amazonaws.AmazonWebServiceResult implements Serializable,
Cloneable {
/**
*
* The timestamp of when this blueprint was created.
*
*/
private java.util.Date createdAt;
/**
*
* The ID of the Amazon DataZone domain where this blueprint exists.
*
*/
private String domainId;
/**
*
* The Amazon Web Services regions in which this blueprint is enabled.
*
*/
private java.util.List enabledRegions;
/**
*
* The ID of the blueprint.
*
*/
private String environmentBlueprintId;
/**
*
* The ARN of the manage access role with which this blueprint is created.
*
*/
private String manageAccessRoleArn;
/**
*
* The provisioning configuration of a blueprint.
*
*/
private java.util.List provisioningConfigurations;
/**
*
* The ARN of the provisioning role with which this blueprint is created.
*
*/
private String provisioningRoleArn;
/**
*
* The regional parameters of the blueprint.
*
*/
private java.util.Map> regionalParameters;
/**
*
* The timestamp of when this blueprint was upated.
*
*/
private java.util.Date updatedAt;
/**
*
* The timestamp of when this blueprint was created.
*
*
* @param createdAt
* The timestamp of when this blueprint was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The timestamp of when this blueprint was created.
*
*
* @return The timestamp of when this blueprint was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The timestamp of when this blueprint was created.
*
*
* @param createdAt
* The timestamp of when this blueprint was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEnvironmentBlueprintConfigurationResult withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The ID of the Amazon DataZone domain where this blueprint exists.
*
*
* @param domainId
* The ID of the Amazon DataZone domain where this blueprint exists.
*/
public void setDomainId(String domainId) {
this.domainId = domainId;
}
/**
*
* The ID of the Amazon DataZone domain where this blueprint exists.
*
*
* @return The ID of the Amazon DataZone domain where this blueprint exists.
*/
public String getDomainId() {
return this.domainId;
}
/**
*
* The ID of the Amazon DataZone domain where this blueprint exists.
*
*
* @param domainId
* The ID of the Amazon DataZone domain where this blueprint exists.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEnvironmentBlueprintConfigurationResult withDomainId(String domainId) {
setDomainId(domainId);
return this;
}
/**
*
* The Amazon Web Services regions in which this blueprint is enabled.
*
*
* @return The Amazon Web Services regions in which this blueprint is enabled.
*/
public java.util.List getEnabledRegions() {
return enabledRegions;
}
/**
*
* The Amazon Web Services regions in which this blueprint is enabled.
*
*
* @param enabledRegions
* The Amazon Web Services regions in which this blueprint is enabled.
*/
public void setEnabledRegions(java.util.Collection enabledRegions) {
if (enabledRegions == null) {
this.enabledRegions = null;
return;
}
this.enabledRegions = new java.util.ArrayList(enabledRegions);
}
/**
*
* The Amazon Web Services regions in which this blueprint is enabled.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setEnabledRegions(java.util.Collection)} or {@link #withEnabledRegions(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param enabledRegions
* The Amazon Web Services regions in which this blueprint is enabled.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEnvironmentBlueprintConfigurationResult withEnabledRegions(String... enabledRegions) {
if (this.enabledRegions == null) {
setEnabledRegions(new java.util.ArrayList(enabledRegions.length));
}
for (String ele : enabledRegions) {
this.enabledRegions.add(ele);
}
return this;
}
/**
*
* The Amazon Web Services regions in which this blueprint is enabled.
*
*
* @param enabledRegions
* The Amazon Web Services regions in which this blueprint is enabled.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEnvironmentBlueprintConfigurationResult withEnabledRegions(java.util.Collection enabledRegions) {
setEnabledRegions(enabledRegions);
return this;
}
/**
*
* The ID of the blueprint.
*
*
* @param environmentBlueprintId
* The ID of the blueprint.
*/
public void setEnvironmentBlueprintId(String environmentBlueprintId) {
this.environmentBlueprintId = environmentBlueprintId;
}
/**
*
* The ID of the blueprint.
*
*
* @return The ID of the blueprint.
*/
public String getEnvironmentBlueprintId() {
return this.environmentBlueprintId;
}
/**
*
* The ID of the blueprint.
*
*
* @param environmentBlueprintId
* The ID of the blueprint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEnvironmentBlueprintConfigurationResult withEnvironmentBlueprintId(String environmentBlueprintId) {
setEnvironmentBlueprintId(environmentBlueprintId);
return this;
}
/**
*
* The ARN of the manage access role with which this blueprint is created.
*
*
* @param manageAccessRoleArn
* The ARN of the manage access role with which this blueprint is created.
*/
public void setManageAccessRoleArn(String manageAccessRoleArn) {
this.manageAccessRoleArn = manageAccessRoleArn;
}
/**
*
* The ARN of the manage access role with which this blueprint is created.
*
*
* @return The ARN of the manage access role with which this blueprint is created.
*/
public String getManageAccessRoleArn() {
return this.manageAccessRoleArn;
}
/**
*
* The ARN of the manage access role with which this blueprint is created.
*
*
* @param manageAccessRoleArn
* The ARN of the manage access role with which this blueprint is created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEnvironmentBlueprintConfigurationResult withManageAccessRoleArn(String manageAccessRoleArn) {
setManageAccessRoleArn(manageAccessRoleArn);
return this;
}
/**
*
* The provisioning configuration of a blueprint.
*
*
* @return The provisioning configuration of a blueprint.
*/
public java.util.List getProvisioningConfigurations() {
return provisioningConfigurations;
}
/**
*
* The provisioning configuration of a blueprint.
*
*
* @param provisioningConfigurations
* The provisioning configuration of a blueprint.
*/
public void setProvisioningConfigurations(java.util.Collection provisioningConfigurations) {
if (provisioningConfigurations == null) {
this.provisioningConfigurations = null;
return;
}
this.provisioningConfigurations = new java.util.ArrayList(provisioningConfigurations);
}
/**
*
* The provisioning configuration of a blueprint.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setProvisioningConfigurations(java.util.Collection)} or
* {@link #withProvisioningConfigurations(java.util.Collection)} if you want to override the existing values.
*
*
* @param provisioningConfigurations
* The provisioning configuration of a blueprint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEnvironmentBlueprintConfigurationResult withProvisioningConfigurations(ProvisioningConfiguration... provisioningConfigurations) {
if (this.provisioningConfigurations == null) {
setProvisioningConfigurations(new java.util.ArrayList(provisioningConfigurations.length));
}
for (ProvisioningConfiguration ele : provisioningConfigurations) {
this.provisioningConfigurations.add(ele);
}
return this;
}
/**
*
* The provisioning configuration of a blueprint.
*
*
* @param provisioningConfigurations
* The provisioning configuration of a blueprint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEnvironmentBlueprintConfigurationResult withProvisioningConfigurations(java.util.Collection provisioningConfigurations) {
setProvisioningConfigurations(provisioningConfigurations);
return this;
}
/**
*
* The ARN of the provisioning role with which this blueprint is created.
*
*
* @param provisioningRoleArn
* The ARN of the provisioning role with which this blueprint is created.
*/
public void setProvisioningRoleArn(String provisioningRoleArn) {
this.provisioningRoleArn = provisioningRoleArn;
}
/**
*
* The ARN of the provisioning role with which this blueprint is created.
*
*
* @return The ARN of the provisioning role with which this blueprint is created.
*/
public String getProvisioningRoleArn() {
return this.provisioningRoleArn;
}
/**
*
* The ARN of the provisioning role with which this blueprint is created.
*
*
* @param provisioningRoleArn
* The ARN of the provisioning role with which this blueprint is created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEnvironmentBlueprintConfigurationResult withProvisioningRoleArn(String provisioningRoleArn) {
setProvisioningRoleArn(provisioningRoleArn);
return this;
}
/**
*
* The regional parameters of the blueprint.
*
*
* @return The regional parameters of the blueprint.
*/
public java.util.Map> getRegionalParameters() {
return regionalParameters;
}
/**
*
* The regional parameters of the blueprint.
*
*
* @param regionalParameters
* The regional parameters of the blueprint.
*/
public void setRegionalParameters(java.util.Map> regionalParameters) {
this.regionalParameters = regionalParameters;
}
/**
*
* The regional parameters of the blueprint.
*
*
* @param regionalParameters
* The regional parameters of the blueprint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEnvironmentBlueprintConfigurationResult withRegionalParameters(java.util.Map> regionalParameters) {
setRegionalParameters(regionalParameters);
return this;
}
/**
* Add a single RegionalParameters entry
*
* @see GetEnvironmentBlueprintConfigurationResult#withRegionalParameters
* @returns a reference to this object so that method calls can be chained together.
*/
public GetEnvironmentBlueprintConfigurationResult addRegionalParametersEntry(String key, java.util.Map value) {
if (null == this.regionalParameters) {
this.regionalParameters = new java.util.HashMap>();
}
if (this.regionalParameters.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.regionalParameters.put(key, value);
return this;
}
/**
* Removes all the entries added into RegionalParameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEnvironmentBlueprintConfigurationResult clearRegionalParametersEntries() {
this.regionalParameters = null;
return this;
}
/**
*
* The timestamp of when this blueprint was upated.
*
*
* @param updatedAt
* The timestamp of when this blueprint was upated.
*/
public void setUpdatedAt(java.util.Date updatedAt) {
this.updatedAt = updatedAt;
}
/**
*
* The timestamp of when this blueprint was upated.
*
*
* @return The timestamp of when this blueprint was upated.
*/
public java.util.Date getUpdatedAt() {
return this.updatedAt;
}
/**
*
* The timestamp of when this blueprint was upated.
*
*
* @param updatedAt
* The timestamp of when this blueprint was upated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEnvironmentBlueprintConfigurationResult withUpdatedAt(java.util.Date updatedAt) {
setUpdatedAt(updatedAt);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getDomainId() != null)
sb.append("DomainId: ").append(getDomainId()).append(",");
if (getEnabledRegions() != null)
sb.append("EnabledRegions: ").append(getEnabledRegions()).append(",");
if (getEnvironmentBlueprintId() != null)
sb.append("EnvironmentBlueprintId: ").append(getEnvironmentBlueprintId()).append(",");
if (getManageAccessRoleArn() != null)
sb.append("ManageAccessRoleArn: ").append(getManageAccessRoleArn()).append(",");
if (getProvisioningConfigurations() != null)
sb.append("ProvisioningConfigurations: ").append(getProvisioningConfigurations()).append(",");
if (getProvisioningRoleArn() != null)
sb.append("ProvisioningRoleArn: ").append(getProvisioningRoleArn()).append(",");
if (getRegionalParameters() != null)
sb.append("RegionalParameters: ").append(getRegionalParameters()).append(",");
if (getUpdatedAt() != null)
sb.append("UpdatedAt: ").append(getUpdatedAt());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetEnvironmentBlueprintConfigurationResult == false)
return false;
GetEnvironmentBlueprintConfigurationResult other = (GetEnvironmentBlueprintConfigurationResult) obj;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getDomainId() == null ^ this.getDomainId() == null)
return false;
if (other.getDomainId() != null && other.getDomainId().equals(this.getDomainId()) == false)
return false;
if (other.getEnabledRegions() == null ^ this.getEnabledRegions() == null)
return false;
if (other.getEnabledRegions() != null && other.getEnabledRegions().equals(this.getEnabledRegions()) == false)
return false;
if (other.getEnvironmentBlueprintId() == null ^ this.getEnvironmentBlueprintId() == null)
return false;
if (other.getEnvironmentBlueprintId() != null && other.getEnvironmentBlueprintId().equals(this.getEnvironmentBlueprintId()) == false)
return false;
if (other.getManageAccessRoleArn() == null ^ this.getManageAccessRoleArn() == null)
return false;
if (other.getManageAccessRoleArn() != null && other.getManageAccessRoleArn().equals(this.getManageAccessRoleArn()) == false)
return false;
if (other.getProvisioningConfigurations() == null ^ this.getProvisioningConfigurations() == null)
return false;
if (other.getProvisioningConfigurations() != null && other.getProvisioningConfigurations().equals(this.getProvisioningConfigurations()) == false)
return false;
if (other.getProvisioningRoleArn() == null ^ this.getProvisioningRoleArn() == null)
return false;
if (other.getProvisioningRoleArn() != null && other.getProvisioningRoleArn().equals(this.getProvisioningRoleArn()) == false)
return false;
if (other.getRegionalParameters() == null ^ this.getRegionalParameters() == null)
return false;
if (other.getRegionalParameters() != null && other.getRegionalParameters().equals(this.getRegionalParameters()) == false)
return false;
if (other.getUpdatedAt() == null ^ this.getUpdatedAt() == null)
return false;
if (other.getUpdatedAt() != null && other.getUpdatedAt().equals(this.getUpdatedAt()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getDomainId() == null) ? 0 : getDomainId().hashCode());
hashCode = prime * hashCode + ((getEnabledRegions() == null) ? 0 : getEnabledRegions().hashCode());
hashCode = prime * hashCode + ((getEnvironmentBlueprintId() == null) ? 0 : getEnvironmentBlueprintId().hashCode());
hashCode = prime * hashCode + ((getManageAccessRoleArn() == null) ? 0 : getManageAccessRoleArn().hashCode());
hashCode = prime * hashCode + ((getProvisioningConfigurations() == null) ? 0 : getProvisioningConfigurations().hashCode());
hashCode = prime * hashCode + ((getProvisioningRoleArn() == null) ? 0 : getProvisioningRoleArn().hashCode());
hashCode = prime * hashCode + ((getRegionalParameters() == null) ? 0 : getRegionalParameters().hashCode());
hashCode = prime * hashCode + ((getUpdatedAt() == null) ? 0 : getUpdatedAt().hashCode());
return hashCode;
}
@Override
public GetEnvironmentBlueprintConfigurationResult clone() {
try {
return (GetEnvironmentBlueprintConfigurationResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}