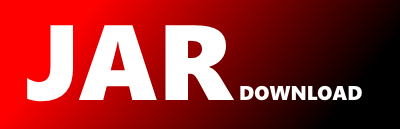
com.amazonaws.services.deadline.AWSDeadlineAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-deadline Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.deadline;
import javax.annotation.Generated;
import com.amazonaws.services.deadline.model.*;
/**
* Interface for accessing AWSDeadlineCloud asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.deadline.AbstractAWSDeadlineAsync} instead.
*
*
*
* The Amazon Web Services Deadline Cloud API provides infrastructure and centralized management for your projects. Use
* the Deadline Cloud API to onboard users, assign projects, and attach permissions specific to their job function.
*
*
* With Deadline Cloud, content production teams can deploy resources for their workforce securely in the cloud,
* reducing the costs of added physical infrastructure. Keep your content production operations secure, while allowing
* your contributors to access the tools they need, such as scalable high-speed storage, licenses, and cost management
* services.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSDeadlineAsync extends AWSDeadline {
/**
*
* Assigns a farm membership level to a member.
*
*
* @param associateMemberToFarmRequest
* @return A Java Future containing the result of the AssociateMemberToFarm operation returned by the service.
* @sample AWSDeadlineAsync.AssociateMemberToFarm
* @see AWS
* API Documentation
*/
java.util.concurrent.Future associateMemberToFarmAsync(AssociateMemberToFarmRequest associateMemberToFarmRequest);
/**
*
* Assigns a farm membership level to a member.
*
*
* @param associateMemberToFarmRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateMemberToFarm operation returned by the service.
* @sample AWSDeadlineAsyncHandler.AssociateMemberToFarm
* @see AWS
* API Documentation
*/
java.util.concurrent.Future associateMemberToFarmAsync(AssociateMemberToFarmRequest associateMemberToFarmRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns a fleet membership level to a member.
*
*
* @param associateMemberToFleetRequest
* @return A Java Future containing the result of the AssociateMemberToFleet operation returned by the service.
* @sample AWSDeadlineAsync.AssociateMemberToFleet
* @see AWS API Documentation
*/
java.util.concurrent.Future associateMemberToFleetAsync(AssociateMemberToFleetRequest associateMemberToFleetRequest);
/**
*
* Assigns a fleet membership level to a member.
*
*
* @param associateMemberToFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateMemberToFleet operation returned by the service.
* @sample AWSDeadlineAsyncHandler.AssociateMemberToFleet
* @see AWS API Documentation
*/
java.util.concurrent.Future associateMemberToFleetAsync(AssociateMemberToFleetRequest associateMemberToFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns a job membership level to a member
*
*
* @param associateMemberToJobRequest
* @return A Java Future containing the result of the AssociateMemberToJob operation returned by the service.
* @sample AWSDeadlineAsync.AssociateMemberToJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future associateMemberToJobAsync(AssociateMemberToJobRequest associateMemberToJobRequest);
/**
*
* Assigns a job membership level to a member
*
*
* @param associateMemberToJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateMemberToJob operation returned by the service.
* @sample AWSDeadlineAsyncHandler.AssociateMemberToJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future associateMemberToJobAsync(AssociateMemberToJobRequest associateMemberToJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns a queue membership level to a member
*
*
* @param associateMemberToQueueRequest
* @return A Java Future containing the result of the AssociateMemberToQueue operation returned by the service.
* @sample AWSDeadlineAsync.AssociateMemberToQueue
* @see AWS API Documentation
*/
java.util.concurrent.Future associateMemberToQueueAsync(AssociateMemberToQueueRequest associateMemberToQueueRequest);
/**
*
* Assigns a queue membership level to a member
*
*
* @param associateMemberToQueueRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateMemberToQueue operation returned by the service.
* @sample AWSDeadlineAsyncHandler.AssociateMemberToQueue
* @see AWS API Documentation
*/
java.util.concurrent.Future associateMemberToQueueAsync(AssociateMemberToQueueRequest associateMemberToQueueRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get Amazon Web Services credentials from the fleet role. The IAM permissions of the credentials are scoped down
* to have read-only access.
*
*
* @param assumeFleetRoleForReadRequest
* @return A Java Future containing the result of the AssumeFleetRoleForRead operation returned by the service.
* @sample AWSDeadlineAsync.AssumeFleetRoleForRead
* @see AWS API Documentation
*/
java.util.concurrent.Future assumeFleetRoleForReadAsync(AssumeFleetRoleForReadRequest assumeFleetRoleForReadRequest);
/**
*
* Get Amazon Web Services credentials from the fleet role. The IAM permissions of the credentials are scoped down
* to have read-only access.
*
*
* @param assumeFleetRoleForReadRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssumeFleetRoleForRead operation returned by the service.
* @sample AWSDeadlineAsyncHandler.AssumeFleetRoleForRead
* @see AWS API Documentation
*/
java.util.concurrent.Future assumeFleetRoleForReadAsync(AssumeFleetRoleForReadRequest assumeFleetRoleForReadRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get credentials from the fleet role for a worker.
*
*
* @param assumeFleetRoleForWorkerRequest
* @return A Java Future containing the result of the AssumeFleetRoleForWorker operation returned by the service.
* @sample AWSDeadlineAsync.AssumeFleetRoleForWorker
* @see AWS API Documentation
*/
java.util.concurrent.Future assumeFleetRoleForWorkerAsync(AssumeFleetRoleForWorkerRequest assumeFleetRoleForWorkerRequest);
/**
*
* Get credentials from the fleet role for a worker.
*
*
* @param assumeFleetRoleForWorkerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssumeFleetRoleForWorker operation returned by the service.
* @sample AWSDeadlineAsyncHandler.AssumeFleetRoleForWorker
* @see AWS API Documentation
*/
java.util.concurrent.Future assumeFleetRoleForWorkerAsync(AssumeFleetRoleForWorkerRequest assumeFleetRoleForWorkerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets Amazon Web Services credentials from the queue role. The IAM permissions of the credentials are scoped down
* to have read-only access.
*
*
* @param assumeQueueRoleForReadRequest
* @return A Java Future containing the result of the AssumeQueueRoleForRead operation returned by the service.
* @sample AWSDeadlineAsync.AssumeQueueRoleForRead
* @see AWS API Documentation
*/
java.util.concurrent.Future assumeQueueRoleForReadAsync(AssumeQueueRoleForReadRequest assumeQueueRoleForReadRequest);
/**
*
* Gets Amazon Web Services credentials from the queue role. The IAM permissions of the credentials are scoped down
* to have read-only access.
*
*
* @param assumeQueueRoleForReadRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssumeQueueRoleForRead operation returned by the service.
* @sample AWSDeadlineAsyncHandler.AssumeQueueRoleForRead
* @see AWS API Documentation
*/
java.util.concurrent.Future assumeQueueRoleForReadAsync(AssumeQueueRoleForReadRequest assumeQueueRoleForReadRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Allows a user to assume a role for a queue.
*
*
* @param assumeQueueRoleForUserRequest
* @return A Java Future containing the result of the AssumeQueueRoleForUser operation returned by the service.
* @sample AWSDeadlineAsync.AssumeQueueRoleForUser
* @see AWS API Documentation
*/
java.util.concurrent.Future assumeQueueRoleForUserAsync(AssumeQueueRoleForUserRequest assumeQueueRoleForUserRequest);
/**
*
* Allows a user to assume a role for a queue.
*
*
* @param assumeQueueRoleForUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssumeQueueRoleForUser operation returned by the service.
* @sample AWSDeadlineAsyncHandler.AssumeQueueRoleForUser
* @see AWS API Documentation
*/
java.util.concurrent.Future assumeQueueRoleForUserAsync(AssumeQueueRoleForUserRequest assumeQueueRoleForUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Allows a worker to assume a queue role.
*
*
* @param assumeQueueRoleForWorkerRequest
* @return A Java Future containing the result of the AssumeQueueRoleForWorker operation returned by the service.
* @sample AWSDeadlineAsync.AssumeQueueRoleForWorker
* @see AWS API Documentation
*/
java.util.concurrent.Future assumeQueueRoleForWorkerAsync(AssumeQueueRoleForWorkerRequest assumeQueueRoleForWorkerRequest);
/**
*
* Allows a worker to assume a queue role.
*
*
* @param assumeQueueRoleForWorkerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssumeQueueRoleForWorker operation returned by the service.
* @sample AWSDeadlineAsyncHandler.AssumeQueueRoleForWorker
* @see AWS API Documentation
*/
java.util.concurrent.Future assumeQueueRoleForWorkerAsync(AssumeQueueRoleForWorkerRequest assumeQueueRoleForWorkerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get batched job details for a worker.
*
*
* @param batchGetJobEntityRequest
* @return A Java Future containing the result of the BatchGetJobEntity operation returned by the service.
* @sample AWSDeadlineAsync.BatchGetJobEntity
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchGetJobEntityAsync(BatchGetJobEntityRequest batchGetJobEntityRequest);
/**
*
* Get batched job details for a worker.
*
*
* @param batchGetJobEntityRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchGetJobEntity operation returned by the service.
* @sample AWSDeadlineAsyncHandler.BatchGetJobEntity
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchGetJobEntityAsync(BatchGetJobEntityRequest batchGetJobEntityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Copies a job template to an Amazon S3 bucket.
*
*
* @param copyJobTemplateRequest
* @return A Java Future containing the result of the CopyJobTemplate operation returned by the service.
* @sample AWSDeadlineAsync.CopyJobTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyJobTemplateAsync(CopyJobTemplateRequest copyJobTemplateRequest);
/**
*
* Copies a job template to an Amazon S3 bucket.
*
*
* @param copyJobTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopyJobTemplate operation returned by the service.
* @sample AWSDeadlineAsyncHandler.CopyJobTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyJobTemplateAsync(CopyJobTemplateRequest copyJobTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a budget to set spending thresholds for your rendering activity.
*
*
* @param createBudgetRequest
* @return A Java Future containing the result of the CreateBudget operation returned by the service.
* @sample AWSDeadlineAsync.CreateBudget
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createBudgetAsync(CreateBudgetRequest createBudgetRequest);
/**
*
* Creates a budget to set spending thresholds for your rendering activity.
*
*
* @param createBudgetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateBudget operation returned by the service.
* @sample AWSDeadlineAsyncHandler.CreateBudget
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createBudgetAsync(CreateBudgetRequest createBudgetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a farm to allow space for queues and fleets. Farms are the space where the components of your renders
* gather and are pieced together in the cloud. Farms contain budgets and allow you to enforce permissions. Deadline
* Cloud farms are a useful container for large projects.
*
*
* @param createFarmRequest
* @return A Java Future containing the result of the CreateFarm operation returned by the service.
* @sample AWSDeadlineAsync.CreateFarm
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFarmAsync(CreateFarmRequest createFarmRequest);
/**
*
* Creates a farm to allow space for queues and fleets. Farms are the space where the components of your renders
* gather and are pieced together in the cloud. Farms contain budgets and allow you to enforce permissions. Deadline
* Cloud farms are a useful container for large projects.
*
*
* @param createFarmRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFarm operation returned by the service.
* @sample AWSDeadlineAsyncHandler.CreateFarm
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFarmAsync(CreateFarmRequest createFarmRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a fleet. Fleets gather information relating to compute, or capacity, for renders within your farms. You
* can choose to manage your own capacity or opt to have fleets fully managed by Deadline Cloud.
*
*
* @param createFleetRequest
* @return A Java Future containing the result of the CreateFleet operation returned by the service.
* @sample AWSDeadlineAsync.CreateFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFleetAsync(CreateFleetRequest createFleetRequest);
/**
*
* Creates a fleet. Fleets gather information relating to compute, or capacity, for renders within your farms. You
* can choose to manage your own capacity or opt to have fleets fully managed by Deadline Cloud.
*
*
* @param createFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFleet operation returned by the service.
* @sample AWSDeadlineAsyncHandler.CreateFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFleetAsync(CreateFleetRequest createFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a job. A job is a render submission submitted by a user. It contains specific job properties outlined as
* steps and tasks.
*
*
* @param createJobRequest
* @return A Java Future containing the result of the CreateJob operation returned by the service.
* @sample AWSDeadlineAsync.CreateJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createJobAsync(CreateJobRequest createJobRequest);
/**
*
* Creates a job. A job is a render submission submitted by a user. It contains specific job properties outlined as
* steps and tasks.
*
*
* @param createJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateJob operation returned by the service.
* @sample AWSDeadlineAsyncHandler.CreateJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createJobAsync(CreateJobRequest createJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a license endpoint to integrate your various licensed software used for rendering on Deadline Cloud.
*
*
* @param createLicenseEndpointRequest
* @return A Java Future containing the result of the CreateLicenseEndpoint operation returned by the service.
* @sample AWSDeadlineAsync.CreateLicenseEndpoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createLicenseEndpointAsync(CreateLicenseEndpointRequest createLicenseEndpointRequest);
/**
*
* Creates a license endpoint to integrate your various licensed software used for rendering on Deadline Cloud.
*
*
* @param createLicenseEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLicenseEndpoint operation returned by the service.
* @sample AWSDeadlineAsyncHandler.CreateLicenseEndpoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createLicenseEndpointAsync(CreateLicenseEndpointRequest createLicenseEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon Web Services Deadline Cloud monitor that you can use to view your farms, queues, and fleets.
* After you submit a job, you can track the progress of the tasks and steps that make up the job, and then download
* the job's results.
*
*
* @param createMonitorRequest
* @return A Java Future containing the result of the CreateMonitor operation returned by the service.
* @sample AWSDeadlineAsync.CreateMonitor
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createMonitorAsync(CreateMonitorRequest createMonitorRequest);
/**
*
* Creates an Amazon Web Services Deadline Cloud monitor that you can use to view your farms, queues, and fleets.
* After you submit a job, you can track the progress of the tasks and steps that make up the job, and then download
* the job's results.
*
*
* @param createMonitorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMonitor operation returned by the service.
* @sample AWSDeadlineAsyncHandler.CreateMonitor
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createMonitorAsync(CreateMonitorRequest createMonitorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a queue to coordinate the order in which jobs run on a farm. A queue can also specify where to pull
* resources and indicate where to output completed jobs.
*
*
* @param createQueueRequest
* @return A Java Future containing the result of the CreateQueue operation returned by the service.
* @sample AWSDeadlineAsync.CreateQueue
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createQueueAsync(CreateQueueRequest createQueueRequest);
/**
*
* Creates a queue to coordinate the order in which jobs run on a farm. A queue can also specify where to pull
* resources and indicate where to output completed jobs.
*
*
* @param createQueueRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateQueue operation returned by the service.
* @sample AWSDeadlineAsyncHandler.CreateQueue
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createQueueAsync(CreateQueueRequest createQueueRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an environment for a queue that defines how jobs in the queue run.
*
*
* @param createQueueEnvironmentRequest
* @return A Java Future containing the result of the CreateQueueEnvironment operation returned by the service.
* @sample AWSDeadlineAsync.CreateQueueEnvironment
* @see AWS API Documentation
*/
java.util.concurrent.Future createQueueEnvironmentAsync(CreateQueueEnvironmentRequest createQueueEnvironmentRequest);
/**
*
* Creates an environment for a queue that defines how jobs in the queue run.
*
*
* @param createQueueEnvironmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateQueueEnvironment operation returned by the service.
* @sample AWSDeadlineAsyncHandler.CreateQueueEnvironment
* @see AWS API Documentation
*/
java.util.concurrent.Future createQueueEnvironmentAsync(CreateQueueEnvironmentRequest createQueueEnvironmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an association between a queue and a fleet.
*
*
* @param createQueueFleetAssociationRequest
* @return A Java Future containing the result of the CreateQueueFleetAssociation operation returned by the service.
* @sample AWSDeadlineAsync.CreateQueueFleetAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future createQueueFleetAssociationAsync(
CreateQueueFleetAssociationRequest createQueueFleetAssociationRequest);
/**
*
* Creates an association between a queue and a fleet.
*
*
* @param createQueueFleetAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateQueueFleetAssociation operation returned by the service.
* @sample AWSDeadlineAsyncHandler.CreateQueueFleetAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future createQueueFleetAssociationAsync(
CreateQueueFleetAssociationRequest createQueueFleetAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a storage profile that specifies the operating system, file type, and file location of resources used on
* a farm.
*
*
* @param createStorageProfileRequest
* @return A Java Future containing the result of the CreateStorageProfile operation returned by the service.
* @sample AWSDeadlineAsync.CreateStorageProfile
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createStorageProfileAsync(CreateStorageProfileRequest createStorageProfileRequest);
/**
*
* Creates a storage profile that specifies the operating system, file type, and file location of resources used on
* a farm.
*
*
* @param createStorageProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateStorageProfile operation returned by the service.
* @sample AWSDeadlineAsyncHandler.CreateStorageProfile
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createStorageProfileAsync(CreateStorageProfileRequest createStorageProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a worker. A worker tells your instance how much processing power (vCPU), and memory (GiB) you’ll need to
* assemble the digital assets held within a particular instance. You can specify certain instance types to use, or
* let the worker know which instances types to exclude.
*
*
* @param createWorkerRequest
* @return A Java Future containing the result of the CreateWorker operation returned by the service.
* @sample AWSDeadlineAsync.CreateWorker
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createWorkerAsync(CreateWorkerRequest createWorkerRequest);
/**
*
* Creates a worker. A worker tells your instance how much processing power (vCPU), and memory (GiB) you’ll need to
* assemble the digital assets held within a particular instance. You can specify certain instance types to use, or
* let the worker know which instances types to exclude.
*
*
* @param createWorkerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateWorker operation returned by the service.
* @sample AWSDeadlineAsyncHandler.CreateWorker
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createWorkerAsync(CreateWorkerRequest createWorkerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a budget.
*
*
* @param deleteBudgetRequest
* @return A Java Future containing the result of the DeleteBudget operation returned by the service.
* @sample AWSDeadlineAsync.DeleteBudget
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteBudgetAsync(DeleteBudgetRequest deleteBudgetRequest);
/**
*
* Deletes a budget.
*
*
* @param deleteBudgetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteBudget operation returned by the service.
* @sample AWSDeadlineAsyncHandler.DeleteBudget
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteBudgetAsync(DeleteBudgetRequest deleteBudgetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a farm.
*
*
* @param deleteFarmRequest
* @return A Java Future containing the result of the DeleteFarm operation returned by the service.
* @sample AWSDeadlineAsync.DeleteFarm
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFarmAsync(DeleteFarmRequest deleteFarmRequest);
/**
*
* Deletes a farm.
*
*
* @param deleteFarmRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFarm operation returned by the service.
* @sample AWSDeadlineAsyncHandler.DeleteFarm
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFarmAsync(DeleteFarmRequest deleteFarmRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a fleet.
*
*
* @param deleteFleetRequest
* @return A Java Future containing the result of the DeleteFleet operation returned by the service.
* @sample AWSDeadlineAsync.DeleteFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFleetAsync(DeleteFleetRequest deleteFleetRequest);
/**
*
* Deletes a fleet.
*
*
* @param deleteFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFleet operation returned by the service.
* @sample AWSDeadlineAsyncHandler.DeleteFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFleetAsync(DeleteFleetRequest deleteFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a license endpoint.
*
*
* @param deleteLicenseEndpointRequest
* @return A Java Future containing the result of the DeleteLicenseEndpoint operation returned by the service.
* @sample AWSDeadlineAsync.DeleteLicenseEndpoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteLicenseEndpointAsync(DeleteLicenseEndpointRequest deleteLicenseEndpointRequest);
/**
*
* Deletes a license endpoint.
*
*
* @param deleteLicenseEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteLicenseEndpoint operation returned by the service.
* @sample AWSDeadlineAsyncHandler.DeleteLicenseEndpoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteLicenseEndpointAsync(DeleteLicenseEndpointRequest deleteLicenseEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a metered product.
*
*
* @param deleteMeteredProductRequest
* @return A Java Future containing the result of the DeleteMeteredProduct operation returned by the service.
* @sample AWSDeadlineAsync.DeleteMeteredProduct
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteMeteredProductAsync(DeleteMeteredProductRequest deleteMeteredProductRequest);
/**
*
* Deletes a metered product.
*
*
* @param deleteMeteredProductRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMeteredProduct operation returned by the service.
* @sample AWSDeadlineAsyncHandler.DeleteMeteredProduct
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteMeteredProductAsync(DeleteMeteredProductRequest deleteMeteredProductRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a Deadline Cloud monitor. After you delete a monitor, you can create a new one and attach farms to the
* monitor.
*
*
* @param deleteMonitorRequest
* @return A Java Future containing the result of the DeleteMonitor operation returned by the service.
* @sample AWSDeadlineAsync.DeleteMonitor
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteMonitorAsync(DeleteMonitorRequest deleteMonitorRequest);
/**
*
* Removes a Deadline Cloud monitor. After you delete a monitor, you can create a new one and attach farms to the
* monitor.
*
*
* @param deleteMonitorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMonitor operation returned by the service.
* @sample AWSDeadlineAsyncHandler.DeleteMonitor
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteMonitorAsync(DeleteMonitorRequest deleteMonitorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a queue.
*
*
* @param deleteQueueRequest
* @return A Java Future containing the result of the DeleteQueue operation returned by the service.
* @sample AWSDeadlineAsync.DeleteQueue
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteQueueAsync(DeleteQueueRequest deleteQueueRequest);
/**
*
* Deletes a queue.
*
*
* @param deleteQueueRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteQueue operation returned by the service.
* @sample AWSDeadlineAsyncHandler.DeleteQueue
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteQueueAsync(DeleteQueueRequest deleteQueueRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a queue environment.
*
*
* @param deleteQueueEnvironmentRequest
* @return A Java Future containing the result of the DeleteQueueEnvironment operation returned by the service.
* @sample AWSDeadlineAsync.DeleteQueueEnvironment
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteQueueEnvironmentAsync(DeleteQueueEnvironmentRequest deleteQueueEnvironmentRequest);
/**
*
* Deletes a queue environment.
*
*
* @param deleteQueueEnvironmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteQueueEnvironment operation returned by the service.
* @sample AWSDeadlineAsyncHandler.DeleteQueueEnvironment
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteQueueEnvironmentAsync(DeleteQueueEnvironmentRequest deleteQueueEnvironmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a queue-fleet association.
*
*
* @param deleteQueueFleetAssociationRequest
* @return A Java Future containing the result of the DeleteQueueFleetAssociation operation returned by the service.
* @sample AWSDeadlineAsync.DeleteQueueFleetAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteQueueFleetAssociationAsync(
DeleteQueueFleetAssociationRequest deleteQueueFleetAssociationRequest);
/**
*
* Deletes a queue-fleet association.
*
*
* @param deleteQueueFleetAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteQueueFleetAssociation operation returned by the service.
* @sample AWSDeadlineAsyncHandler.DeleteQueueFleetAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteQueueFleetAssociationAsync(
DeleteQueueFleetAssociationRequest deleteQueueFleetAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a storage profile.
*
*
* @param deleteStorageProfileRequest
* @return A Java Future containing the result of the DeleteStorageProfile operation returned by the service.
* @sample AWSDeadlineAsync.DeleteStorageProfile
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteStorageProfileAsync(DeleteStorageProfileRequest deleteStorageProfileRequest);
/**
*
* Deletes a storage profile.
*
*
* @param deleteStorageProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteStorageProfile operation returned by the service.
* @sample AWSDeadlineAsyncHandler.DeleteStorageProfile
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteStorageProfileAsync(DeleteStorageProfileRequest deleteStorageProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a worker.
*
*
* @param deleteWorkerRequest
* @return A Java Future containing the result of the DeleteWorker operation returned by the service.
* @sample AWSDeadlineAsync.DeleteWorker
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteWorkerAsync(DeleteWorkerRequest deleteWorkerRequest);
/**
*
* Deletes a worker.
*
*
* @param deleteWorkerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteWorker operation returned by the service.
* @sample AWSDeadlineAsyncHandler.DeleteWorker
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteWorkerAsync(DeleteWorkerRequest deleteWorkerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates a member from a farm.
*
*
* @param disassociateMemberFromFarmRequest
* @return A Java Future containing the result of the DisassociateMemberFromFarm operation returned by the service.
* @sample AWSDeadlineAsync.DisassociateMemberFromFarm
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateMemberFromFarmAsync(
DisassociateMemberFromFarmRequest disassociateMemberFromFarmRequest);
/**
*
* Disassociates a member from a farm.
*
*
* @param disassociateMemberFromFarmRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateMemberFromFarm operation returned by the service.
* @sample AWSDeadlineAsyncHandler.DisassociateMemberFromFarm
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateMemberFromFarmAsync(
DisassociateMemberFromFarmRequest disassociateMemberFromFarmRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates a member from a fleet.
*
*
* @param disassociateMemberFromFleetRequest
* @return A Java Future containing the result of the DisassociateMemberFromFleet operation returned by the service.
* @sample AWSDeadlineAsync.DisassociateMemberFromFleet
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateMemberFromFleetAsync(
DisassociateMemberFromFleetRequest disassociateMemberFromFleetRequest);
/**
*
* Disassociates a member from a fleet.
*
*
* @param disassociateMemberFromFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateMemberFromFleet operation returned by the service.
* @sample AWSDeadlineAsyncHandler.DisassociateMemberFromFleet
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateMemberFromFleetAsync(
DisassociateMemberFromFleetRequest disassociateMemberFromFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates a member from a job.
*
*
* @param disassociateMemberFromJobRequest
* @return A Java Future containing the result of the DisassociateMemberFromJob operation returned by the service.
* @sample AWSDeadlineAsync.DisassociateMemberFromJob
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateMemberFromJobAsync(
DisassociateMemberFromJobRequest disassociateMemberFromJobRequest);
/**
*
* Disassociates a member from a job.
*
*
* @param disassociateMemberFromJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateMemberFromJob operation returned by the service.
* @sample AWSDeadlineAsyncHandler.DisassociateMemberFromJob
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateMemberFromJobAsync(
DisassociateMemberFromJobRequest disassociateMemberFromJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates a member from a queue.
*
*
* @param disassociateMemberFromQueueRequest
* @return A Java Future containing the result of the DisassociateMemberFromQueue operation returned by the service.
* @sample AWSDeadlineAsync.DisassociateMemberFromQueue
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateMemberFromQueueAsync(
DisassociateMemberFromQueueRequest disassociateMemberFromQueueRequest);
/**
*
* Disassociates a member from a queue.
*
*
* @param disassociateMemberFromQueueRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateMemberFromQueue operation returned by the service.
* @sample AWSDeadlineAsyncHandler.DisassociateMemberFromQueue
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateMemberFromQueueAsync(
DisassociateMemberFromQueueRequest disassociateMemberFromQueueRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get a budget.
*
*
* @param getBudgetRequest
* @return A Java Future containing the result of the GetBudget operation returned by the service.
* @sample AWSDeadlineAsync.GetBudget
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getBudgetAsync(GetBudgetRequest getBudgetRequest);
/**
*
* Get a budget.
*
*
* @param getBudgetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBudget operation returned by the service.
* @sample AWSDeadlineAsyncHandler.GetBudget
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getBudgetAsync(GetBudgetRequest getBudgetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get a farm.
*
*
* @param getFarmRequest
* @return A Java Future containing the result of the GetFarm operation returned by the service.
* @sample AWSDeadlineAsync.GetFarm
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getFarmAsync(GetFarmRequest getFarmRequest);
/**
*
* Get a farm.
*
*
* @param getFarmRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetFarm operation returned by the service.
* @sample AWSDeadlineAsyncHandler.GetFarm
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getFarmAsync(GetFarmRequest getFarmRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get a fleet.
*
*
* @param getFleetRequest
* @return A Java Future containing the result of the GetFleet operation returned by the service.
* @sample AWSDeadlineAsync.GetFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getFleetAsync(GetFleetRequest getFleetRequest);
/**
*
* Get a fleet.
*
*
* @param getFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetFleet operation returned by the service.
* @sample AWSDeadlineAsyncHandler.GetFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getFleetAsync(GetFleetRequest getFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a Deadline Cloud job.
*
*
* @param getJobRequest
* @return A Java Future containing the result of the GetJob operation returned by the service.
* @sample AWSDeadlineAsync.GetJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getJobAsync(GetJobRequest getJobRequest);
/**
*
* Gets a Deadline Cloud job.
*
*
* @param getJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetJob operation returned by the service.
* @sample AWSDeadlineAsyncHandler.GetJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getJobAsync(GetJobRequest getJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a licence endpoint.
*
*
* @param getLicenseEndpointRequest
* @return A Java Future containing the result of the GetLicenseEndpoint operation returned by the service.
* @sample AWSDeadlineAsync.GetLicenseEndpoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getLicenseEndpointAsync(GetLicenseEndpointRequest getLicenseEndpointRequest);
/**
*
* Gets a licence endpoint.
*
*
* @param getLicenseEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetLicenseEndpoint operation returned by the service.
* @sample AWSDeadlineAsyncHandler.GetLicenseEndpoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getLicenseEndpointAsync(GetLicenseEndpointRequest getLicenseEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the specified monitor.
*
*
* @param getMonitorRequest
* @return A Java Future containing the result of the GetMonitor operation returned by the service.
* @sample AWSDeadlineAsync.GetMonitor
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getMonitorAsync(GetMonitorRequest getMonitorRequest);
/**
*
* Gets information about the specified monitor.
*
*
* @param getMonitorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMonitor operation returned by the service.
* @sample AWSDeadlineAsyncHandler.GetMonitor
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getMonitorAsync(GetMonitorRequest getMonitorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a queue.
*
*
* @param getQueueRequest
* @return A Java Future containing the result of the GetQueue operation returned by the service.
* @sample AWSDeadlineAsync.GetQueue
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getQueueAsync(GetQueueRequest getQueueRequest);
/**
*
* Gets a queue.
*
*
* @param getQueueRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetQueue operation returned by the service.
* @sample AWSDeadlineAsyncHandler.GetQueue
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getQueueAsync(GetQueueRequest getQueueRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a queue environment.
*
*
* @param getQueueEnvironmentRequest
* @return A Java Future containing the result of the GetQueueEnvironment operation returned by the service.
* @sample AWSDeadlineAsync.GetQueueEnvironment
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getQueueEnvironmentAsync(GetQueueEnvironmentRequest getQueueEnvironmentRequest);
/**
*
* Gets a queue environment.
*
*
* @param getQueueEnvironmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetQueueEnvironment operation returned by the service.
* @sample AWSDeadlineAsyncHandler.GetQueueEnvironment
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getQueueEnvironmentAsync(GetQueueEnvironmentRequest getQueueEnvironmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a queue-fleet association.
*
*
* @param getQueueFleetAssociationRequest
* @return A Java Future containing the result of the GetQueueFleetAssociation operation returned by the service.
* @sample AWSDeadlineAsync.GetQueueFleetAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future getQueueFleetAssociationAsync(GetQueueFleetAssociationRequest getQueueFleetAssociationRequest);
/**
*
* Gets a queue-fleet association.
*
*
* @param getQueueFleetAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetQueueFleetAssociation operation returned by the service.
* @sample AWSDeadlineAsyncHandler.GetQueueFleetAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future getQueueFleetAssociationAsync(GetQueueFleetAssociationRequest getQueueFleetAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a session.
*
*
* @param getSessionRequest
* @return A Java Future containing the result of the GetSession operation returned by the service.
* @sample AWSDeadlineAsync.GetSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSessionAsync(GetSessionRequest getSessionRequest);
/**
*
* Gets a session.
*
*
* @param getSessionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSession operation returned by the service.
* @sample AWSDeadlineAsyncHandler.GetSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSessionAsync(GetSessionRequest getSessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a session action for the job.
*
*
* @param getSessionActionRequest
* @return A Java Future containing the result of the GetSessionAction operation returned by the service.
* @sample AWSDeadlineAsync.GetSessionAction
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSessionActionAsync(GetSessionActionRequest getSessionActionRequest);
/**
*
* Gets a session action for the job.
*
*
* @param getSessionActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSessionAction operation returned by the service.
* @sample AWSDeadlineAsyncHandler.GetSessionAction
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSessionActionAsync(GetSessionActionRequest getSessionActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a set of statistics for queues or farms. Before you can call the
* GetSessionStatisticsAggregation
operation, you must first call the
* StartSessionsStatisticsAggregation
operation. Statistics are available for 1 hour after you call the
* StartSessionsStatisticsAggregation
operation.
*
*
* @param getSessionsStatisticsAggregationRequest
* @return A Java Future containing the result of the GetSessionsStatisticsAggregation operation returned by the
* service.
* @sample AWSDeadlineAsync.GetSessionsStatisticsAggregation
* @see AWS API Documentation
*/
java.util.concurrent.Future getSessionsStatisticsAggregationAsync(
GetSessionsStatisticsAggregationRequest getSessionsStatisticsAggregationRequest);
/**
*
* Gets a set of statistics for queues or farms. Before you can call the
* GetSessionStatisticsAggregation
operation, you must first call the
* StartSessionsStatisticsAggregation
operation. Statistics are available for 1 hour after you call the
* StartSessionsStatisticsAggregation
operation.
*
*
* @param getSessionsStatisticsAggregationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSessionsStatisticsAggregation operation returned by the
* service.
* @sample AWSDeadlineAsyncHandler.GetSessionsStatisticsAggregation
* @see AWS API Documentation
*/
java.util.concurrent.Future getSessionsStatisticsAggregationAsync(
GetSessionsStatisticsAggregationRequest getSessionsStatisticsAggregationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a step.
*
*
* @param getStepRequest
* @return A Java Future containing the result of the GetStep operation returned by the service.
* @sample AWSDeadlineAsync.GetStep
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getStepAsync(GetStepRequest getStepRequest);
/**
*
* Gets a step.
*
*
* @param getStepRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetStep operation returned by the service.
* @sample AWSDeadlineAsyncHandler.GetStep
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getStepAsync(GetStepRequest getStepRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a storage profile.
*
*
* @param getStorageProfileRequest
* @return A Java Future containing the result of the GetStorageProfile operation returned by the service.
* @sample AWSDeadlineAsync.GetStorageProfile
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getStorageProfileAsync(GetStorageProfileRequest getStorageProfileRequest);
/**
*
* Gets a storage profile.
*
*
* @param getStorageProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetStorageProfile operation returned by the service.
* @sample AWSDeadlineAsyncHandler.GetStorageProfile
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getStorageProfileAsync(GetStorageProfileRequest getStorageProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a storage profile for a queue.
*
*
* @param getStorageProfileForQueueRequest
* @return A Java Future containing the result of the GetStorageProfileForQueue operation returned by the service.
* @sample AWSDeadlineAsync.GetStorageProfileForQueue
* @see AWS API Documentation
*/
java.util.concurrent.Future getStorageProfileForQueueAsync(
GetStorageProfileForQueueRequest getStorageProfileForQueueRequest);
/**
*
* Gets a storage profile for a queue.
*
*
* @param getStorageProfileForQueueRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetStorageProfileForQueue operation returned by the service.
* @sample AWSDeadlineAsyncHandler.GetStorageProfileForQueue
* @see AWS API Documentation
*/
java.util.concurrent.Future getStorageProfileForQueueAsync(
GetStorageProfileForQueueRequest getStorageProfileForQueueRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a task.
*
*
* @param getTaskRequest
* @return A Java Future containing the result of the GetTask operation returned by the service.
* @sample AWSDeadlineAsync.GetTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTaskAsync(GetTaskRequest getTaskRequest);
/**
*
* Gets a task.
*
*
* @param getTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTask operation returned by the service.
* @sample AWSDeadlineAsyncHandler.GetTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTaskAsync(GetTaskRequest getTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a worker.
*
*
* @param getWorkerRequest
* @return A Java Future containing the result of the GetWorker operation returned by the service.
* @sample AWSDeadlineAsync.GetWorker
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getWorkerAsync(GetWorkerRequest getWorkerRequest);
/**
*
* Gets a worker.
*
*
* @param getWorkerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetWorker operation returned by the service.
* @sample AWSDeadlineAsyncHandler.GetWorker
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getWorkerAsync(GetWorkerRequest getWorkerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* A list of the available metered products.
*
*
* @param listAvailableMeteredProductsRequest
* @return A Java Future containing the result of the ListAvailableMeteredProducts operation returned by the
* service.
* @sample AWSDeadlineAsync.ListAvailableMeteredProducts
* @see AWS API Documentation
*/
java.util.concurrent.Future listAvailableMeteredProductsAsync(
ListAvailableMeteredProductsRequest listAvailableMeteredProductsRequest);
/**
*
* A list of the available metered products.
*
*
* @param listAvailableMeteredProductsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAvailableMeteredProducts operation returned by the
* service.
* @sample AWSDeadlineAsyncHandler.ListAvailableMeteredProducts
* @see AWS API Documentation
*/
java.util.concurrent.Future listAvailableMeteredProductsAsync(
ListAvailableMeteredProductsRequest listAvailableMeteredProductsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* A list of budgets in a farm.
*
*
* @param listBudgetsRequest
* @return A Java Future containing the result of the ListBudgets operation returned by the service.
* @sample AWSDeadlineAsync.ListBudgets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listBudgetsAsync(ListBudgetsRequest listBudgetsRequest);
/**
*
* A list of budgets in a farm.
*
*
* @param listBudgetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListBudgets operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListBudgets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listBudgetsAsync(ListBudgetsRequest listBudgetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the members of a farm.
*
*
* @param listFarmMembersRequest
* @return A Java Future containing the result of the ListFarmMembers operation returned by the service.
* @sample AWSDeadlineAsync.ListFarmMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFarmMembersAsync(ListFarmMembersRequest listFarmMembersRequest);
/**
*
* Lists the members of a farm.
*
*
* @param listFarmMembersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFarmMembers operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListFarmMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFarmMembersAsync(ListFarmMembersRequest listFarmMembersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists farms.
*
*
* @param listFarmsRequest
* @return A Java Future containing the result of the ListFarms operation returned by the service.
* @sample AWSDeadlineAsync.ListFarms
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFarmsAsync(ListFarmsRequest listFarmsRequest);
/**
*
* Lists farms.
*
*
* @param listFarmsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFarms operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListFarms
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFarmsAsync(ListFarmsRequest listFarmsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists fleet members.
*
*
* @param listFleetMembersRequest
* @return A Java Future containing the result of the ListFleetMembers operation returned by the service.
* @sample AWSDeadlineAsync.ListFleetMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFleetMembersAsync(ListFleetMembersRequest listFleetMembersRequest);
/**
*
* Lists fleet members.
*
*
* @param listFleetMembersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFleetMembers operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListFleetMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFleetMembersAsync(ListFleetMembersRequest listFleetMembersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists fleets.
*
*
* @param listFleetsRequest
* @return A Java Future containing the result of the ListFleets operation returned by the service.
* @sample AWSDeadlineAsync.ListFleets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFleetsAsync(ListFleetsRequest listFleetsRequest);
/**
*
* Lists fleets.
*
*
* @param listFleetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFleets operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListFleets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFleetsAsync(ListFleetsRequest listFleetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists members on a job.
*
*
* @param listJobMembersRequest
* @return A Java Future containing the result of the ListJobMembers operation returned by the service.
* @sample AWSDeadlineAsync.ListJobMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listJobMembersAsync(ListJobMembersRequest listJobMembersRequest);
/**
*
* Lists members on a job.
*
*
* @param listJobMembersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListJobMembers operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListJobMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listJobMembersAsync(ListJobMembersRequest listJobMembersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists jobs.
*
*
* @param listJobsRequest
* @return A Java Future containing the result of the ListJobs operation returned by the service.
* @sample AWSDeadlineAsync.ListJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listJobsAsync(ListJobsRequest listJobsRequest);
/**
*
* Lists jobs.
*
*
* @param listJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListJobs operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listJobsAsync(ListJobsRequest listJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists license endpoints.
*
*
* @param listLicenseEndpointsRequest
* @return A Java Future containing the result of the ListLicenseEndpoints operation returned by the service.
* @sample AWSDeadlineAsync.ListLicenseEndpoints
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listLicenseEndpointsAsync(ListLicenseEndpointsRequest listLicenseEndpointsRequest);
/**
*
* Lists license endpoints.
*
*
* @param listLicenseEndpointsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListLicenseEndpoints operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListLicenseEndpoints
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listLicenseEndpointsAsync(ListLicenseEndpointsRequest listLicenseEndpointsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists metered products.
*
*
* @param listMeteredProductsRequest
* @return A Java Future containing the result of the ListMeteredProducts operation returned by the service.
* @sample AWSDeadlineAsync.ListMeteredProducts
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listMeteredProductsAsync(ListMeteredProductsRequest listMeteredProductsRequest);
/**
*
* Lists metered products.
*
*
* @param listMeteredProductsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMeteredProducts operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListMeteredProducts
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listMeteredProductsAsync(ListMeteredProductsRequest listMeteredProductsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of your monitors in Deadline Cloud.
*
*
* @param listMonitorsRequest
* @return A Java Future containing the result of the ListMonitors operation returned by the service.
* @sample AWSDeadlineAsync.ListMonitors
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listMonitorsAsync(ListMonitorsRequest listMonitorsRequest);
/**
*
* Gets a list of your monitors in Deadline Cloud.
*
*
* @param listMonitorsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMonitors operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListMonitors
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listMonitorsAsync(ListMonitorsRequest listMonitorsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists queue environments.
*
*
* @param listQueueEnvironmentsRequest
* @return A Java Future containing the result of the ListQueueEnvironments operation returned by the service.
* @sample AWSDeadlineAsync.ListQueueEnvironments
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listQueueEnvironmentsAsync(ListQueueEnvironmentsRequest listQueueEnvironmentsRequest);
/**
*
* Lists queue environments.
*
*
* @param listQueueEnvironmentsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListQueueEnvironments operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListQueueEnvironments
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listQueueEnvironmentsAsync(ListQueueEnvironmentsRequest listQueueEnvironmentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists queue-fleet associations.
*
*
* @param listQueueFleetAssociationsRequest
* @return A Java Future containing the result of the ListQueueFleetAssociations operation returned by the service.
* @sample AWSDeadlineAsync.ListQueueFleetAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future listQueueFleetAssociationsAsync(
ListQueueFleetAssociationsRequest listQueueFleetAssociationsRequest);
/**
*
* Lists queue-fleet associations.
*
*
* @param listQueueFleetAssociationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListQueueFleetAssociations operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListQueueFleetAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future listQueueFleetAssociationsAsync(
ListQueueFleetAssociationsRequest listQueueFleetAssociationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the members in a queue.
*
*
* @param listQueueMembersRequest
* @return A Java Future containing the result of the ListQueueMembers operation returned by the service.
* @sample AWSDeadlineAsync.ListQueueMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listQueueMembersAsync(ListQueueMembersRequest listQueueMembersRequest);
/**
*
* Lists the members in a queue.
*
*
* @param listQueueMembersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListQueueMembers operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListQueueMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listQueueMembersAsync(ListQueueMembersRequest listQueueMembersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists queues.
*
*
* @param listQueuesRequest
* @return A Java Future containing the result of the ListQueues operation returned by the service.
* @sample AWSDeadlineAsync.ListQueues
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listQueuesAsync(ListQueuesRequest listQueuesRequest);
/**
*
* Lists queues.
*
*
* @param listQueuesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListQueues operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListQueues
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listQueuesAsync(ListQueuesRequest listQueuesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists session actions.
*
*
* @param listSessionActionsRequest
* @return A Java Future containing the result of the ListSessionActions operation returned by the service.
* @sample AWSDeadlineAsync.ListSessionActions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listSessionActionsAsync(ListSessionActionsRequest listSessionActionsRequest);
/**
*
* Lists session actions.
*
*
* @param listSessionActionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSessionActions operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListSessionActions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listSessionActionsAsync(ListSessionActionsRequest listSessionActionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists sessions.
*
*
* @param listSessionsRequest
* @return A Java Future containing the result of the ListSessions operation returned by the service.
* @sample AWSDeadlineAsync.ListSessions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listSessionsAsync(ListSessionsRequest listSessionsRequest);
/**
*
* Lists sessions.
*
*
* @param listSessionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSessions operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListSessions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listSessionsAsync(ListSessionsRequest listSessionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists sessions for a worker.
*
*
* @param listSessionsForWorkerRequest
* @return A Java Future containing the result of the ListSessionsForWorker operation returned by the service.
* @sample AWSDeadlineAsync.ListSessionsForWorker
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listSessionsForWorkerAsync(ListSessionsForWorkerRequest listSessionsForWorkerRequest);
/**
*
* Lists sessions for a worker.
*
*
* @param listSessionsForWorkerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSessionsForWorker operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListSessionsForWorker
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listSessionsForWorkerAsync(ListSessionsForWorkerRequest listSessionsForWorkerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists step consumers.
*
*
* @param listStepConsumersRequest
* @return A Java Future containing the result of the ListStepConsumers operation returned by the service.
* @sample AWSDeadlineAsync.ListStepConsumers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listStepConsumersAsync(ListStepConsumersRequest listStepConsumersRequest);
/**
*
* Lists step consumers.
*
*
* @param listStepConsumersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListStepConsumers operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListStepConsumers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listStepConsumersAsync(ListStepConsumersRequest listStepConsumersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the dependencies for a step.
*
*
* @param listStepDependenciesRequest
* @return A Java Future containing the result of the ListStepDependencies operation returned by the service.
* @sample AWSDeadlineAsync.ListStepDependencies
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listStepDependenciesAsync(ListStepDependenciesRequest listStepDependenciesRequest);
/**
*
* Lists the dependencies for a step.
*
*
* @param listStepDependenciesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListStepDependencies operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListStepDependencies
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listStepDependenciesAsync(ListStepDependenciesRequest listStepDependenciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists steps for a job.
*
*
* @param listStepsRequest
* @return A Java Future containing the result of the ListSteps operation returned by the service.
* @sample AWSDeadlineAsync.ListSteps
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listStepsAsync(ListStepsRequest listStepsRequest);
/**
*
* Lists steps for a job.
*
*
* @param listStepsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSteps operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListSteps
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listStepsAsync(ListStepsRequest listStepsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists storage profiles.
*
*
* @param listStorageProfilesRequest
* @return A Java Future containing the result of the ListStorageProfiles operation returned by the service.
* @sample AWSDeadlineAsync.ListStorageProfiles
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listStorageProfilesAsync(ListStorageProfilesRequest listStorageProfilesRequest);
/**
*
* Lists storage profiles.
*
*
* @param listStorageProfilesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListStorageProfiles operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListStorageProfiles
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listStorageProfilesAsync(ListStorageProfilesRequest listStorageProfilesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists storage profiles for a queue.
*
*
* @param listStorageProfilesForQueueRequest
* @return A Java Future containing the result of the ListStorageProfilesForQueue operation returned by the service.
* @sample AWSDeadlineAsync.ListStorageProfilesForQueue
* @see AWS API Documentation
*/
java.util.concurrent.Future listStorageProfilesForQueueAsync(
ListStorageProfilesForQueueRequest listStorageProfilesForQueueRequest);
/**
*
* Lists storage profiles for a queue.
*
*
* @param listStorageProfilesForQueueRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListStorageProfilesForQueue operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListStorageProfilesForQueue
* @see AWS API Documentation
*/
java.util.concurrent.Future listStorageProfilesForQueueAsync(
ListStorageProfilesForQueueRequest listStorageProfilesForQueueRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists tags for a resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSDeadlineAsync.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists tags for a resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists tasks for a job.
*
*
* @param listTasksRequest
* @return A Java Future containing the result of the ListTasks operation returned by the service.
* @sample AWSDeadlineAsync.ListTasks
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTasksAsync(ListTasksRequest listTasksRequest);
/**
*
* Lists tasks for a job.
*
*
* @param listTasksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTasks operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListTasks
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTasksAsync(ListTasksRequest listTasksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists workers.
*
*
* @param listWorkersRequest
* @return A Java Future containing the result of the ListWorkers operation returned by the service.
* @sample AWSDeadlineAsync.ListWorkers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listWorkersAsync(ListWorkersRequest listWorkersRequest);
/**
*
* Lists workers.
*
*
* @param listWorkersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListWorkers operation returned by the service.
* @sample AWSDeadlineAsyncHandler.ListWorkers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listWorkersAsync(ListWorkersRequest listWorkersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds a metered product.
*
*
* @param putMeteredProductRequest
* @return A Java Future containing the result of the PutMeteredProduct operation returned by the service.
* @sample AWSDeadlineAsync.PutMeteredProduct
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putMeteredProductAsync(PutMeteredProductRequest putMeteredProductRequest);
/**
*
* Adds a metered product.
*
*
* @param putMeteredProductRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutMeteredProduct operation returned by the service.
* @sample AWSDeadlineAsyncHandler.PutMeteredProduct
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putMeteredProductAsync(PutMeteredProductRequest putMeteredProductRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Searches for jobs.
*
*
* @param searchJobsRequest
* @return A Java Future containing the result of the SearchJobs operation returned by the service.
* @sample AWSDeadlineAsync.SearchJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future searchJobsAsync(SearchJobsRequest searchJobsRequest);
/**
*
* Searches for jobs.
*
*
* @param searchJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchJobs operation returned by the service.
* @sample AWSDeadlineAsyncHandler.SearchJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future searchJobsAsync(SearchJobsRequest searchJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Searches for steps.
*
*
* @param searchStepsRequest
* @return A Java Future containing the result of the SearchSteps operation returned by the service.
* @sample AWSDeadlineAsync.SearchSteps
* @see AWS API
* Documentation
*/
java.util.concurrent.Future searchStepsAsync(SearchStepsRequest searchStepsRequest);
/**
*
* Searches for steps.
*
*
* @param searchStepsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchSteps operation returned by the service.
* @sample AWSDeadlineAsyncHandler.SearchSteps
* @see AWS API
* Documentation
*/
java.util.concurrent.Future searchStepsAsync(SearchStepsRequest searchStepsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Searches for tasks.
*
*
* @param searchTasksRequest
* @return A Java Future containing the result of the SearchTasks operation returned by the service.
* @sample AWSDeadlineAsync.SearchTasks
* @see AWS API
* Documentation
*/
java.util.concurrent.Future searchTasksAsync(SearchTasksRequest searchTasksRequest);
/**
*