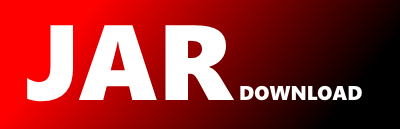
com.amazonaws.services.deadline.model.GetStepResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-deadline Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.deadline.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetStepResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The date and time the resource was created.
*
*/
private java.util.Date createdAt;
/**
*
* The user or system that created this resource.
*
*/
private String createdBy;
/**
*
* The number of dependencies in the step.
*
*/
private DependencyCounts dependencyCounts;
/**
*
* The description of the step.
*
*/
private String description;
/**
*
* The date and time the resource ended running.
*
*/
private java.util.Date endedAt;
/**
*
* The life cycle status of the step.
*
*/
private String lifecycleStatus;
/**
*
* A message that describes the lifecycle status of the step.
*
*/
private String lifecycleStatusMessage;
/**
*
* The name of the step.
*
*/
private String name;
/**
*
* A list of step parameters and the combination expression for the step.
*
*/
private ParameterSpace parameterSpace;
/**
*
* The required capabilities of the step.
*
*/
private StepRequiredCapabilities requiredCapabilities;
/**
*
* The date and time the resource started running.
*
*/
private java.util.Date startedAt;
/**
*
* The step ID.
*
*/
private String stepId;
/**
*
* The task status with which the job started.
*
*/
private String targetTaskRunStatus;
/**
*
* The task run status for the job.
*
*/
private String taskRunStatus;
/**
*
* The number of tasks running on the job.
*
*/
private java.util.Map taskRunStatusCounts;
/**
*
* The date and time the resource was updated.
*
*/
private java.util.Date updatedAt;
/**
*
* The user or system that updated this resource.
*
*/
private String updatedBy;
/**
*
* The date and time the resource was created.
*
*
* @param createdAt
* The date and time the resource was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The date and time the resource was created.
*
*
* @return The date and time the resource was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The date and time the resource was created.
*
*
* @param createdAt
* The date and time the resource was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetStepResult withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The user or system that created this resource.
*
*
* @param createdBy
* The user or system that created this resource.
*/
public void setCreatedBy(String createdBy) {
this.createdBy = createdBy;
}
/**
*
* The user or system that created this resource.
*
*
* @return The user or system that created this resource.
*/
public String getCreatedBy() {
return this.createdBy;
}
/**
*
* The user or system that created this resource.
*
*
* @param createdBy
* The user or system that created this resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetStepResult withCreatedBy(String createdBy) {
setCreatedBy(createdBy);
return this;
}
/**
*
* The number of dependencies in the step.
*
*
* @param dependencyCounts
* The number of dependencies in the step.
*/
public void setDependencyCounts(DependencyCounts dependencyCounts) {
this.dependencyCounts = dependencyCounts;
}
/**
*
* The number of dependencies in the step.
*
*
* @return The number of dependencies in the step.
*/
public DependencyCounts getDependencyCounts() {
return this.dependencyCounts;
}
/**
*
* The number of dependencies in the step.
*
*
* @param dependencyCounts
* The number of dependencies in the step.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetStepResult withDependencyCounts(DependencyCounts dependencyCounts) {
setDependencyCounts(dependencyCounts);
return this;
}
/**
*
* The description of the step.
*
*
* @param description
* The description of the step.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The description of the step.
*
*
* @return The description of the step.
*/
public String getDescription() {
return this.description;
}
/**
*
* The description of the step.
*
*
* @param description
* The description of the step.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetStepResult withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The date and time the resource ended running.
*
*
* @param endedAt
* The date and time the resource ended running.
*/
public void setEndedAt(java.util.Date endedAt) {
this.endedAt = endedAt;
}
/**
*
* The date and time the resource ended running.
*
*
* @return The date and time the resource ended running.
*/
public java.util.Date getEndedAt() {
return this.endedAt;
}
/**
*
* The date and time the resource ended running.
*
*
* @param endedAt
* The date and time the resource ended running.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetStepResult withEndedAt(java.util.Date endedAt) {
setEndedAt(endedAt);
return this;
}
/**
*
* The life cycle status of the step.
*
*
* @param lifecycleStatus
* The life cycle status of the step.
* @see StepLifecycleStatus
*/
public void setLifecycleStatus(String lifecycleStatus) {
this.lifecycleStatus = lifecycleStatus;
}
/**
*
* The life cycle status of the step.
*
*
* @return The life cycle status of the step.
* @see StepLifecycleStatus
*/
public String getLifecycleStatus() {
return this.lifecycleStatus;
}
/**
*
* The life cycle status of the step.
*
*
* @param lifecycleStatus
* The life cycle status of the step.
* @return Returns a reference to this object so that method calls can be chained together.
* @see StepLifecycleStatus
*/
public GetStepResult withLifecycleStatus(String lifecycleStatus) {
setLifecycleStatus(lifecycleStatus);
return this;
}
/**
*
* The life cycle status of the step.
*
*
* @param lifecycleStatus
* The life cycle status of the step.
* @return Returns a reference to this object so that method calls can be chained together.
* @see StepLifecycleStatus
*/
public GetStepResult withLifecycleStatus(StepLifecycleStatus lifecycleStatus) {
this.lifecycleStatus = lifecycleStatus.toString();
return this;
}
/**
*
* A message that describes the lifecycle status of the step.
*
*
* @param lifecycleStatusMessage
* A message that describes the lifecycle status of the step.
*/
public void setLifecycleStatusMessage(String lifecycleStatusMessage) {
this.lifecycleStatusMessage = lifecycleStatusMessage;
}
/**
*
* A message that describes the lifecycle status of the step.
*
*
* @return A message that describes the lifecycle status of the step.
*/
public String getLifecycleStatusMessage() {
return this.lifecycleStatusMessage;
}
/**
*
* A message that describes the lifecycle status of the step.
*
*
* @param lifecycleStatusMessage
* A message that describes the lifecycle status of the step.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetStepResult withLifecycleStatusMessage(String lifecycleStatusMessage) {
setLifecycleStatusMessage(lifecycleStatusMessage);
return this;
}
/**
*
* The name of the step.
*
*
* @param name
* The name of the step.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the step.
*
*
* @return The name of the step.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the step.
*
*
* @param name
* The name of the step.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetStepResult withName(String name) {
setName(name);
return this;
}
/**
*
* A list of step parameters and the combination expression for the step.
*
*
* @param parameterSpace
* A list of step parameters and the combination expression for the step.
*/
public void setParameterSpace(ParameterSpace parameterSpace) {
this.parameterSpace = parameterSpace;
}
/**
*
* A list of step parameters and the combination expression for the step.
*
*
* @return A list of step parameters and the combination expression for the step.
*/
public ParameterSpace getParameterSpace() {
return this.parameterSpace;
}
/**
*
* A list of step parameters and the combination expression for the step.
*
*
* @param parameterSpace
* A list of step parameters and the combination expression for the step.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetStepResult withParameterSpace(ParameterSpace parameterSpace) {
setParameterSpace(parameterSpace);
return this;
}
/**
*
* The required capabilities of the step.
*
*
* @param requiredCapabilities
* The required capabilities of the step.
*/
public void setRequiredCapabilities(StepRequiredCapabilities requiredCapabilities) {
this.requiredCapabilities = requiredCapabilities;
}
/**
*
* The required capabilities of the step.
*
*
* @return The required capabilities of the step.
*/
public StepRequiredCapabilities getRequiredCapabilities() {
return this.requiredCapabilities;
}
/**
*
* The required capabilities of the step.
*
*
* @param requiredCapabilities
* The required capabilities of the step.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetStepResult withRequiredCapabilities(StepRequiredCapabilities requiredCapabilities) {
setRequiredCapabilities(requiredCapabilities);
return this;
}
/**
*
* The date and time the resource started running.
*
*
* @param startedAt
* The date and time the resource started running.
*/
public void setStartedAt(java.util.Date startedAt) {
this.startedAt = startedAt;
}
/**
*
* The date and time the resource started running.
*
*
* @return The date and time the resource started running.
*/
public java.util.Date getStartedAt() {
return this.startedAt;
}
/**
*
* The date and time the resource started running.
*
*
* @param startedAt
* The date and time the resource started running.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetStepResult withStartedAt(java.util.Date startedAt) {
setStartedAt(startedAt);
return this;
}
/**
*
* The step ID.
*
*
* @param stepId
* The step ID.
*/
public void setStepId(String stepId) {
this.stepId = stepId;
}
/**
*
* The step ID.
*
*
* @return The step ID.
*/
public String getStepId() {
return this.stepId;
}
/**
*
* The step ID.
*
*
* @param stepId
* The step ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetStepResult withStepId(String stepId) {
setStepId(stepId);
return this;
}
/**
*
* The task status with which the job started.
*
*
* @param targetTaskRunStatus
* The task status with which the job started.
* @see StepTargetTaskRunStatus
*/
public void setTargetTaskRunStatus(String targetTaskRunStatus) {
this.targetTaskRunStatus = targetTaskRunStatus;
}
/**
*
* The task status with which the job started.
*
*
* @return The task status with which the job started.
* @see StepTargetTaskRunStatus
*/
public String getTargetTaskRunStatus() {
return this.targetTaskRunStatus;
}
/**
*
* The task status with which the job started.
*
*
* @param targetTaskRunStatus
* The task status with which the job started.
* @return Returns a reference to this object so that method calls can be chained together.
* @see StepTargetTaskRunStatus
*/
public GetStepResult withTargetTaskRunStatus(String targetTaskRunStatus) {
setTargetTaskRunStatus(targetTaskRunStatus);
return this;
}
/**
*
* The task status with which the job started.
*
*
* @param targetTaskRunStatus
* The task status with which the job started.
* @return Returns a reference to this object so that method calls can be chained together.
* @see StepTargetTaskRunStatus
*/
public GetStepResult withTargetTaskRunStatus(StepTargetTaskRunStatus targetTaskRunStatus) {
this.targetTaskRunStatus = targetTaskRunStatus.toString();
return this;
}
/**
*
* The task run status for the job.
*
*
* @param taskRunStatus
* The task run status for the job.
* @see TaskRunStatus
*/
public void setTaskRunStatus(String taskRunStatus) {
this.taskRunStatus = taskRunStatus;
}
/**
*
* The task run status for the job.
*
*
* @return The task run status for the job.
* @see TaskRunStatus
*/
public String getTaskRunStatus() {
return this.taskRunStatus;
}
/**
*
* The task run status for the job.
*
*
* @param taskRunStatus
* The task run status for the job.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TaskRunStatus
*/
public GetStepResult withTaskRunStatus(String taskRunStatus) {
setTaskRunStatus(taskRunStatus);
return this;
}
/**
*
* The task run status for the job.
*
*
* @param taskRunStatus
* The task run status for the job.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TaskRunStatus
*/
public GetStepResult withTaskRunStatus(TaskRunStatus taskRunStatus) {
this.taskRunStatus = taskRunStatus.toString();
return this;
}
/**
*
* The number of tasks running on the job.
*
*
* @return The number of tasks running on the job.
*/
public java.util.Map getTaskRunStatusCounts() {
return taskRunStatusCounts;
}
/**
*
* The number of tasks running on the job.
*
*
* @param taskRunStatusCounts
* The number of tasks running on the job.
*/
public void setTaskRunStatusCounts(java.util.Map taskRunStatusCounts) {
this.taskRunStatusCounts = taskRunStatusCounts;
}
/**
*
* The number of tasks running on the job.
*
*
* @param taskRunStatusCounts
* The number of tasks running on the job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetStepResult withTaskRunStatusCounts(java.util.Map taskRunStatusCounts) {
setTaskRunStatusCounts(taskRunStatusCounts);
return this;
}
/**
* Add a single TaskRunStatusCounts entry
*
* @see GetStepResult#withTaskRunStatusCounts
* @returns a reference to this object so that method calls can be chained together.
*/
public GetStepResult addTaskRunStatusCountsEntry(String key, Integer value) {
if (null == this.taskRunStatusCounts) {
this.taskRunStatusCounts = new java.util.HashMap();
}
if (this.taskRunStatusCounts.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.taskRunStatusCounts.put(key, value);
return this;
}
/**
* Removes all the entries added into TaskRunStatusCounts.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetStepResult clearTaskRunStatusCountsEntries() {
this.taskRunStatusCounts = null;
return this;
}
/**
*
* The date and time the resource was updated.
*
*
* @param updatedAt
* The date and time the resource was updated.
*/
public void setUpdatedAt(java.util.Date updatedAt) {
this.updatedAt = updatedAt;
}
/**
*
* The date and time the resource was updated.
*
*
* @return The date and time the resource was updated.
*/
public java.util.Date getUpdatedAt() {
return this.updatedAt;
}
/**
*
* The date and time the resource was updated.
*
*
* @param updatedAt
* The date and time the resource was updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetStepResult withUpdatedAt(java.util.Date updatedAt) {
setUpdatedAt(updatedAt);
return this;
}
/**
*
* The user or system that updated this resource.
*
*
* @param updatedBy
* The user or system that updated this resource.
*/
public void setUpdatedBy(String updatedBy) {
this.updatedBy = updatedBy;
}
/**
*
* The user or system that updated this resource.
*
*
* @return The user or system that updated this resource.
*/
public String getUpdatedBy() {
return this.updatedBy;
}
/**
*
* The user or system that updated this resource.
*
*
* @param updatedBy
* The user or system that updated this resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetStepResult withUpdatedBy(String updatedBy) {
setUpdatedBy(updatedBy);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getCreatedBy() != null)
sb.append("CreatedBy: ").append(getCreatedBy()).append(",");
if (getDependencyCounts() != null)
sb.append("DependencyCounts: ").append(getDependencyCounts()).append(",");
if (getDescription() != null)
sb.append("Description: ").append("***Sensitive Data Redacted***").append(",");
if (getEndedAt() != null)
sb.append("EndedAt: ").append(getEndedAt()).append(",");
if (getLifecycleStatus() != null)
sb.append("LifecycleStatus: ").append(getLifecycleStatus()).append(",");
if (getLifecycleStatusMessage() != null)
sb.append("LifecycleStatusMessage: ").append(getLifecycleStatusMessage()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getParameterSpace() != null)
sb.append("ParameterSpace: ").append(getParameterSpace()).append(",");
if (getRequiredCapabilities() != null)
sb.append("RequiredCapabilities: ").append(getRequiredCapabilities()).append(",");
if (getStartedAt() != null)
sb.append("StartedAt: ").append(getStartedAt()).append(",");
if (getStepId() != null)
sb.append("StepId: ").append(getStepId()).append(",");
if (getTargetTaskRunStatus() != null)
sb.append("TargetTaskRunStatus: ").append(getTargetTaskRunStatus()).append(",");
if (getTaskRunStatus() != null)
sb.append("TaskRunStatus: ").append(getTaskRunStatus()).append(",");
if (getTaskRunStatusCounts() != null)
sb.append("TaskRunStatusCounts: ").append(getTaskRunStatusCounts()).append(",");
if (getUpdatedAt() != null)
sb.append("UpdatedAt: ").append(getUpdatedAt()).append(",");
if (getUpdatedBy() != null)
sb.append("UpdatedBy: ").append(getUpdatedBy());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetStepResult == false)
return false;
GetStepResult other = (GetStepResult) obj;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getCreatedBy() == null ^ this.getCreatedBy() == null)
return false;
if (other.getCreatedBy() != null && other.getCreatedBy().equals(this.getCreatedBy()) == false)
return false;
if (other.getDependencyCounts() == null ^ this.getDependencyCounts() == null)
return false;
if (other.getDependencyCounts() != null && other.getDependencyCounts().equals(this.getDependencyCounts()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getEndedAt() == null ^ this.getEndedAt() == null)
return false;
if (other.getEndedAt() != null && other.getEndedAt().equals(this.getEndedAt()) == false)
return false;
if (other.getLifecycleStatus() == null ^ this.getLifecycleStatus() == null)
return false;
if (other.getLifecycleStatus() != null && other.getLifecycleStatus().equals(this.getLifecycleStatus()) == false)
return false;
if (other.getLifecycleStatusMessage() == null ^ this.getLifecycleStatusMessage() == null)
return false;
if (other.getLifecycleStatusMessage() != null && other.getLifecycleStatusMessage().equals(this.getLifecycleStatusMessage()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getParameterSpace() == null ^ this.getParameterSpace() == null)
return false;
if (other.getParameterSpace() != null && other.getParameterSpace().equals(this.getParameterSpace()) == false)
return false;
if (other.getRequiredCapabilities() == null ^ this.getRequiredCapabilities() == null)
return false;
if (other.getRequiredCapabilities() != null && other.getRequiredCapabilities().equals(this.getRequiredCapabilities()) == false)
return false;
if (other.getStartedAt() == null ^ this.getStartedAt() == null)
return false;
if (other.getStartedAt() != null && other.getStartedAt().equals(this.getStartedAt()) == false)
return false;
if (other.getStepId() == null ^ this.getStepId() == null)
return false;
if (other.getStepId() != null && other.getStepId().equals(this.getStepId()) == false)
return false;
if (other.getTargetTaskRunStatus() == null ^ this.getTargetTaskRunStatus() == null)
return false;
if (other.getTargetTaskRunStatus() != null && other.getTargetTaskRunStatus().equals(this.getTargetTaskRunStatus()) == false)
return false;
if (other.getTaskRunStatus() == null ^ this.getTaskRunStatus() == null)
return false;
if (other.getTaskRunStatus() != null && other.getTaskRunStatus().equals(this.getTaskRunStatus()) == false)
return false;
if (other.getTaskRunStatusCounts() == null ^ this.getTaskRunStatusCounts() == null)
return false;
if (other.getTaskRunStatusCounts() != null && other.getTaskRunStatusCounts().equals(this.getTaskRunStatusCounts()) == false)
return false;
if (other.getUpdatedAt() == null ^ this.getUpdatedAt() == null)
return false;
if (other.getUpdatedAt() != null && other.getUpdatedAt().equals(this.getUpdatedAt()) == false)
return false;
if (other.getUpdatedBy() == null ^ this.getUpdatedBy() == null)
return false;
if (other.getUpdatedBy() != null && other.getUpdatedBy().equals(this.getUpdatedBy()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getCreatedBy() == null) ? 0 : getCreatedBy().hashCode());
hashCode = prime * hashCode + ((getDependencyCounts() == null) ? 0 : getDependencyCounts().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getEndedAt() == null) ? 0 : getEndedAt().hashCode());
hashCode = prime * hashCode + ((getLifecycleStatus() == null) ? 0 : getLifecycleStatus().hashCode());
hashCode = prime * hashCode + ((getLifecycleStatusMessage() == null) ? 0 : getLifecycleStatusMessage().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getParameterSpace() == null) ? 0 : getParameterSpace().hashCode());
hashCode = prime * hashCode + ((getRequiredCapabilities() == null) ? 0 : getRequiredCapabilities().hashCode());
hashCode = prime * hashCode + ((getStartedAt() == null) ? 0 : getStartedAt().hashCode());
hashCode = prime * hashCode + ((getStepId() == null) ? 0 : getStepId().hashCode());
hashCode = prime * hashCode + ((getTargetTaskRunStatus() == null) ? 0 : getTargetTaskRunStatus().hashCode());
hashCode = prime * hashCode + ((getTaskRunStatus() == null) ? 0 : getTaskRunStatus().hashCode());
hashCode = prime * hashCode + ((getTaskRunStatusCounts() == null) ? 0 : getTaskRunStatusCounts().hashCode());
hashCode = prime * hashCode + ((getUpdatedAt() == null) ? 0 : getUpdatedAt().hashCode());
hashCode = prime * hashCode + ((getUpdatedBy() == null) ? 0 : getUpdatedBy().hashCode());
return hashCode;
}
@Override
public GetStepResult clone() {
try {
return (GetStepResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}