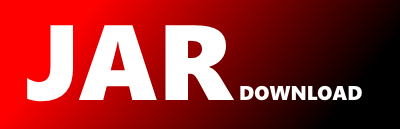
com.amazonaws.services.detective.AmazonDetectiveAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-detective Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.detective;
import javax.annotation.Generated;
import com.amazonaws.services.detective.model.*;
/**
* Interface for accessing Amazon Detective asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.detective.AbstractAmazonDetectiveAsync} instead.
*
*
*
* Detective uses machine learning and purpose-built visualizations to help you to analyze and investigate security
* issues across your Amazon Web Services (Amazon Web Services) workloads. Detective automatically extracts time-based
* events such as login attempts, API calls, and network traffic from CloudTrail and Amazon Virtual Private Cloud
* (Amazon VPC) flow logs. It also extracts findings detected by Amazon GuardDuty.
*
*
* The Detective API primarily supports the creation and management of behavior graphs. A behavior graph contains the
* extracted data from a set of member accounts, and is created and managed by an administrator account.
*
*
* To add a member account to the behavior graph, the administrator account sends an invitation to the account. When the
* account accepts the invitation, it becomes a member account in the behavior graph.
*
*
* Detective is also integrated with Organizations. The organization management account designates the Detective
* administrator account for the organization. That account becomes the administrator account for the organization
* behavior graph. The Detective administrator account is also the delegated administrator account for Detective in
* Organizations.
*
*
* The Detective administrator account can enable any organization account as a member account in the organization
* behavior graph. The organization accounts do not receive invitations. The Detective administrator account can also
* invite other accounts to the organization behavior graph.
*
*
* Every behavior graph is specific to a Region. You can only use the API to manage behavior graphs that belong to the
* Region that is associated with the currently selected endpoint.
*
*
* The administrator account for a behavior graph can use the Detective API to do the following:
*
*
* -
*
* Enable and disable Detective. Enabling Detective creates a new behavior graph.
*
*
* -
*
* View the list of member accounts in a behavior graph.
*
*
* -
*
* Add member accounts to a behavior graph.
*
*
* -
*
* Remove member accounts from a behavior graph.
*
*
* -
*
* Apply tags to a behavior graph.
*
*
*
*
* The organization management account can use the Detective API to select the delegated administrator for Detective.
*
*
* The Detective administrator account for an organization can use the Detective API to do the following:
*
*
* -
*
* Perform all of the functions of an administrator account.
*
*
* -
*
* Determine whether to automatically enable new organization accounts as member accounts in the organization behavior
* graph.
*
*
*
*
* An invited member account can use the Detective API to do the following:
*
*
* -
*
* View the list of behavior graphs that they are invited to.
*
*
* -
*
* Accept an invitation to contribute to a behavior graph.
*
*
* -
*
* Decline an invitation to contribute to a behavior graph.
*
*
* -
*
* Remove their account from a behavior graph.
*
*
*
*
* All API actions are logged as CloudTrail events. See Logging Detective API
* Calls with CloudTrail.
*
*
*
* We replaced the term "master account" with the term "administrator account." An administrator account is used to
* centrally manage multiple accounts. In the case of Detective, the administrator account manages the accounts in their
* behavior graph.
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonDetectiveAsync extends AmazonDetective {
/**
*
* Accepts an invitation for the member account to contribute data to a behavior graph. This operation can only be
* called by an invited member account.
*
*
* The request provides the ARN of behavior graph.
*
*
* The member account status in the graph must be INVITED
.
*
*
* @param acceptInvitationRequest
* @return A Java Future containing the result of the AcceptInvitation operation returned by the service.
* @sample AmazonDetectiveAsync.AcceptInvitation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future acceptInvitationAsync(AcceptInvitationRequest acceptInvitationRequest);
/**
*
* Accepts an invitation for the member account to contribute data to a behavior graph. This operation can only be
* called by an invited member account.
*
*
* The request provides the ARN of behavior graph.
*
*
* The member account status in the graph must be INVITED
.
*
*
* @param acceptInvitationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AcceptInvitation operation returned by the service.
* @sample AmazonDetectiveAsyncHandler.AcceptInvitation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future acceptInvitationAsync(AcceptInvitationRequest acceptInvitationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets data source package information for the behavior graph.
*
*
* @param batchGetGraphMemberDatasourcesRequest
* @return A Java Future containing the result of the BatchGetGraphMemberDatasources operation returned by the
* service.
* @sample AmazonDetectiveAsync.BatchGetGraphMemberDatasources
* @see AWS API Documentation
*/
java.util.concurrent.Future batchGetGraphMemberDatasourcesAsync(
BatchGetGraphMemberDatasourcesRequest batchGetGraphMemberDatasourcesRequest);
/**
*
* Gets data source package information for the behavior graph.
*
*
* @param batchGetGraphMemberDatasourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchGetGraphMemberDatasources operation returned by the
* service.
* @sample AmazonDetectiveAsyncHandler.BatchGetGraphMemberDatasources
* @see AWS API Documentation
*/
java.util.concurrent.Future batchGetGraphMemberDatasourcesAsync(
BatchGetGraphMemberDatasourcesRequest batchGetGraphMemberDatasourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information on the data source package history for an account.
*
*
* @param batchGetMembershipDatasourcesRequest
* @return A Java Future containing the result of the BatchGetMembershipDatasources operation returned by the
* service.
* @sample AmazonDetectiveAsync.BatchGetMembershipDatasources
* @see AWS API Documentation
*/
java.util.concurrent.Future batchGetMembershipDatasourcesAsync(
BatchGetMembershipDatasourcesRequest batchGetMembershipDatasourcesRequest);
/**
*
* Gets information on the data source package history for an account.
*
*
* @param batchGetMembershipDatasourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchGetMembershipDatasources operation returned by the
* service.
* @sample AmazonDetectiveAsyncHandler.BatchGetMembershipDatasources
* @see AWS API Documentation
*/
java.util.concurrent.Future batchGetMembershipDatasourcesAsync(
BatchGetMembershipDatasourcesRequest batchGetMembershipDatasourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new behavior graph for the calling account, and sets that account as the administrator account. This
* operation is called by the account that is enabling Detective.
*
*
* Before you try to enable Detective, make sure that your account has been enrolled in Amazon GuardDuty for at
* least 48 hours. If you do not meet this requirement, you cannot enable Detective. If you do meet the GuardDuty
* prerequisite, then when you make the request to enable Detective, it checks whether your data volume is within
* the Detective quota. If it exceeds the quota, then you cannot enable Detective.
*
*
* The operation also enables Detective for the calling account in the currently selected Region. It returns the ARN
* of the new behavior graph.
*
*
* CreateGraph
triggers a process to create the corresponding data tables for the new behavior graph.
*
*
* An account can only be the administrator account for one behavior graph within a Region. If the same account
* calls CreateGraph
with the same administrator account, it always returns the same behavior graph
* ARN. It does not create a new behavior graph.
*
*
* @param createGraphRequest
* @return A Java Future containing the result of the CreateGraph operation returned by the service.
* @sample AmazonDetectiveAsync.CreateGraph
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createGraphAsync(CreateGraphRequest createGraphRequest);
/**
*
* Creates a new behavior graph for the calling account, and sets that account as the administrator account. This
* operation is called by the account that is enabling Detective.
*
*
* Before you try to enable Detective, make sure that your account has been enrolled in Amazon GuardDuty for at
* least 48 hours. If you do not meet this requirement, you cannot enable Detective. If you do meet the GuardDuty
* prerequisite, then when you make the request to enable Detective, it checks whether your data volume is within
* the Detective quota. If it exceeds the quota, then you cannot enable Detective.
*
*
* The operation also enables Detective for the calling account in the currently selected Region. It returns the ARN
* of the new behavior graph.
*
*
* CreateGraph
triggers a process to create the corresponding data tables for the new behavior graph.
*
*
* An account can only be the administrator account for one behavior graph within a Region. If the same account
* calls CreateGraph
with the same administrator account, it always returns the same behavior graph
* ARN. It does not create a new behavior graph.
*
*
* @param createGraphRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateGraph operation returned by the service.
* @sample AmazonDetectiveAsyncHandler.CreateGraph
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createGraphAsync(CreateGraphRequest createGraphRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* CreateMembers
is used to send invitations to accounts. For the organization behavior graph, the
* Detective administrator account uses CreateMembers
to enable organization accounts as member
* accounts.
*
*
* For invited accounts, CreateMembers
sends a request to invite the specified Amazon Web Services
* accounts to be member accounts in the behavior graph. This operation can only be called by the administrator
* account for a behavior graph.
*
*
* CreateMembers
verifies the accounts and then invites the verified accounts. The administrator can
* optionally specify to not send invitation emails to the member accounts. This would be used when the
* administrator manages their member accounts centrally.
*
*
* For organization accounts in the organization behavior graph, CreateMembers
attempts to enable the
* accounts. The organization accounts do not receive invitations.
*
*
* The request provides the behavior graph ARN and the list of accounts to invite or to enable.
*
*
* The response separates the requested accounts into two lists:
*
*
* -
*
* The accounts that CreateMembers
was able to process. For invited accounts, includes member accounts
* that are being verified, that have passed verification and are to be invited, and that have failed verification.
* For organization accounts in the organization behavior graph, includes accounts that can be enabled and that
* cannot be enabled.
*
*
* -
*
* The accounts that CreateMembers
was unable to process. This list includes accounts that were already
* invited to be member accounts in the behavior graph.
*
*
*
*
* @param createMembersRequest
* @return A Java Future containing the result of the CreateMembers operation returned by the service.
* @sample AmazonDetectiveAsync.CreateMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createMembersAsync(CreateMembersRequest createMembersRequest);
/**
*
* CreateMembers
is used to send invitations to accounts. For the organization behavior graph, the
* Detective administrator account uses CreateMembers
to enable organization accounts as member
* accounts.
*
*
* For invited accounts, CreateMembers
sends a request to invite the specified Amazon Web Services
* accounts to be member accounts in the behavior graph. This operation can only be called by the administrator
* account for a behavior graph.
*
*
* CreateMembers
verifies the accounts and then invites the verified accounts. The administrator can
* optionally specify to not send invitation emails to the member accounts. This would be used when the
* administrator manages their member accounts centrally.
*
*
* For organization accounts in the organization behavior graph, CreateMembers
attempts to enable the
* accounts. The organization accounts do not receive invitations.
*
*
* The request provides the behavior graph ARN and the list of accounts to invite or to enable.
*
*
* The response separates the requested accounts into two lists:
*
*
* -
*
* The accounts that CreateMembers
was able to process. For invited accounts, includes member accounts
* that are being verified, that have passed verification and are to be invited, and that have failed verification.
* For organization accounts in the organization behavior graph, includes accounts that can be enabled and that
* cannot be enabled.
*
*
* -
*
* The accounts that CreateMembers
was unable to process. This list includes accounts that were already
* invited to be member accounts in the behavior graph.
*
*
*
*
* @param createMembersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMembers operation returned by the service.
* @sample AmazonDetectiveAsyncHandler.CreateMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createMembersAsync(CreateMembersRequest createMembersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disables the specified behavior graph and queues it to be deleted. This operation removes the behavior graph from
* each member account's list of behavior graphs.
*
*
* DeleteGraph
can only be called by the administrator account for a behavior graph.
*
*
* @param deleteGraphRequest
* @return A Java Future containing the result of the DeleteGraph operation returned by the service.
* @sample AmazonDetectiveAsync.DeleteGraph
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteGraphAsync(DeleteGraphRequest deleteGraphRequest);
/**
*
* Disables the specified behavior graph and queues it to be deleted. This operation removes the behavior graph from
* each member account's list of behavior graphs.
*
*
* DeleteGraph
can only be called by the administrator account for a behavior graph.
*
*
* @param deleteGraphRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteGraph operation returned by the service.
* @sample AmazonDetectiveAsyncHandler.DeleteGraph
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteGraphAsync(DeleteGraphRequest deleteGraphRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified member accounts from the behavior graph. The removed accounts no longer contribute data to
* the behavior graph. This operation can only be called by the administrator account for the behavior graph.
*
*
* For invited accounts, the removed accounts are deleted from the list of accounts in the behavior graph. To
* restore the account, the administrator account must send another invitation.
*
*
* For organization accounts in the organization behavior graph, the Detective administrator account can always
* enable the organization account again. Organization accounts that are not enabled as member accounts are not
* included in the ListMembers
results for the organization behavior graph.
*
*
* An administrator account cannot use DeleteMembers
to remove their own account from the behavior
* graph. To disable a behavior graph, the administrator account uses the DeleteGraph
API method.
*
*
* @param deleteMembersRequest
* @return A Java Future containing the result of the DeleteMembers operation returned by the service.
* @sample AmazonDetectiveAsync.DeleteMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteMembersAsync(DeleteMembersRequest deleteMembersRequest);
/**
*
* Removes the specified member accounts from the behavior graph. The removed accounts no longer contribute data to
* the behavior graph. This operation can only be called by the administrator account for the behavior graph.
*
*
* For invited accounts, the removed accounts are deleted from the list of accounts in the behavior graph. To
* restore the account, the administrator account must send another invitation.
*
*
* For organization accounts in the organization behavior graph, the Detective administrator account can always
* enable the organization account again. Organization accounts that are not enabled as member accounts are not
* included in the ListMembers
results for the organization behavior graph.
*
*
* An administrator account cannot use DeleteMembers
to remove their own account from the behavior
* graph. To disable a behavior graph, the administrator account uses the DeleteGraph
API method.
*
*
* @param deleteMembersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMembers operation returned by the service.
* @sample AmazonDetectiveAsyncHandler.DeleteMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteMembersAsync(DeleteMembersRequest deleteMembersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the configuration for the organization behavior graph. Currently indicates whether to
* automatically enable new organization accounts as member accounts.
*
*
* Can only be called by the Detective administrator account for the organization.
*
*
* @param describeOrganizationConfigurationRequest
* @return A Java Future containing the result of the DescribeOrganizationConfiguration operation returned by the
* service.
* @sample AmazonDetectiveAsync.DescribeOrganizationConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeOrganizationConfigurationAsync(
DescribeOrganizationConfigurationRequest describeOrganizationConfigurationRequest);
/**
*
* Returns information about the configuration for the organization behavior graph. Currently indicates whether to
* automatically enable new organization accounts as member accounts.
*
*
* Can only be called by the Detective administrator account for the organization.
*
*
* @param describeOrganizationConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeOrganizationConfiguration operation returned by the
* service.
* @sample AmazonDetectiveAsyncHandler.DescribeOrganizationConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeOrganizationConfigurationAsync(
DescribeOrganizationConfigurationRequest describeOrganizationConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the Detective administrator account in the current Region. Deletes the organization behavior graph.
*
*
* Can only be called by the organization management account.
*
*
* Removing the Detective administrator account does not affect the delegated administrator account for Detective in
* Organizations.
*
*
* To remove the delegated administrator account in Organizations, use the Organizations API. Removing the delegated
* administrator account also removes the Detective administrator account in all Regions, except for Regions where
* the Detective administrator account is the organization management account.
*
*
* @param disableOrganizationAdminAccountRequest
* @return A Java Future containing the result of the DisableOrganizationAdminAccount operation returned by the
* service.
* @sample AmazonDetectiveAsync.DisableOrganizationAdminAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future disableOrganizationAdminAccountAsync(
DisableOrganizationAdminAccountRequest disableOrganizationAdminAccountRequest);
/**
*
* Removes the Detective administrator account in the current Region. Deletes the organization behavior graph.
*
*
* Can only be called by the organization management account.
*
*
* Removing the Detective administrator account does not affect the delegated administrator account for Detective in
* Organizations.
*
*
* To remove the delegated administrator account in Organizations, use the Organizations API. Removing the delegated
* administrator account also removes the Detective administrator account in all Regions, except for Regions where
* the Detective administrator account is the organization management account.
*
*
* @param disableOrganizationAdminAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisableOrganizationAdminAccount operation returned by the
* service.
* @sample AmazonDetectiveAsyncHandler.DisableOrganizationAdminAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future disableOrganizationAdminAccountAsync(
DisableOrganizationAdminAccountRequest disableOrganizationAdminAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the member account from the specified behavior graph. This operation can only be called by an invited
* member account that has the ENABLED
status.
*
*
* DisassociateMembership
cannot be called by an organization account in the organization behavior
* graph. For the organization behavior graph, the Detective administrator account determines which organization
* accounts to enable or disable as member accounts.
*
*
* @param disassociateMembershipRequest
* @return A Java Future containing the result of the DisassociateMembership operation returned by the service.
* @sample AmazonDetectiveAsync.DisassociateMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateMembershipAsync(DisassociateMembershipRequest disassociateMembershipRequest);
/**
*
* Removes the member account from the specified behavior graph. This operation can only be called by an invited
* member account that has the ENABLED
status.
*
*
* DisassociateMembership
cannot be called by an organization account in the organization behavior
* graph. For the organization behavior graph, the Detective administrator account determines which organization
* accounts to enable or disable as member accounts.
*
*
* @param disassociateMembershipRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateMembership operation returned by the service.
* @sample AmazonDetectiveAsyncHandler.DisassociateMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateMembershipAsync(DisassociateMembershipRequest disassociateMembershipRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Designates the Detective administrator account for the organization in the current Region.
*
*
* If the account does not have Detective enabled, then enables Detective for that account and creates a new
* behavior graph.
*
*
* Can only be called by the organization management account.
*
*
* If the organization has a delegated administrator account in Organizations, then the Detective administrator
* account must be either the delegated administrator account or the organization management account.
*
*
* If the organization does not have a delegated administrator account in Organizations, then you can choose any
* account in the organization. If you choose an account other than the organization management account, Detective
* calls Organizations to make that account the delegated administrator account for Detective. The organization
* management account cannot be the delegated administrator account.
*
*
* @param enableOrganizationAdminAccountRequest
* @return A Java Future containing the result of the EnableOrganizationAdminAccount operation returned by the
* service.
* @sample AmazonDetectiveAsync.EnableOrganizationAdminAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future enableOrganizationAdminAccountAsync(
EnableOrganizationAdminAccountRequest enableOrganizationAdminAccountRequest);
/**
*
* Designates the Detective administrator account for the organization in the current Region.
*
*
* If the account does not have Detective enabled, then enables Detective for that account and creates a new
* behavior graph.
*
*
* Can only be called by the organization management account.
*
*
* If the organization has a delegated administrator account in Organizations, then the Detective administrator
* account must be either the delegated administrator account or the organization management account.
*
*
* If the organization does not have a delegated administrator account in Organizations, then you can choose any
* account in the organization. If you choose an account other than the organization management account, Detective
* calls Organizations to make that account the delegated administrator account for Detective. The organization
* management account cannot be the delegated administrator account.
*
*
* @param enableOrganizationAdminAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the EnableOrganizationAdminAccount operation returned by the
* service.
* @sample AmazonDetectiveAsyncHandler.EnableOrganizationAdminAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future enableOrganizationAdminAccountAsync(
EnableOrganizationAdminAccountRequest enableOrganizationAdminAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the membership details for specified member accounts for a behavior graph.
*
*
* @param getMembersRequest
* @return A Java Future containing the result of the GetMembers operation returned by the service.
* @sample AmazonDetectiveAsync.GetMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getMembersAsync(GetMembersRequest getMembersRequest);
/**
*
* Returns the membership details for specified member accounts for a behavior graph.
*
*
* @param getMembersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMembers operation returned by the service.
* @sample AmazonDetectiveAsyncHandler.GetMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getMembersAsync(GetMembersRequest getMembersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists data source packages in the behavior graph.
*
*
* @param listDatasourcePackagesRequest
* @return A Java Future containing the result of the ListDatasourcePackages operation returned by the service.
* @sample AmazonDetectiveAsync.ListDatasourcePackages
* @see AWS API Documentation
*/
java.util.concurrent.Future listDatasourcePackagesAsync(ListDatasourcePackagesRequest listDatasourcePackagesRequest);
/**
*
* Lists data source packages in the behavior graph.
*
*
* @param listDatasourcePackagesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDatasourcePackages operation returned by the service.
* @sample AmazonDetectiveAsyncHandler.ListDatasourcePackages
* @see AWS API Documentation
*/
java.util.concurrent.Future listDatasourcePackagesAsync(ListDatasourcePackagesRequest listDatasourcePackagesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the list of behavior graphs that the calling account is an administrator account of. This operation can
* only be called by an administrator account.
*
*
* Because an account can currently only be the administrator of one behavior graph within a Region, the results
* always contain a single behavior graph.
*
*
* @param listGraphsRequest
* @return A Java Future containing the result of the ListGraphs operation returned by the service.
* @sample AmazonDetectiveAsync.ListGraphs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listGraphsAsync(ListGraphsRequest listGraphsRequest);
/**
*
* Returns the list of behavior graphs that the calling account is an administrator account of. This operation can
* only be called by an administrator account.
*
*
* Because an account can currently only be the administrator of one behavior graph within a Region, the results
* always contain a single behavior graph.
*
*
* @param listGraphsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListGraphs operation returned by the service.
* @sample AmazonDetectiveAsyncHandler.ListGraphs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listGraphsAsync(ListGraphsRequest listGraphsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the list of open and accepted behavior graph invitations for the member account. This operation can
* only be called by an invited member account.
*
*
* Open invitations are invitations that the member account has not responded to.
*
*
* The results do not include behavior graphs for which the member account declined the invitation. The results also
* do not include behavior graphs that the member account resigned from or was removed from.
*
*
* @param listInvitationsRequest
* @return A Java Future containing the result of the ListInvitations operation returned by the service.
* @sample AmazonDetectiveAsync.ListInvitations
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listInvitationsAsync(ListInvitationsRequest listInvitationsRequest);
/**
*
* Retrieves the list of open and accepted behavior graph invitations for the member account. This operation can
* only be called by an invited member account.
*
*
* Open invitations are invitations that the member account has not responded to.
*
*
* The results do not include behavior graphs for which the member account declined the invitation. The results also
* do not include behavior graphs that the member account resigned from or was removed from.
*
*
* @param listInvitationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListInvitations operation returned by the service.
* @sample AmazonDetectiveAsyncHandler.ListInvitations
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listInvitationsAsync(ListInvitationsRequest listInvitationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the list of member accounts for a behavior graph.
*
*
* For invited accounts, the results do not include member accounts that were removed from the behavior graph.
*
*
* For the organization behavior graph, the results do not include organization accounts that the Detective
* administrator account has not enabled as member accounts.
*
*
* @param listMembersRequest
* @return A Java Future containing the result of the ListMembers operation returned by the service.
* @sample AmazonDetectiveAsync.ListMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listMembersAsync(ListMembersRequest listMembersRequest);
/**
*
* Retrieves the list of member accounts for a behavior graph.
*
*
* For invited accounts, the results do not include member accounts that were removed from the behavior graph.
*
*
* For the organization behavior graph, the results do not include organization accounts that the Detective
* administrator account has not enabled as member accounts.
*
*
* @param listMembersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMembers operation returned by the service.
* @sample AmazonDetectiveAsyncHandler.ListMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listMembersAsync(ListMembersRequest listMembersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the Detective administrator account for an organization. Can only be called by the
* organization management account.
*
*
* @param listOrganizationAdminAccountsRequest
* @return A Java Future containing the result of the ListOrganizationAdminAccounts operation returned by the
* service.
* @sample AmazonDetectiveAsync.ListOrganizationAdminAccounts
* @see AWS API Documentation
*/
java.util.concurrent.Future listOrganizationAdminAccountsAsync(
ListOrganizationAdminAccountsRequest listOrganizationAdminAccountsRequest);
/**
*
* Returns information about the Detective administrator account for an organization. Can only be called by the
* organization management account.
*
*
* @param listOrganizationAdminAccountsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListOrganizationAdminAccounts operation returned by the
* service.
* @sample AmazonDetectiveAsyncHandler.ListOrganizationAdminAccounts
* @see AWS API Documentation
*/
java.util.concurrent.Future listOrganizationAdminAccountsAsync(
ListOrganizationAdminAccountsRequest listOrganizationAdminAccountsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the tag values that are assigned to a behavior graph.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonDetectiveAsync.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Returns the tag values that are assigned to a behavior graph.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonDetectiveAsyncHandler.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Rejects an invitation to contribute the account data to a behavior graph. This operation must be called by an
* invited member account that has the INVITED
status.
*
*
* RejectInvitation
cannot be called by an organization account in the organization behavior graph. In
* the organization behavior graph, organization accounts do not receive an invitation.
*
*
* @param rejectInvitationRequest
* @return A Java Future containing the result of the RejectInvitation operation returned by the service.
* @sample AmazonDetectiveAsync.RejectInvitation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future rejectInvitationAsync(RejectInvitationRequest rejectInvitationRequest);
/**
*
* Rejects an invitation to contribute the account data to a behavior graph. This operation must be called by an
* invited member account that has the INVITED
status.
*
*
* RejectInvitation
cannot be called by an organization account in the organization behavior graph. In
* the organization behavior graph, organization accounts do not receive an invitation.
*
*
* @param rejectInvitationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RejectInvitation operation returned by the service.
* @sample AmazonDetectiveAsyncHandler.RejectInvitation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future rejectInvitationAsync(RejectInvitationRequest rejectInvitationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sends a request to enable data ingest for a member account that has a status of
* ACCEPTED_BUT_DISABLED
.
*
*
* For valid member accounts, the status is updated as follows.
*
*
* -
*
* If Detective enabled the member account, then the new status is ENABLED
.
*
*
* -
*
* If Detective cannot enable the member account, the status remains ACCEPTED_BUT_DISABLED
.
*
*
*
*
* @param startMonitoringMemberRequest
* @return A Java Future containing the result of the StartMonitoringMember operation returned by the service.
* @sample AmazonDetectiveAsync.StartMonitoringMember
* @see AWS API Documentation
*/
java.util.concurrent.Future startMonitoringMemberAsync(StartMonitoringMemberRequest startMonitoringMemberRequest);
/**
*
* Sends a request to enable data ingest for a member account that has a status of
* ACCEPTED_BUT_DISABLED
.
*
*
* For valid member accounts, the status is updated as follows.
*
*
* -
*
* If Detective enabled the member account, then the new status is ENABLED
.
*
*
* -
*
* If Detective cannot enable the member account, the status remains ACCEPTED_BUT_DISABLED
.
*
*
*
*
* @param startMonitoringMemberRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartMonitoringMember operation returned by the service.
* @sample AmazonDetectiveAsyncHandler.StartMonitoringMember
* @see AWS API Documentation
*/
java.util.concurrent.Future startMonitoringMemberAsync(StartMonitoringMemberRequest startMonitoringMemberRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Applies tag values to a behavior graph.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonDetectiveAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Applies tag values to a behavior graph.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonDetectiveAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes tags from a behavior graph.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonDetectiveAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes tags from a behavior graph.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonDetectiveAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts a data source packages for the behavior graph.
*
*
* @param updateDatasourcePackagesRequest
* @return A Java Future containing the result of the UpdateDatasourcePackages operation returned by the service.
* @sample AmazonDetectiveAsync.UpdateDatasourcePackages
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDatasourcePackagesAsync(UpdateDatasourcePackagesRequest updateDatasourcePackagesRequest);
/**
*
* Starts a data source packages for the behavior graph.
*
*
* @param updateDatasourcePackagesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDatasourcePackages operation returned by the service.
* @sample AmazonDetectiveAsyncHandler.UpdateDatasourcePackages
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDatasourcePackagesAsync(UpdateDatasourcePackagesRequest updateDatasourcePackagesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the configuration for the Organizations integration in the current Region. Can only be called by the
* Detective administrator account for the organization.
*
*
* @param updateOrganizationConfigurationRequest
* @return A Java Future containing the result of the UpdateOrganizationConfiguration operation returned by the
* service.
* @sample AmazonDetectiveAsync.UpdateOrganizationConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateOrganizationConfigurationAsync(
UpdateOrganizationConfigurationRequest updateOrganizationConfigurationRequest);
/**
*
* Updates the configuration for the Organizations integration in the current Region. Can only be called by the
* Detective administrator account for the organization.
*
*
* @param updateOrganizationConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateOrganizationConfiguration operation returned by the
* service.
* @sample AmazonDetectiveAsyncHandler.UpdateOrganizationConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateOrganizationConfigurationAsync(
UpdateOrganizationConfigurationRequest updateOrganizationConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}