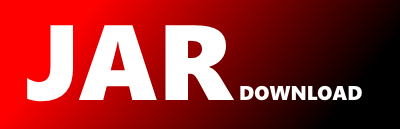
com.amazonaws.services.detective.model.IndicatorDetail Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.detective.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Details about the indicators of compromise which are used to determine if a resource is involved in a security
* incident. An indicator of compromise (IOC) is an artifact observed in or on a network, system, or environment that
* can (with a high level of confidence) identify malicious activity or a security incident. For the list of indicators
* of compromise that are generated by Detective investigations, see Detective
* investigations.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class IndicatorDetail implements Serializable, Cloneable, StructuredPojo {
/**
*
* Details about the indicator of compromise.
*
*/
private TTPsObservedDetail tTPsObservedDetail;
/**
*
* Identifies unusual and impossible user activity for an account.
*
*/
private ImpossibleTravelDetail impossibleTravelDetail;
/**
*
* Suspicious IP addresses that are flagged, which indicates critical or severe threats based on threat intelligence
* by Detective. This indicator is derived from Amazon Web Services threat intelligence.
*
*/
private FlaggedIpAddressDetail flaggedIpAddressDetail;
/**
*
* Contains details about the new geographic location.
*
*/
private NewGeolocationDetail newGeolocationDetail;
/**
*
* Contains details about the new Autonomous System Organization (ASO).
*
*/
private NewAsoDetail newAsoDetail;
/**
*
* Contains details about the new user agent.
*
*/
private NewUserAgentDetail newUserAgentDetail;
/**
*
* Contains details about related findings.
*
*/
private RelatedFindingDetail relatedFindingDetail;
/**
*
* Contains details about related finding groups.
*
*/
private RelatedFindingGroupDetail relatedFindingGroupDetail;
/**
*
* Details about the indicator of compromise.
*
*
* @param tTPsObservedDetail
* Details about the indicator of compromise.
*/
public void setTTPsObservedDetail(TTPsObservedDetail tTPsObservedDetail) {
this.tTPsObservedDetail = tTPsObservedDetail;
}
/**
*
* Details about the indicator of compromise.
*
*
* @return Details about the indicator of compromise.
*/
public TTPsObservedDetail getTTPsObservedDetail() {
return this.tTPsObservedDetail;
}
/**
*
* Details about the indicator of compromise.
*
*
* @param tTPsObservedDetail
* Details about the indicator of compromise.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public IndicatorDetail withTTPsObservedDetail(TTPsObservedDetail tTPsObservedDetail) {
setTTPsObservedDetail(tTPsObservedDetail);
return this;
}
/**
*
* Identifies unusual and impossible user activity for an account.
*
*
* @param impossibleTravelDetail
* Identifies unusual and impossible user activity for an account.
*/
public void setImpossibleTravelDetail(ImpossibleTravelDetail impossibleTravelDetail) {
this.impossibleTravelDetail = impossibleTravelDetail;
}
/**
*
* Identifies unusual and impossible user activity for an account.
*
*
* @return Identifies unusual and impossible user activity for an account.
*/
public ImpossibleTravelDetail getImpossibleTravelDetail() {
return this.impossibleTravelDetail;
}
/**
*
* Identifies unusual and impossible user activity for an account.
*
*
* @param impossibleTravelDetail
* Identifies unusual and impossible user activity for an account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public IndicatorDetail withImpossibleTravelDetail(ImpossibleTravelDetail impossibleTravelDetail) {
setImpossibleTravelDetail(impossibleTravelDetail);
return this;
}
/**
*
* Suspicious IP addresses that are flagged, which indicates critical or severe threats based on threat intelligence
* by Detective. This indicator is derived from Amazon Web Services threat intelligence.
*
*
* @param flaggedIpAddressDetail
* Suspicious IP addresses that are flagged, which indicates critical or severe threats based on threat
* intelligence by Detective. This indicator is derived from Amazon Web Services threat intelligence.
*/
public void setFlaggedIpAddressDetail(FlaggedIpAddressDetail flaggedIpAddressDetail) {
this.flaggedIpAddressDetail = flaggedIpAddressDetail;
}
/**
*
* Suspicious IP addresses that are flagged, which indicates critical or severe threats based on threat intelligence
* by Detective. This indicator is derived from Amazon Web Services threat intelligence.
*
*
* @return Suspicious IP addresses that are flagged, which indicates critical or severe threats based on threat
* intelligence by Detective. This indicator is derived from Amazon Web Services threat intelligence.
*/
public FlaggedIpAddressDetail getFlaggedIpAddressDetail() {
return this.flaggedIpAddressDetail;
}
/**
*
* Suspicious IP addresses that are flagged, which indicates critical or severe threats based on threat intelligence
* by Detective. This indicator is derived from Amazon Web Services threat intelligence.
*
*
* @param flaggedIpAddressDetail
* Suspicious IP addresses that are flagged, which indicates critical or severe threats based on threat
* intelligence by Detective. This indicator is derived from Amazon Web Services threat intelligence.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public IndicatorDetail withFlaggedIpAddressDetail(FlaggedIpAddressDetail flaggedIpAddressDetail) {
setFlaggedIpAddressDetail(flaggedIpAddressDetail);
return this;
}
/**
*
* Contains details about the new geographic location.
*
*
* @param newGeolocationDetail
* Contains details about the new geographic location.
*/
public void setNewGeolocationDetail(NewGeolocationDetail newGeolocationDetail) {
this.newGeolocationDetail = newGeolocationDetail;
}
/**
*
* Contains details about the new geographic location.
*
*
* @return Contains details about the new geographic location.
*/
public NewGeolocationDetail getNewGeolocationDetail() {
return this.newGeolocationDetail;
}
/**
*
* Contains details about the new geographic location.
*
*
* @param newGeolocationDetail
* Contains details about the new geographic location.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public IndicatorDetail withNewGeolocationDetail(NewGeolocationDetail newGeolocationDetail) {
setNewGeolocationDetail(newGeolocationDetail);
return this;
}
/**
*
* Contains details about the new Autonomous System Organization (ASO).
*
*
* @param newAsoDetail
* Contains details about the new Autonomous System Organization (ASO).
*/
public void setNewAsoDetail(NewAsoDetail newAsoDetail) {
this.newAsoDetail = newAsoDetail;
}
/**
*
* Contains details about the new Autonomous System Organization (ASO).
*
*
* @return Contains details about the new Autonomous System Organization (ASO).
*/
public NewAsoDetail getNewAsoDetail() {
return this.newAsoDetail;
}
/**
*
* Contains details about the new Autonomous System Organization (ASO).
*
*
* @param newAsoDetail
* Contains details about the new Autonomous System Organization (ASO).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public IndicatorDetail withNewAsoDetail(NewAsoDetail newAsoDetail) {
setNewAsoDetail(newAsoDetail);
return this;
}
/**
*
* Contains details about the new user agent.
*
*
* @param newUserAgentDetail
* Contains details about the new user agent.
*/
public void setNewUserAgentDetail(NewUserAgentDetail newUserAgentDetail) {
this.newUserAgentDetail = newUserAgentDetail;
}
/**
*
* Contains details about the new user agent.
*
*
* @return Contains details about the new user agent.
*/
public NewUserAgentDetail getNewUserAgentDetail() {
return this.newUserAgentDetail;
}
/**
*
* Contains details about the new user agent.
*
*
* @param newUserAgentDetail
* Contains details about the new user agent.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public IndicatorDetail withNewUserAgentDetail(NewUserAgentDetail newUserAgentDetail) {
setNewUserAgentDetail(newUserAgentDetail);
return this;
}
/**
*
* Contains details about related findings.
*
*
* @param relatedFindingDetail
* Contains details about related findings.
*/
public void setRelatedFindingDetail(RelatedFindingDetail relatedFindingDetail) {
this.relatedFindingDetail = relatedFindingDetail;
}
/**
*
* Contains details about related findings.
*
*
* @return Contains details about related findings.
*/
public RelatedFindingDetail getRelatedFindingDetail() {
return this.relatedFindingDetail;
}
/**
*
* Contains details about related findings.
*
*
* @param relatedFindingDetail
* Contains details about related findings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public IndicatorDetail withRelatedFindingDetail(RelatedFindingDetail relatedFindingDetail) {
setRelatedFindingDetail(relatedFindingDetail);
return this;
}
/**
*
* Contains details about related finding groups.
*
*
* @param relatedFindingGroupDetail
* Contains details about related finding groups.
*/
public void setRelatedFindingGroupDetail(RelatedFindingGroupDetail relatedFindingGroupDetail) {
this.relatedFindingGroupDetail = relatedFindingGroupDetail;
}
/**
*
* Contains details about related finding groups.
*
*
* @return Contains details about related finding groups.
*/
public RelatedFindingGroupDetail getRelatedFindingGroupDetail() {
return this.relatedFindingGroupDetail;
}
/**
*
* Contains details about related finding groups.
*
*
* @param relatedFindingGroupDetail
* Contains details about related finding groups.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public IndicatorDetail withRelatedFindingGroupDetail(RelatedFindingGroupDetail relatedFindingGroupDetail) {
setRelatedFindingGroupDetail(relatedFindingGroupDetail);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTTPsObservedDetail() != null)
sb.append("TTPsObservedDetail: ").append(getTTPsObservedDetail()).append(",");
if (getImpossibleTravelDetail() != null)
sb.append("ImpossibleTravelDetail: ").append(getImpossibleTravelDetail()).append(",");
if (getFlaggedIpAddressDetail() != null)
sb.append("FlaggedIpAddressDetail: ").append(getFlaggedIpAddressDetail()).append(",");
if (getNewGeolocationDetail() != null)
sb.append("NewGeolocationDetail: ").append(getNewGeolocationDetail()).append(",");
if (getNewAsoDetail() != null)
sb.append("NewAsoDetail: ").append(getNewAsoDetail()).append(",");
if (getNewUserAgentDetail() != null)
sb.append("NewUserAgentDetail: ").append(getNewUserAgentDetail()).append(",");
if (getRelatedFindingDetail() != null)
sb.append("RelatedFindingDetail: ").append(getRelatedFindingDetail()).append(",");
if (getRelatedFindingGroupDetail() != null)
sb.append("RelatedFindingGroupDetail: ").append(getRelatedFindingGroupDetail());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof IndicatorDetail == false)
return false;
IndicatorDetail other = (IndicatorDetail) obj;
if (other.getTTPsObservedDetail() == null ^ this.getTTPsObservedDetail() == null)
return false;
if (other.getTTPsObservedDetail() != null && other.getTTPsObservedDetail().equals(this.getTTPsObservedDetail()) == false)
return false;
if (other.getImpossibleTravelDetail() == null ^ this.getImpossibleTravelDetail() == null)
return false;
if (other.getImpossibleTravelDetail() != null && other.getImpossibleTravelDetail().equals(this.getImpossibleTravelDetail()) == false)
return false;
if (other.getFlaggedIpAddressDetail() == null ^ this.getFlaggedIpAddressDetail() == null)
return false;
if (other.getFlaggedIpAddressDetail() != null && other.getFlaggedIpAddressDetail().equals(this.getFlaggedIpAddressDetail()) == false)
return false;
if (other.getNewGeolocationDetail() == null ^ this.getNewGeolocationDetail() == null)
return false;
if (other.getNewGeolocationDetail() != null && other.getNewGeolocationDetail().equals(this.getNewGeolocationDetail()) == false)
return false;
if (other.getNewAsoDetail() == null ^ this.getNewAsoDetail() == null)
return false;
if (other.getNewAsoDetail() != null && other.getNewAsoDetail().equals(this.getNewAsoDetail()) == false)
return false;
if (other.getNewUserAgentDetail() == null ^ this.getNewUserAgentDetail() == null)
return false;
if (other.getNewUserAgentDetail() != null && other.getNewUserAgentDetail().equals(this.getNewUserAgentDetail()) == false)
return false;
if (other.getRelatedFindingDetail() == null ^ this.getRelatedFindingDetail() == null)
return false;
if (other.getRelatedFindingDetail() != null && other.getRelatedFindingDetail().equals(this.getRelatedFindingDetail()) == false)
return false;
if (other.getRelatedFindingGroupDetail() == null ^ this.getRelatedFindingGroupDetail() == null)
return false;
if (other.getRelatedFindingGroupDetail() != null && other.getRelatedFindingGroupDetail().equals(this.getRelatedFindingGroupDetail()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTTPsObservedDetail() == null) ? 0 : getTTPsObservedDetail().hashCode());
hashCode = prime * hashCode + ((getImpossibleTravelDetail() == null) ? 0 : getImpossibleTravelDetail().hashCode());
hashCode = prime * hashCode + ((getFlaggedIpAddressDetail() == null) ? 0 : getFlaggedIpAddressDetail().hashCode());
hashCode = prime * hashCode + ((getNewGeolocationDetail() == null) ? 0 : getNewGeolocationDetail().hashCode());
hashCode = prime * hashCode + ((getNewAsoDetail() == null) ? 0 : getNewAsoDetail().hashCode());
hashCode = prime * hashCode + ((getNewUserAgentDetail() == null) ? 0 : getNewUserAgentDetail().hashCode());
hashCode = prime * hashCode + ((getRelatedFindingDetail() == null) ? 0 : getRelatedFindingDetail().hashCode());
hashCode = prime * hashCode + ((getRelatedFindingGroupDetail() == null) ? 0 : getRelatedFindingGroupDetail().hashCode());
return hashCode;
}
@Override
public IndicatorDetail clone() {
try {
return (IndicatorDetail) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.detective.model.transform.IndicatorDetailMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}