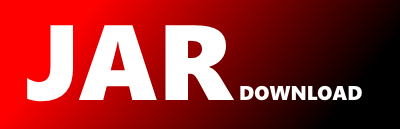
com.amazonaws.services.detective.model.MemberDetail Maven / Gradle / Ivy
Show all versions of aws-java-sdk-detective Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.detective.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Details about a member account in a behavior graph.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class MemberDetail implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Web Services account identifier for the member account.
*
*/
private String accountId;
/**
*
* The Amazon Web Services account root user email address for the member account.
*
*/
private String emailAddress;
/**
*
* The ARN of the behavior graph.
*
*/
private String graphArn;
/**
*
* The Amazon Web Services account identifier of the administrator account for the behavior graph.
*
*/
@Deprecated
private String masterId;
/**
*
* The Amazon Web Services account identifier of the administrator account for the behavior graph.
*
*/
private String administratorId;
/**
*
* The current membership status of the member account. The status can have one of the following values:
*
*
* -
*
* INVITED
- For invited accounts only. Indicates that the member was sent an invitation but has not
* yet responded.
*
*
* -
*
* VERIFICATION_IN_PROGRESS
- For invited accounts only, indicates that Detective is verifying that the
* account identifier and email address provided for the member account match. If they do match, then Detective
* sends the invitation. If the email address and account identifier don't match, then the member cannot be added to
* the behavior graph.
*
*
* For organization accounts in the organization behavior graph, indicates that Detective is verifying that the
* account belongs to the organization.
*
*
* -
*
* VERIFICATION_FAILED
- For invited accounts only. Indicates that the account and email address
* provided for the member account do not match, and Detective did not send an invitation to the account.
*
*
* -
*
* ENABLED
- Indicates that the member account currently contributes data to the behavior graph. For
* invited accounts, the member account accepted the invitation. For organization accounts in the organization
* behavior graph, the Detective administrator account enabled the organization account as a member account.
*
*
* -
*
* ACCEPTED_BUT_DISABLED
- The account accepted the invitation, or was enabled by the Detective
* administrator account, but is prevented from contributing data to the behavior graph. DisabledReason
* provides the reason why the member account is not enabled.
*
*
*
*
* Invited accounts that declined an invitation or that were removed from the behavior graph are not included. In
* the organization behavior graph, organization accounts that the Detective administrator account did not enable
* are not included.
*
*/
private String status;
/**
*
* For member accounts with a status of ACCEPTED_BUT_DISABLED
, the reason that the member account is
* not enabled.
*
*
* The reason can have one of the following values:
*
*
* -
*
* VOLUME_TOO_HIGH
- Indicates that adding the member account would cause the data volume for the
* behavior graph to be too high.
*
*
* -
*
* VOLUME_UNKNOWN
- Indicates that Detective is unable to verify the data volume for the member
* account. This is usually because the member account is not enrolled in Amazon GuardDuty.
*
*
*
*/
private String disabledReason;
/**
*
* For invited accounts, the date and time that Detective sent the invitation to the account. The value is an
* ISO8601 formatted string. For example, 2021-08-18T16:35:56.284Z
.
*
*/
private java.util.Date invitedTime;
/**
*
* The date and time that the member account was last updated. The value is an ISO8601 formatted string. For
* example, 2021-08-18T16:35:56.284Z
.
*
*/
private java.util.Date updatedTime;
/**
*
* The data volume in bytes per day for the member account.
*
*/
@Deprecated
private Long volumeUsageInBytes;
/**
*
* The data and time when the member account data volume was last updated. The value is an ISO8601 formatted string.
* For example, 2021-08-18T16:35:56.284Z
.
*
*/
@Deprecated
private java.util.Date volumeUsageUpdatedTime;
/**
*
* The member account data volume as a percentage of the maximum allowed data volume. 0 indicates 0 percent, and 100
* indicates 100 percent.
*
*
* Note that this is not the percentage of the behavior graph data volume.
*
*
* For example, the data volume for the behavior graph is 80 GB per day. The maximum data volume is 160 GB per day.
* If the data volume for the member account is 40 GB per day, then PercentOfGraphUtilization
is 25. It
* represents 25% of the maximum allowed data volume.
*
*/
@Deprecated
private Double percentOfGraphUtilization;
/**
*
* The date and time when the graph utilization percentage was last updated. The value is an ISO8601 formatted
* string. For example, 2021-08-18T16:35:56.284Z
.
*
*/
@Deprecated
private java.util.Date percentOfGraphUtilizationUpdatedTime;
/**
*
* The type of behavior graph membership.
*
*
* For an organization account in the organization behavior graph, the type is ORGANIZATION
.
*
*
* For an account that was invited to a behavior graph, the type is INVITATION
.
*
*/
private String invitationType;
/**
*
* Details on the volume of usage for each data source package in a behavior graph.
*
*/
private java.util.Map volumeUsageByDatasourcePackage;
/**
*
* The state of a data source package for the behavior graph.
*
*/
private java.util.Map datasourcePackageIngestStates;
/**
*
* The Amazon Web Services account identifier for the member account.
*
*
* @param accountId
* The Amazon Web Services account identifier for the member account.
*/
public void setAccountId(String accountId) {
this.accountId = accountId;
}
/**
*
* The Amazon Web Services account identifier for the member account.
*
*
* @return The Amazon Web Services account identifier for the member account.
*/
public String getAccountId() {
return this.accountId;
}
/**
*
* The Amazon Web Services account identifier for the member account.
*
*
* @param accountId
* The Amazon Web Services account identifier for the member account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MemberDetail withAccountId(String accountId) {
setAccountId(accountId);
return this;
}
/**
*
* The Amazon Web Services account root user email address for the member account.
*
*
* @param emailAddress
* The Amazon Web Services account root user email address for the member account.
*/
public void setEmailAddress(String emailAddress) {
this.emailAddress = emailAddress;
}
/**
*
* The Amazon Web Services account root user email address for the member account.
*
*
* @return The Amazon Web Services account root user email address for the member account.
*/
public String getEmailAddress() {
return this.emailAddress;
}
/**
*
* The Amazon Web Services account root user email address for the member account.
*
*
* @param emailAddress
* The Amazon Web Services account root user email address for the member account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MemberDetail withEmailAddress(String emailAddress) {
setEmailAddress(emailAddress);
return this;
}
/**
*
* The ARN of the behavior graph.
*
*
* @param graphArn
* The ARN of the behavior graph.
*/
public void setGraphArn(String graphArn) {
this.graphArn = graphArn;
}
/**
*
* The ARN of the behavior graph.
*
*
* @return The ARN of the behavior graph.
*/
public String getGraphArn() {
return this.graphArn;
}
/**
*
* The ARN of the behavior graph.
*
*
* @param graphArn
* The ARN of the behavior graph.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MemberDetail withGraphArn(String graphArn) {
setGraphArn(graphArn);
return this;
}
/**
*
* The Amazon Web Services account identifier of the administrator account for the behavior graph.
*
*
* @param masterId
* The Amazon Web Services account identifier of the administrator account for the behavior graph.
*/
@Deprecated
public void setMasterId(String masterId) {
this.masterId = masterId;
}
/**
*
* The Amazon Web Services account identifier of the administrator account for the behavior graph.
*
*
* @return The Amazon Web Services account identifier of the administrator account for the behavior graph.
*/
@Deprecated
public String getMasterId() {
return this.masterId;
}
/**
*
* The Amazon Web Services account identifier of the administrator account for the behavior graph.
*
*
* @param masterId
* The Amazon Web Services account identifier of the administrator account for the behavior graph.
* @return Returns a reference to this object so that method calls can be chained together.
*/
@Deprecated
public MemberDetail withMasterId(String masterId) {
setMasterId(masterId);
return this;
}
/**
*
* The Amazon Web Services account identifier of the administrator account for the behavior graph.
*
*
* @param administratorId
* The Amazon Web Services account identifier of the administrator account for the behavior graph.
*/
public void setAdministratorId(String administratorId) {
this.administratorId = administratorId;
}
/**
*
* The Amazon Web Services account identifier of the administrator account for the behavior graph.
*
*
* @return The Amazon Web Services account identifier of the administrator account for the behavior graph.
*/
public String getAdministratorId() {
return this.administratorId;
}
/**
*
* The Amazon Web Services account identifier of the administrator account for the behavior graph.
*
*
* @param administratorId
* The Amazon Web Services account identifier of the administrator account for the behavior graph.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MemberDetail withAdministratorId(String administratorId) {
setAdministratorId(administratorId);
return this;
}
/**
*
* The current membership status of the member account. The status can have one of the following values:
*
*
* -
*
* INVITED
- For invited accounts only. Indicates that the member was sent an invitation but has not
* yet responded.
*
*
* -
*
* VERIFICATION_IN_PROGRESS
- For invited accounts only, indicates that Detective is verifying that the
* account identifier and email address provided for the member account match. If they do match, then Detective
* sends the invitation. If the email address and account identifier don't match, then the member cannot be added to
* the behavior graph.
*
*
* For organization accounts in the organization behavior graph, indicates that Detective is verifying that the
* account belongs to the organization.
*
*
* -
*
* VERIFICATION_FAILED
- For invited accounts only. Indicates that the account and email address
* provided for the member account do not match, and Detective did not send an invitation to the account.
*
*
* -
*
* ENABLED
- Indicates that the member account currently contributes data to the behavior graph. For
* invited accounts, the member account accepted the invitation. For organization accounts in the organization
* behavior graph, the Detective administrator account enabled the organization account as a member account.
*
*
* -
*
* ACCEPTED_BUT_DISABLED
- The account accepted the invitation, or was enabled by the Detective
* administrator account, but is prevented from contributing data to the behavior graph. DisabledReason
* provides the reason why the member account is not enabled.
*
*
*
*
* Invited accounts that declined an invitation or that were removed from the behavior graph are not included. In
* the organization behavior graph, organization accounts that the Detective administrator account did not enable
* are not included.
*
*
* @param status
* The current membership status of the member account. The status can have one of the following values:
*
* -
*
* INVITED
- For invited accounts only. Indicates that the member was sent an invitation but has
* not yet responded.
*
*
* -
*
* VERIFICATION_IN_PROGRESS
- For invited accounts only, indicates that Detective is verifying
* that the account identifier and email address provided for the member account match. If they do match,
* then Detective sends the invitation. If the email address and account identifier don't match, then the
* member cannot be added to the behavior graph.
*
*
* For organization accounts in the organization behavior graph, indicates that Detective is verifying that
* the account belongs to the organization.
*
*
* -
*
* VERIFICATION_FAILED
- For invited accounts only. Indicates that the account and email address
* provided for the member account do not match, and Detective did not send an invitation to the account.
*
*
* -
*
* ENABLED
- Indicates that the member account currently contributes data to the behavior graph.
* For invited accounts, the member account accepted the invitation. For organization accounts in the
* organization behavior graph, the Detective administrator account enabled the organization account as a
* member account.
*
*
* -
*
* ACCEPTED_BUT_DISABLED
- The account accepted the invitation, or was enabled by the Detective
* administrator account, but is prevented from contributing data to the behavior graph.
* DisabledReason
provides the reason why the member account is not enabled.
*
*
*
*
* Invited accounts that declined an invitation or that were removed from the behavior graph are not
* included. In the organization behavior graph, organization accounts that the Detective administrator
* account did not enable are not included.
* @see MemberStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The current membership status of the member account. The status can have one of the following values:
*
*
* -
*
* INVITED
- For invited accounts only. Indicates that the member was sent an invitation but has not
* yet responded.
*
*
* -
*
* VERIFICATION_IN_PROGRESS
- For invited accounts only, indicates that Detective is verifying that the
* account identifier and email address provided for the member account match. If they do match, then Detective
* sends the invitation. If the email address and account identifier don't match, then the member cannot be added to
* the behavior graph.
*
*
* For organization accounts in the organization behavior graph, indicates that Detective is verifying that the
* account belongs to the organization.
*
*
* -
*
* VERIFICATION_FAILED
- For invited accounts only. Indicates that the account and email address
* provided for the member account do not match, and Detective did not send an invitation to the account.
*
*
* -
*
* ENABLED
- Indicates that the member account currently contributes data to the behavior graph. For
* invited accounts, the member account accepted the invitation. For organization accounts in the organization
* behavior graph, the Detective administrator account enabled the organization account as a member account.
*
*
* -
*
* ACCEPTED_BUT_DISABLED
- The account accepted the invitation, or was enabled by the Detective
* administrator account, but is prevented from contributing data to the behavior graph. DisabledReason
* provides the reason why the member account is not enabled.
*
*
*
*
* Invited accounts that declined an invitation or that were removed from the behavior graph are not included. In
* the organization behavior graph, organization accounts that the Detective administrator account did not enable
* are not included.
*
*
* @return The current membership status of the member account. The status can have one of the following values:
*
* -
*
* INVITED
- For invited accounts only. Indicates that the member was sent an invitation but
* has not yet responded.
*
*
* -
*
* VERIFICATION_IN_PROGRESS
- For invited accounts only, indicates that Detective is verifying
* that the account identifier and email address provided for the member account match. If they do match,
* then Detective sends the invitation. If the email address and account identifier don't match, then the
* member cannot be added to the behavior graph.
*
*
* For organization accounts in the organization behavior graph, indicates that Detective is verifying that
* the account belongs to the organization.
*
*
* -
*
* VERIFICATION_FAILED
- For invited accounts only. Indicates that the account and email
* address provided for the member account do not match, and Detective did not send an invitation to the
* account.
*
*
* -
*
* ENABLED
- Indicates that the member account currently contributes data to the behavior
* graph. For invited accounts, the member account accepted the invitation. For organization accounts in the
* organization behavior graph, the Detective administrator account enabled the organization account as a
* member account.
*
*
* -
*
* ACCEPTED_BUT_DISABLED
- The account accepted the invitation, or was enabled by the Detective
* administrator account, but is prevented from contributing data to the behavior graph.
* DisabledReason
provides the reason why the member account is not enabled.
*
*
*
*
* Invited accounts that declined an invitation or that were removed from the behavior graph are not
* included. In the organization behavior graph, organization accounts that the Detective administrator
* account did not enable are not included.
* @see MemberStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The current membership status of the member account. The status can have one of the following values:
*
*
* -
*
* INVITED
- For invited accounts only. Indicates that the member was sent an invitation but has not
* yet responded.
*
*
* -
*
* VERIFICATION_IN_PROGRESS
- For invited accounts only, indicates that Detective is verifying that the
* account identifier and email address provided for the member account match. If they do match, then Detective
* sends the invitation. If the email address and account identifier don't match, then the member cannot be added to
* the behavior graph.
*
*
* For organization accounts in the organization behavior graph, indicates that Detective is verifying that the
* account belongs to the organization.
*
*
* -
*
* VERIFICATION_FAILED
- For invited accounts only. Indicates that the account and email address
* provided for the member account do not match, and Detective did not send an invitation to the account.
*
*
* -
*
* ENABLED
- Indicates that the member account currently contributes data to the behavior graph. For
* invited accounts, the member account accepted the invitation. For organization accounts in the organization
* behavior graph, the Detective administrator account enabled the organization account as a member account.
*
*
* -
*
* ACCEPTED_BUT_DISABLED
- The account accepted the invitation, or was enabled by the Detective
* administrator account, but is prevented from contributing data to the behavior graph. DisabledReason
* provides the reason why the member account is not enabled.
*
*
*
*
* Invited accounts that declined an invitation or that were removed from the behavior graph are not included. In
* the organization behavior graph, organization accounts that the Detective administrator account did not enable
* are not included.
*
*
* @param status
* The current membership status of the member account. The status can have one of the following values:
*
* -
*
* INVITED
- For invited accounts only. Indicates that the member was sent an invitation but has
* not yet responded.
*
*
* -
*
* VERIFICATION_IN_PROGRESS
- For invited accounts only, indicates that Detective is verifying
* that the account identifier and email address provided for the member account match. If they do match,
* then Detective sends the invitation. If the email address and account identifier don't match, then the
* member cannot be added to the behavior graph.
*
*
* For organization accounts in the organization behavior graph, indicates that Detective is verifying that
* the account belongs to the organization.
*
*
* -
*
* VERIFICATION_FAILED
- For invited accounts only. Indicates that the account and email address
* provided for the member account do not match, and Detective did not send an invitation to the account.
*
*
* -
*
* ENABLED
- Indicates that the member account currently contributes data to the behavior graph.
* For invited accounts, the member account accepted the invitation. For organization accounts in the
* organization behavior graph, the Detective administrator account enabled the organization account as a
* member account.
*
*
* -
*
* ACCEPTED_BUT_DISABLED
- The account accepted the invitation, or was enabled by the Detective
* administrator account, but is prevented from contributing data to the behavior graph.
* DisabledReason
provides the reason why the member account is not enabled.
*
*
*
*
* Invited accounts that declined an invitation or that were removed from the behavior graph are not
* included. In the organization behavior graph, organization accounts that the Detective administrator
* account did not enable are not included.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MemberStatus
*/
public MemberDetail withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The current membership status of the member account. The status can have one of the following values:
*
*
* -
*
* INVITED
- For invited accounts only. Indicates that the member was sent an invitation but has not
* yet responded.
*
*
* -
*
* VERIFICATION_IN_PROGRESS
- For invited accounts only, indicates that Detective is verifying that the
* account identifier and email address provided for the member account match. If they do match, then Detective
* sends the invitation. If the email address and account identifier don't match, then the member cannot be added to
* the behavior graph.
*
*
* For organization accounts in the organization behavior graph, indicates that Detective is verifying that the
* account belongs to the organization.
*
*
* -
*
* VERIFICATION_FAILED
- For invited accounts only. Indicates that the account and email address
* provided for the member account do not match, and Detective did not send an invitation to the account.
*
*
* -
*
* ENABLED
- Indicates that the member account currently contributes data to the behavior graph. For
* invited accounts, the member account accepted the invitation. For organization accounts in the organization
* behavior graph, the Detective administrator account enabled the organization account as a member account.
*
*
* -
*
* ACCEPTED_BUT_DISABLED
- The account accepted the invitation, or was enabled by the Detective
* administrator account, but is prevented from contributing data to the behavior graph. DisabledReason
* provides the reason why the member account is not enabled.
*
*
*
*
* Invited accounts that declined an invitation or that were removed from the behavior graph are not included. In
* the organization behavior graph, organization accounts that the Detective administrator account did not enable
* are not included.
*
*
* @param status
* The current membership status of the member account. The status can have one of the following values:
*
* -
*
* INVITED
- For invited accounts only. Indicates that the member was sent an invitation but has
* not yet responded.
*
*
* -
*
* VERIFICATION_IN_PROGRESS
- For invited accounts only, indicates that Detective is verifying
* that the account identifier and email address provided for the member account match. If they do match,
* then Detective sends the invitation. If the email address and account identifier don't match, then the
* member cannot be added to the behavior graph.
*
*
* For organization accounts in the organization behavior graph, indicates that Detective is verifying that
* the account belongs to the organization.
*
*
* -
*
* VERIFICATION_FAILED
- For invited accounts only. Indicates that the account and email address
* provided for the member account do not match, and Detective did not send an invitation to the account.
*
*
* -
*
* ENABLED
- Indicates that the member account currently contributes data to the behavior graph.
* For invited accounts, the member account accepted the invitation. For organization accounts in the
* organization behavior graph, the Detective administrator account enabled the organization account as a
* member account.
*
*
* -
*
* ACCEPTED_BUT_DISABLED
- The account accepted the invitation, or was enabled by the Detective
* administrator account, but is prevented from contributing data to the behavior graph.
* DisabledReason
provides the reason why the member account is not enabled.
*
*
*
*
* Invited accounts that declined an invitation or that were removed from the behavior graph are not
* included. In the organization behavior graph, organization accounts that the Detective administrator
* account did not enable are not included.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MemberStatus
*/
public MemberDetail withStatus(MemberStatus status) {
this.status = status.toString();
return this;
}
/**
*
* For member accounts with a status of ACCEPTED_BUT_DISABLED
, the reason that the member account is
* not enabled.
*
*
* The reason can have one of the following values:
*
*
* -
*
* VOLUME_TOO_HIGH
- Indicates that adding the member account would cause the data volume for the
* behavior graph to be too high.
*
*
* -
*
* VOLUME_UNKNOWN
- Indicates that Detective is unable to verify the data volume for the member
* account. This is usually because the member account is not enrolled in Amazon GuardDuty.
*
*
*
*
* @param disabledReason
* For member accounts with a status of ACCEPTED_BUT_DISABLED
, the reason that the member
* account is not enabled.
*
* The reason can have one of the following values:
*
*
* -
*
* VOLUME_TOO_HIGH
- Indicates that adding the member account would cause the data volume for
* the behavior graph to be too high.
*
*
* -
*
* VOLUME_UNKNOWN
- Indicates that Detective is unable to verify the data volume for the member
* account. This is usually because the member account is not enrolled in Amazon GuardDuty.
*
*
* @see MemberDisabledReason
*/
public void setDisabledReason(String disabledReason) {
this.disabledReason = disabledReason;
}
/**
*
* For member accounts with a status of ACCEPTED_BUT_DISABLED
, the reason that the member account is
* not enabled.
*
*
* The reason can have one of the following values:
*
*
* -
*
* VOLUME_TOO_HIGH
- Indicates that adding the member account would cause the data volume for the
* behavior graph to be too high.
*
*
* -
*
* VOLUME_UNKNOWN
- Indicates that Detective is unable to verify the data volume for the member
* account. This is usually because the member account is not enrolled in Amazon GuardDuty.
*
*
*
*
* @return For member accounts with a status of ACCEPTED_BUT_DISABLED
, the reason that the member
* account is not enabled.
*
* The reason can have one of the following values:
*
*
* -
*
* VOLUME_TOO_HIGH
- Indicates that adding the member account would cause the data volume for
* the behavior graph to be too high.
*
*
* -
*
* VOLUME_UNKNOWN
- Indicates that Detective is unable to verify the data volume for the member
* account. This is usually because the member account is not enrolled in Amazon GuardDuty.
*
*
* @see MemberDisabledReason
*/
public String getDisabledReason() {
return this.disabledReason;
}
/**
*
* For member accounts with a status of ACCEPTED_BUT_DISABLED
, the reason that the member account is
* not enabled.
*
*
* The reason can have one of the following values:
*
*
* -
*
* VOLUME_TOO_HIGH
- Indicates that adding the member account would cause the data volume for the
* behavior graph to be too high.
*
*
* -
*
* VOLUME_UNKNOWN
- Indicates that Detective is unable to verify the data volume for the member
* account. This is usually because the member account is not enrolled in Amazon GuardDuty.
*
*
*
*
* @param disabledReason
* For member accounts with a status of ACCEPTED_BUT_DISABLED
, the reason that the member
* account is not enabled.
*
* The reason can have one of the following values:
*
*
* -
*
* VOLUME_TOO_HIGH
- Indicates that adding the member account would cause the data volume for
* the behavior graph to be too high.
*
*
* -
*
* VOLUME_UNKNOWN
- Indicates that Detective is unable to verify the data volume for the member
* account. This is usually because the member account is not enrolled in Amazon GuardDuty.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see MemberDisabledReason
*/
public MemberDetail withDisabledReason(String disabledReason) {
setDisabledReason(disabledReason);
return this;
}
/**
*
* For member accounts with a status of ACCEPTED_BUT_DISABLED
, the reason that the member account is
* not enabled.
*
*
* The reason can have one of the following values:
*
*
* -
*
* VOLUME_TOO_HIGH
- Indicates that adding the member account would cause the data volume for the
* behavior graph to be too high.
*
*
* -
*
* VOLUME_UNKNOWN
- Indicates that Detective is unable to verify the data volume for the member
* account. This is usually because the member account is not enrolled in Amazon GuardDuty.
*
*
*
*
* @param disabledReason
* For member accounts with a status of ACCEPTED_BUT_DISABLED
, the reason that the member
* account is not enabled.
*
* The reason can have one of the following values:
*
*
* -
*
* VOLUME_TOO_HIGH
- Indicates that adding the member account would cause the data volume for
* the behavior graph to be too high.
*
*
* -
*
* VOLUME_UNKNOWN
- Indicates that Detective is unable to verify the data volume for the member
* account. This is usually because the member account is not enrolled in Amazon GuardDuty.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see MemberDisabledReason
*/
public MemberDetail withDisabledReason(MemberDisabledReason disabledReason) {
this.disabledReason = disabledReason.toString();
return this;
}
/**
*
* For invited accounts, the date and time that Detective sent the invitation to the account. The value is an
* ISO8601 formatted string. For example, 2021-08-18T16:35:56.284Z
.
*
*
* @param invitedTime
* For invited accounts, the date and time that Detective sent the invitation to the account. The value is an
* ISO8601 formatted string. For example, 2021-08-18T16:35:56.284Z
.
*/
public void setInvitedTime(java.util.Date invitedTime) {
this.invitedTime = invitedTime;
}
/**
*
* For invited accounts, the date and time that Detective sent the invitation to the account. The value is an
* ISO8601 formatted string. For example, 2021-08-18T16:35:56.284Z
.
*
*
* @return For invited accounts, the date and time that Detective sent the invitation to the account. The value is
* an ISO8601 formatted string. For example, 2021-08-18T16:35:56.284Z
.
*/
public java.util.Date getInvitedTime() {
return this.invitedTime;
}
/**
*
* For invited accounts, the date and time that Detective sent the invitation to the account. The value is an
* ISO8601 formatted string. For example, 2021-08-18T16:35:56.284Z
.
*
*
* @param invitedTime
* For invited accounts, the date and time that Detective sent the invitation to the account. The value is an
* ISO8601 formatted string. For example, 2021-08-18T16:35:56.284Z
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MemberDetail withInvitedTime(java.util.Date invitedTime) {
setInvitedTime(invitedTime);
return this;
}
/**
*
* The date and time that the member account was last updated. The value is an ISO8601 formatted string. For
* example, 2021-08-18T16:35:56.284Z
.
*
*
* @param updatedTime
* The date and time that the member account was last updated. The value is an ISO8601 formatted string. For
* example, 2021-08-18T16:35:56.284Z
.
*/
public void setUpdatedTime(java.util.Date updatedTime) {
this.updatedTime = updatedTime;
}
/**
*
* The date and time that the member account was last updated. The value is an ISO8601 formatted string. For
* example, 2021-08-18T16:35:56.284Z
.
*
*
* @return The date and time that the member account was last updated. The value is an ISO8601 formatted string. For
* example, 2021-08-18T16:35:56.284Z
.
*/
public java.util.Date getUpdatedTime() {
return this.updatedTime;
}
/**
*
* The date and time that the member account was last updated. The value is an ISO8601 formatted string. For
* example, 2021-08-18T16:35:56.284Z
.
*
*
* @param updatedTime
* The date and time that the member account was last updated. The value is an ISO8601 formatted string. For
* example, 2021-08-18T16:35:56.284Z
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MemberDetail withUpdatedTime(java.util.Date updatedTime) {
setUpdatedTime(updatedTime);
return this;
}
/**
*
* The data volume in bytes per day for the member account.
*
*
* @param volumeUsageInBytes
* The data volume in bytes per day for the member account.
*/
@Deprecated
public void setVolumeUsageInBytes(Long volumeUsageInBytes) {
this.volumeUsageInBytes = volumeUsageInBytes;
}
/**
*
* The data volume in bytes per day for the member account.
*
*
* @return The data volume in bytes per day for the member account.
*/
@Deprecated
public Long getVolumeUsageInBytes() {
return this.volumeUsageInBytes;
}
/**
*
* The data volume in bytes per day for the member account.
*
*
* @param volumeUsageInBytes
* The data volume in bytes per day for the member account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
@Deprecated
public MemberDetail withVolumeUsageInBytes(Long volumeUsageInBytes) {
setVolumeUsageInBytes(volumeUsageInBytes);
return this;
}
/**
*
* The data and time when the member account data volume was last updated. The value is an ISO8601 formatted string.
* For example, 2021-08-18T16:35:56.284Z
.
*
*
* @param volumeUsageUpdatedTime
* The data and time when the member account data volume was last updated. The value is an ISO8601 formatted
* string. For example, 2021-08-18T16:35:56.284Z
.
*/
@Deprecated
public void setVolumeUsageUpdatedTime(java.util.Date volumeUsageUpdatedTime) {
this.volumeUsageUpdatedTime = volumeUsageUpdatedTime;
}
/**
*
* The data and time when the member account data volume was last updated. The value is an ISO8601 formatted string.
* For example, 2021-08-18T16:35:56.284Z
.
*
*
* @return The data and time when the member account data volume was last updated. The value is an ISO8601 formatted
* string. For example, 2021-08-18T16:35:56.284Z
.
*/
@Deprecated
public java.util.Date getVolumeUsageUpdatedTime() {
return this.volumeUsageUpdatedTime;
}
/**
*
* The data and time when the member account data volume was last updated. The value is an ISO8601 formatted string.
* For example, 2021-08-18T16:35:56.284Z
.
*
*
* @param volumeUsageUpdatedTime
* The data and time when the member account data volume was last updated. The value is an ISO8601 formatted
* string. For example, 2021-08-18T16:35:56.284Z
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
@Deprecated
public MemberDetail withVolumeUsageUpdatedTime(java.util.Date volumeUsageUpdatedTime) {
setVolumeUsageUpdatedTime(volumeUsageUpdatedTime);
return this;
}
/**
*
* The member account data volume as a percentage of the maximum allowed data volume. 0 indicates 0 percent, and 100
* indicates 100 percent.
*
*
* Note that this is not the percentage of the behavior graph data volume.
*
*
* For example, the data volume for the behavior graph is 80 GB per day. The maximum data volume is 160 GB per day.
* If the data volume for the member account is 40 GB per day, then PercentOfGraphUtilization
is 25. It
* represents 25% of the maximum allowed data volume.
*
*
* @param percentOfGraphUtilization
* The member account data volume as a percentage of the maximum allowed data volume. 0 indicates 0 percent,
* and 100 indicates 100 percent.
*
* Note that this is not the percentage of the behavior graph data volume.
*
*
* For example, the data volume for the behavior graph is 80 GB per day. The maximum data volume is 160 GB
* per day. If the data volume for the member account is 40 GB per day, then
* PercentOfGraphUtilization
is 25. It represents 25% of the maximum allowed data volume.
*/
@Deprecated
public void setPercentOfGraphUtilization(Double percentOfGraphUtilization) {
this.percentOfGraphUtilization = percentOfGraphUtilization;
}
/**
*
* The member account data volume as a percentage of the maximum allowed data volume. 0 indicates 0 percent, and 100
* indicates 100 percent.
*
*
* Note that this is not the percentage of the behavior graph data volume.
*
*
* For example, the data volume for the behavior graph is 80 GB per day. The maximum data volume is 160 GB per day.
* If the data volume for the member account is 40 GB per day, then PercentOfGraphUtilization
is 25. It
* represents 25% of the maximum allowed data volume.
*
*
* @return The member account data volume as a percentage of the maximum allowed data volume. 0 indicates 0 percent,
* and 100 indicates 100 percent.
*
* Note that this is not the percentage of the behavior graph data volume.
*
*
* For example, the data volume for the behavior graph is 80 GB per day. The maximum data volume is 160 GB
* per day. If the data volume for the member account is 40 GB per day, then
* PercentOfGraphUtilization
is 25. It represents 25% of the maximum allowed data volume.
*/
@Deprecated
public Double getPercentOfGraphUtilization() {
return this.percentOfGraphUtilization;
}
/**
*
* The member account data volume as a percentage of the maximum allowed data volume. 0 indicates 0 percent, and 100
* indicates 100 percent.
*
*
* Note that this is not the percentage of the behavior graph data volume.
*
*
* For example, the data volume for the behavior graph is 80 GB per day. The maximum data volume is 160 GB per day.
* If the data volume for the member account is 40 GB per day, then PercentOfGraphUtilization
is 25. It
* represents 25% of the maximum allowed data volume.
*
*
* @param percentOfGraphUtilization
* The member account data volume as a percentage of the maximum allowed data volume. 0 indicates 0 percent,
* and 100 indicates 100 percent.
*
* Note that this is not the percentage of the behavior graph data volume.
*
*
* For example, the data volume for the behavior graph is 80 GB per day. The maximum data volume is 160 GB
* per day. If the data volume for the member account is 40 GB per day, then
* PercentOfGraphUtilization
is 25. It represents 25% of the maximum allowed data volume.
* @return Returns a reference to this object so that method calls can be chained together.
*/
@Deprecated
public MemberDetail withPercentOfGraphUtilization(Double percentOfGraphUtilization) {
setPercentOfGraphUtilization(percentOfGraphUtilization);
return this;
}
/**
*
* The date and time when the graph utilization percentage was last updated. The value is an ISO8601 formatted
* string. For example, 2021-08-18T16:35:56.284Z
.
*
*
* @param percentOfGraphUtilizationUpdatedTime
* The date and time when the graph utilization percentage was last updated. The value is an ISO8601
* formatted string. For example, 2021-08-18T16:35:56.284Z
.
*/
@Deprecated
public void setPercentOfGraphUtilizationUpdatedTime(java.util.Date percentOfGraphUtilizationUpdatedTime) {
this.percentOfGraphUtilizationUpdatedTime = percentOfGraphUtilizationUpdatedTime;
}
/**
*
* The date and time when the graph utilization percentage was last updated. The value is an ISO8601 formatted
* string. For example, 2021-08-18T16:35:56.284Z
.
*
*
* @return The date and time when the graph utilization percentage was last updated. The value is an ISO8601
* formatted string. For example, 2021-08-18T16:35:56.284Z
.
*/
@Deprecated
public java.util.Date getPercentOfGraphUtilizationUpdatedTime() {
return this.percentOfGraphUtilizationUpdatedTime;
}
/**
*
* The date and time when the graph utilization percentage was last updated. The value is an ISO8601 formatted
* string. For example, 2021-08-18T16:35:56.284Z
.
*
*
* @param percentOfGraphUtilizationUpdatedTime
* The date and time when the graph utilization percentage was last updated. The value is an ISO8601
* formatted string. For example, 2021-08-18T16:35:56.284Z
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
@Deprecated
public MemberDetail withPercentOfGraphUtilizationUpdatedTime(java.util.Date percentOfGraphUtilizationUpdatedTime) {
setPercentOfGraphUtilizationUpdatedTime(percentOfGraphUtilizationUpdatedTime);
return this;
}
/**
*
* The type of behavior graph membership.
*
*
* For an organization account in the organization behavior graph, the type is ORGANIZATION
.
*
*
* For an account that was invited to a behavior graph, the type is INVITATION
.
*
*
* @param invitationType
* The type of behavior graph membership.
*
* For an organization account in the organization behavior graph, the type is ORGANIZATION
.
*
*
* For an account that was invited to a behavior graph, the type is INVITATION
.
* @see InvitationType
*/
public void setInvitationType(String invitationType) {
this.invitationType = invitationType;
}
/**
*
* The type of behavior graph membership.
*
*
* For an organization account in the organization behavior graph, the type is ORGANIZATION
.
*
*
* For an account that was invited to a behavior graph, the type is INVITATION
.
*
*
* @return The type of behavior graph membership.
*
* For an organization account in the organization behavior graph, the type is ORGANIZATION
.
*
*
* For an account that was invited to a behavior graph, the type is INVITATION
.
* @see InvitationType
*/
public String getInvitationType() {
return this.invitationType;
}
/**
*
* The type of behavior graph membership.
*
*
* For an organization account in the organization behavior graph, the type is ORGANIZATION
.
*
*
* For an account that was invited to a behavior graph, the type is INVITATION
.
*
*
* @param invitationType
* The type of behavior graph membership.
*
* For an organization account in the organization behavior graph, the type is ORGANIZATION
.
*
*
* For an account that was invited to a behavior graph, the type is INVITATION
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InvitationType
*/
public MemberDetail withInvitationType(String invitationType) {
setInvitationType(invitationType);
return this;
}
/**
*
* The type of behavior graph membership.
*
*
* For an organization account in the organization behavior graph, the type is ORGANIZATION
.
*
*
* For an account that was invited to a behavior graph, the type is INVITATION
.
*
*
* @param invitationType
* The type of behavior graph membership.
*
* For an organization account in the organization behavior graph, the type is ORGANIZATION
.
*
*
* For an account that was invited to a behavior graph, the type is INVITATION
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InvitationType
*/
public MemberDetail withInvitationType(InvitationType invitationType) {
this.invitationType = invitationType.toString();
return this;
}
/**
*
* Details on the volume of usage for each data source package in a behavior graph.
*
*
* @return Details on the volume of usage for each data source package in a behavior graph.
*/
public java.util.Map getVolumeUsageByDatasourcePackage() {
return volumeUsageByDatasourcePackage;
}
/**
*
* Details on the volume of usage for each data source package in a behavior graph.
*
*
* @param volumeUsageByDatasourcePackage
* Details on the volume of usage for each data source package in a behavior graph.
*/
public void setVolumeUsageByDatasourcePackage(java.util.Map volumeUsageByDatasourcePackage) {
this.volumeUsageByDatasourcePackage = volumeUsageByDatasourcePackage;
}
/**
*
* Details on the volume of usage for each data source package in a behavior graph.
*
*
* @param volumeUsageByDatasourcePackage
* Details on the volume of usage for each data source package in a behavior graph.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MemberDetail withVolumeUsageByDatasourcePackage(java.util.Map volumeUsageByDatasourcePackage) {
setVolumeUsageByDatasourcePackage(volumeUsageByDatasourcePackage);
return this;
}
/**
* Add a single VolumeUsageByDatasourcePackage entry
*
* @see MemberDetail#withVolumeUsageByDatasourcePackage
* @returns a reference to this object so that method calls can be chained together.
*/
public MemberDetail addVolumeUsageByDatasourcePackageEntry(String key, DatasourcePackageUsageInfo value) {
if (null == this.volumeUsageByDatasourcePackage) {
this.volumeUsageByDatasourcePackage = new java.util.HashMap();
}
if (this.volumeUsageByDatasourcePackage.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.volumeUsageByDatasourcePackage.put(key, value);
return this;
}
/**
* Removes all the entries added into VolumeUsageByDatasourcePackage.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MemberDetail clearVolumeUsageByDatasourcePackageEntries() {
this.volumeUsageByDatasourcePackage = null;
return this;
}
/**
*
* The state of a data source package for the behavior graph.
*
*
* @return The state of a data source package for the behavior graph.
*/
public java.util.Map getDatasourcePackageIngestStates() {
return datasourcePackageIngestStates;
}
/**
*
* The state of a data source package for the behavior graph.
*
*
* @param datasourcePackageIngestStates
* The state of a data source package for the behavior graph.
*/
public void setDatasourcePackageIngestStates(java.util.Map datasourcePackageIngestStates) {
this.datasourcePackageIngestStates = datasourcePackageIngestStates;
}
/**
*
* The state of a data source package for the behavior graph.
*
*
* @param datasourcePackageIngestStates
* The state of a data source package for the behavior graph.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MemberDetail withDatasourcePackageIngestStates(java.util.Map datasourcePackageIngestStates) {
setDatasourcePackageIngestStates(datasourcePackageIngestStates);
return this;
}
/**
* Add a single DatasourcePackageIngestStates entry
*
* @see MemberDetail#withDatasourcePackageIngestStates
* @returns a reference to this object so that method calls can be chained together.
*/
public MemberDetail addDatasourcePackageIngestStatesEntry(String key, String value) {
if (null == this.datasourcePackageIngestStates) {
this.datasourcePackageIngestStates = new java.util.HashMap();
}
if (this.datasourcePackageIngestStates.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.datasourcePackageIngestStates.put(key, value);
return this;
}
/**
* Removes all the entries added into DatasourcePackageIngestStates.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MemberDetail clearDatasourcePackageIngestStatesEntries() {
this.datasourcePackageIngestStates = null;
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAccountId() != null)
sb.append("AccountId: ").append(getAccountId()).append(",");
if (getEmailAddress() != null)
sb.append("EmailAddress: ").append("***Sensitive Data Redacted***").append(",");
if (getGraphArn() != null)
sb.append("GraphArn: ").append(getGraphArn()).append(",");
if (getMasterId() != null)
sb.append("MasterId: ").append(getMasterId()).append(",");
if (getAdministratorId() != null)
sb.append("AdministratorId: ").append(getAdministratorId()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getDisabledReason() != null)
sb.append("DisabledReason: ").append(getDisabledReason()).append(",");
if (getInvitedTime() != null)
sb.append("InvitedTime: ").append(getInvitedTime()).append(",");
if (getUpdatedTime() != null)
sb.append("UpdatedTime: ").append(getUpdatedTime()).append(",");
if (getVolumeUsageInBytes() != null)
sb.append("VolumeUsageInBytes: ").append(getVolumeUsageInBytes()).append(",");
if (getVolumeUsageUpdatedTime() != null)
sb.append("VolumeUsageUpdatedTime: ").append(getVolumeUsageUpdatedTime()).append(",");
if (getPercentOfGraphUtilization() != null)
sb.append("PercentOfGraphUtilization: ").append(getPercentOfGraphUtilization()).append(",");
if (getPercentOfGraphUtilizationUpdatedTime() != null)
sb.append("PercentOfGraphUtilizationUpdatedTime: ").append(getPercentOfGraphUtilizationUpdatedTime()).append(",");
if (getInvitationType() != null)
sb.append("InvitationType: ").append(getInvitationType()).append(",");
if (getVolumeUsageByDatasourcePackage() != null)
sb.append("VolumeUsageByDatasourcePackage: ").append(getVolumeUsageByDatasourcePackage()).append(",");
if (getDatasourcePackageIngestStates() != null)
sb.append("DatasourcePackageIngestStates: ").append(getDatasourcePackageIngestStates());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof MemberDetail == false)
return false;
MemberDetail other = (MemberDetail) obj;
if (other.getAccountId() == null ^ this.getAccountId() == null)
return false;
if (other.getAccountId() != null && other.getAccountId().equals(this.getAccountId()) == false)
return false;
if (other.getEmailAddress() == null ^ this.getEmailAddress() == null)
return false;
if (other.getEmailAddress() != null && other.getEmailAddress().equals(this.getEmailAddress()) == false)
return false;
if (other.getGraphArn() == null ^ this.getGraphArn() == null)
return false;
if (other.getGraphArn() != null && other.getGraphArn().equals(this.getGraphArn()) == false)
return false;
if (other.getMasterId() == null ^ this.getMasterId() == null)
return false;
if (other.getMasterId() != null && other.getMasterId().equals(this.getMasterId()) == false)
return false;
if (other.getAdministratorId() == null ^ this.getAdministratorId() == null)
return false;
if (other.getAdministratorId() != null && other.getAdministratorId().equals(this.getAdministratorId()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getDisabledReason() == null ^ this.getDisabledReason() == null)
return false;
if (other.getDisabledReason() != null && other.getDisabledReason().equals(this.getDisabledReason()) == false)
return false;
if (other.getInvitedTime() == null ^ this.getInvitedTime() == null)
return false;
if (other.getInvitedTime() != null && other.getInvitedTime().equals(this.getInvitedTime()) == false)
return false;
if (other.getUpdatedTime() == null ^ this.getUpdatedTime() == null)
return false;
if (other.getUpdatedTime() != null && other.getUpdatedTime().equals(this.getUpdatedTime()) == false)
return false;
if (other.getVolumeUsageInBytes() == null ^ this.getVolumeUsageInBytes() == null)
return false;
if (other.getVolumeUsageInBytes() != null && other.getVolumeUsageInBytes().equals(this.getVolumeUsageInBytes()) == false)
return false;
if (other.getVolumeUsageUpdatedTime() == null ^ this.getVolumeUsageUpdatedTime() == null)
return false;
if (other.getVolumeUsageUpdatedTime() != null && other.getVolumeUsageUpdatedTime().equals(this.getVolumeUsageUpdatedTime()) == false)
return false;
if (other.getPercentOfGraphUtilization() == null ^ this.getPercentOfGraphUtilization() == null)
return false;
if (other.getPercentOfGraphUtilization() != null && other.getPercentOfGraphUtilization().equals(this.getPercentOfGraphUtilization()) == false)
return false;
if (other.getPercentOfGraphUtilizationUpdatedTime() == null ^ this.getPercentOfGraphUtilizationUpdatedTime() == null)
return false;
if (other.getPercentOfGraphUtilizationUpdatedTime() != null
&& other.getPercentOfGraphUtilizationUpdatedTime().equals(this.getPercentOfGraphUtilizationUpdatedTime()) == false)
return false;
if (other.getInvitationType() == null ^ this.getInvitationType() == null)
return false;
if (other.getInvitationType() != null && other.getInvitationType().equals(this.getInvitationType()) == false)
return false;
if (other.getVolumeUsageByDatasourcePackage() == null ^ this.getVolumeUsageByDatasourcePackage() == null)
return false;
if (other.getVolumeUsageByDatasourcePackage() != null
&& other.getVolumeUsageByDatasourcePackage().equals(this.getVolumeUsageByDatasourcePackage()) == false)
return false;
if (other.getDatasourcePackageIngestStates() == null ^ this.getDatasourcePackageIngestStates() == null)
return false;
if (other.getDatasourcePackageIngestStates() != null
&& other.getDatasourcePackageIngestStates().equals(this.getDatasourcePackageIngestStates()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAccountId() == null) ? 0 : getAccountId().hashCode());
hashCode = prime * hashCode + ((getEmailAddress() == null) ? 0 : getEmailAddress().hashCode());
hashCode = prime * hashCode + ((getGraphArn() == null) ? 0 : getGraphArn().hashCode());
hashCode = prime * hashCode + ((getMasterId() == null) ? 0 : getMasterId().hashCode());
hashCode = prime * hashCode + ((getAdministratorId() == null) ? 0 : getAdministratorId().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getDisabledReason() == null) ? 0 : getDisabledReason().hashCode());
hashCode = prime * hashCode + ((getInvitedTime() == null) ? 0 : getInvitedTime().hashCode());
hashCode = prime * hashCode + ((getUpdatedTime() == null) ? 0 : getUpdatedTime().hashCode());
hashCode = prime * hashCode + ((getVolumeUsageInBytes() == null) ? 0 : getVolumeUsageInBytes().hashCode());
hashCode = prime * hashCode + ((getVolumeUsageUpdatedTime() == null) ? 0 : getVolumeUsageUpdatedTime().hashCode());
hashCode = prime * hashCode + ((getPercentOfGraphUtilization() == null) ? 0 : getPercentOfGraphUtilization().hashCode());
hashCode = prime * hashCode + ((getPercentOfGraphUtilizationUpdatedTime() == null) ? 0 : getPercentOfGraphUtilizationUpdatedTime().hashCode());
hashCode = prime * hashCode + ((getInvitationType() == null) ? 0 : getInvitationType().hashCode());
hashCode = prime * hashCode + ((getVolumeUsageByDatasourcePackage() == null) ? 0 : getVolumeUsageByDatasourcePackage().hashCode());
hashCode = prime * hashCode + ((getDatasourcePackageIngestStates() == null) ? 0 : getDatasourcePackageIngestStates().hashCode());
return hashCode;
}
@Override
public MemberDetail clone() {
try {
return (MemberDetail) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.detective.model.transform.MemberDetailMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}