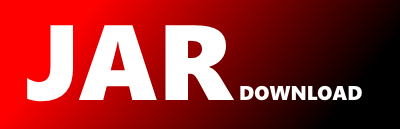
com.amazonaws.services.devicefarm.AWSDeviceFarm Maven / Gradle / Ivy
Show all versions of aws-java-sdk-devicefarm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.devicefarm;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.devicefarm.model.*;
/**
* Interface for accessing AWS Device Farm.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.devicefarm.AbstractAWSDeviceFarm} instead.
*
*
*
* Welcome to the AWS Device Farm API documentation, which contains APIs for:
*
*
* -
*
* Testing on desktop browsers
*
*
* Device Farm makes it possible for you to test your web applications on desktop browsers using Selenium. The APIs for
* desktop browser testing contain TestGrid
in their names. For more information, see Testing Web Applications on Selenium with Device
* Farm.
*
*
* -
*
* Testing on real mobile devices
*
*
* Device Farm makes it possible for you to test apps on physical phones, tablets, and other devices in the cloud. For
* more information, see the Device Farm
* Developer Guide.
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSDeviceFarm {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "devicefarm";
/**
* Overrides the default endpoint for this client ("https://devicefarm.us-west-2.amazonaws.com"). Callers can use
* this method to control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex: "devicefarm.us-west-2.amazonaws.com") or a full URL, including the
* protocol (ex: "https://devicefarm.us-west-2.amazonaws.com"). If the protocol is not specified here, the default
* protocol from this client's {@link ClientConfiguration} will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and a complete list of all available
* endpoints for all AWS services, see: https://docs.aws.amazon.com/sdk-for-java/v1/developer-guide/java-dg-region-selection.html#region-selection-
* choose-endpoint
*
* This method is not threadsafe. An endpoint should be configured when the client is created and before any
* service requests are made. Changing it afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param endpoint
* The endpoint (ex: "devicefarm.us-west-2.amazonaws.com") or a full URL, including the protocol (ex:
* "https://devicefarm.us-west-2.amazonaws.com") of the region specific AWS endpoint this client will
* communicate with.
* @deprecated use {@link AwsClientBuilder#setEndpointConfiguration(AwsClientBuilder.EndpointConfiguration)} for
* example:
* {@code builder.setEndpointConfiguration(new EndpointConfiguration(endpoint, signingRegion));}
*/
@Deprecated
void setEndpoint(String endpoint);
/**
* An alternative to {@link AWSDeviceFarm#setEndpoint(String)}, sets the regional endpoint for this client's service
* calls. Callers can use this method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol. To use http instead, specify it in the
* {@link ClientConfiguration} supplied at construction.
*
* This method is not threadsafe. A region should be configured when the client is created and before any service
* requests are made. Changing it afterwards creates inevitable race conditions for any service requests in transit
* or retrying.
*
* @param region
* The region this client will communicate with. See {@link Region#getRegion(com.amazonaws.regions.Regions)}
* for accessing a given region. Must not be null and must be a region where the service is available.
*
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class, com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
* @see Region#isServiceSupported(String)
* @deprecated use {@link AwsClientBuilder#setRegion(String)}
*/
@Deprecated
void setRegion(Region region);
/**
*
* Creates a device pool.
*
*
* @param createDevicePoolRequest
* Represents a request to the create device pool operation.
* @return Result of the CreateDevicePool operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.CreateDevicePool
* @see AWS
* API Documentation
*/
CreateDevicePoolResult createDevicePool(CreateDevicePoolRequest createDevicePoolRequest);
/**
*
* Creates a profile that can be applied to one or more private fleet device instances.
*
*
* @param createInstanceProfileRequest
* @return Result of the CreateInstanceProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.CreateInstanceProfile
* @see AWS API Documentation
*/
CreateInstanceProfileResult createInstanceProfile(CreateInstanceProfileRequest createInstanceProfileRequest);
/**
*
* Creates a network profile.
*
*
* @param createNetworkProfileRequest
* @return Result of the CreateNetworkProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.CreateNetworkProfile
* @see AWS API Documentation
*/
CreateNetworkProfileResult createNetworkProfile(CreateNetworkProfileRequest createNetworkProfileRequest);
/**
*
* Creates a project.
*
*
* @param createProjectRequest
* Represents a request to the create project operation.
* @return Result of the CreateProject operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws TagOperationException
* The operation was not successful. Try again.
* @sample AWSDeviceFarm.CreateProject
* @see AWS API
* Documentation
*/
CreateProjectResult createProject(CreateProjectRequest createProjectRequest);
/**
*
* Specifies and starts a remote access session.
*
*
* @param createRemoteAccessSessionRequest
* Creates and submits a request to start a remote access session.
* @return Result of the CreateRemoteAccessSession operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.CreateRemoteAccessSession
* @see AWS API Documentation
*/
CreateRemoteAccessSessionResult createRemoteAccessSession(CreateRemoteAccessSessionRequest createRemoteAccessSessionRequest);
/**
*
* Creates a Selenium testing project. Projects are used to track TestGridSession instances.
*
*
* @param createTestGridProjectRequest
* @return Result of the CreateTestGridProject operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws LimitExceededException
* A limit was exceeded.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @sample AWSDeviceFarm.CreateTestGridProject
* @see AWS API Documentation
*/
CreateTestGridProjectResult createTestGridProject(CreateTestGridProjectRequest createTestGridProjectRequest);
/**
*
* Creates a signed, short-term URL that can be passed to a Selenium RemoteWebDriver
constructor.
*
*
* @param createTestGridUrlRequest
* @return Result of the CreateTestGridUrl operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @sample AWSDeviceFarm.CreateTestGridUrl
* @see AWS
* API Documentation
*/
CreateTestGridUrlResult createTestGridUrl(CreateTestGridUrlRequest createTestGridUrlRequest);
/**
*
* Uploads an app or test scripts.
*
*
* @param createUploadRequest
* Represents a request to the create upload operation.
* @return Result of the CreateUpload operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.CreateUpload
* @see AWS API
* Documentation
*/
CreateUploadResult createUpload(CreateUploadRequest createUploadRequest);
/**
*
* Creates a configuration record in Device Farm for your Amazon Virtual Private Cloud (VPC) endpoint.
*
*
* @param createVPCEConfigurationRequest
* @return Result of the CreateVPCEConfiguration operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.CreateVPCEConfiguration
* @see AWS API Documentation
*/
CreateVPCEConfigurationResult createVPCEConfiguration(CreateVPCEConfigurationRequest createVPCEConfigurationRequest);
/**
*
* Deletes a device pool given the pool ARN. Does not allow deletion of curated pools owned by the system.
*
*
* @param deleteDevicePoolRequest
* Represents a request to the delete device pool operation.
* @return Result of the DeleteDevicePool operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.DeleteDevicePool
* @see AWS
* API Documentation
*/
DeleteDevicePoolResult deleteDevicePool(DeleteDevicePoolRequest deleteDevicePoolRequest);
/**
*
* Deletes a profile that can be applied to one or more private device instances.
*
*
* @param deleteInstanceProfileRequest
* @return Result of the DeleteInstanceProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.DeleteInstanceProfile
* @see AWS API Documentation
*/
DeleteInstanceProfileResult deleteInstanceProfile(DeleteInstanceProfileRequest deleteInstanceProfileRequest);
/**
*
* Deletes a network profile.
*
*
* @param deleteNetworkProfileRequest
* @return Result of the DeleteNetworkProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.DeleteNetworkProfile
* @see AWS API Documentation
*/
DeleteNetworkProfileResult deleteNetworkProfile(DeleteNetworkProfileRequest deleteNetworkProfileRequest);
/**
*
* Deletes an AWS Device Farm project, given the project ARN.
*
*
* Deleting this resource does not stop an in-progress run.
*
*
* @param deleteProjectRequest
* Represents a request to the delete project operation.
* @return Result of the DeleteProject operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.DeleteProject
* @see AWS API
* Documentation
*/
DeleteProjectResult deleteProject(DeleteProjectRequest deleteProjectRequest);
/**
*
* Deletes a completed remote access session and its results.
*
*
* @param deleteRemoteAccessSessionRequest
* Represents the request to delete the specified remote access session.
* @return Result of the DeleteRemoteAccessSession operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.DeleteRemoteAccessSession
* @see AWS API Documentation
*/
DeleteRemoteAccessSessionResult deleteRemoteAccessSession(DeleteRemoteAccessSessionRequest deleteRemoteAccessSessionRequest);
/**
*
* Deletes the run, given the run ARN.
*
*
* Deleting this resource does not stop an in-progress run.
*
*
* @param deleteRunRequest
* Represents a request to the delete run operation.
* @return Result of the DeleteRun operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.DeleteRun
* @see AWS API
* Documentation
*/
DeleteRunResult deleteRun(DeleteRunRequest deleteRunRequest);
/**
*
* Deletes a Selenium testing project and all content generated under it.
*
*
*
* You cannot undo this operation.
*
*
*
* You cannot delete a project if it has active sessions.
*
*
*
* @param deleteTestGridProjectRequest
* @return Result of the DeleteTestGridProject operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws CannotDeleteException
* The requested object could not be deleted.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @sample AWSDeviceFarm.DeleteTestGridProject
* @see AWS API Documentation
*/
DeleteTestGridProjectResult deleteTestGridProject(DeleteTestGridProjectRequest deleteTestGridProjectRequest);
/**
*
* Deletes an upload given the upload ARN.
*
*
* @param deleteUploadRequest
* Represents a request to the delete upload operation.
* @return Result of the DeleteUpload operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.DeleteUpload
* @see AWS API
* Documentation
*/
DeleteUploadResult deleteUpload(DeleteUploadRequest deleteUploadRequest);
/**
*
* Deletes a configuration for your Amazon Virtual Private Cloud (VPC) endpoint.
*
*
* @param deleteVPCEConfigurationRequest
* @return Result of the DeleteVPCEConfiguration operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws InvalidOperationException
* There was an error with the update request, or you do not have sufficient permissions to update this VPC
* endpoint configuration.
* @sample AWSDeviceFarm.DeleteVPCEConfiguration
* @see AWS API Documentation
*/
DeleteVPCEConfigurationResult deleteVPCEConfiguration(DeleteVPCEConfigurationRequest deleteVPCEConfigurationRequest);
/**
*
* Returns the number of unmetered iOS or unmetered Android devices that have been purchased by the account.
*
*
* @param getAccountSettingsRequest
* Represents the request sent to retrieve the account settings.
* @return Result of the GetAccountSettings operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.GetAccountSettings
* @see AWS
* API Documentation
*/
GetAccountSettingsResult getAccountSettings(GetAccountSettingsRequest getAccountSettingsRequest);
/**
*
* Gets information about a unique device type.
*
*
* @param getDeviceRequest
* Represents a request to the get device request.
* @return Result of the GetDevice operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.GetDevice
* @see AWS API
* Documentation
*/
GetDeviceResult getDevice(GetDeviceRequest getDeviceRequest);
/**
*
* Returns information about a device instance that belongs to a private device fleet.
*
*
* @param getDeviceInstanceRequest
* @return Result of the GetDeviceInstance operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.GetDeviceInstance
* @see AWS
* API Documentation
*/
GetDeviceInstanceResult getDeviceInstance(GetDeviceInstanceRequest getDeviceInstanceRequest);
/**
*
* Gets information about a device pool.
*
*
* @param getDevicePoolRequest
* Represents a request to the get device pool operation.
* @return Result of the GetDevicePool operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.GetDevicePool
* @see AWS API
* Documentation
*/
GetDevicePoolResult getDevicePool(GetDevicePoolRequest getDevicePoolRequest);
/**
*
* Gets information about compatibility with a device pool.
*
*
* @param getDevicePoolCompatibilityRequest
* Represents a request to the get device pool compatibility operation.
* @return Result of the GetDevicePoolCompatibility operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.GetDevicePoolCompatibility
* @see AWS API Documentation
*/
GetDevicePoolCompatibilityResult getDevicePoolCompatibility(GetDevicePoolCompatibilityRequest getDevicePoolCompatibilityRequest);
/**
*
* Returns information about the specified instance profile.
*
*
* @param getInstanceProfileRequest
* @return Result of the GetInstanceProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.GetInstanceProfile
* @see AWS
* API Documentation
*/
GetInstanceProfileResult getInstanceProfile(GetInstanceProfileRequest getInstanceProfileRequest);
/**
*
* Gets information about a job.
*
*
* @param getJobRequest
* Represents a request to the get job operation.
* @return Result of the GetJob operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.GetJob
* @see AWS API
* Documentation
*/
GetJobResult getJob(GetJobRequest getJobRequest);
/**
*
* Returns information about a network profile.
*
*
* @param getNetworkProfileRequest
* @return Result of the GetNetworkProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.GetNetworkProfile
* @see AWS
* API Documentation
*/
GetNetworkProfileResult getNetworkProfile(GetNetworkProfileRequest getNetworkProfileRequest);
/**
*
* Gets the current status and future status of all offerings purchased by an AWS account. The response indicates
* how many offerings are currently available and the offerings that will be available in the next period. The API
* returns a NotEligible
error if the user is not permitted to invoke the operation. If you must be
* able to invoke this operation, contact [email protected].
*
*
* @param getOfferingStatusRequest
* Represents the request to retrieve the offering status for the specified customer or account.
* @return Result of the GetOfferingStatus operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.GetOfferingStatus
* @see AWS
* API Documentation
*/
GetOfferingStatusResult getOfferingStatus(GetOfferingStatusRequest getOfferingStatusRequest);
/**
*
* Gets information about a project.
*
*
* @param getProjectRequest
* Represents a request to the get project operation.
* @return Result of the GetProject operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.GetProject
* @see AWS API
* Documentation
*/
GetProjectResult getProject(GetProjectRequest getProjectRequest);
/**
*
* Returns a link to a currently running remote access session.
*
*
* @param getRemoteAccessSessionRequest
* Represents the request to get information about the specified remote access session.
* @return Result of the GetRemoteAccessSession operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.GetRemoteAccessSession
* @see AWS API Documentation
*/
GetRemoteAccessSessionResult getRemoteAccessSession(GetRemoteAccessSessionRequest getRemoteAccessSessionRequest);
/**
*
* Gets information about a run.
*
*
* @param getRunRequest
* Represents a request to the get run operation.
* @return Result of the GetRun operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.GetRun
* @see AWS API
* Documentation
*/
GetRunResult getRun(GetRunRequest getRunRequest);
/**
*
* Gets information about a suite.
*
*
* @param getSuiteRequest
* Represents a request to the get suite operation.
* @return Result of the GetSuite operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.GetSuite
* @see AWS API
* Documentation
*/
GetSuiteResult getSuite(GetSuiteRequest getSuiteRequest);
/**
*
* Gets information about a test.
*
*
* @param getTestRequest
* Represents a request to the get test operation.
* @return Result of the GetTest operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.GetTest
* @see AWS API
* Documentation
*/
GetTestResult getTest(GetTestRequest getTestRequest);
/**
*
* Retrieves information about a Selenium testing project.
*
*
* @param getTestGridProjectRequest
* @return Result of the GetTestGridProject operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @sample AWSDeviceFarm.GetTestGridProject
* @see AWS
* API Documentation
*/
GetTestGridProjectResult getTestGridProject(GetTestGridProjectRequest getTestGridProjectRequest);
/**
*
* A session is an instance of a browser created through a RemoteWebDriver
with the URL from
* CreateTestGridUrlResult$url. You can use the following to look up sessions:
*
*
* -
*
* The session ARN (GetTestGridSessionRequest$sessionArn).
*
*
* -
*
* The project ARN and a session ID (GetTestGridSessionRequest$projectArn and
* GetTestGridSessionRequest$sessionId).
*
*
*
*
*
* @param getTestGridSessionRequest
* @return Result of the GetTestGridSession operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @sample AWSDeviceFarm.GetTestGridSession
* @see AWS
* API Documentation
*/
GetTestGridSessionResult getTestGridSession(GetTestGridSessionRequest getTestGridSessionRequest);
/**
*
* Gets information about an upload.
*
*
* @param getUploadRequest
* Represents a request to the get upload operation.
* @return Result of the GetUpload operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.GetUpload
* @see AWS API
* Documentation
*/
GetUploadResult getUpload(GetUploadRequest getUploadRequest);
/**
*
* Returns information about the configuration settings for your Amazon Virtual Private Cloud (VPC) endpoint.
*
*
* @param getVPCEConfigurationRequest
* @return Result of the GetVPCEConfiguration operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.GetVPCEConfiguration
* @see AWS API Documentation
*/
GetVPCEConfigurationResult getVPCEConfiguration(GetVPCEConfigurationRequest getVPCEConfigurationRequest);
/**
*
* Installs an application to the device in a remote access session. For Android applications, the file must be in
* .apk format. For iOS applications, the file must be in .ipa format.
*
*
* @param installToRemoteAccessSessionRequest
* Represents the request to install an Android application (in .apk format) or an iOS application (in .ipa
* format) as part of a remote access session.
* @return Result of the InstallToRemoteAccessSession operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.InstallToRemoteAccessSession
* @see AWS API Documentation
*/
InstallToRemoteAccessSessionResult installToRemoteAccessSession(InstallToRemoteAccessSessionRequest installToRemoteAccessSessionRequest);
/**
*
* Gets information about artifacts.
*
*
* @param listArtifactsRequest
* Represents a request to the list artifacts operation.
* @return Result of the ListArtifacts operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.ListArtifacts
* @see AWS API
* Documentation
*/
ListArtifactsResult listArtifacts(ListArtifactsRequest listArtifactsRequest);
/**
*
* Returns information about the private device instances associated with one or more AWS accounts.
*
*
* @param listDeviceInstancesRequest
* @return Result of the ListDeviceInstances operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.ListDeviceInstances
* @see AWS
* API Documentation
*/
ListDeviceInstancesResult listDeviceInstances(ListDeviceInstancesRequest listDeviceInstancesRequest);
/**
*
* Gets information about device pools.
*
*
* @param listDevicePoolsRequest
* Represents the result of a list device pools request.
* @return Result of the ListDevicePools operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.ListDevicePools
* @see AWS API
* Documentation
*/
ListDevicePoolsResult listDevicePools(ListDevicePoolsRequest listDevicePoolsRequest);
/**
*
* Gets information about unique device types.
*
*
* @param listDevicesRequest
* Represents the result of a list devices request.
* @return Result of the ListDevices operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.ListDevices
* @see AWS API
* Documentation
*/
ListDevicesResult listDevices(ListDevicesRequest listDevicesRequest);
/**
*
* Returns information about all the instance profiles in an AWS account.
*
*
* @param listInstanceProfilesRequest
* @return Result of the ListInstanceProfiles operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.ListInstanceProfiles
* @see AWS API Documentation
*/
ListInstanceProfilesResult listInstanceProfiles(ListInstanceProfilesRequest listInstanceProfilesRequest);
/**
*
* Gets information about jobs for a given test run.
*
*
* @param listJobsRequest
* Represents a request to the list jobs operation.
* @return Result of the ListJobs operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.ListJobs
* @see AWS API
* Documentation
*/
ListJobsResult listJobs(ListJobsRequest listJobsRequest);
/**
*
* Returns the list of available network profiles.
*
*
* @param listNetworkProfilesRequest
* @return Result of the ListNetworkProfiles operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.ListNetworkProfiles
* @see AWS
* API Documentation
*/
ListNetworkProfilesResult listNetworkProfiles(ListNetworkProfilesRequest listNetworkProfilesRequest);
/**
*
* Returns a list of offering promotions. Each offering promotion record contains the ID and description of the
* promotion. The API returns a NotEligible
error if the caller is not permitted to invoke the
* operation. Contact [email protected] if
* you must be able to invoke this operation.
*
*
* @param listOfferingPromotionsRequest
* @return Result of the ListOfferingPromotions operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.ListOfferingPromotions
* @see AWS API Documentation
*/
ListOfferingPromotionsResult listOfferingPromotions(ListOfferingPromotionsRequest listOfferingPromotionsRequest);
/**
*
* Returns a list of all historical purchases, renewals, and system renewal transactions for an AWS account. The
* list is paginated and ordered by a descending timestamp (most recent transactions are first). The API returns a
* NotEligible
error if the user is not permitted to invoke the operation. If you must be able to
* invoke this operation, contact [email protected].
*
*
* @param listOfferingTransactionsRequest
* Represents the request to list the offering transaction history.
* @return Result of the ListOfferingTransactions operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.ListOfferingTransactions
* @see AWS API Documentation
*/
ListOfferingTransactionsResult listOfferingTransactions(ListOfferingTransactionsRequest listOfferingTransactionsRequest);
/**
*
* Returns a list of products or offerings that the user can manage through the API. Each offering record indicates
* the recurring price per unit and the frequency for that offering. The API returns a NotEligible
* error if the user is not permitted to invoke the operation. If you must be able to invoke this operation, contact
* [email protected].
*
*
* @param listOfferingsRequest
* Represents the request to list all offerings.
* @return Result of the ListOfferings operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.ListOfferings
* @see AWS API
* Documentation
*/
ListOfferingsResult listOfferings(ListOfferingsRequest listOfferingsRequest);
/**
*
* Gets information about projects.
*
*
* @param listProjectsRequest
* Represents a request to the list projects operation.
* @return Result of the ListProjects operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.ListProjects
* @see AWS API
* Documentation
*/
ListProjectsResult listProjects(ListProjectsRequest listProjectsRequest);
/**
*
* Returns a list of all currently running remote access sessions.
*
*
* @param listRemoteAccessSessionsRequest
* Represents the request to return information about the remote access session.
* @return Result of the ListRemoteAccessSessions operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.ListRemoteAccessSessions
* @see AWS API Documentation
*/
ListRemoteAccessSessionsResult listRemoteAccessSessions(ListRemoteAccessSessionsRequest listRemoteAccessSessionsRequest);
/**
*
* Gets information about runs, given an AWS Device Farm project ARN.
*
*
* @param listRunsRequest
* Represents a request to the list runs operation.
* @return Result of the ListRuns operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.ListRuns
* @see AWS API
* Documentation
*/
ListRunsResult listRuns(ListRunsRequest listRunsRequest);
/**
*
* Gets information about samples, given an AWS Device Farm job ARN.
*
*
* @param listSamplesRequest
* Represents a request to the list samples operation.
* @return Result of the ListSamples operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.ListSamples
* @see AWS API
* Documentation
*/
ListSamplesResult listSamples(ListSamplesRequest listSamplesRequest);
/**
*
* Gets information about test suites for a given job.
*
*
* @param listSuitesRequest
* Represents a request to the list suites operation.
* @return Result of the ListSuites operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.ListSuites
* @see AWS API
* Documentation
*/
ListSuitesResult listSuites(ListSuitesRequest listSuitesRequest);
/**
*
* List the tags for an AWS Device Farm resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws TagOperationException
* The operation was not successful. Try again.
* @sample AWSDeviceFarm.ListTagsForResource
* @see AWS
* API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Gets a list of all Selenium testing projects in your account.
*
*
* @param listTestGridProjectsRequest
* @return Result of the ListTestGridProjects operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @sample AWSDeviceFarm.ListTestGridProjects
* @see AWS API Documentation
*/
ListTestGridProjectsResult listTestGridProjects(ListTestGridProjectsRequest listTestGridProjectsRequest);
/**
*
* Returns a list of the actions taken in a TestGridSession.
*
*
* @param listTestGridSessionActionsRequest
* @return Result of the ListTestGridSessionActions operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @sample AWSDeviceFarm.ListTestGridSessionActions
* @see AWS API Documentation
*/
ListTestGridSessionActionsResult listTestGridSessionActions(ListTestGridSessionActionsRequest listTestGridSessionActionsRequest);
/**
*
* Retrieves a list of artifacts created during the session.
*
*
* @param listTestGridSessionArtifactsRequest
* @return Result of the ListTestGridSessionArtifacts operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @sample AWSDeviceFarm.ListTestGridSessionArtifacts
* @see AWS API Documentation
*/
ListTestGridSessionArtifactsResult listTestGridSessionArtifacts(ListTestGridSessionArtifactsRequest listTestGridSessionArtifactsRequest);
/**
*
* Retrieves a list of sessions for a TestGridProject.
*
*
* @param listTestGridSessionsRequest
* @return Result of the ListTestGridSessions operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @sample AWSDeviceFarm.ListTestGridSessions
* @see AWS API Documentation
*/
ListTestGridSessionsResult listTestGridSessions(ListTestGridSessionsRequest listTestGridSessionsRequest);
/**
*
* Gets information about tests in a given test suite.
*
*
* @param listTestsRequest
* Represents a request to the list tests operation.
* @return Result of the ListTests operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.ListTests
* @see AWS API
* Documentation
*/
ListTestsResult listTests(ListTestsRequest listTestsRequest);
/**
*
* Gets information about unique problems, such as exceptions or crashes.
*
*
* Unique problems are defined as a single instance of an error across a run, job, or suite. For example, if a call
* in your application consistently raises an exception (OutOfBoundsException in MyActivity.java:386
),
* ListUniqueProblems
returns a single entry instead of many individual entries for that exception.
*
*
* @param listUniqueProblemsRequest
* Represents a request to the list unique problems operation.
* @return Result of the ListUniqueProblems operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.ListUniqueProblems
* @see AWS
* API Documentation
*/
ListUniqueProblemsResult listUniqueProblems(ListUniqueProblemsRequest listUniqueProblemsRequest);
/**
*
* Gets information about uploads, given an AWS Device Farm project ARN.
*
*
* @param listUploadsRequest
* Represents a request to the list uploads operation.
* @return Result of the ListUploads operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.ListUploads
* @see AWS API
* Documentation
*/
ListUploadsResult listUploads(ListUploadsRequest listUploadsRequest);
/**
*
* Returns information about all Amazon Virtual Private Cloud (VPC) endpoint configurations in the AWS account.
*
*
* @param listVPCEConfigurationsRequest
* @return Result of the ListVPCEConfigurations operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.ListVPCEConfigurations
* @see AWS API Documentation
*/
ListVPCEConfigurationsResult listVPCEConfigurations(ListVPCEConfigurationsRequest listVPCEConfigurationsRequest);
/**
*
* Immediately purchases offerings for an AWS account. Offerings renew with the latest total purchased quantity for
* an offering, unless the renewal was overridden. The API returns a NotEligible
error if the user is
* not permitted to invoke the operation. If you must be able to invoke this operation, contact [email protected].
*
*
* @param purchaseOfferingRequest
* Represents a request for a purchase offering.
* @return Result of the PurchaseOffering operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.PurchaseOffering
* @see AWS
* API Documentation
*/
PurchaseOfferingResult purchaseOffering(PurchaseOfferingRequest purchaseOfferingRequest);
/**
*
* Explicitly sets the quantity of devices to renew for an offering, starting from the effectiveDate
of
* the next period. The API returns a NotEligible
error if the user is not permitted to invoke the
* operation. If you must be able to invoke this operation, contact [email protected].
*
*
* @param renewOfferingRequest
* A request that represents an offering renewal.
* @return Result of the RenewOffering operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.RenewOffering
* @see AWS API
* Documentation
*/
RenewOfferingResult renewOffering(RenewOfferingRequest renewOfferingRequest);
/**
*
* Schedules a run.
*
*
* @param scheduleRunRequest
* Represents a request to the schedule run operation.
* @return Result of the ScheduleRun operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws IdempotencyException
* An entity with the same name already exists.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.ScheduleRun
* @see AWS API
* Documentation
*/
ScheduleRunResult scheduleRun(ScheduleRunRequest scheduleRunRequest);
/**
*
* Initiates a stop request for the current job. AWS Device Farm immediately stops the job on the device where tests
* have not started. You are not billed for this device. On the device where tests have started, setup suite and
* teardown suite tests run to completion on the device. You are billed for setup, teardown, and any tests that were
* in progress or already completed.
*
*
* @param stopJobRequest
* @return Result of the StopJob operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.StopJob
* @see AWS API
* Documentation
*/
StopJobResult stopJob(StopJobRequest stopJobRequest);
/**
*
* Ends a specified remote access session.
*
*
* @param stopRemoteAccessSessionRequest
* Represents the request to stop the remote access session.
* @return Result of the StopRemoteAccessSession operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.StopRemoteAccessSession
* @see AWS API Documentation
*/
StopRemoteAccessSessionResult stopRemoteAccessSession(StopRemoteAccessSessionRequest stopRemoteAccessSessionRequest);
/**
*
* Initiates a stop request for the current test run. AWS Device Farm immediately stops the run on devices where
* tests have not started. You are not billed for these devices. On devices where tests have started executing,
* setup suite and teardown suite tests run to completion on those devices. You are billed for setup, teardown, and
* any tests that were in progress or already completed.
*
*
* @param stopRunRequest
* Represents the request to stop a specific run.
* @return Result of the StopRun operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.StopRun
* @see AWS API
* Documentation
*/
StopRunResult stopRun(StopRunRequest stopRunRequest);
/**
*
* Associates the specified tags to a resource with the specified resourceArn
. If existing tags on a
* resource are not specified in the request parameters, they are not changed. When a resource is deleted, the tags
* associated with that resource are also deleted.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws TagOperationException
* The operation was not successful. Try again.
* @throws TooManyTagsException
* The list of tags on the repository is over the limit. The maximum number of tags that can be applied to a
* repository is 50.
* @throws TagPolicyException
* The request doesn't comply with the AWS Identity and Access Management (IAM) tag policy. Correct your
* request and then retry it.
* @sample AWSDeviceFarm.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Deletes the specified tags from a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws TagOperationException
* The operation was not successful. Try again.
* @sample AWSDeviceFarm.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates information about a private device instance.
*
*
* @param updateDeviceInstanceRequest
* @return Result of the UpdateDeviceInstance operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.UpdateDeviceInstance
* @see AWS API Documentation
*/
UpdateDeviceInstanceResult updateDeviceInstance(UpdateDeviceInstanceRequest updateDeviceInstanceRequest);
/**
*
* Modifies the name, description, and rules in a device pool given the attributes and the pool ARN. Rule updates
* are all-or-nothing, meaning they can only be updated as a whole (or not at all).
*
*
* @param updateDevicePoolRequest
* Represents a request to the update device pool operation.
* @return Result of the UpdateDevicePool operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.UpdateDevicePool
* @see AWS
* API Documentation
*/
UpdateDevicePoolResult updateDevicePool(UpdateDevicePoolRequest updateDevicePoolRequest);
/**
*
* Updates information about an existing private device instance profile.
*
*
* @param updateInstanceProfileRequest
* @return Result of the UpdateInstanceProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.UpdateInstanceProfile
* @see AWS API Documentation
*/
UpdateInstanceProfileResult updateInstanceProfile(UpdateInstanceProfileRequest updateInstanceProfileRequest);
/**
*
* Updates the network profile.
*
*
* @param updateNetworkProfileRequest
* @return Result of the UpdateNetworkProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.UpdateNetworkProfile
* @see AWS API Documentation
*/
UpdateNetworkProfileResult updateNetworkProfile(UpdateNetworkProfileRequest updateNetworkProfileRequest);
/**
*
* Modifies the specified project name, given the project ARN and a new name.
*
*
* @param updateProjectRequest
* Represents a request to the update project operation.
* @return Result of the UpdateProject operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.UpdateProject
* @see AWS API
* Documentation
*/
UpdateProjectResult updateProject(UpdateProjectRequest updateProjectRequest);
/**
*
* Change details of a project.
*
*
* @param updateTestGridProjectRequest
* @return Result of the UpdateTestGridProject operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws LimitExceededException
* A limit was exceeded.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @sample AWSDeviceFarm.UpdateTestGridProject
* @see AWS API Documentation
*/
UpdateTestGridProjectResult updateTestGridProject(UpdateTestGridProjectRequest updateTestGridProjectRequest);
/**
*
* Updates an uploaded test spec.
*
*
* @param updateUploadRequest
* @return Result of the UpdateUpload operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @sample AWSDeviceFarm.UpdateUpload
* @see AWS API
* Documentation
*/
UpdateUploadResult updateUpload(UpdateUploadRequest updateUploadRequest);
/**
*
* Updates information about an Amazon Virtual Private Cloud (VPC) endpoint configuration.
*
*
* @param updateVPCEConfigurationRequest
* @return Result of the UpdateVPCEConfiguration operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws InvalidOperationException
* There was an error with the update request, or you do not have sufficient permissions to update this VPC
* endpoint configuration.
* @sample AWSDeviceFarm.UpdateVPCEConfiguration
* @see AWS API Documentation
*/
UpdateVPCEConfigurationResult updateVPCEConfiguration(UpdateVPCEConfigurationRequest updateVPCEConfigurationRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}