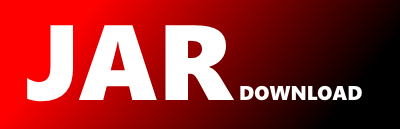
com.amazonaws.services.devicefarm.model.AccountSettings Maven / Gradle / Ivy
Show all versions of aws-java-sdk-devicefarm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.devicefarm.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* A container for account-level settings in AWS Device Farm.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AccountSettings implements Serializable, Cloneable, StructuredPojo {
/**
*
* The AWS account number specified in the AccountSettings
container.
*
*/
private String awsAccountNumber;
/**
*
* Returns the unmetered devices you have purchased or want to purchase.
*
*/
private java.util.Map unmeteredDevices;
/**
*
* Returns the unmetered remote access devices you have purchased or want to purchase.
*
*/
private java.util.Map unmeteredRemoteAccessDevices;
/**
*
* The maximum number of minutes a test run executes before it times out.
*
*/
private Integer maxJobTimeoutMinutes;
/**
*
* Information about an AWS account's usage of free trial device minutes.
*
*/
private TrialMinutes trialMinutes;
/**
*
* The maximum number of device slots that the AWS account can purchase. Each maximum is expressed as an
* offering-id:number
pair, where the offering-id
represents one of the IDs returned by
* the ListOfferings
command.
*
*/
private java.util.Map maxSlots;
/**
*
* The default number of minutes (at the account level) a test run executes before it times out. The default value
* is 150 minutes.
*
*/
private Integer defaultJobTimeoutMinutes;
/**
*
* When set to true
, for private devices, Device Farm does not sign your app again. For public devices,
* Device Farm always signs your apps again.
*
*
* For more information about how Device Farm re-signs your apps, see Do you modify my app? in the AWS Device Farm FAQs.
*
*/
private Boolean skipAppResign;
/**
*
* The AWS account number specified in the AccountSettings
container.
*
*
* @param awsAccountNumber
* The AWS account number specified in the AccountSettings
container.
*/
public void setAwsAccountNumber(String awsAccountNumber) {
this.awsAccountNumber = awsAccountNumber;
}
/**
*
* The AWS account number specified in the AccountSettings
container.
*
*
* @return The AWS account number specified in the AccountSettings
container.
*/
public String getAwsAccountNumber() {
return this.awsAccountNumber;
}
/**
*
* The AWS account number specified in the AccountSettings
container.
*
*
* @param awsAccountNumber
* The AWS account number specified in the AccountSettings
container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AccountSettings withAwsAccountNumber(String awsAccountNumber) {
setAwsAccountNumber(awsAccountNumber);
return this;
}
/**
*
* Returns the unmetered devices you have purchased or want to purchase.
*
*
* @return Returns the unmetered devices you have purchased or want to purchase.
*/
public java.util.Map getUnmeteredDevices() {
return unmeteredDevices;
}
/**
*
* Returns the unmetered devices you have purchased or want to purchase.
*
*
* @param unmeteredDevices
* Returns the unmetered devices you have purchased or want to purchase.
*/
public void setUnmeteredDevices(java.util.Map unmeteredDevices) {
this.unmeteredDevices = unmeteredDevices;
}
/**
*
* Returns the unmetered devices you have purchased or want to purchase.
*
*
* @param unmeteredDevices
* Returns the unmetered devices you have purchased or want to purchase.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AccountSettings withUnmeteredDevices(java.util.Map unmeteredDevices) {
setUnmeteredDevices(unmeteredDevices);
return this;
}
/**
* Add a single UnmeteredDevices entry
*
* @see AccountSettings#withUnmeteredDevices
* @returns a reference to this object so that method calls can be chained together.
*/
public AccountSettings addUnmeteredDevicesEntry(String key, Integer value) {
if (null == this.unmeteredDevices) {
this.unmeteredDevices = new java.util.HashMap();
}
if (this.unmeteredDevices.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.unmeteredDevices.put(key, value);
return this;
}
/**
* Removes all the entries added into UnmeteredDevices.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AccountSettings clearUnmeteredDevicesEntries() {
this.unmeteredDevices = null;
return this;
}
/**
*
* Returns the unmetered remote access devices you have purchased or want to purchase.
*
*
* @return Returns the unmetered remote access devices you have purchased or want to purchase.
*/
public java.util.Map getUnmeteredRemoteAccessDevices() {
return unmeteredRemoteAccessDevices;
}
/**
*
* Returns the unmetered remote access devices you have purchased or want to purchase.
*
*
* @param unmeteredRemoteAccessDevices
* Returns the unmetered remote access devices you have purchased or want to purchase.
*/
public void setUnmeteredRemoteAccessDevices(java.util.Map unmeteredRemoteAccessDevices) {
this.unmeteredRemoteAccessDevices = unmeteredRemoteAccessDevices;
}
/**
*
* Returns the unmetered remote access devices you have purchased or want to purchase.
*
*
* @param unmeteredRemoteAccessDevices
* Returns the unmetered remote access devices you have purchased or want to purchase.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AccountSettings withUnmeteredRemoteAccessDevices(java.util.Map unmeteredRemoteAccessDevices) {
setUnmeteredRemoteAccessDevices(unmeteredRemoteAccessDevices);
return this;
}
/**
* Add a single UnmeteredRemoteAccessDevices entry
*
* @see AccountSettings#withUnmeteredRemoteAccessDevices
* @returns a reference to this object so that method calls can be chained together.
*/
public AccountSettings addUnmeteredRemoteAccessDevicesEntry(String key, Integer value) {
if (null == this.unmeteredRemoteAccessDevices) {
this.unmeteredRemoteAccessDevices = new java.util.HashMap();
}
if (this.unmeteredRemoteAccessDevices.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.unmeteredRemoteAccessDevices.put(key, value);
return this;
}
/**
* Removes all the entries added into UnmeteredRemoteAccessDevices.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AccountSettings clearUnmeteredRemoteAccessDevicesEntries() {
this.unmeteredRemoteAccessDevices = null;
return this;
}
/**
*
* The maximum number of minutes a test run executes before it times out.
*
*
* @param maxJobTimeoutMinutes
* The maximum number of minutes a test run executes before it times out.
*/
public void setMaxJobTimeoutMinutes(Integer maxJobTimeoutMinutes) {
this.maxJobTimeoutMinutes = maxJobTimeoutMinutes;
}
/**
*
* The maximum number of minutes a test run executes before it times out.
*
*
* @return The maximum number of minutes a test run executes before it times out.
*/
public Integer getMaxJobTimeoutMinutes() {
return this.maxJobTimeoutMinutes;
}
/**
*
* The maximum number of minutes a test run executes before it times out.
*
*
* @param maxJobTimeoutMinutes
* The maximum number of minutes a test run executes before it times out.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AccountSettings withMaxJobTimeoutMinutes(Integer maxJobTimeoutMinutes) {
setMaxJobTimeoutMinutes(maxJobTimeoutMinutes);
return this;
}
/**
*
* Information about an AWS account's usage of free trial device minutes.
*
*
* @param trialMinutes
* Information about an AWS account's usage of free trial device minutes.
*/
public void setTrialMinutes(TrialMinutes trialMinutes) {
this.trialMinutes = trialMinutes;
}
/**
*
* Information about an AWS account's usage of free trial device minutes.
*
*
* @return Information about an AWS account's usage of free trial device minutes.
*/
public TrialMinutes getTrialMinutes() {
return this.trialMinutes;
}
/**
*
* Information about an AWS account's usage of free trial device minutes.
*
*
* @param trialMinutes
* Information about an AWS account's usage of free trial device minutes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AccountSettings withTrialMinutes(TrialMinutes trialMinutes) {
setTrialMinutes(trialMinutes);
return this;
}
/**
*
* The maximum number of device slots that the AWS account can purchase. Each maximum is expressed as an
* offering-id:number
pair, where the offering-id
represents one of the IDs returned by
* the ListOfferings
command.
*
*
* @return The maximum number of device slots that the AWS account can purchase. Each maximum is expressed as an
* offering-id:number
pair, where the offering-id
represents one of the IDs
* returned by the ListOfferings
command.
*/
public java.util.Map getMaxSlots() {
return maxSlots;
}
/**
*
* The maximum number of device slots that the AWS account can purchase. Each maximum is expressed as an
* offering-id:number
pair, where the offering-id
represents one of the IDs returned by
* the ListOfferings
command.
*
*
* @param maxSlots
* The maximum number of device slots that the AWS account can purchase. Each maximum is expressed as an
* offering-id:number
pair, where the offering-id
represents one of the IDs
* returned by the ListOfferings
command.
*/
public void setMaxSlots(java.util.Map maxSlots) {
this.maxSlots = maxSlots;
}
/**
*
* The maximum number of device slots that the AWS account can purchase. Each maximum is expressed as an
* offering-id:number
pair, where the offering-id
represents one of the IDs returned by
* the ListOfferings
command.
*
*
* @param maxSlots
* The maximum number of device slots that the AWS account can purchase. Each maximum is expressed as an
* offering-id:number
pair, where the offering-id
represents one of the IDs
* returned by the ListOfferings
command.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AccountSettings withMaxSlots(java.util.Map maxSlots) {
setMaxSlots(maxSlots);
return this;
}
/**
* Add a single MaxSlots entry
*
* @see AccountSettings#withMaxSlots
* @returns a reference to this object so that method calls can be chained together.
*/
public AccountSettings addMaxSlotsEntry(String key, Integer value) {
if (null == this.maxSlots) {
this.maxSlots = new java.util.HashMap();
}
if (this.maxSlots.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.maxSlots.put(key, value);
return this;
}
/**
* Removes all the entries added into MaxSlots.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AccountSettings clearMaxSlotsEntries() {
this.maxSlots = null;
return this;
}
/**
*
* The default number of minutes (at the account level) a test run executes before it times out. The default value
* is 150 minutes.
*
*
* @param defaultJobTimeoutMinutes
* The default number of minutes (at the account level) a test run executes before it times out. The default
* value is 150 minutes.
*/
public void setDefaultJobTimeoutMinutes(Integer defaultJobTimeoutMinutes) {
this.defaultJobTimeoutMinutes = defaultJobTimeoutMinutes;
}
/**
*
* The default number of minutes (at the account level) a test run executes before it times out. The default value
* is 150 minutes.
*
*
* @return The default number of minutes (at the account level) a test run executes before it times out. The default
* value is 150 minutes.
*/
public Integer getDefaultJobTimeoutMinutes() {
return this.defaultJobTimeoutMinutes;
}
/**
*
* The default number of minutes (at the account level) a test run executes before it times out. The default value
* is 150 minutes.
*
*
* @param defaultJobTimeoutMinutes
* The default number of minutes (at the account level) a test run executes before it times out. The default
* value is 150 minutes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AccountSettings withDefaultJobTimeoutMinutes(Integer defaultJobTimeoutMinutes) {
setDefaultJobTimeoutMinutes(defaultJobTimeoutMinutes);
return this;
}
/**
*
* When set to true
, for private devices, Device Farm does not sign your app again. For public devices,
* Device Farm always signs your apps again.
*
*
* For more information about how Device Farm re-signs your apps, see Do you modify my app? in the AWS Device Farm FAQs.
*
*
* @param skipAppResign
* When set to true
, for private devices, Device Farm does not sign your app again. For public
* devices, Device Farm always signs your apps again.
*
* For more information about how Device Farm re-signs your apps, see Do you modify my app? in the AWS Device Farm
* FAQs.
*/
public void setSkipAppResign(Boolean skipAppResign) {
this.skipAppResign = skipAppResign;
}
/**
*
* When set to true
, for private devices, Device Farm does not sign your app again. For public devices,
* Device Farm always signs your apps again.
*
*
* For more information about how Device Farm re-signs your apps, see Do you modify my app? in the AWS Device Farm FAQs.
*
*
* @return When set to true
, for private devices, Device Farm does not sign your app again. For public
* devices, Device Farm always signs your apps again.
*
* For more information about how Device Farm re-signs your apps, see Do you modify my app? in the AWS Device Farm
* FAQs.
*/
public Boolean getSkipAppResign() {
return this.skipAppResign;
}
/**
*
* When set to true
, for private devices, Device Farm does not sign your app again. For public devices,
* Device Farm always signs your apps again.
*
*
* For more information about how Device Farm re-signs your apps, see Do you modify my app? in the AWS Device Farm FAQs.
*
*
* @param skipAppResign
* When set to true
, for private devices, Device Farm does not sign your app again. For public
* devices, Device Farm always signs your apps again.
*
* For more information about how Device Farm re-signs your apps, see Do you modify my app? in the AWS Device Farm
* FAQs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AccountSettings withSkipAppResign(Boolean skipAppResign) {
setSkipAppResign(skipAppResign);
return this;
}
/**
*
* When set to true
, for private devices, Device Farm does not sign your app again. For public devices,
* Device Farm always signs your apps again.
*
*
* For more information about how Device Farm re-signs your apps, see Do you modify my app? in the AWS Device Farm FAQs.
*
*
* @return When set to true
, for private devices, Device Farm does not sign your app again. For public
* devices, Device Farm always signs your apps again.
*
* For more information about how Device Farm re-signs your apps, see Do you modify my app? in the AWS Device Farm
* FAQs.
*/
public Boolean isSkipAppResign() {
return this.skipAppResign;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAwsAccountNumber() != null)
sb.append("AwsAccountNumber: ").append(getAwsAccountNumber()).append(",");
if (getUnmeteredDevices() != null)
sb.append("UnmeteredDevices: ").append(getUnmeteredDevices()).append(",");
if (getUnmeteredRemoteAccessDevices() != null)
sb.append("UnmeteredRemoteAccessDevices: ").append(getUnmeteredRemoteAccessDevices()).append(",");
if (getMaxJobTimeoutMinutes() != null)
sb.append("MaxJobTimeoutMinutes: ").append(getMaxJobTimeoutMinutes()).append(",");
if (getTrialMinutes() != null)
sb.append("TrialMinutes: ").append(getTrialMinutes()).append(",");
if (getMaxSlots() != null)
sb.append("MaxSlots: ").append(getMaxSlots()).append(",");
if (getDefaultJobTimeoutMinutes() != null)
sb.append("DefaultJobTimeoutMinutes: ").append(getDefaultJobTimeoutMinutes()).append(",");
if (getSkipAppResign() != null)
sb.append("SkipAppResign: ").append(getSkipAppResign());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AccountSettings == false)
return false;
AccountSettings other = (AccountSettings) obj;
if (other.getAwsAccountNumber() == null ^ this.getAwsAccountNumber() == null)
return false;
if (other.getAwsAccountNumber() != null && other.getAwsAccountNumber().equals(this.getAwsAccountNumber()) == false)
return false;
if (other.getUnmeteredDevices() == null ^ this.getUnmeteredDevices() == null)
return false;
if (other.getUnmeteredDevices() != null && other.getUnmeteredDevices().equals(this.getUnmeteredDevices()) == false)
return false;
if (other.getUnmeteredRemoteAccessDevices() == null ^ this.getUnmeteredRemoteAccessDevices() == null)
return false;
if (other.getUnmeteredRemoteAccessDevices() != null && other.getUnmeteredRemoteAccessDevices().equals(this.getUnmeteredRemoteAccessDevices()) == false)
return false;
if (other.getMaxJobTimeoutMinutes() == null ^ this.getMaxJobTimeoutMinutes() == null)
return false;
if (other.getMaxJobTimeoutMinutes() != null && other.getMaxJobTimeoutMinutes().equals(this.getMaxJobTimeoutMinutes()) == false)
return false;
if (other.getTrialMinutes() == null ^ this.getTrialMinutes() == null)
return false;
if (other.getTrialMinutes() != null && other.getTrialMinutes().equals(this.getTrialMinutes()) == false)
return false;
if (other.getMaxSlots() == null ^ this.getMaxSlots() == null)
return false;
if (other.getMaxSlots() != null && other.getMaxSlots().equals(this.getMaxSlots()) == false)
return false;
if (other.getDefaultJobTimeoutMinutes() == null ^ this.getDefaultJobTimeoutMinutes() == null)
return false;
if (other.getDefaultJobTimeoutMinutes() != null && other.getDefaultJobTimeoutMinutes().equals(this.getDefaultJobTimeoutMinutes()) == false)
return false;
if (other.getSkipAppResign() == null ^ this.getSkipAppResign() == null)
return false;
if (other.getSkipAppResign() != null && other.getSkipAppResign().equals(this.getSkipAppResign()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAwsAccountNumber() == null) ? 0 : getAwsAccountNumber().hashCode());
hashCode = prime * hashCode + ((getUnmeteredDevices() == null) ? 0 : getUnmeteredDevices().hashCode());
hashCode = prime * hashCode + ((getUnmeteredRemoteAccessDevices() == null) ? 0 : getUnmeteredRemoteAccessDevices().hashCode());
hashCode = prime * hashCode + ((getMaxJobTimeoutMinutes() == null) ? 0 : getMaxJobTimeoutMinutes().hashCode());
hashCode = prime * hashCode + ((getTrialMinutes() == null) ? 0 : getTrialMinutes().hashCode());
hashCode = prime * hashCode + ((getMaxSlots() == null) ? 0 : getMaxSlots().hashCode());
hashCode = prime * hashCode + ((getDefaultJobTimeoutMinutes() == null) ? 0 : getDefaultJobTimeoutMinutes().hashCode());
hashCode = prime * hashCode + ((getSkipAppResign() == null) ? 0 : getSkipAppResign().hashCode());
return hashCode;
}
@Override
public AccountSettings clone() {
try {
return (AccountSettings) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.devicefarm.model.transform.AccountSettingsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}