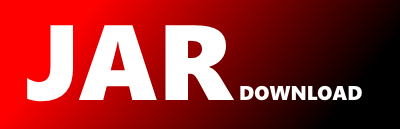
com.amazonaws.services.devicefarm.model.CreateRemoteAccessSessionRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-devicefarm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.devicefarm.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* Creates and submits a request to start a remote access session.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateRemoteAccessSessionRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The Amazon Resource Name (ARN) of the project for which you want to create a remote access session.
*
*/
private String projectArn;
/**
*
* The ARN of the device for which you want to create a remote access session.
*
*/
private String deviceArn;
/**
*
* The Amazon Resource Name (ARN) of the device instance for which you want to create a remote access session.
*
*/
private String instanceArn;
/**
*
* Ignored. The public key of the ssh
key pair you want to use for connecting to remote devices in your
* remote debugging session. This key is required only if remoteDebugEnabled
is set to
* true
.
*
*
* Remote debugging is no longer
* supported.
*
*/
private String sshPublicKey;
/**
*
* Set to true
if you want to access devices remotely for debugging in your remote access session.
*
*
* Remote debugging is no longer
* supported.
*
*/
private Boolean remoteDebugEnabled;
/**
*
* Set to true
to enable remote recording for the remote access session.
*
*/
private Boolean remoteRecordEnabled;
/**
*
* The Amazon Resource Name (ARN) for the app to be recorded in the remote access session.
*
*/
private String remoteRecordAppArn;
/**
*
* The name of the remote access session to create.
*
*/
private String name;
/**
*
* Unique identifier for the client. If you want access to multiple devices on the same client, you should pass the
* same clientId
value in each call to CreateRemoteAccessSession
. This identifier is
* required only if remoteDebugEnabled
is set to true
.
*
*
* Remote debugging is no longer
* supported.
*
*/
private String clientId;
/**
*
* The configuration information for the remote access session request.
*
*/
private CreateRemoteAccessSessionConfiguration configuration;
/**
*
* The interaction mode of the remote access session. Valid values are:
*
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You cannot run
* XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode has the
* fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest framework-based tests
* and watch the screen in this mode.
*
*
*
*/
private String interactionMode;
/**
*
* When set to true
, for private devices, Device Farm does not sign your app again. For public devices,
* Device Farm always signs your apps again.
*
*
* For more information on how Device Farm modifies your uploads during tests, see Do you modify my app?
*
*/
private Boolean skipAppResign;
/**
*
* The Amazon Resource Name (ARN) of the project for which you want to create a remote access session.
*
*
* @param projectArn
* The Amazon Resource Name (ARN) of the project for which you want to create a remote access session.
*/
public void setProjectArn(String projectArn) {
this.projectArn = projectArn;
}
/**
*
* The Amazon Resource Name (ARN) of the project for which you want to create a remote access session.
*
*
* @return The Amazon Resource Name (ARN) of the project for which you want to create a remote access session.
*/
public String getProjectArn() {
return this.projectArn;
}
/**
*
* The Amazon Resource Name (ARN) of the project for which you want to create a remote access session.
*
*
* @param projectArn
* The Amazon Resource Name (ARN) of the project for which you want to create a remote access session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRemoteAccessSessionRequest withProjectArn(String projectArn) {
setProjectArn(projectArn);
return this;
}
/**
*
* The ARN of the device for which you want to create a remote access session.
*
*
* @param deviceArn
* The ARN of the device for which you want to create a remote access session.
*/
public void setDeviceArn(String deviceArn) {
this.deviceArn = deviceArn;
}
/**
*
* The ARN of the device for which you want to create a remote access session.
*
*
* @return The ARN of the device for which you want to create a remote access session.
*/
public String getDeviceArn() {
return this.deviceArn;
}
/**
*
* The ARN of the device for which you want to create a remote access session.
*
*
* @param deviceArn
* The ARN of the device for which you want to create a remote access session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRemoteAccessSessionRequest withDeviceArn(String deviceArn) {
setDeviceArn(deviceArn);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the device instance for which you want to create a remote access session.
*
*
* @param instanceArn
* The Amazon Resource Name (ARN) of the device instance for which you want to create a remote access
* session.
*/
public void setInstanceArn(String instanceArn) {
this.instanceArn = instanceArn;
}
/**
*
* The Amazon Resource Name (ARN) of the device instance for which you want to create a remote access session.
*
*
* @return The Amazon Resource Name (ARN) of the device instance for which you want to create a remote access
* session.
*/
public String getInstanceArn() {
return this.instanceArn;
}
/**
*
* The Amazon Resource Name (ARN) of the device instance for which you want to create a remote access session.
*
*
* @param instanceArn
* The Amazon Resource Name (ARN) of the device instance for which you want to create a remote access
* session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRemoteAccessSessionRequest withInstanceArn(String instanceArn) {
setInstanceArn(instanceArn);
return this;
}
/**
*
* Ignored. The public key of the ssh
key pair you want to use for connecting to remote devices in your
* remote debugging session. This key is required only if remoteDebugEnabled
is set to
* true
.
*
*
* Remote debugging is no longer
* supported.
*
*
* @param sshPublicKey
* Ignored. The public key of the ssh
key pair you want to use for connecting to remote devices
* in your remote debugging session. This key is required only if remoteDebugEnabled
is set to
* true
.
*
* Remote debugging is no
* longer supported.
*/
public void setSshPublicKey(String sshPublicKey) {
this.sshPublicKey = sshPublicKey;
}
/**
*
* Ignored. The public key of the ssh
key pair you want to use for connecting to remote devices in your
* remote debugging session. This key is required only if remoteDebugEnabled
is set to
* true
.
*
*
* Remote debugging is no longer
* supported.
*
*
* @return Ignored. The public key of the ssh
key pair you want to use for connecting to remote devices
* in your remote debugging session. This key is required only if remoteDebugEnabled
is set to
* true
.
*
* Remote debugging is no longer supported.
*/
public String getSshPublicKey() {
return this.sshPublicKey;
}
/**
*
* Ignored. The public key of the ssh
key pair you want to use for connecting to remote devices in your
* remote debugging session. This key is required only if remoteDebugEnabled
is set to
* true
.
*
*
* Remote debugging is no longer
* supported.
*
*
* @param sshPublicKey
* Ignored. The public key of the ssh
key pair you want to use for connecting to remote devices
* in your remote debugging session. This key is required only if remoteDebugEnabled
is set to
* true
.
*
* Remote debugging is no
* longer supported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRemoteAccessSessionRequest withSshPublicKey(String sshPublicKey) {
setSshPublicKey(sshPublicKey);
return this;
}
/**
*
* Set to true
if you want to access devices remotely for debugging in your remote access session.
*
*
* Remote debugging is no longer
* supported.
*
*
* @param remoteDebugEnabled
* Set to true
if you want to access devices remotely for debugging in your remote access
* session.
*
* Remote debugging is no
* longer supported.
*/
public void setRemoteDebugEnabled(Boolean remoteDebugEnabled) {
this.remoteDebugEnabled = remoteDebugEnabled;
}
/**
*
* Set to true
if you want to access devices remotely for debugging in your remote access session.
*
*
* Remote debugging is no longer
* supported.
*
*
* @return Set to true
if you want to access devices remotely for debugging in your remote access
* session.
*
* Remote debugging is no longer supported.
*/
public Boolean getRemoteDebugEnabled() {
return this.remoteDebugEnabled;
}
/**
*
* Set to true
if you want to access devices remotely for debugging in your remote access session.
*
*
* Remote debugging is no longer
* supported.
*
*
* @param remoteDebugEnabled
* Set to true
if you want to access devices remotely for debugging in your remote access
* session.
*
* Remote debugging is no
* longer supported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRemoteAccessSessionRequest withRemoteDebugEnabled(Boolean remoteDebugEnabled) {
setRemoteDebugEnabled(remoteDebugEnabled);
return this;
}
/**
*
* Set to true
if you want to access devices remotely for debugging in your remote access session.
*
*
* Remote debugging is no longer
* supported.
*
*
* @return Set to true
if you want to access devices remotely for debugging in your remote access
* session.
*
* Remote debugging is no longer supported.
*/
public Boolean isRemoteDebugEnabled() {
return this.remoteDebugEnabled;
}
/**
*
* Set to true
to enable remote recording for the remote access session.
*
*
* @param remoteRecordEnabled
* Set to true
to enable remote recording for the remote access session.
*/
public void setRemoteRecordEnabled(Boolean remoteRecordEnabled) {
this.remoteRecordEnabled = remoteRecordEnabled;
}
/**
*
* Set to true
to enable remote recording for the remote access session.
*
*
* @return Set to true
to enable remote recording for the remote access session.
*/
public Boolean getRemoteRecordEnabled() {
return this.remoteRecordEnabled;
}
/**
*
* Set to true
to enable remote recording for the remote access session.
*
*
* @param remoteRecordEnabled
* Set to true
to enable remote recording for the remote access session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRemoteAccessSessionRequest withRemoteRecordEnabled(Boolean remoteRecordEnabled) {
setRemoteRecordEnabled(remoteRecordEnabled);
return this;
}
/**
*
* Set to true
to enable remote recording for the remote access session.
*
*
* @return Set to true
to enable remote recording for the remote access session.
*/
public Boolean isRemoteRecordEnabled() {
return this.remoteRecordEnabled;
}
/**
*
* The Amazon Resource Name (ARN) for the app to be recorded in the remote access session.
*
*
* @param remoteRecordAppArn
* The Amazon Resource Name (ARN) for the app to be recorded in the remote access session.
*/
public void setRemoteRecordAppArn(String remoteRecordAppArn) {
this.remoteRecordAppArn = remoteRecordAppArn;
}
/**
*
* The Amazon Resource Name (ARN) for the app to be recorded in the remote access session.
*
*
* @return The Amazon Resource Name (ARN) for the app to be recorded in the remote access session.
*/
public String getRemoteRecordAppArn() {
return this.remoteRecordAppArn;
}
/**
*
* The Amazon Resource Name (ARN) for the app to be recorded in the remote access session.
*
*
* @param remoteRecordAppArn
* The Amazon Resource Name (ARN) for the app to be recorded in the remote access session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRemoteAccessSessionRequest withRemoteRecordAppArn(String remoteRecordAppArn) {
setRemoteRecordAppArn(remoteRecordAppArn);
return this;
}
/**
*
* The name of the remote access session to create.
*
*
* @param name
* The name of the remote access session to create.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the remote access session to create.
*
*
* @return The name of the remote access session to create.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the remote access session to create.
*
*
* @param name
* The name of the remote access session to create.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRemoteAccessSessionRequest withName(String name) {
setName(name);
return this;
}
/**
*
* Unique identifier for the client. If you want access to multiple devices on the same client, you should pass the
* same clientId
value in each call to CreateRemoteAccessSession
. This identifier is
* required only if remoteDebugEnabled
is set to true
.
*
*
* Remote debugging is no longer
* supported.
*
*
* @param clientId
* Unique identifier for the client. If you want access to multiple devices on the same client, you should
* pass the same clientId
value in each call to CreateRemoteAccessSession
. This
* identifier is required only if remoteDebugEnabled
is set to true
.
*
* Remote debugging is no
* longer supported.
*/
public void setClientId(String clientId) {
this.clientId = clientId;
}
/**
*
* Unique identifier for the client. If you want access to multiple devices on the same client, you should pass the
* same clientId
value in each call to CreateRemoteAccessSession
. This identifier is
* required only if remoteDebugEnabled
is set to true
.
*
*
* Remote debugging is no longer
* supported.
*
*
* @return Unique identifier for the client. If you want access to multiple devices on the same client, you should
* pass the same clientId
value in each call to CreateRemoteAccessSession
. This
* identifier is required only if remoteDebugEnabled
is set to true
.
*
* Remote debugging is no longer supported.
*/
public String getClientId() {
return this.clientId;
}
/**
*
* Unique identifier for the client. If you want access to multiple devices on the same client, you should pass the
* same clientId
value in each call to CreateRemoteAccessSession
. This identifier is
* required only if remoteDebugEnabled
is set to true
.
*
*
* Remote debugging is no longer
* supported.
*
*
* @param clientId
* Unique identifier for the client. If you want access to multiple devices on the same client, you should
* pass the same clientId
value in each call to CreateRemoteAccessSession
. This
* identifier is required only if remoteDebugEnabled
is set to true
.
*
* Remote debugging is no
* longer supported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRemoteAccessSessionRequest withClientId(String clientId) {
setClientId(clientId);
return this;
}
/**
*
* The configuration information for the remote access session request.
*
*
* @param configuration
* The configuration information for the remote access session request.
*/
public void setConfiguration(CreateRemoteAccessSessionConfiguration configuration) {
this.configuration = configuration;
}
/**
*
* The configuration information for the remote access session request.
*
*
* @return The configuration information for the remote access session request.
*/
public CreateRemoteAccessSessionConfiguration getConfiguration() {
return this.configuration;
}
/**
*
* The configuration information for the remote access session request.
*
*
* @param configuration
* The configuration information for the remote access session request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRemoteAccessSessionRequest withConfiguration(CreateRemoteAccessSessionConfiguration configuration) {
setConfiguration(configuration);
return this;
}
/**
*
* The interaction mode of the remote access session. Valid values are:
*
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You cannot run
* XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode has the
* fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest framework-based tests
* and watch the screen in this mode.
*
*
*
*
* @param interactionMode
* The interaction mode of the remote access session. Valid values are:
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You
* cannot run XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode has
* the fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest framework-based
* tests and watch the screen in this mode.
*
*
* @see InteractionMode
*/
public void setInteractionMode(String interactionMode) {
this.interactionMode = interactionMode;
}
/**
*
* The interaction mode of the remote access session. Valid values are:
*
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You cannot run
* XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode has the
* fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest framework-based tests
* and watch the screen in this mode.
*
*
*
*
* @return The interaction mode of the remote access session. Valid values are:
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You
* cannot run XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode has
* the fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest framework-based
* tests and watch the screen in this mode.
*
*
* @see InteractionMode
*/
public String getInteractionMode() {
return this.interactionMode;
}
/**
*
* The interaction mode of the remote access session. Valid values are:
*
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You cannot run
* XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode has the
* fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest framework-based tests
* and watch the screen in this mode.
*
*
*
*
* @param interactionMode
* The interaction mode of the remote access session. Valid values are:
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You
* cannot run XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode has
* the fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest framework-based
* tests and watch the screen in this mode.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see InteractionMode
*/
public CreateRemoteAccessSessionRequest withInteractionMode(String interactionMode) {
setInteractionMode(interactionMode);
return this;
}
/**
*
* The interaction mode of the remote access session. Valid values are:
*
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You cannot run
* XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode has the
* fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest framework-based tests
* and watch the screen in this mode.
*
*
*
*
* @param interactionMode
* The interaction mode of the remote access session. Valid values are:
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You
* cannot run XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode has
* the fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest framework-based
* tests and watch the screen in this mode.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see InteractionMode
*/
public CreateRemoteAccessSessionRequest withInteractionMode(InteractionMode interactionMode) {
this.interactionMode = interactionMode.toString();
return this;
}
/**
*
* When set to true
, for private devices, Device Farm does not sign your app again. For public devices,
* Device Farm always signs your apps again.
*
*
* For more information on how Device Farm modifies your uploads during tests, see Do you modify my app?
*
*
* @param skipAppResign
* When set to true
, for private devices, Device Farm does not sign your app again. For public
* devices, Device Farm always signs your apps again.
*
* For more information on how Device Farm modifies your uploads during tests, see Do you modify my app?
*/
public void setSkipAppResign(Boolean skipAppResign) {
this.skipAppResign = skipAppResign;
}
/**
*
* When set to true
, for private devices, Device Farm does not sign your app again. For public devices,
* Device Farm always signs your apps again.
*
*
* For more information on how Device Farm modifies your uploads during tests, see Do you modify my app?
*
*
* @return When set to true
, for private devices, Device Farm does not sign your app again. For public
* devices, Device Farm always signs your apps again.
*
* For more information on how Device Farm modifies your uploads during tests, see Do you modify my app?
*/
public Boolean getSkipAppResign() {
return this.skipAppResign;
}
/**
*
* When set to true
, for private devices, Device Farm does not sign your app again. For public devices,
* Device Farm always signs your apps again.
*
*
* For more information on how Device Farm modifies your uploads during tests, see Do you modify my app?
*
*
* @param skipAppResign
* When set to true
, for private devices, Device Farm does not sign your app again. For public
* devices, Device Farm always signs your apps again.
*
* For more information on how Device Farm modifies your uploads during tests, see Do you modify my app?
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRemoteAccessSessionRequest withSkipAppResign(Boolean skipAppResign) {
setSkipAppResign(skipAppResign);
return this;
}
/**
*
* When set to true
, for private devices, Device Farm does not sign your app again. For public devices,
* Device Farm always signs your apps again.
*
*
* For more information on how Device Farm modifies your uploads during tests, see Do you modify my app?
*
*
* @return When set to true
, for private devices, Device Farm does not sign your app again. For public
* devices, Device Farm always signs your apps again.
*
* For more information on how Device Farm modifies your uploads during tests, see Do you modify my app?
*/
public Boolean isSkipAppResign() {
return this.skipAppResign;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getProjectArn() != null)
sb.append("ProjectArn: ").append(getProjectArn()).append(",");
if (getDeviceArn() != null)
sb.append("DeviceArn: ").append(getDeviceArn()).append(",");
if (getInstanceArn() != null)
sb.append("InstanceArn: ").append(getInstanceArn()).append(",");
if (getSshPublicKey() != null)
sb.append("SshPublicKey: ").append(getSshPublicKey()).append(",");
if (getRemoteDebugEnabled() != null)
sb.append("RemoteDebugEnabled: ").append(getRemoteDebugEnabled()).append(",");
if (getRemoteRecordEnabled() != null)
sb.append("RemoteRecordEnabled: ").append(getRemoteRecordEnabled()).append(",");
if (getRemoteRecordAppArn() != null)
sb.append("RemoteRecordAppArn: ").append(getRemoteRecordAppArn()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getClientId() != null)
sb.append("ClientId: ").append(getClientId()).append(",");
if (getConfiguration() != null)
sb.append("Configuration: ").append(getConfiguration()).append(",");
if (getInteractionMode() != null)
sb.append("InteractionMode: ").append(getInteractionMode()).append(",");
if (getSkipAppResign() != null)
sb.append("SkipAppResign: ").append(getSkipAppResign());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateRemoteAccessSessionRequest == false)
return false;
CreateRemoteAccessSessionRequest other = (CreateRemoteAccessSessionRequest) obj;
if (other.getProjectArn() == null ^ this.getProjectArn() == null)
return false;
if (other.getProjectArn() != null && other.getProjectArn().equals(this.getProjectArn()) == false)
return false;
if (other.getDeviceArn() == null ^ this.getDeviceArn() == null)
return false;
if (other.getDeviceArn() != null && other.getDeviceArn().equals(this.getDeviceArn()) == false)
return false;
if (other.getInstanceArn() == null ^ this.getInstanceArn() == null)
return false;
if (other.getInstanceArn() != null && other.getInstanceArn().equals(this.getInstanceArn()) == false)
return false;
if (other.getSshPublicKey() == null ^ this.getSshPublicKey() == null)
return false;
if (other.getSshPublicKey() != null && other.getSshPublicKey().equals(this.getSshPublicKey()) == false)
return false;
if (other.getRemoteDebugEnabled() == null ^ this.getRemoteDebugEnabled() == null)
return false;
if (other.getRemoteDebugEnabled() != null && other.getRemoteDebugEnabled().equals(this.getRemoteDebugEnabled()) == false)
return false;
if (other.getRemoteRecordEnabled() == null ^ this.getRemoteRecordEnabled() == null)
return false;
if (other.getRemoteRecordEnabled() != null && other.getRemoteRecordEnabled().equals(this.getRemoteRecordEnabled()) == false)
return false;
if (other.getRemoteRecordAppArn() == null ^ this.getRemoteRecordAppArn() == null)
return false;
if (other.getRemoteRecordAppArn() != null && other.getRemoteRecordAppArn().equals(this.getRemoteRecordAppArn()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getClientId() == null ^ this.getClientId() == null)
return false;
if (other.getClientId() != null && other.getClientId().equals(this.getClientId()) == false)
return false;
if (other.getConfiguration() == null ^ this.getConfiguration() == null)
return false;
if (other.getConfiguration() != null && other.getConfiguration().equals(this.getConfiguration()) == false)
return false;
if (other.getInteractionMode() == null ^ this.getInteractionMode() == null)
return false;
if (other.getInteractionMode() != null && other.getInteractionMode().equals(this.getInteractionMode()) == false)
return false;
if (other.getSkipAppResign() == null ^ this.getSkipAppResign() == null)
return false;
if (other.getSkipAppResign() != null && other.getSkipAppResign().equals(this.getSkipAppResign()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getProjectArn() == null) ? 0 : getProjectArn().hashCode());
hashCode = prime * hashCode + ((getDeviceArn() == null) ? 0 : getDeviceArn().hashCode());
hashCode = prime * hashCode + ((getInstanceArn() == null) ? 0 : getInstanceArn().hashCode());
hashCode = prime * hashCode + ((getSshPublicKey() == null) ? 0 : getSshPublicKey().hashCode());
hashCode = prime * hashCode + ((getRemoteDebugEnabled() == null) ? 0 : getRemoteDebugEnabled().hashCode());
hashCode = prime * hashCode + ((getRemoteRecordEnabled() == null) ? 0 : getRemoteRecordEnabled().hashCode());
hashCode = prime * hashCode + ((getRemoteRecordAppArn() == null) ? 0 : getRemoteRecordAppArn().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getClientId() == null) ? 0 : getClientId().hashCode());
hashCode = prime * hashCode + ((getConfiguration() == null) ? 0 : getConfiguration().hashCode());
hashCode = prime * hashCode + ((getInteractionMode() == null) ? 0 : getInteractionMode().hashCode());
hashCode = prime * hashCode + ((getSkipAppResign() == null) ? 0 : getSkipAppResign().hashCode());
return hashCode;
}
@Override
public CreateRemoteAccessSessionRequest clone() {
return (CreateRemoteAccessSessionRequest) super.clone();
}
}