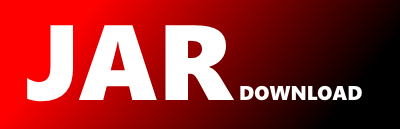
com.amazonaws.services.devicefarm.model.CustomerArtifactPaths Maven / Gradle / Ivy
Show all versions of aws-java-sdk-devicefarm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.devicefarm.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* A JSON object that specifies the paths where the artifacts generated by the customer's tests, on the device or in the
* test environment, are pulled from.
*
*
* Specify deviceHostPaths
and optionally specify either iosPaths
or androidPaths
* .
*
*
* For web app tests, you can specify both iosPaths
and androidPaths
.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CustomerArtifactPaths implements Serializable, Cloneable, StructuredPojo {
/**
*
* Comma-separated list of paths on the iOS device where the artifacts generated by the customer's tests are pulled
* from.
*
*/
private java.util.List iosPaths;
/**
*
* Comma-separated list of paths on the Android device where the artifacts generated by the customer's tests are
* pulled from.
*
*/
private java.util.List androidPaths;
/**
*
* Comma-separated list of paths in the test execution environment where the artifacts generated by the customer's
* tests are pulled from.
*
*/
private java.util.List deviceHostPaths;
/**
*
* Comma-separated list of paths on the iOS device where the artifacts generated by the customer's tests are pulled
* from.
*
*
* @return Comma-separated list of paths on the iOS device where the artifacts generated by the customer's tests are
* pulled from.
*/
public java.util.List getIosPaths() {
return iosPaths;
}
/**
*
* Comma-separated list of paths on the iOS device where the artifacts generated by the customer's tests are pulled
* from.
*
*
* @param iosPaths
* Comma-separated list of paths on the iOS device where the artifacts generated by the customer's tests are
* pulled from.
*/
public void setIosPaths(java.util.Collection iosPaths) {
if (iosPaths == null) {
this.iosPaths = null;
return;
}
this.iosPaths = new java.util.ArrayList(iosPaths);
}
/**
*
* Comma-separated list of paths on the iOS device where the artifacts generated by the customer's tests are pulled
* from.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setIosPaths(java.util.Collection)} or {@link #withIosPaths(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param iosPaths
* Comma-separated list of paths on the iOS device where the artifacts generated by the customer's tests are
* pulled from.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CustomerArtifactPaths withIosPaths(String... iosPaths) {
if (this.iosPaths == null) {
setIosPaths(new java.util.ArrayList(iosPaths.length));
}
for (String ele : iosPaths) {
this.iosPaths.add(ele);
}
return this;
}
/**
*
* Comma-separated list of paths on the iOS device where the artifacts generated by the customer's tests are pulled
* from.
*
*
* @param iosPaths
* Comma-separated list of paths on the iOS device where the artifacts generated by the customer's tests are
* pulled from.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CustomerArtifactPaths withIosPaths(java.util.Collection iosPaths) {
setIosPaths(iosPaths);
return this;
}
/**
*
* Comma-separated list of paths on the Android device where the artifacts generated by the customer's tests are
* pulled from.
*
*
* @return Comma-separated list of paths on the Android device where the artifacts generated by the customer's tests
* are pulled from.
*/
public java.util.List getAndroidPaths() {
return androidPaths;
}
/**
*
* Comma-separated list of paths on the Android device where the artifacts generated by the customer's tests are
* pulled from.
*
*
* @param androidPaths
* Comma-separated list of paths on the Android device where the artifacts generated by the customer's tests
* are pulled from.
*/
public void setAndroidPaths(java.util.Collection androidPaths) {
if (androidPaths == null) {
this.androidPaths = null;
return;
}
this.androidPaths = new java.util.ArrayList(androidPaths);
}
/**
*
* Comma-separated list of paths on the Android device where the artifacts generated by the customer's tests are
* pulled from.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAndroidPaths(java.util.Collection)} or {@link #withAndroidPaths(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param androidPaths
* Comma-separated list of paths on the Android device where the artifacts generated by the customer's tests
* are pulled from.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CustomerArtifactPaths withAndroidPaths(String... androidPaths) {
if (this.androidPaths == null) {
setAndroidPaths(new java.util.ArrayList(androidPaths.length));
}
for (String ele : androidPaths) {
this.androidPaths.add(ele);
}
return this;
}
/**
*
* Comma-separated list of paths on the Android device where the artifacts generated by the customer's tests are
* pulled from.
*
*
* @param androidPaths
* Comma-separated list of paths on the Android device where the artifacts generated by the customer's tests
* are pulled from.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CustomerArtifactPaths withAndroidPaths(java.util.Collection androidPaths) {
setAndroidPaths(androidPaths);
return this;
}
/**
*
* Comma-separated list of paths in the test execution environment where the artifacts generated by the customer's
* tests are pulled from.
*
*
* @return Comma-separated list of paths in the test execution environment where the artifacts generated by the
* customer's tests are pulled from.
*/
public java.util.List getDeviceHostPaths() {
return deviceHostPaths;
}
/**
*
* Comma-separated list of paths in the test execution environment where the artifacts generated by the customer's
* tests are pulled from.
*
*
* @param deviceHostPaths
* Comma-separated list of paths in the test execution environment where the artifacts generated by the
* customer's tests are pulled from.
*/
public void setDeviceHostPaths(java.util.Collection deviceHostPaths) {
if (deviceHostPaths == null) {
this.deviceHostPaths = null;
return;
}
this.deviceHostPaths = new java.util.ArrayList(deviceHostPaths);
}
/**
*
* Comma-separated list of paths in the test execution environment where the artifacts generated by the customer's
* tests are pulled from.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDeviceHostPaths(java.util.Collection)} or {@link #withDeviceHostPaths(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param deviceHostPaths
* Comma-separated list of paths in the test execution environment where the artifacts generated by the
* customer's tests are pulled from.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CustomerArtifactPaths withDeviceHostPaths(String... deviceHostPaths) {
if (this.deviceHostPaths == null) {
setDeviceHostPaths(new java.util.ArrayList(deviceHostPaths.length));
}
for (String ele : deviceHostPaths) {
this.deviceHostPaths.add(ele);
}
return this;
}
/**
*
* Comma-separated list of paths in the test execution environment where the artifacts generated by the customer's
* tests are pulled from.
*
*
* @param deviceHostPaths
* Comma-separated list of paths in the test execution environment where the artifacts generated by the
* customer's tests are pulled from.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CustomerArtifactPaths withDeviceHostPaths(java.util.Collection deviceHostPaths) {
setDeviceHostPaths(deviceHostPaths);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getIosPaths() != null)
sb.append("IosPaths: ").append(getIosPaths()).append(",");
if (getAndroidPaths() != null)
sb.append("AndroidPaths: ").append(getAndroidPaths()).append(",");
if (getDeviceHostPaths() != null)
sb.append("DeviceHostPaths: ").append(getDeviceHostPaths());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CustomerArtifactPaths == false)
return false;
CustomerArtifactPaths other = (CustomerArtifactPaths) obj;
if (other.getIosPaths() == null ^ this.getIosPaths() == null)
return false;
if (other.getIosPaths() != null && other.getIosPaths().equals(this.getIosPaths()) == false)
return false;
if (other.getAndroidPaths() == null ^ this.getAndroidPaths() == null)
return false;
if (other.getAndroidPaths() != null && other.getAndroidPaths().equals(this.getAndroidPaths()) == false)
return false;
if (other.getDeviceHostPaths() == null ^ this.getDeviceHostPaths() == null)
return false;
if (other.getDeviceHostPaths() != null && other.getDeviceHostPaths().equals(this.getDeviceHostPaths()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getIosPaths() == null) ? 0 : getIosPaths().hashCode());
hashCode = prime * hashCode + ((getAndroidPaths() == null) ? 0 : getAndroidPaths().hashCode());
hashCode = prime * hashCode + ((getDeviceHostPaths() == null) ? 0 : getDeviceHostPaths().hashCode());
return hashCode;
}
@Override
public CustomerArtifactPaths clone() {
try {
return (CustomerArtifactPaths) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.devicefarm.model.transform.CustomerArtifactPathsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}