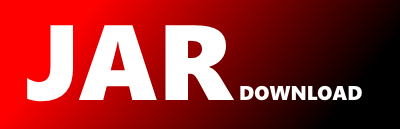
com.amazonaws.services.devicefarm.model.GetDevicePoolCompatibilityRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-devicefarm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.devicefarm.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* Represents a request to the get device pool compatibility operation.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetDevicePoolCompatibilityRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The device pool's ARN.
*
*/
private String devicePoolArn;
/**
*
* The ARN of the app that is associated with the specified device pool.
*
*/
private String appArn;
/**
*
* The test type for the specified device pool.
*
*
* Allowed values include the following:
*
*
* -
*
* BUILTIN_FUZZ.
*
*
* -
*
* BUILTIN_EXPLORER. For Android, an app explorer that traverses an Android app, interacting with it and capturing
* screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT.
*
*
* -
*
* APPIUM_JAVA_TESTNG.
*
*
* -
*
* APPIUM_PYTHON.
*
*
* -
*
* APPIUM_NODE.
*
*
* -
*
* APPIUM_RUBY.
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT.
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG.
*
*
* -
*
* APPIUM_WEB_PYTHON.
*
*
* -
*
* APPIUM_WEB_NODE.
*
*
* -
*
* APPIUM_WEB_RUBY.
*
*
* -
*
* CALABASH.
*
*
* -
*
* INSTRUMENTATION.
*
*
* -
*
* UIAUTOMATION.
*
*
* -
*
* UIAUTOMATOR.
*
*
* -
*
* XCTEST.
*
*
* -
*
* XCTEST_UI.
*
*
*
*/
private String testType;
/**
*
* Information about the uploaded test to be run against the device pool.
*
*/
private ScheduleRunTest test;
/**
*
* An object that contains information about the settings for a run.
*
*/
private ScheduleRunConfiguration configuration;
/**
*
* The device pool's ARN.
*
*
* @param devicePoolArn
* The device pool's ARN.
*/
public void setDevicePoolArn(String devicePoolArn) {
this.devicePoolArn = devicePoolArn;
}
/**
*
* The device pool's ARN.
*
*
* @return The device pool's ARN.
*/
public String getDevicePoolArn() {
return this.devicePoolArn;
}
/**
*
* The device pool's ARN.
*
*
* @param devicePoolArn
* The device pool's ARN.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDevicePoolCompatibilityRequest withDevicePoolArn(String devicePoolArn) {
setDevicePoolArn(devicePoolArn);
return this;
}
/**
*
* The ARN of the app that is associated with the specified device pool.
*
*
* @param appArn
* The ARN of the app that is associated with the specified device pool.
*/
public void setAppArn(String appArn) {
this.appArn = appArn;
}
/**
*
* The ARN of the app that is associated with the specified device pool.
*
*
* @return The ARN of the app that is associated with the specified device pool.
*/
public String getAppArn() {
return this.appArn;
}
/**
*
* The ARN of the app that is associated with the specified device pool.
*
*
* @param appArn
* The ARN of the app that is associated with the specified device pool.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDevicePoolCompatibilityRequest withAppArn(String appArn) {
setAppArn(appArn);
return this;
}
/**
*
* The test type for the specified device pool.
*
*
* Allowed values include the following:
*
*
* -
*
* BUILTIN_FUZZ.
*
*
* -
*
* BUILTIN_EXPLORER. For Android, an app explorer that traverses an Android app, interacting with it and capturing
* screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT.
*
*
* -
*
* APPIUM_JAVA_TESTNG.
*
*
* -
*
* APPIUM_PYTHON.
*
*
* -
*
* APPIUM_NODE.
*
*
* -
*
* APPIUM_RUBY.
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT.
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG.
*
*
* -
*
* APPIUM_WEB_PYTHON.
*
*
* -
*
* APPIUM_WEB_NODE.
*
*
* -
*
* APPIUM_WEB_RUBY.
*
*
* -
*
* CALABASH.
*
*
* -
*
* INSTRUMENTATION.
*
*
* -
*
* UIAUTOMATION.
*
*
* -
*
* UIAUTOMATOR.
*
*
* -
*
* XCTEST.
*
*
* -
*
* XCTEST_UI.
*
*
*
*
* @param testType
* The test type for the specified device pool.
*
* Allowed values include the following:
*
*
* -
*
* BUILTIN_FUZZ.
*
*
* -
*
* BUILTIN_EXPLORER. For Android, an app explorer that traverses an Android app, interacting with it and
* capturing screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT.
*
*
* -
*
* APPIUM_JAVA_TESTNG.
*
*
* -
*
* APPIUM_PYTHON.
*
*
* -
*
* APPIUM_NODE.
*
*
* -
*
* APPIUM_RUBY.
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT.
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG.
*
*
* -
*
* APPIUM_WEB_PYTHON.
*
*
* -
*
* APPIUM_WEB_NODE.
*
*
* -
*
* APPIUM_WEB_RUBY.
*
*
* -
*
* CALABASH.
*
*
* -
*
* INSTRUMENTATION.
*
*
* -
*
* UIAUTOMATION.
*
*
* -
*
* UIAUTOMATOR.
*
*
* -
*
* XCTEST.
*
*
* -
*
* XCTEST_UI.
*
*
* @see TestType
*/
public void setTestType(String testType) {
this.testType = testType;
}
/**
*
* The test type for the specified device pool.
*
*
* Allowed values include the following:
*
*
* -
*
* BUILTIN_FUZZ.
*
*
* -
*
* BUILTIN_EXPLORER. For Android, an app explorer that traverses an Android app, interacting with it and capturing
* screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT.
*
*
* -
*
* APPIUM_JAVA_TESTNG.
*
*
* -
*
* APPIUM_PYTHON.
*
*
* -
*
* APPIUM_NODE.
*
*
* -
*
* APPIUM_RUBY.
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT.
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG.
*
*
* -
*
* APPIUM_WEB_PYTHON.
*
*
* -
*
* APPIUM_WEB_NODE.
*
*
* -
*
* APPIUM_WEB_RUBY.
*
*
* -
*
* CALABASH.
*
*
* -
*
* INSTRUMENTATION.
*
*
* -
*
* UIAUTOMATION.
*
*
* -
*
* UIAUTOMATOR.
*
*
* -
*
* XCTEST.
*
*
* -
*
* XCTEST_UI.
*
*
*
*
* @return The test type for the specified device pool.
*
* Allowed values include the following:
*
*
* -
*
* BUILTIN_FUZZ.
*
*
* -
*
* BUILTIN_EXPLORER. For Android, an app explorer that traverses an Android app, interacting with it and
* capturing screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT.
*
*
* -
*
* APPIUM_JAVA_TESTNG.
*
*
* -
*
* APPIUM_PYTHON.
*
*
* -
*
* APPIUM_NODE.
*
*
* -
*
* APPIUM_RUBY.
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT.
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG.
*
*
* -
*
* APPIUM_WEB_PYTHON.
*
*
* -
*
* APPIUM_WEB_NODE.
*
*
* -
*
* APPIUM_WEB_RUBY.
*
*
* -
*
* CALABASH.
*
*
* -
*
* INSTRUMENTATION.
*
*
* -
*
* UIAUTOMATION.
*
*
* -
*
* UIAUTOMATOR.
*
*
* -
*
* XCTEST.
*
*
* -
*
* XCTEST_UI.
*
*
* @see TestType
*/
public String getTestType() {
return this.testType;
}
/**
*
* The test type for the specified device pool.
*
*
* Allowed values include the following:
*
*
* -
*
* BUILTIN_FUZZ.
*
*
* -
*
* BUILTIN_EXPLORER. For Android, an app explorer that traverses an Android app, interacting with it and capturing
* screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT.
*
*
* -
*
* APPIUM_JAVA_TESTNG.
*
*
* -
*
* APPIUM_PYTHON.
*
*
* -
*
* APPIUM_NODE.
*
*
* -
*
* APPIUM_RUBY.
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT.
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG.
*
*
* -
*
* APPIUM_WEB_PYTHON.
*
*
* -
*
* APPIUM_WEB_NODE.
*
*
* -
*
* APPIUM_WEB_RUBY.
*
*
* -
*
* CALABASH.
*
*
* -
*
* INSTRUMENTATION.
*
*
* -
*
* UIAUTOMATION.
*
*
* -
*
* UIAUTOMATOR.
*
*
* -
*
* XCTEST.
*
*
* -
*
* XCTEST_UI.
*
*
*
*
* @param testType
* The test type for the specified device pool.
*
* Allowed values include the following:
*
*
* -
*
* BUILTIN_FUZZ.
*
*
* -
*
* BUILTIN_EXPLORER. For Android, an app explorer that traverses an Android app, interacting with it and
* capturing screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT.
*
*
* -
*
* APPIUM_JAVA_TESTNG.
*
*
* -
*
* APPIUM_PYTHON.
*
*
* -
*
* APPIUM_NODE.
*
*
* -
*
* APPIUM_RUBY.
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT.
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG.
*
*
* -
*
* APPIUM_WEB_PYTHON.
*
*
* -
*
* APPIUM_WEB_NODE.
*
*
* -
*
* APPIUM_WEB_RUBY.
*
*
* -
*
* CALABASH.
*
*
* -
*
* INSTRUMENTATION.
*
*
* -
*
* UIAUTOMATION.
*
*
* -
*
* UIAUTOMATOR.
*
*
* -
*
* XCTEST.
*
*
* -
*
* XCTEST_UI.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see TestType
*/
public GetDevicePoolCompatibilityRequest withTestType(String testType) {
setTestType(testType);
return this;
}
/**
*
* The test type for the specified device pool.
*
*
* Allowed values include the following:
*
*
* -
*
* BUILTIN_FUZZ.
*
*
* -
*
* BUILTIN_EXPLORER. For Android, an app explorer that traverses an Android app, interacting with it and capturing
* screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT.
*
*
* -
*
* APPIUM_JAVA_TESTNG.
*
*
* -
*
* APPIUM_PYTHON.
*
*
* -
*
* APPIUM_NODE.
*
*
* -
*
* APPIUM_RUBY.
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT.
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG.
*
*
* -
*
* APPIUM_WEB_PYTHON.
*
*
* -
*
* APPIUM_WEB_NODE.
*
*
* -
*
* APPIUM_WEB_RUBY.
*
*
* -
*
* CALABASH.
*
*
* -
*
* INSTRUMENTATION.
*
*
* -
*
* UIAUTOMATION.
*
*
* -
*
* UIAUTOMATOR.
*
*
* -
*
* XCTEST.
*
*
* -
*
* XCTEST_UI.
*
*
*
*
* @param testType
* The test type for the specified device pool.
*
* Allowed values include the following:
*
*
* -
*
* BUILTIN_FUZZ.
*
*
* -
*
* BUILTIN_EXPLORER. For Android, an app explorer that traverses an Android app, interacting with it and
* capturing screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT.
*
*
* -
*
* APPIUM_JAVA_TESTNG.
*
*
* -
*
* APPIUM_PYTHON.
*
*
* -
*
* APPIUM_NODE.
*
*
* -
*
* APPIUM_RUBY.
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT.
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG.
*
*
* -
*
* APPIUM_WEB_PYTHON.
*
*
* -
*
* APPIUM_WEB_NODE.
*
*
* -
*
* APPIUM_WEB_RUBY.
*
*
* -
*
* CALABASH.
*
*
* -
*
* INSTRUMENTATION.
*
*
* -
*
* UIAUTOMATION.
*
*
* -
*
* UIAUTOMATOR.
*
*
* -
*
* XCTEST.
*
*
* -
*
* XCTEST_UI.
*
*
* @see TestType
*/
public void setTestType(TestType testType) {
withTestType(testType);
}
/**
*
* The test type for the specified device pool.
*
*
* Allowed values include the following:
*
*
* -
*
* BUILTIN_FUZZ.
*
*
* -
*
* BUILTIN_EXPLORER. For Android, an app explorer that traverses an Android app, interacting with it and capturing
* screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT.
*
*
* -
*
* APPIUM_JAVA_TESTNG.
*
*
* -
*
* APPIUM_PYTHON.
*
*
* -
*
* APPIUM_NODE.
*
*
* -
*
* APPIUM_RUBY.
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT.
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG.
*
*
* -
*
* APPIUM_WEB_PYTHON.
*
*
* -
*
* APPIUM_WEB_NODE.
*
*
* -
*
* APPIUM_WEB_RUBY.
*
*
* -
*
* CALABASH.
*
*
* -
*
* INSTRUMENTATION.
*
*
* -
*
* UIAUTOMATION.
*
*
* -
*
* UIAUTOMATOR.
*
*
* -
*
* XCTEST.
*
*
* -
*
* XCTEST_UI.
*
*
*
*
* @param testType
* The test type for the specified device pool.
*
* Allowed values include the following:
*
*
* -
*
* BUILTIN_FUZZ.
*
*
* -
*
* BUILTIN_EXPLORER. For Android, an app explorer that traverses an Android app, interacting with it and
* capturing screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT.
*
*
* -
*
* APPIUM_JAVA_TESTNG.
*
*
* -
*
* APPIUM_PYTHON.
*
*
* -
*
* APPIUM_NODE.
*
*
* -
*
* APPIUM_RUBY.
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT.
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG.
*
*
* -
*
* APPIUM_WEB_PYTHON.
*
*
* -
*
* APPIUM_WEB_NODE.
*
*
* -
*
* APPIUM_WEB_RUBY.
*
*
* -
*
* CALABASH.
*
*
* -
*
* INSTRUMENTATION.
*
*
* -
*
* UIAUTOMATION.
*
*
* -
*
* UIAUTOMATOR.
*
*
* -
*
* XCTEST.
*
*
* -
*
* XCTEST_UI.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see TestType
*/
public GetDevicePoolCompatibilityRequest withTestType(TestType testType) {
this.testType = testType.toString();
return this;
}
/**
*
* Information about the uploaded test to be run against the device pool.
*
*
* @param test
* Information about the uploaded test to be run against the device pool.
*/
public void setTest(ScheduleRunTest test) {
this.test = test;
}
/**
*
* Information about the uploaded test to be run against the device pool.
*
*
* @return Information about the uploaded test to be run against the device pool.
*/
public ScheduleRunTest getTest() {
return this.test;
}
/**
*
* Information about the uploaded test to be run against the device pool.
*
*
* @param test
* Information about the uploaded test to be run against the device pool.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDevicePoolCompatibilityRequest withTest(ScheduleRunTest test) {
setTest(test);
return this;
}
/**
*
* An object that contains information about the settings for a run.
*
*
* @param configuration
* An object that contains information about the settings for a run.
*/
public void setConfiguration(ScheduleRunConfiguration configuration) {
this.configuration = configuration;
}
/**
*
* An object that contains information about the settings for a run.
*
*
* @return An object that contains information about the settings for a run.
*/
public ScheduleRunConfiguration getConfiguration() {
return this.configuration;
}
/**
*
* An object that contains information about the settings for a run.
*
*
* @param configuration
* An object that contains information about the settings for a run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDevicePoolCompatibilityRequest withConfiguration(ScheduleRunConfiguration configuration) {
setConfiguration(configuration);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDevicePoolArn() != null)
sb.append("DevicePoolArn: ").append(getDevicePoolArn()).append(",");
if (getAppArn() != null)
sb.append("AppArn: ").append(getAppArn()).append(",");
if (getTestType() != null)
sb.append("TestType: ").append(getTestType()).append(",");
if (getTest() != null)
sb.append("Test: ").append(getTest()).append(",");
if (getConfiguration() != null)
sb.append("Configuration: ").append(getConfiguration());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetDevicePoolCompatibilityRequest == false)
return false;
GetDevicePoolCompatibilityRequest other = (GetDevicePoolCompatibilityRequest) obj;
if (other.getDevicePoolArn() == null ^ this.getDevicePoolArn() == null)
return false;
if (other.getDevicePoolArn() != null && other.getDevicePoolArn().equals(this.getDevicePoolArn()) == false)
return false;
if (other.getAppArn() == null ^ this.getAppArn() == null)
return false;
if (other.getAppArn() != null && other.getAppArn().equals(this.getAppArn()) == false)
return false;
if (other.getTestType() == null ^ this.getTestType() == null)
return false;
if (other.getTestType() != null && other.getTestType().equals(this.getTestType()) == false)
return false;
if (other.getTest() == null ^ this.getTest() == null)
return false;
if (other.getTest() != null && other.getTest().equals(this.getTest()) == false)
return false;
if (other.getConfiguration() == null ^ this.getConfiguration() == null)
return false;
if (other.getConfiguration() != null && other.getConfiguration().equals(this.getConfiguration()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDevicePoolArn() == null) ? 0 : getDevicePoolArn().hashCode());
hashCode = prime * hashCode + ((getAppArn() == null) ? 0 : getAppArn().hashCode());
hashCode = prime * hashCode + ((getTestType() == null) ? 0 : getTestType().hashCode());
hashCode = prime * hashCode + ((getTest() == null) ? 0 : getTest().hashCode());
hashCode = prime * hashCode + ((getConfiguration() == null) ? 0 : getConfiguration().hashCode());
return hashCode;
}
@Override
public GetDevicePoolCompatibilityRequest clone() {
return (GetDevicePoolCompatibilityRequest) super.clone();
}
}