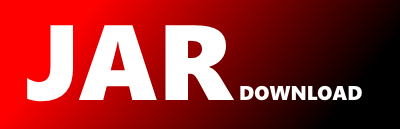
com.amazonaws.services.devicefarm.model.ScheduleRunConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-devicefarm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.devicefarm.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Represents the settings for a run. Includes things like location, radio states, auxiliary apps, and network profiles.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ScheduleRunConfiguration implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ARN of the extra data for the run. The extra data is a .zip file that AWS Device Farm extracts to external
* data for Android or the app's sandbox for iOS.
*
*/
private String extraDataPackageArn;
/**
*
* Reserved for internal use.
*
*/
private String networkProfileArn;
/**
*
* Information about the locale that is used for the run.
*
*/
private String locale;
/**
*
* Information about the location that is used for the run.
*
*/
private Location location;
/**
*
* An array of ARNs for your VPC endpoint configurations.
*
*/
private java.util.List vpceConfigurationArns;
/**
*
* Input CustomerArtifactPaths
object for the scheduled run configuration.
*
*/
private CustomerArtifactPaths customerArtifactPaths;
/**
*
* Information about the radio states for the run.
*
*/
private Radios radios;
/**
*
* A list of upload ARNs for app packages to be installed with your app.
*
*/
private java.util.List auxiliaryApps;
/**
*
* Specifies the billing method for a test run: metered
or unmetered
. If the parameter is
* not specified, the default value is metered
.
*
*
*
* If you have purchased unmetered device slots, you must set this parameter to unmetered
to make use
* of them. Otherwise, your run counts against your metered time.
*
*
*/
private String billingMethod;
/**
*
* The ARN of the extra data for the run. The extra data is a .zip file that AWS Device Farm extracts to external
* data for Android or the app's sandbox for iOS.
*
*
* @param extraDataPackageArn
* The ARN of the extra data for the run. The extra data is a .zip file that AWS Device Farm extracts to
* external data for Android or the app's sandbox for iOS.
*/
public void setExtraDataPackageArn(String extraDataPackageArn) {
this.extraDataPackageArn = extraDataPackageArn;
}
/**
*
* The ARN of the extra data for the run. The extra data is a .zip file that AWS Device Farm extracts to external
* data for Android or the app's sandbox for iOS.
*
*
* @return The ARN of the extra data for the run. The extra data is a .zip file that AWS Device Farm extracts to
* external data for Android or the app's sandbox for iOS.
*/
public String getExtraDataPackageArn() {
return this.extraDataPackageArn;
}
/**
*
* The ARN of the extra data for the run. The extra data is a .zip file that AWS Device Farm extracts to external
* data for Android or the app's sandbox for iOS.
*
*
* @param extraDataPackageArn
* The ARN of the extra data for the run. The extra data is a .zip file that AWS Device Farm extracts to
* external data for Android or the app's sandbox for iOS.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScheduleRunConfiguration withExtraDataPackageArn(String extraDataPackageArn) {
setExtraDataPackageArn(extraDataPackageArn);
return this;
}
/**
*
* Reserved for internal use.
*
*
* @param networkProfileArn
* Reserved for internal use.
*/
public void setNetworkProfileArn(String networkProfileArn) {
this.networkProfileArn = networkProfileArn;
}
/**
*
* Reserved for internal use.
*
*
* @return Reserved for internal use.
*/
public String getNetworkProfileArn() {
return this.networkProfileArn;
}
/**
*
* Reserved for internal use.
*
*
* @param networkProfileArn
* Reserved for internal use.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScheduleRunConfiguration withNetworkProfileArn(String networkProfileArn) {
setNetworkProfileArn(networkProfileArn);
return this;
}
/**
*
* Information about the locale that is used for the run.
*
*
* @param locale
* Information about the locale that is used for the run.
*/
public void setLocale(String locale) {
this.locale = locale;
}
/**
*
* Information about the locale that is used for the run.
*
*
* @return Information about the locale that is used for the run.
*/
public String getLocale() {
return this.locale;
}
/**
*
* Information about the locale that is used for the run.
*
*
* @param locale
* Information about the locale that is used for the run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScheduleRunConfiguration withLocale(String locale) {
setLocale(locale);
return this;
}
/**
*
* Information about the location that is used for the run.
*
*
* @param location
* Information about the location that is used for the run.
*/
public void setLocation(Location location) {
this.location = location;
}
/**
*
* Information about the location that is used for the run.
*
*
* @return Information about the location that is used for the run.
*/
public Location getLocation() {
return this.location;
}
/**
*
* Information about the location that is used for the run.
*
*
* @param location
* Information about the location that is used for the run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScheduleRunConfiguration withLocation(Location location) {
setLocation(location);
return this;
}
/**
*
* An array of ARNs for your VPC endpoint configurations.
*
*
* @return An array of ARNs for your VPC endpoint configurations.
*/
public java.util.List getVpceConfigurationArns() {
return vpceConfigurationArns;
}
/**
*
* An array of ARNs for your VPC endpoint configurations.
*
*
* @param vpceConfigurationArns
* An array of ARNs for your VPC endpoint configurations.
*/
public void setVpceConfigurationArns(java.util.Collection vpceConfigurationArns) {
if (vpceConfigurationArns == null) {
this.vpceConfigurationArns = null;
return;
}
this.vpceConfigurationArns = new java.util.ArrayList(vpceConfigurationArns);
}
/**
*
* An array of ARNs for your VPC endpoint configurations.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setVpceConfigurationArns(java.util.Collection)} or
* {@link #withVpceConfigurationArns(java.util.Collection)} if you want to override the existing values.
*
*
* @param vpceConfigurationArns
* An array of ARNs for your VPC endpoint configurations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScheduleRunConfiguration withVpceConfigurationArns(String... vpceConfigurationArns) {
if (this.vpceConfigurationArns == null) {
setVpceConfigurationArns(new java.util.ArrayList(vpceConfigurationArns.length));
}
for (String ele : vpceConfigurationArns) {
this.vpceConfigurationArns.add(ele);
}
return this;
}
/**
*
* An array of ARNs for your VPC endpoint configurations.
*
*
* @param vpceConfigurationArns
* An array of ARNs for your VPC endpoint configurations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScheduleRunConfiguration withVpceConfigurationArns(java.util.Collection vpceConfigurationArns) {
setVpceConfigurationArns(vpceConfigurationArns);
return this;
}
/**
*
* Input CustomerArtifactPaths
object for the scheduled run configuration.
*
*
* @param customerArtifactPaths
* Input CustomerArtifactPaths
object for the scheduled run configuration.
*/
public void setCustomerArtifactPaths(CustomerArtifactPaths customerArtifactPaths) {
this.customerArtifactPaths = customerArtifactPaths;
}
/**
*
* Input CustomerArtifactPaths
object for the scheduled run configuration.
*
*
* @return Input CustomerArtifactPaths
object for the scheduled run configuration.
*/
public CustomerArtifactPaths getCustomerArtifactPaths() {
return this.customerArtifactPaths;
}
/**
*
* Input CustomerArtifactPaths
object for the scheduled run configuration.
*
*
* @param customerArtifactPaths
* Input CustomerArtifactPaths
object for the scheduled run configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScheduleRunConfiguration withCustomerArtifactPaths(CustomerArtifactPaths customerArtifactPaths) {
setCustomerArtifactPaths(customerArtifactPaths);
return this;
}
/**
*
* Information about the radio states for the run.
*
*
* @param radios
* Information about the radio states for the run.
*/
public void setRadios(Radios radios) {
this.radios = radios;
}
/**
*
* Information about the radio states for the run.
*
*
* @return Information about the radio states for the run.
*/
public Radios getRadios() {
return this.radios;
}
/**
*
* Information about the radio states for the run.
*
*
* @param radios
* Information about the radio states for the run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScheduleRunConfiguration withRadios(Radios radios) {
setRadios(radios);
return this;
}
/**
*
* A list of upload ARNs for app packages to be installed with your app.
*
*
* @return A list of upload ARNs for app packages to be installed with your app.
*/
public java.util.List getAuxiliaryApps() {
return auxiliaryApps;
}
/**
*
* A list of upload ARNs for app packages to be installed with your app.
*
*
* @param auxiliaryApps
* A list of upload ARNs for app packages to be installed with your app.
*/
public void setAuxiliaryApps(java.util.Collection auxiliaryApps) {
if (auxiliaryApps == null) {
this.auxiliaryApps = null;
return;
}
this.auxiliaryApps = new java.util.ArrayList(auxiliaryApps);
}
/**
*
* A list of upload ARNs for app packages to be installed with your app.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAuxiliaryApps(java.util.Collection)} or {@link #withAuxiliaryApps(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param auxiliaryApps
* A list of upload ARNs for app packages to be installed with your app.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScheduleRunConfiguration withAuxiliaryApps(String... auxiliaryApps) {
if (this.auxiliaryApps == null) {
setAuxiliaryApps(new java.util.ArrayList(auxiliaryApps.length));
}
for (String ele : auxiliaryApps) {
this.auxiliaryApps.add(ele);
}
return this;
}
/**
*
* A list of upload ARNs for app packages to be installed with your app.
*
*
* @param auxiliaryApps
* A list of upload ARNs for app packages to be installed with your app.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScheduleRunConfiguration withAuxiliaryApps(java.util.Collection auxiliaryApps) {
setAuxiliaryApps(auxiliaryApps);
return this;
}
/**
*
* Specifies the billing method for a test run: metered
or unmetered
. If the parameter is
* not specified, the default value is metered
.
*
*
*
* If you have purchased unmetered device slots, you must set this parameter to unmetered
to make use
* of them. Otherwise, your run counts against your metered time.
*
*
*
* @param billingMethod
* Specifies the billing method for a test run: metered
or unmetered
. If the
* parameter is not specified, the default value is metered
.
*
* If you have purchased unmetered device slots, you must set this parameter to unmetered
to
* make use of them. Otherwise, your run counts against your metered time.
*
* @see BillingMethod
*/
public void setBillingMethod(String billingMethod) {
this.billingMethod = billingMethod;
}
/**
*
* Specifies the billing method for a test run: metered
or unmetered
. If the parameter is
* not specified, the default value is metered
.
*
*
*
* If you have purchased unmetered device slots, you must set this parameter to unmetered
to make use
* of them. Otherwise, your run counts against your metered time.
*
*
*
* @return Specifies the billing method for a test run: metered
or unmetered
. If the
* parameter is not specified, the default value is metered
.
*
* If you have purchased unmetered device slots, you must set this parameter to unmetered
to
* make use of them. Otherwise, your run counts against your metered time.
*
* @see BillingMethod
*/
public String getBillingMethod() {
return this.billingMethod;
}
/**
*
* Specifies the billing method for a test run: metered
or unmetered
. If the parameter is
* not specified, the default value is metered
.
*
*
*
* If you have purchased unmetered device slots, you must set this parameter to unmetered
to make use
* of them. Otherwise, your run counts against your metered time.
*
*
*
* @param billingMethod
* Specifies the billing method for a test run: metered
or unmetered
. If the
* parameter is not specified, the default value is metered
.
*
* If you have purchased unmetered device slots, you must set this parameter to unmetered
to
* make use of them. Otherwise, your run counts against your metered time.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see BillingMethod
*/
public ScheduleRunConfiguration withBillingMethod(String billingMethod) {
setBillingMethod(billingMethod);
return this;
}
/**
*
* Specifies the billing method for a test run: metered
or unmetered
. If the parameter is
* not specified, the default value is metered
.
*
*
*
* If you have purchased unmetered device slots, you must set this parameter to unmetered
to make use
* of them. Otherwise, your run counts against your metered time.
*
*
*
* @param billingMethod
* Specifies the billing method for a test run: metered
or unmetered
. If the
* parameter is not specified, the default value is metered
.
*
* If you have purchased unmetered device slots, you must set this parameter to unmetered
to
* make use of them. Otherwise, your run counts against your metered time.
*
* @see BillingMethod
*/
public void setBillingMethod(BillingMethod billingMethod) {
withBillingMethod(billingMethod);
}
/**
*
* Specifies the billing method for a test run: metered
or unmetered
. If the parameter is
* not specified, the default value is metered
.
*
*
*
* If you have purchased unmetered device slots, you must set this parameter to unmetered
to make use
* of them. Otherwise, your run counts against your metered time.
*
*
*
* @param billingMethod
* Specifies the billing method for a test run: metered
or unmetered
. If the
* parameter is not specified, the default value is metered
.
*
* If you have purchased unmetered device slots, you must set this parameter to unmetered
to
* make use of them. Otherwise, your run counts against your metered time.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see BillingMethod
*/
public ScheduleRunConfiguration withBillingMethod(BillingMethod billingMethod) {
this.billingMethod = billingMethod.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getExtraDataPackageArn() != null)
sb.append("ExtraDataPackageArn: ").append(getExtraDataPackageArn()).append(",");
if (getNetworkProfileArn() != null)
sb.append("NetworkProfileArn: ").append(getNetworkProfileArn()).append(",");
if (getLocale() != null)
sb.append("Locale: ").append(getLocale()).append(",");
if (getLocation() != null)
sb.append("Location: ").append(getLocation()).append(",");
if (getVpceConfigurationArns() != null)
sb.append("VpceConfigurationArns: ").append(getVpceConfigurationArns()).append(",");
if (getCustomerArtifactPaths() != null)
sb.append("CustomerArtifactPaths: ").append(getCustomerArtifactPaths()).append(",");
if (getRadios() != null)
sb.append("Radios: ").append(getRadios()).append(",");
if (getAuxiliaryApps() != null)
sb.append("AuxiliaryApps: ").append(getAuxiliaryApps()).append(",");
if (getBillingMethod() != null)
sb.append("BillingMethod: ").append(getBillingMethod());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ScheduleRunConfiguration == false)
return false;
ScheduleRunConfiguration other = (ScheduleRunConfiguration) obj;
if (other.getExtraDataPackageArn() == null ^ this.getExtraDataPackageArn() == null)
return false;
if (other.getExtraDataPackageArn() != null && other.getExtraDataPackageArn().equals(this.getExtraDataPackageArn()) == false)
return false;
if (other.getNetworkProfileArn() == null ^ this.getNetworkProfileArn() == null)
return false;
if (other.getNetworkProfileArn() != null && other.getNetworkProfileArn().equals(this.getNetworkProfileArn()) == false)
return false;
if (other.getLocale() == null ^ this.getLocale() == null)
return false;
if (other.getLocale() != null && other.getLocale().equals(this.getLocale()) == false)
return false;
if (other.getLocation() == null ^ this.getLocation() == null)
return false;
if (other.getLocation() != null && other.getLocation().equals(this.getLocation()) == false)
return false;
if (other.getVpceConfigurationArns() == null ^ this.getVpceConfigurationArns() == null)
return false;
if (other.getVpceConfigurationArns() != null && other.getVpceConfigurationArns().equals(this.getVpceConfigurationArns()) == false)
return false;
if (other.getCustomerArtifactPaths() == null ^ this.getCustomerArtifactPaths() == null)
return false;
if (other.getCustomerArtifactPaths() != null && other.getCustomerArtifactPaths().equals(this.getCustomerArtifactPaths()) == false)
return false;
if (other.getRadios() == null ^ this.getRadios() == null)
return false;
if (other.getRadios() != null && other.getRadios().equals(this.getRadios()) == false)
return false;
if (other.getAuxiliaryApps() == null ^ this.getAuxiliaryApps() == null)
return false;
if (other.getAuxiliaryApps() != null && other.getAuxiliaryApps().equals(this.getAuxiliaryApps()) == false)
return false;
if (other.getBillingMethod() == null ^ this.getBillingMethod() == null)
return false;
if (other.getBillingMethod() != null && other.getBillingMethod().equals(this.getBillingMethod()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getExtraDataPackageArn() == null) ? 0 : getExtraDataPackageArn().hashCode());
hashCode = prime * hashCode + ((getNetworkProfileArn() == null) ? 0 : getNetworkProfileArn().hashCode());
hashCode = prime * hashCode + ((getLocale() == null) ? 0 : getLocale().hashCode());
hashCode = prime * hashCode + ((getLocation() == null) ? 0 : getLocation().hashCode());
hashCode = prime * hashCode + ((getVpceConfigurationArns() == null) ? 0 : getVpceConfigurationArns().hashCode());
hashCode = prime * hashCode + ((getCustomerArtifactPaths() == null) ? 0 : getCustomerArtifactPaths().hashCode());
hashCode = prime * hashCode + ((getRadios() == null) ? 0 : getRadios().hashCode());
hashCode = prime * hashCode + ((getAuxiliaryApps() == null) ? 0 : getAuxiliaryApps().hashCode());
hashCode = prime * hashCode + ((getBillingMethod() == null) ? 0 : getBillingMethod().hashCode());
return hashCode;
}
@Override
public ScheduleRunConfiguration clone() {
try {
return (ScheduleRunConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.devicefarm.model.transform.ScheduleRunConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}