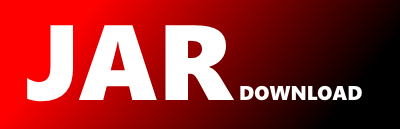
com.amazonaws.services.devicefarm.model.ScheduleRunTest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-devicefarm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.devicefarm.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Represents test settings. This data structure is passed in as the test parameter to ScheduleRun. For an example of
* the JSON request syntax, see ScheduleRun.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ScheduleRunTest implements Serializable, Cloneable, StructuredPojo {
/**
*
* The test's type.
*
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER. For Android, an app explorer that traverses an Android app, interacting with it and capturing
* screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
*
*/
private String type;
/**
*
* The ARN of the uploaded test to be run.
*
*/
private String testPackageArn;
/**
*
* The ARN of the YAML-formatted test specification.
*
*/
private String testSpecArn;
/**
*
* The test's filter.
*
*/
private String filter;
/**
*
* The test's parameters, such as test framework parameters and fixture settings. Parameters are represented by
* name-value pairs of strings.
*
*
* For all tests:
*
*
* -
*
* app_performance_monitoring
: Performance monitoring is enabled by default. Set this parameter to
* false to disable it.
*
*
*
*
* For Calabash tests:
*
*
* -
*
* profile: A cucumber profile (for example, my_profile_name
).
*
*
* -
*
* tags: You can limit execution to features or scenarios that have (or don't have) certain tags (for example, @smoke
* or @smoke,~@wip).
*
*
*
*
* For Appium tests (all types):
*
*
* -
*
* appium_version: The Appium version. Currently supported values are 1.6.5 (and later), latest, and default.
*
*
* -
*
* latest runs the latest Appium version supported by Device Farm (1.9.1).
*
*
* -
*
* For default, Device Farm selects a compatible version of Appium for the device. The current behavior is to run
* 1.7.2 on Android devices and iOS 9 and earlier and 1.7.2 for iOS 10 and later.
*
*
* -
*
* This behavior is subject to change.
*
*
*
*
*
*
* For fuzz tests (Android only):
*
*
* -
*
* event_count: The number of events, between 1 and 10000, that the UI fuzz test should perform.
*
*
* -
*
* throttle: The time, in ms, between 0 and 1000, that the UI fuzz test should wait between events.
*
*
* -
*
* seed: A seed to use for randomizing the UI fuzz test. Using the same seed value between tests ensures identical
* event sequences.
*
*
*
*
* For Explorer tests:
*
*
* -
*
* username: A user name to use if the Explorer encounters a login form. If not supplied, no user name is inserted.
*
*
* -
*
* password: A password to use if the Explorer encounters a login form. If not supplied, no password is inserted.
*
*
*
*
* For Instrumentation:
*
*
* -
*
* filter: A test filter string. Examples:
*
*
* -
*
* Running a single test case: com.android.abc.Test1
*
*
* -
*
* Running a single test: com.android.abc.Test1#smoke
*
*
* -
*
* Running multiple tests: com.android.abc.Test1,com.android.abc.Test2
*
*
*
*
*
*
* For XCTest and XCTestUI:
*
*
* -
*
* filter: A test filter string. Examples:
*
*
* -
*
* Running a single test class: LoginTests
*
*
* -
*
* Running a multiple test classes: LoginTests,SmokeTests
*
*
* -
*
* Running a single test: LoginTests/testValid
*
*
* -
*
* Running multiple tests: LoginTests/testValid,LoginTests/testInvalid
*
*
*
*
*
*
* For UIAutomator:
*
*
* -
*
* filter: A test filter string. Examples:
*
*
* -
*
* Running a single test case: com.android.abc.Test1
*
*
* -
*
* Running a single test: com.android.abc.Test1#smoke
*
*
* -
*
* Running multiple tests: com.android.abc.Test1,com.android.abc.Test2
*
*
*
*
*
*/
private java.util.Map parameters;
/**
*
* The test's type.
*
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER. For Android, an app explorer that traverses an Android app, interacting with it and capturing
* screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
*
*
* @param type
* The test's type.
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER. For Android, an app explorer that traverses an Android app, interacting with it and
* capturing screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
* @see TestType
*/
public void setType(String type) {
this.type = type;
}
/**
*
* The test's type.
*
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER. For Android, an app explorer that traverses an Android app, interacting with it and capturing
* screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
*
*
* @return The test's type.
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER. For Android, an app explorer that traverses an Android app, interacting with it and
* capturing screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
* @see TestType
*/
public String getType() {
return this.type;
}
/**
*
* The test's type.
*
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER. For Android, an app explorer that traverses an Android app, interacting with it and capturing
* screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
*
*
* @param type
* The test's type.
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER. For Android, an app explorer that traverses an Android app, interacting with it and
* capturing screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see TestType
*/
public ScheduleRunTest withType(String type) {
setType(type);
return this;
}
/**
*
* The test's type.
*
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER. For Android, an app explorer that traverses an Android app, interacting with it and capturing
* screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
*
*
* @param type
* The test's type.
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER. For Android, an app explorer that traverses an Android app, interacting with it and
* capturing screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
* @see TestType
*/
public void setType(TestType type) {
withType(type);
}
/**
*
* The test's type.
*
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER. For Android, an app explorer that traverses an Android app, interacting with it and capturing
* screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
*
*
* @param type
* The test's type.
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER. For Android, an app explorer that traverses an Android app, interacting with it and
* capturing screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see TestType
*/
public ScheduleRunTest withType(TestType type) {
this.type = type.toString();
return this;
}
/**
*
* The ARN of the uploaded test to be run.
*
*
* @param testPackageArn
* The ARN of the uploaded test to be run.
*/
public void setTestPackageArn(String testPackageArn) {
this.testPackageArn = testPackageArn;
}
/**
*
* The ARN of the uploaded test to be run.
*
*
* @return The ARN of the uploaded test to be run.
*/
public String getTestPackageArn() {
return this.testPackageArn;
}
/**
*
* The ARN of the uploaded test to be run.
*
*
* @param testPackageArn
* The ARN of the uploaded test to be run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScheduleRunTest withTestPackageArn(String testPackageArn) {
setTestPackageArn(testPackageArn);
return this;
}
/**
*
* The ARN of the YAML-formatted test specification.
*
*
* @param testSpecArn
* The ARN of the YAML-formatted test specification.
*/
public void setTestSpecArn(String testSpecArn) {
this.testSpecArn = testSpecArn;
}
/**
*
* The ARN of the YAML-formatted test specification.
*
*
* @return The ARN of the YAML-formatted test specification.
*/
public String getTestSpecArn() {
return this.testSpecArn;
}
/**
*
* The ARN of the YAML-formatted test specification.
*
*
* @param testSpecArn
* The ARN of the YAML-formatted test specification.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScheduleRunTest withTestSpecArn(String testSpecArn) {
setTestSpecArn(testSpecArn);
return this;
}
/**
*
* The test's filter.
*
*
* @param filter
* The test's filter.
*/
public void setFilter(String filter) {
this.filter = filter;
}
/**
*
* The test's filter.
*
*
* @return The test's filter.
*/
public String getFilter() {
return this.filter;
}
/**
*
* The test's filter.
*
*
* @param filter
* The test's filter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScheduleRunTest withFilter(String filter) {
setFilter(filter);
return this;
}
/**
*
* The test's parameters, such as test framework parameters and fixture settings. Parameters are represented by
* name-value pairs of strings.
*
*
* For all tests:
*
*
* -
*
* app_performance_monitoring
: Performance monitoring is enabled by default. Set this parameter to
* false to disable it.
*
*
*
*
* For Calabash tests:
*
*
* -
*
* profile: A cucumber profile (for example, my_profile_name
).
*
*
* -
*
* tags: You can limit execution to features or scenarios that have (or don't have) certain tags (for example, @smoke
* or @smoke,~@wip).
*
*
*
*
* For Appium tests (all types):
*
*
* -
*
* appium_version: The Appium version. Currently supported values are 1.6.5 (and later), latest, and default.
*
*
* -
*
* latest runs the latest Appium version supported by Device Farm (1.9.1).
*
*
* -
*
* For default, Device Farm selects a compatible version of Appium for the device. The current behavior is to run
* 1.7.2 on Android devices and iOS 9 and earlier and 1.7.2 for iOS 10 and later.
*
*
* -
*
* This behavior is subject to change.
*
*
*
*
*
*
* For fuzz tests (Android only):
*
*
* -
*
* event_count: The number of events, between 1 and 10000, that the UI fuzz test should perform.
*
*
* -
*
* throttle: The time, in ms, between 0 and 1000, that the UI fuzz test should wait between events.
*
*
* -
*
* seed: A seed to use for randomizing the UI fuzz test. Using the same seed value between tests ensures identical
* event sequences.
*
*
*
*
* For Explorer tests:
*
*
* -
*
* username: A user name to use if the Explorer encounters a login form. If not supplied, no user name is inserted.
*
*
* -
*
* password: A password to use if the Explorer encounters a login form. If not supplied, no password is inserted.
*
*
*
*
* For Instrumentation:
*
*
* -
*
* filter: A test filter string. Examples:
*
*
* -
*
* Running a single test case: com.android.abc.Test1
*
*
* -
*
* Running a single test: com.android.abc.Test1#smoke
*
*
* -
*
* Running multiple tests: com.android.abc.Test1,com.android.abc.Test2
*
*
*
*
*
*
* For XCTest and XCTestUI:
*
*
* -
*
* filter: A test filter string. Examples:
*
*
* -
*
* Running a single test class: LoginTests
*
*
* -
*
* Running a multiple test classes: LoginTests,SmokeTests
*
*
* -
*
* Running a single test: LoginTests/testValid
*
*
* -
*
* Running multiple tests: LoginTests/testValid,LoginTests/testInvalid
*
*
*
*
*
*
* For UIAutomator:
*
*
* -
*
* filter: A test filter string. Examples:
*
*
* -
*
* Running a single test case: com.android.abc.Test1
*
*
* -
*
* Running a single test: com.android.abc.Test1#smoke
*
*
* -
*
* Running multiple tests: com.android.abc.Test1,com.android.abc.Test2
*
*
*
*
*
*
* @return The test's parameters, such as test framework parameters and fixture settings. Parameters are represented
* by name-value pairs of strings.
*
* For all tests:
*
*
* -
*
* app_performance_monitoring
: Performance monitoring is enabled by default. Set this parameter
* to false to disable it.
*
*
*
*
* For Calabash tests:
*
*
* -
*
* profile: A cucumber profile (for example, my_profile_name
).
*
*
* -
*
* tags: You can limit execution to features or scenarios that have (or don't have) certain tags (for
* example, @smoke or @smoke,~@wip).
*
*
*
*
* For Appium tests (all types):
*
*
* -
*
* appium_version: The Appium version. Currently supported values are 1.6.5 (and later), latest, and
* default.
*
*
* -
*
* latest runs the latest Appium version supported by Device Farm (1.9.1).
*
*
* -
*
* For default, Device Farm selects a compatible version of Appium for the device. The current behavior is
* to run 1.7.2 on Android devices and iOS 9 and earlier and 1.7.2 for iOS 10 and later.
*
*
* -
*
* This behavior is subject to change.
*
*
*
*
*
*
* For fuzz tests (Android only):
*
*
* -
*
* event_count: The number of events, between 1 and 10000, that the UI fuzz test should perform.
*
*
* -
*
* throttle: The time, in ms, between 0 and 1000, that the UI fuzz test should wait between events.
*
*
* -
*
* seed: A seed to use for randomizing the UI fuzz test. Using the same seed value between tests ensures
* identical event sequences.
*
*
*
*
* For Explorer tests:
*
*
* -
*
* username: A user name to use if the Explorer encounters a login form. If not supplied, no user name is
* inserted.
*
*
* -
*
* password: A password to use if the Explorer encounters a login form. If not supplied, no password is
* inserted.
*
*
*
*
* For Instrumentation:
*
*
* -
*
* filter: A test filter string. Examples:
*
*
* -
*
* Running a single test case: com.android.abc.Test1
*
*
* -
*
* Running a single test: com.android.abc.Test1#smoke
*
*
* -
*
* Running multiple tests: com.android.abc.Test1,com.android.abc.Test2
*
*
*
*
*
*
* For XCTest and XCTestUI:
*
*
* -
*
* filter: A test filter string. Examples:
*
*
* -
*
* Running a single test class: LoginTests
*
*
* -
*
* Running a multiple test classes: LoginTests,SmokeTests
*
*
* -
*
* Running a single test: LoginTests/testValid
*
*
* -
*
* Running multiple tests: LoginTests/testValid,LoginTests/testInvalid
*
*
*
*
*
*
* For UIAutomator:
*
*
* -
*
* filter: A test filter string. Examples:
*
*
* -
*
* Running a single test case: com.android.abc.Test1
*
*
* -
*
* Running a single test: com.android.abc.Test1#smoke
*
*
* -
*
* Running multiple tests: com.android.abc.Test1,com.android.abc.Test2
*
*
*
*
*/
public java.util.Map getParameters() {
return parameters;
}
/**
*
* The test's parameters, such as test framework parameters and fixture settings. Parameters are represented by
* name-value pairs of strings.
*
*
* For all tests:
*
*
* -
*
* app_performance_monitoring
: Performance monitoring is enabled by default. Set this parameter to
* false to disable it.
*
*
*
*
* For Calabash tests:
*
*
* -
*
* profile: A cucumber profile (for example, my_profile_name
).
*
*
* -
*
* tags: You can limit execution to features or scenarios that have (or don't have) certain tags (for example, @smoke
* or @smoke,~@wip).
*
*
*
*
* For Appium tests (all types):
*
*
* -
*
* appium_version: The Appium version. Currently supported values are 1.6.5 (and later), latest, and default.
*
*
* -
*
* latest runs the latest Appium version supported by Device Farm (1.9.1).
*
*
* -
*
* For default, Device Farm selects a compatible version of Appium for the device. The current behavior is to run
* 1.7.2 on Android devices and iOS 9 and earlier and 1.7.2 for iOS 10 and later.
*
*
* -
*
* This behavior is subject to change.
*
*
*
*
*
*
* For fuzz tests (Android only):
*
*
* -
*
* event_count: The number of events, between 1 and 10000, that the UI fuzz test should perform.
*
*
* -
*
* throttle: The time, in ms, between 0 and 1000, that the UI fuzz test should wait between events.
*
*
* -
*
* seed: A seed to use for randomizing the UI fuzz test. Using the same seed value between tests ensures identical
* event sequences.
*
*
*
*
* For Explorer tests:
*
*
* -
*
* username: A user name to use if the Explorer encounters a login form. If not supplied, no user name is inserted.
*
*
* -
*
* password: A password to use if the Explorer encounters a login form. If not supplied, no password is inserted.
*
*
*
*
* For Instrumentation:
*
*
* -
*
* filter: A test filter string. Examples:
*
*
* -
*
* Running a single test case: com.android.abc.Test1
*
*
* -
*
* Running a single test: com.android.abc.Test1#smoke
*
*
* -
*
* Running multiple tests: com.android.abc.Test1,com.android.abc.Test2
*
*
*
*
*
*
* For XCTest and XCTestUI:
*
*
* -
*
* filter: A test filter string. Examples:
*
*
* -
*
* Running a single test class: LoginTests
*
*
* -
*
* Running a multiple test classes: LoginTests,SmokeTests
*
*
* -
*
* Running a single test: LoginTests/testValid
*
*
* -
*
* Running multiple tests: LoginTests/testValid,LoginTests/testInvalid
*
*
*
*
*
*
* For UIAutomator:
*
*
* -
*
* filter: A test filter string. Examples:
*
*
* -
*
* Running a single test case: com.android.abc.Test1
*
*
* -
*
* Running a single test: com.android.abc.Test1#smoke
*
*
* -
*
* Running multiple tests: com.android.abc.Test1,com.android.abc.Test2
*
*
*
*
*
*
* @param parameters
* The test's parameters, such as test framework parameters and fixture settings. Parameters are represented
* by name-value pairs of strings.
*
* For all tests:
*
*
* -
*
* app_performance_monitoring
: Performance monitoring is enabled by default. Set this parameter
* to false to disable it.
*
*
*
*
* For Calabash tests:
*
*
* -
*
* profile: A cucumber profile (for example, my_profile_name
).
*
*
* -
*
* tags: You can limit execution to features or scenarios that have (or don't have) certain tags (for
* example, @smoke or @smoke,~@wip).
*
*
*
*
* For Appium tests (all types):
*
*
* -
*
* appium_version: The Appium version. Currently supported values are 1.6.5 (and later), latest, and default.
*
*
* -
*
* latest runs the latest Appium version supported by Device Farm (1.9.1).
*
*
* -
*
* For default, Device Farm selects a compatible version of Appium for the device. The current behavior is to
* run 1.7.2 on Android devices and iOS 9 and earlier and 1.7.2 for iOS 10 and later.
*
*
* -
*
* This behavior is subject to change.
*
*
*
*
*
*
* For fuzz tests (Android only):
*
*
* -
*
* event_count: The number of events, between 1 and 10000, that the UI fuzz test should perform.
*
*
* -
*
* throttle: The time, in ms, between 0 and 1000, that the UI fuzz test should wait between events.
*
*
* -
*
* seed: A seed to use for randomizing the UI fuzz test. Using the same seed value between tests ensures
* identical event sequences.
*
*
*
*
* For Explorer tests:
*
*
* -
*
* username: A user name to use if the Explorer encounters a login form. If not supplied, no user name is
* inserted.
*
*
* -
*
* password: A password to use if the Explorer encounters a login form. If not supplied, no password is
* inserted.
*
*
*
*
* For Instrumentation:
*
*
* -
*
* filter: A test filter string. Examples:
*
*
* -
*
* Running a single test case: com.android.abc.Test1
*
*
* -
*
* Running a single test: com.android.abc.Test1#smoke
*
*
* -
*
* Running multiple tests: com.android.abc.Test1,com.android.abc.Test2
*
*
*
*
*
*
* For XCTest and XCTestUI:
*
*
* -
*
* filter: A test filter string. Examples:
*
*
* -
*
* Running a single test class: LoginTests
*
*
* -
*
* Running a multiple test classes: LoginTests,SmokeTests
*
*
* -
*
* Running a single test: LoginTests/testValid
*
*
* -
*
* Running multiple tests: LoginTests/testValid,LoginTests/testInvalid
*
*
*
*
*
*
* For UIAutomator:
*
*
* -
*
* filter: A test filter string. Examples:
*
*
* -
*
* Running a single test case: com.android.abc.Test1
*
*
* -
*
* Running a single test: com.android.abc.Test1#smoke
*
*
* -
*
* Running multiple tests: com.android.abc.Test1,com.android.abc.Test2
*
*
*
*
*/
public void setParameters(java.util.Map parameters) {
this.parameters = parameters;
}
/**
*
* The test's parameters, such as test framework parameters and fixture settings. Parameters are represented by
* name-value pairs of strings.
*
*
* For all tests:
*
*
* -
*
* app_performance_monitoring
: Performance monitoring is enabled by default. Set this parameter to
* false to disable it.
*
*
*
*
* For Calabash tests:
*
*
* -
*
* profile: A cucumber profile (for example, my_profile_name
).
*
*
* -
*
* tags: You can limit execution to features or scenarios that have (or don't have) certain tags (for example, @smoke
* or @smoke,~@wip).
*
*
*
*
* For Appium tests (all types):
*
*
* -
*
* appium_version: The Appium version. Currently supported values are 1.6.5 (and later), latest, and default.
*
*
* -
*
* latest runs the latest Appium version supported by Device Farm (1.9.1).
*
*
* -
*
* For default, Device Farm selects a compatible version of Appium for the device. The current behavior is to run
* 1.7.2 on Android devices and iOS 9 and earlier and 1.7.2 for iOS 10 and later.
*
*
* -
*
* This behavior is subject to change.
*
*
*
*
*
*
* For fuzz tests (Android only):
*
*
* -
*
* event_count: The number of events, between 1 and 10000, that the UI fuzz test should perform.
*
*
* -
*
* throttle: The time, in ms, between 0 and 1000, that the UI fuzz test should wait between events.
*
*
* -
*
* seed: A seed to use for randomizing the UI fuzz test. Using the same seed value between tests ensures identical
* event sequences.
*
*
*
*
* For Explorer tests:
*
*
* -
*
* username: A user name to use if the Explorer encounters a login form. If not supplied, no user name is inserted.
*
*
* -
*
* password: A password to use if the Explorer encounters a login form. If not supplied, no password is inserted.
*
*
*
*
* For Instrumentation:
*
*
* -
*
* filter: A test filter string. Examples:
*
*
* -
*
* Running a single test case: com.android.abc.Test1
*
*
* -
*
* Running a single test: com.android.abc.Test1#smoke
*
*
* -
*
* Running multiple tests: com.android.abc.Test1,com.android.abc.Test2
*
*
*
*
*
*
* For XCTest and XCTestUI:
*
*
* -
*
* filter: A test filter string. Examples:
*
*
* -
*
* Running a single test class: LoginTests
*
*
* -
*
* Running a multiple test classes: LoginTests,SmokeTests
*
*
* -
*
* Running a single test: LoginTests/testValid
*
*
* -
*
* Running multiple tests: LoginTests/testValid,LoginTests/testInvalid
*
*
*
*
*
*
* For UIAutomator:
*
*
* -
*
* filter: A test filter string. Examples:
*
*
* -
*
* Running a single test case: com.android.abc.Test1
*
*
* -
*
* Running a single test: com.android.abc.Test1#smoke
*
*
* -
*
* Running multiple tests: com.android.abc.Test1,com.android.abc.Test2
*
*
*
*
*
*
* @param parameters
* The test's parameters, such as test framework parameters and fixture settings. Parameters are represented
* by name-value pairs of strings.
*
* For all tests:
*
*
* -
*
* app_performance_monitoring
: Performance monitoring is enabled by default. Set this parameter
* to false to disable it.
*
*
*
*
* For Calabash tests:
*
*
* -
*
* profile: A cucumber profile (for example, my_profile_name
).
*
*
* -
*
* tags: You can limit execution to features or scenarios that have (or don't have) certain tags (for
* example, @smoke or @smoke,~@wip).
*
*
*
*
* For Appium tests (all types):
*
*
* -
*
* appium_version: The Appium version. Currently supported values are 1.6.5 (and later), latest, and default.
*
*
* -
*
* latest runs the latest Appium version supported by Device Farm (1.9.1).
*
*
* -
*
* For default, Device Farm selects a compatible version of Appium for the device. The current behavior is to
* run 1.7.2 on Android devices and iOS 9 and earlier and 1.7.2 for iOS 10 and later.
*
*
* -
*
* This behavior is subject to change.
*
*
*
*
*
*
* For fuzz tests (Android only):
*
*
* -
*
* event_count: The number of events, between 1 and 10000, that the UI fuzz test should perform.
*
*
* -
*
* throttle: The time, in ms, between 0 and 1000, that the UI fuzz test should wait between events.
*
*
* -
*
* seed: A seed to use for randomizing the UI fuzz test. Using the same seed value between tests ensures
* identical event sequences.
*
*
*
*
* For Explorer tests:
*
*
* -
*
* username: A user name to use if the Explorer encounters a login form. If not supplied, no user name is
* inserted.
*
*
* -
*
* password: A password to use if the Explorer encounters a login form. If not supplied, no password is
* inserted.
*
*
*
*
* For Instrumentation:
*
*
* -
*
* filter: A test filter string. Examples:
*
*
* -
*
* Running a single test case: com.android.abc.Test1
*
*
* -
*
* Running a single test: com.android.abc.Test1#smoke
*
*
* -
*
* Running multiple tests: com.android.abc.Test1,com.android.abc.Test2
*
*
*
*
*
*
* For XCTest and XCTestUI:
*
*
* -
*
* filter: A test filter string. Examples:
*
*
* -
*
* Running a single test class: LoginTests
*
*
* -
*
* Running a multiple test classes: LoginTests,SmokeTests
*
*
* -
*
* Running a single test: LoginTests/testValid
*
*
* -
*
* Running multiple tests: LoginTests/testValid,LoginTests/testInvalid
*
*
*
*
*
*
* For UIAutomator:
*
*
* -
*
* filter: A test filter string. Examples:
*
*
* -
*
* Running a single test case: com.android.abc.Test1
*
*
* -
*
* Running a single test: com.android.abc.Test1#smoke
*
*
* -
*
* Running multiple tests: com.android.abc.Test1,com.android.abc.Test2
*
*
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScheduleRunTest withParameters(java.util.Map parameters) {
setParameters(parameters);
return this;
}
/**
* Add a single Parameters entry
*
* @see ScheduleRunTest#withParameters
* @returns a reference to this object so that method calls can be chained together.
*/
public ScheduleRunTest addParametersEntry(String key, String value) {
if (null == this.parameters) {
this.parameters = new java.util.HashMap();
}
if (this.parameters.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.parameters.put(key, value);
return this;
}
/**
* Removes all the entries added into Parameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScheduleRunTest clearParametersEntries() {
this.parameters = null;
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getType() != null)
sb.append("Type: ").append(getType()).append(",");
if (getTestPackageArn() != null)
sb.append("TestPackageArn: ").append(getTestPackageArn()).append(",");
if (getTestSpecArn() != null)
sb.append("TestSpecArn: ").append(getTestSpecArn()).append(",");
if (getFilter() != null)
sb.append("Filter: ").append(getFilter()).append(",");
if (getParameters() != null)
sb.append("Parameters: ").append(getParameters());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ScheduleRunTest == false)
return false;
ScheduleRunTest other = (ScheduleRunTest) obj;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null && other.getType().equals(this.getType()) == false)
return false;
if (other.getTestPackageArn() == null ^ this.getTestPackageArn() == null)
return false;
if (other.getTestPackageArn() != null && other.getTestPackageArn().equals(this.getTestPackageArn()) == false)
return false;
if (other.getTestSpecArn() == null ^ this.getTestSpecArn() == null)
return false;
if (other.getTestSpecArn() != null && other.getTestSpecArn().equals(this.getTestSpecArn()) == false)
return false;
if (other.getFilter() == null ^ this.getFilter() == null)
return false;
if (other.getFilter() != null && other.getFilter().equals(this.getFilter()) == false)
return false;
if (other.getParameters() == null ^ this.getParameters() == null)
return false;
if (other.getParameters() != null && other.getParameters().equals(this.getParameters()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getType() == null) ? 0 : getType().hashCode());
hashCode = prime * hashCode + ((getTestPackageArn() == null) ? 0 : getTestPackageArn().hashCode());
hashCode = prime * hashCode + ((getTestSpecArn() == null) ? 0 : getTestSpecArn().hashCode());
hashCode = prime * hashCode + ((getFilter() == null) ? 0 : getFilter().hashCode());
hashCode = prime * hashCode + ((getParameters() == null) ? 0 : getParameters().hashCode());
return hashCode;
}
@Override
public ScheduleRunTest clone() {
try {
return (ScheduleRunTest) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.devicefarm.model.transform.ScheduleRunTestMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}