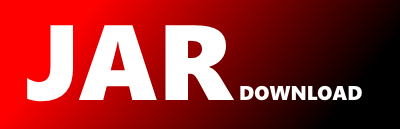
com.amazonaws.services.devicefarm.AWSDeviceFarmAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-devicefarm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.devicefarm;
import javax.annotation.Generated;
import com.amazonaws.services.devicefarm.model.*;
/**
* Interface for accessing AWS Device Farm asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.devicefarm.AbstractAWSDeviceFarmAsync} instead.
*
*
*
* Welcome to the AWS Device Farm API documentation, which contains APIs for:
*
*
* -
*
* Testing on desktop browsers
*
*
* Device Farm makes it possible for you to test your web applications on desktop browsers using Selenium. The APIs for
* desktop browser testing contain TestGrid
in their names. For more information, see Testing Web Applications on Selenium with Device
* Farm.
*
*
* -
*
* Testing on real mobile devices
*
*
* Device Farm makes it possible for you to test apps on physical phones, tablets, and other devices in the cloud. For
* more information, see the Device Farm
* Developer Guide.
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSDeviceFarmAsync extends AWSDeviceFarm {
/**
*
* Creates a device pool.
*
*
* @param createDevicePoolRequest
* Represents a request to the create device pool operation.
* @return A Java Future containing the result of the CreateDevicePool operation returned by the service.
* @sample AWSDeviceFarmAsync.CreateDevicePool
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDevicePoolAsync(CreateDevicePoolRequest createDevicePoolRequest);
/**
*
* Creates a device pool.
*
*
* @param createDevicePoolRequest
* Represents a request to the create device pool operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDevicePool operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.CreateDevicePool
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDevicePoolAsync(CreateDevicePoolRequest createDevicePoolRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a profile that can be applied to one or more private fleet device instances.
*
*
* @param createInstanceProfileRequest
* @return A Java Future containing the result of the CreateInstanceProfile operation returned by the service.
* @sample AWSDeviceFarmAsync.CreateInstanceProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future createInstanceProfileAsync(CreateInstanceProfileRequest createInstanceProfileRequest);
/**
*
* Creates a profile that can be applied to one or more private fleet device instances.
*
*
* @param createInstanceProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateInstanceProfile operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.CreateInstanceProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future createInstanceProfileAsync(CreateInstanceProfileRequest createInstanceProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a network profile.
*
*
* @param createNetworkProfileRequest
* @return A Java Future containing the result of the CreateNetworkProfile operation returned by the service.
* @sample AWSDeviceFarmAsync.CreateNetworkProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future createNetworkProfileAsync(CreateNetworkProfileRequest createNetworkProfileRequest);
/**
*
* Creates a network profile.
*
*
* @param createNetworkProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateNetworkProfile operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.CreateNetworkProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future createNetworkProfileAsync(CreateNetworkProfileRequest createNetworkProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a project.
*
*
* @param createProjectRequest
* Represents a request to the create project operation.
* @return A Java Future containing the result of the CreateProject operation returned by the service.
* @sample AWSDeviceFarmAsync.CreateProject
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createProjectAsync(CreateProjectRequest createProjectRequest);
/**
*
* Creates a project.
*
*
* @param createProjectRequest
* Represents a request to the create project operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateProject operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.CreateProject
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createProjectAsync(CreateProjectRequest createProjectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Specifies and starts a remote access session.
*
*
* @param createRemoteAccessSessionRequest
* Creates and submits a request to start a remote access session.
* @return A Java Future containing the result of the CreateRemoteAccessSession operation returned by the service.
* @sample AWSDeviceFarmAsync.CreateRemoteAccessSession
* @see AWS API Documentation
*/
java.util.concurrent.Future createRemoteAccessSessionAsync(
CreateRemoteAccessSessionRequest createRemoteAccessSessionRequest);
/**
*
* Specifies and starts a remote access session.
*
*
* @param createRemoteAccessSessionRequest
* Creates and submits a request to start a remote access session.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRemoteAccessSession operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.CreateRemoteAccessSession
* @see AWS API Documentation
*/
java.util.concurrent.Future createRemoteAccessSessionAsync(
CreateRemoteAccessSessionRequest createRemoteAccessSessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a Selenium testing project. Projects are used to track TestGridSession instances.
*
*
* @param createTestGridProjectRequest
* @return A Java Future containing the result of the CreateTestGridProject operation returned by the service.
* @sample AWSDeviceFarmAsync.CreateTestGridProject
* @see AWS API Documentation
*/
java.util.concurrent.Future createTestGridProjectAsync(CreateTestGridProjectRequest createTestGridProjectRequest);
/**
*
* Creates a Selenium testing project. Projects are used to track TestGridSession instances.
*
*
* @param createTestGridProjectRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTestGridProject operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.CreateTestGridProject
* @see AWS API Documentation
*/
java.util.concurrent.Future createTestGridProjectAsync(CreateTestGridProjectRequest createTestGridProjectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a signed, short-term URL that can be passed to a Selenium RemoteWebDriver
constructor.
*
*
* @param createTestGridUrlRequest
* @return A Java Future containing the result of the CreateTestGridUrl operation returned by the service.
* @sample AWSDeviceFarmAsync.CreateTestGridUrl
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createTestGridUrlAsync(CreateTestGridUrlRequest createTestGridUrlRequest);
/**
*
* Creates a signed, short-term URL that can be passed to a Selenium RemoteWebDriver
constructor.
*
*
* @param createTestGridUrlRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTestGridUrl operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.CreateTestGridUrl
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createTestGridUrlAsync(CreateTestGridUrlRequest createTestGridUrlRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Uploads an app or test scripts.
*
*
* @param createUploadRequest
* Represents a request to the create upload operation.
* @return A Java Future containing the result of the CreateUpload operation returned by the service.
* @sample AWSDeviceFarmAsync.CreateUpload
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createUploadAsync(CreateUploadRequest createUploadRequest);
/**
*
* Uploads an app or test scripts.
*
*
* @param createUploadRequest
* Represents a request to the create upload operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateUpload operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.CreateUpload
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createUploadAsync(CreateUploadRequest createUploadRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a configuration record in Device Farm for your Amazon Virtual Private Cloud (VPC) endpoint.
*
*
* @param createVPCEConfigurationRequest
* @return A Java Future containing the result of the CreateVPCEConfiguration operation returned by the service.
* @sample AWSDeviceFarmAsync.CreateVPCEConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createVPCEConfigurationAsync(CreateVPCEConfigurationRequest createVPCEConfigurationRequest);
/**
*
* Creates a configuration record in Device Farm for your Amazon Virtual Private Cloud (VPC) endpoint.
*
*
* @param createVPCEConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateVPCEConfiguration operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.CreateVPCEConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createVPCEConfigurationAsync(CreateVPCEConfigurationRequest createVPCEConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a device pool given the pool ARN. Does not allow deletion of curated pools owned by the system.
*
*
* @param deleteDevicePoolRequest
* Represents a request to the delete device pool operation.
* @return A Java Future containing the result of the DeleteDevicePool operation returned by the service.
* @sample AWSDeviceFarmAsync.DeleteDevicePool
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDevicePoolAsync(DeleteDevicePoolRequest deleteDevicePoolRequest);
/**
*
* Deletes a device pool given the pool ARN. Does not allow deletion of curated pools owned by the system.
*
*
* @param deleteDevicePoolRequest
* Represents a request to the delete device pool operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDevicePool operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.DeleteDevicePool
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDevicePoolAsync(DeleteDevicePoolRequest deleteDevicePoolRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a profile that can be applied to one or more private device instances.
*
*
* @param deleteInstanceProfileRequest
* @return A Java Future containing the result of the DeleteInstanceProfile operation returned by the service.
* @sample AWSDeviceFarmAsync.DeleteInstanceProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteInstanceProfileAsync(DeleteInstanceProfileRequest deleteInstanceProfileRequest);
/**
*
* Deletes a profile that can be applied to one or more private device instances.
*
*
* @param deleteInstanceProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteInstanceProfile operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.DeleteInstanceProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteInstanceProfileAsync(DeleteInstanceProfileRequest deleteInstanceProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a network profile.
*
*
* @param deleteNetworkProfileRequest
* @return A Java Future containing the result of the DeleteNetworkProfile operation returned by the service.
* @sample AWSDeviceFarmAsync.DeleteNetworkProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteNetworkProfileAsync(DeleteNetworkProfileRequest deleteNetworkProfileRequest);
/**
*
* Deletes a network profile.
*
*
* @param deleteNetworkProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteNetworkProfile operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.DeleteNetworkProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteNetworkProfileAsync(DeleteNetworkProfileRequest deleteNetworkProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an AWS Device Farm project, given the project ARN.
*
*
* Deleting this resource does not stop an in-progress run.
*
*
* @param deleteProjectRequest
* Represents a request to the delete project operation.
* @return A Java Future containing the result of the DeleteProject operation returned by the service.
* @sample AWSDeviceFarmAsync.DeleteProject
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteProjectAsync(DeleteProjectRequest deleteProjectRequest);
/**
*
* Deletes an AWS Device Farm project, given the project ARN.
*
*
* Deleting this resource does not stop an in-progress run.
*
*
* @param deleteProjectRequest
* Represents a request to the delete project operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteProject operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.DeleteProject
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteProjectAsync(DeleteProjectRequest deleteProjectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a completed remote access session and its results.
*
*
* @param deleteRemoteAccessSessionRequest
* Represents the request to delete the specified remote access session.
* @return A Java Future containing the result of the DeleteRemoteAccessSession operation returned by the service.
* @sample AWSDeviceFarmAsync.DeleteRemoteAccessSession
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRemoteAccessSessionAsync(
DeleteRemoteAccessSessionRequest deleteRemoteAccessSessionRequest);
/**
*
* Deletes a completed remote access session and its results.
*
*
* @param deleteRemoteAccessSessionRequest
* Represents the request to delete the specified remote access session.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRemoteAccessSession operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.DeleteRemoteAccessSession
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRemoteAccessSessionAsync(
DeleteRemoteAccessSessionRequest deleteRemoteAccessSessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the run, given the run ARN.
*
*
* Deleting this resource does not stop an in-progress run.
*
*
* @param deleteRunRequest
* Represents a request to the delete run operation.
* @return A Java Future containing the result of the DeleteRun operation returned by the service.
* @sample AWSDeviceFarmAsync.DeleteRun
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteRunAsync(DeleteRunRequest deleteRunRequest);
/**
*
* Deletes the run, given the run ARN.
*
*
* Deleting this resource does not stop an in-progress run.
*
*
* @param deleteRunRequest
* Represents a request to the delete run operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRun operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.DeleteRun
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteRunAsync(DeleteRunRequest deleteRunRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a Selenium testing project and all content generated under it.
*
*
*
* You cannot undo this operation.
*
*
*
* You cannot delete a project if it has active sessions.
*
*
*
* @param deleteTestGridProjectRequest
* @return A Java Future containing the result of the DeleteTestGridProject operation returned by the service.
* @sample AWSDeviceFarmAsync.DeleteTestGridProject
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteTestGridProjectAsync(DeleteTestGridProjectRequest deleteTestGridProjectRequest);
/**
*
* Deletes a Selenium testing project and all content generated under it.
*
*
*
* You cannot undo this operation.
*
*
*
* You cannot delete a project if it has active sessions.
*
*
*
* @param deleteTestGridProjectRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTestGridProject operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.DeleteTestGridProject
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteTestGridProjectAsync(DeleteTestGridProjectRequest deleteTestGridProjectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an upload given the upload ARN.
*
*
* @param deleteUploadRequest
* Represents a request to the delete upload operation.
* @return A Java Future containing the result of the DeleteUpload operation returned by the service.
* @sample AWSDeviceFarmAsync.DeleteUpload
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteUploadAsync(DeleteUploadRequest deleteUploadRequest);
/**
*
* Deletes an upload given the upload ARN.
*
*
* @param deleteUploadRequest
* Represents a request to the delete upload operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteUpload operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.DeleteUpload
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteUploadAsync(DeleteUploadRequest deleteUploadRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a configuration for your Amazon Virtual Private Cloud (VPC) endpoint.
*
*
* @param deleteVPCEConfigurationRequest
* @return A Java Future containing the result of the DeleteVPCEConfiguration operation returned by the service.
* @sample AWSDeviceFarmAsync.DeleteVPCEConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteVPCEConfigurationAsync(DeleteVPCEConfigurationRequest deleteVPCEConfigurationRequest);
/**
*
* Deletes a configuration for your Amazon Virtual Private Cloud (VPC) endpoint.
*
*
* @param deleteVPCEConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVPCEConfiguration operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.DeleteVPCEConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteVPCEConfigurationAsync(DeleteVPCEConfigurationRequest deleteVPCEConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the number of unmetered iOS or unmetered Android devices that have been purchased by the account.
*
*
* @param getAccountSettingsRequest
* Represents the request sent to retrieve the account settings.
* @return A Java Future containing the result of the GetAccountSettings operation returned by the service.
* @sample AWSDeviceFarmAsync.GetAccountSettings
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getAccountSettingsAsync(GetAccountSettingsRequest getAccountSettingsRequest);
/**
*
* Returns the number of unmetered iOS or unmetered Android devices that have been purchased by the account.
*
*
* @param getAccountSettingsRequest
* Represents the request sent to retrieve the account settings.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAccountSettings operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.GetAccountSettings
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getAccountSettingsAsync(GetAccountSettingsRequest getAccountSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about a unique device type.
*
*
* @param getDeviceRequest
* Represents a request to the get device request.
* @return A Java Future containing the result of the GetDevice operation returned by the service.
* @sample AWSDeviceFarmAsync.GetDevice
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDeviceAsync(GetDeviceRequest getDeviceRequest);
/**
*
* Gets information about a unique device type.
*
*
* @param getDeviceRequest
* Represents a request to the get device request.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDevice operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.GetDevice
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDeviceAsync(GetDeviceRequest getDeviceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a device instance that belongs to a private device fleet.
*
*
* @param getDeviceInstanceRequest
* @return A Java Future containing the result of the GetDeviceInstance operation returned by the service.
* @sample AWSDeviceFarmAsync.GetDeviceInstance
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getDeviceInstanceAsync(GetDeviceInstanceRequest getDeviceInstanceRequest);
/**
*
* Returns information about a device instance that belongs to a private device fleet.
*
*
* @param getDeviceInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDeviceInstance operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.GetDeviceInstance
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getDeviceInstanceAsync(GetDeviceInstanceRequest getDeviceInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about a device pool.
*
*
* @param getDevicePoolRequest
* Represents a request to the get device pool operation.
* @return A Java Future containing the result of the GetDevicePool operation returned by the service.
* @sample AWSDeviceFarmAsync.GetDevicePool
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDevicePoolAsync(GetDevicePoolRequest getDevicePoolRequest);
/**
*
* Gets information about a device pool.
*
*
* @param getDevicePoolRequest
* Represents a request to the get device pool operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDevicePool operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.GetDevicePool
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDevicePoolAsync(GetDevicePoolRequest getDevicePoolRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about compatibility with a device pool.
*
*
* @param getDevicePoolCompatibilityRequest
* Represents a request to the get device pool compatibility operation.
* @return A Java Future containing the result of the GetDevicePoolCompatibility operation returned by the service.
* @sample AWSDeviceFarmAsync.GetDevicePoolCompatibility
* @see AWS API Documentation
*/
java.util.concurrent.Future getDevicePoolCompatibilityAsync(
GetDevicePoolCompatibilityRequest getDevicePoolCompatibilityRequest);
/**
*
* Gets information about compatibility with a device pool.
*
*
* @param getDevicePoolCompatibilityRequest
* Represents a request to the get device pool compatibility operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDevicePoolCompatibility operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.GetDevicePoolCompatibility
* @see AWS API Documentation
*/
java.util.concurrent.Future getDevicePoolCompatibilityAsync(
GetDevicePoolCompatibilityRequest getDevicePoolCompatibilityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the specified instance profile.
*
*
* @param getInstanceProfileRequest
* @return A Java Future containing the result of the GetInstanceProfile operation returned by the service.
* @sample AWSDeviceFarmAsync.GetInstanceProfile
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getInstanceProfileAsync(GetInstanceProfileRequest getInstanceProfileRequest);
/**
*
* Returns information about the specified instance profile.
*
*
* @param getInstanceProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetInstanceProfile operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.GetInstanceProfile
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getInstanceProfileAsync(GetInstanceProfileRequest getInstanceProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about a job.
*
*
* @param getJobRequest
* Represents a request to the get job operation.
* @return A Java Future containing the result of the GetJob operation returned by the service.
* @sample AWSDeviceFarmAsync.GetJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getJobAsync(GetJobRequest getJobRequest);
/**
*
* Gets information about a job.
*
*
* @param getJobRequest
* Represents a request to the get job operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetJob operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.GetJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getJobAsync(GetJobRequest getJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a network profile.
*
*
* @param getNetworkProfileRequest
* @return A Java Future containing the result of the GetNetworkProfile operation returned by the service.
* @sample AWSDeviceFarmAsync.GetNetworkProfile
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getNetworkProfileAsync(GetNetworkProfileRequest getNetworkProfileRequest);
/**
*
* Returns information about a network profile.
*
*
* @param getNetworkProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetNetworkProfile operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.GetNetworkProfile
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getNetworkProfileAsync(GetNetworkProfileRequest getNetworkProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the current status and future status of all offerings purchased by an AWS account. The response indicates
* how many offerings are currently available and the offerings that will be available in the next period. The API
* returns a NotEligible
error if the user is not permitted to invoke the operation. If you must be
* able to invoke this operation, contact [email protected].
*
*
* @param getOfferingStatusRequest
* Represents the request to retrieve the offering status for the specified customer or account.
* @return A Java Future containing the result of the GetOfferingStatus operation returned by the service.
* @sample AWSDeviceFarmAsync.GetOfferingStatus
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getOfferingStatusAsync(GetOfferingStatusRequest getOfferingStatusRequest);
/**
*
* Gets the current status and future status of all offerings purchased by an AWS account. The response indicates
* how many offerings are currently available and the offerings that will be available in the next period. The API
* returns a NotEligible
error if the user is not permitted to invoke the operation. If you must be
* able to invoke this operation, contact [email protected].
*
*
* @param getOfferingStatusRequest
* Represents the request to retrieve the offering status for the specified customer or account.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetOfferingStatus operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.GetOfferingStatus
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getOfferingStatusAsync(GetOfferingStatusRequest getOfferingStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about a project.
*
*
* @param getProjectRequest
* Represents a request to the get project operation.
* @return A Java Future containing the result of the GetProject operation returned by the service.
* @sample AWSDeviceFarmAsync.GetProject
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getProjectAsync(GetProjectRequest getProjectRequest);
/**
*
* Gets information about a project.
*
*
* @param getProjectRequest
* Represents a request to the get project operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetProject operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.GetProject
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getProjectAsync(GetProjectRequest getProjectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a link to a currently running remote access session.
*
*
* @param getRemoteAccessSessionRequest
* Represents the request to get information about the specified remote access session.
* @return A Java Future containing the result of the GetRemoteAccessSession operation returned by the service.
* @sample AWSDeviceFarmAsync.GetRemoteAccessSession
* @see AWS API Documentation
*/
java.util.concurrent.Future getRemoteAccessSessionAsync(GetRemoteAccessSessionRequest getRemoteAccessSessionRequest);
/**
*
* Returns a link to a currently running remote access session.
*
*
* @param getRemoteAccessSessionRequest
* Represents the request to get information about the specified remote access session.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetRemoteAccessSession operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.GetRemoteAccessSession
* @see AWS API Documentation
*/
java.util.concurrent.Future getRemoteAccessSessionAsync(GetRemoteAccessSessionRequest getRemoteAccessSessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about a run.
*
*
* @param getRunRequest
* Represents a request to the get run operation.
* @return A Java Future containing the result of the GetRun operation returned by the service.
* @sample AWSDeviceFarmAsync.GetRun
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getRunAsync(GetRunRequest getRunRequest);
/**
*
* Gets information about a run.
*
*
* @param getRunRequest
* Represents a request to the get run operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetRun operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.GetRun
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getRunAsync(GetRunRequest getRunRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about a suite.
*
*
* @param getSuiteRequest
* Represents a request to the get suite operation.
* @return A Java Future containing the result of the GetSuite operation returned by the service.
* @sample AWSDeviceFarmAsync.GetSuite
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSuiteAsync(GetSuiteRequest getSuiteRequest);
/**
*
* Gets information about a suite.
*
*
* @param getSuiteRequest
* Represents a request to the get suite operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSuite operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.GetSuite
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSuiteAsync(GetSuiteRequest getSuiteRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about a test.
*
*
* @param getTestRequest
* Represents a request to the get test operation.
* @return A Java Future containing the result of the GetTest operation returned by the service.
* @sample AWSDeviceFarmAsync.GetTest
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTestAsync(GetTestRequest getTestRequest);
/**
*
* Gets information about a test.
*
*
* @param getTestRequest
* Represents a request to the get test operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTest operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.GetTest
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTestAsync(GetTestRequest getTestRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about a Selenium testing project.
*
*
* @param getTestGridProjectRequest
* @return A Java Future containing the result of the GetTestGridProject operation returned by the service.
* @sample AWSDeviceFarmAsync.GetTestGridProject
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getTestGridProjectAsync(GetTestGridProjectRequest getTestGridProjectRequest);
/**
*
* Retrieves information about a Selenium testing project.
*
*
* @param getTestGridProjectRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTestGridProject operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.GetTestGridProject
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getTestGridProjectAsync(GetTestGridProjectRequest getTestGridProjectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* A session is an instance of a browser created through a RemoteWebDriver
with the URL from
* CreateTestGridUrlResult$url. You can use the following to look up sessions:
*
*
* -
*
* The session ARN (GetTestGridSessionRequest$sessionArn).
*
*
* -
*
* The project ARN and a session ID (GetTestGridSessionRequest$projectArn and
* GetTestGridSessionRequest$sessionId).
*
*
*
*
*
* @param getTestGridSessionRequest
* @return A Java Future containing the result of the GetTestGridSession operation returned by the service.
* @sample AWSDeviceFarmAsync.GetTestGridSession
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getTestGridSessionAsync(GetTestGridSessionRequest getTestGridSessionRequest);
/**
*
* A session is an instance of a browser created through a RemoteWebDriver
with the URL from
* CreateTestGridUrlResult$url. You can use the following to look up sessions:
*
*
* -
*
* The session ARN (GetTestGridSessionRequest$sessionArn).
*
*
* -
*
* The project ARN and a session ID (GetTestGridSessionRequest$projectArn and
* GetTestGridSessionRequest$sessionId).
*
*
*
*
*
* @param getTestGridSessionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTestGridSession operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.GetTestGridSession
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getTestGridSessionAsync(GetTestGridSessionRequest getTestGridSessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about an upload.
*
*
* @param getUploadRequest
* Represents a request to the get upload operation.
* @return A Java Future containing the result of the GetUpload operation returned by the service.
* @sample AWSDeviceFarmAsync.GetUpload
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getUploadAsync(GetUploadRequest getUploadRequest);
/**
*
* Gets information about an upload.
*
*
* @param getUploadRequest
* Represents a request to the get upload operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetUpload operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.GetUpload
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getUploadAsync(GetUploadRequest getUploadRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the configuration settings for your Amazon Virtual Private Cloud (VPC) endpoint.
*
*
* @param getVPCEConfigurationRequest
* @return A Java Future containing the result of the GetVPCEConfiguration operation returned by the service.
* @sample AWSDeviceFarmAsync.GetVPCEConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getVPCEConfigurationAsync(GetVPCEConfigurationRequest getVPCEConfigurationRequest);
/**
*
* Returns information about the configuration settings for your Amazon Virtual Private Cloud (VPC) endpoint.
*
*
* @param getVPCEConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetVPCEConfiguration operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.GetVPCEConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getVPCEConfigurationAsync(GetVPCEConfigurationRequest getVPCEConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Installs an application to the device in a remote access session. For Android applications, the file must be in
* .apk format. For iOS applications, the file must be in .ipa format.
*
*
* @param installToRemoteAccessSessionRequest
* Represents the request to install an Android application (in .apk format) or an iOS application (in .ipa
* format) as part of a remote access session.
* @return A Java Future containing the result of the InstallToRemoteAccessSession operation returned by the
* service.
* @sample AWSDeviceFarmAsync.InstallToRemoteAccessSession
* @see AWS API Documentation
*/
java.util.concurrent.Future installToRemoteAccessSessionAsync(
InstallToRemoteAccessSessionRequest installToRemoteAccessSessionRequest);
/**
*
* Installs an application to the device in a remote access session. For Android applications, the file must be in
* .apk format. For iOS applications, the file must be in .ipa format.
*
*
* @param installToRemoteAccessSessionRequest
* Represents the request to install an Android application (in .apk format) or an iOS application (in .ipa
* format) as part of a remote access session.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the InstallToRemoteAccessSession operation returned by the
* service.
* @sample AWSDeviceFarmAsyncHandler.InstallToRemoteAccessSession
* @see AWS API Documentation
*/
java.util.concurrent.Future installToRemoteAccessSessionAsync(
InstallToRemoteAccessSessionRequest installToRemoteAccessSessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about artifacts.
*
*
* @param listArtifactsRequest
* Represents a request to the list artifacts operation.
* @return A Java Future containing the result of the ListArtifacts operation returned by the service.
* @sample AWSDeviceFarmAsync.ListArtifacts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listArtifactsAsync(ListArtifactsRequest listArtifactsRequest);
/**
*
* Gets information about artifacts.
*
*
* @param listArtifactsRequest
* Represents a request to the list artifacts operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListArtifacts operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListArtifacts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listArtifactsAsync(ListArtifactsRequest listArtifactsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the private device instances associated with one or more AWS accounts.
*
*
* @param listDeviceInstancesRequest
* @return A Java Future containing the result of the ListDeviceInstances operation returned by the service.
* @sample AWSDeviceFarmAsync.ListDeviceInstances
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDeviceInstancesAsync(ListDeviceInstancesRequest listDeviceInstancesRequest);
/**
*
* Returns information about the private device instances associated with one or more AWS accounts.
*
*
* @param listDeviceInstancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDeviceInstances operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListDeviceInstances
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDeviceInstancesAsync(ListDeviceInstancesRequest listDeviceInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about device pools.
*
*
* @param listDevicePoolsRequest
* Represents the result of a list device pools request.
* @return A Java Future containing the result of the ListDevicePools operation returned by the service.
* @sample AWSDeviceFarmAsync.ListDevicePools
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDevicePoolsAsync(ListDevicePoolsRequest listDevicePoolsRequest);
/**
*
* Gets information about device pools.
*
*
* @param listDevicePoolsRequest
* Represents the result of a list device pools request.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDevicePools operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListDevicePools
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDevicePoolsAsync(ListDevicePoolsRequest listDevicePoolsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about unique device types.
*
*
* @param listDevicesRequest
* Represents the result of a list devices request.
* @return A Java Future containing the result of the ListDevices operation returned by the service.
* @sample AWSDeviceFarmAsync.ListDevices
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDevicesAsync(ListDevicesRequest listDevicesRequest);
/**
*
* Gets information about unique device types.
*
*
* @param listDevicesRequest
* Represents the result of a list devices request.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDevices operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListDevices
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDevicesAsync(ListDevicesRequest listDevicesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about all the instance profiles in an AWS account.
*
*
* @param listInstanceProfilesRequest
* @return A Java Future containing the result of the ListInstanceProfiles operation returned by the service.
* @sample AWSDeviceFarmAsync.ListInstanceProfiles
* @see AWS API Documentation
*/
java.util.concurrent.Future listInstanceProfilesAsync(ListInstanceProfilesRequest listInstanceProfilesRequest);
/**
*
* Returns information about all the instance profiles in an AWS account.
*
*
* @param listInstanceProfilesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListInstanceProfiles operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListInstanceProfiles
* @see AWS API Documentation
*/
java.util.concurrent.Future listInstanceProfilesAsync(ListInstanceProfilesRequest listInstanceProfilesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about jobs for a given test run.
*
*
* @param listJobsRequest
* Represents a request to the list jobs operation.
* @return A Java Future containing the result of the ListJobs operation returned by the service.
* @sample AWSDeviceFarmAsync.ListJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listJobsAsync(ListJobsRequest listJobsRequest);
/**
*
* Gets information about jobs for a given test run.
*
*
* @param listJobsRequest
* Represents a request to the list jobs operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListJobs operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listJobsAsync(ListJobsRequest listJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the list of available network profiles.
*
*
* @param listNetworkProfilesRequest
* @return A Java Future containing the result of the ListNetworkProfiles operation returned by the service.
* @sample AWSDeviceFarmAsync.ListNetworkProfiles
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listNetworkProfilesAsync(ListNetworkProfilesRequest listNetworkProfilesRequest);
/**
*
* Returns the list of available network profiles.
*
*
* @param listNetworkProfilesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListNetworkProfiles operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListNetworkProfiles
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listNetworkProfilesAsync(ListNetworkProfilesRequest listNetworkProfilesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of offering promotions. Each offering promotion record contains the ID and description of the
* promotion. The API returns a NotEligible
error if the caller is not permitted to invoke the
* operation. Contact [email protected] if
* you must be able to invoke this operation.
*
*
* @param listOfferingPromotionsRequest
* @return A Java Future containing the result of the ListOfferingPromotions operation returned by the service.
* @sample AWSDeviceFarmAsync.ListOfferingPromotions
* @see AWS API Documentation
*/
java.util.concurrent.Future listOfferingPromotionsAsync(ListOfferingPromotionsRequest listOfferingPromotionsRequest);
/**
*
* Returns a list of offering promotions. Each offering promotion record contains the ID and description of the
* promotion. The API returns a NotEligible
error if the caller is not permitted to invoke the
* operation. Contact [email protected] if
* you must be able to invoke this operation.
*
*
* @param listOfferingPromotionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListOfferingPromotions operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListOfferingPromotions
* @see AWS API Documentation
*/
java.util.concurrent.Future listOfferingPromotionsAsync(ListOfferingPromotionsRequest listOfferingPromotionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all historical purchases, renewals, and system renewal transactions for an AWS account. The
* list is paginated and ordered by a descending timestamp (most recent transactions are first). The API returns a
* NotEligible
error if the user is not permitted to invoke the operation. If you must be able to
* invoke this operation, contact [email protected].
*
*
* @param listOfferingTransactionsRequest
* Represents the request to list the offering transaction history.
* @return A Java Future containing the result of the ListOfferingTransactions operation returned by the service.
* @sample AWSDeviceFarmAsync.ListOfferingTransactions
* @see AWS API Documentation
*/
java.util.concurrent.Future listOfferingTransactionsAsync(ListOfferingTransactionsRequest listOfferingTransactionsRequest);
/**
*
* Returns a list of all historical purchases, renewals, and system renewal transactions for an AWS account. The
* list is paginated and ordered by a descending timestamp (most recent transactions are first). The API returns a
* NotEligible
error if the user is not permitted to invoke the operation. If you must be able to
* invoke this operation, contact [email protected].
*
*
* @param listOfferingTransactionsRequest
* Represents the request to list the offering transaction history.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListOfferingTransactions operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListOfferingTransactions
* @see AWS API Documentation
*/
java.util.concurrent.Future listOfferingTransactionsAsync(ListOfferingTransactionsRequest listOfferingTransactionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of products or offerings that the user can manage through the API. Each offering record indicates
* the recurring price per unit and the frequency for that offering. The API returns a NotEligible
* error if the user is not permitted to invoke the operation. If you must be able to invoke this operation, contact
* [email protected].
*
*
* @param listOfferingsRequest
* Represents the request to list all offerings.
* @return A Java Future containing the result of the ListOfferings operation returned by the service.
* @sample AWSDeviceFarmAsync.ListOfferings
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listOfferingsAsync(ListOfferingsRequest listOfferingsRequest);
/**
*
* Returns a list of products or offerings that the user can manage through the API. Each offering record indicates
* the recurring price per unit and the frequency for that offering. The API returns a NotEligible
* error if the user is not permitted to invoke the operation. If you must be able to invoke this operation, contact
* [email protected].
*
*
* @param listOfferingsRequest
* Represents the request to list all offerings.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListOfferings operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListOfferings
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listOfferingsAsync(ListOfferingsRequest listOfferingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about projects.
*
*
* @param listProjectsRequest
* Represents a request to the list projects operation.
* @return A Java Future containing the result of the ListProjects operation returned by the service.
* @sample AWSDeviceFarmAsync.ListProjects
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listProjectsAsync(ListProjectsRequest listProjectsRequest);
/**
*
* Gets information about projects.
*
*
* @param listProjectsRequest
* Represents a request to the list projects operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListProjects operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListProjects
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listProjectsAsync(ListProjectsRequest listProjectsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all currently running remote access sessions.
*
*
* @param listRemoteAccessSessionsRequest
* Represents the request to return information about the remote access session.
* @return A Java Future containing the result of the ListRemoteAccessSessions operation returned by the service.
* @sample AWSDeviceFarmAsync.ListRemoteAccessSessions
* @see AWS API Documentation
*/
java.util.concurrent.Future listRemoteAccessSessionsAsync(ListRemoteAccessSessionsRequest listRemoteAccessSessionsRequest);
/**
*
* Returns a list of all currently running remote access sessions.
*
*
* @param listRemoteAccessSessionsRequest
* Represents the request to return information about the remote access session.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRemoteAccessSessions operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListRemoteAccessSessions
* @see AWS API Documentation
*/
java.util.concurrent.Future listRemoteAccessSessionsAsync(ListRemoteAccessSessionsRequest listRemoteAccessSessionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about runs, given an AWS Device Farm project ARN.
*
*
* @param listRunsRequest
* Represents a request to the list runs operation.
* @return A Java Future containing the result of the ListRuns operation returned by the service.
* @sample AWSDeviceFarmAsync.ListRuns
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listRunsAsync(ListRunsRequest listRunsRequest);
/**
*
* Gets information about runs, given an AWS Device Farm project ARN.
*
*
* @param listRunsRequest
* Represents a request to the list runs operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRuns operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListRuns
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listRunsAsync(ListRunsRequest listRunsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about samples, given an AWS Device Farm job ARN.
*
*
* @param listSamplesRequest
* Represents a request to the list samples operation.
* @return A Java Future containing the result of the ListSamples operation returned by the service.
* @sample AWSDeviceFarmAsync.ListSamples
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listSamplesAsync(ListSamplesRequest listSamplesRequest);
/**
*
* Gets information about samples, given an AWS Device Farm job ARN.
*
*
* @param listSamplesRequest
* Represents a request to the list samples operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSamples operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListSamples
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listSamplesAsync(ListSamplesRequest listSamplesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about test suites for a given job.
*
*
* @param listSuitesRequest
* Represents a request to the list suites operation.
* @return A Java Future containing the result of the ListSuites operation returned by the service.
* @sample AWSDeviceFarmAsync.ListSuites
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listSuitesAsync(ListSuitesRequest listSuitesRequest);
/**
*
* Gets information about test suites for a given job.
*
*
* @param listSuitesRequest
* Represents a request to the list suites operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSuites operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListSuites
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listSuitesAsync(ListSuitesRequest listSuitesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List the tags for an AWS Device Farm resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSDeviceFarmAsync.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* List the tags for an AWS Device Farm resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of all Selenium testing projects in your account.
*
*
* @param listTestGridProjectsRequest
* @return A Java Future containing the result of the ListTestGridProjects operation returned by the service.
* @sample AWSDeviceFarmAsync.ListTestGridProjects
* @see AWS API Documentation
*/
java.util.concurrent.Future listTestGridProjectsAsync(ListTestGridProjectsRequest listTestGridProjectsRequest);
/**
*
* Gets a list of all Selenium testing projects in your account.
*
*
* @param listTestGridProjectsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTestGridProjects operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListTestGridProjects
* @see AWS API Documentation
*/
java.util.concurrent.Future listTestGridProjectsAsync(ListTestGridProjectsRequest listTestGridProjectsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of the actions taken in a TestGridSession.
*
*
* @param listTestGridSessionActionsRequest
* @return A Java Future containing the result of the ListTestGridSessionActions operation returned by the service.
* @sample AWSDeviceFarmAsync.ListTestGridSessionActions
* @see AWS API Documentation
*/
java.util.concurrent.Future listTestGridSessionActionsAsync(
ListTestGridSessionActionsRequest listTestGridSessionActionsRequest);
/**
*
* Returns a list of the actions taken in a TestGridSession.
*
*
* @param listTestGridSessionActionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTestGridSessionActions operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListTestGridSessionActions
* @see AWS API Documentation
*/
java.util.concurrent.Future listTestGridSessionActionsAsync(
ListTestGridSessionActionsRequest listTestGridSessionActionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of artifacts created during the session.
*
*
* @param listTestGridSessionArtifactsRequest
* @return A Java Future containing the result of the ListTestGridSessionArtifacts operation returned by the
* service.
* @sample AWSDeviceFarmAsync.ListTestGridSessionArtifacts
* @see AWS API Documentation
*/
java.util.concurrent.Future listTestGridSessionArtifactsAsync(
ListTestGridSessionArtifactsRequest listTestGridSessionArtifactsRequest);
/**
*
* Retrieves a list of artifacts created during the session.
*
*
* @param listTestGridSessionArtifactsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTestGridSessionArtifacts operation returned by the
* service.
* @sample AWSDeviceFarmAsyncHandler.ListTestGridSessionArtifacts
* @see AWS API Documentation
*/
java.util.concurrent.Future listTestGridSessionArtifactsAsync(
ListTestGridSessionArtifactsRequest listTestGridSessionArtifactsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of sessions for a TestGridProject.
*
*
* @param listTestGridSessionsRequest
* @return A Java Future containing the result of the ListTestGridSessions operation returned by the service.
* @sample AWSDeviceFarmAsync.ListTestGridSessions
* @see AWS API Documentation
*/
java.util.concurrent.Future listTestGridSessionsAsync(ListTestGridSessionsRequest listTestGridSessionsRequest);
/**
*
* Retrieves a list of sessions for a TestGridProject.
*
*
* @param listTestGridSessionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTestGridSessions operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListTestGridSessions
* @see AWS API Documentation
*/
java.util.concurrent.Future listTestGridSessionsAsync(ListTestGridSessionsRequest listTestGridSessionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about tests in a given test suite.
*
*
* @param listTestsRequest
* Represents a request to the list tests operation.
* @return A Java Future containing the result of the ListTests operation returned by the service.
* @sample AWSDeviceFarmAsync.ListTests
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTestsAsync(ListTestsRequest listTestsRequest);
/**
*
* Gets information about tests in a given test suite.
*
*
* @param listTestsRequest
* Represents a request to the list tests operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTests operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListTests
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTestsAsync(ListTestsRequest listTestsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about unique problems, such as exceptions or crashes.
*
*
* Unique problems are defined as a single instance of an error across a run, job, or suite. For example, if a call
* in your application consistently raises an exception (OutOfBoundsException in MyActivity.java:386
),
* ListUniqueProblems
returns a single entry instead of many individual entries for that exception.
*
*
* @param listUniqueProblemsRequest
* Represents a request to the list unique problems operation.
* @return A Java Future containing the result of the ListUniqueProblems operation returned by the service.
* @sample AWSDeviceFarmAsync.ListUniqueProblems
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listUniqueProblemsAsync(ListUniqueProblemsRequest listUniqueProblemsRequest);
/**
*
* Gets information about unique problems, such as exceptions or crashes.
*
*
* Unique problems are defined as a single instance of an error across a run, job, or suite. For example, if a call
* in your application consistently raises an exception (OutOfBoundsException in MyActivity.java:386
),
* ListUniqueProblems
returns a single entry instead of many individual entries for that exception.
*
*
* @param listUniqueProblemsRequest
* Represents a request to the list unique problems operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListUniqueProblems operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListUniqueProblems
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listUniqueProblemsAsync(ListUniqueProblemsRequest listUniqueProblemsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about uploads, given an AWS Device Farm project ARN.
*
*
* @param listUploadsRequest
* Represents a request to the list uploads operation.
* @return A Java Future containing the result of the ListUploads operation returned by the service.
* @sample AWSDeviceFarmAsync.ListUploads
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listUploadsAsync(ListUploadsRequest listUploadsRequest);
/**
*
* Gets information about uploads, given an AWS Device Farm project ARN.
*
*
* @param listUploadsRequest
* Represents a request to the list uploads operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListUploads operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListUploads
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listUploadsAsync(ListUploadsRequest listUploadsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about all Amazon Virtual Private Cloud (VPC) endpoint configurations in the AWS account.
*
*
* @param listVPCEConfigurationsRequest
* @return A Java Future containing the result of the ListVPCEConfigurations operation returned by the service.
* @sample AWSDeviceFarmAsync.ListVPCEConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listVPCEConfigurationsAsync(ListVPCEConfigurationsRequest listVPCEConfigurationsRequest);
/**
*
* Returns information about all Amazon Virtual Private Cloud (VPC) endpoint configurations in the AWS account.
*
*
* @param listVPCEConfigurationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListVPCEConfigurations operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ListVPCEConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listVPCEConfigurationsAsync(ListVPCEConfigurationsRequest listVPCEConfigurationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Immediately purchases offerings for an AWS account. Offerings renew with the latest total purchased quantity for
* an offering, unless the renewal was overridden. The API returns a NotEligible
error if the user is
* not permitted to invoke the operation. If you must be able to invoke this operation, contact [email protected].
*
*
* @param purchaseOfferingRequest
* Represents a request for a purchase offering.
* @return A Java Future containing the result of the PurchaseOffering operation returned by the service.
* @sample AWSDeviceFarmAsync.PurchaseOffering
* @see AWS
* API Documentation
*/
java.util.concurrent.Future purchaseOfferingAsync(PurchaseOfferingRequest purchaseOfferingRequest);
/**
*
* Immediately purchases offerings for an AWS account. Offerings renew with the latest total purchased quantity for
* an offering, unless the renewal was overridden. The API returns a NotEligible
error if the user is
* not permitted to invoke the operation. If you must be able to invoke this operation, contact [email protected].
*
*
* @param purchaseOfferingRequest
* Represents a request for a purchase offering.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PurchaseOffering operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.PurchaseOffering
* @see AWS
* API Documentation
*/
java.util.concurrent.Future purchaseOfferingAsync(PurchaseOfferingRequest purchaseOfferingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Explicitly sets the quantity of devices to renew for an offering, starting from the effectiveDate
of
* the next period. The API returns a NotEligible
error if the user is not permitted to invoke the
* operation. If you must be able to invoke this operation, contact [email protected].
*
*
* @param renewOfferingRequest
* A request that represents an offering renewal.
* @return A Java Future containing the result of the RenewOffering operation returned by the service.
* @sample AWSDeviceFarmAsync.RenewOffering
* @see AWS API
* Documentation
*/
java.util.concurrent.Future renewOfferingAsync(RenewOfferingRequest renewOfferingRequest);
/**
*
* Explicitly sets the quantity of devices to renew for an offering, starting from the effectiveDate
of
* the next period. The API returns a NotEligible
error if the user is not permitted to invoke the
* operation. If you must be able to invoke this operation, contact [email protected].
*
*
* @param renewOfferingRequest
* A request that represents an offering renewal.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RenewOffering operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.RenewOffering
* @see AWS API
* Documentation
*/
java.util.concurrent.Future renewOfferingAsync(RenewOfferingRequest renewOfferingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Schedules a run.
*
*
* @param scheduleRunRequest
* Represents a request to the schedule run operation.
* @return A Java Future containing the result of the ScheduleRun operation returned by the service.
* @sample AWSDeviceFarmAsync.ScheduleRun
* @see AWS API
* Documentation
*/
java.util.concurrent.Future scheduleRunAsync(ScheduleRunRequest scheduleRunRequest);
/**
*
* Schedules a run.
*
*
* @param scheduleRunRequest
* Represents a request to the schedule run operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ScheduleRun operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.ScheduleRun
* @see AWS API
* Documentation
*/
java.util.concurrent.Future scheduleRunAsync(ScheduleRunRequest scheduleRunRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Initiates a stop request for the current job. AWS Device Farm immediately stops the job on the device where tests
* have not started. You are not billed for this device. On the device where tests have started, setup suite and
* teardown suite tests run to completion on the device. You are billed for setup, teardown, and any tests that were
* in progress or already completed.
*
*
* @param stopJobRequest
* @return A Java Future containing the result of the StopJob operation returned by the service.
* @sample AWSDeviceFarmAsync.StopJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopJobAsync(StopJobRequest stopJobRequest);
/**
*
* Initiates a stop request for the current job. AWS Device Farm immediately stops the job on the device where tests
* have not started. You are not billed for this device. On the device where tests have started, setup suite and
* teardown suite tests run to completion on the device. You are billed for setup, teardown, and any tests that were
* in progress or already completed.
*
*
* @param stopJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopJob operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.StopJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopJobAsync(StopJobRequest stopJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Ends a specified remote access session.
*
*
* @param stopRemoteAccessSessionRequest
* Represents the request to stop the remote access session.
* @return A Java Future containing the result of the StopRemoteAccessSession operation returned by the service.
* @sample AWSDeviceFarmAsync.StopRemoteAccessSession
* @see AWS API Documentation
*/
java.util.concurrent.Future stopRemoteAccessSessionAsync(StopRemoteAccessSessionRequest stopRemoteAccessSessionRequest);
/**
*
* Ends a specified remote access session.
*
*
* @param stopRemoteAccessSessionRequest
* Represents the request to stop the remote access session.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopRemoteAccessSession operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.StopRemoteAccessSession
* @see AWS API Documentation
*/
java.util.concurrent.Future stopRemoteAccessSessionAsync(StopRemoteAccessSessionRequest stopRemoteAccessSessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Initiates a stop request for the current test run. AWS Device Farm immediately stops the run on devices where
* tests have not started. You are not billed for these devices. On devices where tests have started executing,
* setup suite and teardown suite tests run to completion on those devices. You are billed for setup, teardown, and
* any tests that were in progress or already completed.
*
*
* @param stopRunRequest
* Represents the request to stop a specific run.
* @return A Java Future containing the result of the StopRun operation returned by the service.
* @sample AWSDeviceFarmAsync.StopRun
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopRunAsync(StopRunRequest stopRunRequest);
/**
*
* Initiates a stop request for the current test run. AWS Device Farm immediately stops the run on devices where
* tests have not started. You are not billed for these devices. On devices where tests have started executing,
* setup suite and teardown suite tests run to completion on those devices. You are billed for setup, teardown, and
* any tests that were in progress or already completed.
*
*
* @param stopRunRequest
* Represents the request to stop a specific run.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopRun operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.StopRun
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopRunAsync(StopRunRequest stopRunRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates the specified tags to a resource with the specified resourceArn
. If existing tags on a
* resource are not specified in the request parameters, they are not changed. When a resource is deleted, the tags
* associated with that resource are also deleted.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSDeviceFarmAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Associates the specified tags to a resource with the specified resourceArn
. If existing tags on a
* resource are not specified in the request parameters, they are not changed. When a resource is deleted, the tags
* associated with that resource are also deleted.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified tags from a resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSDeviceFarmAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Deletes the specified tags from a resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates information about a private device instance.
*
*
* @param updateDeviceInstanceRequest
* @return A Java Future containing the result of the UpdateDeviceInstance operation returned by the service.
* @sample AWSDeviceFarmAsync.UpdateDeviceInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDeviceInstanceAsync(UpdateDeviceInstanceRequest updateDeviceInstanceRequest);
/**
*
* Updates information about a private device instance.
*
*
* @param updateDeviceInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDeviceInstance operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.UpdateDeviceInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDeviceInstanceAsync(UpdateDeviceInstanceRequest updateDeviceInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the name, description, and rules in a device pool given the attributes and the pool ARN. Rule updates
* are all-or-nothing, meaning they can only be updated as a whole (or not at all).
*
*
* @param updateDevicePoolRequest
* Represents a request to the update device pool operation.
* @return A Java Future containing the result of the UpdateDevicePool operation returned by the service.
* @sample AWSDeviceFarmAsync.UpdateDevicePool
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateDevicePoolAsync(UpdateDevicePoolRequest updateDevicePoolRequest);
/**
*
* Modifies the name, description, and rules in a device pool given the attributes and the pool ARN. Rule updates
* are all-or-nothing, meaning they can only be updated as a whole (or not at all).
*
*
* @param updateDevicePoolRequest
* Represents a request to the update device pool operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDevicePool operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.UpdateDevicePool
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateDevicePoolAsync(UpdateDevicePoolRequest updateDevicePoolRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates information about an existing private device instance profile.
*
*
* @param updateInstanceProfileRequest
* @return A Java Future containing the result of the UpdateInstanceProfile operation returned by the service.
* @sample AWSDeviceFarmAsync.UpdateInstanceProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future updateInstanceProfileAsync(UpdateInstanceProfileRequest updateInstanceProfileRequest);
/**
*
* Updates information about an existing private device instance profile.
*
*
* @param updateInstanceProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateInstanceProfile operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.UpdateInstanceProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future updateInstanceProfileAsync(UpdateInstanceProfileRequest updateInstanceProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the network profile.
*
*
* @param updateNetworkProfileRequest
* @return A Java Future containing the result of the UpdateNetworkProfile operation returned by the service.
* @sample AWSDeviceFarmAsync.UpdateNetworkProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future updateNetworkProfileAsync(UpdateNetworkProfileRequest updateNetworkProfileRequest);
/**
*
* Updates the network profile.
*
*
* @param updateNetworkProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateNetworkProfile operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.UpdateNetworkProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future updateNetworkProfileAsync(UpdateNetworkProfileRequest updateNetworkProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the specified project name, given the project ARN and a new name.
*
*
* @param updateProjectRequest
* Represents a request to the update project operation.
* @return A Java Future containing the result of the UpdateProject operation returned by the service.
* @sample AWSDeviceFarmAsync.UpdateProject
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateProjectAsync(UpdateProjectRequest updateProjectRequest);
/**
*
* Modifies the specified project name, given the project ARN and a new name.
*
*
* @param updateProjectRequest
* Represents a request to the update project operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateProject operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.UpdateProject
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateProjectAsync(UpdateProjectRequest updateProjectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Change details of a project.
*
*
* @param updateTestGridProjectRequest
* @return A Java Future containing the result of the UpdateTestGridProject operation returned by the service.
* @sample AWSDeviceFarmAsync.UpdateTestGridProject
* @see AWS API Documentation
*/
java.util.concurrent.Future updateTestGridProjectAsync(UpdateTestGridProjectRequest updateTestGridProjectRequest);
/**
*
* Change details of a project.
*
*
* @param updateTestGridProjectRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateTestGridProject operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.UpdateTestGridProject
* @see AWS API Documentation
*/
java.util.concurrent.Future updateTestGridProjectAsync(UpdateTestGridProjectRequest updateTestGridProjectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an uploaded test spec.
*
*
* @param updateUploadRequest
* @return A Java Future containing the result of the UpdateUpload operation returned by the service.
* @sample AWSDeviceFarmAsync.UpdateUpload
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateUploadAsync(UpdateUploadRequest updateUploadRequest);
/**
*
* Updates an uploaded test spec.
*
*
* @param updateUploadRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateUpload operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.UpdateUpload
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateUploadAsync(UpdateUploadRequest updateUploadRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates information about an Amazon Virtual Private Cloud (VPC) endpoint configuration.
*
*
* @param updateVPCEConfigurationRequest
* @return A Java Future containing the result of the UpdateVPCEConfiguration operation returned by the service.
* @sample AWSDeviceFarmAsync.UpdateVPCEConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateVPCEConfigurationAsync(UpdateVPCEConfigurationRequest updateVPCEConfigurationRequest);
/**
*
* Updates information about an Amazon Virtual Private Cloud (VPC) endpoint configuration.
*
*
* @param updateVPCEConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateVPCEConfiguration operation returned by the service.
* @sample AWSDeviceFarmAsyncHandler.UpdateVPCEConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateVPCEConfigurationAsync(UpdateVPCEConfigurationRequest updateVPCEConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}