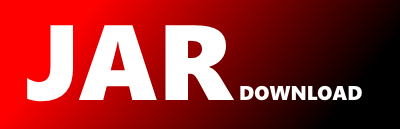
com.amazonaws.services.directconnect.AmazonDirectConnectAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-directconnect Show documentation
/*
* Copyright 2011-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.directconnect;
import com.amazonaws.services.directconnect.model.*;
/**
* Interface for accessing AWS Direct Connect asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.directconnect.AbstractAmazonDirectConnectAsync} instead.
*
*
*
* AWS Direct Connect links your internal network to an AWS Direct Connect location over a standard 1 gigabit or 10
* gigabit Ethernet fiber-optic cable. One end of the cable is connected to your router, the other to an AWS Direct
* Connect router. With this connection in place, you can create virtual interfaces directly to the AWS cloud (for
* example, to Amazon Elastic Compute Cloud (Amazon EC2) and Amazon Simple Storage Service (Amazon S3)) and to Amazon
* Virtual Private Cloud (Amazon VPC), bypassing Internet service providers in your network path. An AWS Direct Connect
* location provides access to AWS in the region it is associated with, as well as access to other US regions. For
* example, you can provision a single connection to any AWS Direct Connect location in the US and use it to access
* public AWS services in all US Regions and AWS GovCloud (US).
*
*/
public interface AmazonDirectConnectAsync extends AmazonDirectConnect {
/**
*
* Creates a hosted connection on an interconnect.
*
*
* Allocates a VLAN number and a specified amount of bandwidth for use by a hosted connection on the given
* interconnect.
*
*
*
* This is intended for use by AWS Direct Connect partners only.
*
*
*
* @param allocateConnectionOnInterconnectRequest
* Container for the parameters to the AllocateConnectionOnInterconnect operation.
* @return A Java Future containing the result of the AllocateConnectionOnInterconnect operation returned by the
* service.
* @sample AmazonDirectConnectAsync.AllocateConnectionOnInterconnect
*/
java.util.concurrent.Future allocateConnectionOnInterconnectAsync(
AllocateConnectionOnInterconnectRequest allocateConnectionOnInterconnectRequest);
/**
*
* Creates a hosted connection on an interconnect.
*
*
* Allocates a VLAN number and a specified amount of bandwidth for use by a hosted connection on the given
* interconnect.
*
*
*
* This is intended for use by AWS Direct Connect partners only.
*
*
*
* @param allocateConnectionOnInterconnectRequest
* Container for the parameters to the AllocateConnectionOnInterconnect operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AllocateConnectionOnInterconnect operation returned by the
* service.
* @sample AmazonDirectConnectAsyncHandler.AllocateConnectionOnInterconnect
*/
java.util.concurrent.Future allocateConnectionOnInterconnectAsync(
AllocateConnectionOnInterconnectRequest allocateConnectionOnInterconnectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provisions a private virtual interface to be owned by a different customer.
*
*
* The owner of a connection calls this function to provision a private virtual interface which will be owned by
* another AWS customer.
*
*
* Virtual interfaces created using this function must be confirmed by the virtual interface owner by calling
* ConfirmPrivateVirtualInterface. Until this step has been completed, the virtual interface will be in 'Confirming'
* state, and will not be available for handling traffic.
*
*
* @param allocatePrivateVirtualInterfaceRequest
* Container for the parameters to the AllocatePrivateVirtualInterface operation.
* @return A Java Future containing the result of the AllocatePrivateVirtualInterface operation returned by the
* service.
* @sample AmazonDirectConnectAsync.AllocatePrivateVirtualInterface
*/
java.util.concurrent.Future allocatePrivateVirtualInterfaceAsync(
AllocatePrivateVirtualInterfaceRequest allocatePrivateVirtualInterfaceRequest);
/**
*
* Provisions a private virtual interface to be owned by a different customer.
*
*
* The owner of a connection calls this function to provision a private virtual interface which will be owned by
* another AWS customer.
*
*
* Virtual interfaces created using this function must be confirmed by the virtual interface owner by calling
* ConfirmPrivateVirtualInterface. Until this step has been completed, the virtual interface will be in 'Confirming'
* state, and will not be available for handling traffic.
*
*
* @param allocatePrivateVirtualInterfaceRequest
* Container for the parameters to the AllocatePrivateVirtualInterface operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AllocatePrivateVirtualInterface operation returned by the
* service.
* @sample AmazonDirectConnectAsyncHandler.AllocatePrivateVirtualInterface
*/
java.util.concurrent.Future allocatePrivateVirtualInterfaceAsync(
AllocatePrivateVirtualInterfaceRequest allocatePrivateVirtualInterfaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provisions a public virtual interface to be owned by a different customer.
*
*
* The owner of a connection calls this function to provision a public virtual interface which will be owned by
* another AWS customer.
*
*
* Virtual interfaces created using this function must be confirmed by the virtual interface owner by calling
* ConfirmPublicVirtualInterface. Until this step has been completed, the virtual interface will be in 'Confirming'
* state, and will not be available for handling traffic.
*
*
* @param allocatePublicVirtualInterfaceRequest
* Container for the parameters to the AllocatePublicVirtualInterface operation.
* @return A Java Future containing the result of the AllocatePublicVirtualInterface operation returned by the
* service.
* @sample AmazonDirectConnectAsync.AllocatePublicVirtualInterface
*/
java.util.concurrent.Future allocatePublicVirtualInterfaceAsync(
AllocatePublicVirtualInterfaceRequest allocatePublicVirtualInterfaceRequest);
/**
*
* Provisions a public virtual interface to be owned by a different customer.
*
*
* The owner of a connection calls this function to provision a public virtual interface which will be owned by
* another AWS customer.
*
*
* Virtual interfaces created using this function must be confirmed by the virtual interface owner by calling
* ConfirmPublicVirtualInterface. Until this step has been completed, the virtual interface will be in 'Confirming'
* state, and will not be available for handling traffic.
*
*
* @param allocatePublicVirtualInterfaceRequest
* Container for the parameters to the AllocatePublicVirtualInterface operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AllocatePublicVirtualInterface operation returned by the
* service.
* @sample AmazonDirectConnectAsyncHandler.AllocatePublicVirtualInterface
*/
java.util.concurrent.Future allocatePublicVirtualInterfaceAsync(
AllocatePublicVirtualInterfaceRequest allocatePublicVirtualInterfaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Confirm the creation of a hosted connection on an interconnect.
*
*
* Upon creation, the hosted connection is initially in the 'Ordering' state, and will remain in this state until
* the owner calls ConfirmConnection to confirm creation of the hosted connection.
*
*
* @param confirmConnectionRequest
* Container for the parameters to the ConfirmConnection operation.
* @return A Java Future containing the result of the ConfirmConnection operation returned by the service.
* @sample AmazonDirectConnectAsync.ConfirmConnection
*/
java.util.concurrent.Future confirmConnectionAsync(ConfirmConnectionRequest confirmConnectionRequest);
/**
*
* Confirm the creation of a hosted connection on an interconnect.
*
*
* Upon creation, the hosted connection is initially in the 'Ordering' state, and will remain in this state until
* the owner calls ConfirmConnection to confirm creation of the hosted connection.
*
*
* @param confirmConnectionRequest
* Container for the parameters to the ConfirmConnection operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ConfirmConnection operation returned by the service.
* @sample AmazonDirectConnectAsyncHandler.ConfirmConnection
*/
java.util.concurrent.Future confirmConnectionAsync(ConfirmConnectionRequest confirmConnectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Accept ownership of a private virtual interface created by another customer.
*
*
* After the virtual interface owner calls this function, the virtual interface will be created and attached to the
* given virtual private gateway, and will be available for handling traffic.
*
*
* @param confirmPrivateVirtualInterfaceRequest
* Container for the parameters to the ConfirmPrivateVirtualInterface operation.
* @return A Java Future containing the result of the ConfirmPrivateVirtualInterface operation returned by the
* service.
* @sample AmazonDirectConnectAsync.ConfirmPrivateVirtualInterface
*/
java.util.concurrent.Future confirmPrivateVirtualInterfaceAsync(
ConfirmPrivateVirtualInterfaceRequest confirmPrivateVirtualInterfaceRequest);
/**
*
* Accept ownership of a private virtual interface created by another customer.
*
*
* After the virtual interface owner calls this function, the virtual interface will be created and attached to the
* given virtual private gateway, and will be available for handling traffic.
*
*
* @param confirmPrivateVirtualInterfaceRequest
* Container for the parameters to the ConfirmPrivateVirtualInterface operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ConfirmPrivateVirtualInterface operation returned by the
* service.
* @sample AmazonDirectConnectAsyncHandler.ConfirmPrivateVirtualInterface
*/
java.util.concurrent.Future confirmPrivateVirtualInterfaceAsync(
ConfirmPrivateVirtualInterfaceRequest confirmPrivateVirtualInterfaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Accept ownership of a public virtual interface created by another customer.
*
*
* After the virtual interface owner calls this function, the specified virtual interface will be created and made
* available for handling traffic.
*
*
* @param confirmPublicVirtualInterfaceRequest
* Container for the parameters to the ConfirmPublicVirtualInterface operation.
* @return A Java Future containing the result of the ConfirmPublicVirtualInterface operation returned by the
* service.
* @sample AmazonDirectConnectAsync.ConfirmPublicVirtualInterface
*/
java.util.concurrent.Future confirmPublicVirtualInterfaceAsync(
ConfirmPublicVirtualInterfaceRequest confirmPublicVirtualInterfaceRequest);
/**
*
* Accept ownership of a public virtual interface created by another customer.
*
*
* After the virtual interface owner calls this function, the specified virtual interface will be created and made
* available for handling traffic.
*
*
* @param confirmPublicVirtualInterfaceRequest
* Container for the parameters to the ConfirmPublicVirtualInterface operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ConfirmPublicVirtualInterface operation returned by the
* service.
* @sample AmazonDirectConnectAsyncHandler.ConfirmPublicVirtualInterface
*/
java.util.concurrent.Future confirmPublicVirtualInterfaceAsync(
ConfirmPublicVirtualInterfaceRequest confirmPublicVirtualInterfaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new connection between the customer network and a specific AWS Direct Connect location.
*
*
* A connection links your internal network to an AWS Direct Connect location over a standard 1 gigabit or 10
* gigabit Ethernet fiber-optic cable. One end of the cable is connected to your router, the other to an AWS Direct
* Connect router. An AWS Direct Connect location provides access to Amazon Web Services in the region it is
* associated with. You can establish connections with AWS Direct Connect locations in multiple regions, but a
* connection in one region does not provide connectivity to other regions.
*
*
* @param createConnectionRequest
* Container for the parameters to the CreateConnection operation.
* @return A Java Future containing the result of the CreateConnection operation returned by the service.
* @sample AmazonDirectConnectAsync.CreateConnection
*/
java.util.concurrent.Future createConnectionAsync(CreateConnectionRequest createConnectionRequest);
/**
*
* Creates a new connection between the customer network and a specific AWS Direct Connect location.
*
*
* A connection links your internal network to an AWS Direct Connect location over a standard 1 gigabit or 10
* gigabit Ethernet fiber-optic cable. One end of the cable is connected to your router, the other to an AWS Direct
* Connect router. An AWS Direct Connect location provides access to Amazon Web Services in the region it is
* associated with. You can establish connections with AWS Direct Connect locations in multiple regions, but a
* connection in one region does not provide connectivity to other regions.
*
*
* @param createConnectionRequest
* Container for the parameters to the CreateConnection operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateConnection operation returned by the service.
* @sample AmazonDirectConnectAsyncHandler.CreateConnection
*/
java.util.concurrent.Future createConnectionAsync(CreateConnectionRequest createConnectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new interconnect between a AWS Direct Connect partner's network and a specific AWS Direct Connect
* location.
*
*
* An interconnect is a connection which is capable of hosting other connections. The AWS Direct Connect partner can
* use an interconnect to provide sub-1Gbps AWS Direct Connect service to tier 2 customers who do not have their own
* connections. Like a standard connection, an interconnect links the AWS Direct Connect partner's network to an AWS
* Direct Connect location over a standard 1 Gbps or 10 Gbps Ethernet fiber-optic cable. One end is connected to the
* partner's router, the other to an AWS Direct Connect router.
*
*
* For each end customer, the AWS Direct Connect partner provisions a connection on their interconnect by calling
* AllocateConnectionOnInterconnect. The end customer can then connect to AWS resources by creating a virtual
* interface on their connection, using the VLAN assigned to them by the AWS Direct Connect partner.
*
*
*
* This is intended for use by AWS Direct Connect partners only.
*
*
*
* @param createInterconnectRequest
* Container for the parameters to the CreateInterconnect operation.
* @return A Java Future containing the result of the CreateInterconnect operation returned by the service.
* @sample AmazonDirectConnectAsync.CreateInterconnect
*/
java.util.concurrent.Future createInterconnectAsync(CreateInterconnectRequest createInterconnectRequest);
/**
*
* Creates a new interconnect between a AWS Direct Connect partner's network and a specific AWS Direct Connect
* location.
*
*
* An interconnect is a connection which is capable of hosting other connections. The AWS Direct Connect partner can
* use an interconnect to provide sub-1Gbps AWS Direct Connect service to tier 2 customers who do not have their own
* connections. Like a standard connection, an interconnect links the AWS Direct Connect partner's network to an AWS
* Direct Connect location over a standard 1 Gbps or 10 Gbps Ethernet fiber-optic cable. One end is connected to the
* partner's router, the other to an AWS Direct Connect router.
*
*
* For each end customer, the AWS Direct Connect partner provisions a connection on their interconnect by calling
* AllocateConnectionOnInterconnect. The end customer can then connect to AWS resources by creating a virtual
* interface on their connection, using the VLAN assigned to them by the AWS Direct Connect partner.
*
*
*
* This is intended for use by AWS Direct Connect partners only.
*
*
*
* @param createInterconnectRequest
* Container for the parameters to the CreateInterconnect operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateInterconnect operation returned by the service.
* @sample AmazonDirectConnectAsyncHandler.CreateInterconnect
*/
java.util.concurrent.Future createInterconnectAsync(CreateInterconnectRequest createInterconnectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new private virtual interface. A virtual interface is the VLAN that transports AWS Direct Connect
* traffic. A private virtual interface supports sending traffic to a single virtual private cloud (VPC).
*
*
* @param createPrivateVirtualInterfaceRequest
* Container for the parameters to the CreatePrivateVirtualInterface operation.
* @return A Java Future containing the result of the CreatePrivateVirtualInterface operation returned by the
* service.
* @sample AmazonDirectConnectAsync.CreatePrivateVirtualInterface
*/
java.util.concurrent.Future createPrivateVirtualInterfaceAsync(
CreatePrivateVirtualInterfaceRequest createPrivateVirtualInterfaceRequest);
/**
*
* Creates a new private virtual interface. A virtual interface is the VLAN that transports AWS Direct Connect
* traffic. A private virtual interface supports sending traffic to a single virtual private cloud (VPC).
*
*
* @param createPrivateVirtualInterfaceRequest
* Container for the parameters to the CreatePrivateVirtualInterface operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePrivateVirtualInterface operation returned by the
* service.
* @sample AmazonDirectConnectAsyncHandler.CreatePrivateVirtualInterface
*/
java.util.concurrent.Future createPrivateVirtualInterfaceAsync(
CreatePrivateVirtualInterfaceRequest createPrivateVirtualInterfaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new public virtual interface. A virtual interface is the VLAN that transports AWS Direct Connect
* traffic. A public virtual interface supports sending traffic to public services of AWS such as Amazon Simple
* Storage Service (Amazon S3).
*
*
* @param createPublicVirtualInterfaceRequest
* Container for the parameters to the CreatePublicVirtualInterface operation.
* @return A Java Future containing the result of the CreatePublicVirtualInterface operation returned by the
* service.
* @sample AmazonDirectConnectAsync.CreatePublicVirtualInterface
*/
java.util.concurrent.Future createPublicVirtualInterfaceAsync(
CreatePublicVirtualInterfaceRequest createPublicVirtualInterfaceRequest);
/**
*
* Creates a new public virtual interface. A virtual interface is the VLAN that transports AWS Direct Connect
* traffic. A public virtual interface supports sending traffic to public services of AWS such as Amazon Simple
* Storage Service (Amazon S3).
*
*
* @param createPublicVirtualInterfaceRequest
* Container for the parameters to the CreatePublicVirtualInterface operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePublicVirtualInterface operation returned by the
* service.
* @sample AmazonDirectConnectAsyncHandler.CreatePublicVirtualInterface
*/
java.util.concurrent.Future createPublicVirtualInterfaceAsync(
CreatePublicVirtualInterfaceRequest createPublicVirtualInterfaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the connection.
*
*
* Deleting a connection only stops the AWS Direct Connect port hour and data transfer charges. You need to cancel
* separately with the providers any services or charges for cross-connects or network circuits that connect you to
* the AWS Direct Connect location.
*
*
* @param deleteConnectionRequest
* Container for the parameters to the DeleteConnection operation.
* @return A Java Future containing the result of the DeleteConnection operation returned by the service.
* @sample AmazonDirectConnectAsync.DeleteConnection
*/
java.util.concurrent.Future deleteConnectionAsync(DeleteConnectionRequest deleteConnectionRequest);
/**
*
* Deletes the connection.
*
*
* Deleting a connection only stops the AWS Direct Connect port hour and data transfer charges. You need to cancel
* separately with the providers any services or charges for cross-connects or network circuits that connect you to
* the AWS Direct Connect location.
*
*
* @param deleteConnectionRequest
* Container for the parameters to the DeleteConnection operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteConnection operation returned by the service.
* @sample AmazonDirectConnectAsyncHandler.DeleteConnection
*/
java.util.concurrent.Future deleteConnectionAsync(DeleteConnectionRequest deleteConnectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified interconnect.
*
*
*
* This is intended for use by AWS Direct Connect partners only.
*
*
*
* @param deleteInterconnectRequest
* Container for the parameters to the DeleteInterconnect operation.
* @return A Java Future containing the result of the DeleteInterconnect operation returned by the service.
* @sample AmazonDirectConnectAsync.DeleteInterconnect
*/
java.util.concurrent.Future deleteInterconnectAsync(DeleteInterconnectRequest deleteInterconnectRequest);
/**
*
* Deletes the specified interconnect.
*
*
*
* This is intended for use by AWS Direct Connect partners only.
*
*
*
* @param deleteInterconnectRequest
* Container for the parameters to the DeleteInterconnect operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteInterconnect operation returned by the service.
* @sample AmazonDirectConnectAsyncHandler.DeleteInterconnect
*/
java.util.concurrent.Future deleteInterconnectAsync(DeleteInterconnectRequest deleteInterconnectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a virtual interface.
*
*
* @param deleteVirtualInterfaceRequest
* Container for the parameters to the DeleteVirtualInterface operation.
* @return A Java Future containing the result of the DeleteVirtualInterface operation returned by the service.
* @sample AmazonDirectConnectAsync.DeleteVirtualInterface
*/
java.util.concurrent.Future deleteVirtualInterfaceAsync(DeleteVirtualInterfaceRequest deleteVirtualInterfaceRequest);
/**
*
* Deletes a virtual interface.
*
*
* @param deleteVirtualInterfaceRequest
* Container for the parameters to the DeleteVirtualInterface operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVirtualInterface operation returned by the service.
* @sample AmazonDirectConnectAsyncHandler.DeleteVirtualInterface
*/
java.util.concurrent.Future deleteVirtualInterfaceAsync(DeleteVirtualInterfaceRequest deleteVirtualInterfaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the LOA-CFA for a Connection.
*
*
* The Letter of Authorization - Connecting Facility Assignment (LOA-CFA) is a document that your APN partner or
* service provider uses when establishing your cross connect to AWS at the colocation facility. For more
* information, see Requesting
* Cross Connects at AWS Direct Connect Locations in the AWS Direct Connect user guide.
*
*
* @param describeConnectionLoaRequest
* Container for the parameters to the DescribeConnectionLoa operation.
* @return A Java Future containing the result of the DescribeConnectionLoa operation returned by the service.
* @sample AmazonDirectConnectAsync.DescribeConnectionLoa
*/
java.util.concurrent.Future describeConnectionLoaAsync(DescribeConnectionLoaRequest describeConnectionLoaRequest);
/**
*
* Returns the LOA-CFA for a Connection.
*
*
* The Letter of Authorization - Connecting Facility Assignment (LOA-CFA) is a document that your APN partner or
* service provider uses when establishing your cross connect to AWS at the colocation facility. For more
* information, see Requesting
* Cross Connects at AWS Direct Connect Locations in the AWS Direct Connect user guide.
*
*
* @param describeConnectionLoaRequest
* Container for the parameters to the DescribeConnectionLoa operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeConnectionLoa operation returned by the service.
* @sample AmazonDirectConnectAsyncHandler.DescribeConnectionLoa
*/
java.util.concurrent.Future describeConnectionLoaAsync(DescribeConnectionLoaRequest describeConnectionLoaRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Displays all connections in this region.
*
*
* If a connection ID is provided, the call returns only that particular connection.
*
*
* @param describeConnectionsRequest
* Container for the parameters to the DescribeConnections operation.
* @return A Java Future containing the result of the DescribeConnections operation returned by the service.
* @sample AmazonDirectConnectAsync.DescribeConnections
*/
java.util.concurrent.Future describeConnectionsAsync(DescribeConnectionsRequest describeConnectionsRequest);
/**
*
* Displays all connections in this region.
*
*
* If a connection ID is provided, the call returns only that particular connection.
*
*
* @param describeConnectionsRequest
* Container for the parameters to the DescribeConnections operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeConnections operation returned by the service.
* @sample AmazonDirectConnectAsyncHandler.DescribeConnections
*/
java.util.concurrent.Future describeConnectionsAsync(DescribeConnectionsRequest describeConnectionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeConnections operation.
*
* @see #describeConnectionsAsync(DescribeConnectionsRequest)
*/
java.util.concurrent.Future describeConnectionsAsync();
/**
* Simplified method form for invoking the DescribeConnections operation with an AsyncHandler.
*
* @see #describeConnectionsAsync(DescribeConnectionsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeConnectionsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Return a list of connections that have been provisioned on the given interconnect.
*
*
*
* This is intended for use by AWS Direct Connect partners only.
*
*
*
* @param describeConnectionsOnInterconnectRequest
* Container for the parameters to the DescribeConnectionsOnInterconnect operation.
* @return A Java Future containing the result of the DescribeConnectionsOnInterconnect operation returned by the
* service.
* @sample AmazonDirectConnectAsync.DescribeConnectionsOnInterconnect
*/
java.util.concurrent.Future describeConnectionsOnInterconnectAsync(
DescribeConnectionsOnInterconnectRequest describeConnectionsOnInterconnectRequest);
/**
*
* Return a list of connections that have been provisioned on the given interconnect.
*
*
*
* This is intended for use by AWS Direct Connect partners only.
*
*
*
* @param describeConnectionsOnInterconnectRequest
* Container for the parameters to the DescribeConnectionsOnInterconnect operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeConnectionsOnInterconnect operation returned by the
* service.
* @sample AmazonDirectConnectAsyncHandler.DescribeConnectionsOnInterconnect
*/
java.util.concurrent.Future describeConnectionsOnInterconnectAsync(
DescribeConnectionsOnInterconnectRequest describeConnectionsOnInterconnectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the LOA-CFA for an Interconnect.
*
*
* The Letter of Authorization - Connecting Facility Assignment (LOA-CFA) is a document that is used when
* establishing your cross connect to AWS at the colocation facility. For more information, see Requesting Cross Connects at AWS
* Direct Connect Locations in the AWS Direct Connect user guide.
*
*
* @param describeInterconnectLoaRequest
* Container for the parameters to the DescribeInterconnectLoa operation.
* @return A Java Future containing the result of the DescribeInterconnectLoa operation returned by the service.
* @sample AmazonDirectConnectAsync.DescribeInterconnectLoa
*/
java.util.concurrent.Future describeInterconnectLoaAsync(DescribeInterconnectLoaRequest describeInterconnectLoaRequest);
/**
*
* Returns the LOA-CFA for an Interconnect.
*
*
* The Letter of Authorization - Connecting Facility Assignment (LOA-CFA) is a document that is used when
* establishing your cross connect to AWS at the colocation facility. For more information, see Requesting Cross Connects at AWS
* Direct Connect Locations in the AWS Direct Connect user guide.
*
*
* @param describeInterconnectLoaRequest
* Container for the parameters to the DescribeInterconnectLoa operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeInterconnectLoa operation returned by the service.
* @sample AmazonDirectConnectAsyncHandler.DescribeInterconnectLoa
*/
java.util.concurrent.Future describeInterconnectLoaAsync(DescribeInterconnectLoaRequest describeInterconnectLoaRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of interconnects owned by the AWS account.
*
*
* If an interconnect ID is provided, it will only return this particular interconnect.
*
*
* @param describeInterconnectsRequest
* Container for the parameters to the DescribeInterconnects operation.
* @return A Java Future containing the result of the DescribeInterconnects operation returned by the service.
* @sample AmazonDirectConnectAsync.DescribeInterconnects
*/
java.util.concurrent.Future describeInterconnectsAsync(DescribeInterconnectsRequest describeInterconnectsRequest);
/**
*
* Returns a list of interconnects owned by the AWS account.
*
*
* If an interconnect ID is provided, it will only return this particular interconnect.
*
*
* @param describeInterconnectsRequest
* Container for the parameters to the DescribeInterconnects operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeInterconnects operation returned by the service.
* @sample AmazonDirectConnectAsyncHandler.DescribeInterconnects
*/
java.util.concurrent.Future describeInterconnectsAsync(DescribeInterconnectsRequest describeInterconnectsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeInterconnects operation.
*
* @see #describeInterconnectsAsync(DescribeInterconnectsRequest)
*/
java.util.concurrent.Future describeInterconnectsAsync();
/**
* Simplified method form for invoking the DescribeInterconnects operation with an AsyncHandler.
*
* @see #describeInterconnectsAsync(DescribeInterconnectsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeInterconnectsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the list of AWS Direct Connect locations in the current AWS region. These are the locations that may be
* selected when calling CreateConnection or CreateInterconnect.
*
*
* @param describeLocationsRequest
* @return A Java Future containing the result of the DescribeLocations operation returned by the service.
* @sample AmazonDirectConnectAsync.DescribeLocations
*/
java.util.concurrent.Future describeLocationsAsync(DescribeLocationsRequest describeLocationsRequest);
/**
*
* Returns the list of AWS Direct Connect locations in the current AWS region. These are the locations that may be
* selected when calling CreateConnection or CreateInterconnect.
*
*
* @param describeLocationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeLocations operation returned by the service.
* @sample AmazonDirectConnectAsyncHandler.DescribeLocations
*/
java.util.concurrent.Future describeLocationsAsync(DescribeLocationsRequest describeLocationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeLocations operation.
*
* @see #describeLocationsAsync(DescribeLocationsRequest)
*/
java.util.concurrent.Future describeLocationsAsync();
/**
* Simplified method form for invoking the DescribeLocations operation with an AsyncHandler.
*
* @see #describeLocationsAsync(DescribeLocationsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeLocationsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the tags associated with the specified Direct Connect resources.
*
*
* @param describeTagsRequest
* Container for the parameters to the DescribeTags operation.
* @return A Java Future containing the result of the DescribeTags operation returned by the service.
* @sample AmazonDirectConnectAsync.DescribeTags
*/
java.util.concurrent.Future describeTagsAsync(DescribeTagsRequest describeTagsRequest);
/**
*
* Describes the tags associated with the specified Direct Connect resources.
*
*
* @param describeTagsRequest
* Container for the parameters to the DescribeTags operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTags operation returned by the service.
* @sample AmazonDirectConnectAsyncHandler.DescribeTags
*/
java.util.concurrent.Future describeTagsAsync(DescribeTagsRequest describeTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of virtual private gateways owned by the AWS account.
*
*
* You can create one or more AWS Direct Connect private virtual interfaces linking to a virtual private gateway. A
* virtual private gateway can be managed via Amazon Virtual Private Cloud (VPC) console or the EC2
* CreateVpnGateway action.
*
*
* @param describeVirtualGatewaysRequest
* @return A Java Future containing the result of the DescribeVirtualGateways operation returned by the service.
* @sample AmazonDirectConnectAsync.DescribeVirtualGateways
*/
java.util.concurrent.Future describeVirtualGatewaysAsync(DescribeVirtualGatewaysRequest describeVirtualGatewaysRequest);
/**
*
* Returns a list of virtual private gateways owned by the AWS account.
*
*
* You can create one or more AWS Direct Connect private virtual interfaces linking to a virtual private gateway. A
* virtual private gateway can be managed via Amazon Virtual Private Cloud (VPC) console or the EC2
* CreateVpnGateway action.
*
*
* @param describeVirtualGatewaysRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeVirtualGateways operation returned by the service.
* @sample AmazonDirectConnectAsyncHandler.DescribeVirtualGateways
*/
java.util.concurrent.Future describeVirtualGatewaysAsync(DescribeVirtualGatewaysRequest describeVirtualGatewaysRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeVirtualGateways operation.
*
* @see #describeVirtualGatewaysAsync(DescribeVirtualGatewaysRequest)
*/
java.util.concurrent.Future describeVirtualGatewaysAsync();
/**
* Simplified method form for invoking the DescribeVirtualGateways operation with an AsyncHandler.
*
* @see #describeVirtualGatewaysAsync(DescribeVirtualGatewaysRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeVirtualGatewaysAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Displays all virtual interfaces for an AWS account. Virtual interfaces deleted fewer than 15 minutes before
* DescribeVirtualInterfaces is called are also returned. If a connection ID is included then only virtual
* interfaces associated with this connection will be returned. If a virtual interface ID is included then only a
* single virtual interface will be returned.
*
*
* A virtual interface (VLAN) transmits the traffic between the AWS Direct Connect location and the customer.
*
*
* If a connection ID is provided, only virtual interfaces provisioned on the specified connection will be returned.
* If a virtual interface ID is provided, only this particular virtual interface will be returned.
*
*
* @param describeVirtualInterfacesRequest
* Container for the parameters to the DescribeVirtualInterfaces operation.
* @return A Java Future containing the result of the DescribeVirtualInterfaces operation returned by the service.
* @sample AmazonDirectConnectAsync.DescribeVirtualInterfaces
*/
java.util.concurrent.Future describeVirtualInterfacesAsync(
DescribeVirtualInterfacesRequest describeVirtualInterfacesRequest);
/**
*
* Displays all virtual interfaces for an AWS account. Virtual interfaces deleted fewer than 15 minutes before
* DescribeVirtualInterfaces is called are also returned. If a connection ID is included then only virtual
* interfaces associated with this connection will be returned. If a virtual interface ID is included then only a
* single virtual interface will be returned.
*
*
* A virtual interface (VLAN) transmits the traffic between the AWS Direct Connect location and the customer.
*
*
* If a connection ID is provided, only virtual interfaces provisioned on the specified connection will be returned.
* If a virtual interface ID is provided, only this particular virtual interface will be returned.
*
*
* @param describeVirtualInterfacesRequest
* Container for the parameters to the DescribeVirtualInterfaces operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeVirtualInterfaces operation returned by the service.
* @sample AmazonDirectConnectAsyncHandler.DescribeVirtualInterfaces
*/
java.util.concurrent.Future describeVirtualInterfacesAsync(
DescribeVirtualInterfacesRequest describeVirtualInterfacesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeVirtualInterfaces operation.
*
* @see #describeVirtualInterfacesAsync(DescribeVirtualInterfacesRequest)
*/
java.util.concurrent.Future describeVirtualInterfacesAsync();
/**
* Simplified method form for invoking the DescribeVirtualInterfaces operation with an AsyncHandler.
*
* @see #describeVirtualInterfacesAsync(DescribeVirtualInterfacesRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeVirtualInterfacesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds the specified tags to the specified Direct Connect resource. Each Direct Connect resource can have a maximum
* of 50 tags.
*
*
* Each tag consists of a key and an optional value. If a tag with the same key is already associated with the
* Direct Connect resource, this action updates its value.
*
*
* @param tagResourceRequest
* Container for the parameters to the TagResource operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonDirectConnectAsync.TagResource
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds the specified tags to the specified Direct Connect resource. Each Direct Connect resource can have a maximum
* of 50 tags.
*
*
* Each tag consists of a key and an optional value. If a tag with the same key is already associated with the
* Direct Connect resource, this action updates its value.
*
*
* @param tagResourceRequest
* Container for the parameters to the TagResource operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonDirectConnectAsyncHandler.TagResource
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes one or more tags from the specified Direct Connect resource.
*
*
* @param untagResourceRequest
* Container for the parameters to the UntagResource operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonDirectConnectAsync.UntagResource
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes one or more tags from the specified Direct Connect resource.
*
*
* @param untagResourceRequest
* Container for the parameters to the UntagResource operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonDirectConnectAsyncHandler.UntagResource
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}