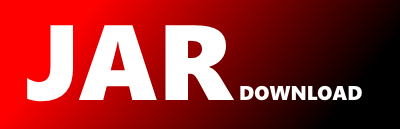
com.amazonaws.services.directconnect.model.AllocatePrivateVirtualInterfaceResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-directconnect Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.directconnect.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Information about a virtual interface.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AllocatePrivateVirtualInterfaceResult extends com.amazonaws.AmazonWebServiceResult implements Serializable,
Cloneable {
/**
*
* The ID of the Amazon Web Services account that owns the virtual interface.
*
*/
private String ownerAccount;
/**
*
* The ID of the virtual interface.
*
*/
private String virtualInterfaceId;
/**
*
* The location of the connection.
*
*/
private String location;
/**
*
* The ID of the connection.
*
*/
private String connectionId;
/**
*
* The type of virtual interface. The possible values are private
, public
and
* transit
.
*
*/
private String virtualInterfaceType;
/**
*
* The name of the virtual interface assigned by the customer network. The name has a maximum of 100 characters. The
* following are valid characters: a-z, 0-9 and a hyphen (-).
*
*/
private String virtualInterfaceName;
/**
*
* The ID of the VLAN.
*
*/
private Integer vlan;
/**
*
* The autonomous system (AS) number for Border Gateway Protocol (BGP) configuration.
*
*
* The valid values are 1-2147483647.
*
*/
private Integer asn;
/**
*
* The autonomous system number (ASN) for the Amazon side of the connection.
*
*/
private Long amazonSideAsn;
/**
*
* The authentication key for BGP configuration. This string has a minimum length of 6 characters and and a maximun
* lenth of 80 characters.
*
*/
private String authKey;
/**
*
* The IP address assigned to the Amazon interface.
*
*/
private String amazonAddress;
/**
*
* The IP address assigned to the customer interface.
*
*/
private String customerAddress;
/**
*
* The address family for the BGP peer.
*
*/
private String addressFamily;
/**
*
* The state of the virtual interface. The following are the possible values:
*
*
* -
*
* confirming
: The creation of the virtual interface is pending confirmation from the virtual interface
* owner. If the owner of the virtual interface is different from the owner of the connection on which it is
* provisioned, then the virtual interface will remain in this state until it is confirmed by the virtual interface
* owner.
*
*
* -
*
* verifying
: This state only applies to public virtual interfaces. Each public virtual interface needs
* validation before the virtual interface can be created.
*
*
* -
*
* pending
: A virtual interface is in this state from the time that it is created until the virtual
* interface is ready to forward traffic.
*
*
* -
*
* available
: A virtual interface that is able to forward traffic.
*
*
* -
*
* down
: A virtual interface that is BGP down.
*
*
* -
*
* deleting
: A virtual interface is in this state immediately after calling
* DeleteVirtualInterface until it can no longer forward traffic.
*
*
* -
*
* deleted
: A virtual interface that cannot forward traffic.
*
*
* -
*
* rejected
: The virtual interface owner has declined creation of the virtual interface. If a virtual
* interface in the Confirming
state is deleted by the virtual interface owner, the virtual interface
* enters the Rejected
state.
*
*
* -
*
* unknown
: The state of the virtual interface is not available.
*
*
*
*/
private String virtualInterfaceState;
/**
*
* The customer router configuration.
*
*/
private String customerRouterConfig;
/**
*
* The maximum transmission unit (MTU), in bytes. The supported values are 1500 and 8500. The default value is 1500
*
*/
private Integer mtu;
/**
*
* Indicates whether jumbo frames are supported.
*
*/
private Boolean jumboFrameCapable;
/**
*
* The ID of the virtual private gateway. Applies only to private virtual interfaces.
*
*/
private String virtualGatewayId;
/**
*
* The ID of the Direct Connect gateway.
*
*/
private String directConnectGatewayId;
/**
*
* The routes to be advertised to the Amazon Web Services network in this Region. Applies to public virtual
* interfaces.
*
*/
private com.amazonaws.internal.SdkInternalList routeFilterPrefixes;
/**
*
* The BGP peers configured on this virtual interface.
*
*/
private com.amazonaws.internal.SdkInternalList bgpPeers;
/**
*
* The Amazon Web Services Region where the virtual interface is located.
*
*/
private String region;
/**
*
* The Direct Connect endpoint that terminates the physical connection.
*
*/
private String awsDeviceV2;
/**
*
* The Direct Connect endpoint that terminates the logical connection. This device might be different than the
* device that terminates the physical connection.
*
*/
private String awsLogicalDeviceId;
/**
*
* The tags associated with the virtual interface.
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* Indicates whether SiteLink is enabled.
*
*/
private Boolean siteLinkEnabled;
/**
*
* The ID of the Amazon Web Services account that owns the virtual interface.
*
*
* @param ownerAccount
* The ID of the Amazon Web Services account that owns the virtual interface.
*/
public void setOwnerAccount(String ownerAccount) {
this.ownerAccount = ownerAccount;
}
/**
*
* The ID of the Amazon Web Services account that owns the virtual interface.
*
*
* @return The ID of the Amazon Web Services account that owns the virtual interface.
*/
public String getOwnerAccount() {
return this.ownerAccount;
}
/**
*
* The ID of the Amazon Web Services account that owns the virtual interface.
*
*
* @param ownerAccount
* The ID of the Amazon Web Services account that owns the virtual interface.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withOwnerAccount(String ownerAccount) {
setOwnerAccount(ownerAccount);
return this;
}
/**
*
* The ID of the virtual interface.
*
*
* @param virtualInterfaceId
* The ID of the virtual interface.
*/
public void setVirtualInterfaceId(String virtualInterfaceId) {
this.virtualInterfaceId = virtualInterfaceId;
}
/**
*
* The ID of the virtual interface.
*
*
* @return The ID of the virtual interface.
*/
public String getVirtualInterfaceId() {
return this.virtualInterfaceId;
}
/**
*
* The ID of the virtual interface.
*
*
* @param virtualInterfaceId
* The ID of the virtual interface.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withVirtualInterfaceId(String virtualInterfaceId) {
setVirtualInterfaceId(virtualInterfaceId);
return this;
}
/**
*
* The location of the connection.
*
*
* @param location
* The location of the connection.
*/
public void setLocation(String location) {
this.location = location;
}
/**
*
* The location of the connection.
*
*
* @return The location of the connection.
*/
public String getLocation() {
return this.location;
}
/**
*
* The location of the connection.
*
*
* @param location
* The location of the connection.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withLocation(String location) {
setLocation(location);
return this;
}
/**
*
* The ID of the connection.
*
*
* @param connectionId
* The ID of the connection.
*/
public void setConnectionId(String connectionId) {
this.connectionId = connectionId;
}
/**
*
* The ID of the connection.
*
*
* @return The ID of the connection.
*/
public String getConnectionId() {
return this.connectionId;
}
/**
*
* The ID of the connection.
*
*
* @param connectionId
* The ID of the connection.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withConnectionId(String connectionId) {
setConnectionId(connectionId);
return this;
}
/**
*
* The type of virtual interface. The possible values are private
, public
and
* transit
.
*
*
* @param virtualInterfaceType
* The type of virtual interface. The possible values are private
, public
and
* transit
.
*/
public void setVirtualInterfaceType(String virtualInterfaceType) {
this.virtualInterfaceType = virtualInterfaceType;
}
/**
*
* The type of virtual interface. The possible values are private
, public
and
* transit
.
*
*
* @return The type of virtual interface. The possible values are private
, public
and
* transit
.
*/
public String getVirtualInterfaceType() {
return this.virtualInterfaceType;
}
/**
*
* The type of virtual interface. The possible values are private
, public
and
* transit
.
*
*
* @param virtualInterfaceType
* The type of virtual interface. The possible values are private
, public
and
* transit
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withVirtualInterfaceType(String virtualInterfaceType) {
setVirtualInterfaceType(virtualInterfaceType);
return this;
}
/**
*
* The name of the virtual interface assigned by the customer network. The name has a maximum of 100 characters. The
* following are valid characters: a-z, 0-9 and a hyphen (-).
*
*
* @param virtualInterfaceName
* The name of the virtual interface assigned by the customer network. The name has a maximum of 100
* characters. The following are valid characters: a-z, 0-9 and a hyphen (-).
*/
public void setVirtualInterfaceName(String virtualInterfaceName) {
this.virtualInterfaceName = virtualInterfaceName;
}
/**
*
* The name of the virtual interface assigned by the customer network. The name has a maximum of 100 characters. The
* following are valid characters: a-z, 0-9 and a hyphen (-).
*
*
* @return The name of the virtual interface assigned by the customer network. The name has a maximum of 100
* characters. The following are valid characters: a-z, 0-9 and a hyphen (-).
*/
public String getVirtualInterfaceName() {
return this.virtualInterfaceName;
}
/**
*
* The name of the virtual interface assigned by the customer network. The name has a maximum of 100 characters. The
* following are valid characters: a-z, 0-9 and a hyphen (-).
*
*
* @param virtualInterfaceName
* The name of the virtual interface assigned by the customer network. The name has a maximum of 100
* characters. The following are valid characters: a-z, 0-9 and a hyphen (-).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withVirtualInterfaceName(String virtualInterfaceName) {
setVirtualInterfaceName(virtualInterfaceName);
return this;
}
/**
*
* The ID of the VLAN.
*
*
* @param vlan
* The ID of the VLAN.
*/
public void setVlan(Integer vlan) {
this.vlan = vlan;
}
/**
*
* The ID of the VLAN.
*
*
* @return The ID of the VLAN.
*/
public Integer getVlan() {
return this.vlan;
}
/**
*
* The ID of the VLAN.
*
*
* @param vlan
* The ID of the VLAN.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withVlan(Integer vlan) {
setVlan(vlan);
return this;
}
/**
*
* The autonomous system (AS) number for Border Gateway Protocol (BGP) configuration.
*
*
* The valid values are 1-2147483647.
*
*
* @param asn
* The autonomous system (AS) number for Border Gateway Protocol (BGP) configuration.
*
* The valid values are 1-2147483647.
*/
public void setAsn(Integer asn) {
this.asn = asn;
}
/**
*
* The autonomous system (AS) number for Border Gateway Protocol (BGP) configuration.
*
*
* The valid values are 1-2147483647.
*
*
* @return The autonomous system (AS) number for Border Gateway Protocol (BGP) configuration.
*
* The valid values are 1-2147483647.
*/
public Integer getAsn() {
return this.asn;
}
/**
*
* The autonomous system (AS) number for Border Gateway Protocol (BGP) configuration.
*
*
* The valid values are 1-2147483647.
*
*
* @param asn
* The autonomous system (AS) number for Border Gateway Protocol (BGP) configuration.
*
* The valid values are 1-2147483647.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withAsn(Integer asn) {
setAsn(asn);
return this;
}
/**
*
* The autonomous system number (ASN) for the Amazon side of the connection.
*
*
* @param amazonSideAsn
* The autonomous system number (ASN) for the Amazon side of the connection.
*/
public void setAmazonSideAsn(Long amazonSideAsn) {
this.amazonSideAsn = amazonSideAsn;
}
/**
*
* The autonomous system number (ASN) for the Amazon side of the connection.
*
*
* @return The autonomous system number (ASN) for the Amazon side of the connection.
*/
public Long getAmazonSideAsn() {
return this.amazonSideAsn;
}
/**
*
* The autonomous system number (ASN) for the Amazon side of the connection.
*
*
* @param amazonSideAsn
* The autonomous system number (ASN) for the Amazon side of the connection.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withAmazonSideAsn(Long amazonSideAsn) {
setAmazonSideAsn(amazonSideAsn);
return this;
}
/**
*
* The authentication key for BGP configuration. This string has a minimum length of 6 characters and and a maximun
* lenth of 80 characters.
*
*
* @param authKey
* The authentication key for BGP configuration. This string has a minimum length of 6 characters and and a
* maximun lenth of 80 characters.
*/
public void setAuthKey(String authKey) {
this.authKey = authKey;
}
/**
*
* The authentication key for BGP configuration. This string has a minimum length of 6 characters and and a maximun
* lenth of 80 characters.
*
*
* @return The authentication key for BGP configuration. This string has a minimum length of 6 characters and and a
* maximun lenth of 80 characters.
*/
public String getAuthKey() {
return this.authKey;
}
/**
*
* The authentication key for BGP configuration. This string has a minimum length of 6 characters and and a maximun
* lenth of 80 characters.
*
*
* @param authKey
* The authentication key for BGP configuration. This string has a minimum length of 6 characters and and a
* maximun lenth of 80 characters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withAuthKey(String authKey) {
setAuthKey(authKey);
return this;
}
/**
*
* The IP address assigned to the Amazon interface.
*
*
* @param amazonAddress
* The IP address assigned to the Amazon interface.
*/
public void setAmazonAddress(String amazonAddress) {
this.amazonAddress = amazonAddress;
}
/**
*
* The IP address assigned to the Amazon interface.
*
*
* @return The IP address assigned to the Amazon interface.
*/
public String getAmazonAddress() {
return this.amazonAddress;
}
/**
*
* The IP address assigned to the Amazon interface.
*
*
* @param amazonAddress
* The IP address assigned to the Amazon interface.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withAmazonAddress(String amazonAddress) {
setAmazonAddress(amazonAddress);
return this;
}
/**
*
* The IP address assigned to the customer interface.
*
*
* @param customerAddress
* The IP address assigned to the customer interface.
*/
public void setCustomerAddress(String customerAddress) {
this.customerAddress = customerAddress;
}
/**
*
* The IP address assigned to the customer interface.
*
*
* @return The IP address assigned to the customer interface.
*/
public String getCustomerAddress() {
return this.customerAddress;
}
/**
*
* The IP address assigned to the customer interface.
*
*
* @param customerAddress
* The IP address assigned to the customer interface.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withCustomerAddress(String customerAddress) {
setCustomerAddress(customerAddress);
return this;
}
/**
*
* The address family for the BGP peer.
*
*
* @param addressFamily
* The address family for the BGP peer.
* @see AddressFamily
*/
public void setAddressFamily(String addressFamily) {
this.addressFamily = addressFamily;
}
/**
*
* The address family for the BGP peer.
*
*
* @return The address family for the BGP peer.
* @see AddressFamily
*/
public String getAddressFamily() {
return this.addressFamily;
}
/**
*
* The address family for the BGP peer.
*
*
* @param addressFamily
* The address family for the BGP peer.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AddressFamily
*/
public AllocatePrivateVirtualInterfaceResult withAddressFamily(String addressFamily) {
setAddressFamily(addressFamily);
return this;
}
/**
*
* The address family for the BGP peer.
*
*
* @param addressFamily
* The address family for the BGP peer.
* @see AddressFamily
*/
public void setAddressFamily(AddressFamily addressFamily) {
withAddressFamily(addressFamily);
}
/**
*
* The address family for the BGP peer.
*
*
* @param addressFamily
* The address family for the BGP peer.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AddressFamily
*/
public AllocatePrivateVirtualInterfaceResult withAddressFamily(AddressFamily addressFamily) {
this.addressFamily = addressFamily.toString();
return this;
}
/**
*
* The state of the virtual interface. The following are the possible values:
*
*
* -
*
* confirming
: The creation of the virtual interface is pending confirmation from the virtual interface
* owner. If the owner of the virtual interface is different from the owner of the connection on which it is
* provisioned, then the virtual interface will remain in this state until it is confirmed by the virtual interface
* owner.
*
*
* -
*
* verifying
: This state only applies to public virtual interfaces. Each public virtual interface needs
* validation before the virtual interface can be created.
*
*
* -
*
* pending
: A virtual interface is in this state from the time that it is created until the virtual
* interface is ready to forward traffic.
*
*
* -
*
* available
: A virtual interface that is able to forward traffic.
*
*
* -
*
* down
: A virtual interface that is BGP down.
*
*
* -
*
* deleting
: A virtual interface is in this state immediately after calling
* DeleteVirtualInterface until it can no longer forward traffic.
*
*
* -
*
* deleted
: A virtual interface that cannot forward traffic.
*
*
* -
*
* rejected
: The virtual interface owner has declined creation of the virtual interface. If a virtual
* interface in the Confirming
state is deleted by the virtual interface owner, the virtual interface
* enters the Rejected
state.
*
*
* -
*
* unknown
: The state of the virtual interface is not available.
*
*
*
*
* @param virtualInterfaceState
* The state of the virtual interface. The following are the possible values:
*
* -
*
* confirming
: The creation of the virtual interface is pending confirmation from the virtual
* interface owner. If the owner of the virtual interface is different from the owner of the connection on
* which it is provisioned, then the virtual interface will remain in this state until it is confirmed by the
* virtual interface owner.
*
*
* -
*
* verifying
: This state only applies to public virtual interfaces. Each public virtual
* interface needs validation before the virtual interface can be created.
*
*
* -
*
* pending
: A virtual interface is in this state from the time that it is created until the
* virtual interface is ready to forward traffic.
*
*
* -
*
* available
: A virtual interface that is able to forward traffic.
*
*
* -
*
* down
: A virtual interface that is BGP down.
*
*
* -
*
* deleting
: A virtual interface is in this state immediately after calling
* DeleteVirtualInterface until it can no longer forward traffic.
*
*
* -
*
* deleted
: A virtual interface that cannot forward traffic.
*
*
* -
*
* rejected
: The virtual interface owner has declined creation of the virtual interface. If a
* virtual interface in the Confirming
state is deleted by the virtual interface owner, the
* virtual interface enters the Rejected
state.
*
*
* -
*
* unknown
: The state of the virtual interface is not available.
*
*
* @see VirtualInterfaceState
*/
public void setVirtualInterfaceState(String virtualInterfaceState) {
this.virtualInterfaceState = virtualInterfaceState;
}
/**
*
* The state of the virtual interface. The following are the possible values:
*
*
* -
*
* confirming
: The creation of the virtual interface is pending confirmation from the virtual interface
* owner. If the owner of the virtual interface is different from the owner of the connection on which it is
* provisioned, then the virtual interface will remain in this state until it is confirmed by the virtual interface
* owner.
*
*
* -
*
* verifying
: This state only applies to public virtual interfaces. Each public virtual interface needs
* validation before the virtual interface can be created.
*
*
* -
*
* pending
: A virtual interface is in this state from the time that it is created until the virtual
* interface is ready to forward traffic.
*
*
* -
*
* available
: A virtual interface that is able to forward traffic.
*
*
* -
*
* down
: A virtual interface that is BGP down.
*
*
* -
*
* deleting
: A virtual interface is in this state immediately after calling
* DeleteVirtualInterface until it can no longer forward traffic.
*
*
* -
*
* deleted
: A virtual interface that cannot forward traffic.
*
*
* -
*
* rejected
: The virtual interface owner has declined creation of the virtual interface. If a virtual
* interface in the Confirming
state is deleted by the virtual interface owner, the virtual interface
* enters the Rejected
state.
*
*
* -
*
* unknown
: The state of the virtual interface is not available.
*
*
*
*
* @return The state of the virtual interface. The following are the possible values:
*
* -
*
* confirming
: The creation of the virtual interface is pending confirmation from the virtual
* interface owner. If the owner of the virtual interface is different from the owner of the connection on
* which it is provisioned, then the virtual interface will remain in this state until it is confirmed by
* the virtual interface owner.
*
*
* -
*
* verifying
: This state only applies to public virtual interfaces. Each public virtual
* interface needs validation before the virtual interface can be created.
*
*
* -
*
* pending
: A virtual interface is in this state from the time that it is created until the
* virtual interface is ready to forward traffic.
*
*
* -
*
* available
: A virtual interface that is able to forward traffic.
*
*
* -
*
* down
: A virtual interface that is BGP down.
*
*
* -
*
* deleting
: A virtual interface is in this state immediately after calling
* DeleteVirtualInterface until it can no longer forward traffic.
*
*
* -
*
* deleted
: A virtual interface that cannot forward traffic.
*
*
* -
*
* rejected
: The virtual interface owner has declined creation of the virtual interface. If a
* virtual interface in the Confirming
state is deleted by the virtual interface owner, the
* virtual interface enters the Rejected
state.
*
*
* -
*
* unknown
: The state of the virtual interface is not available.
*
*
* @see VirtualInterfaceState
*/
public String getVirtualInterfaceState() {
return this.virtualInterfaceState;
}
/**
*
* The state of the virtual interface. The following are the possible values:
*
*
* -
*
* confirming
: The creation of the virtual interface is pending confirmation from the virtual interface
* owner. If the owner of the virtual interface is different from the owner of the connection on which it is
* provisioned, then the virtual interface will remain in this state until it is confirmed by the virtual interface
* owner.
*
*
* -
*
* verifying
: This state only applies to public virtual interfaces. Each public virtual interface needs
* validation before the virtual interface can be created.
*
*
* -
*
* pending
: A virtual interface is in this state from the time that it is created until the virtual
* interface is ready to forward traffic.
*
*
* -
*
* available
: A virtual interface that is able to forward traffic.
*
*
* -
*
* down
: A virtual interface that is BGP down.
*
*
* -
*
* deleting
: A virtual interface is in this state immediately after calling
* DeleteVirtualInterface until it can no longer forward traffic.
*
*
* -
*
* deleted
: A virtual interface that cannot forward traffic.
*
*
* -
*
* rejected
: The virtual interface owner has declined creation of the virtual interface. If a virtual
* interface in the Confirming
state is deleted by the virtual interface owner, the virtual interface
* enters the Rejected
state.
*
*
* -
*
* unknown
: The state of the virtual interface is not available.
*
*
*
*
* @param virtualInterfaceState
* The state of the virtual interface. The following are the possible values:
*
* -
*
* confirming
: The creation of the virtual interface is pending confirmation from the virtual
* interface owner. If the owner of the virtual interface is different from the owner of the connection on
* which it is provisioned, then the virtual interface will remain in this state until it is confirmed by the
* virtual interface owner.
*
*
* -
*
* verifying
: This state only applies to public virtual interfaces. Each public virtual
* interface needs validation before the virtual interface can be created.
*
*
* -
*
* pending
: A virtual interface is in this state from the time that it is created until the
* virtual interface is ready to forward traffic.
*
*
* -
*
* available
: A virtual interface that is able to forward traffic.
*
*
* -
*
* down
: A virtual interface that is BGP down.
*
*
* -
*
* deleting
: A virtual interface is in this state immediately after calling
* DeleteVirtualInterface until it can no longer forward traffic.
*
*
* -
*
* deleted
: A virtual interface that cannot forward traffic.
*
*
* -
*
* rejected
: The virtual interface owner has declined creation of the virtual interface. If a
* virtual interface in the Confirming
state is deleted by the virtual interface owner, the
* virtual interface enters the Rejected
state.
*
*
* -
*
* unknown
: The state of the virtual interface is not available.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see VirtualInterfaceState
*/
public AllocatePrivateVirtualInterfaceResult withVirtualInterfaceState(String virtualInterfaceState) {
setVirtualInterfaceState(virtualInterfaceState);
return this;
}
/**
*
* The state of the virtual interface. The following are the possible values:
*
*
* -
*
* confirming
: The creation of the virtual interface is pending confirmation from the virtual interface
* owner. If the owner of the virtual interface is different from the owner of the connection on which it is
* provisioned, then the virtual interface will remain in this state until it is confirmed by the virtual interface
* owner.
*
*
* -
*
* verifying
: This state only applies to public virtual interfaces. Each public virtual interface needs
* validation before the virtual interface can be created.
*
*
* -
*
* pending
: A virtual interface is in this state from the time that it is created until the virtual
* interface is ready to forward traffic.
*
*
* -
*
* available
: A virtual interface that is able to forward traffic.
*
*
* -
*
* down
: A virtual interface that is BGP down.
*
*
* -
*
* deleting
: A virtual interface is in this state immediately after calling
* DeleteVirtualInterface until it can no longer forward traffic.
*
*
* -
*
* deleted
: A virtual interface that cannot forward traffic.
*
*
* -
*
* rejected
: The virtual interface owner has declined creation of the virtual interface. If a virtual
* interface in the Confirming
state is deleted by the virtual interface owner, the virtual interface
* enters the Rejected
state.
*
*
* -
*
* unknown
: The state of the virtual interface is not available.
*
*
*
*
* @param virtualInterfaceState
* The state of the virtual interface. The following are the possible values:
*
* -
*
* confirming
: The creation of the virtual interface is pending confirmation from the virtual
* interface owner. If the owner of the virtual interface is different from the owner of the connection on
* which it is provisioned, then the virtual interface will remain in this state until it is confirmed by the
* virtual interface owner.
*
*
* -
*
* verifying
: This state only applies to public virtual interfaces. Each public virtual
* interface needs validation before the virtual interface can be created.
*
*
* -
*
* pending
: A virtual interface is in this state from the time that it is created until the
* virtual interface is ready to forward traffic.
*
*
* -
*
* available
: A virtual interface that is able to forward traffic.
*
*
* -
*
* down
: A virtual interface that is BGP down.
*
*
* -
*
* deleting
: A virtual interface is in this state immediately after calling
* DeleteVirtualInterface until it can no longer forward traffic.
*
*
* -
*
* deleted
: A virtual interface that cannot forward traffic.
*
*
* -
*
* rejected
: The virtual interface owner has declined creation of the virtual interface. If a
* virtual interface in the Confirming
state is deleted by the virtual interface owner, the
* virtual interface enters the Rejected
state.
*
*
* -
*
* unknown
: The state of the virtual interface is not available.
*
*
* @see VirtualInterfaceState
*/
public void setVirtualInterfaceState(VirtualInterfaceState virtualInterfaceState) {
withVirtualInterfaceState(virtualInterfaceState);
}
/**
*
* The state of the virtual interface. The following are the possible values:
*
*
* -
*
* confirming
: The creation of the virtual interface is pending confirmation from the virtual interface
* owner. If the owner of the virtual interface is different from the owner of the connection on which it is
* provisioned, then the virtual interface will remain in this state until it is confirmed by the virtual interface
* owner.
*
*
* -
*
* verifying
: This state only applies to public virtual interfaces. Each public virtual interface needs
* validation before the virtual interface can be created.
*
*
* -
*
* pending
: A virtual interface is in this state from the time that it is created until the virtual
* interface is ready to forward traffic.
*
*
* -
*
* available
: A virtual interface that is able to forward traffic.
*
*
* -
*
* down
: A virtual interface that is BGP down.
*
*
* -
*
* deleting
: A virtual interface is in this state immediately after calling
* DeleteVirtualInterface until it can no longer forward traffic.
*
*
* -
*
* deleted
: A virtual interface that cannot forward traffic.
*
*
* -
*
* rejected
: The virtual interface owner has declined creation of the virtual interface. If a virtual
* interface in the Confirming
state is deleted by the virtual interface owner, the virtual interface
* enters the Rejected
state.
*
*
* -
*
* unknown
: The state of the virtual interface is not available.
*
*
*
*
* @param virtualInterfaceState
* The state of the virtual interface. The following are the possible values:
*
* -
*
* confirming
: The creation of the virtual interface is pending confirmation from the virtual
* interface owner. If the owner of the virtual interface is different from the owner of the connection on
* which it is provisioned, then the virtual interface will remain in this state until it is confirmed by the
* virtual interface owner.
*
*
* -
*
* verifying
: This state only applies to public virtual interfaces. Each public virtual
* interface needs validation before the virtual interface can be created.
*
*
* -
*
* pending
: A virtual interface is in this state from the time that it is created until the
* virtual interface is ready to forward traffic.
*
*
* -
*
* available
: A virtual interface that is able to forward traffic.
*
*
* -
*
* down
: A virtual interface that is BGP down.
*
*
* -
*
* deleting
: A virtual interface is in this state immediately after calling
* DeleteVirtualInterface until it can no longer forward traffic.
*
*
* -
*
* deleted
: A virtual interface that cannot forward traffic.
*
*
* -
*
* rejected
: The virtual interface owner has declined creation of the virtual interface. If a
* virtual interface in the Confirming
state is deleted by the virtual interface owner, the
* virtual interface enters the Rejected
state.
*
*
* -
*
* unknown
: The state of the virtual interface is not available.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see VirtualInterfaceState
*/
public AllocatePrivateVirtualInterfaceResult withVirtualInterfaceState(VirtualInterfaceState virtualInterfaceState) {
this.virtualInterfaceState = virtualInterfaceState.toString();
return this;
}
/**
*
* The customer router configuration.
*
*
* @param customerRouterConfig
* The customer router configuration.
*/
public void setCustomerRouterConfig(String customerRouterConfig) {
this.customerRouterConfig = customerRouterConfig;
}
/**
*
* The customer router configuration.
*
*
* @return The customer router configuration.
*/
public String getCustomerRouterConfig() {
return this.customerRouterConfig;
}
/**
*
* The customer router configuration.
*
*
* @param customerRouterConfig
* The customer router configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withCustomerRouterConfig(String customerRouterConfig) {
setCustomerRouterConfig(customerRouterConfig);
return this;
}
/**
*
* The maximum transmission unit (MTU), in bytes. The supported values are 1500 and 8500. The default value is 1500
*
*
* @param mtu
* The maximum transmission unit (MTU), in bytes. The supported values are 1500 and 8500. The default value
* is 1500
*/
public void setMtu(Integer mtu) {
this.mtu = mtu;
}
/**
*
* The maximum transmission unit (MTU), in bytes. The supported values are 1500 and 8500. The default value is 1500
*
*
* @return The maximum transmission unit (MTU), in bytes. The supported values are 1500 and 8500. The default value
* is 1500
*/
public Integer getMtu() {
return this.mtu;
}
/**
*
* The maximum transmission unit (MTU), in bytes. The supported values are 1500 and 8500. The default value is 1500
*
*
* @param mtu
* The maximum transmission unit (MTU), in bytes. The supported values are 1500 and 8500. The default value
* is 1500
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withMtu(Integer mtu) {
setMtu(mtu);
return this;
}
/**
*
* Indicates whether jumbo frames are supported.
*
*
* @param jumboFrameCapable
* Indicates whether jumbo frames are supported.
*/
public void setJumboFrameCapable(Boolean jumboFrameCapable) {
this.jumboFrameCapable = jumboFrameCapable;
}
/**
*
* Indicates whether jumbo frames are supported.
*
*
* @return Indicates whether jumbo frames are supported.
*/
public Boolean getJumboFrameCapable() {
return this.jumboFrameCapable;
}
/**
*
* Indicates whether jumbo frames are supported.
*
*
* @param jumboFrameCapable
* Indicates whether jumbo frames are supported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withJumboFrameCapable(Boolean jumboFrameCapable) {
setJumboFrameCapable(jumboFrameCapable);
return this;
}
/**
*
* Indicates whether jumbo frames are supported.
*
*
* @return Indicates whether jumbo frames are supported.
*/
public Boolean isJumboFrameCapable() {
return this.jumboFrameCapable;
}
/**
*
* The ID of the virtual private gateway. Applies only to private virtual interfaces.
*
*
* @param virtualGatewayId
* The ID of the virtual private gateway. Applies only to private virtual interfaces.
*/
public void setVirtualGatewayId(String virtualGatewayId) {
this.virtualGatewayId = virtualGatewayId;
}
/**
*
* The ID of the virtual private gateway. Applies only to private virtual interfaces.
*
*
* @return The ID of the virtual private gateway. Applies only to private virtual interfaces.
*/
public String getVirtualGatewayId() {
return this.virtualGatewayId;
}
/**
*
* The ID of the virtual private gateway. Applies only to private virtual interfaces.
*
*
* @param virtualGatewayId
* The ID of the virtual private gateway. Applies only to private virtual interfaces.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withVirtualGatewayId(String virtualGatewayId) {
setVirtualGatewayId(virtualGatewayId);
return this;
}
/**
*
* The ID of the Direct Connect gateway.
*
*
* @param directConnectGatewayId
* The ID of the Direct Connect gateway.
*/
public void setDirectConnectGatewayId(String directConnectGatewayId) {
this.directConnectGatewayId = directConnectGatewayId;
}
/**
*
* The ID of the Direct Connect gateway.
*
*
* @return The ID of the Direct Connect gateway.
*/
public String getDirectConnectGatewayId() {
return this.directConnectGatewayId;
}
/**
*
* The ID of the Direct Connect gateway.
*
*
* @param directConnectGatewayId
* The ID of the Direct Connect gateway.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withDirectConnectGatewayId(String directConnectGatewayId) {
setDirectConnectGatewayId(directConnectGatewayId);
return this;
}
/**
*
* The routes to be advertised to the Amazon Web Services network in this Region. Applies to public virtual
* interfaces.
*
*
* @return The routes to be advertised to the Amazon Web Services network in this Region. Applies to public virtual
* interfaces.
*/
public java.util.List getRouteFilterPrefixes() {
if (routeFilterPrefixes == null) {
routeFilterPrefixes = new com.amazonaws.internal.SdkInternalList();
}
return routeFilterPrefixes;
}
/**
*
* The routes to be advertised to the Amazon Web Services network in this Region. Applies to public virtual
* interfaces.
*
*
* @param routeFilterPrefixes
* The routes to be advertised to the Amazon Web Services network in this Region. Applies to public virtual
* interfaces.
*/
public void setRouteFilterPrefixes(java.util.Collection routeFilterPrefixes) {
if (routeFilterPrefixes == null) {
this.routeFilterPrefixes = null;
return;
}
this.routeFilterPrefixes = new com.amazonaws.internal.SdkInternalList(routeFilterPrefixes);
}
/**
*
* The routes to be advertised to the Amazon Web Services network in this Region. Applies to public virtual
* interfaces.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRouteFilterPrefixes(java.util.Collection)} or {@link #withRouteFilterPrefixes(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param routeFilterPrefixes
* The routes to be advertised to the Amazon Web Services network in this Region. Applies to public virtual
* interfaces.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withRouteFilterPrefixes(RouteFilterPrefix... routeFilterPrefixes) {
if (this.routeFilterPrefixes == null) {
setRouteFilterPrefixes(new com.amazonaws.internal.SdkInternalList(routeFilterPrefixes.length));
}
for (RouteFilterPrefix ele : routeFilterPrefixes) {
this.routeFilterPrefixes.add(ele);
}
return this;
}
/**
*
* The routes to be advertised to the Amazon Web Services network in this Region. Applies to public virtual
* interfaces.
*
*
* @param routeFilterPrefixes
* The routes to be advertised to the Amazon Web Services network in this Region. Applies to public virtual
* interfaces.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withRouteFilterPrefixes(java.util.Collection routeFilterPrefixes) {
setRouteFilterPrefixes(routeFilterPrefixes);
return this;
}
/**
*
* The BGP peers configured on this virtual interface.
*
*
* @return The BGP peers configured on this virtual interface.
*/
public java.util.List getBgpPeers() {
if (bgpPeers == null) {
bgpPeers = new com.amazonaws.internal.SdkInternalList();
}
return bgpPeers;
}
/**
*
* The BGP peers configured on this virtual interface.
*
*
* @param bgpPeers
* The BGP peers configured on this virtual interface.
*/
public void setBgpPeers(java.util.Collection bgpPeers) {
if (bgpPeers == null) {
this.bgpPeers = null;
return;
}
this.bgpPeers = new com.amazonaws.internal.SdkInternalList(bgpPeers);
}
/**
*
* The BGP peers configured on this virtual interface.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setBgpPeers(java.util.Collection)} or {@link #withBgpPeers(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param bgpPeers
* The BGP peers configured on this virtual interface.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withBgpPeers(BGPPeer... bgpPeers) {
if (this.bgpPeers == null) {
setBgpPeers(new com.amazonaws.internal.SdkInternalList(bgpPeers.length));
}
for (BGPPeer ele : bgpPeers) {
this.bgpPeers.add(ele);
}
return this;
}
/**
*
* The BGP peers configured on this virtual interface.
*
*
* @param bgpPeers
* The BGP peers configured on this virtual interface.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withBgpPeers(java.util.Collection bgpPeers) {
setBgpPeers(bgpPeers);
return this;
}
/**
*
* The Amazon Web Services Region where the virtual interface is located.
*
*
* @param region
* The Amazon Web Services Region where the virtual interface is located.
*/
public void setRegion(String region) {
this.region = region;
}
/**
*
* The Amazon Web Services Region where the virtual interface is located.
*
*
* @return The Amazon Web Services Region where the virtual interface is located.
*/
public String getRegion() {
return this.region;
}
/**
*
* The Amazon Web Services Region where the virtual interface is located.
*
*
* @param region
* The Amazon Web Services Region where the virtual interface is located.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withRegion(String region) {
setRegion(region);
return this;
}
/**
*
* The Direct Connect endpoint that terminates the physical connection.
*
*
* @param awsDeviceV2
* The Direct Connect endpoint that terminates the physical connection.
*/
public void setAwsDeviceV2(String awsDeviceV2) {
this.awsDeviceV2 = awsDeviceV2;
}
/**
*
* The Direct Connect endpoint that terminates the physical connection.
*
*
* @return The Direct Connect endpoint that terminates the physical connection.
*/
public String getAwsDeviceV2() {
return this.awsDeviceV2;
}
/**
*
* The Direct Connect endpoint that terminates the physical connection.
*
*
* @param awsDeviceV2
* The Direct Connect endpoint that terminates the physical connection.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withAwsDeviceV2(String awsDeviceV2) {
setAwsDeviceV2(awsDeviceV2);
return this;
}
/**
*
* The Direct Connect endpoint that terminates the logical connection. This device might be different than the
* device that terminates the physical connection.
*
*
* @param awsLogicalDeviceId
* The Direct Connect endpoint that terminates the logical connection. This device might be different than
* the device that terminates the physical connection.
*/
public void setAwsLogicalDeviceId(String awsLogicalDeviceId) {
this.awsLogicalDeviceId = awsLogicalDeviceId;
}
/**
*
* The Direct Connect endpoint that terminates the logical connection. This device might be different than the
* device that terminates the physical connection.
*
*
* @return The Direct Connect endpoint that terminates the logical connection. This device might be different than
* the device that terminates the physical connection.
*/
public String getAwsLogicalDeviceId() {
return this.awsLogicalDeviceId;
}
/**
*
* The Direct Connect endpoint that terminates the logical connection. This device might be different than the
* device that terminates the physical connection.
*
*
* @param awsLogicalDeviceId
* The Direct Connect endpoint that terminates the logical connection. This device might be different than
* the device that terminates the physical connection.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withAwsLogicalDeviceId(String awsLogicalDeviceId) {
setAwsLogicalDeviceId(awsLogicalDeviceId);
return this;
}
/**
*
* The tags associated with the virtual interface.
*
*
* @return The tags associated with the virtual interface.
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* The tags associated with the virtual interface.
*
*
* @param tags
* The tags associated with the virtual interface.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* The tags associated with the virtual interface.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The tags associated with the virtual interface.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The tags associated with the virtual interface.
*
*
* @param tags
* The tags associated with the virtual interface.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* Indicates whether SiteLink is enabled.
*
*
* @param siteLinkEnabled
* Indicates whether SiteLink is enabled.
*/
public void setSiteLinkEnabled(Boolean siteLinkEnabled) {
this.siteLinkEnabled = siteLinkEnabled;
}
/**
*
* Indicates whether SiteLink is enabled.
*
*
* @return Indicates whether SiteLink is enabled.
*/
public Boolean getSiteLinkEnabled() {
return this.siteLinkEnabled;
}
/**
*
* Indicates whether SiteLink is enabled.
*
*
* @param siteLinkEnabled
* Indicates whether SiteLink is enabled.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocatePrivateVirtualInterfaceResult withSiteLinkEnabled(Boolean siteLinkEnabled) {
setSiteLinkEnabled(siteLinkEnabled);
return this;
}
/**
*
* Indicates whether SiteLink is enabled.
*
*
* @return Indicates whether SiteLink is enabled.
*/
public Boolean isSiteLinkEnabled() {
return this.siteLinkEnabled;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getOwnerAccount() != null)
sb.append("OwnerAccount: ").append(getOwnerAccount()).append(",");
if (getVirtualInterfaceId() != null)
sb.append("VirtualInterfaceId: ").append(getVirtualInterfaceId()).append(",");
if (getLocation() != null)
sb.append("Location: ").append(getLocation()).append(",");
if (getConnectionId() != null)
sb.append("ConnectionId: ").append(getConnectionId()).append(",");
if (getVirtualInterfaceType() != null)
sb.append("VirtualInterfaceType: ").append(getVirtualInterfaceType()).append(",");
if (getVirtualInterfaceName() != null)
sb.append("VirtualInterfaceName: ").append(getVirtualInterfaceName()).append(",");
if (getVlan() != null)
sb.append("Vlan: ").append(getVlan()).append(",");
if (getAsn() != null)
sb.append("Asn: ").append(getAsn()).append(",");
if (getAmazonSideAsn() != null)
sb.append("AmazonSideAsn: ").append(getAmazonSideAsn()).append(",");
if (getAuthKey() != null)
sb.append("AuthKey: ").append(getAuthKey()).append(",");
if (getAmazonAddress() != null)
sb.append("AmazonAddress: ").append(getAmazonAddress()).append(",");
if (getCustomerAddress() != null)
sb.append("CustomerAddress: ").append(getCustomerAddress()).append(",");
if (getAddressFamily() != null)
sb.append("AddressFamily: ").append(getAddressFamily()).append(",");
if (getVirtualInterfaceState() != null)
sb.append("VirtualInterfaceState: ").append(getVirtualInterfaceState()).append(",");
if (getCustomerRouterConfig() != null)
sb.append("CustomerRouterConfig: ").append(getCustomerRouterConfig()).append(",");
if (getMtu() != null)
sb.append("Mtu: ").append(getMtu()).append(",");
if (getJumboFrameCapable() != null)
sb.append("JumboFrameCapable: ").append(getJumboFrameCapable()).append(",");
if (getVirtualGatewayId() != null)
sb.append("VirtualGatewayId: ").append(getVirtualGatewayId()).append(",");
if (getDirectConnectGatewayId() != null)
sb.append("DirectConnectGatewayId: ").append(getDirectConnectGatewayId()).append(",");
if (getRouteFilterPrefixes() != null)
sb.append("RouteFilterPrefixes: ").append(getRouteFilterPrefixes()).append(",");
if (getBgpPeers() != null)
sb.append("BgpPeers: ").append(getBgpPeers()).append(",");
if (getRegion() != null)
sb.append("Region: ").append(getRegion()).append(",");
if (getAwsDeviceV2() != null)
sb.append("AwsDeviceV2: ").append(getAwsDeviceV2()).append(",");
if (getAwsLogicalDeviceId() != null)
sb.append("AwsLogicalDeviceId: ").append(getAwsLogicalDeviceId()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getSiteLinkEnabled() != null)
sb.append("SiteLinkEnabled: ").append(getSiteLinkEnabled());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AllocatePrivateVirtualInterfaceResult == false)
return false;
AllocatePrivateVirtualInterfaceResult other = (AllocatePrivateVirtualInterfaceResult) obj;
if (other.getOwnerAccount() == null ^ this.getOwnerAccount() == null)
return false;
if (other.getOwnerAccount() != null && other.getOwnerAccount().equals(this.getOwnerAccount()) == false)
return false;
if (other.getVirtualInterfaceId() == null ^ this.getVirtualInterfaceId() == null)
return false;
if (other.getVirtualInterfaceId() != null && other.getVirtualInterfaceId().equals(this.getVirtualInterfaceId()) == false)
return false;
if (other.getLocation() == null ^ this.getLocation() == null)
return false;
if (other.getLocation() != null && other.getLocation().equals(this.getLocation()) == false)
return false;
if (other.getConnectionId() == null ^ this.getConnectionId() == null)
return false;
if (other.getConnectionId() != null && other.getConnectionId().equals(this.getConnectionId()) == false)
return false;
if (other.getVirtualInterfaceType() == null ^ this.getVirtualInterfaceType() == null)
return false;
if (other.getVirtualInterfaceType() != null && other.getVirtualInterfaceType().equals(this.getVirtualInterfaceType()) == false)
return false;
if (other.getVirtualInterfaceName() == null ^ this.getVirtualInterfaceName() == null)
return false;
if (other.getVirtualInterfaceName() != null && other.getVirtualInterfaceName().equals(this.getVirtualInterfaceName()) == false)
return false;
if (other.getVlan() == null ^ this.getVlan() == null)
return false;
if (other.getVlan() != null && other.getVlan().equals(this.getVlan()) == false)
return false;
if (other.getAsn() == null ^ this.getAsn() == null)
return false;
if (other.getAsn() != null && other.getAsn().equals(this.getAsn()) == false)
return false;
if (other.getAmazonSideAsn() == null ^ this.getAmazonSideAsn() == null)
return false;
if (other.getAmazonSideAsn() != null && other.getAmazonSideAsn().equals(this.getAmazonSideAsn()) == false)
return false;
if (other.getAuthKey() == null ^ this.getAuthKey() == null)
return false;
if (other.getAuthKey() != null && other.getAuthKey().equals(this.getAuthKey()) == false)
return false;
if (other.getAmazonAddress() == null ^ this.getAmazonAddress() == null)
return false;
if (other.getAmazonAddress() != null && other.getAmazonAddress().equals(this.getAmazonAddress()) == false)
return false;
if (other.getCustomerAddress() == null ^ this.getCustomerAddress() == null)
return false;
if (other.getCustomerAddress() != null && other.getCustomerAddress().equals(this.getCustomerAddress()) == false)
return false;
if (other.getAddressFamily() == null ^ this.getAddressFamily() == null)
return false;
if (other.getAddressFamily() != null && other.getAddressFamily().equals(this.getAddressFamily()) == false)
return false;
if (other.getVirtualInterfaceState() == null ^ this.getVirtualInterfaceState() == null)
return false;
if (other.getVirtualInterfaceState() != null && other.getVirtualInterfaceState().equals(this.getVirtualInterfaceState()) == false)
return false;
if (other.getCustomerRouterConfig() == null ^ this.getCustomerRouterConfig() == null)
return false;
if (other.getCustomerRouterConfig() != null && other.getCustomerRouterConfig().equals(this.getCustomerRouterConfig()) == false)
return false;
if (other.getMtu() == null ^ this.getMtu() == null)
return false;
if (other.getMtu() != null && other.getMtu().equals(this.getMtu()) == false)
return false;
if (other.getJumboFrameCapable() == null ^ this.getJumboFrameCapable() == null)
return false;
if (other.getJumboFrameCapable() != null && other.getJumboFrameCapable().equals(this.getJumboFrameCapable()) == false)
return false;
if (other.getVirtualGatewayId() == null ^ this.getVirtualGatewayId() == null)
return false;
if (other.getVirtualGatewayId() != null && other.getVirtualGatewayId().equals(this.getVirtualGatewayId()) == false)
return false;
if (other.getDirectConnectGatewayId() == null ^ this.getDirectConnectGatewayId() == null)
return false;
if (other.getDirectConnectGatewayId() != null && other.getDirectConnectGatewayId().equals(this.getDirectConnectGatewayId()) == false)
return false;
if (other.getRouteFilterPrefixes() == null ^ this.getRouteFilterPrefixes() == null)
return false;
if (other.getRouteFilterPrefixes() != null && other.getRouteFilterPrefixes().equals(this.getRouteFilterPrefixes()) == false)
return false;
if (other.getBgpPeers() == null ^ this.getBgpPeers() == null)
return false;
if (other.getBgpPeers() != null && other.getBgpPeers().equals(this.getBgpPeers()) == false)
return false;
if (other.getRegion() == null ^ this.getRegion() == null)
return false;
if (other.getRegion() != null && other.getRegion().equals(this.getRegion()) == false)
return false;
if (other.getAwsDeviceV2() == null ^ this.getAwsDeviceV2() == null)
return false;
if (other.getAwsDeviceV2() != null && other.getAwsDeviceV2().equals(this.getAwsDeviceV2()) == false)
return false;
if (other.getAwsLogicalDeviceId() == null ^ this.getAwsLogicalDeviceId() == null)
return false;
if (other.getAwsLogicalDeviceId() != null && other.getAwsLogicalDeviceId().equals(this.getAwsLogicalDeviceId()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getSiteLinkEnabled() == null ^ this.getSiteLinkEnabled() == null)
return false;
if (other.getSiteLinkEnabled() != null && other.getSiteLinkEnabled().equals(this.getSiteLinkEnabled()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getOwnerAccount() == null) ? 0 : getOwnerAccount().hashCode());
hashCode = prime * hashCode + ((getVirtualInterfaceId() == null) ? 0 : getVirtualInterfaceId().hashCode());
hashCode = prime * hashCode + ((getLocation() == null) ? 0 : getLocation().hashCode());
hashCode = prime * hashCode + ((getConnectionId() == null) ? 0 : getConnectionId().hashCode());
hashCode = prime * hashCode + ((getVirtualInterfaceType() == null) ? 0 : getVirtualInterfaceType().hashCode());
hashCode = prime * hashCode + ((getVirtualInterfaceName() == null) ? 0 : getVirtualInterfaceName().hashCode());
hashCode = prime * hashCode + ((getVlan() == null) ? 0 : getVlan().hashCode());
hashCode = prime * hashCode + ((getAsn() == null) ? 0 : getAsn().hashCode());
hashCode = prime * hashCode + ((getAmazonSideAsn() == null) ? 0 : getAmazonSideAsn().hashCode());
hashCode = prime * hashCode + ((getAuthKey() == null) ? 0 : getAuthKey().hashCode());
hashCode = prime * hashCode + ((getAmazonAddress() == null) ? 0 : getAmazonAddress().hashCode());
hashCode = prime * hashCode + ((getCustomerAddress() == null) ? 0 : getCustomerAddress().hashCode());
hashCode = prime * hashCode + ((getAddressFamily() == null) ? 0 : getAddressFamily().hashCode());
hashCode = prime * hashCode + ((getVirtualInterfaceState() == null) ? 0 : getVirtualInterfaceState().hashCode());
hashCode = prime * hashCode + ((getCustomerRouterConfig() == null) ? 0 : getCustomerRouterConfig().hashCode());
hashCode = prime * hashCode + ((getMtu() == null) ? 0 : getMtu().hashCode());
hashCode = prime * hashCode + ((getJumboFrameCapable() == null) ? 0 : getJumboFrameCapable().hashCode());
hashCode = prime * hashCode + ((getVirtualGatewayId() == null) ? 0 : getVirtualGatewayId().hashCode());
hashCode = prime * hashCode + ((getDirectConnectGatewayId() == null) ? 0 : getDirectConnectGatewayId().hashCode());
hashCode = prime * hashCode + ((getRouteFilterPrefixes() == null) ? 0 : getRouteFilterPrefixes().hashCode());
hashCode = prime * hashCode + ((getBgpPeers() == null) ? 0 : getBgpPeers().hashCode());
hashCode = prime * hashCode + ((getRegion() == null) ? 0 : getRegion().hashCode());
hashCode = prime * hashCode + ((getAwsDeviceV2() == null) ? 0 : getAwsDeviceV2().hashCode());
hashCode = prime * hashCode + ((getAwsLogicalDeviceId() == null) ? 0 : getAwsLogicalDeviceId().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getSiteLinkEnabled() == null) ? 0 : getSiteLinkEnabled().hashCode());
return hashCode;
}
@Override
public AllocatePrivateVirtualInterfaceResult clone() {
try {
return (AllocatePrivateVirtualInterfaceResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}