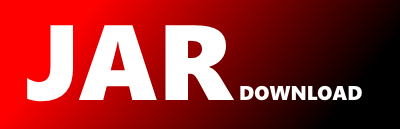
com.amazonaws.services.directconnect.model.BGPPeer Maven / Gradle / Ivy
Show all versions of aws-java-sdk-directconnect Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.directconnect.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Information about a BGP peer.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class BGPPeer implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ID of the BGP peer.
*
*/
private String bgpPeerId;
/**
*
* The autonomous system (AS) number for Border Gateway Protocol (BGP) configuration.
*
*/
private Integer asn;
/**
*
* The authentication key for BGP configuration. This string has a minimum length of 6 characters and and a maximun
* lenth of 80 characters.
*
*/
private String authKey;
/**
*
* The address family for the BGP peer.
*
*/
private String addressFamily;
/**
*
* The IP address assigned to the Amazon interface.
*
*/
private String amazonAddress;
/**
*
* The IP address assigned to the customer interface.
*
*/
private String customerAddress;
/**
*
* The state of the BGP peer. The following are the possible values:
*
*
* -
*
* verifying
: The BGP peering addresses or ASN require validation before the BGP peer can be created.
* This state applies only to public virtual interfaces.
*
*
* -
*
* pending
: The BGP peer is created, and remains in this state until it is ready to be established.
*
*
* -
*
* available
: The BGP peer is ready to be established.
*
*
* -
*
* deleting
: The BGP peer is being deleted.
*
*
* -
*
* deleted
: The BGP peer is deleted and cannot be established.
*
*
*
*/
private String bgpPeerState;
/**
*
* The status of the BGP peer. The following are the possible values:
*
*
* -
*
* up
: The BGP peer is established. This state does not indicate the state of the routing function.
* Ensure that you are receiving routes over the BGP session.
*
*
* -
*
* down
: The BGP peer is down.
*
*
* -
*
* unknown
: The BGP peer status is not available.
*
*
*
*/
private String bgpStatus;
/**
*
* The Direct Connect endpoint that terminates the BGP peer.
*
*/
private String awsDeviceV2;
/**
*
* The Direct Connect endpoint that terminates the logical connection. This device might be different than the
* device that terminates the physical connection.
*
*/
private String awsLogicalDeviceId;
/**
*
* The ID of the BGP peer.
*
*
* @param bgpPeerId
* The ID of the BGP peer.
*/
public void setBgpPeerId(String bgpPeerId) {
this.bgpPeerId = bgpPeerId;
}
/**
*
* The ID of the BGP peer.
*
*
* @return The ID of the BGP peer.
*/
public String getBgpPeerId() {
return this.bgpPeerId;
}
/**
*
* The ID of the BGP peer.
*
*
* @param bgpPeerId
* The ID of the BGP peer.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BGPPeer withBgpPeerId(String bgpPeerId) {
setBgpPeerId(bgpPeerId);
return this;
}
/**
*
* The autonomous system (AS) number for Border Gateway Protocol (BGP) configuration.
*
*
* @param asn
* The autonomous system (AS) number for Border Gateway Protocol (BGP) configuration.
*/
public void setAsn(Integer asn) {
this.asn = asn;
}
/**
*
* The autonomous system (AS) number for Border Gateway Protocol (BGP) configuration.
*
*
* @return The autonomous system (AS) number for Border Gateway Protocol (BGP) configuration.
*/
public Integer getAsn() {
return this.asn;
}
/**
*
* The autonomous system (AS) number for Border Gateway Protocol (BGP) configuration.
*
*
* @param asn
* The autonomous system (AS) number for Border Gateway Protocol (BGP) configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BGPPeer withAsn(Integer asn) {
setAsn(asn);
return this;
}
/**
*
* The authentication key for BGP configuration. This string has a minimum length of 6 characters and and a maximun
* lenth of 80 characters.
*
*
* @param authKey
* The authentication key for BGP configuration. This string has a minimum length of 6 characters and and a
* maximun lenth of 80 characters.
*/
public void setAuthKey(String authKey) {
this.authKey = authKey;
}
/**
*
* The authentication key for BGP configuration. This string has a minimum length of 6 characters and and a maximun
* lenth of 80 characters.
*
*
* @return The authentication key for BGP configuration. This string has a minimum length of 6 characters and and a
* maximun lenth of 80 characters.
*/
public String getAuthKey() {
return this.authKey;
}
/**
*
* The authentication key for BGP configuration. This string has a minimum length of 6 characters and and a maximun
* lenth of 80 characters.
*
*
* @param authKey
* The authentication key for BGP configuration. This string has a minimum length of 6 characters and and a
* maximun lenth of 80 characters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BGPPeer withAuthKey(String authKey) {
setAuthKey(authKey);
return this;
}
/**
*
* The address family for the BGP peer.
*
*
* @param addressFamily
* The address family for the BGP peer.
* @see AddressFamily
*/
public void setAddressFamily(String addressFamily) {
this.addressFamily = addressFamily;
}
/**
*
* The address family for the BGP peer.
*
*
* @return The address family for the BGP peer.
* @see AddressFamily
*/
public String getAddressFamily() {
return this.addressFamily;
}
/**
*
* The address family for the BGP peer.
*
*
* @param addressFamily
* The address family for the BGP peer.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AddressFamily
*/
public BGPPeer withAddressFamily(String addressFamily) {
setAddressFamily(addressFamily);
return this;
}
/**
*
* The address family for the BGP peer.
*
*
* @param addressFamily
* The address family for the BGP peer.
* @see AddressFamily
*/
public void setAddressFamily(AddressFamily addressFamily) {
withAddressFamily(addressFamily);
}
/**
*
* The address family for the BGP peer.
*
*
* @param addressFamily
* The address family for the BGP peer.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AddressFamily
*/
public BGPPeer withAddressFamily(AddressFamily addressFamily) {
this.addressFamily = addressFamily.toString();
return this;
}
/**
*
* The IP address assigned to the Amazon interface.
*
*
* @param amazonAddress
* The IP address assigned to the Amazon interface.
*/
public void setAmazonAddress(String amazonAddress) {
this.amazonAddress = amazonAddress;
}
/**
*
* The IP address assigned to the Amazon interface.
*
*
* @return The IP address assigned to the Amazon interface.
*/
public String getAmazonAddress() {
return this.amazonAddress;
}
/**
*
* The IP address assigned to the Amazon interface.
*
*
* @param amazonAddress
* The IP address assigned to the Amazon interface.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BGPPeer withAmazonAddress(String amazonAddress) {
setAmazonAddress(amazonAddress);
return this;
}
/**
*
* The IP address assigned to the customer interface.
*
*
* @param customerAddress
* The IP address assigned to the customer interface.
*/
public void setCustomerAddress(String customerAddress) {
this.customerAddress = customerAddress;
}
/**
*
* The IP address assigned to the customer interface.
*
*
* @return The IP address assigned to the customer interface.
*/
public String getCustomerAddress() {
return this.customerAddress;
}
/**
*
* The IP address assigned to the customer interface.
*
*
* @param customerAddress
* The IP address assigned to the customer interface.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BGPPeer withCustomerAddress(String customerAddress) {
setCustomerAddress(customerAddress);
return this;
}
/**
*
* The state of the BGP peer. The following are the possible values:
*
*
* -
*
* verifying
: The BGP peering addresses or ASN require validation before the BGP peer can be created.
* This state applies only to public virtual interfaces.
*
*
* -
*
* pending
: The BGP peer is created, and remains in this state until it is ready to be established.
*
*
* -
*
* available
: The BGP peer is ready to be established.
*
*
* -
*
* deleting
: The BGP peer is being deleted.
*
*
* -
*
* deleted
: The BGP peer is deleted and cannot be established.
*
*
*
*
* @param bgpPeerState
* The state of the BGP peer. The following are the possible values:
*
* -
*
* verifying
: The BGP peering addresses or ASN require validation before the BGP peer can be
* created. This state applies only to public virtual interfaces.
*
*
* -
*
* pending
: The BGP peer is created, and remains in this state until it is ready to be
* established.
*
*
* -
*
* available
: The BGP peer is ready to be established.
*
*
* -
*
* deleting
: The BGP peer is being deleted.
*
*
* -
*
* deleted
: The BGP peer is deleted and cannot be established.
*
*
* @see BGPPeerState
*/
public void setBgpPeerState(String bgpPeerState) {
this.bgpPeerState = bgpPeerState;
}
/**
*
* The state of the BGP peer. The following are the possible values:
*
*
* -
*
* verifying
: The BGP peering addresses or ASN require validation before the BGP peer can be created.
* This state applies only to public virtual interfaces.
*
*
* -
*
* pending
: The BGP peer is created, and remains in this state until it is ready to be established.
*
*
* -
*
* available
: The BGP peer is ready to be established.
*
*
* -
*
* deleting
: The BGP peer is being deleted.
*
*
* -
*
* deleted
: The BGP peer is deleted and cannot be established.
*
*
*
*
* @return The state of the BGP peer. The following are the possible values:
*
* -
*
* verifying
: The BGP peering addresses or ASN require validation before the BGP peer can be
* created. This state applies only to public virtual interfaces.
*
*
* -
*
* pending
: The BGP peer is created, and remains in this state until it is ready to be
* established.
*
*
* -
*
* available
: The BGP peer is ready to be established.
*
*
* -
*
* deleting
: The BGP peer is being deleted.
*
*
* -
*
* deleted
: The BGP peer is deleted and cannot be established.
*
*
* @see BGPPeerState
*/
public String getBgpPeerState() {
return this.bgpPeerState;
}
/**
*
* The state of the BGP peer. The following are the possible values:
*
*
* -
*
* verifying
: The BGP peering addresses or ASN require validation before the BGP peer can be created.
* This state applies only to public virtual interfaces.
*
*
* -
*
* pending
: The BGP peer is created, and remains in this state until it is ready to be established.
*
*
* -
*
* available
: The BGP peer is ready to be established.
*
*
* -
*
* deleting
: The BGP peer is being deleted.
*
*
* -
*
* deleted
: The BGP peer is deleted and cannot be established.
*
*
*
*
* @param bgpPeerState
* The state of the BGP peer. The following are the possible values:
*
* -
*
* verifying
: The BGP peering addresses or ASN require validation before the BGP peer can be
* created. This state applies only to public virtual interfaces.
*
*
* -
*
* pending
: The BGP peer is created, and remains in this state until it is ready to be
* established.
*
*
* -
*
* available
: The BGP peer is ready to be established.
*
*
* -
*
* deleting
: The BGP peer is being deleted.
*
*
* -
*
* deleted
: The BGP peer is deleted and cannot be established.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see BGPPeerState
*/
public BGPPeer withBgpPeerState(String bgpPeerState) {
setBgpPeerState(bgpPeerState);
return this;
}
/**
*
* The state of the BGP peer. The following are the possible values:
*
*
* -
*
* verifying
: The BGP peering addresses or ASN require validation before the BGP peer can be created.
* This state applies only to public virtual interfaces.
*
*
* -
*
* pending
: The BGP peer is created, and remains in this state until it is ready to be established.
*
*
* -
*
* available
: The BGP peer is ready to be established.
*
*
* -
*
* deleting
: The BGP peer is being deleted.
*
*
* -
*
* deleted
: The BGP peer is deleted and cannot be established.
*
*
*
*
* @param bgpPeerState
* The state of the BGP peer. The following are the possible values:
*
* -
*
* verifying
: The BGP peering addresses or ASN require validation before the BGP peer can be
* created. This state applies only to public virtual interfaces.
*
*
* -
*
* pending
: The BGP peer is created, and remains in this state until it is ready to be
* established.
*
*
* -
*
* available
: The BGP peer is ready to be established.
*
*
* -
*
* deleting
: The BGP peer is being deleted.
*
*
* -
*
* deleted
: The BGP peer is deleted and cannot be established.
*
*
* @see BGPPeerState
*/
public void setBgpPeerState(BGPPeerState bgpPeerState) {
withBgpPeerState(bgpPeerState);
}
/**
*
* The state of the BGP peer. The following are the possible values:
*
*
* -
*
* verifying
: The BGP peering addresses or ASN require validation before the BGP peer can be created.
* This state applies only to public virtual interfaces.
*
*
* -
*
* pending
: The BGP peer is created, and remains in this state until it is ready to be established.
*
*
* -
*
* available
: The BGP peer is ready to be established.
*
*
* -
*
* deleting
: The BGP peer is being deleted.
*
*
* -
*
* deleted
: The BGP peer is deleted and cannot be established.
*
*
*
*
* @param bgpPeerState
* The state of the BGP peer. The following are the possible values:
*
* -
*
* verifying
: The BGP peering addresses or ASN require validation before the BGP peer can be
* created. This state applies only to public virtual interfaces.
*
*
* -
*
* pending
: The BGP peer is created, and remains in this state until it is ready to be
* established.
*
*
* -
*
* available
: The BGP peer is ready to be established.
*
*
* -
*
* deleting
: The BGP peer is being deleted.
*
*
* -
*
* deleted
: The BGP peer is deleted and cannot be established.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see BGPPeerState
*/
public BGPPeer withBgpPeerState(BGPPeerState bgpPeerState) {
this.bgpPeerState = bgpPeerState.toString();
return this;
}
/**
*
* The status of the BGP peer. The following are the possible values:
*
*
* -
*
* up
: The BGP peer is established. This state does not indicate the state of the routing function.
* Ensure that you are receiving routes over the BGP session.
*
*
* -
*
* down
: The BGP peer is down.
*
*
* -
*
* unknown
: The BGP peer status is not available.
*
*
*
*
* @param bgpStatus
* The status of the BGP peer. The following are the possible values:
*
* -
*
* up
: The BGP peer is established. This state does not indicate the state of the routing
* function. Ensure that you are receiving routes over the BGP session.
*
*
* -
*
* down
: The BGP peer is down.
*
*
* -
*
* unknown
: The BGP peer status is not available.
*
*
* @see BGPStatus
*/
public void setBgpStatus(String bgpStatus) {
this.bgpStatus = bgpStatus;
}
/**
*
* The status of the BGP peer. The following are the possible values:
*
*
* -
*
* up
: The BGP peer is established. This state does not indicate the state of the routing function.
* Ensure that you are receiving routes over the BGP session.
*
*
* -
*
* down
: The BGP peer is down.
*
*
* -
*
* unknown
: The BGP peer status is not available.
*
*
*
*
* @return The status of the BGP peer. The following are the possible values:
*
* -
*
* up
: The BGP peer is established. This state does not indicate the state of the routing
* function. Ensure that you are receiving routes over the BGP session.
*
*
* -
*
* down
: The BGP peer is down.
*
*
* -
*
* unknown
: The BGP peer status is not available.
*
*
* @see BGPStatus
*/
public String getBgpStatus() {
return this.bgpStatus;
}
/**
*
* The status of the BGP peer. The following are the possible values:
*
*
* -
*
* up
: The BGP peer is established. This state does not indicate the state of the routing function.
* Ensure that you are receiving routes over the BGP session.
*
*
* -
*
* down
: The BGP peer is down.
*
*
* -
*
* unknown
: The BGP peer status is not available.
*
*
*
*
* @param bgpStatus
* The status of the BGP peer. The following are the possible values:
*
* -
*
* up
: The BGP peer is established. This state does not indicate the state of the routing
* function. Ensure that you are receiving routes over the BGP session.
*
*
* -
*
* down
: The BGP peer is down.
*
*
* -
*
* unknown
: The BGP peer status is not available.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see BGPStatus
*/
public BGPPeer withBgpStatus(String bgpStatus) {
setBgpStatus(bgpStatus);
return this;
}
/**
*
* The status of the BGP peer. The following are the possible values:
*
*
* -
*
* up
: The BGP peer is established. This state does not indicate the state of the routing function.
* Ensure that you are receiving routes over the BGP session.
*
*
* -
*
* down
: The BGP peer is down.
*
*
* -
*
* unknown
: The BGP peer status is not available.
*
*
*
*
* @param bgpStatus
* The status of the BGP peer. The following are the possible values:
*
* -
*
* up
: The BGP peer is established. This state does not indicate the state of the routing
* function. Ensure that you are receiving routes over the BGP session.
*
*
* -
*
* down
: The BGP peer is down.
*
*
* -
*
* unknown
: The BGP peer status is not available.
*
*
* @see BGPStatus
*/
public void setBgpStatus(BGPStatus bgpStatus) {
withBgpStatus(bgpStatus);
}
/**
*
* The status of the BGP peer. The following are the possible values:
*
*
* -
*
* up
: The BGP peer is established. This state does not indicate the state of the routing function.
* Ensure that you are receiving routes over the BGP session.
*
*
* -
*
* down
: The BGP peer is down.
*
*
* -
*
* unknown
: The BGP peer status is not available.
*
*
*
*
* @param bgpStatus
* The status of the BGP peer. The following are the possible values:
*
* -
*
* up
: The BGP peer is established. This state does not indicate the state of the routing
* function. Ensure that you are receiving routes over the BGP session.
*
*
* -
*
* down
: The BGP peer is down.
*
*
* -
*
* unknown
: The BGP peer status is not available.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see BGPStatus
*/
public BGPPeer withBgpStatus(BGPStatus bgpStatus) {
this.bgpStatus = bgpStatus.toString();
return this;
}
/**
*
* The Direct Connect endpoint that terminates the BGP peer.
*
*
* @param awsDeviceV2
* The Direct Connect endpoint that terminates the BGP peer.
*/
public void setAwsDeviceV2(String awsDeviceV2) {
this.awsDeviceV2 = awsDeviceV2;
}
/**
*
* The Direct Connect endpoint that terminates the BGP peer.
*
*
* @return The Direct Connect endpoint that terminates the BGP peer.
*/
public String getAwsDeviceV2() {
return this.awsDeviceV2;
}
/**
*
* The Direct Connect endpoint that terminates the BGP peer.
*
*
* @param awsDeviceV2
* The Direct Connect endpoint that terminates the BGP peer.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BGPPeer withAwsDeviceV2(String awsDeviceV2) {
setAwsDeviceV2(awsDeviceV2);
return this;
}
/**
*
* The Direct Connect endpoint that terminates the logical connection. This device might be different than the
* device that terminates the physical connection.
*
*
* @param awsLogicalDeviceId
* The Direct Connect endpoint that terminates the logical connection. This device might be different than
* the device that terminates the physical connection.
*/
public void setAwsLogicalDeviceId(String awsLogicalDeviceId) {
this.awsLogicalDeviceId = awsLogicalDeviceId;
}
/**
*
* The Direct Connect endpoint that terminates the logical connection. This device might be different than the
* device that terminates the physical connection.
*
*
* @return The Direct Connect endpoint that terminates the logical connection. This device might be different than
* the device that terminates the physical connection.
*/
public String getAwsLogicalDeviceId() {
return this.awsLogicalDeviceId;
}
/**
*
* The Direct Connect endpoint that terminates the logical connection. This device might be different than the
* device that terminates the physical connection.
*
*
* @param awsLogicalDeviceId
* The Direct Connect endpoint that terminates the logical connection. This device might be different than
* the device that terminates the physical connection.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BGPPeer withAwsLogicalDeviceId(String awsLogicalDeviceId) {
setAwsLogicalDeviceId(awsLogicalDeviceId);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getBgpPeerId() != null)
sb.append("BgpPeerId: ").append(getBgpPeerId()).append(",");
if (getAsn() != null)
sb.append("Asn: ").append(getAsn()).append(",");
if (getAuthKey() != null)
sb.append("AuthKey: ").append(getAuthKey()).append(",");
if (getAddressFamily() != null)
sb.append("AddressFamily: ").append(getAddressFamily()).append(",");
if (getAmazonAddress() != null)
sb.append("AmazonAddress: ").append(getAmazonAddress()).append(",");
if (getCustomerAddress() != null)
sb.append("CustomerAddress: ").append(getCustomerAddress()).append(",");
if (getBgpPeerState() != null)
sb.append("BgpPeerState: ").append(getBgpPeerState()).append(",");
if (getBgpStatus() != null)
sb.append("BgpStatus: ").append(getBgpStatus()).append(",");
if (getAwsDeviceV2() != null)
sb.append("AwsDeviceV2: ").append(getAwsDeviceV2()).append(",");
if (getAwsLogicalDeviceId() != null)
sb.append("AwsLogicalDeviceId: ").append(getAwsLogicalDeviceId());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof BGPPeer == false)
return false;
BGPPeer other = (BGPPeer) obj;
if (other.getBgpPeerId() == null ^ this.getBgpPeerId() == null)
return false;
if (other.getBgpPeerId() != null && other.getBgpPeerId().equals(this.getBgpPeerId()) == false)
return false;
if (other.getAsn() == null ^ this.getAsn() == null)
return false;
if (other.getAsn() != null && other.getAsn().equals(this.getAsn()) == false)
return false;
if (other.getAuthKey() == null ^ this.getAuthKey() == null)
return false;
if (other.getAuthKey() != null && other.getAuthKey().equals(this.getAuthKey()) == false)
return false;
if (other.getAddressFamily() == null ^ this.getAddressFamily() == null)
return false;
if (other.getAddressFamily() != null && other.getAddressFamily().equals(this.getAddressFamily()) == false)
return false;
if (other.getAmazonAddress() == null ^ this.getAmazonAddress() == null)
return false;
if (other.getAmazonAddress() != null && other.getAmazonAddress().equals(this.getAmazonAddress()) == false)
return false;
if (other.getCustomerAddress() == null ^ this.getCustomerAddress() == null)
return false;
if (other.getCustomerAddress() != null && other.getCustomerAddress().equals(this.getCustomerAddress()) == false)
return false;
if (other.getBgpPeerState() == null ^ this.getBgpPeerState() == null)
return false;
if (other.getBgpPeerState() != null && other.getBgpPeerState().equals(this.getBgpPeerState()) == false)
return false;
if (other.getBgpStatus() == null ^ this.getBgpStatus() == null)
return false;
if (other.getBgpStatus() != null && other.getBgpStatus().equals(this.getBgpStatus()) == false)
return false;
if (other.getAwsDeviceV2() == null ^ this.getAwsDeviceV2() == null)
return false;
if (other.getAwsDeviceV2() != null && other.getAwsDeviceV2().equals(this.getAwsDeviceV2()) == false)
return false;
if (other.getAwsLogicalDeviceId() == null ^ this.getAwsLogicalDeviceId() == null)
return false;
if (other.getAwsLogicalDeviceId() != null && other.getAwsLogicalDeviceId().equals(this.getAwsLogicalDeviceId()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getBgpPeerId() == null) ? 0 : getBgpPeerId().hashCode());
hashCode = prime * hashCode + ((getAsn() == null) ? 0 : getAsn().hashCode());
hashCode = prime * hashCode + ((getAuthKey() == null) ? 0 : getAuthKey().hashCode());
hashCode = prime * hashCode + ((getAddressFamily() == null) ? 0 : getAddressFamily().hashCode());
hashCode = prime * hashCode + ((getAmazonAddress() == null) ? 0 : getAmazonAddress().hashCode());
hashCode = prime * hashCode + ((getCustomerAddress() == null) ? 0 : getCustomerAddress().hashCode());
hashCode = prime * hashCode + ((getBgpPeerState() == null) ? 0 : getBgpPeerState().hashCode());
hashCode = prime * hashCode + ((getBgpStatus() == null) ? 0 : getBgpStatus().hashCode());
hashCode = prime * hashCode + ((getAwsDeviceV2() == null) ? 0 : getAwsDeviceV2().hashCode());
hashCode = prime * hashCode + ((getAwsLogicalDeviceId() == null) ? 0 : getAwsLogicalDeviceId().hashCode());
return hashCode;
}
@Override
public BGPPeer clone() {
try {
return (BGPPeer) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.directconnect.model.transform.BGPPeerMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}