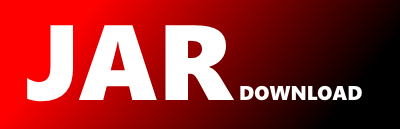
com.amazonaws.services.directory.AWSDirectoryService Maven / Gradle / Ivy
Show all versions of aws-java-sdk-directory Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.directory;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.directory.model.*;
/**
* Interface for accessing Directory Service.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.directory.AbstractAWSDirectoryService} instead.
*
*
* AWS Directory Service
*
* AWS Directory Service is a web service that makes it easy for you to setup and run directories in the AWS cloud, or
* connect your AWS resources with an existing on-premises Microsoft Active Directory. This guide provides detailed
* information about AWS Directory Service operations, data types, parameters, and errors. For information about AWS
* Directory Services features, see AWS Directory Service and the
* AWS Directory Service
* Administration Guide.
*
*
*
* AWS provides SDKs that consist of libraries and sample code for various programming languages and platforms (Java,
* Ruby, .Net, iOS, Android, etc.). The SDKs provide a convenient way to create programmatic access to AWS Directory
* Service and other AWS services. For more information about the AWS SDKs, including how to download and install them,
* see Tools for Amazon Web Services.
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSDirectoryService {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "ds";
/**
* Overrides the default endpoint for this client ("https://ds.us-east-1.amazonaws.com"). Callers can use this
* method to control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex: "ds.us-east-1.amazonaws.com") or a full URL, including the protocol
* (ex: "https://ds.us-east-1.amazonaws.com"). If the protocol is not specified here, the default protocol from this
* client's {@link ClientConfiguration} will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and a complete list of all available
* endpoints for all AWS services, see: https://docs.aws.amazon.com/sdk-for-java/v1/developer-guide/java-dg-region-selection.html#region-selection-
* choose-endpoint
*
* This method is not threadsafe. An endpoint should be configured when the client is created and before any
* service requests are made. Changing it afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param endpoint
* The endpoint (ex: "ds.us-east-1.amazonaws.com") or a full URL, including the protocol (ex:
* "https://ds.us-east-1.amazonaws.com") of the region specific AWS endpoint this client will communicate
* with.
* @deprecated use {@link AwsClientBuilder#setEndpointConfiguration(AwsClientBuilder.EndpointConfiguration)} for
* example:
* {@code builder.setEndpointConfiguration(new EndpointConfiguration(endpoint, signingRegion));}
*/
@Deprecated
void setEndpoint(String endpoint);
/**
* An alternative to {@link AWSDirectoryService#setEndpoint(String)}, sets the regional endpoint for this client's
* service calls. Callers can use this method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol. To use http instead, specify it in the
* {@link ClientConfiguration} supplied at construction.
*
* This method is not threadsafe. A region should be configured when the client is created and before any service
* requests are made. Changing it afterwards creates inevitable race conditions for any service requests in transit
* or retrying.
*
* @param region
* The region this client will communicate with. See {@link Region#getRegion(com.amazonaws.regions.Regions)}
* for accessing a given region. Must not be null and must be a region where the service is available.
*
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class, com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
* @see Region#isServiceSupported(String)
* @deprecated use {@link AwsClientBuilder#setRegion(String)}
*/
@Deprecated
void setRegion(Region region);
/**
*
* Accepts a directory sharing request that was sent from the directory owner account.
*
*
* @param acceptSharedDirectoryRequest
* @return Result of the AcceptSharedDirectory operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws DirectoryAlreadySharedException
* The specified directory has already been shared with this AWS account.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.AcceptSharedDirectory
* @see AWS API
* Documentation
*/
AcceptSharedDirectoryResult acceptSharedDirectory(AcceptSharedDirectoryRequest acceptSharedDirectoryRequest);
/**
*
* If the DNS server for your on-premises domain uses a publicly addressable IP address, you must add a CIDR address
* block to correctly route traffic to and from your Microsoft AD on Amazon Web Services. AddIpRoutes adds
* this address block. You can also use AddIpRoutes to facilitate routing traffic that uses public IP ranges
* from your Microsoft AD on AWS to a peer VPC.
*
*
* Before you call AddIpRoutes, ensure that all of the required permissions have been explicitly granted
* through a policy. For details about what permissions are required to run the AddIpRoutes operation, see AWS
* Directory Service API Permissions: Actions, Resources, and Conditions Reference.
*
*
* @param addIpRoutesRequest
* @return Result of the AddIpRoutes operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws EntityAlreadyExistsException
* The specified entity already exists.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws IpRouteLimitExceededException
* The maximum allowed number of IP addresses was exceeded. The default limit is 100 IP address blocks.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.AddIpRoutes
* @see AWS API
* Documentation
*/
AddIpRoutesResult addIpRoutes(AddIpRoutesRequest addIpRoutesRequest);
/**
*
* Adds or overwrites one or more tags for the specified directory. Each directory can have a maximum of 50 tags.
* Each tag consists of a key and optional value. Tag keys must be unique to each resource.
*
*
* @param addTagsToResourceRequest
* @return Result of the AddTagsToResource operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws TagLimitExceededException
* The maximum allowed number of tags was exceeded.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.AddTagsToResource
* @see AWS API
* Documentation
*/
AddTagsToResourceResult addTagsToResource(AddTagsToResourceRequest addTagsToResourceRequest);
/**
*
* Cancels an in-progress schema extension to a Microsoft AD directory. Once a schema extension has started
* replicating to all domain controllers, the task can no longer be canceled. A schema extension can be canceled
* during any of the following states; Initializing
, CreatingSnapshot
, and
* UpdatingSchema
.
*
*
* @param cancelSchemaExtensionRequest
* @return Result of the CancelSchemaExtension operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.CancelSchemaExtension
* @see AWS API
* Documentation
*/
CancelSchemaExtensionResult cancelSchemaExtension(CancelSchemaExtensionRequest cancelSchemaExtensionRequest);
/**
*
* Creates an AD Connector to connect to an on-premises directory.
*
*
* Before you call ConnectDirectory
, ensure that all of the required permissions have been explicitly
* granted through a policy. For details about what permissions are required to run the
* ConnectDirectory
operation, see AWS Directory Service API Permissions: Actions, Resources, and Conditions Reference.
*
*
* @param connectDirectoryRequest
* Contains the inputs for the ConnectDirectory operation.
* @return Result of the ConnectDirectory operation returned by the service.
* @throws DirectoryLimitExceededException
* The maximum number of directories in the region has been reached. You can use the
* GetDirectoryLimits operation to determine your directory limits in the region.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.ConnectDirectory
* @see AWS API
* Documentation
*/
ConnectDirectoryResult connectDirectory(ConnectDirectoryRequest connectDirectoryRequest);
/**
*
* Creates an alias for a directory and assigns the alias to the directory. The alias is used to construct the
* access URL for the directory, such as http://<alias>.awsapps.com
.
*
*
*
* After an alias has been created, it cannot be deleted or reused, so this operation should only be used when
* absolutely necessary.
*
*
*
* @param createAliasRequest
* Contains the inputs for the CreateAlias operation.
* @return Result of the CreateAlias operation returned by the service.
* @throws EntityAlreadyExistsException
* The specified entity already exists.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.CreateAlias
* @see AWS API
* Documentation
*/
CreateAliasResult createAlias(CreateAliasRequest createAliasRequest);
/**
*
* Creates a computer account in the specified directory, and joins the computer to the directory.
*
*
* @param createComputerRequest
* Contains the inputs for the CreateComputer operation.
* @return Result of the CreateComputer operation returned by the service.
* @throws AuthenticationFailedException
* An authentication error occurred.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws EntityAlreadyExistsException
* The specified entity already exists.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.CreateComputer
* @see AWS API
* Documentation
*/
CreateComputerResult createComputer(CreateComputerRequest createComputerRequest);
/**
*
* Creates a conditional forwarder associated with your AWS directory. Conditional forwarders are required in order
* to set up a trust relationship with another domain. The conditional forwarder points to the trusted domain.
*
*
* @param createConditionalForwarderRequest
* Initiates the creation of a conditional forwarder for your AWS Directory Service for Microsoft Active
* Directory. Conditional forwarders are required in order to set up a trust relationship with another
* domain.
* @return Result of the CreateConditionalForwarder operation returned by the service.
* @throws EntityAlreadyExistsException
* The specified entity already exists.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.CreateConditionalForwarder
* @see AWS
* API Documentation
*/
CreateConditionalForwarderResult createConditionalForwarder(CreateConditionalForwarderRequest createConditionalForwarderRequest);
/**
*
* Creates a Simple AD directory. For more information, see Simple Active
* Directory in the AWS Directory Service Admin Guide.
*
*
* Before you call CreateDirectory
, ensure that all of the required permissions have been explicitly
* granted through a policy. For details about what permissions are required to run the CreateDirectory
* operation, see AWS Directory Service API Permissions: Actions, Resources, and Conditions Reference.
*
*
* @param createDirectoryRequest
* Contains the inputs for the CreateDirectory operation.
* @return Result of the CreateDirectory operation returned by the service.
* @throws DirectoryLimitExceededException
* The maximum number of directories in the region has been reached. You can use the
* GetDirectoryLimits operation to determine your directory limits in the region.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.CreateDirectory
* @see AWS API
* Documentation
*/
CreateDirectoryResult createDirectory(CreateDirectoryRequest createDirectoryRequest);
/**
*
* Creates a subscription to forward real-time Directory Service domain controller security logs to the specified
* Amazon CloudWatch log group in your AWS account.
*
*
* @param createLogSubscriptionRequest
* @return Result of the CreateLogSubscription operation returned by the service.
* @throws EntityAlreadyExistsException
* The specified entity already exists.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws InsufficientPermissionsException
* The account does not have sufficient permission to perform the operation.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.CreateLogSubscription
* @see AWS API
* Documentation
*/
CreateLogSubscriptionResult createLogSubscription(CreateLogSubscriptionRequest createLogSubscriptionRequest);
/**
*
* Creates a Microsoft AD directory in the AWS Cloud. For more information, see AWS Managed
* Microsoft AD in the AWS Directory Service Admin Guide.
*
*
* Before you call CreateMicrosoftAD, ensure that all of the required permissions have been explicitly
* granted through a policy. For details about what permissions are required to run the CreateMicrosoftAD
* operation, see AWS Directory Service API Permissions: Actions, Resources, and Conditions Reference.
*
*
* @param createMicrosoftADRequest
* Creates an AWS Managed Microsoft AD directory.
* @return Result of the CreateMicrosoftAD operation returned by the service.
* @throws DirectoryLimitExceededException
* The maximum number of directories in the region has been reached. You can use the
* GetDirectoryLimits operation to determine your directory limits in the region.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @throws UnsupportedOperationException
* The operation is not supported.
* @sample AWSDirectoryService.CreateMicrosoftAD
* @see AWS API
* Documentation
*/
CreateMicrosoftADResult createMicrosoftAD(CreateMicrosoftADRequest createMicrosoftADRequest);
/**
*
* Creates a snapshot of a Simple AD or Microsoft AD directory in the AWS cloud.
*
*
*
* You cannot take snapshots of AD Connector directories.
*
*
*
* @param createSnapshotRequest
* Contains the inputs for the CreateSnapshot operation.
* @return Result of the CreateSnapshot operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws SnapshotLimitExceededException
* The maximum number of manual snapshots for the directory has been reached. You can use the
* GetSnapshotLimits operation to determine the snapshot limits for a directory.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.CreateSnapshot
* @see AWS API
* Documentation
*/
CreateSnapshotResult createSnapshot(CreateSnapshotRequest createSnapshotRequest);
/**
*
* AWS Directory Service for Microsoft Active Directory allows you to configure trust relationships. For example,
* you can establish a trust between your AWS Managed Microsoft AD directory, and your existing on-premises
* Microsoft Active Directory. This would allow you to provide users and groups access to resources in either
* domain, with a single set of credentials.
*
*
* This action initiates the creation of the AWS side of a trust relationship between an AWS Managed Microsoft AD
* directory and an external domain. You can create either a forest trust or an external trust.
*
*
* @param createTrustRequest
* AWS Directory Service for Microsoft Active Directory allows you to configure trust relationships. For
* example, you can establish a trust between your AWS Managed Microsoft AD directory, and your existing
* on-premises Microsoft Active Directory. This would allow you to provide users and groups access to
* resources in either domain, with a single set of credentials.
*
* This action initiates the creation of the AWS side of a trust relationship between an AWS Managed
* Microsoft AD directory and an external domain.
* @return Result of the CreateTrust operation returned by the service.
* @throws EntityAlreadyExistsException
* The specified entity already exists.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @throws UnsupportedOperationException
* The operation is not supported.
* @sample AWSDirectoryService.CreateTrust
* @see AWS API
* Documentation
*/
CreateTrustResult createTrust(CreateTrustRequest createTrustRequest);
/**
*
* Deletes a conditional forwarder that has been set up for your AWS directory.
*
*
* @param deleteConditionalForwarderRequest
* Deletes a conditional forwarder.
* @return Result of the DeleteConditionalForwarder operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.DeleteConditionalForwarder
* @see AWS
* API Documentation
*/
DeleteConditionalForwarderResult deleteConditionalForwarder(DeleteConditionalForwarderRequest deleteConditionalForwarderRequest);
/**
*
* Deletes an AWS Directory Service directory.
*
*
* Before you call DeleteDirectory
, ensure that all of the required permissions have been explicitly
* granted through a policy. For details about what permissions are required to run the DeleteDirectory
* operation, see AWS Directory Service API Permissions: Actions, Resources, and Conditions Reference.
*
*
* @param deleteDirectoryRequest
* Contains the inputs for the DeleteDirectory operation.
* @return Result of the DeleteDirectory operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.DeleteDirectory
* @see AWS API
* Documentation
*/
DeleteDirectoryResult deleteDirectory(DeleteDirectoryRequest deleteDirectoryRequest);
/**
*
* Deletes the specified log subscription.
*
*
* @param deleteLogSubscriptionRequest
* @return Result of the DeleteLogSubscription operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.DeleteLogSubscription
* @see AWS API
* Documentation
*/
DeleteLogSubscriptionResult deleteLogSubscription(DeleteLogSubscriptionRequest deleteLogSubscriptionRequest);
/**
*
* Deletes a directory snapshot.
*
*
* @param deleteSnapshotRequest
* Contains the inputs for the DeleteSnapshot operation.
* @return Result of the DeleteSnapshot operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.DeleteSnapshot
* @see AWS API
* Documentation
*/
DeleteSnapshotResult deleteSnapshot(DeleteSnapshotRequest deleteSnapshotRequest);
/**
*
* Deletes an existing trust relationship between your AWS Managed Microsoft AD directory and an external domain.
*
*
* @param deleteTrustRequest
* Deletes the local side of an existing trust relationship between the AWS Managed Microsoft AD directory
* and the external domain.
* @return Result of the DeleteTrust operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @throws UnsupportedOperationException
* The operation is not supported.
* @sample AWSDirectoryService.DeleteTrust
* @see AWS API
* Documentation
*/
DeleteTrustResult deleteTrust(DeleteTrustRequest deleteTrustRequest);
/**
*
* Deletes from the system the certificate that was registered for a secured LDAP connection.
*
*
* @param deregisterCertificateRequest
* @return Result of the DeregisterCertificate operation returned by the service.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws CertificateDoesNotExistException
* The certificate is not present in the system for describe or deregister activities.
* @throws CertificateInUseException
* The certificate is being used for the LDAP security connection and cannot be removed without disabling
* LDAP security.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.DeregisterCertificate
* @see AWS API
* Documentation
*/
DeregisterCertificateResult deregisterCertificate(DeregisterCertificateRequest deregisterCertificateRequest);
/**
*
* Removes the specified directory as a publisher to the specified SNS topic.
*
*
* @param deregisterEventTopicRequest
* Removes the specified directory as a publisher to the specified SNS topic.
* @return Result of the DeregisterEventTopic operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.DeregisterEventTopic
* @see AWS API
* Documentation
*/
DeregisterEventTopicResult deregisterEventTopic(DeregisterEventTopicRequest deregisterEventTopicRequest);
/**
*
* Displays information about the certificate registered for a secured LDAP connection.
*
*
* @param describeCertificateRequest
* @return Result of the DescribeCertificate operation returned by the service.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws CertificateDoesNotExistException
* The certificate is not present in the system for describe or deregister activities.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.DescribeCertificate
* @see AWS API
* Documentation
*/
DescribeCertificateResult describeCertificate(DescribeCertificateRequest describeCertificateRequest);
/**
*
* Obtains information about the conditional forwarders for this account.
*
*
* If no input parameters are provided for RemoteDomainNames, this request describes all conditional forwarders for
* the specified directory ID.
*
*
* @param describeConditionalForwardersRequest
* Describes a conditional forwarder.
* @return Result of the DescribeConditionalForwarders operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.DescribeConditionalForwarders
* @see AWS API Documentation
*/
DescribeConditionalForwardersResult describeConditionalForwarders(DescribeConditionalForwardersRequest describeConditionalForwardersRequest);
/**
*
* Obtains information about the directories that belong to this account.
*
*
* You can retrieve information about specific directories by passing the directory identifiers in the
* DirectoryIds
parameter. Otherwise, all directories that belong to the current account are returned.
*
*
* This operation supports pagination with the use of the NextToken
request and response parameters. If
* more results are available, the DescribeDirectoriesResult.NextToken
member contains a token that you
* pass in the next call to DescribeDirectories to retrieve the next set of items.
*
*
* You can also specify a maximum number of return results with the Limit
parameter.
*
*
* @param describeDirectoriesRequest
* Contains the inputs for the DescribeDirectories operation.
* @return Result of the DescribeDirectories operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.DescribeDirectories
* @see AWS API
* Documentation
*/
DescribeDirectoriesResult describeDirectories(DescribeDirectoriesRequest describeDirectoriesRequest);
/**
* Simplified method form for invoking the DescribeDirectories operation.
*
* @see #describeDirectories(DescribeDirectoriesRequest)
*/
DescribeDirectoriesResult describeDirectories();
/**
*
* Provides information about any domain controllers in your directory.
*
*
* @param describeDomainControllersRequest
* @return Result of the DescribeDomainControllers operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @throws UnsupportedOperationException
* The operation is not supported.
* @sample AWSDirectoryService.DescribeDomainControllers
* @see AWS
* API Documentation
*/
DescribeDomainControllersResult describeDomainControllers(DescribeDomainControllersRequest describeDomainControllersRequest);
/**
*
* Obtains information about which SNS topics receive status messages from the specified directory.
*
*
* If no input parameters are provided, such as DirectoryId or TopicName, this request describes all of the
* associations in the account.
*
*
* @param describeEventTopicsRequest
* Describes event topics.
* @return Result of the DescribeEventTopics operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.DescribeEventTopics
* @see AWS API
* Documentation
*/
DescribeEventTopicsResult describeEventTopics(DescribeEventTopicsRequest describeEventTopicsRequest);
/**
*
* Describes the status of LDAP security for the specified directory.
*
*
* @param describeLDAPSSettingsRequest
* @return Result of the DescribeLDAPSSettings operation returned by the service.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.DescribeLDAPSSettings
* @see AWS API
* Documentation
*/
DescribeLDAPSSettingsResult describeLDAPSSettings(DescribeLDAPSSettingsRequest describeLDAPSSettingsRequest);
/**
*
* Returns the shared directories in your account.
*
*
* @param describeSharedDirectoriesRequest
* @return Result of the DescribeSharedDirectories operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.DescribeSharedDirectories
* @see AWS
* API Documentation
*/
DescribeSharedDirectoriesResult describeSharedDirectories(DescribeSharedDirectoriesRequest describeSharedDirectoriesRequest);
/**
*
* Obtains information about the directory snapshots that belong to this account.
*
*
* This operation supports pagination with the use of the NextToken request and response parameters. If more
* results are available, the DescribeSnapshots.NextToken member contains a token that you pass in the next
* call to DescribeSnapshots to retrieve the next set of items.
*
*
* You can also specify a maximum number of return results with the Limit parameter.
*
*
* @param describeSnapshotsRequest
* Contains the inputs for the DescribeSnapshots operation.
* @return Result of the DescribeSnapshots operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.DescribeSnapshots
* @see AWS API
* Documentation
*/
DescribeSnapshotsResult describeSnapshots(DescribeSnapshotsRequest describeSnapshotsRequest);
/**
* Simplified method form for invoking the DescribeSnapshots operation.
*
* @see #describeSnapshots(DescribeSnapshotsRequest)
*/
DescribeSnapshotsResult describeSnapshots();
/**
*
* Obtains information about the trust relationships for this account.
*
*
* If no input parameters are provided, such as DirectoryId or TrustIds, this request describes all the trust
* relationships belonging to the account.
*
*
* @param describeTrustsRequest
* Describes the trust relationships for a particular AWS Managed Microsoft AD directory. If no input
* parameters are are provided, such as directory ID or trust ID, this request describes all the trust
* relationships.
* @return Result of the DescribeTrusts operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @throws UnsupportedOperationException
* The operation is not supported.
* @sample AWSDirectoryService.DescribeTrusts
* @see AWS API
* Documentation
*/
DescribeTrustsResult describeTrusts(DescribeTrustsRequest describeTrustsRequest);
/**
*
* Deactivates LDAP secure calls for the specified directory.
*
*
* @param disableLDAPSRequest
* @return Result of the DisableLDAPS operation returned by the service.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws InvalidLDAPSStatusException
* The LDAP activities could not be performed because they are limited by the LDAPS status.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.DisableLDAPS
* @see AWS API
* Documentation
*/
DisableLDAPSResult disableLDAPS(DisableLDAPSRequest disableLDAPSRequest);
/**
*
* Disables multi-factor authentication (MFA) with the Remote Authentication Dial In User Service (RADIUS) server
* for an AD Connector or Microsoft AD directory.
*
*
* @param disableRadiusRequest
* Contains the inputs for the DisableRadius operation.
* @return Result of the DisableRadius operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.DisableRadius
* @see AWS API
* Documentation
*/
DisableRadiusResult disableRadius(DisableRadiusRequest disableRadiusRequest);
/**
*
* Disables single-sign on for a directory.
*
*
* @param disableSsoRequest
* Contains the inputs for the DisableSso operation.
* @return Result of the DisableSso operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InsufficientPermissionsException
* The account does not have sufficient permission to perform the operation.
* @throws AuthenticationFailedException
* An authentication error occurred.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.DisableSso
* @see AWS API
* Documentation
*/
DisableSsoResult disableSso(DisableSsoRequest disableSsoRequest);
/**
*
* Activates the switch for the specific directory to always use LDAP secure calls.
*
*
* @param enableLDAPSRequest
* @return Result of the EnableLDAPS operation returned by the service.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws NoAvailableCertificateException
* The LDAP activities could not be performed because at least one valid certificate must be registered with
* the system.
* @throws InvalidLDAPSStatusException
* The LDAP activities could not be performed because they are limited by the LDAPS status.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.EnableLDAPS
* @see AWS API
* Documentation
*/
EnableLDAPSResult enableLDAPS(EnableLDAPSRequest enableLDAPSRequest);
/**
*
* Enables multi-factor authentication (MFA) with the Remote Authentication Dial In User Service (RADIUS) server for
* an AD Connector or Microsoft AD directory.
*
*
* @param enableRadiusRequest
* Contains the inputs for the EnableRadius operation.
* @return Result of the EnableRadius operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws EntityAlreadyExistsException
* The specified entity already exists.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.EnableRadius
* @see AWS API
* Documentation
*/
EnableRadiusResult enableRadius(EnableRadiusRequest enableRadiusRequest);
/**
*
* Enables single sign-on for a directory. Single sign-on allows users in your directory to access certain AWS
* services from a computer joined to the directory without having to enter their credentials separately.
*
*
* @param enableSsoRequest
* Contains the inputs for the EnableSso operation.
* @return Result of the EnableSso operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InsufficientPermissionsException
* The account does not have sufficient permission to perform the operation.
* @throws AuthenticationFailedException
* An authentication error occurred.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.EnableSso
* @see AWS API
* Documentation
*/
EnableSsoResult enableSso(EnableSsoRequest enableSsoRequest);
/**
*
* Obtains directory limit information for the current Region.
*
*
* @param getDirectoryLimitsRequest
* Contains the inputs for the GetDirectoryLimits operation.
* @return Result of the GetDirectoryLimits operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.GetDirectoryLimits
* @see AWS API
* Documentation
*/
GetDirectoryLimitsResult getDirectoryLimits(GetDirectoryLimitsRequest getDirectoryLimitsRequest);
/**
* Simplified method form for invoking the GetDirectoryLimits operation.
*
* @see #getDirectoryLimits(GetDirectoryLimitsRequest)
*/
GetDirectoryLimitsResult getDirectoryLimits();
/**
*
* Obtains the manual snapshot limits for a directory.
*
*
* @param getSnapshotLimitsRequest
* Contains the inputs for the GetSnapshotLimits operation.
* @return Result of the GetSnapshotLimits operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.GetSnapshotLimits
* @see AWS API
* Documentation
*/
GetSnapshotLimitsResult getSnapshotLimits(GetSnapshotLimitsRequest getSnapshotLimitsRequest);
/**
*
* For the specified directory, lists all the certificates registered for a secured LDAP connection.
*
*
* @param listCertificatesRequest
* @return Result of the ListCertificates operation returned by the service.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.ListCertificates
* @see AWS API
* Documentation
*/
ListCertificatesResult listCertificates(ListCertificatesRequest listCertificatesRequest);
/**
*
* Lists the address blocks that you have added to a directory.
*
*
* @param listIpRoutesRequest
* @return Result of the ListIpRoutes operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.ListIpRoutes
* @see AWS API
* Documentation
*/
ListIpRoutesResult listIpRoutes(ListIpRoutesRequest listIpRoutesRequest);
/**
*
* Lists the active log subscriptions for the AWS account.
*
*
* @param listLogSubscriptionsRequest
* @return Result of the ListLogSubscriptions operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.ListLogSubscriptions
* @see AWS API
* Documentation
*/
ListLogSubscriptionsResult listLogSubscriptions(ListLogSubscriptionsRequest listLogSubscriptionsRequest);
/**
*
* Lists all schema extensions applied to a Microsoft AD Directory.
*
*
* @param listSchemaExtensionsRequest
* @return Result of the ListSchemaExtensions operation returned by the service.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.ListSchemaExtensions
* @see AWS API
* Documentation
*/
ListSchemaExtensionsResult listSchemaExtensions(ListSchemaExtensionsRequest listSchemaExtensionsRequest);
/**
*
* Lists all tags on a directory.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.ListTagsForResource
* @see AWS API
* Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Registers a certificate for secured LDAP connection.
*
*
* @param registerCertificateRequest
* @return Result of the RegisterCertificate operation returned by the service.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws InvalidCertificateException
* The certificate PEM that was provided has incorrect encoding.
* @throws CertificateLimitExceededException
* The certificate could not be added because the certificate limit has been reached.
* @throws CertificateAlreadyExistsException
* The certificate has already been registered into the system.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.RegisterCertificate
* @see AWS API
* Documentation
*/
RegisterCertificateResult registerCertificate(RegisterCertificateRequest registerCertificateRequest);
/**
*
* Associates a directory with an SNS topic. This establishes the directory as a publisher to the specified SNS
* topic. You can then receive email or text (SMS) messages when the status of your directory changes. You get
* notified if your directory goes from an Active status to an Impaired or Inoperable status. You also receive a
* notification when the directory returns to an Active status.
*
*
* @param registerEventTopicRequest
* Registers a new event topic.
* @return Result of the RegisterEventTopic operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.RegisterEventTopic
* @see AWS API
* Documentation
*/
RegisterEventTopicResult registerEventTopic(RegisterEventTopicRequest registerEventTopicRequest);
/**
*
* Rejects a directory sharing request that was sent from the directory owner account.
*
*
* @param rejectSharedDirectoryRequest
* @return Result of the RejectSharedDirectory operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws DirectoryAlreadySharedException
* The specified directory has already been shared with this AWS account.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.RejectSharedDirectory
* @see AWS API
* Documentation
*/
RejectSharedDirectoryResult rejectSharedDirectory(RejectSharedDirectoryRequest rejectSharedDirectoryRequest);
/**
*
* Removes IP address blocks from a directory.
*
*
* @param removeIpRoutesRequest
* @return Result of the RemoveIpRoutes operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.RemoveIpRoutes
* @see AWS API
* Documentation
*/
RemoveIpRoutesResult removeIpRoutes(RemoveIpRoutesRequest removeIpRoutesRequest);
/**
*
* Removes tags from a directory.
*
*
* @param removeTagsFromResourceRequest
* @return Result of the RemoveTagsFromResource operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.RemoveTagsFromResource
* @see AWS API
* Documentation
*/
RemoveTagsFromResourceResult removeTagsFromResource(RemoveTagsFromResourceRequest removeTagsFromResourceRequest);
/**
*
* Resets the password for any user in your AWS Managed Microsoft AD or Simple AD directory.
*
*
* You can reset the password for any user in your directory with the following exceptions:
*
*
* -
*
* For Simple AD, you cannot reset the password for any user that is a member of either the Domain Admins or
* Enterprise Admins group except for the administrator user.
*
*
* -
*
* For AWS Managed Microsoft AD, you can only reset the password for a user that is in an OU based off of the
* NetBIOS name that you typed when you created your directory. For example, you cannot reset the password for a
* user in the AWS Reserved OU. For more information about the OU structure for an AWS Managed Microsoft AD
* directory, see What Gets Created in the AWS Directory Service Administration Guide.
*
*
*
*
* @param resetUserPasswordRequest
* @return Result of the ResetUserPassword operation returned by the service.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws UserDoesNotExistException
* The user provided a username that does not exist in your directory.
* @throws InvalidPasswordException
* The new password provided by the user does not meet the password complexity requirements defined in your
* directory.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.ResetUserPassword
* @see AWS API
* Documentation
*/
ResetUserPasswordResult resetUserPassword(ResetUserPasswordRequest resetUserPasswordRequest);
/**
*
* Restores a directory using an existing directory snapshot.
*
*
* When you restore a directory from a snapshot, any changes made to the directory after the snapshot date are
* overwritten.
*
*
* This action returns as soon as the restore operation is initiated. You can monitor the progress of the restore
* operation by calling the DescribeDirectories operation with the directory identifier. When the
* DirectoryDescription.Stage value changes to Active
, the restore operation is complete.
*
*
* @param restoreFromSnapshotRequest
* An object representing the inputs for the RestoreFromSnapshot operation.
* @return Result of the RestoreFromSnapshot operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.RestoreFromSnapshot
* @see AWS API
* Documentation
*/
RestoreFromSnapshotResult restoreFromSnapshot(RestoreFromSnapshotRequest restoreFromSnapshotRequest);
/**
*
* Shares a specified directory (DirectoryId
) in your AWS account (directory owner) with another AWS
* account (directory consumer). With this operation you can use your directory from any AWS account and from any
* Amazon VPC within an AWS Region.
*
*
* When you share your AWS Managed Microsoft AD directory, AWS Directory Service creates a shared directory in the
* directory consumer account. This shared directory contains the metadata to provide access to the directory within
* the directory owner account. The shared directory is visible in all VPCs in the directory consumer account.
*
*
* The ShareMethod
parameter determines whether the specified directory can be shared between AWS
* accounts inside the same AWS organization (ORGANIZATIONS
). It also determines whether you can share
* the directory with any other AWS account either inside or outside of the organization (HANDSHAKE
).
*
*
* The ShareNotes
parameter is only used when HANDSHAKE
is called, which sends a directory
* sharing request to the directory consumer.
*
*
* @param shareDirectoryRequest
* @return Result of the ShareDirectory operation returned by the service.
* @throws DirectoryAlreadySharedException
* The specified directory has already been shared with this AWS account.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidTargetException
* The specified shared target is not valid.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ShareLimitExceededException
* The maximum number of AWS accounts that you can share with this directory has been reached.
* @throws OrganizationsException
* Exception encountered while trying to access your AWS organization.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.ShareDirectory
* @see AWS API
* Documentation
*/
ShareDirectoryResult shareDirectory(ShareDirectoryRequest shareDirectoryRequest);
/**
*
* Applies a schema extension to a Microsoft AD directory.
*
*
* @param startSchemaExtensionRequest
* @return Result of the StartSchemaExtension operation returned by the service.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws SnapshotLimitExceededException
* The maximum number of manual snapshots for the directory has been reached. You can use the
* GetSnapshotLimits operation to determine the snapshot limits for a directory.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.StartSchemaExtension
* @see AWS API
* Documentation
*/
StartSchemaExtensionResult startSchemaExtension(StartSchemaExtensionRequest startSchemaExtensionRequest);
/**
*
* Stops the directory sharing between the directory owner and consumer accounts.
*
*
* @param unshareDirectoryRequest
* @return Result of the UnshareDirectory operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidTargetException
* The specified shared target is not valid.
* @throws DirectoryNotSharedException
* The specified directory has not been shared with this AWS account.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.UnshareDirectory
* @see AWS API
* Documentation
*/
UnshareDirectoryResult unshareDirectory(UnshareDirectoryRequest unshareDirectoryRequest);
/**
*
* Updates a conditional forwarder that has been set up for your AWS directory.
*
*
* @param updateConditionalForwarderRequest
* Updates a conditional forwarder.
* @return Result of the UpdateConditionalForwarder operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.UpdateConditionalForwarder
* @see AWS
* API Documentation
*/
UpdateConditionalForwarderResult updateConditionalForwarder(UpdateConditionalForwarderRequest updateConditionalForwarderRequest);
/**
*
* Adds or removes domain controllers to or from the directory. Based on the difference between current value and
* new value (provided through this API call), domain controllers will be added or removed. It may take up to 45
* minutes for any new domain controllers to become fully active once the requested number of domain controllers is
* updated. During this time, you cannot make another update request.
*
*
* @param updateNumberOfDomainControllersRequest
* @return Result of the UpdateNumberOfDomainControllers operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws DomainControllerLimitExceededException
* The maximum allowed number of domain controllers per directory was exceeded. The default limit per
* directory is 20 domain controllers.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.UpdateNumberOfDomainControllers
* @see AWS API Documentation
*/
UpdateNumberOfDomainControllersResult updateNumberOfDomainControllers(UpdateNumberOfDomainControllersRequest updateNumberOfDomainControllersRequest);
/**
*
* Updates the Remote Authentication Dial In User Service (RADIUS) server information for an AD Connector or
* Microsoft AD directory.
*
*
* @param updateRadiusRequest
* Contains the inputs for the UpdateRadius operation.
* @return Result of the UpdateRadius operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.UpdateRadius
* @see AWS API
* Documentation
*/
UpdateRadiusResult updateRadius(UpdateRadiusRequest updateRadiusRequest);
/**
*
* Updates the trust that has been set up between your AWS Managed Microsoft AD directory and an on-premises Active
* Directory.
*
*
* @param updateTrustRequest
* @return Result of the UpdateTrust operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @sample AWSDirectoryService.UpdateTrust
* @see AWS API
* Documentation
*/
UpdateTrustResult updateTrust(UpdateTrustRequest updateTrustRequest);
/**
*
* AWS Directory Service for Microsoft Active Directory allows you to configure and verify trust relationships.
*
*
* This action verifies a trust relationship between your AWS Managed Microsoft AD directory and an external domain.
*
*
* @param verifyTrustRequest
* Initiates the verification of an existing trust relationship between an AWS Managed Microsoft AD directory
* and an external domain.
* @return Result of the VerifyTrust operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in AWS Directory Service.
* @throws UnsupportedOperationException
* The operation is not supported.
* @sample AWSDirectoryService.VerifyTrust
* @see AWS API
* Documentation
*/
VerifyTrustResult verifyTrust(VerifyTrustRequest verifyTrustRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}