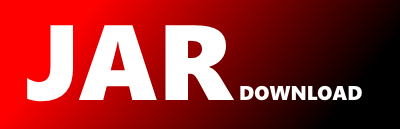
com.amazonaws.services.directory.AWSDirectoryServiceAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-directory Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.directory;
import javax.annotation.Generated;
import com.amazonaws.services.directory.model.*;
/**
* Interface for accessing Directory Service asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.directory.AbstractAWSDirectoryServiceAsync} instead.
*
*
* AWS Directory Service
*
* AWS Directory Service is a web service that makes it easy for you to setup and run directories in the AWS cloud, or
* connect your AWS resources with an existing on-premises Microsoft Active Directory. This guide provides detailed
* information about AWS Directory Service operations, data types, parameters, and errors. For information about AWS
* Directory Services features, see AWS Directory Service and the
* AWS Directory Service
* Administration Guide.
*
*
*
* AWS provides SDKs that consist of libraries and sample code for various programming languages and platforms (Java,
* Ruby, .Net, iOS, Android, etc.). The SDKs provide a convenient way to create programmatic access to AWS Directory
* Service and other AWS services. For more information about the AWS SDKs, including how to download and install them,
* see Tools for Amazon Web Services.
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSDirectoryServiceAsync extends AWSDirectoryService {
/**
*
* Accepts a directory sharing request that was sent from the directory owner account.
*
*
* @param acceptSharedDirectoryRequest
* @return A Java Future containing the result of the AcceptSharedDirectory operation returned by the service.
* @sample AWSDirectoryServiceAsync.AcceptSharedDirectory
* @see AWS API
* Documentation
*/
java.util.concurrent.Future acceptSharedDirectoryAsync(AcceptSharedDirectoryRequest acceptSharedDirectoryRequest);
/**
*
* Accepts a directory sharing request that was sent from the directory owner account.
*
*
* @param acceptSharedDirectoryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AcceptSharedDirectory operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.AcceptSharedDirectory
* @see AWS API
* Documentation
*/
java.util.concurrent.Future acceptSharedDirectoryAsync(AcceptSharedDirectoryRequest acceptSharedDirectoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* If the DNS server for your on-premises domain uses a publicly addressable IP address, you must add a CIDR address
* block to correctly route traffic to and from your Microsoft AD on Amazon Web Services. AddIpRoutes adds
* this address block. You can also use AddIpRoutes to facilitate routing traffic that uses public IP ranges
* from your Microsoft AD on AWS to a peer VPC.
*
*
* Before you call AddIpRoutes, ensure that all of the required permissions have been explicitly granted
* through a policy. For details about what permissions are required to run the AddIpRoutes operation, see AWS
* Directory Service API Permissions: Actions, Resources, and Conditions Reference.
*
*
* @param addIpRoutesRequest
* @return A Java Future containing the result of the AddIpRoutes operation returned by the service.
* @sample AWSDirectoryServiceAsync.AddIpRoutes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addIpRoutesAsync(AddIpRoutesRequest addIpRoutesRequest);
/**
*
* If the DNS server for your on-premises domain uses a publicly addressable IP address, you must add a CIDR address
* block to correctly route traffic to and from your Microsoft AD on Amazon Web Services. AddIpRoutes adds
* this address block. You can also use AddIpRoutes to facilitate routing traffic that uses public IP ranges
* from your Microsoft AD on AWS to a peer VPC.
*
*
* Before you call AddIpRoutes, ensure that all of the required permissions have been explicitly granted
* through a policy. For details about what permissions are required to run the AddIpRoutes operation, see AWS
* Directory Service API Permissions: Actions, Resources, and Conditions Reference.
*
*
* @param addIpRoutesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddIpRoutes operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.AddIpRoutes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addIpRoutesAsync(AddIpRoutesRequest addIpRoutesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds or overwrites one or more tags for the specified directory. Each directory can have a maximum of 50 tags.
* Each tag consists of a key and optional value. Tag keys must be unique to each resource.
*
*
* @param addTagsToResourceRequest
* @return A Java Future containing the result of the AddTagsToResource operation returned by the service.
* @sample AWSDirectoryServiceAsync.AddTagsToResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addTagsToResourceAsync(AddTagsToResourceRequest addTagsToResourceRequest);
/**
*
* Adds or overwrites one or more tags for the specified directory. Each directory can have a maximum of 50 tags.
* Each tag consists of a key and optional value. Tag keys must be unique to each resource.
*
*
* @param addTagsToResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddTagsToResource operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.AddTagsToResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addTagsToResourceAsync(AddTagsToResourceRequest addTagsToResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels an in-progress schema extension to a Microsoft AD directory. Once a schema extension has started
* replicating to all domain controllers, the task can no longer be canceled. A schema extension can be canceled
* during any of the following states; Initializing
, CreatingSnapshot
, and
* UpdatingSchema
.
*
*
* @param cancelSchemaExtensionRequest
* @return A Java Future containing the result of the CancelSchemaExtension operation returned by the service.
* @sample AWSDirectoryServiceAsync.CancelSchemaExtension
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelSchemaExtensionAsync(CancelSchemaExtensionRequest cancelSchemaExtensionRequest);
/**
*
* Cancels an in-progress schema extension to a Microsoft AD directory. Once a schema extension has started
* replicating to all domain controllers, the task can no longer be canceled. A schema extension can be canceled
* during any of the following states; Initializing
, CreatingSnapshot
, and
* UpdatingSchema
.
*
*
* @param cancelSchemaExtensionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelSchemaExtension operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.CancelSchemaExtension
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelSchemaExtensionAsync(CancelSchemaExtensionRequest cancelSchemaExtensionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an AD Connector to connect to an on-premises directory.
*
*
* Before you call ConnectDirectory
, ensure that all of the required permissions have been explicitly
* granted through a policy. For details about what permissions are required to run the
* ConnectDirectory
operation, see AWS Directory Service API Permissions: Actions, Resources, and Conditions Reference.
*
*
* @param connectDirectoryRequest
* Contains the inputs for the ConnectDirectory operation.
* @return A Java Future containing the result of the ConnectDirectory operation returned by the service.
* @sample AWSDirectoryServiceAsync.ConnectDirectory
* @see AWS API
* Documentation
*/
java.util.concurrent.Future connectDirectoryAsync(ConnectDirectoryRequest connectDirectoryRequest);
/**
*
* Creates an AD Connector to connect to an on-premises directory.
*
*
* Before you call ConnectDirectory
, ensure that all of the required permissions have been explicitly
* granted through a policy. For details about what permissions are required to run the
* ConnectDirectory
operation, see AWS Directory Service API Permissions: Actions, Resources, and Conditions Reference.
*
*
* @param connectDirectoryRequest
* Contains the inputs for the ConnectDirectory operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ConnectDirectory operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.ConnectDirectory
* @see AWS API
* Documentation
*/
java.util.concurrent.Future connectDirectoryAsync(ConnectDirectoryRequest connectDirectoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an alias for a directory and assigns the alias to the directory. The alias is used to construct the
* access URL for the directory, such as http://<alias>.awsapps.com
.
*
*
*
* After an alias has been created, it cannot be deleted or reused, so this operation should only be used when
* absolutely necessary.
*
*
*
* @param createAliasRequest
* Contains the inputs for the CreateAlias operation.
* @return A Java Future containing the result of the CreateAlias operation returned by the service.
* @sample AWSDirectoryServiceAsync.CreateAlias
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAliasAsync(CreateAliasRequest createAliasRequest);
/**
*
* Creates an alias for a directory and assigns the alias to the directory. The alias is used to construct the
* access URL for the directory, such as http://<alias>.awsapps.com
.
*
*
*
* After an alias has been created, it cannot be deleted or reused, so this operation should only be used when
* absolutely necessary.
*
*
*
* @param createAliasRequest
* Contains the inputs for the CreateAlias operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAlias operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.CreateAlias
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAliasAsync(CreateAliasRequest createAliasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a computer account in the specified directory, and joins the computer to the directory.
*
*
* @param createComputerRequest
* Contains the inputs for the CreateComputer operation.
* @return A Java Future containing the result of the CreateComputer operation returned by the service.
* @sample AWSDirectoryServiceAsync.CreateComputer
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createComputerAsync(CreateComputerRequest createComputerRequest);
/**
*
* Creates a computer account in the specified directory, and joins the computer to the directory.
*
*
* @param createComputerRequest
* Contains the inputs for the CreateComputer operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateComputer operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.CreateComputer
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createComputerAsync(CreateComputerRequest createComputerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a conditional forwarder associated with your AWS directory. Conditional forwarders are required in order
* to set up a trust relationship with another domain. The conditional forwarder points to the trusted domain.
*
*
* @param createConditionalForwarderRequest
* Initiates the creation of a conditional forwarder for your AWS Directory Service for Microsoft Active
* Directory. Conditional forwarders are required in order to set up a trust relationship with another
* domain.
* @return A Java Future containing the result of the CreateConditionalForwarder operation returned by the service.
* @sample AWSDirectoryServiceAsync.CreateConditionalForwarder
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createConditionalForwarderAsync(
CreateConditionalForwarderRequest createConditionalForwarderRequest);
/**
*
* Creates a conditional forwarder associated with your AWS directory. Conditional forwarders are required in order
* to set up a trust relationship with another domain. The conditional forwarder points to the trusted domain.
*
*
* @param createConditionalForwarderRequest
* Initiates the creation of a conditional forwarder for your AWS Directory Service for Microsoft Active
* Directory. Conditional forwarders are required in order to set up a trust relationship with another
* domain.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateConditionalForwarder operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.CreateConditionalForwarder
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createConditionalForwarderAsync(
CreateConditionalForwarderRequest createConditionalForwarderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a Simple AD directory. For more information, see Simple Active
* Directory in the AWS Directory Service Admin Guide.
*
*
* Before you call CreateDirectory
, ensure that all of the required permissions have been explicitly
* granted through a policy. For details about what permissions are required to run the CreateDirectory
* operation, see AWS Directory Service API Permissions: Actions, Resources, and Conditions Reference.
*
*
* @param createDirectoryRequest
* Contains the inputs for the CreateDirectory operation.
* @return A Java Future containing the result of the CreateDirectory operation returned by the service.
* @sample AWSDirectoryServiceAsync.CreateDirectory
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDirectoryAsync(CreateDirectoryRequest createDirectoryRequest);
/**
*
* Creates a Simple AD directory. For more information, see Simple Active
* Directory in the AWS Directory Service Admin Guide.
*
*
* Before you call CreateDirectory
, ensure that all of the required permissions have been explicitly
* granted through a policy. For details about what permissions are required to run the CreateDirectory
* operation, see AWS Directory Service API Permissions: Actions, Resources, and Conditions Reference.
*
*
* @param createDirectoryRequest
* Contains the inputs for the CreateDirectory operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDirectory operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.CreateDirectory
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDirectoryAsync(CreateDirectoryRequest createDirectoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a subscription to forward real-time Directory Service domain controller security logs to the specified
* Amazon CloudWatch log group in your AWS account.
*
*
* @param createLogSubscriptionRequest
* @return A Java Future containing the result of the CreateLogSubscription operation returned by the service.
* @sample AWSDirectoryServiceAsync.CreateLogSubscription
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createLogSubscriptionAsync(CreateLogSubscriptionRequest createLogSubscriptionRequest);
/**
*
* Creates a subscription to forward real-time Directory Service domain controller security logs to the specified
* Amazon CloudWatch log group in your AWS account.
*
*
* @param createLogSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLogSubscription operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.CreateLogSubscription
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createLogSubscriptionAsync(CreateLogSubscriptionRequest createLogSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a Microsoft AD directory in the AWS Cloud. For more information, see AWS Managed
* Microsoft AD in the AWS Directory Service Admin Guide.
*
*
* Before you call CreateMicrosoftAD, ensure that all of the required permissions have been explicitly
* granted through a policy. For details about what permissions are required to run the CreateMicrosoftAD
* operation, see AWS Directory Service API Permissions: Actions, Resources, and Conditions Reference.
*
*
* @param createMicrosoftADRequest
* Creates an AWS Managed Microsoft AD directory.
* @return A Java Future containing the result of the CreateMicrosoftAD operation returned by the service.
* @sample AWSDirectoryServiceAsync.CreateMicrosoftAD
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createMicrosoftADAsync(CreateMicrosoftADRequest createMicrosoftADRequest);
/**
*
* Creates a Microsoft AD directory in the AWS Cloud. For more information, see AWS Managed
* Microsoft AD in the AWS Directory Service Admin Guide.
*
*
* Before you call CreateMicrosoftAD, ensure that all of the required permissions have been explicitly
* granted through a policy. For details about what permissions are required to run the CreateMicrosoftAD
* operation, see AWS Directory Service API Permissions: Actions, Resources, and Conditions Reference.
*
*
* @param createMicrosoftADRequest
* Creates an AWS Managed Microsoft AD directory.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMicrosoftAD operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.CreateMicrosoftAD
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createMicrosoftADAsync(CreateMicrosoftADRequest createMicrosoftADRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a snapshot of a Simple AD or Microsoft AD directory in the AWS cloud.
*
*
*
* You cannot take snapshots of AD Connector directories.
*
*
*
* @param createSnapshotRequest
* Contains the inputs for the CreateSnapshot operation.
* @return A Java Future containing the result of the CreateSnapshot operation returned by the service.
* @sample AWSDirectoryServiceAsync.CreateSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createSnapshotAsync(CreateSnapshotRequest createSnapshotRequest);
/**
*
* Creates a snapshot of a Simple AD or Microsoft AD directory in the AWS cloud.
*
*
*
* You cannot take snapshots of AD Connector directories.
*
*
*
* @param createSnapshotRequest
* Contains the inputs for the CreateSnapshot operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSnapshot operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.CreateSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createSnapshotAsync(CreateSnapshotRequest createSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* AWS Directory Service for Microsoft Active Directory allows you to configure trust relationships. For example,
* you can establish a trust between your AWS Managed Microsoft AD directory, and your existing on-premises
* Microsoft Active Directory. This would allow you to provide users and groups access to resources in either
* domain, with a single set of credentials.
*
*
* This action initiates the creation of the AWS side of a trust relationship between an AWS Managed Microsoft AD
* directory and an external domain. You can create either a forest trust or an external trust.
*
*
* @param createTrustRequest
* AWS Directory Service for Microsoft Active Directory allows you to configure trust relationships. For
* example, you can establish a trust between your AWS Managed Microsoft AD directory, and your existing
* on-premises Microsoft Active Directory. This would allow you to provide users and groups access to
* resources in either domain, with a single set of credentials.
*
* This action initiates the creation of the AWS side of a trust relationship between an AWS Managed
* Microsoft AD directory and an external domain.
* @return A Java Future containing the result of the CreateTrust operation returned by the service.
* @sample AWSDirectoryServiceAsync.CreateTrust
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTrustAsync(CreateTrustRequest createTrustRequest);
/**
*
* AWS Directory Service for Microsoft Active Directory allows you to configure trust relationships. For example,
* you can establish a trust between your AWS Managed Microsoft AD directory, and your existing on-premises
* Microsoft Active Directory. This would allow you to provide users and groups access to resources in either
* domain, with a single set of credentials.
*
*
* This action initiates the creation of the AWS side of a trust relationship between an AWS Managed Microsoft AD
* directory and an external domain. You can create either a forest trust or an external trust.
*
*
* @param createTrustRequest
* AWS Directory Service for Microsoft Active Directory allows you to configure trust relationships. For
* example, you can establish a trust between your AWS Managed Microsoft AD directory, and your existing
* on-premises Microsoft Active Directory. This would allow you to provide users and groups access to
* resources in either domain, with a single set of credentials.
*
* This action initiates the creation of the AWS side of a trust relationship between an AWS Managed
* Microsoft AD directory and an external domain.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTrust operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.CreateTrust
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTrustAsync(CreateTrustRequest createTrustRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a conditional forwarder that has been set up for your AWS directory.
*
*
* @param deleteConditionalForwarderRequest
* Deletes a conditional forwarder.
* @return A Java Future containing the result of the DeleteConditionalForwarder operation returned by the service.
* @sample AWSDirectoryServiceAsync.DeleteConditionalForwarder
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteConditionalForwarderAsync(
DeleteConditionalForwarderRequest deleteConditionalForwarderRequest);
/**
*
* Deletes a conditional forwarder that has been set up for your AWS directory.
*
*
* @param deleteConditionalForwarderRequest
* Deletes a conditional forwarder.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteConditionalForwarder operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.DeleteConditionalForwarder
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteConditionalForwarderAsync(
DeleteConditionalForwarderRequest deleteConditionalForwarderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an AWS Directory Service directory.
*
*
* Before you call DeleteDirectory
, ensure that all of the required permissions have been explicitly
* granted through a policy. For details about what permissions are required to run the DeleteDirectory
* operation, see AWS Directory Service API Permissions: Actions, Resources, and Conditions Reference.
*
*
* @param deleteDirectoryRequest
* Contains the inputs for the DeleteDirectory operation.
* @return A Java Future containing the result of the DeleteDirectory operation returned by the service.
* @sample AWSDirectoryServiceAsync.DeleteDirectory
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDirectoryAsync(DeleteDirectoryRequest deleteDirectoryRequest);
/**
*
* Deletes an AWS Directory Service directory.
*
*
* Before you call DeleteDirectory
, ensure that all of the required permissions have been explicitly
* granted through a policy. For details about what permissions are required to run the DeleteDirectory
* operation, see AWS Directory Service API Permissions: Actions, Resources, and Conditions Reference.
*
*
* @param deleteDirectoryRequest
* Contains the inputs for the DeleteDirectory operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDirectory operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.DeleteDirectory
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDirectoryAsync(DeleteDirectoryRequest deleteDirectoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified log subscription.
*
*
* @param deleteLogSubscriptionRequest
* @return A Java Future containing the result of the DeleteLogSubscription operation returned by the service.
* @sample AWSDirectoryServiceAsync.DeleteLogSubscription
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteLogSubscriptionAsync(DeleteLogSubscriptionRequest deleteLogSubscriptionRequest);
/**
*
* Deletes the specified log subscription.
*
*
* @param deleteLogSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteLogSubscription operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.DeleteLogSubscription
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteLogSubscriptionAsync(DeleteLogSubscriptionRequest deleteLogSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a directory snapshot.
*
*
* @param deleteSnapshotRequest
* Contains the inputs for the DeleteSnapshot operation.
* @return A Java Future containing the result of the DeleteSnapshot operation returned by the service.
* @sample AWSDirectoryServiceAsync.DeleteSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteSnapshotAsync(DeleteSnapshotRequest deleteSnapshotRequest);
/**
*
* Deletes a directory snapshot.
*
*
* @param deleteSnapshotRequest
* Contains the inputs for the DeleteSnapshot operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSnapshot operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.DeleteSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteSnapshotAsync(DeleteSnapshotRequest deleteSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing trust relationship between your AWS Managed Microsoft AD directory and an external domain.
*
*
* @param deleteTrustRequest
* Deletes the local side of an existing trust relationship between the AWS Managed Microsoft AD directory
* and the external domain.
* @return A Java Future containing the result of the DeleteTrust operation returned by the service.
* @sample AWSDirectoryServiceAsync.DeleteTrust
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteTrustAsync(DeleteTrustRequest deleteTrustRequest);
/**
*
* Deletes an existing trust relationship between your AWS Managed Microsoft AD directory and an external domain.
*
*
* @param deleteTrustRequest
* Deletes the local side of an existing trust relationship between the AWS Managed Microsoft AD directory
* and the external domain.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTrust operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.DeleteTrust
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteTrustAsync(DeleteTrustRequest deleteTrustRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes from the system the certificate that was registered for a secured LDAP connection.
*
*
* @param deregisterCertificateRequest
* @return A Java Future containing the result of the DeregisterCertificate operation returned by the service.
* @sample AWSDirectoryServiceAsync.DeregisterCertificate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deregisterCertificateAsync(DeregisterCertificateRequest deregisterCertificateRequest);
/**
*
* Deletes from the system the certificate that was registered for a secured LDAP connection.
*
*
* @param deregisterCertificateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeregisterCertificate operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.DeregisterCertificate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deregisterCertificateAsync(DeregisterCertificateRequest deregisterCertificateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified directory as a publisher to the specified SNS topic.
*
*
* @param deregisterEventTopicRequest
* Removes the specified directory as a publisher to the specified SNS topic.
* @return A Java Future containing the result of the DeregisterEventTopic operation returned by the service.
* @sample AWSDirectoryServiceAsync.DeregisterEventTopic
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deregisterEventTopicAsync(DeregisterEventTopicRequest deregisterEventTopicRequest);
/**
*
* Removes the specified directory as a publisher to the specified SNS topic.
*
*
* @param deregisterEventTopicRequest
* Removes the specified directory as a publisher to the specified SNS topic.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeregisterEventTopic operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.DeregisterEventTopic
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deregisterEventTopicAsync(DeregisterEventTopicRequest deregisterEventTopicRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Displays information about the certificate registered for a secured LDAP connection.
*
*
* @param describeCertificateRequest
* @return A Java Future containing the result of the DescribeCertificate operation returned by the service.
* @sample AWSDirectoryServiceAsync.DescribeCertificate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeCertificateAsync(DescribeCertificateRequest describeCertificateRequest);
/**
*
* Displays information about the certificate registered for a secured LDAP connection.
*
*
* @param describeCertificateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeCertificate operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.DescribeCertificate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeCertificateAsync(DescribeCertificateRequest describeCertificateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Obtains information about the conditional forwarders for this account.
*
*
* If no input parameters are provided for RemoteDomainNames, this request describes all conditional forwarders for
* the specified directory ID.
*
*
* @param describeConditionalForwardersRequest
* Describes a conditional forwarder.
* @return A Java Future containing the result of the DescribeConditionalForwarders operation returned by the
* service.
* @sample AWSDirectoryServiceAsync.DescribeConditionalForwarders
* @see AWS API Documentation
*/
java.util.concurrent.Future describeConditionalForwardersAsync(
DescribeConditionalForwardersRequest describeConditionalForwardersRequest);
/**
*
* Obtains information about the conditional forwarders for this account.
*
*
* If no input parameters are provided for RemoteDomainNames, this request describes all conditional forwarders for
* the specified directory ID.
*
*
* @param describeConditionalForwardersRequest
* Describes a conditional forwarder.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeConditionalForwarders operation returned by the
* service.
* @sample AWSDirectoryServiceAsyncHandler.DescribeConditionalForwarders
* @see AWS API Documentation
*/
java.util.concurrent.Future describeConditionalForwardersAsync(
DescribeConditionalForwardersRequest describeConditionalForwardersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Obtains information about the directories that belong to this account.
*
*
* You can retrieve information about specific directories by passing the directory identifiers in the
* DirectoryIds
parameter. Otherwise, all directories that belong to the current account are returned.
*
*
* This operation supports pagination with the use of the NextToken
request and response parameters. If
* more results are available, the DescribeDirectoriesResult.NextToken
member contains a token that you
* pass in the next call to DescribeDirectories to retrieve the next set of items.
*
*
* You can also specify a maximum number of return results with the Limit
parameter.
*
*
* @param describeDirectoriesRequest
* Contains the inputs for the DescribeDirectories operation.
* @return A Java Future containing the result of the DescribeDirectories operation returned by the service.
* @sample AWSDirectoryServiceAsync.DescribeDirectories
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDirectoriesAsync(DescribeDirectoriesRequest describeDirectoriesRequest);
/**
*
* Obtains information about the directories that belong to this account.
*
*
* You can retrieve information about specific directories by passing the directory identifiers in the
* DirectoryIds
parameter. Otherwise, all directories that belong to the current account are returned.
*
*
* This operation supports pagination with the use of the NextToken
request and response parameters. If
* more results are available, the DescribeDirectoriesResult.NextToken
member contains a token that you
* pass in the next call to DescribeDirectories to retrieve the next set of items.
*
*
* You can also specify a maximum number of return results with the Limit
parameter.
*
*
* @param describeDirectoriesRequest
* Contains the inputs for the DescribeDirectories operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDirectories operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.DescribeDirectories
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDirectoriesAsync(DescribeDirectoriesRequest describeDirectoriesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeDirectories operation.
*
* @see #describeDirectoriesAsync(DescribeDirectoriesRequest)
*/
java.util.concurrent.Future describeDirectoriesAsync();
/**
* Simplified method form for invoking the DescribeDirectories operation with an AsyncHandler.
*
* @see #describeDirectoriesAsync(DescribeDirectoriesRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeDirectoriesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides information about any domain controllers in your directory.
*
*
* @param describeDomainControllersRequest
* @return A Java Future containing the result of the DescribeDomainControllers operation returned by the service.
* @sample AWSDirectoryServiceAsync.DescribeDomainControllers
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDomainControllersAsync(
DescribeDomainControllersRequest describeDomainControllersRequest);
/**
*
* Provides information about any domain controllers in your directory.
*
*
* @param describeDomainControllersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDomainControllers operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.DescribeDomainControllers
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDomainControllersAsync(
DescribeDomainControllersRequest describeDomainControllersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Obtains information about which SNS topics receive status messages from the specified directory.
*
*
* If no input parameters are provided, such as DirectoryId or TopicName, this request describes all of the
* associations in the account.
*
*
* @param describeEventTopicsRequest
* Describes event topics.
* @return A Java Future containing the result of the DescribeEventTopics operation returned by the service.
* @sample AWSDirectoryServiceAsync.DescribeEventTopics
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEventTopicsAsync(DescribeEventTopicsRequest describeEventTopicsRequest);
/**
*
* Obtains information about which SNS topics receive status messages from the specified directory.
*
*
* If no input parameters are provided, such as DirectoryId or TopicName, this request describes all of the
* associations in the account.
*
*
* @param describeEventTopicsRequest
* Describes event topics.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEventTopics operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.DescribeEventTopics
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEventTopicsAsync(DescribeEventTopicsRequest describeEventTopicsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the status of LDAP security for the specified directory.
*
*
* @param describeLDAPSSettingsRequest
* @return A Java Future containing the result of the DescribeLDAPSSettings operation returned by the service.
* @sample AWSDirectoryServiceAsync.DescribeLDAPSSettings
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeLDAPSSettingsAsync(DescribeLDAPSSettingsRequest describeLDAPSSettingsRequest);
/**
*
* Describes the status of LDAP security for the specified directory.
*
*
* @param describeLDAPSSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeLDAPSSettings operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.DescribeLDAPSSettings
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeLDAPSSettingsAsync(DescribeLDAPSSettingsRequest describeLDAPSSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the shared directories in your account.
*
*
* @param describeSharedDirectoriesRequest
* @return A Java Future containing the result of the DescribeSharedDirectories operation returned by the service.
* @sample AWSDirectoryServiceAsync.DescribeSharedDirectories
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeSharedDirectoriesAsync(
DescribeSharedDirectoriesRequest describeSharedDirectoriesRequest);
/**
*
* Returns the shared directories in your account.
*
*
* @param describeSharedDirectoriesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSharedDirectories operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.DescribeSharedDirectories
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeSharedDirectoriesAsync(
DescribeSharedDirectoriesRequest describeSharedDirectoriesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Obtains information about the directory snapshots that belong to this account.
*
*
* This operation supports pagination with the use of the NextToken request and response parameters. If more
* results are available, the DescribeSnapshots.NextToken member contains a token that you pass in the next
* call to DescribeSnapshots to retrieve the next set of items.
*
*
* You can also specify a maximum number of return results with the Limit parameter.
*
*
* @param describeSnapshotsRequest
* Contains the inputs for the DescribeSnapshots operation.
* @return A Java Future containing the result of the DescribeSnapshots operation returned by the service.
* @sample AWSDirectoryServiceAsync.DescribeSnapshots
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeSnapshotsAsync(DescribeSnapshotsRequest describeSnapshotsRequest);
/**
*
* Obtains information about the directory snapshots that belong to this account.
*
*
* This operation supports pagination with the use of the NextToken request and response parameters. If more
* results are available, the DescribeSnapshots.NextToken member contains a token that you pass in the next
* call to DescribeSnapshots to retrieve the next set of items.
*
*
* You can also specify a maximum number of return results with the Limit parameter.
*
*
* @param describeSnapshotsRequest
* Contains the inputs for the DescribeSnapshots operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSnapshots operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.DescribeSnapshots
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeSnapshotsAsync(DescribeSnapshotsRequest describeSnapshotsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeSnapshots operation.
*
* @see #describeSnapshotsAsync(DescribeSnapshotsRequest)
*/
java.util.concurrent.Future describeSnapshotsAsync();
/**
* Simplified method form for invoking the DescribeSnapshots operation with an AsyncHandler.
*
* @see #describeSnapshotsAsync(DescribeSnapshotsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeSnapshotsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Obtains information about the trust relationships for this account.
*
*
* If no input parameters are provided, such as DirectoryId or TrustIds, this request describes all the trust
* relationships belonging to the account.
*
*
* @param describeTrustsRequest
* Describes the trust relationships for a particular AWS Managed Microsoft AD directory. If no input
* parameters are are provided, such as directory ID or trust ID, this request describes all the trust
* relationships.
* @return A Java Future containing the result of the DescribeTrusts operation returned by the service.
* @sample AWSDirectoryServiceAsync.DescribeTrusts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeTrustsAsync(DescribeTrustsRequest describeTrustsRequest);
/**
*
* Obtains information about the trust relationships for this account.
*
*
* If no input parameters are provided, such as DirectoryId or TrustIds, this request describes all the trust
* relationships belonging to the account.
*
*
* @param describeTrustsRequest
* Describes the trust relationships for a particular AWS Managed Microsoft AD directory. If no input
* parameters are are provided, such as directory ID or trust ID, this request describes all the trust
* relationships.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTrusts operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.DescribeTrusts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeTrustsAsync(DescribeTrustsRequest describeTrustsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deactivates LDAP secure calls for the specified directory.
*
*
* @param disableLDAPSRequest
* @return A Java Future containing the result of the DisableLDAPS operation returned by the service.
* @sample AWSDirectoryServiceAsync.DisableLDAPS
* @see AWS API
* Documentation
*/
java.util.concurrent.Future disableLDAPSAsync(DisableLDAPSRequest disableLDAPSRequest);
/**
*
* Deactivates LDAP secure calls for the specified directory.
*
*
* @param disableLDAPSRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisableLDAPS operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.DisableLDAPS
* @see AWS API
* Documentation
*/
java.util.concurrent.Future disableLDAPSAsync(DisableLDAPSRequest disableLDAPSRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disables multi-factor authentication (MFA) with the Remote Authentication Dial In User Service (RADIUS) server
* for an AD Connector or Microsoft AD directory.
*
*
* @param disableRadiusRequest
* Contains the inputs for the DisableRadius operation.
* @return A Java Future containing the result of the DisableRadius operation returned by the service.
* @sample AWSDirectoryServiceAsync.DisableRadius
* @see AWS API
* Documentation
*/
java.util.concurrent.Future disableRadiusAsync(DisableRadiusRequest disableRadiusRequest);
/**
*
* Disables multi-factor authentication (MFA) with the Remote Authentication Dial In User Service (RADIUS) server
* for an AD Connector or Microsoft AD directory.
*
*
* @param disableRadiusRequest
* Contains the inputs for the DisableRadius operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisableRadius operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.DisableRadius
* @see AWS API
* Documentation
*/
java.util.concurrent.Future disableRadiusAsync(DisableRadiusRequest disableRadiusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disables single-sign on for a directory.
*
*
* @param disableSsoRequest
* Contains the inputs for the DisableSso operation.
* @return A Java Future containing the result of the DisableSso operation returned by the service.
* @sample AWSDirectoryServiceAsync.DisableSso
* @see AWS API
* Documentation
*/
java.util.concurrent.Future disableSsoAsync(DisableSsoRequest disableSsoRequest);
/**
*
* Disables single-sign on for a directory.
*
*
* @param disableSsoRequest
* Contains the inputs for the DisableSso operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisableSso operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.DisableSso
* @see AWS API
* Documentation
*/
java.util.concurrent.Future disableSsoAsync(DisableSsoRequest disableSsoRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Activates the switch for the specific directory to always use LDAP secure calls.
*
*
* @param enableLDAPSRequest
* @return A Java Future containing the result of the EnableLDAPS operation returned by the service.
* @sample AWSDirectoryServiceAsync.EnableLDAPS
* @see AWS API
* Documentation
*/
java.util.concurrent.Future enableLDAPSAsync(EnableLDAPSRequest enableLDAPSRequest);
/**
*
* Activates the switch for the specific directory to always use LDAP secure calls.
*
*
* @param enableLDAPSRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the EnableLDAPS operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.EnableLDAPS
* @see AWS API
* Documentation
*/
java.util.concurrent.Future enableLDAPSAsync(EnableLDAPSRequest enableLDAPSRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables multi-factor authentication (MFA) with the Remote Authentication Dial In User Service (RADIUS) server for
* an AD Connector or Microsoft AD directory.
*
*
* @param enableRadiusRequest
* Contains the inputs for the EnableRadius operation.
* @return A Java Future containing the result of the EnableRadius operation returned by the service.
* @sample AWSDirectoryServiceAsync.EnableRadius
* @see AWS API
* Documentation
*/
java.util.concurrent.Future enableRadiusAsync(EnableRadiusRequest enableRadiusRequest);
/**
*
* Enables multi-factor authentication (MFA) with the Remote Authentication Dial In User Service (RADIUS) server for
* an AD Connector or Microsoft AD directory.
*
*
* @param enableRadiusRequest
* Contains the inputs for the EnableRadius operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the EnableRadius operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.EnableRadius
* @see AWS API
* Documentation
*/
java.util.concurrent.Future enableRadiusAsync(EnableRadiusRequest enableRadiusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables single sign-on for a directory. Single sign-on allows users in your directory to access certain AWS
* services from a computer joined to the directory without having to enter their credentials separately.
*
*
* @param enableSsoRequest
* Contains the inputs for the EnableSso operation.
* @return A Java Future containing the result of the EnableSso operation returned by the service.
* @sample AWSDirectoryServiceAsync.EnableSso
* @see AWS API
* Documentation
*/
java.util.concurrent.Future enableSsoAsync(EnableSsoRequest enableSsoRequest);
/**
*
* Enables single sign-on for a directory. Single sign-on allows users in your directory to access certain AWS
* services from a computer joined to the directory without having to enter their credentials separately.
*
*
* @param enableSsoRequest
* Contains the inputs for the EnableSso operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the EnableSso operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.EnableSso
* @see AWS API
* Documentation
*/
java.util.concurrent.Future enableSsoAsync(EnableSsoRequest enableSsoRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Obtains directory limit information for the current Region.
*
*
* @param getDirectoryLimitsRequest
* Contains the inputs for the GetDirectoryLimits operation.
* @return A Java Future containing the result of the GetDirectoryLimits operation returned by the service.
* @sample AWSDirectoryServiceAsync.GetDirectoryLimits
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDirectoryLimitsAsync(GetDirectoryLimitsRequest getDirectoryLimitsRequest);
/**
*
* Obtains directory limit information for the current Region.
*
*
* @param getDirectoryLimitsRequest
* Contains the inputs for the GetDirectoryLimits operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDirectoryLimits operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.GetDirectoryLimits
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDirectoryLimitsAsync(GetDirectoryLimitsRequest getDirectoryLimitsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the GetDirectoryLimits operation.
*
* @see #getDirectoryLimitsAsync(GetDirectoryLimitsRequest)
*/
java.util.concurrent.Future getDirectoryLimitsAsync();
/**
* Simplified method form for invoking the GetDirectoryLimits operation with an AsyncHandler.
*
* @see #getDirectoryLimitsAsync(GetDirectoryLimitsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future getDirectoryLimitsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Obtains the manual snapshot limits for a directory.
*
*
* @param getSnapshotLimitsRequest
* Contains the inputs for the GetSnapshotLimits operation.
* @return A Java Future containing the result of the GetSnapshotLimits operation returned by the service.
* @sample AWSDirectoryServiceAsync.GetSnapshotLimits
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSnapshotLimitsAsync(GetSnapshotLimitsRequest getSnapshotLimitsRequest);
/**
*
* Obtains the manual snapshot limits for a directory.
*
*
* @param getSnapshotLimitsRequest
* Contains the inputs for the GetSnapshotLimits operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSnapshotLimits operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.GetSnapshotLimits
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSnapshotLimitsAsync(GetSnapshotLimitsRequest getSnapshotLimitsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* For the specified directory, lists all the certificates registered for a secured LDAP connection.
*
*
* @param listCertificatesRequest
* @return A Java Future containing the result of the ListCertificates operation returned by the service.
* @sample AWSDirectoryServiceAsync.ListCertificates
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listCertificatesAsync(ListCertificatesRequest listCertificatesRequest);
/**
*
* For the specified directory, lists all the certificates registered for a secured LDAP connection.
*
*
* @param listCertificatesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListCertificates operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.ListCertificates
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listCertificatesAsync(ListCertificatesRequest listCertificatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the address blocks that you have added to a directory.
*
*
* @param listIpRoutesRequest
* @return A Java Future containing the result of the ListIpRoutes operation returned by the service.
* @sample AWSDirectoryServiceAsync.ListIpRoutes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listIpRoutesAsync(ListIpRoutesRequest listIpRoutesRequest);
/**
*
* Lists the address blocks that you have added to a directory.
*
*
* @param listIpRoutesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListIpRoutes operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.ListIpRoutes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listIpRoutesAsync(ListIpRoutesRequest listIpRoutesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the active log subscriptions for the AWS account.
*
*
* @param listLogSubscriptionsRequest
* @return A Java Future containing the result of the ListLogSubscriptions operation returned by the service.
* @sample AWSDirectoryServiceAsync.ListLogSubscriptions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listLogSubscriptionsAsync(ListLogSubscriptionsRequest listLogSubscriptionsRequest);
/**
*
* Lists the active log subscriptions for the AWS account.
*
*
* @param listLogSubscriptionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListLogSubscriptions operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.ListLogSubscriptions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listLogSubscriptionsAsync(ListLogSubscriptionsRequest listLogSubscriptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all schema extensions applied to a Microsoft AD Directory.
*
*
* @param listSchemaExtensionsRequest
* @return A Java Future containing the result of the ListSchemaExtensions operation returned by the service.
* @sample AWSDirectoryServiceAsync.ListSchemaExtensions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listSchemaExtensionsAsync(ListSchemaExtensionsRequest listSchemaExtensionsRequest);
/**
*
* Lists all schema extensions applied to a Microsoft AD Directory.
*
*
* @param listSchemaExtensionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSchemaExtensions operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.ListSchemaExtensions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listSchemaExtensionsAsync(ListSchemaExtensionsRequest listSchemaExtensionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all tags on a directory.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSDirectoryServiceAsync.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists all tags on a directory.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Registers a certificate for secured LDAP connection.
*
*
* @param registerCertificateRequest
* @return A Java Future containing the result of the RegisterCertificate operation returned by the service.
* @sample AWSDirectoryServiceAsync.RegisterCertificate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future registerCertificateAsync(RegisterCertificateRequest registerCertificateRequest);
/**
*
* Registers a certificate for secured LDAP connection.
*
*
* @param registerCertificateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RegisterCertificate operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.RegisterCertificate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future registerCertificateAsync(RegisterCertificateRequest registerCertificateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a directory with an SNS topic. This establishes the directory as a publisher to the specified SNS
* topic. You can then receive email or text (SMS) messages when the status of your directory changes. You get
* notified if your directory goes from an Active status to an Impaired or Inoperable status. You also receive a
* notification when the directory returns to an Active status.
*
*
* @param registerEventTopicRequest
* Registers a new event topic.
* @return A Java Future containing the result of the RegisterEventTopic operation returned by the service.
* @sample AWSDirectoryServiceAsync.RegisterEventTopic
* @see AWS API
* Documentation
*/
java.util.concurrent.Future registerEventTopicAsync(RegisterEventTopicRequest registerEventTopicRequest);
/**
*
* Associates a directory with an SNS topic. This establishes the directory as a publisher to the specified SNS
* topic. You can then receive email or text (SMS) messages when the status of your directory changes. You get
* notified if your directory goes from an Active status to an Impaired or Inoperable status. You also receive a
* notification when the directory returns to an Active status.
*
*
* @param registerEventTopicRequest
* Registers a new event topic.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RegisterEventTopic operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.RegisterEventTopic
* @see AWS API
* Documentation
*/
java.util.concurrent.Future registerEventTopicAsync(RegisterEventTopicRequest registerEventTopicRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Rejects a directory sharing request that was sent from the directory owner account.
*
*
* @param rejectSharedDirectoryRequest
* @return A Java Future containing the result of the RejectSharedDirectory operation returned by the service.
* @sample AWSDirectoryServiceAsync.RejectSharedDirectory
* @see AWS API
* Documentation
*/
java.util.concurrent.Future rejectSharedDirectoryAsync(RejectSharedDirectoryRequest rejectSharedDirectoryRequest);
/**
*
* Rejects a directory sharing request that was sent from the directory owner account.
*
*
* @param rejectSharedDirectoryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RejectSharedDirectory operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.RejectSharedDirectory
* @see AWS API
* Documentation
*/
java.util.concurrent.Future rejectSharedDirectoryAsync(RejectSharedDirectoryRequest rejectSharedDirectoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes IP address blocks from a directory.
*
*
* @param removeIpRoutesRequest
* @return A Java Future containing the result of the RemoveIpRoutes operation returned by the service.
* @sample AWSDirectoryServiceAsync.RemoveIpRoutes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future removeIpRoutesAsync(RemoveIpRoutesRequest removeIpRoutesRequest);
/**
*
* Removes IP address blocks from a directory.
*
*
* @param removeIpRoutesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveIpRoutes operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.RemoveIpRoutes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future removeIpRoutesAsync(RemoveIpRoutesRequest removeIpRoutesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes tags from a directory.
*
*
* @param removeTagsFromResourceRequest
* @return A Java Future containing the result of the RemoveTagsFromResource operation returned by the service.
* @sample AWSDirectoryServiceAsync.RemoveTagsFromResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future removeTagsFromResourceAsync(RemoveTagsFromResourceRequest removeTagsFromResourceRequest);
/**
*
* Removes tags from a directory.
*
*
* @param removeTagsFromResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveTagsFromResource operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.RemoveTagsFromResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future removeTagsFromResourceAsync(RemoveTagsFromResourceRequest removeTagsFromResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Resets the password for any user in your AWS Managed Microsoft AD or Simple AD directory.
*
*
* You can reset the password for any user in your directory with the following exceptions:
*
*
* -
*
* For Simple AD, you cannot reset the password for any user that is a member of either the Domain Admins or
* Enterprise Admins group except for the administrator user.
*
*
* -
*
* For AWS Managed Microsoft AD, you can only reset the password for a user that is in an OU based off of the
* NetBIOS name that you typed when you created your directory. For example, you cannot reset the password for a
* user in the AWS Reserved OU. For more information about the OU structure for an AWS Managed Microsoft AD
* directory, see What Gets Created in the AWS Directory Service Administration Guide.
*
*
*
*
* @param resetUserPasswordRequest
* @return A Java Future containing the result of the ResetUserPassword operation returned by the service.
* @sample AWSDirectoryServiceAsync.ResetUserPassword
* @see AWS API
* Documentation
*/
java.util.concurrent.Future resetUserPasswordAsync(ResetUserPasswordRequest resetUserPasswordRequest);
/**
*
* Resets the password for any user in your AWS Managed Microsoft AD or Simple AD directory.
*
*
* You can reset the password for any user in your directory with the following exceptions:
*
*
* -
*
* For Simple AD, you cannot reset the password for any user that is a member of either the Domain Admins or
* Enterprise Admins group except for the administrator user.
*
*
* -
*
* For AWS Managed Microsoft AD, you can only reset the password for a user that is in an OU based off of the
* NetBIOS name that you typed when you created your directory. For example, you cannot reset the password for a
* user in the AWS Reserved OU. For more information about the OU structure for an AWS Managed Microsoft AD
* directory, see What Gets Created in the AWS Directory Service Administration Guide.
*
*
*
*
* @param resetUserPasswordRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ResetUserPassword operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.ResetUserPassword
* @see AWS API
* Documentation
*/
java.util.concurrent.Future resetUserPasswordAsync(ResetUserPasswordRequest resetUserPasswordRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Restores a directory using an existing directory snapshot.
*
*
* When you restore a directory from a snapshot, any changes made to the directory after the snapshot date are
* overwritten.
*
*
* This action returns as soon as the restore operation is initiated. You can monitor the progress of the restore
* operation by calling the DescribeDirectories operation with the directory identifier. When the
* DirectoryDescription.Stage value changes to Active
, the restore operation is complete.
*
*
* @param restoreFromSnapshotRequest
* An object representing the inputs for the RestoreFromSnapshot operation.
* @return A Java Future containing the result of the RestoreFromSnapshot operation returned by the service.
* @sample AWSDirectoryServiceAsync.RestoreFromSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future restoreFromSnapshotAsync(RestoreFromSnapshotRequest restoreFromSnapshotRequest);
/**
*
* Restores a directory using an existing directory snapshot.
*
*
* When you restore a directory from a snapshot, any changes made to the directory after the snapshot date are
* overwritten.
*
*
* This action returns as soon as the restore operation is initiated. You can monitor the progress of the restore
* operation by calling the DescribeDirectories operation with the directory identifier. When the
* DirectoryDescription.Stage value changes to Active
, the restore operation is complete.
*
*
* @param restoreFromSnapshotRequest
* An object representing the inputs for the RestoreFromSnapshot operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RestoreFromSnapshot operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.RestoreFromSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future restoreFromSnapshotAsync(RestoreFromSnapshotRequest restoreFromSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Shares a specified directory (DirectoryId
) in your AWS account (directory owner) with another AWS
* account (directory consumer). With this operation you can use your directory from any AWS account and from any
* Amazon VPC within an AWS Region.
*
*
* When you share your AWS Managed Microsoft AD directory, AWS Directory Service creates a shared directory in the
* directory consumer account. This shared directory contains the metadata to provide access to the directory within
* the directory owner account. The shared directory is visible in all VPCs in the directory consumer account.
*
*
* The ShareMethod
parameter determines whether the specified directory can be shared between AWS
* accounts inside the same AWS organization (ORGANIZATIONS
). It also determines whether you can share
* the directory with any other AWS account either inside or outside of the organization (HANDSHAKE
).
*
*
* The ShareNotes
parameter is only used when HANDSHAKE
is called, which sends a directory
* sharing request to the directory consumer.
*
*
* @param shareDirectoryRequest
* @return A Java Future containing the result of the ShareDirectory operation returned by the service.
* @sample AWSDirectoryServiceAsync.ShareDirectory
* @see AWS API
* Documentation
*/
java.util.concurrent.Future shareDirectoryAsync(ShareDirectoryRequest shareDirectoryRequest);
/**
*
* Shares a specified directory (DirectoryId
) in your AWS account (directory owner) with another AWS
* account (directory consumer). With this operation you can use your directory from any AWS account and from any
* Amazon VPC within an AWS Region.
*
*
* When you share your AWS Managed Microsoft AD directory, AWS Directory Service creates a shared directory in the
* directory consumer account. This shared directory contains the metadata to provide access to the directory within
* the directory owner account. The shared directory is visible in all VPCs in the directory consumer account.
*
*
* The ShareMethod
parameter determines whether the specified directory can be shared between AWS
* accounts inside the same AWS organization (ORGANIZATIONS
). It also determines whether you can share
* the directory with any other AWS account either inside or outside of the organization (HANDSHAKE
).
*
*
* The ShareNotes
parameter is only used when HANDSHAKE
is called, which sends a directory
* sharing request to the directory consumer.
*
*
* @param shareDirectoryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ShareDirectory operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.ShareDirectory
* @see AWS API
* Documentation
*/
java.util.concurrent.Future shareDirectoryAsync(ShareDirectoryRequest shareDirectoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Applies a schema extension to a Microsoft AD directory.
*
*
* @param startSchemaExtensionRequest
* @return A Java Future containing the result of the StartSchemaExtension operation returned by the service.
* @sample AWSDirectoryServiceAsync.StartSchemaExtension
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startSchemaExtensionAsync(StartSchemaExtensionRequest startSchemaExtensionRequest);
/**
*
* Applies a schema extension to a Microsoft AD directory.
*
*
* @param startSchemaExtensionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartSchemaExtension operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.StartSchemaExtension
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startSchemaExtensionAsync(StartSchemaExtensionRequest startSchemaExtensionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops the directory sharing between the directory owner and consumer accounts.
*
*
* @param unshareDirectoryRequest
* @return A Java Future containing the result of the UnshareDirectory operation returned by the service.
* @sample AWSDirectoryServiceAsync.UnshareDirectory
* @see AWS API
* Documentation
*/
java.util.concurrent.Future unshareDirectoryAsync(UnshareDirectoryRequest unshareDirectoryRequest);
/**
*
* Stops the directory sharing between the directory owner and consumer accounts.
*
*
* @param unshareDirectoryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UnshareDirectory operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.UnshareDirectory
* @see AWS API
* Documentation
*/
java.util.concurrent.Future unshareDirectoryAsync(UnshareDirectoryRequest unshareDirectoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a conditional forwarder that has been set up for your AWS directory.
*
*
* @param updateConditionalForwarderRequest
* Updates a conditional forwarder.
* @return A Java Future containing the result of the UpdateConditionalForwarder operation returned by the service.
* @sample AWSDirectoryServiceAsync.UpdateConditionalForwarder
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateConditionalForwarderAsync(
UpdateConditionalForwarderRequest updateConditionalForwarderRequest);
/**
*
* Updates a conditional forwarder that has been set up for your AWS directory.
*
*
* @param updateConditionalForwarderRequest
* Updates a conditional forwarder.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateConditionalForwarder operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.UpdateConditionalForwarder
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateConditionalForwarderAsync(
UpdateConditionalForwarderRequest updateConditionalForwarderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds or removes domain controllers to or from the directory. Based on the difference between current value and
* new value (provided through this API call), domain controllers will be added or removed. It may take up to 45
* minutes for any new domain controllers to become fully active once the requested number of domain controllers is
* updated. During this time, you cannot make another update request.
*
*
* @param updateNumberOfDomainControllersRequest
* @return A Java Future containing the result of the UpdateNumberOfDomainControllers operation returned by the
* service.
* @sample AWSDirectoryServiceAsync.UpdateNumberOfDomainControllers
* @see AWS API Documentation
*/
java.util.concurrent.Future updateNumberOfDomainControllersAsync(
UpdateNumberOfDomainControllersRequest updateNumberOfDomainControllersRequest);
/**
*
* Adds or removes domain controllers to or from the directory. Based on the difference between current value and
* new value (provided through this API call), domain controllers will be added or removed. It may take up to 45
* minutes for any new domain controllers to become fully active once the requested number of domain controllers is
* updated. During this time, you cannot make another update request.
*
*
* @param updateNumberOfDomainControllersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateNumberOfDomainControllers operation returned by the
* service.
* @sample AWSDirectoryServiceAsyncHandler.UpdateNumberOfDomainControllers
* @see AWS API Documentation
*/
java.util.concurrent.Future updateNumberOfDomainControllersAsync(
UpdateNumberOfDomainControllersRequest updateNumberOfDomainControllersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the Remote Authentication Dial In User Service (RADIUS) server information for an AD Connector or
* Microsoft AD directory.
*
*
* @param updateRadiusRequest
* Contains the inputs for the UpdateRadius operation.
* @return A Java Future containing the result of the UpdateRadius operation returned by the service.
* @sample AWSDirectoryServiceAsync.UpdateRadius
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateRadiusAsync(UpdateRadiusRequest updateRadiusRequest);
/**
*
* Updates the Remote Authentication Dial In User Service (RADIUS) server information for an AD Connector or
* Microsoft AD directory.
*
*
* @param updateRadiusRequest
* Contains the inputs for the UpdateRadius operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateRadius operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.UpdateRadius
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateRadiusAsync(UpdateRadiusRequest updateRadiusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the trust that has been set up between your AWS Managed Microsoft AD directory and an on-premises Active
* Directory.
*
*
* @param updateTrustRequest
* @return A Java Future containing the result of the UpdateTrust operation returned by the service.
* @sample AWSDirectoryServiceAsync.UpdateTrust
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateTrustAsync(UpdateTrustRequest updateTrustRequest);
/**
*
* Updates the trust that has been set up between your AWS Managed Microsoft AD directory and an on-premises Active
* Directory.
*
*
* @param updateTrustRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateTrust operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.UpdateTrust
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateTrustAsync(UpdateTrustRequest updateTrustRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* AWS Directory Service for Microsoft Active Directory allows you to configure and verify trust relationships.
*
*
* This action verifies a trust relationship between your AWS Managed Microsoft AD directory and an external domain.
*
*
* @param verifyTrustRequest
* Initiates the verification of an existing trust relationship between an AWS Managed Microsoft AD directory
* and an external domain.
* @return A Java Future containing the result of the VerifyTrust operation returned by the service.
* @sample AWSDirectoryServiceAsync.VerifyTrust
* @see AWS API
* Documentation
*/
java.util.concurrent.Future verifyTrustAsync(VerifyTrustRequest verifyTrustRequest);
/**
*
* AWS Directory Service for Microsoft Active Directory allows you to configure and verify trust relationships.
*
*
* This action verifies a trust relationship between your AWS Managed Microsoft AD directory and an external domain.
*
*
* @param verifyTrustRequest
* Initiates the verification of an existing trust relationship between an AWS Managed Microsoft AD directory
* and an external domain.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the VerifyTrust operation returned by the service.
* @sample AWSDirectoryServiceAsyncHandler.VerifyTrust
* @see AWS API
* Documentation
*/
java.util.concurrent.Future verifyTrustAsync(VerifyTrustRequest verifyTrustRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}