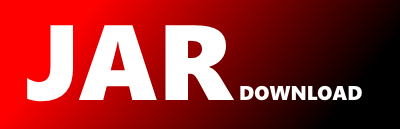
com.amazonaws.services.directory.AWSDirectoryServiceClient Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.directory;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.directory.AWSDirectoryServiceClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.directory.model.*;
import com.amazonaws.services.directory.model.transform.*;
/**
* Client for accessing Directory Service. All service calls made using this client are blocking, and will not return
* until the service call completes.
*
* Directory Service
*
* Directory Service is a web service that makes it easy for you to setup and run directories in the Amazon Web Services
* cloud, or connect your Amazon Web Services resources with an existing self-managed Microsoft Active Directory. This
* guide provides detailed information about Directory Service operations, data types, parameters, and errors. For
* information about Directory Services features, see Directory
* Service and the Directory
* Service Administration Guide.
*
*
*
* Amazon Web Services provides SDKs that consist of libraries and sample code for various programming languages and
* platforms (Java, Ruby, .Net, iOS, Android, etc.). The SDKs provide a convenient way to create programmatic access to
* Directory Service and other Amazon Web Services services. For more information about the Amazon Web Services SDKs,
* including how to download and install them, see Tools for Amazon Web
* Services.
*
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSDirectoryServiceClient extends AmazonWebServiceClient implements AWSDirectoryService {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSDirectoryService.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "ds";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("DirectoryAlreadySharedException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.DirectoryAlreadySharedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("DirectoryUnavailableException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.DirectoryUnavailableExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidLDAPSStatusException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.InvalidLDAPSStatusExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidCertificateException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.InvalidCertificateExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("DirectoryNotSharedException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.DirectoryNotSharedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("RegionLimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.RegionLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("EntityDoesNotExistException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.EntityDoesNotExistExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("DirectoryLimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.DirectoryLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("DirectoryDoesNotExistException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.DirectoryDoesNotExistExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidNextTokenException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.InvalidNextTokenExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("CertificateAlreadyExistsException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.CertificateAlreadyExistsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("DirectoryAlreadyInRegionException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.DirectoryAlreadyInRegionExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("DomainControllerLimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.DomainControllerLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UserDoesNotExistException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.UserDoesNotExistExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidPasswordException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.InvalidPasswordExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnsupportedOperationException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.UnsupportedOperationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ClientException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.ClientExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("IncompatibleSettingsException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.IncompatibleSettingsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AuthenticationFailedException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.AuthenticationFailedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("DirectoryInDesiredStateException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.DirectoryInDesiredStateExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InsufficientPermissionsException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.InsufficientPermissionsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("CertificateLimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.CertificateLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("OrganizationsException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.OrganizationsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidParameterException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.InvalidParameterExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("CertificateDoesNotExistException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.CertificateDoesNotExistExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.ServiceExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("SnapshotLimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.SnapshotLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("EntityAlreadyExistsException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.EntityAlreadyExistsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidClientAuthStatusException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.InvalidClientAuthStatusExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnsupportedSettingsException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.UnsupportedSettingsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("NoAvailableCertificateException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.NoAvailableCertificateExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidTargetException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.InvalidTargetExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("IpRouteLimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.IpRouteLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TagLimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.TagLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ShareLimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.ShareLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("CertificateInUseException").withExceptionUnmarshaller(
com.amazonaws.services.directory.model.transform.CertificateInUseExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.directory.model.AWSDirectoryServiceException.class));
/**
* Constructs a new client to invoke service methods on Directory Service. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AWSDirectoryServiceClientBuilder#defaultClient()}
*/
@Deprecated
public AWSDirectoryServiceClient() {
this(DefaultAWSCredentialsProviderChain.getInstance(), configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Directory Service. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientConfiguration
* The client configuration options controlling how this client connects to Directory Service (ex: proxy
* settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AWSDirectoryServiceClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSDirectoryServiceClient(ClientConfiguration clientConfiguration) {
this(DefaultAWSCredentialsProviderChain.getInstance(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on Directory Service using the specified AWS account
* credentials.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @deprecated use {@link AWSDirectoryServiceClientBuilder#withCredentials(AWSCredentialsProvider)} for example:
* {@code AWSDirectoryServiceClientBuilder.standard().withCredentials(new AWSStaticCredentialsProvider(awsCredentials)).build();}
*/
@Deprecated
public AWSDirectoryServiceClient(AWSCredentials awsCredentials) {
this(awsCredentials, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Directory Service using the specified AWS account
* credentials and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to Directory Service (ex: proxy
* settings, retry counts, etc.).
* @deprecated use {@link AWSDirectoryServiceClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSDirectoryServiceClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSDirectoryServiceClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
this.advancedConfig = AdvancedConfig.EMPTY;
init();
}
/**
* Constructs a new client to invoke service methods on Directory Service using the specified AWS account
* credentials provider.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @deprecated use {@link AWSDirectoryServiceClientBuilder#withCredentials(AWSCredentialsProvider)}
*/
@Deprecated
public AWSDirectoryServiceClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Directory Service using the specified AWS account
* credentials provider and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to Directory Service (ex: proxy
* settings, retry counts, etc.).
* @deprecated use {@link AWSDirectoryServiceClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSDirectoryServiceClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSDirectoryServiceClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on Directory Service using the specified AWS account
* credentials provider, client configuration options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to Directory Service (ex: proxy
* settings, retry counts, etc.).
* @param requestMetricCollector
* optional request metric collector
* @deprecated use {@link AWSDirectoryServiceClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSDirectoryServiceClientBuilder#withClientConfiguration(ClientConfiguration)} and
* {@link AWSDirectoryServiceClientBuilder#withMetricsCollector(RequestMetricCollector)}
*/
@Deprecated
public AWSDirectoryServiceClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
this.advancedConfig = AdvancedConfig.EMPTY;
init();
}
public static AWSDirectoryServiceClientBuilder builder() {
return AWSDirectoryServiceClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on Directory Service using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSDirectoryServiceClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on Directory Service using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSDirectoryServiceClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("https://ds.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/directory/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/directory/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Accepts a directory sharing request that was sent from the directory owner account.
*
*
* @param acceptSharedDirectoryRequest
* @return Result of the AcceptSharedDirectory operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws DirectoryAlreadySharedException
* The specified directory has already been shared with this Amazon Web Services account.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.AcceptSharedDirectory
* @see AWS API
* Documentation
*/
@Override
public AcceptSharedDirectoryResult acceptSharedDirectory(AcceptSharedDirectoryRequest request) {
request = beforeClientExecution(request);
return executeAcceptSharedDirectory(request);
}
@SdkInternalApi
final AcceptSharedDirectoryResult executeAcceptSharedDirectory(AcceptSharedDirectoryRequest acceptSharedDirectoryRequest) {
ExecutionContext executionContext = createExecutionContext(acceptSharedDirectoryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AcceptSharedDirectoryRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(acceptSharedDirectoryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AcceptSharedDirectory");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AcceptSharedDirectoryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* If the DNS server for your self-managed domain uses a publicly addressable IP address, you must add a CIDR
* address block to correctly route traffic to and from your Microsoft AD on Amazon Web Services. AddIpRoutes
* adds this address block. You can also use AddIpRoutes to facilitate routing traffic that uses public IP
* ranges from your Microsoft AD on Amazon Web Services to a peer VPC.
*
*
* Before you call AddIpRoutes, ensure that all of the required permissions have been explicitly granted
* through a policy. For details about what permissions are required to run the AddIpRoutes operation, see
* Directory Service API Permissions: Actions, Resources, and Conditions Reference.
*
*
* @param addIpRoutesRequest
* @return Result of the AddIpRoutes operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws EntityAlreadyExistsException
* The specified entity already exists.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws IpRouteLimitExceededException
* The maximum allowed number of IP addresses was exceeded. The default limit is 100 IP address blocks.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.AddIpRoutes
* @see AWS API
* Documentation
*/
@Override
public AddIpRoutesResult addIpRoutes(AddIpRoutesRequest request) {
request = beforeClientExecution(request);
return executeAddIpRoutes(request);
}
@SdkInternalApi
final AddIpRoutesResult executeAddIpRoutes(AddIpRoutesRequest addIpRoutesRequest) {
ExecutionContext executionContext = createExecutionContext(addIpRoutesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AddIpRoutesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(addIpRoutesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AddIpRoutes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new AddIpRoutesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds two domain controllers in the specified Region for the specified directory.
*
*
* @param addRegionRequest
* @return Result of the AddRegion operation returned by the service.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws DirectoryAlreadyInRegionException
* The Region you specified is the same Region where the Managed Microsoft AD directory was created. Specify
* a different Region and try again.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws RegionLimitExceededException
* You have reached the limit for maximum number of simultaneous Region replications per directory.
* @throws AccessDeniedException
* Client authentication is not available in this region at this time.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.AddRegion
* @see AWS API
* Documentation
*/
@Override
public AddRegionResult addRegion(AddRegionRequest request) {
request = beforeClientExecution(request);
return executeAddRegion(request);
}
@SdkInternalApi
final AddRegionResult executeAddRegion(AddRegionRequest addRegionRequest) {
ExecutionContext executionContext = createExecutionContext(addRegionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AddRegionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(addRegionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AddRegion");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new AddRegionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds or overwrites one or more tags for the specified directory. Each directory can have a maximum of 50 tags.
* Each tag consists of a key and optional value. Tag keys must be unique to each resource.
*
*
* @param addTagsToResourceRequest
* @return Result of the AddTagsToResource operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws TagLimitExceededException
* The maximum allowed number of tags was exceeded.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.AddTagsToResource
* @see AWS API
* Documentation
*/
@Override
public AddTagsToResourceResult addTagsToResource(AddTagsToResourceRequest request) {
request = beforeClientExecution(request);
return executeAddTagsToResource(request);
}
@SdkInternalApi
final AddTagsToResourceResult executeAddTagsToResource(AddTagsToResourceRequest addTagsToResourceRequest) {
ExecutionContext executionContext = createExecutionContext(addTagsToResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AddTagsToResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(addTagsToResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AddTagsToResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new AddTagsToResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Cancels an in-progress schema extension to a Microsoft AD directory. Once a schema extension has started
* replicating to all domain controllers, the task can no longer be canceled. A schema extension can be canceled
* during any of the following states; Initializing
, CreatingSnapshot
, and
* UpdatingSchema
.
*
*
* @param cancelSchemaExtensionRequest
* @return Result of the CancelSchemaExtension operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.CancelSchemaExtension
* @see AWS API
* Documentation
*/
@Override
public CancelSchemaExtensionResult cancelSchemaExtension(CancelSchemaExtensionRequest request) {
request = beforeClientExecution(request);
return executeCancelSchemaExtension(request);
}
@SdkInternalApi
final CancelSchemaExtensionResult executeCancelSchemaExtension(CancelSchemaExtensionRequest cancelSchemaExtensionRequest) {
ExecutionContext executionContext = createExecutionContext(cancelSchemaExtensionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CancelSchemaExtensionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(cancelSchemaExtensionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CancelSchemaExtension");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CancelSchemaExtensionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an AD Connector to connect to a self-managed directory.
*
*
* Before you call ConnectDirectory
, ensure that all of the required permissions have been explicitly
* granted through a policy. For details about what permissions are required to run the
* ConnectDirectory
operation, see Directory Service API Permissions: Actions, Resources, and Conditions Reference.
*
*
* @param connectDirectoryRequest
* Contains the inputs for the ConnectDirectory operation.
* @return Result of the ConnectDirectory operation returned by the service.
* @throws DirectoryLimitExceededException
* The maximum number of directories in the region has been reached. You can use the
* GetDirectoryLimits operation to determine your directory limits in the region.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.ConnectDirectory
* @see AWS API
* Documentation
*/
@Override
public ConnectDirectoryResult connectDirectory(ConnectDirectoryRequest request) {
request = beforeClientExecution(request);
return executeConnectDirectory(request);
}
@SdkInternalApi
final ConnectDirectoryResult executeConnectDirectory(ConnectDirectoryRequest connectDirectoryRequest) {
ExecutionContext executionContext = createExecutionContext(connectDirectoryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ConnectDirectoryRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(connectDirectoryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ConnectDirectory");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ConnectDirectoryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an alias for a directory and assigns the alias to the directory. The alias is used to construct the
* access URL for the directory, such as http://<alias>.awsapps.com
.
*
*
*
* After an alias has been created, it cannot be deleted or reused, so this operation should only be used when
* absolutely necessary.
*
*
*
* @param createAliasRequest
* Contains the inputs for the CreateAlias operation.
* @return Result of the CreateAlias operation returned by the service.
* @throws EntityAlreadyExistsException
* The specified entity already exists.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.CreateAlias
* @see AWS API
* Documentation
*/
@Override
public CreateAliasResult createAlias(CreateAliasRequest request) {
request = beforeClientExecution(request);
return executeCreateAlias(request);
}
@SdkInternalApi
final CreateAliasResult executeCreateAlias(CreateAliasRequest createAliasRequest) {
ExecutionContext executionContext = createExecutionContext(createAliasRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAliasRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createAliasRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAlias");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateAliasResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Active Directory computer object in the specified directory.
*
*
* @param createComputerRequest
* Contains the inputs for the CreateComputer operation.
* @return Result of the CreateComputer operation returned by the service.
* @throws AuthenticationFailedException
* An authentication error occurred.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws EntityAlreadyExistsException
* The specified entity already exists.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.CreateComputer
* @see AWS API
* Documentation
*/
@Override
public CreateComputerResult createComputer(CreateComputerRequest request) {
request = beforeClientExecution(request);
return executeCreateComputer(request);
}
@SdkInternalApi
final CreateComputerResult executeCreateComputer(CreateComputerRequest createComputerRequest) {
ExecutionContext executionContext = createExecutionContext(createComputerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateComputerRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createComputerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateComputer");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateComputerResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a conditional forwarder associated with your Amazon Web Services directory. Conditional forwarders are
* required in order to set up a trust relationship with another domain. The conditional forwarder points to the
* trusted domain.
*
*
* @param createConditionalForwarderRequest
* Initiates the creation of a conditional forwarder for your Directory Service for Microsoft Active
* Directory. Conditional forwarders are required in order to set up a trust relationship with another
* domain.
* @return Result of the CreateConditionalForwarder operation returned by the service.
* @throws EntityAlreadyExistsException
* The specified entity already exists.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.CreateConditionalForwarder
* @see AWS
* API Documentation
*/
@Override
public CreateConditionalForwarderResult createConditionalForwarder(CreateConditionalForwarderRequest request) {
request = beforeClientExecution(request);
return executeCreateConditionalForwarder(request);
}
@SdkInternalApi
final CreateConditionalForwarderResult executeCreateConditionalForwarder(CreateConditionalForwarderRequest createConditionalForwarderRequest) {
ExecutionContext executionContext = createExecutionContext(createConditionalForwarderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateConditionalForwarderRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createConditionalForwarderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateConditionalForwarder");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateConditionalForwarderResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a Simple AD directory. For more information, see Simple Active
* Directory in the Directory Service Admin Guide.
*
*
* Before you call CreateDirectory
, ensure that all of the required permissions have been explicitly
* granted through a policy. For details about what permissions are required to run the CreateDirectory
* operation, see Directory Service API Permissions: Actions, Resources, and Conditions Reference.
*
*
* @param createDirectoryRequest
* Contains the inputs for the CreateDirectory operation.
* @return Result of the CreateDirectory operation returned by the service.
* @throws DirectoryLimitExceededException
* The maximum number of directories in the region has been reached. You can use the
* GetDirectoryLimits operation to determine your directory limits in the region.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.CreateDirectory
* @see AWS API
* Documentation
*/
@Override
public CreateDirectoryResult createDirectory(CreateDirectoryRequest request) {
request = beforeClientExecution(request);
return executeCreateDirectory(request);
}
@SdkInternalApi
final CreateDirectoryResult executeCreateDirectory(CreateDirectoryRequest createDirectoryRequest) {
ExecutionContext executionContext = createExecutionContext(createDirectoryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDirectoryRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createDirectoryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDirectory");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateDirectoryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a subscription to forward real-time Directory Service domain controller security logs to the specified
* Amazon CloudWatch log group in your Amazon Web Services account.
*
*
* @param createLogSubscriptionRequest
* @return Result of the CreateLogSubscription operation returned by the service.
* @throws EntityAlreadyExistsException
* The specified entity already exists.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws InsufficientPermissionsException
* The account does not have sufficient permission to perform the operation.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.CreateLogSubscription
* @see AWS API
* Documentation
*/
@Override
public CreateLogSubscriptionResult createLogSubscription(CreateLogSubscriptionRequest request) {
request = beforeClientExecution(request);
return executeCreateLogSubscription(request);
}
@SdkInternalApi
final CreateLogSubscriptionResult executeCreateLogSubscription(CreateLogSubscriptionRequest createLogSubscriptionRequest) {
ExecutionContext executionContext = createExecutionContext(createLogSubscriptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateLogSubscriptionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createLogSubscriptionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateLogSubscription");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateLogSubscriptionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a Microsoft AD directory in the Amazon Web Services Cloud. For more information, see Managed
* Microsoft AD in the Directory Service Admin Guide.
*
*
* Before you call CreateMicrosoftAD, ensure that all of the required permissions have been explicitly
* granted through a policy. For details about what permissions are required to run the CreateMicrosoftAD
* operation, see Directory Service API Permissions: Actions, Resources, and Conditions Reference.
*
*
* @param createMicrosoftADRequest
* Creates an Managed Microsoft AD directory.
* @return Result of the CreateMicrosoftAD operation returned by the service.
* @throws DirectoryLimitExceededException
* The maximum number of directories in the region has been reached. You can use the
* GetDirectoryLimits operation to determine your directory limits in the region.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @throws UnsupportedOperationException
* The operation is not supported.
* @sample AWSDirectoryService.CreateMicrosoftAD
* @see AWS API
* Documentation
*/
@Override
public CreateMicrosoftADResult createMicrosoftAD(CreateMicrosoftADRequest request) {
request = beforeClientExecution(request);
return executeCreateMicrosoftAD(request);
}
@SdkInternalApi
final CreateMicrosoftADResult executeCreateMicrosoftAD(CreateMicrosoftADRequest createMicrosoftADRequest) {
ExecutionContext executionContext = createExecutionContext(createMicrosoftADRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMicrosoftADRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createMicrosoftADRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMicrosoftAD");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateMicrosoftADResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a snapshot of a Simple AD or Microsoft AD directory in the Amazon Web Services cloud.
*
*
*
* You cannot take snapshots of AD Connector directories.
*
*
*
* @param createSnapshotRequest
* Contains the inputs for the CreateSnapshot operation.
* @return Result of the CreateSnapshot operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws SnapshotLimitExceededException
* The maximum number of manual snapshots for the directory has been reached. You can use the
* GetSnapshotLimits operation to determine the snapshot limits for a directory.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.CreateSnapshot
* @see AWS API
* Documentation
*/
@Override
public CreateSnapshotResult createSnapshot(CreateSnapshotRequest request) {
request = beforeClientExecution(request);
return executeCreateSnapshot(request);
}
@SdkInternalApi
final CreateSnapshotResult executeCreateSnapshot(CreateSnapshotRequest createSnapshotRequest) {
ExecutionContext executionContext = createExecutionContext(createSnapshotRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSnapshotRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createSnapshotRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateSnapshot");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateSnapshotResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Directory Service for Microsoft Active Directory allows you to configure trust relationships. For example, you
* can establish a trust between your Managed Microsoft AD directory, and your existing self-managed Microsoft
* Active Directory. This would allow you to provide users and groups access to resources in either domain, with a
* single set of credentials.
*
*
* This action initiates the creation of the Amazon Web Services side of a trust relationship between an Managed
* Microsoft AD directory and an external domain. You can create either a forest trust or an external trust.
*
*
* @param createTrustRequest
* Directory Service for Microsoft Active Directory allows you to configure trust relationships. For example,
* you can establish a trust between your Managed Microsoft AD directory, and your existing self-managed
* Microsoft Active Directory. This would allow you to provide users and groups access to resources in either
* domain, with a single set of credentials.
*
* This action initiates the creation of the Amazon Web Services side of a trust relationship between an
* Managed Microsoft AD directory and an external domain.
* @return Result of the CreateTrust operation returned by the service.
* @throws EntityAlreadyExistsException
* The specified entity already exists.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @throws UnsupportedOperationException
* The operation is not supported.
* @sample AWSDirectoryService.CreateTrust
* @see AWS API
* Documentation
*/
@Override
public CreateTrustResult createTrust(CreateTrustRequest request) {
request = beforeClientExecution(request);
return executeCreateTrust(request);
}
@SdkInternalApi
final CreateTrustResult executeCreateTrust(CreateTrustRequest createTrustRequest) {
ExecutionContext executionContext = createExecutionContext(createTrustRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateTrustRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createTrustRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateTrust");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateTrustResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a conditional forwarder that has been set up for your Amazon Web Services directory.
*
*
* @param deleteConditionalForwarderRequest
* Deletes a conditional forwarder.
* @return Result of the DeleteConditionalForwarder operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.DeleteConditionalForwarder
* @see AWS
* API Documentation
*/
@Override
public DeleteConditionalForwarderResult deleteConditionalForwarder(DeleteConditionalForwarderRequest request) {
request = beforeClientExecution(request);
return executeDeleteConditionalForwarder(request);
}
@SdkInternalApi
final DeleteConditionalForwarderResult executeDeleteConditionalForwarder(DeleteConditionalForwarderRequest deleteConditionalForwarderRequest) {
ExecutionContext executionContext = createExecutionContext(deleteConditionalForwarderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteConditionalForwarderRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteConditionalForwarderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteConditionalForwarder");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteConditionalForwarderResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an Directory Service directory.
*
*
* Before you call DeleteDirectory
, ensure that all of the required permissions have been explicitly
* granted through a policy. For details about what permissions are required to run the DeleteDirectory
* operation, see Directory Service API Permissions: Actions, Resources, and Conditions Reference.
*
*
* @param deleteDirectoryRequest
* Contains the inputs for the DeleteDirectory operation.
* @return Result of the DeleteDirectory operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.DeleteDirectory
* @see AWS API
* Documentation
*/
@Override
public DeleteDirectoryResult deleteDirectory(DeleteDirectoryRequest request) {
request = beforeClientExecution(request);
return executeDeleteDirectory(request);
}
@SdkInternalApi
final DeleteDirectoryResult executeDeleteDirectory(DeleteDirectoryRequest deleteDirectoryRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDirectoryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDirectoryRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteDirectoryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDirectory");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteDirectoryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified log subscription.
*
*
* @param deleteLogSubscriptionRequest
* @return Result of the DeleteLogSubscription operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.DeleteLogSubscription
* @see AWS API
* Documentation
*/
@Override
public DeleteLogSubscriptionResult deleteLogSubscription(DeleteLogSubscriptionRequest request) {
request = beforeClientExecution(request);
return executeDeleteLogSubscription(request);
}
@SdkInternalApi
final DeleteLogSubscriptionResult executeDeleteLogSubscription(DeleteLogSubscriptionRequest deleteLogSubscriptionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteLogSubscriptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteLogSubscriptionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteLogSubscriptionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteLogSubscription");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteLogSubscriptionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a directory snapshot.
*
*
* @param deleteSnapshotRequest
* Contains the inputs for the DeleteSnapshot operation.
* @return Result of the DeleteSnapshot operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.DeleteSnapshot
* @see AWS API
* Documentation
*/
@Override
public DeleteSnapshotResult deleteSnapshot(DeleteSnapshotRequest request) {
request = beforeClientExecution(request);
return executeDeleteSnapshot(request);
}
@SdkInternalApi
final DeleteSnapshotResult executeDeleteSnapshot(DeleteSnapshotRequest deleteSnapshotRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSnapshotRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteSnapshotRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteSnapshotRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteSnapshot");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteSnapshotResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an existing trust relationship between your Managed Microsoft AD directory and an external domain.
*
*
* @param deleteTrustRequest
* Deletes the local side of an existing trust relationship between the Managed Microsoft AD directory and
* the external domain.
* @return Result of the DeleteTrust operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @throws UnsupportedOperationException
* The operation is not supported.
* @sample AWSDirectoryService.DeleteTrust
* @see AWS API
* Documentation
*/
@Override
public DeleteTrustResult deleteTrust(DeleteTrustRequest request) {
request = beforeClientExecution(request);
return executeDeleteTrust(request);
}
@SdkInternalApi
final DeleteTrustResult executeDeleteTrust(DeleteTrustRequest deleteTrustRequest) {
ExecutionContext executionContext = createExecutionContext(deleteTrustRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteTrustRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteTrustRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteTrust");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteTrustResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes from the system the certificate that was registered for secure LDAP or client certificate authentication.
*
*
* @param deregisterCertificateRequest
* @return Result of the DeregisterCertificate operation returned by the service.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws CertificateDoesNotExistException
* The certificate is not present in the system for describe or deregister activities.
* @throws CertificateInUseException
* The certificate is being used for the LDAP security connection and cannot be removed without disabling
* LDAP security.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.DeregisterCertificate
* @see AWS API
* Documentation
*/
@Override
public DeregisterCertificateResult deregisterCertificate(DeregisterCertificateRequest request) {
request = beforeClientExecution(request);
return executeDeregisterCertificate(request);
}
@SdkInternalApi
final DeregisterCertificateResult executeDeregisterCertificate(DeregisterCertificateRequest deregisterCertificateRequest) {
ExecutionContext executionContext = createExecutionContext(deregisterCertificateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeregisterCertificateRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deregisterCertificateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeregisterCertificate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeregisterCertificateResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the specified directory as a publisher to the specified Amazon SNS topic.
*
*
* @param deregisterEventTopicRequest
* Removes the specified directory as a publisher to the specified Amazon SNS topic.
* @return Result of the DeregisterEventTopic operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.DeregisterEventTopic
* @see AWS API
* Documentation
*/
@Override
public DeregisterEventTopicResult deregisterEventTopic(DeregisterEventTopicRequest request) {
request = beforeClientExecution(request);
return executeDeregisterEventTopic(request);
}
@SdkInternalApi
final DeregisterEventTopicResult executeDeregisterEventTopic(DeregisterEventTopicRequest deregisterEventTopicRequest) {
ExecutionContext executionContext = createExecutionContext(deregisterEventTopicRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeregisterEventTopicRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deregisterEventTopicRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeregisterEventTopic");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeregisterEventTopicResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Displays information about the certificate registered for secure LDAP or client certificate authentication.
*
*
* @param describeCertificateRequest
* @return Result of the DescribeCertificate operation returned by the service.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws CertificateDoesNotExistException
* The certificate is not present in the system for describe or deregister activities.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.DescribeCertificate
* @see AWS API
* Documentation
*/
@Override
public DescribeCertificateResult describeCertificate(DescribeCertificateRequest request) {
request = beforeClientExecution(request);
return executeDescribeCertificate(request);
}
@SdkInternalApi
final DescribeCertificateResult executeDescribeCertificate(DescribeCertificateRequest describeCertificateRequest) {
ExecutionContext executionContext = createExecutionContext(describeCertificateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeCertificateRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeCertificateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeCertificate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeCertificateResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about the type of client authentication for the specified directory, if the type is
* specified. If no type is specified, information about all client authentication types that are supported for the
* specified directory is retrieved. Currently, only SmartCard
is supported.
*
*
* @param describeClientAuthenticationSettingsRequest
* @return Result of the DescribeClientAuthenticationSettings operation returned by the service.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws AccessDeniedException
* Client authentication is not available in this region at this time.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.DescribeClientAuthenticationSettings
* @see AWS API Documentation
*/
@Override
public DescribeClientAuthenticationSettingsResult describeClientAuthenticationSettings(DescribeClientAuthenticationSettingsRequest request) {
request = beforeClientExecution(request);
return executeDescribeClientAuthenticationSettings(request);
}
@SdkInternalApi
final DescribeClientAuthenticationSettingsResult executeDescribeClientAuthenticationSettings(
DescribeClientAuthenticationSettingsRequest describeClientAuthenticationSettingsRequest) {
ExecutionContext executionContext = createExecutionContext(describeClientAuthenticationSettingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeClientAuthenticationSettingsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeClientAuthenticationSettingsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeClientAuthenticationSettings");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeClientAuthenticationSettingsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Obtains information about the conditional forwarders for this account.
*
*
* If no input parameters are provided for RemoteDomainNames, this request describes all conditional forwarders for
* the specified directory ID.
*
*
* @param describeConditionalForwardersRequest
* Describes a conditional forwarder.
* @return Result of the DescribeConditionalForwarders operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.DescribeConditionalForwarders
* @see AWS API Documentation
*/
@Override
public DescribeConditionalForwardersResult describeConditionalForwarders(DescribeConditionalForwardersRequest request) {
request = beforeClientExecution(request);
return executeDescribeConditionalForwarders(request);
}
@SdkInternalApi
final DescribeConditionalForwardersResult executeDescribeConditionalForwarders(DescribeConditionalForwardersRequest describeConditionalForwardersRequest) {
ExecutionContext executionContext = createExecutionContext(describeConditionalForwardersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeConditionalForwardersRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeConditionalForwardersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeConditionalForwarders");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeConditionalForwardersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Obtains information about the directories that belong to this account.
*
*
* You can retrieve information about specific directories by passing the directory identifiers in the
* DirectoryIds
parameter. Otherwise, all directories that belong to the current account are returned.
*
*
* This operation supports pagination with the use of the NextToken
request and response parameters. If
* more results are available, the DescribeDirectoriesResult.NextToken
member contains a token that you
* pass in the next call to DescribeDirectories to retrieve the next set of items.
*
*
* You can also specify a maximum number of return results with the Limit
parameter.
*
*
* @param describeDirectoriesRequest
* Contains the inputs for the DescribeDirectories operation.
* @return Result of the DescribeDirectories operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.DescribeDirectories
* @see AWS API
* Documentation
*/
@Override
public DescribeDirectoriesResult describeDirectories(DescribeDirectoriesRequest request) {
request = beforeClientExecution(request);
return executeDescribeDirectories(request);
}
@SdkInternalApi
final DescribeDirectoriesResult executeDescribeDirectories(DescribeDirectoriesRequest describeDirectoriesRequest) {
ExecutionContext executionContext = createExecutionContext(describeDirectoriesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDirectoriesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeDirectoriesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDirectories");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeDirectoriesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeDirectoriesResult describeDirectories() {
return describeDirectories(new DescribeDirectoriesRequest());
}
/**
*
* Provides information about any domain controllers in your directory.
*
*
* @param describeDomainControllersRequest
* @return Result of the DescribeDomainControllers operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @throws UnsupportedOperationException
* The operation is not supported.
* @sample AWSDirectoryService.DescribeDomainControllers
* @see AWS
* API Documentation
*/
@Override
public DescribeDomainControllersResult describeDomainControllers(DescribeDomainControllersRequest request) {
request = beforeClientExecution(request);
return executeDescribeDomainControllers(request);
}
@SdkInternalApi
final DescribeDomainControllersResult executeDescribeDomainControllers(DescribeDomainControllersRequest describeDomainControllersRequest) {
ExecutionContext executionContext = createExecutionContext(describeDomainControllersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDomainControllersRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeDomainControllersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDomainControllers");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeDomainControllersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Obtains information about which Amazon SNS topics receive status messages from the specified directory.
*
*
* If no input parameters are provided, such as DirectoryId or TopicName, this request describes all of the
* associations in the account.
*
*
* @param describeEventTopicsRequest
* Describes event topics.
* @return Result of the DescribeEventTopics operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.DescribeEventTopics
* @see AWS API
* Documentation
*/
@Override
public DescribeEventTopicsResult describeEventTopics(DescribeEventTopicsRequest request) {
request = beforeClientExecution(request);
return executeDescribeEventTopics(request);
}
@SdkInternalApi
final DescribeEventTopicsResult executeDescribeEventTopics(DescribeEventTopicsRequest describeEventTopicsRequest) {
ExecutionContext executionContext = createExecutionContext(describeEventTopicsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeEventTopicsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeEventTopicsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeEventTopics");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeEventTopicsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the status of LDAP security for the specified directory.
*
*
* @param describeLDAPSSettingsRequest
* @return Result of the DescribeLDAPSSettings operation returned by the service.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.DescribeLDAPSSettings
* @see AWS API
* Documentation
*/
@Override
public DescribeLDAPSSettingsResult describeLDAPSSettings(DescribeLDAPSSettingsRequest request) {
request = beforeClientExecution(request);
return executeDescribeLDAPSSettings(request);
}
@SdkInternalApi
final DescribeLDAPSSettingsResult executeDescribeLDAPSSettings(DescribeLDAPSSettingsRequest describeLDAPSSettingsRequest) {
ExecutionContext executionContext = createExecutionContext(describeLDAPSSettingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeLDAPSSettingsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeLDAPSSettingsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeLDAPSSettings");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeLDAPSSettingsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Provides information about the Regions that are configured for multi-Region replication.
*
*
* @param describeRegionsRequest
* @return Result of the DescribeRegions operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws AccessDeniedException
* Client authentication is not available in this region at this time.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.DescribeRegions
* @see AWS API
* Documentation
*/
@Override
public DescribeRegionsResult describeRegions(DescribeRegionsRequest request) {
request = beforeClientExecution(request);
return executeDescribeRegions(request);
}
@SdkInternalApi
final DescribeRegionsResult executeDescribeRegions(DescribeRegionsRequest describeRegionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeRegionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeRegionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeRegionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeRegions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeRegionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about the configurable settings for the specified directory.
*
*
* @param describeSettingsRequest
* @return Result of the DescribeSettings operation returned by the service.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.DescribeSettings
* @see AWS API
* Documentation
*/
@Override
public DescribeSettingsResult describeSettings(DescribeSettingsRequest request) {
request = beforeClientExecution(request);
return executeDescribeSettings(request);
}
@SdkInternalApi
final DescribeSettingsResult executeDescribeSettings(DescribeSettingsRequest describeSettingsRequest) {
ExecutionContext executionContext = createExecutionContext(describeSettingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeSettingsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeSettingsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeSettings");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeSettingsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the shared directories in your account.
*
*
* @param describeSharedDirectoriesRequest
* @return Result of the DescribeSharedDirectories operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.DescribeSharedDirectories
* @see AWS
* API Documentation
*/
@Override
public DescribeSharedDirectoriesResult describeSharedDirectories(DescribeSharedDirectoriesRequest request) {
request = beforeClientExecution(request);
return executeDescribeSharedDirectories(request);
}
@SdkInternalApi
final DescribeSharedDirectoriesResult executeDescribeSharedDirectories(DescribeSharedDirectoriesRequest describeSharedDirectoriesRequest) {
ExecutionContext executionContext = createExecutionContext(describeSharedDirectoriesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeSharedDirectoriesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeSharedDirectoriesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeSharedDirectories");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeSharedDirectoriesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Obtains information about the directory snapshots that belong to this account.
*
*
* This operation supports pagination with the use of the NextToken request and response parameters. If more
* results are available, the DescribeSnapshots.NextToken member contains a token that you pass in the next
* call to DescribeSnapshots to retrieve the next set of items.
*
*
* You can also specify a maximum number of return results with the Limit parameter.
*
*
* @param describeSnapshotsRequest
* Contains the inputs for the DescribeSnapshots operation.
* @return Result of the DescribeSnapshots operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.DescribeSnapshots
* @see AWS API
* Documentation
*/
@Override
public DescribeSnapshotsResult describeSnapshots(DescribeSnapshotsRequest request) {
request = beforeClientExecution(request);
return executeDescribeSnapshots(request);
}
@SdkInternalApi
final DescribeSnapshotsResult executeDescribeSnapshots(DescribeSnapshotsRequest describeSnapshotsRequest) {
ExecutionContext executionContext = createExecutionContext(describeSnapshotsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeSnapshotsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeSnapshotsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeSnapshots");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeSnapshotsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeSnapshotsResult describeSnapshots() {
return describeSnapshots(new DescribeSnapshotsRequest());
}
/**
*
* Obtains information about the trust relationships for this account.
*
*
* If no input parameters are provided, such as DirectoryId or TrustIds, this request describes all the trust
* relationships belonging to the account.
*
*
* @param describeTrustsRequest
* Describes the trust relationships for a particular Managed Microsoft AD directory. If no input parameters
* are provided, such as directory ID or trust ID, this request describes all the trust relationships.
* @return Result of the DescribeTrusts operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @throws UnsupportedOperationException
* The operation is not supported.
* @sample AWSDirectoryService.DescribeTrusts
* @see AWS API
* Documentation
*/
@Override
public DescribeTrustsResult describeTrusts(DescribeTrustsRequest request) {
request = beforeClientExecution(request);
return executeDescribeTrusts(request);
}
@SdkInternalApi
final DescribeTrustsResult executeDescribeTrusts(DescribeTrustsRequest describeTrustsRequest) {
ExecutionContext executionContext = createExecutionContext(describeTrustsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeTrustsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeTrustsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeTrusts");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeTrustsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the updates of a directory for a particular update type.
*
*
* @param describeUpdateDirectoryRequest
* @return Result of the DescribeUpdateDirectory operation returned by the service.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws AccessDeniedException
* Client authentication is not available in this region at this time.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @sample AWSDirectoryService.DescribeUpdateDirectory
* @see AWS API
* Documentation
*/
@Override
public DescribeUpdateDirectoryResult describeUpdateDirectory(DescribeUpdateDirectoryRequest request) {
request = beforeClientExecution(request);
return executeDescribeUpdateDirectory(request);
}
@SdkInternalApi
final DescribeUpdateDirectoryResult executeDescribeUpdateDirectory(DescribeUpdateDirectoryRequest describeUpdateDirectoryRequest) {
ExecutionContext executionContext = createExecutionContext(describeUpdateDirectoryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeUpdateDirectoryRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeUpdateDirectoryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeUpdateDirectory");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeUpdateDirectoryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disables alternative client authentication methods for the specified directory.
*
*
* @param disableClientAuthenticationRequest
* @return Result of the DisableClientAuthentication operation returned by the service.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws InvalidClientAuthStatusException
* Client authentication is already enabled.
* @throws AccessDeniedException
* Client authentication is not available in this region at this time.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.DisableClientAuthentication
* @see AWS
* API Documentation
*/
@Override
public DisableClientAuthenticationResult disableClientAuthentication(DisableClientAuthenticationRequest request) {
request = beforeClientExecution(request);
return executeDisableClientAuthentication(request);
}
@SdkInternalApi
final DisableClientAuthenticationResult executeDisableClientAuthentication(DisableClientAuthenticationRequest disableClientAuthenticationRequest) {
ExecutionContext executionContext = createExecutionContext(disableClientAuthenticationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisableClientAuthenticationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disableClientAuthenticationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisableClientAuthentication");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisableClientAuthenticationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deactivates LDAP secure calls for the specified directory.
*
*
* @param disableLDAPSRequest
* @return Result of the DisableLDAPS operation returned by the service.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws InvalidLDAPSStatusException
* The LDAP activities could not be performed because they are limited by the LDAPS status.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.DisableLDAPS
* @see AWS API
* Documentation
*/
@Override
public DisableLDAPSResult disableLDAPS(DisableLDAPSRequest request) {
request = beforeClientExecution(request);
return executeDisableLDAPS(request);
}
@SdkInternalApi
final DisableLDAPSResult executeDisableLDAPS(DisableLDAPSRequest disableLDAPSRequest) {
ExecutionContext executionContext = createExecutionContext(disableLDAPSRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisableLDAPSRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(disableLDAPSRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisableLDAPS");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DisableLDAPSResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disables multi-factor authentication (MFA) with the Remote Authentication Dial In User Service (RADIUS) server
* for an AD Connector or Microsoft AD directory.
*
*
* @param disableRadiusRequest
* Contains the inputs for the DisableRadius operation.
* @return Result of the DisableRadius operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.DisableRadius
* @see AWS API
* Documentation
*/
@Override
public DisableRadiusResult disableRadius(DisableRadiusRequest request) {
request = beforeClientExecution(request);
return executeDisableRadius(request);
}
@SdkInternalApi
final DisableRadiusResult executeDisableRadius(DisableRadiusRequest disableRadiusRequest) {
ExecutionContext executionContext = createExecutionContext(disableRadiusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisableRadiusRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(disableRadiusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisableRadius");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DisableRadiusResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disables single-sign on for a directory.
*
*
* @param disableSsoRequest
* Contains the inputs for the DisableSso operation.
* @return Result of the DisableSso operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InsufficientPermissionsException
* The account does not have sufficient permission to perform the operation.
* @throws AuthenticationFailedException
* An authentication error occurred.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.DisableSso
* @see AWS API
* Documentation
*/
@Override
public DisableSsoResult disableSso(DisableSsoRequest request) {
request = beforeClientExecution(request);
return executeDisableSso(request);
}
@SdkInternalApi
final DisableSsoResult executeDisableSso(DisableSsoRequest disableSsoRequest) {
ExecutionContext executionContext = createExecutionContext(disableSsoRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisableSsoRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(disableSsoRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisableSso");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new DisableSsoResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enables alternative client authentication methods for the specified directory.
*
*
* @param enableClientAuthenticationRequest
* @return Result of the EnableClientAuthentication operation returned by the service.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws InvalidClientAuthStatusException
* Client authentication is already enabled.
* @throws AccessDeniedException
* Client authentication is not available in this region at this time.
* @throws NoAvailableCertificateException
* Client authentication setup could not be completed because at least one valid certificate must be
* registered in the system.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.EnableClientAuthentication
* @see AWS
* API Documentation
*/
@Override
public EnableClientAuthenticationResult enableClientAuthentication(EnableClientAuthenticationRequest request) {
request = beforeClientExecution(request);
return executeEnableClientAuthentication(request);
}
@SdkInternalApi
final EnableClientAuthenticationResult executeEnableClientAuthentication(EnableClientAuthenticationRequest enableClientAuthenticationRequest) {
ExecutionContext executionContext = createExecutionContext(enableClientAuthenticationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new EnableClientAuthenticationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(enableClientAuthenticationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "EnableClientAuthentication");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new EnableClientAuthenticationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Activates the switch for the specific directory to always use LDAP secure calls.
*
*
* @param enableLDAPSRequest
* @return Result of the EnableLDAPS operation returned by the service.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws NoAvailableCertificateException
* Client authentication setup could not be completed because at least one valid certificate must be
* registered in the system.
* @throws InvalidLDAPSStatusException
* The LDAP activities could not be performed because they are limited by the LDAPS status.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.EnableLDAPS
* @see AWS API
* Documentation
*/
@Override
public EnableLDAPSResult enableLDAPS(EnableLDAPSRequest request) {
request = beforeClientExecution(request);
return executeEnableLDAPS(request);
}
@SdkInternalApi
final EnableLDAPSResult executeEnableLDAPS(EnableLDAPSRequest enableLDAPSRequest) {
ExecutionContext executionContext = createExecutionContext(enableLDAPSRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new EnableLDAPSRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(enableLDAPSRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "EnableLDAPS");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new EnableLDAPSResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enables multi-factor authentication (MFA) with the Remote Authentication Dial In User Service (RADIUS) server for
* an AD Connector or Microsoft AD directory.
*
*
* @param enableRadiusRequest
* Contains the inputs for the EnableRadius operation.
* @return Result of the EnableRadius operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws EntityAlreadyExistsException
* The specified entity already exists.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.EnableRadius
* @see AWS API
* Documentation
*/
@Override
public EnableRadiusResult enableRadius(EnableRadiusRequest request) {
request = beforeClientExecution(request);
return executeEnableRadius(request);
}
@SdkInternalApi
final EnableRadiusResult executeEnableRadius(EnableRadiusRequest enableRadiusRequest) {
ExecutionContext executionContext = createExecutionContext(enableRadiusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new EnableRadiusRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(enableRadiusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "EnableRadius");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new EnableRadiusResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enables single sign-on for a directory. Single sign-on allows users in your directory to access certain Amazon
* Web Services services from a computer joined to the directory without having to enter their credentials
* separately.
*
*
* @param enableSsoRequest
* Contains the inputs for the EnableSso operation.
* @return Result of the EnableSso operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InsufficientPermissionsException
* The account does not have sufficient permission to perform the operation.
* @throws AuthenticationFailedException
* An authentication error occurred.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.EnableSso
* @see AWS API
* Documentation
*/
@Override
public EnableSsoResult enableSso(EnableSsoRequest request) {
request = beforeClientExecution(request);
return executeEnableSso(request);
}
@SdkInternalApi
final EnableSsoResult executeEnableSso(EnableSsoRequest enableSsoRequest) {
ExecutionContext executionContext = createExecutionContext(enableSsoRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new EnableSsoRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(enableSsoRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "EnableSso");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new EnableSsoResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Obtains directory limit information for the current Region.
*
*
* @param getDirectoryLimitsRequest
* Contains the inputs for the GetDirectoryLimits operation.
* @return Result of the GetDirectoryLimits operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.GetDirectoryLimits
* @see AWS API
* Documentation
*/
@Override
public GetDirectoryLimitsResult getDirectoryLimits(GetDirectoryLimitsRequest request) {
request = beforeClientExecution(request);
return executeGetDirectoryLimits(request);
}
@SdkInternalApi
final GetDirectoryLimitsResult executeGetDirectoryLimits(GetDirectoryLimitsRequest getDirectoryLimitsRequest) {
ExecutionContext executionContext = createExecutionContext(getDirectoryLimitsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDirectoryLimitsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getDirectoryLimitsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDirectoryLimits");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetDirectoryLimitsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public GetDirectoryLimitsResult getDirectoryLimits() {
return getDirectoryLimits(new GetDirectoryLimitsRequest());
}
/**
*
* Obtains the manual snapshot limits for a directory.
*
*
* @param getSnapshotLimitsRequest
* Contains the inputs for the GetSnapshotLimits operation.
* @return Result of the GetSnapshotLimits operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.GetSnapshotLimits
* @see AWS API
* Documentation
*/
@Override
public GetSnapshotLimitsResult getSnapshotLimits(GetSnapshotLimitsRequest request) {
request = beforeClientExecution(request);
return executeGetSnapshotLimits(request);
}
@SdkInternalApi
final GetSnapshotLimitsResult executeGetSnapshotLimits(GetSnapshotLimitsRequest getSnapshotLimitsRequest) {
ExecutionContext executionContext = createExecutionContext(getSnapshotLimitsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSnapshotLimitsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getSnapshotLimitsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSnapshotLimits");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetSnapshotLimitsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* For the specified directory, lists all the certificates registered for a secure LDAP or client certificate
* authentication.
*
*
* @param listCertificatesRequest
* @return Result of the ListCertificates operation returned by the service.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.ListCertificates
* @see AWS API
* Documentation
*/
@Override
public ListCertificatesResult listCertificates(ListCertificatesRequest request) {
request = beforeClientExecution(request);
return executeListCertificates(request);
}
@SdkInternalApi
final ListCertificatesResult executeListCertificates(ListCertificatesRequest listCertificatesRequest) {
ExecutionContext executionContext = createExecutionContext(listCertificatesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListCertificatesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listCertificatesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListCertificates");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListCertificatesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the address blocks that you have added to a directory.
*
*
* @param listIpRoutesRequest
* @return Result of the ListIpRoutes operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.ListIpRoutes
* @see AWS API
* Documentation
*/
@Override
public ListIpRoutesResult listIpRoutes(ListIpRoutesRequest request) {
request = beforeClientExecution(request);
return executeListIpRoutes(request);
}
@SdkInternalApi
final ListIpRoutesResult executeListIpRoutes(ListIpRoutesRequest listIpRoutesRequest) {
ExecutionContext executionContext = createExecutionContext(listIpRoutesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListIpRoutesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listIpRoutesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListIpRoutes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListIpRoutesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the active log subscriptions for the Amazon Web Services account.
*
*
* @param listLogSubscriptionsRequest
* @return Result of the ListLogSubscriptions operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.ListLogSubscriptions
* @see AWS API
* Documentation
*/
@Override
public ListLogSubscriptionsResult listLogSubscriptions(ListLogSubscriptionsRequest request) {
request = beforeClientExecution(request);
return executeListLogSubscriptions(request);
}
@SdkInternalApi
final ListLogSubscriptionsResult executeListLogSubscriptions(ListLogSubscriptionsRequest listLogSubscriptionsRequest) {
ExecutionContext executionContext = createExecutionContext(listLogSubscriptionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListLogSubscriptionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listLogSubscriptionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListLogSubscriptions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListLogSubscriptionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all schema extensions applied to a Microsoft AD Directory.
*
*
* @param listSchemaExtensionsRequest
* @return Result of the ListSchemaExtensions operation returned by the service.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.ListSchemaExtensions
* @see AWS API
* Documentation
*/
@Override
public ListSchemaExtensionsResult listSchemaExtensions(ListSchemaExtensionsRequest request) {
request = beforeClientExecution(request);
return executeListSchemaExtensions(request);
}
@SdkInternalApi
final ListSchemaExtensionsResult executeListSchemaExtensions(ListSchemaExtensionsRequest listSchemaExtensionsRequest) {
ExecutionContext executionContext = createExecutionContext(listSchemaExtensionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListSchemaExtensionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listSchemaExtensionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListSchemaExtensions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListSchemaExtensionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all tags on a directory.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidNextTokenException
* The NextToken
value is not valid.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.ListTagsForResource
* @see AWS API
* Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Registers a certificate for a secure LDAP or client certificate authentication.
*
*
* @param registerCertificateRequest
* @return Result of the RegisterCertificate operation returned by the service.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws InvalidCertificateException
* The certificate PEM that was provided has incorrect encoding.
* @throws CertificateLimitExceededException
* The certificate could not be added because the certificate limit has been reached.
* @throws CertificateAlreadyExistsException
* The certificate has already been registered into the system.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.RegisterCertificate
* @see AWS API
* Documentation
*/
@Override
public RegisterCertificateResult registerCertificate(RegisterCertificateRequest request) {
request = beforeClientExecution(request);
return executeRegisterCertificate(request);
}
@SdkInternalApi
final RegisterCertificateResult executeRegisterCertificate(RegisterCertificateRequest registerCertificateRequest) {
ExecutionContext executionContext = createExecutionContext(registerCertificateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RegisterCertificateRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(registerCertificateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RegisterCertificate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new RegisterCertificateResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates a directory with an Amazon SNS topic. This establishes the directory as a publisher to the specified
* Amazon SNS topic. You can then receive email or text (SMS) messages when the status of your directory changes.
* You get notified if your directory goes from an Active status to an Impaired or Inoperable status. You also
* receive a notification when the directory returns to an Active status.
*
*
* @param registerEventTopicRequest
* Registers a new event topic.
* @return Result of the RegisterEventTopic operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.RegisterEventTopic
* @see AWS API
* Documentation
*/
@Override
public RegisterEventTopicResult registerEventTopic(RegisterEventTopicRequest request) {
request = beforeClientExecution(request);
return executeRegisterEventTopic(request);
}
@SdkInternalApi
final RegisterEventTopicResult executeRegisterEventTopic(RegisterEventTopicRequest registerEventTopicRequest) {
ExecutionContext executionContext = createExecutionContext(registerEventTopicRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RegisterEventTopicRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(registerEventTopicRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RegisterEventTopic");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new RegisterEventTopicResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Rejects a directory sharing request that was sent from the directory owner account.
*
*
* @param rejectSharedDirectoryRequest
* @return Result of the RejectSharedDirectory operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws DirectoryAlreadySharedException
* The specified directory has already been shared with this Amazon Web Services account.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.RejectSharedDirectory
* @see AWS API
* Documentation
*/
@Override
public RejectSharedDirectoryResult rejectSharedDirectory(RejectSharedDirectoryRequest request) {
request = beforeClientExecution(request);
return executeRejectSharedDirectory(request);
}
@SdkInternalApi
final RejectSharedDirectoryResult executeRejectSharedDirectory(RejectSharedDirectoryRequest rejectSharedDirectoryRequest) {
ExecutionContext executionContext = createExecutionContext(rejectSharedDirectoryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RejectSharedDirectoryRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(rejectSharedDirectoryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RejectSharedDirectory");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new RejectSharedDirectoryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes IP address blocks from a directory.
*
*
* @param removeIpRoutesRequest
* @return Result of the RemoveIpRoutes operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.RemoveIpRoutes
* @see AWS API
* Documentation
*/
@Override
public RemoveIpRoutesResult removeIpRoutes(RemoveIpRoutesRequest request) {
request = beforeClientExecution(request);
return executeRemoveIpRoutes(request);
}
@SdkInternalApi
final RemoveIpRoutesResult executeRemoveIpRoutes(RemoveIpRoutesRequest removeIpRoutesRequest) {
ExecutionContext executionContext = createExecutionContext(removeIpRoutesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RemoveIpRoutesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(removeIpRoutesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RemoveIpRoutes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new RemoveIpRoutesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Stops all replication and removes the domain controllers from the specified Region. You cannot remove the primary
* Region with this operation. Instead, use the DeleteDirectory
API.
*
*
* @param removeRegionRequest
* @return Result of the RemoveRegion operation returned by the service.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws AccessDeniedException
* Client authentication is not available in this region at this time.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.RemoveRegion
* @see AWS API
* Documentation
*/
@Override
public RemoveRegionResult removeRegion(RemoveRegionRequest request) {
request = beforeClientExecution(request);
return executeRemoveRegion(request);
}
@SdkInternalApi
final RemoveRegionResult executeRemoveRegion(RemoveRegionRequest removeRegionRequest) {
ExecutionContext executionContext = createExecutionContext(removeRegionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RemoveRegionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(removeRegionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RemoveRegion");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new RemoveRegionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes tags from a directory.
*
*
* @param removeTagsFromResourceRequest
* @return Result of the RemoveTagsFromResource operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.RemoveTagsFromResource
* @see AWS API
* Documentation
*/
@Override
public RemoveTagsFromResourceResult removeTagsFromResource(RemoveTagsFromResourceRequest request) {
request = beforeClientExecution(request);
return executeRemoveTagsFromResource(request);
}
@SdkInternalApi
final RemoveTagsFromResourceResult executeRemoveTagsFromResource(RemoveTagsFromResourceRequest removeTagsFromResourceRequest) {
ExecutionContext executionContext = createExecutionContext(removeTagsFromResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RemoveTagsFromResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(removeTagsFromResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RemoveTagsFromResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new RemoveTagsFromResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Resets the password for any user in your Managed Microsoft AD or Simple AD directory.
*
*
* You can reset the password for any user in your directory with the following exceptions:
*
*
* -
*
* For Simple AD, you cannot reset the password for any user that is a member of either the Domain Admins or
* Enterprise Admins group except for the administrator user.
*
*
* -
*
* For Managed Microsoft AD, you can only reset the password for a user that is in an OU based off of the NetBIOS
* name that you typed when you created your directory. For example, you cannot reset the password for a user in the
* Amazon Web Services Reserved OU. For more information about the OU structure for an Managed Microsoft AD
* directory, see What Gets Created in the Directory Service Administration Guide.
*
*
*
*
* @param resetUserPasswordRequest
* @return Result of the ResetUserPassword operation returned by the service.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws UserDoesNotExistException
* The user provided a username that does not exist in your directory.
* @throws InvalidPasswordException
* The new password provided by the user does not meet the password complexity requirements defined in your
* directory.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.ResetUserPassword
* @see AWS API
* Documentation
*/
@Override
public ResetUserPasswordResult resetUserPassword(ResetUserPasswordRequest request) {
request = beforeClientExecution(request);
return executeResetUserPassword(request);
}
@SdkInternalApi
final ResetUserPasswordResult executeResetUserPassword(ResetUserPasswordRequest resetUserPasswordRequest) {
ExecutionContext executionContext = createExecutionContext(resetUserPasswordRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ResetUserPasswordRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(resetUserPasswordRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ResetUserPassword");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ResetUserPasswordResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Restores a directory using an existing directory snapshot.
*
*
* When you restore a directory from a snapshot, any changes made to the directory after the snapshot date are
* overwritten.
*
*
* This action returns as soon as the restore operation is initiated. You can monitor the progress of the restore
* operation by calling the DescribeDirectories operation with the directory identifier. When the
* DirectoryDescription.Stage value changes to Active
, the restore operation is complete.
*
*
* @param restoreFromSnapshotRequest
* An object representing the inputs for the RestoreFromSnapshot operation.
* @return Result of the RestoreFromSnapshot operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.RestoreFromSnapshot
* @see AWS API
* Documentation
*/
@Override
public RestoreFromSnapshotResult restoreFromSnapshot(RestoreFromSnapshotRequest request) {
request = beforeClientExecution(request);
return executeRestoreFromSnapshot(request);
}
@SdkInternalApi
final RestoreFromSnapshotResult executeRestoreFromSnapshot(RestoreFromSnapshotRequest restoreFromSnapshotRequest) {
ExecutionContext executionContext = createExecutionContext(restoreFromSnapshotRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RestoreFromSnapshotRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(restoreFromSnapshotRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RestoreFromSnapshot");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new RestoreFromSnapshotResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Shares a specified directory (DirectoryId
) in your Amazon Web Services account (directory owner)
* with another Amazon Web Services account (directory consumer). With this operation you can use your directory
* from any Amazon Web Services account and from any Amazon VPC within an Amazon Web Services Region.
*
*
* When you share your Managed Microsoft AD directory, Directory Service creates a shared directory in the directory
* consumer account. This shared directory contains the metadata to provide access to the directory within the
* directory owner account. The shared directory is visible in all VPCs in the directory consumer account.
*
*
* The ShareMethod
parameter determines whether the specified directory can be shared between Amazon
* Web Services accounts inside the same Amazon Web Services organization (ORGANIZATIONS
). It also
* determines whether you can share the directory with any other Amazon Web Services account either inside or
* outside of the organization (HANDSHAKE
).
*
*
* The ShareNotes
parameter is only used when HANDSHAKE
is called, which sends a directory
* sharing request to the directory consumer.
*
*
* @param shareDirectoryRequest
* @return Result of the ShareDirectory operation returned by the service.
* @throws DirectoryAlreadySharedException
* The specified directory has already been shared with this Amazon Web Services account.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidTargetException
* The specified shared target is not valid.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ShareLimitExceededException
* The maximum number of Amazon Web Services accounts that you can share with this directory has been
* reached.
* @throws OrganizationsException
* Exception encountered while trying to access your Amazon Web Services organization.
* @throws AccessDeniedException
* Client authentication is not available in this region at this time.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.ShareDirectory
* @see AWS API
* Documentation
*/
@Override
public ShareDirectoryResult shareDirectory(ShareDirectoryRequest request) {
request = beforeClientExecution(request);
return executeShareDirectory(request);
}
@SdkInternalApi
final ShareDirectoryResult executeShareDirectory(ShareDirectoryRequest shareDirectoryRequest) {
ExecutionContext executionContext = createExecutionContext(shareDirectoryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ShareDirectoryRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(shareDirectoryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ShareDirectory");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ShareDirectoryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Applies a schema extension to a Microsoft AD directory.
*
*
* @param startSchemaExtensionRequest
* @return Result of the StartSchemaExtension operation returned by the service.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws SnapshotLimitExceededException
* The maximum number of manual snapshots for the directory has been reached. You can use the
* GetSnapshotLimits operation to determine the snapshot limits for a directory.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.StartSchemaExtension
* @see AWS API
* Documentation
*/
@Override
public StartSchemaExtensionResult startSchemaExtension(StartSchemaExtensionRequest request) {
request = beforeClientExecution(request);
return executeStartSchemaExtension(request);
}
@SdkInternalApi
final StartSchemaExtensionResult executeStartSchemaExtension(StartSchemaExtensionRequest startSchemaExtensionRequest) {
ExecutionContext executionContext = createExecutionContext(startSchemaExtensionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartSchemaExtensionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startSchemaExtensionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartSchemaExtension");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StartSchemaExtensionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Stops the directory sharing between the directory owner and consumer accounts.
*
*
* @param unshareDirectoryRequest
* @return Result of the UnshareDirectory operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidTargetException
* The specified shared target is not valid.
* @throws DirectoryNotSharedException
* The specified directory has not been shared with this Amazon Web Services account.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.UnshareDirectory
* @see AWS API
* Documentation
*/
@Override
public UnshareDirectoryResult unshareDirectory(UnshareDirectoryRequest request) {
request = beforeClientExecution(request);
return executeUnshareDirectory(request);
}
@SdkInternalApi
final UnshareDirectoryResult executeUnshareDirectory(UnshareDirectoryRequest unshareDirectoryRequest) {
ExecutionContext executionContext = createExecutionContext(unshareDirectoryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UnshareDirectoryRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(unshareDirectoryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UnshareDirectory");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UnshareDirectoryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a conditional forwarder that has been set up for your Amazon Web Services directory.
*
*
* @param updateConditionalForwarderRequest
* Updates a conditional forwarder.
* @return Result of the UpdateConditionalForwarder operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.UpdateConditionalForwarder
* @see AWS
* API Documentation
*/
@Override
public UpdateConditionalForwarderResult updateConditionalForwarder(UpdateConditionalForwarderRequest request) {
request = beforeClientExecution(request);
return executeUpdateConditionalForwarder(request);
}
@SdkInternalApi
final UpdateConditionalForwarderResult executeUpdateConditionalForwarder(UpdateConditionalForwarderRequest updateConditionalForwarderRequest) {
ExecutionContext executionContext = createExecutionContext(updateConditionalForwarderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateConditionalForwarderRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateConditionalForwarderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateConditionalForwarder");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateConditionalForwarderResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the directory for a particular update type.
*
*
* @param updateDirectorySetupRequest
* @return Result of the UpdateDirectorySetup operation returned by the service.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws DirectoryInDesiredStateException
* The directory is already updated to desired update type settings.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws SnapshotLimitExceededException
* The maximum number of manual snapshots for the directory has been reached. You can use the
* GetSnapshotLimits operation to determine the snapshot limits for a directory.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws AccessDeniedException
* Client authentication is not available in this region at this time.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.UpdateDirectorySetup
* @see AWS API
* Documentation
*/
@Override
public UpdateDirectorySetupResult updateDirectorySetup(UpdateDirectorySetupRequest request) {
request = beforeClientExecution(request);
return executeUpdateDirectorySetup(request);
}
@SdkInternalApi
final UpdateDirectorySetupResult executeUpdateDirectorySetup(UpdateDirectorySetupRequest updateDirectorySetupRequest) {
ExecutionContext executionContext = createExecutionContext(updateDirectorySetupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateDirectorySetupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateDirectorySetupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateDirectorySetup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateDirectorySetupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds or removes domain controllers to or from the directory. Based on the difference between current value and
* new value (provided through this API call), domain controllers will be added or removed. It may take up to 45
* minutes for any new domain controllers to become fully active once the requested number of domain controllers is
* updated. During this time, you cannot make another update request.
*
*
* @param updateNumberOfDomainControllersRequest
* @return Result of the UpdateNumberOfDomainControllers operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws DomainControllerLimitExceededException
* The maximum allowed number of domain controllers per directory was exceeded. The default limit per
* directory is 20 domain controllers.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.UpdateNumberOfDomainControllers
* @see AWS API Documentation
*/
@Override
public UpdateNumberOfDomainControllersResult updateNumberOfDomainControllers(UpdateNumberOfDomainControllersRequest request) {
request = beforeClientExecution(request);
return executeUpdateNumberOfDomainControllers(request);
}
@SdkInternalApi
final UpdateNumberOfDomainControllersResult executeUpdateNumberOfDomainControllers(
UpdateNumberOfDomainControllersRequest updateNumberOfDomainControllersRequest) {
ExecutionContext executionContext = createExecutionContext(updateNumberOfDomainControllersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateNumberOfDomainControllersRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateNumberOfDomainControllersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateNumberOfDomainControllers");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateNumberOfDomainControllersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the Remote Authentication Dial In User Service (RADIUS) server information for an AD Connector or
* Microsoft AD directory.
*
*
* @param updateRadiusRequest
* Contains the inputs for the UpdateRadius operation.
* @return Result of the UpdateRadius operation returned by the service.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.UpdateRadius
* @see AWS API
* Documentation
*/
@Override
public UpdateRadiusResult updateRadius(UpdateRadiusRequest request) {
request = beforeClientExecution(request);
return executeUpdateRadius(request);
}
@SdkInternalApi
final UpdateRadiusResult executeUpdateRadius(UpdateRadiusRequest updateRadiusRequest) {
ExecutionContext executionContext = createExecutionContext(updateRadiusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateRadiusRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateRadiusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateRadius");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateRadiusResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the configurable settings for the specified directory.
*
*
* @param updateSettingsRequest
* @return Result of the UpdateSettings operation returned by the service.
* @throws DirectoryDoesNotExistException
* The specified directory does not exist in the system.
* @throws UnsupportedOperationException
* The operation is not supported.
* @throws DirectoryUnavailableException
* The specified directory is unavailable or could not be found.
* @throws IncompatibleSettingsException
* The specified directory setting is not compatible with other settings.
* @throws UnsupportedSettingsException
* The specified directory setting is not supported.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.UpdateSettings
* @see AWS API
* Documentation
*/
@Override
public UpdateSettingsResult updateSettings(UpdateSettingsRequest request) {
request = beforeClientExecution(request);
return executeUpdateSettings(request);
}
@SdkInternalApi
final UpdateSettingsResult executeUpdateSettings(UpdateSettingsRequest updateSettingsRequest) {
ExecutionContext executionContext = createExecutionContext(updateSettingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateSettingsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateSettingsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateSettings");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateSettingsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the trust that has been set up between your Managed Microsoft AD directory and an self-managed Active
* Directory.
*
*
* @param updateTrustRequest
* @return Result of the UpdateTrust operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @sample AWSDirectoryService.UpdateTrust
* @see AWS API
* Documentation
*/
@Override
public UpdateTrustResult updateTrust(UpdateTrustRequest request) {
request = beforeClientExecution(request);
return executeUpdateTrust(request);
}
@SdkInternalApi
final UpdateTrustResult executeUpdateTrust(UpdateTrustRequest updateTrustRequest) {
ExecutionContext executionContext = createExecutionContext(updateTrustRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateTrustRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateTrustRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateTrust");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateTrustResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Directory Service for Microsoft Active Directory allows you to configure and verify trust relationships.
*
*
* This action verifies a trust relationship between your Managed Microsoft AD directory and an external domain.
*
*
* @param verifyTrustRequest
* Initiates the verification of an existing trust relationship between an Managed Microsoft AD directory and
* an external domain.
* @return Result of the VerifyTrust operation returned by the service.
* @throws EntityDoesNotExistException
* The specified entity could not be found.
* @throws InvalidParameterException
* One or more parameters are not valid.
* @throws ClientException
* A client exception has occurred.
* @throws ServiceException
* An exception has occurred in Directory Service.
* @throws UnsupportedOperationException
* The operation is not supported.
* @sample AWSDirectoryService.VerifyTrust
* @see AWS API
* Documentation
*/
@Override
public VerifyTrustResult verifyTrust(VerifyTrustRequest request) {
request = beforeClientExecution(request);
return executeVerifyTrust(request);
}
@SdkInternalApi
final VerifyTrustResult executeVerifyTrust(VerifyTrustRequest verifyTrustRequest) {
ExecutionContext executionContext = createExecutionContext(verifyTrustRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new VerifyTrustRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(verifyTrustRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Directory Service");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "VerifyTrust");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new VerifyTrustResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}