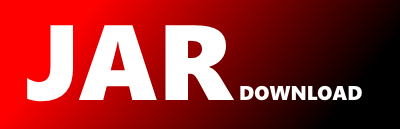
com.amazonaws.services.directory.model.SettingEntry Maven / Gradle / Ivy
Show all versions of aws-java-sdk-directory Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.directory.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Contains information about the specified configurable setting for a directory.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class SettingEntry implements Serializable, Cloneable, StructuredPojo {
/**
*
* The type, or category, of a directory setting. Similar settings have the same type. For example,
* Protocol
, Cipher
, or Certificate-Based Authentication
.
*
*/
private String type;
/**
*
* The name of the directory setting. For example:
*
*
* TLS_1_0
*
*/
private String name;
/**
*
* The valid range of values for the directory setting. These values depend on the DataType
of your
* directory.
*
*/
private String allowedValues;
/**
*
* The value of the directory setting that is applied to the directory.
*
*/
private String appliedValue;
/**
*
* The value that was last requested for the directory setting.
*
*/
private String requestedValue;
/**
*
* The overall status of the request to update the directory setting request. If the directory setting is deployed
* in more than one region, and the request fails in any region, the overall status is Failed
.
*
*/
private String requestStatus;
/**
*
* Details about the status of the request to update the directory setting. If the directory setting is deployed in
* more than one region, status is returned for the request in each region where the setting is deployed.
*
*/
private java.util.Map requestDetailedStatus;
/**
*
* The last status message for the directory status request.
*
*/
private String requestStatusMessage;
/**
*
* The date and time when the directory setting was last updated.
*
*/
private java.util.Date lastUpdatedDateTime;
/**
*
* The date and time when the request to update a directory setting was last submitted.
*
*/
private java.util.Date lastRequestedDateTime;
/**
*
* The data type of a directory setting. This is used to define the AllowedValues
of a setting. For
* example a data type can be Boolean
, DurationInSeconds
, or Enum
.
*
*/
private String dataType;
/**
*
* The type, or category, of a directory setting. Similar settings have the same type. For example,
* Protocol
, Cipher
, or Certificate-Based Authentication
.
*
*
* @param type
* The type, or category, of a directory setting. Similar settings have the same type. For example,
* Protocol
, Cipher
, or Certificate-Based Authentication
.
*/
public void setType(String type) {
this.type = type;
}
/**
*
* The type, or category, of a directory setting. Similar settings have the same type. For example,
* Protocol
, Cipher
, or Certificate-Based Authentication
.
*
*
* @return The type, or category, of a directory setting. Similar settings have the same type. For example,
* Protocol
, Cipher
, or Certificate-Based Authentication
.
*/
public String getType() {
return this.type;
}
/**
*
* The type, or category, of a directory setting. Similar settings have the same type. For example,
* Protocol
, Cipher
, or Certificate-Based Authentication
.
*
*
* @param type
* The type, or category, of a directory setting. Similar settings have the same type. For example,
* Protocol
, Cipher
, or Certificate-Based Authentication
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SettingEntry withType(String type) {
setType(type);
return this;
}
/**
*
* The name of the directory setting. For example:
*
*
* TLS_1_0
*
*
* @param name
* The name of the directory setting. For example:
*
* TLS_1_0
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the directory setting. For example:
*
*
* TLS_1_0
*
*
* @return The name of the directory setting. For example:
*
* TLS_1_0
*/
public String getName() {
return this.name;
}
/**
*
* The name of the directory setting. For example:
*
*
* TLS_1_0
*
*
* @param name
* The name of the directory setting. For example:
*
* TLS_1_0
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SettingEntry withName(String name) {
setName(name);
return this;
}
/**
*
* The valid range of values for the directory setting. These values depend on the DataType
of your
* directory.
*
*
* @param allowedValues
* The valid range of values for the directory setting. These values depend on the DataType
of
* your directory.
*/
public void setAllowedValues(String allowedValues) {
this.allowedValues = allowedValues;
}
/**
*
* The valid range of values for the directory setting. These values depend on the DataType
of your
* directory.
*
*
* @return The valid range of values for the directory setting. These values depend on the DataType
of
* your directory.
*/
public String getAllowedValues() {
return this.allowedValues;
}
/**
*
* The valid range of values for the directory setting. These values depend on the DataType
of your
* directory.
*
*
* @param allowedValues
* The valid range of values for the directory setting. These values depend on the DataType
of
* your directory.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SettingEntry withAllowedValues(String allowedValues) {
setAllowedValues(allowedValues);
return this;
}
/**
*
* The value of the directory setting that is applied to the directory.
*
*
* @param appliedValue
* The value of the directory setting that is applied to the directory.
*/
public void setAppliedValue(String appliedValue) {
this.appliedValue = appliedValue;
}
/**
*
* The value of the directory setting that is applied to the directory.
*
*
* @return The value of the directory setting that is applied to the directory.
*/
public String getAppliedValue() {
return this.appliedValue;
}
/**
*
* The value of the directory setting that is applied to the directory.
*
*
* @param appliedValue
* The value of the directory setting that is applied to the directory.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SettingEntry withAppliedValue(String appliedValue) {
setAppliedValue(appliedValue);
return this;
}
/**
*
* The value that was last requested for the directory setting.
*
*
* @param requestedValue
* The value that was last requested for the directory setting.
*/
public void setRequestedValue(String requestedValue) {
this.requestedValue = requestedValue;
}
/**
*
* The value that was last requested for the directory setting.
*
*
* @return The value that was last requested for the directory setting.
*/
public String getRequestedValue() {
return this.requestedValue;
}
/**
*
* The value that was last requested for the directory setting.
*
*
* @param requestedValue
* The value that was last requested for the directory setting.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SettingEntry withRequestedValue(String requestedValue) {
setRequestedValue(requestedValue);
return this;
}
/**
*
* The overall status of the request to update the directory setting request. If the directory setting is deployed
* in more than one region, and the request fails in any region, the overall status is Failed
.
*
*
* @param requestStatus
* The overall status of the request to update the directory setting request. If the directory setting is
* deployed in more than one region, and the request fails in any region, the overall status is
* Failed
.
* @see DirectoryConfigurationStatus
*/
public void setRequestStatus(String requestStatus) {
this.requestStatus = requestStatus;
}
/**
*
* The overall status of the request to update the directory setting request. If the directory setting is deployed
* in more than one region, and the request fails in any region, the overall status is Failed
.
*
*
* @return The overall status of the request to update the directory setting request. If the directory setting is
* deployed in more than one region, and the request fails in any region, the overall status is
* Failed
.
* @see DirectoryConfigurationStatus
*/
public String getRequestStatus() {
return this.requestStatus;
}
/**
*
* The overall status of the request to update the directory setting request. If the directory setting is deployed
* in more than one region, and the request fails in any region, the overall status is Failed
.
*
*
* @param requestStatus
* The overall status of the request to update the directory setting request. If the directory setting is
* deployed in more than one region, and the request fails in any region, the overall status is
* Failed
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DirectoryConfigurationStatus
*/
public SettingEntry withRequestStatus(String requestStatus) {
setRequestStatus(requestStatus);
return this;
}
/**
*
* The overall status of the request to update the directory setting request. If the directory setting is deployed
* in more than one region, and the request fails in any region, the overall status is Failed
.
*
*
* @param requestStatus
* The overall status of the request to update the directory setting request. If the directory setting is
* deployed in more than one region, and the request fails in any region, the overall status is
* Failed
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DirectoryConfigurationStatus
*/
public SettingEntry withRequestStatus(DirectoryConfigurationStatus requestStatus) {
this.requestStatus = requestStatus.toString();
return this;
}
/**
*
* Details about the status of the request to update the directory setting. If the directory setting is deployed in
* more than one region, status is returned for the request in each region where the setting is deployed.
*
*
* @return Details about the status of the request to update the directory setting. If the directory setting is
* deployed in more than one region, status is returned for the request in each region where the setting is
* deployed.
*/
public java.util.Map getRequestDetailedStatus() {
return requestDetailedStatus;
}
/**
*
* Details about the status of the request to update the directory setting. If the directory setting is deployed in
* more than one region, status is returned for the request in each region where the setting is deployed.
*
*
* @param requestDetailedStatus
* Details about the status of the request to update the directory setting. If the directory setting is
* deployed in more than one region, status is returned for the request in each region where the setting is
* deployed.
*/
public void setRequestDetailedStatus(java.util.Map requestDetailedStatus) {
this.requestDetailedStatus = requestDetailedStatus;
}
/**
*
* Details about the status of the request to update the directory setting. If the directory setting is deployed in
* more than one region, status is returned for the request in each region where the setting is deployed.
*
*
* @param requestDetailedStatus
* Details about the status of the request to update the directory setting. If the directory setting is
* deployed in more than one region, status is returned for the request in each region where the setting is
* deployed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SettingEntry withRequestDetailedStatus(java.util.Map requestDetailedStatus) {
setRequestDetailedStatus(requestDetailedStatus);
return this;
}
/**
* Add a single RequestDetailedStatus entry
*
* @see SettingEntry#withRequestDetailedStatus
* @returns a reference to this object so that method calls can be chained together.
*/
public SettingEntry addRequestDetailedStatusEntry(String key, String value) {
if (null == this.requestDetailedStatus) {
this.requestDetailedStatus = new java.util.HashMap();
}
if (this.requestDetailedStatus.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.requestDetailedStatus.put(key, value);
return this;
}
/**
* Removes all the entries added into RequestDetailedStatus.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SettingEntry clearRequestDetailedStatusEntries() {
this.requestDetailedStatus = null;
return this;
}
/**
*
* The last status message for the directory status request.
*
*
* @param requestStatusMessage
* The last status message for the directory status request.
*/
public void setRequestStatusMessage(String requestStatusMessage) {
this.requestStatusMessage = requestStatusMessage;
}
/**
*
* The last status message for the directory status request.
*
*
* @return The last status message for the directory status request.
*/
public String getRequestStatusMessage() {
return this.requestStatusMessage;
}
/**
*
* The last status message for the directory status request.
*
*
* @param requestStatusMessage
* The last status message for the directory status request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SettingEntry withRequestStatusMessage(String requestStatusMessage) {
setRequestStatusMessage(requestStatusMessage);
return this;
}
/**
*
* The date and time when the directory setting was last updated.
*
*
* @param lastUpdatedDateTime
* The date and time when the directory setting was last updated.
*/
public void setLastUpdatedDateTime(java.util.Date lastUpdatedDateTime) {
this.lastUpdatedDateTime = lastUpdatedDateTime;
}
/**
*
* The date and time when the directory setting was last updated.
*
*
* @return The date and time when the directory setting was last updated.
*/
public java.util.Date getLastUpdatedDateTime() {
return this.lastUpdatedDateTime;
}
/**
*
* The date and time when the directory setting was last updated.
*
*
* @param lastUpdatedDateTime
* The date and time when the directory setting was last updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SettingEntry withLastUpdatedDateTime(java.util.Date lastUpdatedDateTime) {
setLastUpdatedDateTime(lastUpdatedDateTime);
return this;
}
/**
*
* The date and time when the request to update a directory setting was last submitted.
*
*
* @param lastRequestedDateTime
* The date and time when the request to update a directory setting was last submitted.
*/
public void setLastRequestedDateTime(java.util.Date lastRequestedDateTime) {
this.lastRequestedDateTime = lastRequestedDateTime;
}
/**
*
* The date and time when the request to update a directory setting was last submitted.
*
*
* @return The date and time when the request to update a directory setting was last submitted.
*/
public java.util.Date getLastRequestedDateTime() {
return this.lastRequestedDateTime;
}
/**
*
* The date and time when the request to update a directory setting was last submitted.
*
*
* @param lastRequestedDateTime
* The date and time when the request to update a directory setting was last submitted.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SettingEntry withLastRequestedDateTime(java.util.Date lastRequestedDateTime) {
setLastRequestedDateTime(lastRequestedDateTime);
return this;
}
/**
*
* The data type of a directory setting. This is used to define the AllowedValues
of a setting. For
* example a data type can be Boolean
, DurationInSeconds
, or Enum
.
*
*
* @param dataType
* The data type of a directory setting. This is used to define the AllowedValues
of a setting.
* For example a data type can be Boolean
, DurationInSeconds
, or Enum
.
*/
public void setDataType(String dataType) {
this.dataType = dataType;
}
/**
*
* The data type of a directory setting. This is used to define the AllowedValues
of a setting. For
* example a data type can be Boolean
, DurationInSeconds
, or Enum
.
*
*
* @return The data type of a directory setting. This is used to define the AllowedValues
of a setting.
* For example a data type can be Boolean
, DurationInSeconds
, or Enum
* .
*/
public String getDataType() {
return this.dataType;
}
/**
*
* The data type of a directory setting. This is used to define the AllowedValues
of a setting. For
* example a data type can be Boolean
, DurationInSeconds
, or Enum
.
*
*
* @param dataType
* The data type of a directory setting. This is used to define the AllowedValues
of a setting.
* For example a data type can be Boolean
, DurationInSeconds
, or Enum
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SettingEntry withDataType(String dataType) {
setDataType(dataType);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getType() != null)
sb.append("Type: ").append(getType()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getAllowedValues() != null)
sb.append("AllowedValues: ").append(getAllowedValues()).append(",");
if (getAppliedValue() != null)
sb.append("AppliedValue: ").append(getAppliedValue()).append(",");
if (getRequestedValue() != null)
sb.append("RequestedValue: ").append(getRequestedValue()).append(",");
if (getRequestStatus() != null)
sb.append("RequestStatus: ").append(getRequestStatus()).append(",");
if (getRequestDetailedStatus() != null)
sb.append("RequestDetailedStatus: ").append(getRequestDetailedStatus()).append(",");
if (getRequestStatusMessage() != null)
sb.append("RequestStatusMessage: ").append(getRequestStatusMessage()).append(",");
if (getLastUpdatedDateTime() != null)
sb.append("LastUpdatedDateTime: ").append(getLastUpdatedDateTime()).append(",");
if (getLastRequestedDateTime() != null)
sb.append("LastRequestedDateTime: ").append(getLastRequestedDateTime()).append(",");
if (getDataType() != null)
sb.append("DataType: ").append(getDataType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof SettingEntry == false)
return false;
SettingEntry other = (SettingEntry) obj;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null && other.getType().equals(this.getType()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getAllowedValues() == null ^ this.getAllowedValues() == null)
return false;
if (other.getAllowedValues() != null && other.getAllowedValues().equals(this.getAllowedValues()) == false)
return false;
if (other.getAppliedValue() == null ^ this.getAppliedValue() == null)
return false;
if (other.getAppliedValue() != null && other.getAppliedValue().equals(this.getAppliedValue()) == false)
return false;
if (other.getRequestedValue() == null ^ this.getRequestedValue() == null)
return false;
if (other.getRequestedValue() != null && other.getRequestedValue().equals(this.getRequestedValue()) == false)
return false;
if (other.getRequestStatus() == null ^ this.getRequestStatus() == null)
return false;
if (other.getRequestStatus() != null && other.getRequestStatus().equals(this.getRequestStatus()) == false)
return false;
if (other.getRequestDetailedStatus() == null ^ this.getRequestDetailedStatus() == null)
return false;
if (other.getRequestDetailedStatus() != null && other.getRequestDetailedStatus().equals(this.getRequestDetailedStatus()) == false)
return false;
if (other.getRequestStatusMessage() == null ^ this.getRequestStatusMessage() == null)
return false;
if (other.getRequestStatusMessage() != null && other.getRequestStatusMessage().equals(this.getRequestStatusMessage()) == false)
return false;
if (other.getLastUpdatedDateTime() == null ^ this.getLastUpdatedDateTime() == null)
return false;
if (other.getLastUpdatedDateTime() != null && other.getLastUpdatedDateTime().equals(this.getLastUpdatedDateTime()) == false)
return false;
if (other.getLastRequestedDateTime() == null ^ this.getLastRequestedDateTime() == null)
return false;
if (other.getLastRequestedDateTime() != null && other.getLastRequestedDateTime().equals(this.getLastRequestedDateTime()) == false)
return false;
if (other.getDataType() == null ^ this.getDataType() == null)
return false;
if (other.getDataType() != null && other.getDataType().equals(this.getDataType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getType() == null) ? 0 : getType().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getAllowedValues() == null) ? 0 : getAllowedValues().hashCode());
hashCode = prime * hashCode + ((getAppliedValue() == null) ? 0 : getAppliedValue().hashCode());
hashCode = prime * hashCode + ((getRequestedValue() == null) ? 0 : getRequestedValue().hashCode());
hashCode = prime * hashCode + ((getRequestStatus() == null) ? 0 : getRequestStatus().hashCode());
hashCode = prime * hashCode + ((getRequestDetailedStatus() == null) ? 0 : getRequestDetailedStatus().hashCode());
hashCode = prime * hashCode + ((getRequestStatusMessage() == null) ? 0 : getRequestStatusMessage().hashCode());
hashCode = prime * hashCode + ((getLastUpdatedDateTime() == null) ? 0 : getLastUpdatedDateTime().hashCode());
hashCode = prime * hashCode + ((getLastRequestedDateTime() == null) ? 0 : getLastRequestedDateTime().hashCode());
hashCode = prime * hashCode + ((getDataType() == null) ? 0 : getDataType().hashCode());
return hashCode;
}
@Override
public SettingEntry clone() {
try {
return (SettingEntry) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.directory.model.transform.SettingEntryMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}