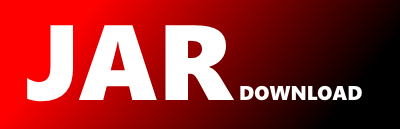
com.amazonaws.services.dlm.model.CreateLifecyclePolicyRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-dlm Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.dlm.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateLifecyclePolicyRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The Amazon Resource Name (ARN) of the IAM role used to run the operations specified by the lifecycle policy.
*
*/
private String executionRoleArn;
/**
*
* A description of the lifecycle policy. The characters ^[0-9A-Za-z _-]+$ are supported.
*
*/
private String description;
/**
*
* The desired activation state of the lifecycle policy after creation.
*
*/
private String state;
/**
*
* The configuration details of the lifecycle policy.
*
*/
private PolicyDetails policyDetails;
/**
*
* The tags to apply to the lifecycle policy during creation.
*
*/
private java.util.Map tags;
/**
*
* The Amazon Resource Name (ARN) of the IAM role used to run the operations specified by the lifecycle policy.
*
*
* @param executionRoleArn
* The Amazon Resource Name (ARN) of the IAM role used to run the operations specified by the lifecycle
* policy.
*/
public void setExecutionRoleArn(String executionRoleArn) {
this.executionRoleArn = executionRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role used to run the operations specified by the lifecycle policy.
*
*
* @return The Amazon Resource Name (ARN) of the IAM role used to run the operations specified by the lifecycle
* policy.
*/
public String getExecutionRoleArn() {
return this.executionRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role used to run the operations specified by the lifecycle policy.
*
*
* @param executionRoleArn
* The Amazon Resource Name (ARN) of the IAM role used to run the operations specified by the lifecycle
* policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateLifecyclePolicyRequest withExecutionRoleArn(String executionRoleArn) {
setExecutionRoleArn(executionRoleArn);
return this;
}
/**
*
* A description of the lifecycle policy. The characters ^[0-9A-Za-z _-]+$ are supported.
*
*
* @param description
* A description of the lifecycle policy. The characters ^[0-9A-Za-z _-]+$ are supported.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the lifecycle policy. The characters ^[0-9A-Za-z _-]+$ are supported.
*
*
* @return A description of the lifecycle policy. The characters ^[0-9A-Za-z _-]+$ are supported.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the lifecycle policy. The characters ^[0-9A-Za-z _-]+$ are supported.
*
*
* @param description
* A description of the lifecycle policy. The characters ^[0-9A-Za-z _-]+$ are supported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateLifecyclePolicyRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The desired activation state of the lifecycle policy after creation.
*
*
* @param state
* The desired activation state of the lifecycle policy after creation.
* @see SettablePolicyStateValues
*/
public void setState(String state) {
this.state = state;
}
/**
*
* The desired activation state of the lifecycle policy after creation.
*
*
* @return The desired activation state of the lifecycle policy after creation.
* @see SettablePolicyStateValues
*/
public String getState() {
return this.state;
}
/**
*
* The desired activation state of the lifecycle policy after creation.
*
*
* @param state
* The desired activation state of the lifecycle policy after creation.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SettablePolicyStateValues
*/
public CreateLifecyclePolicyRequest withState(String state) {
setState(state);
return this;
}
/**
*
* The desired activation state of the lifecycle policy after creation.
*
*
* @param state
* The desired activation state of the lifecycle policy after creation.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SettablePolicyStateValues
*/
public CreateLifecyclePolicyRequest withState(SettablePolicyStateValues state) {
this.state = state.toString();
return this;
}
/**
*
* The configuration details of the lifecycle policy.
*
*
* @param policyDetails
* The configuration details of the lifecycle policy.
*/
public void setPolicyDetails(PolicyDetails policyDetails) {
this.policyDetails = policyDetails;
}
/**
*
* The configuration details of the lifecycle policy.
*
*
* @return The configuration details of the lifecycle policy.
*/
public PolicyDetails getPolicyDetails() {
return this.policyDetails;
}
/**
*
* The configuration details of the lifecycle policy.
*
*
* @param policyDetails
* The configuration details of the lifecycle policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateLifecyclePolicyRequest withPolicyDetails(PolicyDetails policyDetails) {
setPolicyDetails(policyDetails);
return this;
}
/**
*
* The tags to apply to the lifecycle policy during creation.
*
*
* @return The tags to apply to the lifecycle policy during creation.
*/
public java.util.Map getTags() {
return tags;
}
/**
*
* The tags to apply to the lifecycle policy during creation.
*
*
* @param tags
* The tags to apply to the lifecycle policy during creation.
*/
public void setTags(java.util.Map tags) {
this.tags = tags;
}
/**
*
* The tags to apply to the lifecycle policy during creation.
*
*
* @param tags
* The tags to apply to the lifecycle policy during creation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateLifecyclePolicyRequest withTags(java.util.Map tags) {
setTags(tags);
return this;
}
/**
* Add a single Tags entry
*
* @see CreateLifecyclePolicyRequest#withTags
* @returns a reference to this object so that method calls can be chained together.
*/
public CreateLifecyclePolicyRequest addTagsEntry(String key, String value) {
if (null == this.tags) {
this.tags = new java.util.HashMap();
}
if (this.tags.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.tags.put(key, value);
return this;
}
/**
* Removes all the entries added into Tags.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateLifecyclePolicyRequest clearTagsEntries() {
this.tags = null;
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getExecutionRoleArn() != null)
sb.append("ExecutionRoleArn: ").append(getExecutionRoleArn()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getState() != null)
sb.append("State: ").append(getState()).append(",");
if (getPolicyDetails() != null)
sb.append("PolicyDetails: ").append(getPolicyDetails()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateLifecyclePolicyRequest == false)
return false;
CreateLifecyclePolicyRequest other = (CreateLifecyclePolicyRequest) obj;
if (other.getExecutionRoleArn() == null ^ this.getExecutionRoleArn() == null)
return false;
if (other.getExecutionRoleArn() != null && other.getExecutionRoleArn().equals(this.getExecutionRoleArn()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getState() == null ^ this.getState() == null)
return false;
if (other.getState() != null && other.getState().equals(this.getState()) == false)
return false;
if (other.getPolicyDetails() == null ^ this.getPolicyDetails() == null)
return false;
if (other.getPolicyDetails() != null && other.getPolicyDetails().equals(this.getPolicyDetails()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getExecutionRoleArn() == null) ? 0 : getExecutionRoleArn().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getState() == null) ? 0 : getState().hashCode());
hashCode = prime * hashCode + ((getPolicyDetails() == null) ? 0 : getPolicyDetails().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CreateLifecyclePolicyRequest clone() {
return (CreateLifecyclePolicyRequest) super.clone();
}
}